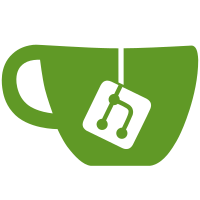
17 changed files with 514 additions and 55 deletions
@ -0,0 +1,81 @@ |
|||||
|
/********************************************************* |
||||
|
********************************************************* |
||||
|
******************** ******************* |
||||
|
************* ************ |
||||
|
******* _oo0oo_ ******* |
||||
|
*** o8888888o *** |
||||
|
* 88" . "88 * |
||||
|
* (| -_- |) * |
||||
|
* 0\ = /0 * |
||||
|
* ___/`---'\___ * |
||||
|
* .' \\| |// '. *
|
||||
|
* / \\||| : |||// \ *
|
||||
|
* / _||||| -:- |||||- \ * |
||||
|
* | | \\\ - /// | | *
|
||||
|
* | \_| ''\---/'' |_/ | * |
||||
|
* \ .-\__ '-' ___/-. / * |
||||
|
* ___'. .' /--.--\ `. .'___ * |
||||
|
* ."" '< `.___\_<|>_/___.' >' "". * |
||||
|
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
||||
|
* \ \ `_. \_ __\ /__ _/ .-` / / * |
||||
|
* =====`-.____`.___ \_____/___.-`___.-'===== * |
||||
|
* `=---=' * |
||||
|
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
||||
|
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
||||
|
*********************************************************/ |
||||
|
package com.yxt.anrui.as.feign.sms; |
||||
|
|
||||
|
import com.baomidou.mybatisplus.annotation.TableName; |
||||
|
import com.yxt.common.core.domain.BaseEntity; |
||||
|
import io.swagger.annotations.ApiModel; |
||||
|
import io.swagger.annotations.ApiModelProperty; |
||||
|
import lombok.Data; |
||||
|
|
||||
|
import java.math.BigDecimal; |
||||
|
|
||||
|
/** |
||||
|
* Project: yxt-sms(价格策略) <br/> |
||||
|
* File: SmsPricestrategy.java <br/> |
||||
|
* Class: com.yxt.sms.api.smspricestrategy.SmsPricestrategy <br/> |
||||
|
* Description: 价格策略. <br/> |
||||
|
* Copyright: Copyright (c) 2011 <br/> |
||||
|
* Company: https://gitee.com/liuzp315 <br/>
|
||||
|
* Makedate: 2024-03-28 14:32:24 <br/> |
||||
|
* |
||||
|
* @author liupopo |
||||
|
* @version 1.0 |
||||
|
* @since 1.0 |
||||
|
*/ |
||||
|
@Data |
||||
|
@ApiModel(value = "商品售价列表", description = "商品售价列表") |
||||
|
@TableName("sms_goods") |
||||
|
public class SmsGoods extends BaseEntity { |
||||
|
private static final long serialVersionUID = 1L; |
||||
|
|
||||
|
@ApiModelProperty("商品ID") |
||||
|
private String goodsID; // 商品ID
|
||||
|
@ApiModelProperty("商品类别sid") |
||||
|
private String goodsTypeSid; // 商品类别sid
|
||||
|
@ApiModelProperty("商品类别名称") |
||||
|
private String goodsTypeName; // 商品类别名称
|
||||
|
@ApiModelProperty("商品基础信息Sid") |
||||
|
private String goodsSpuSid; // 商品基础信息Sid
|
||||
|
@ApiModelProperty("商品名称") |
||||
|
private String goodsSpuName; // 商品名称
|
||||
|
@ApiModelProperty("商品SkuSid") |
||||
|
private String goodsSkuSid; // 商品SkuSid
|
||||
|
@ApiModelProperty("商品Sku名称") |
||||
|
private String goodsSkuTitle; // 商品Sku名称
|
||||
|
@ApiModelProperty("商品编码(图号)") |
||||
|
private String goodsSkuCode; // 商品编码(图号)
|
||||
|
@ApiModelProperty("规格型号") |
||||
|
private String goodsSkuOwnSpec; // 规格型号
|
||||
|
@ApiModelProperty("供应商sid") |
||||
|
private String supplierSid; // 供应商sid
|
||||
|
@ApiModelProperty("供应商名称") |
||||
|
private String supplierName; // 供应商名称
|
||||
|
@ApiModelProperty("计量单位") |
||||
|
private String unit; // 计量单位
|
||||
|
@ApiModelProperty("组织全路径") |
||||
|
private BigDecimal price; // 销售单价
|
||||
|
} |
@ -0,0 +1,27 @@ |
|||||
|
package com.yxt.anrui.as.feign.sms; |
||||
|
|
||||
|
import com.yxt.anrui.as.feign.base.basemanufacturer.BaseManufacturerFeignFallback; |
||||
|
import com.yxt.anrui.as.feign.fms.FmsReceivesettle; |
||||
|
import com.yxt.anrui.as.feign.fms.FmsReceivesettleDto; |
||||
|
import com.yxt.common.core.result.ResultBean; |
||||
|
import io.swagger.annotations.ApiOperation; |
||||
|
import org.springframework.cloud.openfeign.FeignClient; |
||||
|
import org.springframework.web.bind.annotation.*; |
||||
|
|
||||
|
/** |
||||
|
* @author Fan |
||||
|
* @description |
||||
|
* @date 2024/4/28 13:40 |
||||
|
*/ |
||||
|
@FeignClient( |
||||
|
contextId = "yxt-sms-SmsGoods", |
||||
|
name = "yxt-sms", |
||||
|
path = "apiadmin/v1/smsGoods", |
||||
|
fallback = BaseManufacturerFeignFallback.class |
||||
|
) |
||||
|
public interface SmsGoodsFeign { |
||||
|
|
||||
|
@ApiOperation("根据goodsID获取一条记录") |
||||
|
@GetMapping("/fetchEntityByGoodsID") |
||||
|
public ResultBean<SmsGoods> fetchEntityByGoodsID(@RequestParam("goodsID") String goodsID); |
||||
|
} |
@ -0,0 +1,26 @@ |
|||||
|
package com.yxt.anrui.as.feign.wms.wmsoldinventory; |
||||
|
|
||||
|
import com.yxt.anrui.as.feign.wms.wmsinventorybill.WmsInventoryBillDto; |
||||
|
import com.yxt.anrui.as.feign.wms.wmsinventorybill.WmsInventoryBillFeignFallback; |
||||
|
import com.yxt.common.core.result.ResultBean; |
||||
|
import io.swagger.annotations.ApiOperation; |
||||
|
import org.springframework.cloud.openfeign.FeignClient; |
||||
|
import org.springframework.web.bind.annotation.PostMapping; |
||||
|
import org.springframework.web.bind.annotation.RequestBody; |
||||
|
|
||||
|
/** |
||||
|
* @description: 旧件 |
||||
|
* @author: dimengzhe |
||||
|
* @date: 2024/3/7 |
||||
|
**/ |
||||
|
@FeignClient( |
||||
|
contextId = "yxt-wms-WmsOldInventory", |
||||
|
name = "yxt-wms", |
||||
|
path = "/apiadmin/WmsOldInventory", |
||||
|
fallback = WmsInventoryBillFeignFallback.class) |
||||
|
public interface WmsOldInventoryFeign { |
||||
|
|
||||
|
@ApiOperation("竣工推送待入库旧件") |
||||
|
@PostMapping("/pushOldStayInvent") |
||||
|
ResultBean pushOldStayInvent(@RequestBody WmsOldInventoryPush push); |
||||
|
} |
@ -0,0 +1,48 @@ |
|||||
|
package com.yxt.anrui.as.feign.wms.wmsoldinventory; |
||||
|
|
||||
|
import lombok.Data; |
||||
|
|
||||
|
import java.util.ArrayList; |
||||
|
import java.util.List; |
||||
|
|
||||
|
/** |
||||
|
* @description: |
||||
|
* @author: dimengzhe |
||||
|
* @date: 2024/4/28 |
||||
|
**/ |
||||
|
@Data |
||||
|
public class WmsOldInventoryPush { |
||||
|
|
||||
|
private String sid; |
||||
|
private String remarks; |
||||
|
private String createBySid; |
||||
|
//商品ID
|
||||
|
private String goodsID; |
||||
|
//商品Sku名称
|
||||
|
private String goodsSkuTitle; |
||||
|
//商品编码(图号)
|
||||
|
private String goodsSkuCode; |
||||
|
//厂家
|
||||
|
private String manufacturerName; |
||||
|
//规格型号
|
||||
|
private String goodsSkuOwnSpec; |
||||
|
//计量单位
|
||||
|
private String unit; |
||||
|
//数量
|
||||
|
private String count; |
||||
|
//已入库数量
|
||||
|
private String inCount; |
||||
|
//维修工单编号
|
||||
|
private String billNo; |
||||
|
//客户名称
|
||||
|
private String customerName; |
||||
|
// 车牌号
|
||||
|
private String vehMark; |
||||
|
//车架号
|
||||
|
private String vinNo; |
||||
|
//旧件回收说明
|
||||
|
private String shortss; |
||||
|
//旧件照片
|
||||
|
private List<String> photoList = new ArrayList<>(); |
||||
|
|
||||
|
} |
@ -0,0 +1,29 @@ |
|||||
|
package com.yxt.sms.apiadmin; |
||||
|
|
||||
|
import com.yxt.common.core.result.ResultBean; |
||||
|
import com.yxt.sms.biz.smsgoods.SmsGoods; |
||||
|
import com.yxt.sms.biz.smsgoods.SmsGoodsService; |
||||
|
import io.swagger.annotations.Api; |
||||
|
import io.swagger.annotations.ApiOperation; |
||||
|
import org.springframework.web.bind.annotation.*; |
||||
|
|
||||
|
/** |
||||
|
* @author Fan |
||||
|
* @description |
||||
|
* @date 2024/6/20 14:06 |
||||
|
*/ |
||||
|
@Api(tags = "商品售价列表") |
||||
|
@RestController |
||||
|
@RequestMapping("/apiadmin/v1/smsGoods") |
||||
|
public class SmsGoodsRest { |
||||
|
private SmsGoodsService smsGoodsService; |
||||
|
|
||||
|
@ApiOperation("根据goodsID获取一条记录") |
||||
|
@GetMapping("/fetchEntityByGoodsID") |
||||
|
public ResultBean<SmsGoods> fetchEntityByGoodsID(@RequestParam("goodsID") String goodsID) { |
||||
|
ResultBean rb = ResultBean.fireFail(); |
||||
|
SmsGoods goods = smsGoodsService.fetchEntityByGoodsID(goodsID); |
||||
|
return rb.success().setData(goods); |
||||
|
} |
||||
|
|
||||
|
} |
@ -0,0 +1,81 @@ |
|||||
|
/********************************************************* |
||||
|
********************************************************* |
||||
|
******************** ******************* |
||||
|
************* ************ |
||||
|
******* _oo0oo_ ******* |
||||
|
*** o8888888o *** |
||||
|
* 88" . "88 * |
||||
|
* (| -_- |) * |
||||
|
* 0\ = /0 * |
||||
|
* ___/`---'\___ * |
||||
|
* .' \\| |// '. *
|
||||
|
* / \\||| : |||// \ *
|
||||
|
* / _||||| -:- |||||- \ * |
||||
|
* | | \\\ - /// | | *
|
||||
|
* | \_| ''\---/'' |_/ | * |
||||
|
* \ .-\__ '-' ___/-. / * |
||||
|
* ___'. .' /--.--\ `. .'___ * |
||||
|
* ."" '< `.___\_<|>_/___.' >' "". * |
||||
|
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
||||
|
* \ \ `_. \_ __\ /__ _/ .-` / / * |
||||
|
* =====`-.____`.___ \_____/___.-`___.-'===== * |
||||
|
* `=---=' * |
||||
|
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
||||
|
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
||||
|
*********************************************************/ |
||||
|
package com.yxt.sms.biz.smsgoods; |
||||
|
|
||||
|
import com.baomidou.mybatisplus.annotation.TableName; |
||||
|
import com.yxt.common.core.domain.BaseEntity; |
||||
|
import io.swagger.annotations.ApiModel; |
||||
|
import io.swagger.annotations.ApiModelProperty; |
||||
|
import lombok.Data; |
||||
|
|
||||
|
import java.math.BigDecimal; |
||||
|
|
||||
|
/** |
||||
|
* Project: yxt-sms(价格策略) <br/> |
||||
|
* File: SmsPricestrategy.java <br/> |
||||
|
* Class: com.yxt.sms.api.smspricestrategy.SmsPricestrategy <br/> |
||||
|
* Description: 价格策略. <br/> |
||||
|
* Copyright: Copyright (c) 2011 <br/> |
||||
|
* Company: https://gitee.com/liuzp315 <br/>
|
||||
|
* Makedate: 2024-03-28 14:32:24 <br/> |
||||
|
* |
||||
|
* @author liupopo |
||||
|
* @version 1.0 |
||||
|
* @since 1.0 |
||||
|
*/ |
||||
|
@Data |
||||
|
@ApiModel(value = "商品售价列表", description = "商品售价列表") |
||||
|
@TableName("sms_goods") |
||||
|
public class SmsGoods extends BaseEntity { |
||||
|
private static final long serialVersionUID = 1L; |
||||
|
|
||||
|
@ApiModelProperty("商品ID") |
||||
|
private String goodsID; // 商品ID
|
||||
|
@ApiModelProperty("商品类别sid") |
||||
|
private String goodsTypeSid; // 商品类别sid
|
||||
|
@ApiModelProperty("商品类别名称") |
||||
|
private String goodsTypeName; // 商品类别名称
|
||||
|
@ApiModelProperty("商品基础信息Sid") |
||||
|
private String goodsSpuSid; // 商品基础信息Sid
|
||||
|
@ApiModelProperty("商品名称") |
||||
|
private String goodsSpuName; // 商品名称
|
||||
|
@ApiModelProperty("商品SkuSid") |
||||
|
private String goodsSkuSid; // 商品SkuSid
|
||||
|
@ApiModelProperty("商品Sku名称") |
||||
|
private String goodsSkuTitle; // 商品Sku名称
|
||||
|
@ApiModelProperty("商品编码(图号)") |
||||
|
private String goodsSkuCode; // 商品编码(图号)
|
||||
|
@ApiModelProperty("规格型号") |
||||
|
private String goodsSkuOwnSpec; // 规格型号
|
||||
|
@ApiModelProperty("供应商sid") |
||||
|
private String supplierSid; // 供应商sid
|
||||
|
@ApiModelProperty("供应商名称") |
||||
|
private String supplierName; // 供应商名称
|
||||
|
@ApiModelProperty("计量单位") |
||||
|
private String unit; // 计量单位
|
||||
|
@ApiModelProperty("组织全路径") |
||||
|
private BigDecimal price; // 销售单价
|
||||
|
} |
@ -0,0 +1,45 @@ |
|||||
|
/********************************************************* |
||||
|
********************************************************* |
||||
|
******************** ******************* |
||||
|
************* ************ |
||||
|
******* _oo0oo_ ******* |
||||
|
*** o8888888o *** |
||||
|
* 88" . "88 * |
||||
|
* (| -_- |) * |
||||
|
* 0\ = /0 * |
||||
|
* ___/`---'\___ * |
||||
|
* .' \\| |// '. *
|
||||
|
* / \\||| : |||// \ *
|
||||
|
* / _||||| -:- |||||- \ * |
||||
|
* | | \\\ - /// | | *
|
||||
|
* | \_| ''\---/'' |_/ | * |
||||
|
* \ .-\__ '-' ___/-. / * |
||||
|
* ___'. .' /--.--\ `. .'___ * |
||||
|
* ."" '< `.___\_<|>_/___.' >' "". * |
||||
|
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
||||
|
* \ \ `_. \_ __\ /__ _/ .-` / / * |
||||
|
* =====`-.____`.___ \_____/___.-`___.-'===== * |
||||
|
* `=---=' * |
||||
|
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
||||
|
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
||||
|
*********************************************************/ |
||||
|
package com.yxt.sms.biz.smsgoods; |
||||
|
|
||||
|
import com.baomidou.mybatisplus.core.conditions.Wrapper; |
||||
|
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||
|
import com.baomidou.mybatisplus.core.toolkit.Constants; |
||||
|
import com.yxt.sms.biz.smspricestrategy.SmsPricestrategyVo; |
||||
|
import org.apache.ibatis.annotations.Mapper; |
||||
|
import org.apache.ibatis.annotations.Param; |
||||
|
import org.apache.ibatis.annotations.Select; |
||||
|
|
||||
|
import java.util.List; |
||||
|
|
||||
|
|
||||
|
@Mapper |
||||
|
public interface SmsGoodsMapper extends BaseMapper<SmsGoods> { |
||||
|
|
||||
|
@Select("select * from sms_goods where goodsID =#{goodsID}") |
||||
|
SmsGoods fetchEntityByGoodsID(@Param("goodsID") String goodsID); |
||||
|
} |
@ -0,0 +1,7 @@ |
|||||
|
<?xml version="1.0" encoding="UTF-8" ?> |
||||
|
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
||||
|
<mapper namespace="com.yxt.sms.biz.smsgoods.SmsGoodsMapper"> |
||||
|
<!-- <where> ${ew.sqlSegment} </where>--> |
||||
|
<!-- ${ew.customSqlSegment} --> |
||||
|
|
||||
|
</mapper> |
@ -0,0 +1,46 @@ |
|||||
|
/********************************************************* |
||||
|
********************************************************* |
||||
|
******************** ******************* |
||||
|
************* ************ |
||||
|
******* _oo0oo_ ******* |
||||
|
*** o8888888o *** |
||||
|
* 88" . "88 * |
||||
|
* (| -_- |) * |
||||
|
* 0\ = /0 * |
||||
|
* ___/`---'\___ * |
||||
|
* .' \\| |// '. *
|
||||
|
* / \\||| : |||// \ *
|
||||
|
* / _||||| -:- |||||- \ * |
||||
|
* | | \\\ - /// | | *
|
||||
|
* | \_| ''\---/'' |_/ | * |
||||
|
* \ .-\__ '-' ___/-. / * |
||||
|
* ___'. .' /--.--\ `. .'___ * |
||||
|
* ."" '< `.___\_<|>_/___.' >' "". * |
||||
|
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
||||
|
* \ \ `_. \_ __\ /__ _/ .-` / / * |
||||
|
* =====`-.____`.___ \_____/___.-`___.-'===== * |
||||
|
* `=---=' * |
||||
|
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
||||
|
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
||||
|
*********************************************************/ |
||||
|
package com.yxt.sms.biz.smsgoods; |
||||
|
|
||||
|
import cn.hutool.core.bean.BeanUtil; |
||||
|
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||
|
import com.yxt.common.base.service.MybatisBaseService; |
||||
|
import com.yxt.common.base.utils.PagerUtil; |
||||
|
import com.yxt.common.core.query.PagerQuery; |
||||
|
import com.yxt.common.core.vo.PagerVo; |
||||
|
import com.yxt.sms.biz.smspricestrategy.*; |
||||
|
import org.springframework.stereotype.Service; |
||||
|
|
||||
|
|
||||
|
@Service |
||||
|
public class SmsGoodsService extends MybatisBaseService<SmsGoodsMapper, SmsGoods> { |
||||
|
|
||||
|
|
||||
|
public SmsGoods fetchEntityByGoodsID(String goodsID) { |
||||
|
return baseMapper.fetchEntityByGoodsID(goodsID); |
||||
|
} |
||||
|
} |
@ -0,0 +1,22 @@ |
|||||
|
package com.yxt.sms.biz.smssalesbill; |
||||
|
|
||||
|
import io.swagger.annotations.ApiModelProperty; |
||||
|
import lombok.Data; |
||||
|
|
||||
|
/** |
||||
|
* @author Fan |
||||
|
* @description |
||||
|
* @date 2024/6/20 11:09 |
||||
|
*/ |
||||
|
@Data |
||||
|
public class SmsAttachitemVo { |
||||
|
@ApiModelProperty("附加项目sid") |
||||
|
private String aitemsid; |
||||
|
@ApiModelProperty("附加项目名称") |
||||
|
private String aitemName; |
||||
|
@ApiModelProperty("销售价") |
||||
|
private String price; |
||||
|
@ApiModelProperty("备注") |
||||
|
private String remarks; |
||||
|
|
||||
|
} |
Loading…
Reference in new issue