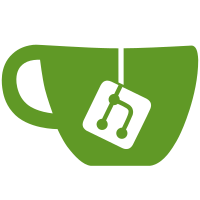
5 changed files with 3 additions and 173 deletions
@ -1,35 +0,0 @@ |
|||||
package com.yxt.supplier.feign.portal.sysorganization; |
|
||||
|
|
||||
import com.yxt.common.core.result.ResultBean; |
|
||||
import io.swagger.annotations.Api; |
|
||||
import io.swagger.annotations.ApiOperation; |
|
||||
import org.springframework.cloud.openfeign.FeignClient; |
|
||||
import org.springframework.web.bind.annotation.GetMapping; |
|
||||
import org.springframework.web.bind.annotation.PathVariable; |
|
||||
import org.springframework.web.bind.annotation.ResponseBody; |
|
||||
|
|
||||
/** |
|
||||
* Project: anrui_portal(门户建设) <br/> |
|
||||
* File: SysOrganizationFeign.java <br/> |
|
||||
* Class: com.yxt.anrui.portal.api.sysorganization.SysOrganizationFeign <br/> |
|
||||
* Description: 组织机构表. <br/> |
|
||||
* Copyright: Copyright (c) 2011 <br/> |
|
||||
* Company: https://gitee.com/liuzp315 <br/>
|
|
||||
* Makedate: 2021-08-03 00:24:28 <br/> |
|
||||
* |
|
||||
* @author liupopo |
|
||||
* @version 1.0 |
|
||||
* @since 1.0 |
|
||||
*/ |
|
||||
@Api(tags = "组织机构表") |
|
||||
@FeignClient( |
|
||||
contextId = "anrui-portal-SysOrganization", |
|
||||
name = "anrui-portal", |
|
||||
path = "v1/sysorganization") |
|
||||
public interface SysOrganizationFeign { |
|
||||
|
|
||||
@ApiOperation("获取一条记录 根据sid") |
|
||||
@ResponseBody |
|
||||
@GetMapping("/fetchBySid/{sid}") |
|
||||
public ResultBean<SysOrganizationVo> fetchBySid(@PathVariable("sid") String sid); |
|
||||
} |
|
@ -1,88 +0,0 @@ |
|||||
package com.yxt.supplier.feign.portal.sysorganization; |
|
||||
|
|
||||
|
|
||||
import com.yxt.common.core.vo.Vo; |
|
||||
import io.swagger.annotations.ApiModel; |
|
||||
import io.swagger.annotations.ApiModelProperty; |
|
||||
import lombok.Data; |
|
||||
|
|
||||
import java.util.ArrayList; |
|
||||
import java.util.List; |
|
||||
|
|
||||
/** |
|
||||
* Project: anrui_portal(门户建设) <br/> |
|
||||
* File: SysOrganizationVo.java <br/> |
|
||||
* Class: com.yxt.anrui.portal.api.sysorganization.SysOrganizationVo <br/> |
|
||||
* Description: 组织机构表 视图数据对象. <br/> |
|
||||
* Copyright: Copyright (c) 2011 <br/> |
|
||||
* Company: https://gitee.com/liuzp315 <br/>
|
|
||||
* Makedate: 2021-08-03 00:24:28 <br/> |
|
||||
* |
|
||||
* @author liupopo |
|
||||
* @version 1.0 |
|
||||
* @since 1.0 |
|
||||
*/ |
|
||||
@ApiModel(value = "组织机构表 视图数据对象", description = "组织机构表 视图数据对象") |
|
||||
@Data |
|
||||
public class SysOrganizationVo implements Vo { |
|
||||
@ApiModelProperty("部门/组织名称") |
|
||||
private String name; |
|
||||
|
|
||||
@ApiModelProperty("父(部门/组织) sid") |
|
||||
private String psid; |
|
||||
|
|
||||
@ApiModelProperty("联系电话") |
|
||||
private String linkPhone; |
|
||||
|
|
||||
@ApiModelProperty("联系人") |
|
||||
private String linkPerson; |
|
||||
|
|
||||
@ApiModelProperty("部门sid全路径") |
|
||||
private String orgSidPath; |
|
||||
|
|
||||
@ApiModelProperty("排序") |
|
||||
private Integer sort; |
|
||||
|
|
||||
@ApiModelProperty("地址") |
|
||||
private String addrs; |
|
||||
|
|
||||
@ApiModelProperty("地理位置经纬度") |
|
||||
private String jwd; |
|
||||
|
|
||||
@ApiModelProperty("二维码") |
|
||||
private String qrText; |
|
||||
|
|
||||
@ApiModelProperty("限制本部门成员查看通讯录:限制开启后,本部门成员只能看到限定范围内的通讯录不能看到所有通讯录,仅可见自己") |
|
||||
private Integer limitOrgMember; |
|
||||
|
|
||||
@ApiModelProperty("部门编码") |
|
||||
private String orgCode; |
|
||||
@ApiModelProperty("sid") |
|
||||
private String sid; |
|
||||
@ApiModelProperty("子集") |
|
||||
private List<SysOrganizationVo> children = new ArrayList<>(); |
|
||||
@ApiModelProperty("主管人员名称") |
|
||||
private String zgNames; |
|
||||
@ApiModelProperty("分管人员名称") |
|
||||
private String fgNames; |
|
||||
@ApiModelProperty("主管人员sid") |
|
||||
private String zgStaffSid; |
|
||||
@ApiModelProperty("分管人员sid") |
|
||||
private String fgStaffSid; |
|
||||
@ApiModelProperty("组织简称") |
|
||||
private String orgAbbre; |
|
||||
@ApiModelProperty("是否是部门(0否,1是)") |
|
||||
private Integer isDept; |
|
||||
@ApiModelProperty("组织属性key") |
|
||||
private String orgAttributeKey; |
|
||||
@ApiModelProperty("组织属性value") |
|
||||
private String orgAttributeValue; |
|
||||
@ApiModelProperty("管理层级key") |
|
||||
private String orgLevelKey; |
|
||||
@ApiModelProperty("管理层级value") |
|
||||
private String orgLevelValue; |
|
||||
@ApiModelProperty("其他编码") |
|
||||
private String otherCode; |
|
||||
@ApiModelProperty("主管用户sid") |
|
||||
private String managerSid; |
|
||||
} |
|
@ -1,25 +0,0 @@ |
|||||
package com.yxt.supplier.feign.portal.sysstafforg; |
|
||||
|
|
||||
import com.yxt.common.core.result.ResultBean; |
|
||||
import io.swagger.annotations.ApiOperation; |
|
||||
import org.springframework.cloud.openfeign.FeignClient; |
|
||||
import org.springframework.web.bind.annotation.GetMapping; |
|
||||
import org.springframework.web.bind.annotation.RequestParam; |
|
||||
import org.springframework.web.bind.annotation.ResponseBody; |
|
||||
|
|
||||
/** |
|
||||
* @description: 人员与机构 |
|
||||
* @author: dimengzhe |
|
||||
* @date: 2024/3/6 |
|
||||
**/ |
|
||||
@FeignClient( |
|
||||
contextId = "anrui-portal-SysStaffOrg", |
|
||||
name = "anrui-portal", |
|
||||
path = "v1/sysstafforg") |
|
||||
public interface SysStaffOrgFeign { |
|
||||
|
|
||||
@GetMapping("/getOrgSidByPath") |
|
||||
@ResponseBody |
|
||||
@ApiOperation("根据用户组织全路径获取用户的分公司sid") |
|
||||
ResultBean<String> getOrgSidByPath(@RequestParam("orgPath") String orgPath); |
|
||||
} |
|
Loading…
Reference in new issue