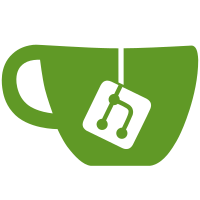
3 changed files with 627 additions and 0 deletions
@ -0,0 +1,44 @@ |
|||
import request from '@/utils/request' |
|||
// eslint-disable-next-line no-unused-vars
|
|||
import qs from 'qs' |
|||
|
|||
// 获取token
|
|||
export function getCallToken() { |
|||
return request({ url: '/call/getCallToken', method: 'GET', }) |
|||
} |
|||
|
|||
// 获取子系统列表
|
|||
export function getCallSonList() { |
|||
return request({ url: '/call/getCallSonList', method: 'GET', }) |
|||
} |
|||
|
|||
// 子系统布防
|
|||
export function getCallArm() { |
|||
return request({ url: '/call/getCallArm', method: 'GET', }) |
|||
} |
|||
|
|||
// 子系统撤防
|
|||
export function getCallDisArm() { |
|||
return request({ url: '/call/getCallDisArm', method: 'GET', }) |
|||
} |
|||
|
|||
// 创建消息消费者
|
|||
export function createCallConsumer() { |
|||
return request({ url: '/call/createCallConsumer', method: 'GET', }) |
|||
} |
|||
|
|||
// 获取消息列表
|
|||
export function getCallMessageList(data) { |
|||
return request({ url: '/call/getCallMessageList', method: 'GET', params: data }) |
|||
} |
|||
|
|||
// 提交消息偏移量
|
|||
export function doCallMessageOffsets(data) { |
|||
return request({ url: '/call/doCallMessageOffsets', method: 'GET', params: data }) |
|||
} |
|||
|
|||
|
|||
// 获取所有防区状态
|
|||
export function getCallZoneStatusList(data) { |
|||
return request({ url: '/call/getCallZoneStatusList', method: 'GET'}) |
|||
} |
@ -0,0 +1,566 @@ |
|||
<template> |
|||
<div class="webindex"> |
|||
<div class="zongcons"> |
|||
<div class="maps"> |
|||
<div class="wenboxs"> |
|||
<div class="wenbox"> |
|||
<div class="tubiao"><span class="myiconfont icon-shishijiance1 tolzi"></span></div> |
|||
<div class="tos"> |
|||
<div class="tit nums">河北仓库<span class="num">3</span>家</div> |
|||
<div class="tit nums">货物数<span class="num">4575</span>个</div> |
|||
</div> |
|||
</div> |
|||
<div class="wenbox"> |
|||
<div class="tubiao"><span class="myiconfont icon-louyuzidonghua tolzi"></span></div> |
|||
<div class="tos"> |
|||
<div class="tit nums">湖北仓库<span class="num">3</span>家</div> |
|||
<div class="tit nums">货物数<span class="num">2575</span>个</div> |
|||
</div> |
|||
</div> |
|||
<div class="wenbox"> |
|||
<div class="tubiao"><span class="myiconfont icon-shebeitaizhang tolzi"></span></div> |
|||
<div class="tos"> |
|||
<div class="tit nums">黑龙江<span class="num">3</span>家</div> |
|||
<div class="tit nums">货物数<span class="num">4575</span>个</div> |
|||
</div> |
|||
</div> |
|||
<div class="wenbox"> |
|||
<div class="tubiao"><span class="myiconfont icon-zhihuilouyu tolzi"></span></div> |
|||
<div class="tos"> |
|||
<div class="tit nums">上海仓库<span class="num">1</span>家</div> |
|||
<div class="tit nums">货物数<span class="num">123</span>个</div> |
|||
</div> |
|||
</div> |
|||
<div class="wenbox"> |
|||
<div class="tubiao"><span class="myiconfont icon-rizhi2 tolzi"></span></div> |
|||
<div class="tos"> |
|||
<div class="tit nums">江苏仓库<span class="num">1</span>家</div> |
|||
<div class="tit nums">货物数<span class="num">126</span>个</div> |
|||
</div> |
|||
</div> |
|||
|
|||
|
|||
|
|||
</div> |
|||
<home-map style="height:600px"></home-map> |
|||
</div> |
|||
</div> |
|||
|
|||
|
|||
<div class="rightbars tops"> |
|||
<!-- 第1个 --> |
|||
<!-- 第2个 --> |
|||
<div class="topboxs"> |
|||
<div class="topbox"> |
|||
<div class="toptitle" @click="getToken">获取token</div> |
|||
<div class="mids"> |
|||
<div class="lefts"> |
|||
<div class="icon myiconfont iconbox iconbox_blue"></div> |
|||
</div> |
|||
<div class="rights"> |
|||
<div class="note" @click="getSonList">获取子系统列表<span class="bluezi">36</span></div> |
|||
<div class="note" @click="subSystemArm">子系统布防:<span class="huizi">16</span></div> |
|||
<div class="note" @click="subSystemDisArm">子系统撤防:<span class="huizi">16</span></div> |
|||
<div class="note"><span class="redzi"></span></div> |
|||
</div> |
|||
<div class="bar"> |
|||
</div> |
|||
</div> |
|||
</div> |
|||
</div> |
|||
<!-- 第3个 --> |
|||
<div class="topboxs"> |
|||
<div class="topbox"> |
|||
<div class="toptitle" @click="createConsumer">创建消息消费者</div> |
|||
<div class="mids"> |
|||
<div class="lefts"> |
|||
<div class="icon myiconfont iconbox iconbox_zi"></div> |
|||
</div> |
|||
<div class="rights"> |
|||
<div class="note" @click="getMessageList">拉取消息:<span class="bluezi">38</span></div> |
|||
<div class="note" @click="doMessageOffsets">手动提交消息偏移量:<span class="huizi">15</span></div> |
|||
<div class="note">消费者ID:<span class="redzi">{{consumerId}}</span></div> |
|||
</div> |
|||
<div class="bar"> |
|||
</div> |
|||
</div> |
|||
</div> |
|||
</div> |
|||
<!-- 第4个 --> |
|||
<div class="topboxs"> |
|||
<div class="topbox"> |
|||
<div class="toptitle" @click="getZoneStatusList">获取所有防区状态</div> |
|||
<div class="mids"> |
|||
<div class="lefts"> |
|||
<div class="icon myiconfont iconbox iconbox_red"></div> |
|||
</div> |
|||
<div class="rights"> |
|||
<div class="note">正常:<span class="bluezi">26</span></div> |
|||
<div class="note">离线:<span class="huizi">12</span></div> |
|||
<div class="note">报警:<span class="redzi">11</span></div> |
|||
</div> |
|||
<div class="bar"> |
|||
</div> |
|||
</div> |
|||
</div> |
|||
</div> |
|||
|
|||
<!-- 11 --> |
|||
</div> |
|||
|
|||
</div> |
|||
</template> |
|||
|
|||
<script> |
|||
import { login1 } from '@/api/system/user/login.js' |
|||
import { getCallToken, getCallSonList, getCallArm, getCallDisArm, createCallConsumer, getCallMessageList, doCallMessageOffsets,getCallZoneStatusList } from '@/api/call.js' |
|||
import * as echarts from "echarts"; |
|||
import PieChartrenyuan from '@/views/echarts/components/PieChartrenyuan' |
|||
import HomeMap from '@/views/dashboard/components/HomeMap' |
|||
export default { |
|||
name: 'index', |
|||
components: { |
|||
PieChartrenyuan, HomeMap, |
|||
}, |
|||
created() { |
|||
var _self = this |
|||
}, |
|||
data() { |
|||
return { |
|||
token: '', |
|||
consumerId: '', |
|||
Datalista: [ |
|||
{ title: '您有新任务了', createTime: '2023-05-23' }, |
|||
{ title: '您有新任务了', createTime: '2023-05-23' }, |
|||
{ title: '您有新任务了', createTime: '2023-05-23' }, |
|||
{ title: '您有新任务了', createTime: '2023-05-23' }, |
|||
{ title: '您有新任务了', createTime: '2023-05-23' }, |
|||
], |
|||
Datalist: [ |
|||
{ title: '您有新消息了', createTime: '2023-05-23' }, |
|||
{ title: '您有新消息了', createTime: '2023-05-23' }, |
|||
{ title: '您有新消息了', createTime: '2023-05-23' }, |
|||
{ title: '您有新消息了', createTime: '2023-05-23' }, |
|||
{ title: '您有新消息了', createTime: '2023-05-23' }, |
|||
], |
|||
} |
|||
}, |
|||
methods: { |
|||
getToken() { |
|||
getCallToken().then(res => { |
|||
console.log(res) |
|||
}) |
|||
}, |
|||
getSonList() { |
|||
getCallSonList().then(res => { |
|||
console.log(res) |
|||
}) |
|||
}, |
|||
subSystemArm() { |
|||
getCallArm().then(res => { |
|||
console.log(res) |
|||
}) |
|||
}, |
|||
subSystemDisArm() { |
|||
getCallDisArm().then(res => { |
|||
console.log(res) |
|||
}) |
|||
}, |
|||
createConsumer() { |
|||
createCallConsumer().then(res => { |
|||
this.consumerId = res.data.consumerId |
|||
}) |
|||
}, |
|||
getMessageList() { |
|||
getCallMessageList({ consumerId: this.consumerId }).then(res => { |
|||
console.log(res) |
|||
}) |
|||
}, |
|||
doMessageOffsets() { |
|||
doCallMessageOffsets({ consumerId: this.consumerId }).then(res => { |
|||
console.log(res) |
|||
}) |
|||
}, |
|||
getZoneStatusList(){ |
|||
getCallZoneStatusList().then(res => { |
|||
console.log(res) |
|||
}) |
|||
}, |
|||
toMore() { |
|||
this.$router.push({ |
|||
path: '/baojingchaxun/baojingchaxunList', |
|||
}) |
|||
}, |
|||
getMessage() { |
|||
|
|||
this.$notify({ |
|||
title: '报警消息', |
|||
dangerouslyUseHTMLString: true, |
|||
message: " <div style='display: flex;flex-direction: row;flex-wrap: nowrap;justify-content: flex-start;align-items:center;margin: 0 auto;height:60px;line-height: 60px;'><img style='width:40px;height:40px;margin: 0 10px 0 0;' src='/image/yujing.png'>" + "<span style='display: inline-block;margin: 0 0px 0 0;height:40px;line-height: 40px;font-weight: bold;'>新的报警待处理</span></div>", |
|||
position: 'bottom-right', |
|||
onClick: () => { |
|||
alert("这是一条新订单") |
|||
}, |
|||
duration: 3000, |
|||
}) |
|||
}, |
|||
} |
|||
} |
|||
</script> |
|||
|
|||
<style lang="scss" scoped> |
|||
.zongcons { |
|||
margin: 0; |
|||
padding: 0; |
|||
flex: 1; |
|||
width: 100%; |
|||
height: calc(100% - 160px); |
|||
} |
|||
|
|||
.rightbars {} |
|||
|
|||
.zongcons .maps { |
|||
width: 100%; |
|||
height: 605px; |
|||
padding: 40px 0 0 100px; |
|||
text-align: right; |
|||
} |
|||
|
|||
.webindex { |
|||
display: flex; |
|||
flex-direction: row; |
|||
flex-wrap: nowrap; |
|||
justify-content: flex-start; |
|||
align-items: flex-start; |
|||
width: 100%; |
|||
padding: 20px; |
|||
height: 100%; |
|||
overflow-y: auto; |
|||
background: radial-gradient(#fff, #f5f7f4, #fff); |
|||
} |
|||
|
|||
.tops { |
|||
width: 470px; |
|||
// display: flex; |
|||
// flex-direction: row; |
|||
// flex-wrap: nowrap; |
|||
// justify-content: flex-start;align-items: flex-start; |
|||
padding: 0 0 0 20px; |
|||
margin: 0px; |
|||
} |
|||
|
|||
.tops .topboxs { |
|||
width: 100%; |
|||
|
|||
padding: 0px 0px 15px 0 !important; |
|||
|
|||
} |
|||
|
|||
.topbox { |
|||
// width: 25%; |
|||
//margin: 20px 20 0 0 !important; |
|||
background-color: #fff; |
|||
box-shadow: 0px 0px 10px #E9E9E9; |
|||
border-radius: 5px; |
|||
padding: 20px 10px 21px 10px; |
|||
} |
|||
|
|||
// .tops .topbox:last-of-type { |
|||
// margin: 0; |
|||
// } |
|||
.topbox .toptitle { |
|||
font-size: 20px; |
|||
padding: 10px 20px; |
|||
font-weight: bold; |
|||
color: #333; |
|||
font-size: 16px; |
|||
border-bottom: 1px solid #ececee; |
|||
} |
|||
|
|||
.topbox .mids { |
|||
display: flex; |
|||
flex-direction: row; |
|||
flex-wrap: nowrap; |
|||
justify-content: flex-start; |
|||
align-items: center; |
|||
margin: 0; |
|||
padding: 35px 20px 38px 20px; |
|||
// padding: 20px ; |
|||
} |
|||
|
|||
.topbox .mids .lefts { |
|||
width: 100px; |
|||
} |
|||
|
|||
.topbox .mids .lefts .iconbox { |
|||
border-radius: 10px; |
|||
line-height: 80px; |
|||
width: 80px; |
|||
height: 80px; |
|||
color: #fff; |
|||
font-size: 36px; |
|||
text-align: center; |
|||
} |
|||
|
|||
.iconbox_red { |
|||
background-color: #df2f07; |
|||
} |
|||
|
|||
.iconbox_yellow { |
|||
background-color: #ff7521; |
|||
} |
|||
|
|||
|
|||
.iconbox_green { |
|||
background-color: #07C160; |
|||
} |
|||
|
|||
.iconbox_blue { |
|||
background-color: #3f9bfa; |
|||
} |
|||
|
|||
.iconbox_zi { |
|||
background-color: #6421ff; |
|||
} |
|||
|
|||
.iconbox_fen { |
|||
background-color: #9b2efb; |
|||
} |
|||
|
|||
.topbox .mids .rights { |
|||
flex: 1; |
|||
padding: 2px 0 2px 10px; |
|||
} |
|||
|
|||
.topbox .mids .rights .note { |
|||
color: #333; |
|||
font-size: 14px; |
|||
padding: 0 0 3px 0; |
|||
} |
|||
|
|||
.topbox .mids .rights .note span { |
|||
font-size: 20px; |
|||
padding: 0 5px; |
|||
} |
|||
|
|||
/* 饼图部分 */ |
|||
.tbars { |
|||
display: flex; |
|||
flex-direction: row; |
|||
flex-wrap: nowrap; |
|||
justify-content: flex-start; |
|||
align-items: flex-start; |
|||
margin: 0; |
|||
padding: 20px 0 0 0; |
|||
} |
|||
|
|||
.tbar { |
|||
width: 25%; |
|||
margin: 0 20px 0 0; |
|||
background-color: #fff; |
|||
box-shadow: 0px 0px 10px #E9E9E9; |
|||
border-radius: 5px; |
|||
padding: 10px; |
|||
} |
|||
|
|||
.tbar:last-of-type { |
|||
margin: 0; |
|||
} |
|||
|
|||
.tbar0 { |
|||
border-bottom: 0px solid #032ab8; |
|||
} |
|||
|
|||
.tbar .title { |
|||
font-size: 20px; |
|||
padding: 10px 20px; |
|||
font-weight: bold; |
|||
color: #333; |
|||
font-size: 16px; |
|||
border-bottom: 1px solid #ececee; |
|||
} |
|||
|
|||
.tbar .title span { |
|||
padding: 0 10px 0 0; |
|||
} |
|||
|
|||
.tbar .bar { |
|||
display: flex; |
|||
flex-direction: row; |
|||
flex-wrap: nowrap; |
|||
justify-content: flex-start; |
|||
align-items: center; |
|||
margin: 0; |
|||
padding: 0px; |
|||
height: 190px; |
|||
|
|||
} |
|||
|
|||
.con { |
|||
padding: 10px 0 0 0; |
|||
display: flex; |
|||
flex-direction: row; |
|||
flex-wrap: nowrap; |
|||
justify-content: space-around; |
|||
} |
|||
|
|||
.news { |
|||
width: calc(100% / 2); |
|||
margin: 0 20px 0 0; |
|||
background-color: #fff; |
|||
box-shadow: 0px 0px 10px #E9E9E9; |
|||
border-radius: 5px; |
|||
padding: 10px; |
|||
} |
|||
|
|||
.news:last-of-type { |
|||
margin: 0; |
|||
} |
|||
|
|||
.news .title { |
|||
display: flex; |
|||
flex-direction: row; |
|||
flex-wrap: nowrap; |
|||
justify-content: space-between; |
|||
align-items: center; |
|||
padding: 10px 10px 10px 20px; |
|||
border-bottom: 1px solid #ececee; |
|||
} |
|||
|
|||
.news .title .newstits { |
|||
font-weight: bold; |
|||
color: #333; |
|||
font-size: 16px; |
|||
} |
|||
|
|||
.news .title .more { |
|||
text-align: right; |
|||
cursor: pointer; |
|||
color: #6c6a6a; |
|||
font-size: 14px; |
|||
} |
|||
|
|||
.news .content { |
|||
padding: 10px 0; |
|||
height: 120px; |
|||
// height:160px; |
|||
overflow: hidden; |
|||
} |
|||
|
|||
.news .content .newsli { |
|||
display: flex; |
|||
flex-direction: row; |
|||
flex-wrap: nowrap; |
|||
justify-content: space-between; |
|||
margin: 0px 0px; |
|||
padding: 5px 10px; |
|||
border-bottom: 1px solid #ececee; |
|||
} |
|||
|
|||
.news .content .newsli .dot { |
|||
padding: 0px 8px 0 0; |
|||
font-size: 18px; |
|||
height: 25px; |
|||
line-height: 25px; |
|||
color: #047cf7; |
|||
} |
|||
|
|||
.news .content .newsli .tit { |
|||
padding: 0px 0; |
|||
font-size: 14px; |
|||
flex: 3; |
|||
height: 25px; |
|||
overflow: hidden; |
|||
line-height: 25px; |
|||
cursor: pointer; |
|||
} |
|||
|
|||
// .news .content .newsli .tel{padding: 10px 0;color: #84d1f5;flex: 1;text-align: center;} |
|||
.news .content .newsli .time { |
|||
padding: 0px 0; |
|||
color: #666666; |
|||
text-align: right; |
|||
font-size: 14px; |
|||
width: 100px; |
|||
height: 25px; |
|||
line-height: 25px; |
|||
} |
|||
|
|||
///// |
|||
|
|||
.wenboxs { |
|||
padding: 20px; |
|||
margin: 0; |
|||
position: absolute; |
|||
z-index: 600; |
|||
top: 20px; |
|||
left: 20px; |
|||
// background-color: rgba($color: #ffffff, $alpha: 0.7); |
|||
// box-shadow: 0px 0px 6px #0d27c2; |
|||
background-color: #fff; |
|||
box-shadow: 0px 0px 10px #E9E9E9; |
|||
border-radius: 5px; |
|||
} |
|||
|
|||
.wenboxs .wenbox { |
|||
display: flex; |
|||
flex-direction: row; |
|||
flex-wrap: nowrap; |
|||
justify-content: flex-start; |
|||
align-items: center; |
|||
margin: 0; |
|||
font-size: 18px; |
|||
color: #8d8e90; |
|||
padding: 10px; |
|||
border-bottom: 1px solid #E9E9E9; |
|||
} |
|||
|
|||
.wenboxs .wenbox:last-of-type { |
|||
border-bottom: 0px solid #E9E9E9; |
|||
} |
|||
|
|||
.wenbox .tubiao { |
|||
margin: 0 5px; |
|||
font-size: 18px; |
|||
// color: #b7d8fa; |
|||
padding: 0 5px 0 0; |
|||
} |
|||
|
|||
.wenbox .tubiao .tolzi { |
|||
color: #3f9bfa; |
|||
font-size: 40px; |
|||
} |
|||
|
|||
.bluezi { |
|||
// color: #042d6a; |
|||
font-size: 18px !important; |
|||
} |
|||
|
|||
.wenbox .tos { |
|||
margin: 0; |
|||
font-size: 14px; |
|||
color: #474848; |
|||
padding: 0; |
|||
text-align: left; |
|||
} |
|||
|
|||
.wenbox .tos .tit { |
|||
margin: 0; |
|||
font-size: 14px; |
|||
// color: #6f7172; |
|||
padding: 0 0 10px 0; |
|||
} |
|||
|
|||
.wenbox .tos .nums { |
|||
margin: 0; |
|||
padding: 0; |
|||
} |
|||
|
|||
.wenbox .tos .nums .num { |
|||
font-size: 20px; |
|||
padding: 0 5px; |
|||
font-weight: bold; |
|||
display: inline-block; |
|||
color: #fc6f04; |
|||
} |
|||
</style> |
Loading…
Reference in new issue