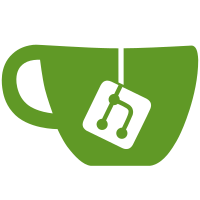
32 changed files with 358 additions and 1331 deletions
@ -0,0 +1,67 @@ |
|||||
|
import axios from 'axios' |
||||
|
import { MessageBox, Message } from 'element-ui' |
||||
|
import store from '@/store' |
||||
|
import { getToken, getStorage } from '@/utils/auth' |
||||
|
|
||||
|
// create an axios instance
|
||||
|
console.log(process.env.VUE_APP_URL) |
||||
|
const service = axios.create({ |
||||
|
baseURL: '/mockapi', // url = base url + request url
|
||||
|
// timeout: 5000 // request timeout
|
||||
|
headers:{'Content-Type':'application/x-www-form-urlencoded;'} |
||||
|
}) |
||||
|
|
||||
|
// request interceptor
|
||||
|
service.interceptors.request.use( |
||||
|
config => { |
||||
|
config.params = { |
||||
|
...config.params, |
||||
|
_t: Date.parse(new Date()) / 1000 |
||||
|
} |
||||
|
if (getStorage()) { |
||||
|
|
||||
|
config.headers['token'] = getStorage() |
||||
|
} |
||||
|
return config |
||||
|
}, |
||||
|
error => { |
||||
|
console.log(error) // for debug
|
||||
|
return Promise.reject(error) |
||||
|
} |
||||
|
) |
||||
|
|
||||
|
// response interceptor
|
||||
|
service.interceptors.response.use( |
||||
|
|
||||
|
response => { |
||||
|
const res = response.data |
||||
|
if(res.type !=undefined){ |
||||
|
return res |
||||
|
} |
||||
|
|
||||
|
if(res.contractNo !=undefined){ |
||||
|
return res |
||||
|
} |
||||
|
// if the custom code is not 20000, it is judged as an error.
|
||||
|
if (res.code != 200) { |
||||
|
if (res.msg == "请重新登录") { |
||||
|
// window.location.href = 'http://39.104.100.138:8082/'
|
||||
|
} else { |
||||
|
alert(res.msg); |
||||
|
} |
||||
|
} |
||||
|
return res |
||||
|
}, |
||||
|
error => { |
||||
|
console.log('err' + error) // for debug
|
||||
|
Message({ |
||||
|
message: error.message, |
||||
|
type: 'error', |
||||
|
duration: 5 * 1000 |
||||
|
}) |
||||
|
|
||||
|
return Promise.reject(error) |
||||
|
} |
||||
|
) |
||||
|
|
||||
|
export default service |
@ -0,0 +1,13 @@ |
|||||
|
<template> |
||||
|
|
||||
|
</template> |
||||
|
|
||||
|
<script> |
||||
|
export default { |
||||
|
name: "confirmLender" |
||||
|
} |
||||
|
</script> |
||||
|
|
||||
|
<style scoped> |
||||
|
|
||||
|
</style> |
@ -0,0 +1,129 @@ |
|||||
|
<template> |
||||
|
<div class="app-container"> |
||||
|
<!-- <div class="tab-header webtop">--> |
||||
|
<!-- <div>价格维护</div>--> |
||||
|
<!-- <div>--> |
||||
|
<!-- <el-button type="info" size="small" @click="handleReturn()">关闭</el-button>--> |
||||
|
<!-- </div>--> |
||||
|
<!-- </div>--> |
||||
|
<!-- <div class="listconadd">--> |
||||
|
<!-- <el-form ref="printForm" :model="temp" label-position="right" class="formadd" :rules="rules">--> |
||||
|
<!-- <el-row class="tleftb_one">--> |
||||
|
<!-- <el-col :span="6" class="tleftb">--> |
||||
|
<!-- <span>车型名称</span>--> |
||||
|
<!-- </el-col>--> |
||||
|
<!-- <el-col :span="18">--> |
||||
|
<!-- <el-form-item>--> |
||||
|
<!-- <span>{{ temp.modelName }}</span>--> |
||||
|
<!-- </el-form-item>--> |
||||
|
<!-- </el-col>--> |
||||
|
<!-- </el-row>--> |
||||
|
<!-- <el-row>--> |
||||
|
<!-- <el-col :span="6" class="tleftb">--> |
||||
|
<!-- <span>常用配置</span>--> |
||||
|
<!-- </el-col>--> |
||||
|
<!-- <el-col :span="18">--> |
||||
|
<!-- <el-form-item>--> |
||||
|
<!-- <span>{{ temp.configName }}</span>--> |
||||
|
<!-- </el-form-item>--> |
||||
|
<!-- </el-col>--> |
||||
|
<!-- </el-row>--> |
||||
|
<!-- <el-row>--> |
||||
|
<!-- <el-col :span="6" class="tleftb">--> |
||||
|
<!-- <span>更多配置</span>--> |
||||
|
<!-- </el-col>--> |
||||
|
<!-- <el-col :span="18">--> |
||||
|
<!-- <el-form-item>--> |
||||
|
<!-- <span>{{ temp.otherConfig }}</span>--> |
||||
|
<!-- </el-form-item>--> |
||||
|
<!-- </el-col>--> |
||||
|
<!-- </el-row>--> |
||||
|
<!-- <el-row>--> |
||||
|
<!-- <el-col :span="6" class="tleftb">--> |
||||
|
<!-- <span>厂家结算价(万元)</span>--> |
||||
|
<!-- </el-col>--> |
||||
|
<!-- <el-col :span="6">--> |
||||
|
<!-- <el-form-item>--> |
||||
|
<!-- <el-input v-model="temp.manufactorSettlementPrice" maxlength="125" placeholder="请输入" class="addinputw" clearable/>--> |
||||
|
<!-- </el-form-item>--> |
||||
|
<!-- </el-col>--> |
||||
|
<!-- <el-col :span="6" class="tleftb">--> |
||||
|
<!-- <span>销售指导价(万元)</span>--> |
||||
|
<!-- </el-col>--> |
||||
|
<!-- <el-col :span="6">--> |
||||
|
<!-- <el-form-item>--> |
||||
|
<!-- <el-input v-model="temp.guidedPrice" maxlength="125" placeholder="请输入" class="addinputw" clearable/>--> |
||||
|
<!-- </el-form-item>--> |
||||
|
<!-- </el-col>--> |
||||
|
<!-- </el-row>--> |
||||
|
<!-- </el-form>--> |
||||
|
<!-- <div class="form_btn">--> |
||||
|
<!-- <el-button type="primary" size="small" @click="handleCreate()">确认</el-button>--> |
||||
|
<!-- <el-button type="info" size="small" @click="handleReturn()">返回</el-button>--> |
||||
|
<!-- </div>--> |
||||
|
<!-- </div>--> |
||||
|
</div> |
||||
|
</template> |
||||
|
|
||||
|
<script> |
||||
|
export default { |
||||
|
name: "printContract", |
||||
|
data() { |
||||
|
return { |
||||
|
temp: { |
||||
|
|
||||
|
}, |
||||
|
rules: {} |
||||
|
} |
||||
|
}, |
||||
|
methods: { |
||||
|
// showPrint(sid) { |
||||
|
// this.$nextTick(() => { |
||||
|
// this.$refs['weihuForm'].clearValidate() |
||||
|
// }) |
||||
|
// fetchBySid(sid).then(resp => { |
||||
|
// if (resp.success) { |
||||
|
// const data = resp.data |
||||
|
// this.temp = data |
||||
|
// this.temp.sid = sid |
||||
|
// } |
||||
|
// }) |
||||
|
// }, |
||||
|
// 返回 |
||||
|
// handleReturn(isreload) { |
||||
|
// if (isreload === 'true') this.$emit('reloadlist') |
||||
|
// this.temp = {} |
||||
|
// this.$emit('doback') |
||||
|
// }, |
||||
|
// // 保存修改数据 |
||||
|
// handleCreate() { |
||||
|
// this.$refs['printForm'].validate(valid => { |
||||
|
// if (valid) { |
||||
|
// const temp = { |
||||
|
// |
||||
|
// } |
||||
|
// // update(temp).then(resp => { |
||||
|
// // if (resp.success) { |
||||
|
// // const data = resp.data |
||||
|
// // this.temp = data |
||||
|
// // this.handleReturn('true') |
||||
|
// // } |
||||
|
// // }) |
||||
|
// } |
||||
|
// }) |
||||
|
// } |
||||
|
|
||||
|
} |
||||
|
} |
||||
|
</script> |
||||
|
|
||||
|
<style scoped> |
||||
|
/*.tleftb_one {*/ |
||||
|
/* border-top: 1px solid #e0e3eb;*/ |
||||
|
/*}*/ |
||||
|
|
||||
|
/*.form_btn {*/ |
||||
|
/* display: flex;*/ |
||||
|
/* justify-content: center;*/ |
||||
|
/*}*/ |
||||
|
</style> |
@ -1,643 +0,0 @@ |
|||||
<template> |
|
||||
<div class="app-container"> |
|
||||
<div class="tab-header webtop"> |
|
||||
<div>{{ viewTitle }}</div> |
|
||||
<div> |
|
||||
<el-button type="primary" size="small" @click="handleCreate()">保存</el-button> |
|
||||
<el-button type="primary" size="small">下载</el-button> |
|
||||
<el-button type="info" size="small" @click="handleReturn()">返回</el-button> |
|
||||
</div> |
|
||||
</div> |
|
||||
<div class="listconadd"> |
|
||||
<el-form ref="dataForm" :model="temp" label-position="right" label-width="190px" class="formadd" :rules="rules"> |
|
||||
<div class="title">合格证台账信息</div> |
|
||||
<el-row> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">合格证流水号:</span> |
|
||||
<el-input v-model="temp.certificationSerialNum" maxlength="125" placeholder="合格证流水号" disabled class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item prop="certificationNo"> |
|
||||
<span slot="label">合格证编号:</span> |
|
||||
<el-input v-model="temp.certificationNo" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item prop="certificateDate"> |
|
||||
<span slot="label">发证日期:</span> |
|
||||
<el-date-picker v-model="temp.certificateDate" type="date" class="addinputw" format="yyyy-MM-dd" value-format="yyyy-MM-dd" placeholder="选择日期时间"/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item prop="certificateSituation"> |
|
||||
<span slot="label">合格证情况:</span> |
|
||||
<el-select v-model="temp.certificateSituation" class="addinputw" placeholder="请选择合格证情况" @change="getHeGeZheng"> |
|
||||
<el-option v-for="item in Situation" :key="item.dictKey" :label="item.dictValue" :value="item.dictKey"/> |
|
||||
</el-select> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item prop="toCardDate"> |
|
||||
<span slot="label">到证日期:</span> |
|
||||
<el-date-picker v-model="temp.toCardDate" type="date" class="addinputw" format="yyyy-MM-dd" value-format="yyyy-MM-dd" placeholder="选择日期时间"/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item prop="receiveDate"> |
|
||||
<span slot="label">领取日期:</span> |
|
||||
<el-date-picker v-model="temp.receiveDate" type="date" class="addinputw" format="yyyy-MM-dd" value-format="yyyy-MM-dd" placeholder="选择日期时间"/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item prop="receiver"> |
|
||||
<span slot="label">领取人:</span> |
|
||||
<el-input v-model="temp.receiver" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
<el-col :span="12"/> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="24"> |
|
||||
<el-form-item prop="remarks"> |
|
||||
<span slot="label">备注:</span> |
|
||||
<el-input v-model="temp.remarks" type="textarea" :rows="3" maxlength="125" placeholder="" class="addinputwda" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="24"> |
|
||||
<el-form-item prop="certificatePhoto"> |
|
||||
<span slot="label">合格证照片:</span> |
|
||||
<ImageUpload types="0001" style="float:left;" v-model="temp.certificatePhoto"/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
</el-row> |
|
||||
<div class="title">合格证详细信息</div> |
|
||||
<el-row> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">底盘ID:</span> |
|
||||
<el-input v-model="temp.chassisId" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">转向类型:</span> |
|
||||
<el-input v-model="temp.steeringType" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">制造日期:</span> |
|
||||
<el-date-picker v-model="temp.manufactureDate" type="date" format="yyyy-MM-dd" class="addinputw" value-format="yyyy-MM-dd" placeholder="选择日期时间"/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">排放标准:</span> |
|
||||
<el-input v-model="temp.emissionStandard" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">轴距:</span> |
|
||||
<el-input v-model="temp.wheelbase" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">驾驶室准乘人数:</span> |
|
||||
<el-input v-model="temp.seatingCapacity" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">轴数:</span> |
|
||||
<el-input v-model="temp.axleNum" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">燃料类型:</span> |
|
||||
<el-input v-model="temp.fuelType" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">额定载客人数:</span> |
|
||||
<el-input v-model="temp.limitPassenger" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">车身颜色:</span> |
|
||||
<el-input v-model="temp.carColor" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">制造厂商:</span> |
|
||||
<el-autocomplete v-model="temp.manufacturer" :fetch-suggestions="querySearchAsync3" class="addinputw" placeholder="请输入制造厂商" @select="handleSelect3"> |
|
||||
<i slot="suffix" class="el-icon-edit el-input__icon" @click="inputclear3"/> |
|
||||
<template slot-scope="{ item }"> |
|
||||
<div style="paddingg:5px;background-color:Azure;"> |
|
||||
<span>制造厂商:</span> |
|
||||
<span style="color:red">{{ item.manufacturerName }}</span><br> |
|
||||
</div> |
|
||||
</template> |
|
||||
</el-autocomplete> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">发动机型号:</span> |
|
||||
<el-input v-model="temp.engineType" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">整备质量:</span> |
|
||||
<el-input v-model="temp.saddleMass" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">发动机号:</span> |
|
||||
<el-input v-model="temp.engineNo" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">最高设计时速:</span> |
|
||||
<el-input v-model="temp.speedLimit" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">合格印章:</span> |
|
||||
<el-input v-model="temp.qualifySeal" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">车辆名称:</span> |
|
||||
<el-input v-model="temp.carName" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">排量:</span> |
|
||||
<el-input v-model="temp.displacement" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">CGS印章:</span> |
|
||||
<el-input v-model="temp.cgsseal" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">功率:</span> |
|
||||
<el-input v-model="temp.power" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">轮胎数:</span> |
|
||||
<el-input v-model="temp.tyreNum" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">总质量:</span> |
|
||||
<el-input v-model="temp.totalWeight" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">车辆品牌:</span> |
|
||||
<el-select v-model="temp.carBrand" class="addinputw" placeholder="请选择车辆型号"> |
|
||||
<el-option v-for="item in carBrandes" :key="item.sid" :label="item.brandName" :value="item.sid"/> |
|
||||
</el-select> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">车辆型号:</span> |
|
||||
<el-select v-model="temp.carModel" class="addinputw" placeholder="请选择车辆型号"> |
|
||||
<el-option v-for="item in Model" :key="item.sid" :label="item.carModel" :value="item.carModel"/> |
|
||||
</el-select> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">车架号:</span> |
|
||||
<el-autocomplete v-model="temp.vinNo" :fetch-suggestions="querySearchAsync" class="addinputw" placeholder="请输入车架号" @select="handleSelect"> |
|
||||
<i slot="suffix" class="el-icon-edit el-input__icon"/> |
|
||||
<template slot-scope="{ item }"> |
|
||||
<div style="paddingg:5px;background-color:Azure;"> |
|
||||
<span>车架号:</span> |
|
||||
<span style="color:red">{{ item.vinNo }}</span><br> |
|
||||
</div> |
|
||||
</template> |
|
||||
</el-autocomplete> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
<el-col :span="12"> |
|
||||
<el-form-item> |
|
||||
<span slot="label">底盘型号:</span> |
|
||||
<el-input v-model="temp.chassisModel" maxlength="125" placeholder="" class="addinputw" clearable/> |
|
||||
</el-form-item> |
|
||||
</el-col> |
|
||||
</el-row> |
|
||||
</el-form> |
|
||||
</div> |
|
||||
</div> |
|
||||
</template> |
|
||||
|
|
||||
<script> |
|
||||
import { mapGetters } from 'vuex' |
|
||||
import { |
|
||||
SaveList, |
|
||||
Update, |
|
||||
details |
|
||||
} from '@/api/cheliang/basevehiclecertificate' |
|
||||
import { |
|
||||
selectDownPlus |
|
||||
} from '@/api/cheliang/basevehiclemodel' |
|
||||
import { |
|
||||
namesDownBySid |
|
||||
} from '@/api/cheliang/basevehiclemodel' |
|
||||
import { |
|
||||
namesDown |
|
||||
} from '@/api/cheliang/basevehicle' |
|
||||
import { |
|
||||
getNamesDownes |
|
||||
} from '@/api/cheliang/basemanufacturer' |
|
||||
import { getChe } from '@/utils/baocun' |
|
||||
import { typeValues } from '@/api/cheliang/dictcommons' |
|
||||
import ImageUpload from '@/components/uploadFile/ManyImageUpload' // 上传文件 |
|
||||
export default { |
|
||||
name: 'hegezhengAdd', |
|
||||
components: { ImageUpload }, |
|
||||
data() { |
|
||||
return { |
|
||||
viewTitle: '', |
|
||||
// --按钮菜单------- |
|
||||
menuState: { |
|
||||
add: false, // 添加 |
|
||||
edit: false, // 编辑 |
|
||||
delete: false, // 删除 |
|
||||
view: false, // 查看 |
|
||||
audit: false, // 审核 |
|
||||
input: false, // 导入 |
|
||||
output: false, // 导出 |
|
||||
upload: false, // 同步 |
|
||||
release: false // 下发 |
|
||||
}, |
|
||||
FormLoading: false, |
|
||||
listLoading: false, |
|
||||
temp: { |
|
||||
certificateSituation: '', |
|
||||
certificateSituationValue: '', |
|
||||
certificatePhoto: [] |
|
||||
}, // 添加和修改 |
|
||||
templook: {}, // 查看实体 |
|
||||
textMap: { |
|
||||
update: '修改', |
|
||||
create: '创建' |
|
||||
}, |
|
||||
certificateSituation: 'certificateSituation', |
|
||||
Situation: [], |
|
||||
stateId: 0, |
|
||||
dialogFormVisible: false, // 添加修改对话框状态 |
|
||||
dialogFormShowVisible: false, // 查看对话框默认关闭状态 |
|
||||
dialogStatus: '', // 对话框状态 |
|
||||
fenzuOptions: [], |
|
||||
attachTypeYingyezhizhao: [], |
|
||||
props: { |
|
||||
value: 'id', |
|
||||
label: 'name', |
|
||||
children: 'children', |
|
||||
checkStrictly: true |
|
||||
}, |
|
||||
carBrandes: [], |
|
||||
ChangShangsid: '', |
|
||||
CJsid: '', |
|
||||
vinNosse: [], |
|
||||
Model: [], |
|
||||
vSid: null, |
|
||||
rules: { |
|
||||
certificationNo: [ |
|
||||
{ required: true, message: '请填写合格证编号', trigger: 'blur' } |
|
||||
], |
|
||||
certificateDate: [ |
|
||||
{ required: true, message: '请选择发证日期', trigger: 'blur' } |
|
||||
], |
|
||||
certificateSituation: [ |
|
||||
{ required: true, message: '请选择合格证情况', trigger: 'blur' } |
|
||||
], |
|
||||
toCardDate: [ |
|
||||
{ required: true, message: '请选择到证日期', trigger: 'blur' } |
|
||||
], |
|
||||
receiveDate: [ |
|
||||
{ required: true, message: '请选择领取日期', trigger: 'blur' } |
|
||||
], |
|
||||
receiver: [ |
|
||||
{ required: true, message: '请填写领取人', trigger: 'blur' } |
|
||||
], |
|
||||
remarks: [{ required: true, message: '请填写备注', trigger: 'blur' }] |
|
||||
// certificatePhoto: [ |
|
||||
// { required: true, message: '请选择合格证照片', trigger: 'blur' }, |
|
||||
// ], |
|
||||
} |
|
||||
// ------------------------------------ |
|
||||
} |
|
||||
}, |
|
||||
computed: { |
|
||||
...mapGetters([ |
|
||||
'id', |
|
||||
'roles', |
|
||||
'rolesIds', |
|
||||
'departmentId', |
|
||||
'departmentCode' |
|
||||
]) |
|
||||
}, |
|
||||
created() { |
|
||||
this.setDate() |
|
||||
this.getXChangshang() |
|
||||
// this.getCheXingHao() |
|
||||
this.getCheJia() |
|
||||
this.vSid = getChe() |
|
||||
console.log('999999999999999999999', this.vSid) |
|
||||
// 初始化变量 |
|
||||
// this.init() |
|
||||
// 加载列表 |
|
||||
// this.getList() |
|
||||
}, |
|
||||
methods: { |
|
||||
init() { |
|
||||
this.stateId = this.$route.params.id |
|
||||
if (this.stateId !== '0') { |
|
||||
details(this.stateId).then((response) => { |
|
||||
if (response.code === '200') { |
|
||||
this.temp = response.data |
|
||||
this.getPinPainting(response.data.manufacturer) |
|
||||
console.log(this.temp.carBrand, 1111) |
|
||||
} |
|
||||
}) |
|
||||
} |
|
||||
this.setDate() |
|
||||
this.getXChangshang() |
|
||||
// this.getCheXingHao() |
|
||||
this.getCheJia() |
|
||||
}, |
|
||||
showAdd() { |
|
||||
this.$nextTick(() => { |
|
||||
this.$refs['dataForm'].clearValidate() |
|
||||
}) |
|
||||
this.dialogStatus = 'add' |
|
||||
this.viewTitle = '新增合格证台账信息' |
|
||||
}, |
|
||||
|
|
||||
showEdit(sid, row) { |
|
||||
this.$nextTick(() => { |
|
||||
this.$refs['dataForm'].clearValidate() |
|
||||
}) |
|
||||
this.viewTitle = '修改车型详细信息' |
|
||||
this.dialogStatus = 'update' |
|
||||
this.temp.sid = sid |
|
||||
details(sid).then(resp => { |
|
||||
const data = resp.data |
|
||||
this.temp = data |
|
||||
}).catch(e => { |
|
||||
this.temp = row |
|
||||
}) |
|
||||
}, |
|
||||
|
|
||||
getCheXingHao(sid) { |
|
||||
var sid = { |
|
||||
sid: sid |
|
||||
} |
|
||||
console.log('wowopwpwp', sid) |
|
||||
selectDownPlus(sid).then((res) => { |
|
||||
console.log(res) |
|
||||
if (res.code === '200') { |
|
||||
this.Model = res.data |
|
||||
} |
|
||||
}) |
|
||||
}, |
|
||||
// 车架号 |
|
||||
getCheJia() { |
|
||||
namesDown({ vinNo: this.temp.vinNo }).then((response) => { |
|
||||
if (response.code === '200') { |
|
||||
this.vinNosse = response.data |
|
||||
} |
|
||||
}) |
|
||||
}, |
|
||||
// 选择数据 |
|
||||
handleSelect(e) { |
|
||||
this.temp.vinNo = e.vinNo |
|
||||
this.CJsid = e.sid |
|
||||
this.temp.vehicleSid = this.CJsid |
|
||||
}, |
|
||||
// 文本模糊查询 |
|
||||
querySearchAsync(queryString, cb) { |
|
||||
console.log('查询条件:', queryString) |
|
||||
if ( |
|
||||
queryString !== null && |
|
||||
queryString !== undefined && |
|
||||
queryString !== '' |
|
||||
) { |
|
||||
namesDown({ |
|
||||
name: queryString |
|
||||
}).then((response) => { |
|
||||
if (response.code === '200') { |
|
||||
console.log('1111111111111', response) |
|
||||
this.datas = response.data |
|
||||
cb(response.data) |
|
||||
} |
|
||||
}) |
|
||||
} else { |
|
||||
cb(this.vinNosse) |
|
||||
} |
|
||||
}, |
|
||||
// 厂商 |
|
||||
getXChangshang() { |
|
||||
getNamesDownes({ name: this.temp.manufacturer }).then((res) => { |
|
||||
if (res.code === '200') { |
|
||||
console.log('777777777777', res) |
|
||||
this.facturer = res.data |
|
||||
} |
|
||||
}) |
|
||||
}, |
|
||||
handleSelect3(e) { |
|
||||
console.log('46588888888888', e) |
|
||||
this.temp.manufacturer = e.manufacturerName |
|
||||
this.ChangShangsid = e.sid |
|
||||
this.getPinPainting(this.ChangShangsid) |
|
||||
}, |
|
||||
// 清空信息 |
|
||||
inputclear3() { |
|
||||
this.manufacturerName = '' |
|
||||
this.brandCode = '' |
|
||||
}, |
|
||||
// 文本模糊查询 |
|
||||
querySearchAsync3(queryString, cb) { |
|
||||
console.log('查询条件:', queryString) |
|
||||
|
|
||||
if (queryString !== null && queryString !== undefined && queryString !== '') { |
|
||||
getNamesDownes({ |
|
||||
name: queryString |
|
||||
}).then((response) => { |
|
||||
if (response.code === '200') { |
|
||||
console.log('1111111111111', response) |
|
||||
this.datas = response.data |
|
||||
cb(response.data) |
|
||||
} |
|
||||
}) |
|
||||
} else { |
|
||||
cb(this.facturer) |
|
||||
} |
|
||||
}, |
|
||||
// 品牌 |
|
||||
getPinPainting(sid) { |
|
||||
var manufacturerSid = { |
|
||||
manufacturerSid: sid |
|
||||
} |
|
||||
namesDownBySid(manufacturerSid).then((res) => { |
|
||||
if (res.code === '200') { |
|
||||
console.log('品牌数据', res) |
|
||||
this.carBrandes = res.data |
|
||||
this.carBrandes.forEach((e) => { |
|
||||
this.getCheXingHao(e.sid) |
|
||||
}) |
|
||||
} |
|
||||
}) |
|
||||
}, |
|
||||
// |
|
||||
getHeGeZheng(value) { |
|
||||
let bb = null |
|
||||
this.Situation.forEach(e => { |
|
||||
if (e.dictKey === value) { |
|
||||
bb = { |
|
||||
type: 'certificateSituation', |
|
||||
name: e.dictValue, |
|
||||
vaule: e.dictKey |
|
||||
} |
|
||||
} |
|
||||
}) |
|
||||
this.temp.certificateSituationValue = bb.name |
|
||||
console.log('value值', this.temp.certificateSituationValue) |
|
||||
}, |
|
||||
setDate() { |
|
||||
typeValues({ type: this.certificateSituation }).then((res) => { |
|
||||
console.log(res) |
|
||||
if (res.code === '200') { |
|
||||
this.Situation = res.data |
|
||||
} |
|
||||
}) |
|
||||
}, |
|
||||
// ----------------------------------方法-------------------- |
|
||||
// 返回 |
|
||||
handleReturn(isreload) { |
|
||||
if (isreload === 'true') this.$emit('reloadlist') |
|
||||
this.$emit('doback') |
|
||||
}, |
|
||||
// 提交添加数据 |
|
||||
handleCreate() { |
|
||||
// console.log('提交:' + JSON.stringify(this.temp)) |
|
||||
this.$refs['dataForm'].validate((valid) => { |
|
||||
if (valid) { |
|
||||
this.FormLoading = true |
|
||||
if (this.dialogStatus === 'add') { |
|
||||
console.log('3333333333333', this.temp) |
|
||||
// this.temp.certificatePhoto = this.attachTypeYingyezhizhao |
|
||||
SaveList(this.temp).then((response) => { |
|
||||
console.log('数据聚聚聚' + JSON.stringify(response)) |
|
||||
this.FormLoading = false |
|
||||
if (response.code === '200') { |
|
||||
this.dialogFormVisible = false |
|
||||
this.$notify({ |
|
||||
title: '提示', |
|
||||
message: '添加成功', |
|
||||
type: 'success', |
|
||||
duration: 2000 |
|
||||
}) |
|
||||
this.handleReturn('true') |
|
||||
} else { |
|
||||
this.$notify({ |
|
||||
title: '提示', |
|
||||
message: '添加失败', |
|
||||
type: 'error', |
|
||||
duration: 2000 |
|
||||
}) |
|
||||
} |
|
||||
}) |
|
||||
} else { |
|
||||
// this.temp.certificatePhoto = this.attachTypeYingyezhizhao |
|
||||
Update(this.temp).then((response) => { |
|
||||
this.FormLoading = false |
|
||||
if (response.code === '200') { |
|
||||
// this.getList() |
|
||||
this.dialogFormVisible = false |
|
||||
this.$notify({ |
|
||||
title: '提示', |
|
||||
message: '修改成功', |
|
||||
type: 'success', |
|
||||
duration: 2000 |
|
||||
}) |
|
||||
this.handleReturn('true') |
|
||||
} else { |
|
||||
this.$notify({ |
|
||||
title: '失败', |
|
||||
message: '修改失败', |
|
||||
type: 'error' |
|
||||
}) |
|
||||
} |
|
||||
}) |
|
||||
} |
|
||||
} |
|
||||
}) |
|
||||
} |
|
||||
} |
|
||||
} |
|
||||
</script> |
|
||||
<style lang="scss" scoped> |
|
||||
.addinputwda { |
|
||||
width: 600px; |
|
||||
} |
|
||||
</style> |
|
@ -1,178 +0,0 @@ |
|||||
<template> |
|
||||
<div class="app-container"> |
|
||||
<div class="tab-header webtop"> |
|
||||
<div>合格证台账详情信息</div> |
|
||||
<div> |
|
||||
<el-button type="primary" size="small">下载</el-button> |
|
||||
<el-button type="info" size="small" @click="handleReturn()">返回</el-button> |
|
||||
</div> |
|
||||
</div> |
|
||||
<div class="listconadd"> |
|
||||
<el-form ref="dataForm" :model="temp" label-position="right" label-width="190px" class="forminfo" :rules="rules"> |
|
||||
<div class="title">合格证台账详情信息</div> |
|
||||
<el-row> |
|
||||
<el-col :span="4" class="trightb">合格证流水号:</el-col> |
|
||||
<el-col :span="8"> {{ temp.certificationSerialNum }}</el-col> |
|
||||
<el-col :span="4" class="trightb">合格证编号:</el-col> |
|
||||
<el-col :span="8" class="tleft"> {{ temp.certificationNo }}</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="4" class="trightb">发证日期:</el-col> |
|
||||
<el-col :span="8"> {{ temp.certificateDate }}</el-col> |
|
||||
<el-col :span="4" class="trightb">合格证情况:</el-col> |
|
||||
<el-col :span="8" class="tleft"> {{ temp.certificateSituationValue }}</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="4" class="trightb">到证日期:</el-col> |
|
||||
<el-col :span="8"> {{ temp.toCardDate }}</el-col> |
|
||||
<el-col :span="4" class="trightb">领取日期:</el-col> |
|
||||
<el-col :span="8" class="tleft"> {{ temp.receiveDate }}</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="4" class="trightb">领取人:</el-col> |
|
||||
<el-col :span="8"> {{ temp.receiver }}</el-col> |
|
||||
<el-col :span="4" class="trightb"/> |
|
||||
<el-col :span="8" class="tleft"/> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="4" class="trightb">备注:</el-col> |
|
||||
<el-col :span="20"> {{ temp.remarks }}</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="4" class="trightb">合格证照片:</el-col> |
|
||||
<img v-for="(map_item,index) in temp.certificatePhoto" style="width: 100px; height: 100px;margin-right: 5px;" :key="index" :src="map_item"> |
|
||||
</el-row> |
|
||||
<div class="title">合格证详细信息</div> |
|
||||
<el-row> |
|
||||
<el-col :span="4" class="trightb">底盘ID:</el-col> |
|
||||
<el-col :span="8"> {{ temp.chassisId }}</el-col> |
|
||||
<el-col :span="4" class="trightb">转向类型:</el-col> |
|
||||
<el-col :span="8" class="tleft"> {{ temp.steeringType }}</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="4" class="trightb">制造日期:</el-col> |
|
||||
<el-col :span="8"> {{ temp.manufactureDate }}</el-col> |
|
||||
<el-col :span="4" class="trightb">排放标准:</el-col> |
|
||||
<el-col :span="8" class="tleft"> {{ temp.emissionStandard }}</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="4" class="trightb">轴距:</el-col> |
|
||||
<el-col :span="8"> {{ temp.wheelbase }}</el-col> |
|
||||
<el-col :span="4" class="trightb">驾驶室准乘人数:</el-col> |
|
||||
<el-col :span="8" class="tleft"> {{ temp.seatingCapacity }}</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="4" class="trightb">轴数:</el-col> |
|
||||
<el-col :span="8"> {{ temp.axleNum }}</el-col> |
|
||||
<el-col :span="4" class="trightb">燃料类型:</el-col> |
|
||||
<el-col :span="8" class="tleft"> {{ temp.fuelType }}</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="4" class="trightb">额定载客人数:</el-col> |
|
||||
<el-col :span="8"> {{ temp.limitPassenger }}</el-col> |
|
||||
<el-col :span="4" class="trightb">车身颜色:</el-col> |
|
||||
<el-col :span="8" class="tleft"> {{ temp.carColor }}</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="4" class="trightb">制造厂商:</el-col> |
|
||||
<el-col :span="8"> {{ temp.manufacturer }}</el-col> |
|
||||
<el-col :span="4" class="trightb">发动机型号:</el-col> |
|
||||
<el-col :span="8" class="tleft"> {{ temp.engineType }}</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="4" class="trightb">整备质量:</el-col> |
|
||||
<el-col :span="8"> {{ temp.saddleMass }}</el-col> |
|
||||
<el-col :span="4" class="trightb">发动机号:</el-col> |
|
||||
<el-col :span="8" class="tleft"> {{ temp.engineNo }}</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="4" class="trightb">最高设计时速:</el-col> |
|
||||
<el-col :span="8"> {{ temp.speedLimit }}</el-col> |
|
||||
<el-col :span="4" class="trightb">合格印章:</el-col> |
|
||||
<el-col :span="8" class="tleft"> {{ temp.qualifySeal }}</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="4" class="trightb">车辆名称:</el-col> |
|
||||
<el-col :span="8"> {{ temp.carName }}</el-col> |
|
||||
<el-col :span="4" class="trightb">排量:</el-col> |
|
||||
<el-col :span="8" class="tleft"> {{ temp.displacement }}</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="4" class="trightb">CGS印章:</el-col> |
|
||||
<el-col :span="8"> {{ temp.cgsseal }}</el-col> |
|
||||
<el-col :span="4" class="trightb">车辆型号:</el-col> |
|
||||
<el-col :span="8" class="tleft"> {{ temp.carModel }}</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="4" class="trightb">车架号:</el-col> |
|
||||
<el-col :span="8"> {{ temp.vinNo }}</el-col> |
|
||||
<el-col :span="4" class="trightb">总质量:</el-col> |
|
||||
<el-col :span="8" class="tleft"> {{ temp.totalWeight }}</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="4" class="trightb">轮胎数:</el-col> |
|
||||
<el-col :span="8"> {{ temp.tyreNum }}</el-col> |
|
||||
<el-col :span="4" class="trightb">车辆品牌:</el-col> |
|
||||
<el-col :span="8" class="tleft"> {{ temp.carBrand }}</el-col> |
|
||||
</el-row> |
|
||||
<el-row> |
|
||||
<el-col :span="4" class="trightb">功率:</el-col> |
|
||||
<el-col :span="8"> {{ temp.power }}</el-col> |
|
||||
<el-col :span="4" class="trightb">底盘型号:</el-col> |
|
||||
<el-col :span="8" class="tleft"> {{ temp.chassisModel }}</el-col> |
|
||||
</el-row> |
|
||||
|
|
||||
</el-form> |
|
||||
</div> |
|
||||
</div> |
|
||||
</template> |
|
||||
|
|
||||
<script> |
|
||||
import { details } from '@/api/cheliang/basevehiclecertificate' |
|
||||
export default { |
|
||||
name: 'hegezhengInfo', |
|
||||
// components: { }, |
|
||||
data() { |
|
||||
return { |
|
||||
FormLoading: false, |
|
||||
listLoading: false, |
|
||||
temp: {}, // 添加和修改 |
|
||||
templook: {}, // 查看实体 |
|
||||
textMap: { |
|
||||
update: '修改', |
|
||||
create: '创建' |
|
||||
}, |
|
||||
tempDate: {}, |
|
||||
stateId: 0, |
|
||||
dialogFormVisible: false, // 添加修改对话框状态 |
|
||||
dialogFormShowVisible: false, // 查看对话框默认关闭状态 |
|
||||
dialogStatus: '', // 对话框状态 |
|
||||
fenzuOptions: [], |
|
||||
rules: {} |
|
||||
} |
|
||||
}, |
|
||||
methods: { |
|
||||
// 返回 |
|
||||
handleReturn() { |
|
||||
this.temp = {} |
|
||||
this.$emit('doback') |
|
||||
// this.$router.go(-1) |
|
||||
}, |
|
||||
// 打开查看 |
|
||||
showInfo(sid) { |
|
||||
details(sid).then((response) => { |
|
||||
console.log('0000000000000') |
|
||||
if (response.code === '200') { |
|
||||
this.temp = response.data |
|
||||
console.log(this.temp, 555555555555) |
|
||||
} |
|
||||
}) |
|
||||
} |
|
||||
} |
|
||||
} |
|
||||
</script> |
|
||||
<style lang="scss" scoped> |
|
||||
.addinputwda { |
|
||||
width: 600px; |
|
||||
} |
|
||||
</style> |
|
@ -1,364 +0,0 @@ |
|||||
<template> |
|
||||
<div class="app-container"> |
|
||||
<div v-show="viewState === 1"> |
|
||||
<div class="tab-header webtop"> |
|
||||
<div>合格证台账</div> |
|
||||
<div> |
|
||||
<el-button type="primary" size="small" @click="handleCreate()">新增</el-button> |
|
||||
<el-button type="primary" size="small" @click="handleUpdate()">修改</el-button> |
|
||||
<el-button type="danger" size="small" @click="handleDelete()">删除</el-button> |
|
||||
<!-- <el-button type="primary" size="small" @click="handleDaoRu()">导入</el-button> --> |
|
||||
<el-button type="success" size="small" @click="handleDaoChu()">导出</el-button> |
|
||||
</div> |
|
||||
</div> |
|
||||
<div class="searchcon"> |
|
||||
<el-button size="small" class="searchbtn" @click="clicksearchShow">{{ searchxianshitit }}</el-button> |
|
||||
<div v-show="isSearchShow" class="search"> |
|
||||
<el-form ref="listQueryform" :inline="true" :model="listQuery" label-width="100px" class="tab-header"> |
|
||||
<el-form-item label="合格证编号"> |
|
||||
<el-input v-model="listQuery.certificationNo" placeholder="请输入合格证编号" clearable class="filter-item"/> |
|
||||
</el-form-item> |
|
||||
<el-form-item label="发证日期"> |
|
||||
<el-date-picker v-model="listQuery.certificateDate" type="date" class="addinputw" format="yyyy-MM-dd" value-format="yyyy-MM-dd" placeholder="选择日期时间"/> |
|
||||
</el-form-item> |
|
||||
<el-form-item label="合格证情况"> |
|
||||
<el-select v-model="listQuery.certificateSituation" class="addinputw" clearable placeholder="请选择合格证情况" style="width: 200px;"> |
|
||||
<el-option v-for="item in Situation" :key="item.dictKey" :label="item.dictValue" :value="item.dictKey"/> |
|
||||
</el-select> |
|
||||
</el-form-item> |
|
||||
<el-button type="primary" @click="handleFilter">查询</el-button> |
|
||||
</el-form> |
|
||||
</div> |
|
||||
</div> |
|
||||
<div class="listtop"> |
|
||||
<div class="tit">合格证台账信息列表</div> |
|
||||
<pageye v-show="total>0" :total="total" :page.sync="listQuery.current" :limit.sync="listQuery.size" class="pagination" @pagination="getList"/> |
|
||||
</div> |
|
||||
<div class=""> |
|
||||
<el-table :key="tableKey" v-loading="listLoading" :data="list" border style="width: 100%;" @selection-change="handleSelectionChange"> |
|
||||
<el-table-column width="50px" type="selection" align="center"/> |
|
||||
<el-table-column width="80px" label="序号" type="index" :index="indexMethod" align="center"/> |
|
||||
<el-table-column label="合格证编号" align="center"> |
|
||||
<template slot-scope="scope"> |
|
||||
<span>{{ scope.row.certificationNo }}</span> |
|
||||
</template> |
|
||||
</el-table-column> |
|
||||
<el-table-column label="发证日期" align="center"> |
|
||||
<template slot-scope="scope"> |
|
||||
<span>{{ scope.row.certificateDate }}</span> |
|
||||
</template> |
|
||||
</el-table-column> |
|
||||
<el-table-column label="合格证情况" align="center"> |
|
||||
<template slot-scope="scope"> |
|
||||
<span>{{ scope.row.certificateSituationValue }}</span> |
|
||||
</template> |
|
||||
</el-table-column> |
|
||||
<el-table-column label="到证日期" align="center"> |
|
||||
<template slot-scope="scope"> |
|
||||
<span>{{ scope.row.toCardDate }}</span> |
|
||||
</template> |
|
||||
</el-table-column> |
|
||||
<el-table-column label="领取日期" align="center"> |
|
||||
<template slot-scope="scope"> |
|
||||
<span>{{ scope.row.receiveDate }}</span> |
|
||||
</template> |
|
||||
</el-table-column> |
|
||||
<el-table-column label="领取人" align="center"> |
|
||||
<template slot-scope="scope"> |
|
||||
<span>{{ scope.row.receiver }}</span> |
|
||||
</template> |
|
||||
</el-table-column> |
|
||||
<el-table-column label="备注" align="center"> |
|
||||
<template slot-scope="scope"> |
|
||||
<span>{{ scope.row.remarks }}</span> |
|
||||
</template> |
|
||||
</el-table-column> |
|
||||
<el-table-column label="合格证照片" align="center"> |
|
||||
<template slot-scope="scope"> |
|
||||
<el-button size="mini" type="primary" @click="handleLock(scope.row)">查看</el-button> |
|
||||
</template> |
|
||||
</el-table-column> |
|
||||
<el-table-column label="操作" align="center" width="280px" class-name="small-padding fixed-width"> |
|
||||
<template slot-scope="{row}"> |
|
||||
<el-button size="mini" type="primary" @click="handleCheck(row)">查看</el-button> |
|
||||
<el-button size="mini" type="primary" @click="cheLiangXiangXi(row)">车辆信息</el-button> |
|
||||
</template> |
|
||||
</el-table-column> |
|
||||
</el-table> |
|
||||
</div> |
|
||||
<div class="pages"> |
|
||||
<!-- 翻页 --> |
|
||||
<pagination v-show="total>0" :total="total" :page.sync="listQuery.current" :limit.sync="listQuery.size" class="pagination" @pagination="getList"/> |
|
||||
</div> |
|
||||
<el-dialog :title="textMap[dialogStatus]" :visible.sync="dialogFormVisible" width="1000px" :close-on-click-modal="false"> |
|
||||
<div class="result-cont"> |
|
||||
<el-carousel indicator-position="outside" style="height: 500px;"> |
|
||||
<el-carousel-item v-for="item in imgs" :key="item" style="height: 500px;"> |
|
||||
<img style="width: 100%; height: 500px;" :src="item"> |
|
||||
</el-carousel-item> |
|
||||
</el-carousel> |
|
||||
</div> |
|
||||
</el-dialog> |
|
||||
</div> |
|
||||
<hegezhengAdd v-show="viewState == 2 || viewState == 3" ref="divadd" @doback="resetState" @reloadlist="getList"/> |
|
||||
<hegezhengInfo v-show="viewState == 4" ref="divinfo" @doback="resetState"/> |
|
||||
</div> |
|
||||
</template> |
|
||||
|
|
||||
<script> |
|
||||
import { |
|
||||
pagerList, |
|
||||
deleteBySids, |
|
||||
basefinbankExportExcel |
|
||||
} from '@/api/cheliang/basevehiclecertificate' |
|
||||
import { typeValues } from '@/api/cheliang/dictcommons' |
|
||||
import Pagination from '@/components/pagination' |
|
||||
import pageye from '@/components/pagination/pageye' |
|
||||
import hegezhengAdd from './hegezhengAdd' |
|
||||
import hegezhengInfo from './hegezhengInfo' |
|
||||
|
|
||||
export default { |
|
||||
name: 'hegezhenggaunli', |
|
||||
components: { |
|
||||
Pagination, |
|
||||
pageye, |
|
||||
hegezhengAdd, |
|
||||
hegezhengInfo |
|
||||
}, |
|
||||
data() { |
|
||||
return { |
|
||||
viewState: 1, |
|
||||
isSearchShow: false, |
|
||||
searchxianshitit: '显示查询条件', |
|
||||
imgs: [], |
|
||||
// 查询 ----------- |
|
||||
tableKey: 0, |
|
||||
list: [], |
|
||||
sids: [], |
|
||||
total: 1, |
|
||||
FormLoading: false, |
|
||||
listLoading: false, |
|
||||
listQuery: { |
|
||||
certificationNo: '', |
|
||||
certificateDateL: '', |
|
||||
certificateSituation: '', |
|
||||
current: 1, |
|
||||
size: 20 |
|
||||
}, |
|
||||
certificateSituation: 'certificateSituation', |
|
||||
Situation: [], |
|
||||
selectDate: undefined, |
|
||||
temp: {}, // 添加和修改 |
|
||||
templook: {}, // 查看实体 |
|
||||
textMap: { |
|
||||
update: '编辑', |
|
||||
create: '创建' |
|
||||
}, |
|
||||
dialogFormVisible: false, // 添加修改对话框状态 |
|
||||
dialogFormShowVisible: false, // 查看对话框默认关闭状态 |
|
||||
dialogStatus: '', // 对话框状态 |
|
||||
typeOptions: [], |
|
||||
rules: {} |
|
||||
} |
|
||||
}, |
|
||||
created() { |
|
||||
// 初始化变量 |
|
||||
this.init() |
|
||||
// 加载列表 |
|
||||
this.getList() |
|
||||
}, |
|
||||
methods: { |
|
||||
// 搜索条件效果 |
|
||||
clicksearchShow() { |
|
||||
this.isSearchShow = !this.isSearchShow |
|
||||
if (this.isSearchShow) { |
|
||||
this.searchxianshitit = '隐藏查询条件' |
|
||||
} else { |
|
||||
this.searchxianshitit = '显示查询条件' |
|
||||
} |
|
||||
}, |
|
||||
init() { |
|
||||
this.setDate() |
|
||||
}, |
|
||||
// 序号 |
|
||||
indexMethod(index) { |
|
||||
var pagestart = (this.listQuery.current - 1) * this.listQuery.size |
|
||||
var pageindex = index + 1 + pagestart |
|
||||
return pageindex |
|
||||
}, |
|
||||
resetState() { |
|
||||
this.viewState = 1 |
|
||||
}, |
|
||||
setDate() { |
|
||||
typeValues({ |
|
||||
type: this.certificateSituation |
|
||||
}).then((res) => { |
|
||||
console.log(res) |
|
||||
if (res.code === '200') { |
|
||||
this.Situation = res.data |
|
||||
} |
|
||||
}) |
|
||||
}, |
|
||||
// 查询列表信息 |
|
||||
getList() { |
|
||||
this.listLoading = true |
|
||||
pagerList({ |
|
||||
current: this.listQuery.current, |
|
||||
size: this.listQuery.size, |
|
||||
params: { |
|
||||
certificationNo: this.listQuery.certificationNo, |
|
||||
certificateDateL: this.listQuery.certificateDateL, |
|
||||
certificateSituation: this.listQuery.certificateSituation |
|
||||
} |
|
||||
}).then((response) => { |
|
||||
console.log('列表查询结果:', response) |
|
||||
this.listLoading = false |
|
||||
if ( |
|
||||
response.code === '200' && |
|
||||
response.data && |
|
||||
response.data.total > 0 |
|
||||
) { |
|
||||
this.list = response.data.records |
|
||||
this.total = response.data.total |
|
||||
} else { |
|
||||
this.list = [] |
|
||||
this.total = 0 |
|
||||
} |
|
||||
}) |
|
||||
}, |
|
||||
// 查询按钮 |
|
||||
handleFilter() { |
|
||||
this.listQuery.current = 1 |
|
||||
this.getList() |
|
||||
}, |
|
||||
handleSelectionChange(row) { |
|
||||
const aa = [] |
|
||||
row.forEach((element) => { |
|
||||
aa.push(element.sid) |
|
||||
}) |
|
||||
this.sids = aa |
|
||||
}, |
|
||||
// 打开添加 |
|
||||
handleCreate() { |
|
||||
this.viewState = 2 |
|
||||
this.$refs['divadd'].showAdd() |
|
||||
}, |
|
||||
// 打开 |
|
||||
cheLiangXiangXi(row) { |
|
||||
console.log('0222221133', row) |
|
||||
this.$router.push({ |
|
||||
path: '/cheliang/cheliangtaizhangInfo', |
|
||||
query: { |
|
||||
sid: row.vehicleSid |
|
||||
} |
|
||||
}) |
|
||||
}, |
|
||||
// 打开修改 |
|
||||
handleUpdate() { |
|
||||
if (this.sids.length === 1) { |
|
||||
this.dialogStatus = 'update' |
|
||||
this.viewState = 3 |
|
||||
const sid = this.sids[0] |
|
||||
const row = this.row |
|
||||
this.$refs['divadd'].showEdit(sid, row) |
|
||||
} else if (this.sids.length > 1) { |
|
||||
this.$notify({ |
|
||||
title: '提示', |
|
||||
message: '不能选中多个合格证台账修改!!', |
|
||||
type: 'info', |
|
||||
duration: 2000 |
|
||||
}) |
|
||||
} else { |
|
||||
this.$notify({ |
|
||||
title: '提示', |
|
||||
message: '没有选择合格证台账!!', |
|
||||
type: 'error', |
|
||||
duration: 2000 |
|
||||
}) |
|
||||
return |
|
||||
} |
|
||||
}, |
|
||||
// 打开查看 |
|
||||
handleCheck(row) { |
|
||||
console.log('222222', row) |
|
||||
this.viewState = 4 |
|
||||
const sid = row.sid |
|
||||
this.$refs['divinfo'].showInfo(sid) |
|
||||
}, |
|
||||
// 根据本行ID删除数据 |
|
||||
handleDelete() { |
|
||||
if (this.sids.length > 0) { |
|
||||
deleteBySids(this.sids).then((response) => { |
|
||||
if (response.code === '200') { |
|
||||
this.$notify({ |
|
||||
title: '提示', |
|
||||
message: '删除成功', |
|
||||
type: 'success', |
|
||||
duration: 2000 |
|
||||
}) |
|
||||
this.getList() |
|
||||
} else { |
|
||||
this.$notify({ |
|
||||
title: '失败', |
|
||||
message: response.msg, |
|
||||
type: 'error' |
|
||||
}) |
|
||||
} |
|
||||
}) |
|
||||
} else { |
|
||||
this.$notify({ |
|
||||
title: '提示', |
|
||||
message: '没有选择合格证台账!!', |
|
||||
type: 'error', |
|
||||
duration: 2000 |
|
||||
}) |
|
||||
return |
|
||||
} |
|
||||
}, |
|
||||
// 导出 |
|
||||
handleDaoChu() { |
|
||||
basefinbankExportExcel(this.sids).then((res) => { |
|
||||
const blob = new Blob([res], { |
|
||||
type: 'application/vnd.ms-excel' |
|
||||
}) |
|
||||
const objectUrl = URL.createObjectURL(blob) |
|
||||
window.location.href = objectUrl |
|
||||
this.$notify({ |
|
||||
title: '提示', |
|
||||
message: '导出成功', |
|
||||
type: 'success', |
|
||||
duration: 2000 |
|
||||
}) |
|
||||
}) |
|
||||
}, |
|
||||
handleLock(row) { |
|
||||
this.imgs = row.certificatePhoto |
|
||||
this.dialogFormVisible = true |
|
||||
this.dialogTitle = '查看' |
|
||||
} |
|
||||
} |
|
||||
} |
|
||||
</script> |
|
||||
<style scoped> |
|
||||
/deep/ .el-collapse { |
|
||||
border-top: 0px solid #e6ebf5; |
|
||||
border-bottom: 0px solid #e6ebf5; |
|
||||
} |
|
||||
|
|
||||
/deep/ .el-collapse-item__content { |
|
||||
margin: 0; |
|
||||
padding: 0; |
|
||||
} |
|
||||
|
|
||||
/deep/ .el-collapse-item__wrap { |
|
||||
border-bottom: 0px solid #ebeef5; |
|
||||
} |
|
||||
|
|
||||
/deep/ .el-collapse-item__header { |
|
||||
border-bottom: 0px solid #e6ebf5; |
|
||||
} |
|
||||
|
|
||||
.searchli { |
|
||||
padding: 5px 100px; |
|
||||
} |
|
||||
</style> |
|
Loading…
Reference in new issue