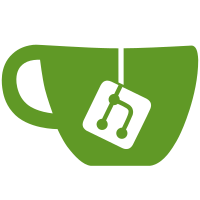
5 changed files with 86 additions and 16 deletions
@ -0,0 +1,27 @@ |
|||
package com.yxt.supervise.monitor.api.vo; |
|||
|
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.io.Serializable; |
|||
|
|||
/** |
|||
* @author Exrickx |
|||
*/ |
|||
@Data |
|||
public class PageVo implements Serializable { |
|||
|
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty(value = "页号") |
|||
private int current; |
|||
|
|||
@ApiModelProperty(value = "页面大小") |
|||
private int size; |
|||
|
|||
@ApiModelProperty(value = "排序字段") |
|||
private String sort; |
|||
|
|||
@ApiModelProperty(value = "排序方式 asc/desc") |
|||
private String order; |
|||
} |
@ -1,28 +1,64 @@ |
|||
package com.yxt.supervise.monitor.biz.service.impl; |
|||
|
|||
import com.alibaba.fastjson.JSONObject; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.supervise.monitor.api.entity.Device; |
|||
import com.yxt.supervise.monitor.biz.mapper.YDeviceMapper; |
|||
import com.yxt.supervise.monitor.biz.service.YDeviceService; |
|||
import com.yxt.supervise.monitor.biz.util.HttpUtils; |
|||
import org.springframework.stereotype.Service; |
|||
import springfox.documentation.spring.web.json.Json; |
|||
|
|||
import javax.annotation.Resource; |
|||
import java.util.HashMap; |
|||
import java.util.Map; |
|||
|
|||
@Service |
|||
public class IYDeviceServiceImpl extends ServiceImpl<YDeviceMapper, Device> implements YDeviceService { |
|||
|
|||
@Resource YDeviceMapper yDeviceMapper; |
|||
@Resource |
|||
YDeviceMapper yDeviceMapper; |
|||
|
|||
|
|||
@Override |
|||
public IPage<Device> getDevicePage(Map<String,String> searchVo){ |
|||
public IPage<Device> getDevicePage(Map<String, String> searchVo) { |
|||
return yDeviceMapper.getDevicePage(); |
|||
} |
|||
|
|||
@Override |
|||
public String createDevice(Device device){ |
|||
return null; |
|||
public ResultBean createDevice(Device device) { |
|||
ResultBean rb = ResultBean.fireSuccess(); |
|||
|
|||
// 先给海康接口添加设备 如果失败直接返回失败
|
|||
String url = "https://api2.hik-cloud.com/api/v1/open/basic/devices/create"; |
|||
Map<String, Object> param = new HashMap<>(); |
|||
param.put("deviceSerial", "Q16362484"); // 设备序列号 string
|
|||
param.put("groupNo", "A1181"); // 组编号 string
|
|||
param.put("validateCode", device.getVCode()); // 验证码 string
|
|||
String paramJson = JSONObject.toJSONString(param); |
|||
String result = HttpUtils.sendPostJson(url, paramJson, "35ad3e80-1de4-4477-827e-0473320cf644"); |
|||
JSONObject jsonObject = JSONObject.parseObject(result); |
|||
if ("200".equals(jsonObject.get("code").toString())) { |
|||
return rb.setData(this.save(device)); |
|||
} else { |
|||
rb.setData(jsonObject.get("message")); |
|||
return rb; |
|||
} |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean createDeviceGroup(Device device) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
|
|||
String url = "https://api2.hik-cloud.com/api/v1/open/basic/groups/create"; |
|||
Map<String, Object> param = new HashMap<>(); |
|||
param.put("groupName", "测试添加"); // 设备序列号 string
|
|||
param.put("groupNo", "A1181"); // 组编号 string
|
|||
String paramJson = JSONObject.toJSONString(param); |
|||
String result = HttpUtils.sendPostJson(url, paramJson, "35ad3e80-1de4-4477-827e-0473320cf644"); |
|||
rb.setData(result); |
|||
return rb; |
|||
} |
|||
} |
|||
|
Loading…
Reference in new issue