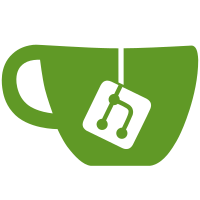
18 changed files with 453 additions and 38 deletions
@ -0,0 +1,40 @@ |
|||
package com.yxt.anrui.crm.api.crmcustomertemp; |
|||
|
|||
import com.fasterxml.jackson.annotation.JsonProperty; |
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2024/1/30 |
|||
**/ |
|||
@Data |
|||
public class CustomerSecondSalesDto implements Dto { |
|||
private static final long serialVersionUID = -1955772984622113809L; |
|||
|
|||
@ApiModelProperty("客户sid") |
|||
private String customerSid; |
|||
@ApiModelProperty("客户名称") |
|||
private String customerName; |
|||
@ApiModelProperty("客户类型") |
|||
private String customerType; |
|||
private String customerTypeKey; |
|||
@ApiModelProperty("联系电话") |
|||
private String mobile; |
|||
@ApiModelProperty("证件类型") |
|||
private String certificateTypeKey; |
|||
private String certificateType; |
|||
@ApiModelProperty("证件号码") |
|||
@JsonProperty("IDNumber") |
|||
private String IDNumber; |
|||
@ApiModelProperty("证件有效期") |
|||
private String endDate; |
|||
@ApiModelProperty("证件地址") |
|||
private String certificateAddress; |
|||
|
|||
private String userSid; |
|||
|
|||
private String orgPath; |
|||
} |
@ -0,0 +1,35 @@ |
|||
package com.yxt.anrui.riskcenter.api.loansecondarysalescustomer; |
|||
|
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2024/1/30 |
|||
**/ |
|||
@Data |
|||
public class LoanSecondarySalesCustomer extends BaseEntity { |
|||
private static final long serialVersionUID = -4647743383421777867L; |
|||
|
|||
private String mainSid; |
|||
@ApiModelProperty("客户sid") |
|||
private String customerSid; |
|||
@ApiModelProperty("客户名称") |
|||
private String customerName; |
|||
@ApiModelProperty("客户类型") |
|||
private String customerType; |
|||
private String customerTypeKey; |
|||
@ApiModelProperty("联系电话") |
|||
private String mobile; |
|||
@ApiModelProperty("证件类型") |
|||
private String certificateTypeKey; |
|||
private String certificateType; |
|||
@ApiModelProperty("证件号码") |
|||
private String IDNumber; |
|||
@ApiModelProperty("证件有效期") |
|||
private String endDate; |
|||
@ApiModelProperty("证件地址") |
|||
private String certificateAddress; |
|||
} |
@ -0,0 +1,38 @@ |
|||
package com.yxt.anrui.riskcenter.api.loansecondarysalescustomer; |
|||
|
|||
import com.fasterxml.jackson.annotation.JsonProperty; |
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2024/1/30 |
|||
**/ |
|||
@Data |
|||
public class LoanSecondarySalesCustomerDto implements Dto { |
|||
private static final long serialVersionUID = 4377892244342062357L; |
|||
|
|||
private String mainSid; |
|||
|
|||
@ApiModelProperty("客户sid") |
|||
private String customerSid; |
|||
@ApiModelProperty("客户名称") |
|||
private String customerName; |
|||
@ApiModelProperty("客户类型") |
|||
private String customerType; |
|||
private String customerTypeKey; |
|||
@ApiModelProperty("联系电话") |
|||
private String mobile; |
|||
@ApiModelProperty("证件类型") |
|||
private String certificateTypeKey; |
|||
private String certificateType; |
|||
@ApiModelProperty("证件号码") |
|||
@JsonProperty("IDNumber") |
|||
private String IDNumber; |
|||
@ApiModelProperty("证件有效期") |
|||
private String endDate; |
|||
@ApiModelProperty("证件地址") |
|||
private String certificateAddress; |
|||
} |
@ -0,0 +1,31 @@ |
|||
package com.yxt.anrui.riskcenter.api.loansecondarysalescustomer; |
|||
|
|||
import com.yxt.anrui.riskcenter.api.loansecondarysalesapply.LoanSecondarySalesApplyFeignFallback; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
import org.springframework.web.bind.annotation.GetMapping; |
|||
import org.springframework.web.bind.annotation.PostMapping; |
|||
import org.springframework.web.bind.annotation.RequestBody; |
|||
import org.springframework.web.bind.annotation.RequestParam; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2024/1/30 |
|||
**/ |
|||
@FeignClient( |
|||
contextId = "anrui-riskcenter-LoanSecondarySalesCustomer", |
|||
name = "anrui-riskcenter", |
|||
path = "v1/LoanSecondarySalesCustomer", |
|||
fallback = LoanSecondarySalesCustomerFeignFallback.class) |
|||
public interface LoanSecondarySalesCustomerFeign { |
|||
|
|||
@ApiOperation("新增或修改") |
|||
@PostMapping("/saveOrUpdate") |
|||
ResultBean saveOrUpdate(@RequestBody LoanSecondarySalesCustomerDto dto); |
|||
|
|||
@ApiOperation("初始化") |
|||
@GetMapping("/getDetails") |
|||
ResultBean<SalesInitVo> getDetails(@RequestParam("sid") String sid); |
|||
} |
@ -0,0 +1,12 @@ |
|||
package com.yxt.anrui.riskcenter.api.loansecondarysalescustomer; |
|||
|
|||
import org.springframework.stereotype.Component; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2024/1/30 |
|||
**/ |
|||
@Component |
|||
public class LoanSecondarySalesCustomerFeignFallback { |
|||
} |
@ -0,0 +1,48 @@ |
|||
package com.yxt.anrui.riskcenter.api.loansecondarysalescustomer; |
|||
|
|||
import com.fasterxml.jackson.annotation.JsonProperty; |
|||
import com.yxt.anrui.riskcenter.api.loansecondarysalesveh.LoanSecondarySalesVehVo; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.ArrayList; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2024/1/30 |
|||
**/ |
|||
@Data |
|||
public class SalesInitVo { |
|||
|
|||
private String mainSid; |
|||
@ApiModelProperty("销售部门") |
|||
private String createDept; |
|||
@ApiModelProperty("销售日期") |
|||
private String saleDate; |
|||
@ApiModelProperty("销售价格") |
|||
private String salePrice; |
|||
|
|||
@ApiModelProperty("客户sid") |
|||
private String customerSid; |
|||
@ApiModelProperty("客户名称") |
|||
private String customerName; |
|||
@ApiModelProperty("客户类型") |
|||
private String customerType; |
|||
private String customerTypeKey; |
|||
@ApiModelProperty("联系电话") |
|||
private String mobile; |
|||
@ApiModelProperty("证件类型") |
|||
private String certificateTypeKey; |
|||
private String certificateType; |
|||
@ApiModelProperty("证件号码") |
|||
@JsonProperty("IDNumber") |
|||
private String IDNumber; |
|||
@ApiModelProperty("证件有效期") |
|||
private String endDate; |
|||
@ApiModelProperty("证件地址") |
|||
private String certificateAddress; |
|||
@ApiModelProperty("车辆列表") |
|||
private List<LoanSecondarySalesVehVo> loanSecondarySalesVehVoList = new ArrayList<>(); |
|||
} |
@ -0,0 +1,15 @@ |
|||
package com.yxt.anrui.riskcenter.biz.loansecondarysalescustomer; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.yxt.anrui.riskcenter.api.loansecondarysalescustomer.LoanSecondarySalesCustomer; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2024/1/30 |
|||
**/ |
|||
@Mapper |
|||
public interface LoanSecondarySalesCustomerMapper extends BaseMapper<LoanSecondarySalesCustomer> { |
|||
LoanSecondarySalesCustomer selectByMainSid(String mainSid); |
|||
} |
@ -0,0 +1,10 @@ |
|||
<?xml version="1.0" encoding="UTF-8" ?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.anrui.riskcenter.biz.loansecondarysalescustomer.LoanSecondarySalesCustomerMapper"> |
|||
<select id="selectByMainSid" |
|||
resultType="com.yxt.anrui.riskcenter.api.loansecondarysalescustomer.LoanSecondarySalesCustomer"> |
|||
select * |
|||
from loan_secondary_sales_customer |
|||
where mainSid = #{mainSid} |
|||
</select> |
|||
</mapper> |
@ -0,0 +1,34 @@ |
|||
package com.yxt.anrui.riskcenter.biz.loansecondarysalescustomer; |
|||
|
|||
import com.yxt.anrui.riskcenter.api.loansecondarysalescustomer.LoanSecondarySalesCustomerDto; |
|||
import com.yxt.anrui.riskcenter.api.loansecondarysalescustomer.LoanSecondarySalesCustomerFeign; |
|||
import com.yxt.anrui.riskcenter.api.loansecondarysalescustomer.SalesInitVo; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import io.swagger.annotations.Api; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.web.bind.annotation.RequestMapping; |
|||
import org.springframework.web.bind.annotation.RestController; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2024/1/30 |
|||
**/ |
|||
@RestController |
|||
@RequestMapping("v1/LoanSecondarySalesCustomer") |
|||
@Api(tags = "二次销售补充客户信息") |
|||
public class LoanSecondarySalesCustomerRest implements LoanSecondarySalesCustomerFeign { |
|||
|
|||
@Autowired |
|||
private LoanSecondarySalesCustomerService loanSecondarySalesCustomerService; |
|||
|
|||
@Override |
|||
public ResultBean saveOrUpdate(LoanSecondarySalesCustomerDto dto) { |
|||
return loanSecondarySalesCustomerService.saveSalesCustomer(dto); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<SalesInitVo> getDetails(String sid) { |
|||
return loanSecondarySalesCustomerService.getDetails(sid); |
|||
} |
|||
} |
@ -0,0 +1,83 @@ |
|||
package com.yxt.anrui.riskcenter.biz.loansecondarysalescustomer; |
|||
|
|||
import cn.hutool.core.bean.BeanUtil; |
|||
import com.yxt.anrui.crm.api.crmcustomer.CrmCustomer; |
|||
import com.yxt.anrui.crm.api.crmcustomertemp.CrmCustomerTemp; |
|||
import com.yxt.anrui.crm.api.crmcustomertemp.CrmCustomerTempFeign; |
|||
import com.yxt.anrui.crm.api.crmcustomertemp.CustomerSecondSalesDto; |
|||
import com.yxt.anrui.riskcenter.api.loansecondarysalesapply.LoanSecondarySalesApply; |
|||
import com.yxt.anrui.riskcenter.api.loansecondarysalescustomer.LoanSecondarySalesCustomer; |
|||
import com.yxt.anrui.riskcenter.api.loansecondarysalescustomer.LoanSecondarySalesCustomerDto; |
|||
import com.yxt.anrui.riskcenter.api.loansecondarysalescustomer.SalesInitVo; |
|||
import com.yxt.anrui.riskcenter.biz.loansecondarysalesapply.LoanSecondarySalesApplyService; |
|||
import com.yxt.common.base.service.MybatisBaseService; |
|||
import com.yxt.common.base.utils.StringUtils; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2024/1/30 |
|||
**/ |
|||
@Service |
|||
public class LoanSecondarySalesCustomerService extends MybatisBaseService<LoanSecondarySalesCustomerMapper, LoanSecondarySalesCustomer> { |
|||
|
|||
@Autowired |
|||
private CrmCustomerTempFeign crmCustomerTempFeign; |
|||
@Autowired |
|||
private LoanSecondarySalesApplyService loanSecondarySalesApplyService; |
|||
|
|||
public ResultBean saveSalesCustomer(LoanSecondarySalesCustomerDto dto) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
LoanSecondarySalesCustomer loanSecondarySalesCustomer = baseMapper.selectByMainSid(dto.getMainSid()); |
|||
String customerSid = dto.getCustomerSid(); |
|||
LoanSecondarySalesApply loanSecondarySalesApply = loanSecondarySalesApplyService.fetchBySid(dto.getMainSid()); |
|||
String userSid = loanSecondarySalesApply.getCreateBySid(); |
|||
String orgPath = loanSecondarySalesApply.getOrgSidPath(); |
|||
if (loanSecondarySalesCustomer == null) { |
|||
//新增客户
|
|||
CustomerSecondSalesDto customerSecondSalesDto = new CustomerSecondSalesDto(); |
|||
BeanUtil.copyProperties(dto, customerSecondSalesDto); |
|||
customerSecondSalesDto.setUserSid(userSid); |
|||
customerSecondSalesDto.setOrgPath(orgPath); |
|||
ResultBean<String> resultBean = crmCustomerTempFeign.updateTemp(customerSecondSalesDto); |
|||
if (!resultBean.getSuccess()) { |
|||
return rb.setMsg(resultBean.getMsg()); |
|||
} |
|||
customerSid = resultBean.getData(); |
|||
loanSecondarySalesCustomer = new LoanSecondarySalesCustomer(); |
|||
BeanUtil.copyProperties(dto, loanSecondarySalesCustomer); |
|||
loanSecondarySalesCustomer.setCustomerSid(customerSid); |
|||
baseMapper.insert(loanSecondarySalesCustomer); |
|||
} else { |
|||
//新增客户
|
|||
CustomerSecondSalesDto customerSecondSalesDto = new CustomerSecondSalesDto(); |
|||
BeanUtil.copyProperties(dto, customerSecondSalesDto); |
|||
customerSecondSalesDto.setUserSid(userSid); |
|||
customerSecondSalesDto.setOrgPath(orgPath); |
|||
ResultBean<String> resultBean = crmCustomerTempFeign.updateTemp(customerSecondSalesDto); |
|||
if (!resultBean.getSuccess()) { |
|||
return rb.setMsg(resultBean.getMsg()); |
|||
} |
|||
customerSid = resultBean.getData(); |
|||
BeanUtil.copyProperties(dto, loanSecondarySalesCustomer); |
|||
loanSecondarySalesCustomer.setCustomerSid(customerSid); |
|||
baseMapper.updateById(loanSecondarySalesCustomer); |
|||
} |
|||
return rb.success(); |
|||
} |
|||
|
|||
public ResultBean<SalesInitVo> getDetails(String sid) { |
|||
ResultBean<SalesInitVo> rb = ResultBean.fireFail(); |
|||
SalesInitVo salesInitVo = new SalesInitVo(); |
|||
LoanSecondarySalesCustomer loanSecondarySalesCustomer = baseMapper.selectByMainSid(sid); |
|||
if (loanSecondarySalesCustomer != null) { |
|||
BeanUtil.copyProperties(loanSecondarySalesCustomer, salesInitVo); |
|||
} else { |
|||
salesInitVo.setMainSid(sid); |
|||
} |
|||
return rb.success().setData(salesInitVo); |
|||
} |
|||
} |
Loading…
Reference in new issue