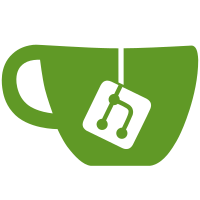
121 changed files with 7449 additions and 130 deletions
@ -0,0 +1,98 @@ |
|||
import request from '@/utils/request' |
|||
|
|||
export default { |
|||
// 查询分页列表
|
|||
listPage: function(params) { |
|||
return request({ |
|||
url: '/riskcenter/v1/LoanDiff/listPage', |
|||
method: 'post', |
|||
data: params, |
|||
headers: { 'Content-Type': 'application/json' } |
|||
}) |
|||
}, |
|||
saveOrUpdate: function(data) { |
|||
return request({ |
|||
url: '/riskcenter/v1/LoanDiff/update', |
|||
method: 'post', |
|||
data: data, |
|||
headers: { 'Content-Type': 'application/json' } |
|||
}) |
|||
}, |
|||
fetchBySid: function(data) { |
|||
return request({ |
|||
url: '/riskcenter/v1/LoanDiff/details', |
|||
method: 'get', |
|||
params: data |
|||
}) |
|||
}, |
|||
// 提交流程
|
|||
submit: function(params) { |
|||
return request({ |
|||
url: '/riskcenter/v1/LoanDiff/submitDiffApply', |
|||
method: 'post', |
|||
data: params, |
|||
headers: { 'Content-Type': 'application/json' } |
|||
}) |
|||
}, |
|||
// 流程审批(同意)
|
|||
complete: function(params) { |
|||
return request({ |
|||
url: '/riskcenter/v1/LoanDiff/complete', |
|||
method: 'post', |
|||
data: params, |
|||
headers: { 'Content-Type': 'application/json' } |
|||
}) |
|||
}, |
|||
// 流程审批(加签)
|
|||
delegate: function(params) { |
|||
return request({ |
|||
url: '/riskcenter/v1/LoanDiff/delegate', |
|||
method: 'post', |
|||
data: params, |
|||
headers: { 'Content-Type': 'application/json' } |
|||
}) |
|||
}, |
|||
// 流程审批(驳回)
|
|||
reject: function(params) { |
|||
return request({ |
|||
url: '/riskcenter/v1/LoanDiff/reject', |
|||
method: 'post', |
|||
data: params, |
|||
headers: { 'Content-Type': 'application/json' } |
|||
}) |
|||
}, |
|||
// 流程审批(终止)
|
|||
breakProcess: function(params) { |
|||
return request({ |
|||
url: '/riskcenter/v1/LoanDiff/breakProcess', |
|||
method: 'post', |
|||
data: params, |
|||
headers: { 'Content-Type': 'application/json' } |
|||
}) |
|||
}, |
|||
// 流程审批(撤回)
|
|||
revokeProcess: function(params) { |
|||
return request({ |
|||
url: '/riskcenter/v1/LoanDiff/revokeProcess', |
|||
method: 'post', |
|||
data: params, |
|||
headers: { 'Content-Type': 'application/json' } |
|||
}) |
|||
}, |
|||
// 审批流程(同意)获取下一环节
|
|||
getNextNodesForSubmit: function(data) { |
|||
return request({ |
|||
url: '/riskcenter/v1/LoanDiff/getNextNodesForSubmit', |
|||
method: 'get', |
|||
params: data |
|||
}) |
|||
}, |
|||
// 审批流程(驳回)获取上一环节
|
|||
getPreviousNodesForReject: function(data) { |
|||
return request({ |
|||
url: '/riskcenter/v1/LoanDiff/getPreviousNodesForReject', |
|||
method: 'get', |
|||
params: data |
|||
}) |
|||
} |
|||
} |
@ -0,0 +1,582 @@ |
|||
<template> |
|||
<div class="app-container"> |
|||
<div class="tab-header webtop"> |
|||
<div>{{ viewTitle }}</div> |
|||
<div> |
|||
<el-button type="primary" size="small" :disabled="submitdisabled" @click="saveOrUpdate()">保存</el-button> |
|||
<el-button type="info" size="small" @click="handleReturn()">关闭</el-button> |
|||
</div> |
|||
</div> |
|||
<div class="listconadd"> |
|||
<el-form ref="form_obj" :model="formobj" :rules="rules" class="formaddcopy02"> |
|||
<el-row style="border-top: 1px solid #e0e3eb"> |
|||
<el-col :span="24"> |
|||
<div class="span-sty"><span class="icon">*</span>生成合同设置</div> |
|||
<el-form-item> |
|||
<el-radio-group class="addinputInfo" :disabled="contractDisabled" style="font-size: 1px" v-model="contractKey"> |
|||
<el-radio label="001">一车一合同</el-radio> |
|||
<el-radio label="002">一贷款人一合同</el-radio> |
|||
</el-radio-group> |
|||
</el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<div class="title">还款协议</div> |
|||
<div class="title">借款人乙方</div> |
|||
<el-row> |
|||
<el-col :span="8"> |
|||
<div class="span-sty"><span><span class="icon">*</span>借款人姓名</span><el-checkbox style="margin-left: 5px" v-model="formobj.actualBuyer">实际购车人</el-checkbox></div> |
|||
<el-form-item><span class="addinputInfo">{{ formobj.lenderNam }}</span></el-form-item> |
|||
</el-col> |
|||
<el-col :span="8"> |
|||
<div class="span-sty"><span class="icon">*</span>身份证号</div> |
|||
<el-form-item prop="idNumber"><el-input class="addinputInfo" style="width: 40%" v-model="formobj.idNumber" clearable placeholder=""/></el-form-item> |
|||
</el-col> |
|||
<el-col :span="8"> |
|||
<div class="span-sty"><span class="icon">*</span>电话</div> |
|||
<el-form-item><span class="addinputInfo">{{ formobj.mobile }}</span></el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<el-row> |
|||
<el-col :span="8"> |
|||
<div class="span-sty">借款人职业</div> |
|||
<el-form-item> |
|||
<el-select class="addinputInfo" v-model="formobj.lenderJobKey" placeholder="请选择" clearable filterable @change="lenderJobChange"> |
|||
<el-option v-for="item in career_list" :key="item.dictKey" :label="item.dictValue" :value="item.dictKey"></el-option> |
|||
</el-select> |
|||
</el-form-item> |
|||
</el-col> |
|||
<el-col :span="8"> |
|||
<div class="span-sty"><span class="icon">*</span>婚姻状况</div> |
|||
<el-form-item prop="marriageTypeKey"> |
|||
<el-select class="addinputInfo" v-model="formobj.marriageTypeKey" placeholder="请选择" clearable filterable @change="marriageChange"> |
|||
<el-option v-for="item in marital_list" :key="item.dictKey" :label="item.dictValue" :value="item.dictKey"></el-option> |
|||
</el-select> |
|||
</el-form-item> |
|||
</el-col> |
|||
<el-col :span="8"> |
|||
<div class="span-sty">有无子女</div> |
|||
<el-form-item prop="childrenKey"> |
|||
<el-radio-group class="addinputInfo" style="font-size: 1px" v-model="formobj.childrenKey" @change="childrenChange"> |
|||
<el-radio label="1">有</el-radio> |
|||
<el-radio label="0">无</el-radio> |
|||
</el-radio-group> |
|||
</el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<el-row> |
|||
<el-col :span="24"> |
|||
<div class="span-sty"><span class="icon">*</span>借款人户籍地址</div> |
|||
<el-form-item prop="koseki"> |
|||
<el-input class="addinputInfo addinputw" v-model="formobj.koseki" clearable placeholder=""/> |
|||
</el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<el-row> |
|||
<el-col :span="24"> |
|||
<div class="span-sty"><span class="icon">*</span>借款人现住址</div> |
|||
<el-form-item prop="address"> |
|||
<el-input class="addinputInfo addinputw" v-model="formobj.address" clearable placeholder=""/> |
|||
</el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<div v-if="formobj.marriageTypeKey == '0004'"> |
|||
<div class="title">配偶(丙方)</div> |
|||
<el-row> |
|||
<el-col :span="8"> |
|||
<div class="span-sty"><span class="icon">*</span>配偶姓名</div> |
|||
<el-form-item prop="spouseName"> |
|||
<el-input class="addinputInfo" style="width: 40%" v-model="formobj.spouseName" clearable placeholder=""/> |
|||
</el-form-item> |
|||
</el-col> |
|||
<el-col :span="8"> |
|||
<div class="span-sty"><span class="icon">*</span>身份证号</div> |
|||
<el-form-item prop="spouseIdCard"> |
|||
<el-input class="addinputInfo" style="width: 40%" v-model="formobj.spouseIdCard" clearable placeholder=""/> |
|||
</el-form-item> |
|||
</el-col> |
|||
<el-col :span="8"> |
|||
<div class="span-sty"><span class="icon">*</span>电话</div> |
|||
<el-form-item prop="spouseMobile"> |
|||
<el-input class="addinputInfo" style="width: 40%" v-model="formobj.spouseMobile" clearable placeholder=""/> |
|||
</el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<el-row> |
|||
<el-col :span="8"> |
|||
<div class="span-sty">配偶职业</div> |
|||
<el-form-item> |
|||
<el-select class="addinputInfo" v-model="formobj.spouseJobKey" placeholder="请选择" clearable filterable @change="spouseChange"> |
|||
<el-option v-for="item in career_list" :key="item.dictKey" :label="item.dictValue" :value="item.dictKey"></el-option> |
|||
</el-select> |
|||
</el-form-item> |
|||
</el-col> |
|||
<el-col :span="8"> |
|||
<div class="span-sty"><span class="icon">*</span>户籍地址</div> |
|||
<el-form-item prop="spouseRegisterAddress"> |
|||
<el-input class="addinputInfo addinputw" v-model="formobj.spouseRegisterAddress" clearable placeholder=""/> |
|||
</el-form-item> |
|||
</el-col> |
|||
<el-col :span="8"> |
|||
<div class="span-sty"><span class="icon">*</span>现住址</div> |
|||
<el-form-item prop="spouseAddress"> |
|||
<el-input class="addinputInfo addinputw" v-model="formobj.spouseAddress" clearable placeholder=""/> |
|||
</el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
</div> |
|||
<div class="title titleOne"> |
|||
<div>其他人员(戊方、己方,若借款人不是实际购车人,则必须录入实际购车人,且作为担保人)</div> |
|||
<el-button type="primary" class="btntopblueline" size="mini" @click="addPerson">添加</el-button> |
|||
</div> |
|||
<el-table :key="tableKey" :data="formobj.otherPersonnel" :index="index" border style="width: 100%"> |
|||
<el-table-column fixed width="60" label="序号" type="index" :index="index + 1" align="center"/> |
|||
<el-table-column label="操作" align="center" width="80"> |
|||
<template slot-scope="scope"> |
|||
<el-button type="danger" size="mini" @click="deletePerson(scope.$index)">删除</el-button> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column label="人员身份" align="center"> |
|||
<template slot-scope="scope"> |
|||
<el-select v-model="scope.row.personnelType" placeholder="请选择" @change="identityChange($event, scope.row)" clearable filterable> |
|||
<el-option v-for="item in identity_list" :key="item.dictKey" :label="item.dictValue" :value="item.dictValue"></el-option> |
|||
</el-select> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column label="姓名" align="center"> |
|||
<template slot-scope="scope"> |
|||
<el-input v-model="scope.row.name" clearable placeholder=""></el-input> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column label="身份证号码" align="center"> |
|||
<template slot-scope="scope"> |
|||
<el-input v-model="scope.row.idCard" clearable placeholder=""></el-input> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column label="电话" align="center"> |
|||
<template slot-scope="scope"> |
|||
<el-input v-model="scope.row.mobile" clearable placeholder=""></el-input> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column label="户籍地址" align="center"> |
|||
<template slot-scope="scope"> |
|||
<el-input v-model="scope.row.residentAddress" clearable placeholder=""></el-input> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column label="现住址" align="center"> |
|||
<template slot-scope="scope"> |
|||
<el-input v-model="scope.row.address" clearable placeholder=""></el-input> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column label="工作单位" align="center"> |
|||
<template slot-scope="scope"> |
|||
<el-input v-model="scope.row.company" clearable placeholder=""></el-input> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column label="职业" align="center"> |
|||
<template slot-scope="scope"> |
|||
<el-input v-model="scope.row.job" clearable placeholder=""></el-input> |
|||
</template> |
|||
</el-table-column> |
|||
</el-table> |
|||
<el-row> |
|||
<el-col :span="12"> |
|||
<div class="span-sty"><span class="icon">*</span>车辆登记在</div> |
|||
<el-form-item prop="vehRegis"> |
|||
<el-radio-group class="addinputInfo" style="font-size: 1px" v-model="formobj.vehRegis"> |
|||
<el-radio label="1">客户名下</el-radio> |
|||
<el-radio label="2">挂靠公司名下</el-radio> |
|||
</el-radio-group> |
|||
</el-form-item> |
|||
</el-col> |
|||
<el-col :span="12"> |
|||
<div v-if="formobj.vehRegis == '2'"> |
|||
<div class="span-sty"><span class="icon">*</span>挂靠公司是否担保</div> |
|||
<el-form-item prop="isSecurity"> |
|||
<el-radio-group class="addinputInfo" style="font-size: 1px" v-model="formobj.isSecurity"> |
|||
<el-radio label="0">是</el-radio> |
|||
<el-radio label="1">否</el-radio> |
|||
</el-radio-group> |
|||
</el-form-item> |
|||
</div> |
|||
</el-col> |
|||
</el-row> |
|||
<div v-if="formobj.vehRegis == '2'"> |
|||
<div class="title">挂靠公司信息(丁方)</div> |
|||
<el-row> |
|||
<el-col :span="24"> |
|||
<div class="span-sty">选择挂靠运输公司</div> |
|||
<el-form-item> |
|||
<el-select v-model="formobj.accCompName" class="addinputInfo" filterable clearable placeholder="" @change="changeBusiness"> |
|||
<el-option v-for="item in business_list" :key="item.taxpayerNo" :label="item.businessName" :value="item.businessName"/> |
|||
</el-select> |
|||
</el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<el-row> |
|||
<el-col :span="12"> |
|||
<div class="span-sty"><span class="icon">*</span>挂靠公司名称</div> |
|||
<el-form-item> |
|||
<el-input class="addinputInfo addinputw" v-model="formobj.accCompName" clearable placeholder=""/> |
|||
</el-form-item> |
|||
</el-col> |
|||
<el-col :span="12"> |
|||
<div class="span-sty"><span class="icon">*</span>统一社会信用代码</div> |
|||
<el-form-item> |
|||
<el-input class="addinputInfo" style="width: 30%" v-model="formobj.accCompRegistNum" clearable placeholder=""/> |
|||
</el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<el-row> |
|||
<el-col :span="12"> |
|||
<div class="span-sty">负责人</div> |
|||
<el-form-item> |
|||
<el-input class="addinputInfo" style="width: 30%" v-model="formobj.accCompContract" clearable placeholder=""/> |
|||
</el-form-item> |
|||
</el-col> |
|||
<el-col :span="12"> |
|||
<div class="span-sty">电话</div> |
|||
<el-form-item> |
|||
<el-input class="addinputInfo" style="width: 30%" v-model="formobj.accComphone" clearable placeholder=""/> |
|||
</el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<el-row> |
|||
<el-col :span="12"> |
|||
<div class="span-sty">注册地址</div> |
|||
<el-form-item> |
|||
<el-input class="addinputInfo addinputw" v-model="formobj.accCompAddress" clearable placeholder=""/> |
|||
</el-form-item> |
|||
</el-col> |
|||
<el-col :span="12"> |
|||
<div class="span-sty">实际经营地址</div> |
|||
<el-form-item> |
|||
<el-input class="addinputInfo addinputw" v-model="formobj.accCompxAddress" clearable placeholder=""/> |
|||
</el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
</div> |
|||
<div class="title">车辆及上装采购合同</div> |
|||
<el-row> |
|||
<el-col :span="24"> |
|||
<div class="span-sty"><span class="icon">*</span>挂车或上装是否公司采购</div> |
|||
<el-form-item> |
|||
<el-radio-group class="addinputInfo" style="font-size: 1px" v-model="formobj.isCompBuy"> |
|||
<el-radio label="0">是</el-radio> |
|||
<el-radio label="1">否</el-radio> |
|||
</el-radio-group> |
|||
</el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
</el-form> |
|||
</div> |
|||
</div> |
|||
</template> |
|||
|
|||
<script> |
|||
import req from '@/api/homevisitpreparation/homevisitpreparation' |
|||
import { selectInvoingByOrgPath, typeValues } from '@/api/Common/dictcommons' |
|||
|
|||
export default { |
|||
name: 'HomeVisitPreparationAdd', |
|||
data() { |
|||
return { |
|||
viewTitle: '', |
|||
submitdisabled: false, |
|||
contractDisabled: false, |
|||
contractKey: '', |
|||
tableKey: 0, |
|||
index: 0, |
|||
career_list: [], |
|||
marital_list: [], |
|||
identity_list: [], |
|||
business_list: [], |
|||
formobj: { |
|||
accCompAddress: '', |
|||
accCompContract: '', |
|||
accCompName: '', |
|||
accCompRegistNum: '', |
|||
accComphone: '', |
|||
accCompxAddress: '', |
|||
actualBuyer: false, |
|||
address: '', |
|||
bankCardImages: [], |
|||
bankNumber: '', |
|||
children: '', |
|||
childrenKey: '', |
|||
emergencyContactMobile: '', |
|||
emergencyContactName: '', |
|||
emergencyContactType: '', |
|||
emergencyContactTypeKey: '', |
|||
idNumber: '', |
|||
invoBank: '', |
|||
isCompBuy: '', |
|||
isSecurity: '', |
|||
koseki: '', |
|||
lenderJob: '', |
|||
lenderJobKey: '', |
|||
lenderNam: '', |
|||
lenderSid: '', |
|||
marriageType: '', |
|||
marriageTypeKey: '', |
|||
mobile: '', |
|||
otherPersonnel: [], |
|||
sid: '', |
|||
spouseAddress: '', |
|||
spouseIdCard: '', |
|||
spouseJob: '', |
|||
spouseJobKey: '', |
|||
spouseMobile: '', |
|||
spouseName: '', |
|||
spouseRegisterAddress: '', |
|||
vehRegis: '' |
|||
}, |
|||
rules: { |
|||
idNumber: [{ required: true, message: '借款人身份证号不能为空', trigger: 'blur' }], |
|||
marriageTypeKey: [{ required: true, message: '婚姻情况不能为空', trigger: 'change' }], |
|||
childrenKey: [{ required: true, message: '有无子女不能为空', trigger: 'change' }], |
|||
koseki: [{ required: true, message: '借款人户籍地址不能为空', trigger: 'blur' }], |
|||
address: [{ required: true, message: '借款人现住址不能为空', trigger: 'blur' }], |
|||
vehRegis: [{ required: true, message: '车辆登记不能为空', trigger: 'change' }], |
|||
isSecurity: [{ required: true, message: '挂靠公司是否担保不能为空', trigger: 'change' }], |
|||
spouseName: [{ required: true, message: '配偶姓名不能为空', trigger: 'blur' }], |
|||
spouseIdCard: [{ required: true, message: '配偶身份证号不能为空', trigger: 'blur' }], |
|||
spouseMobile: [{ required: true, message: '配偶电话不能为空', trigger: 'blur' }], |
|||
spouseRegisterAddress: [{ required: true, message: '配偶户籍地址不能为空', trigger: 'blur' }], |
|||
spouseAddress: [{ required: true, message: '配偶现住址不能为空', trigger: 'blur' }] |
|||
} |
|||
} |
|||
}, |
|||
methods: { |
|||
init() { |
|||
typeValues({ type: 'careerType' }).then((res) => { |
|||
if (res.success) { |
|||
this.career_list = res.data |
|||
} |
|||
}) |
|||
typeValues({ type: 'maritalstatus' }).then((res) => { |
|||
if (res.success) { |
|||
this.marital_list = res.data |
|||
} |
|||
}) |
|||
typeValues({ type: 'identity2' }).then((res) => { |
|||
if (res.success) { |
|||
this.identity_list = res.data |
|||
} |
|||
}) |
|||
selectInvoingByOrgPath({ orgPath: window.sessionStorage.getItem('defaultOrgPath') }).then((res) => { |
|||
if (res.success) { |
|||
this.business_list = res.data |
|||
} |
|||
}) |
|||
}, |
|||
showInfo(sid) { |
|||
this.init() |
|||
this.viewTitle = '贷款合同信息补充' |
|||
this.$nextTick(() => { |
|||
this.$refs['form_obj'].clearValidate() |
|||
}) |
|||
req.fetchDetailsBySid({ sid: sid }).then((resp) => { |
|||
if (resp.success) { |
|||
this.formobj = resp.data |
|||
this.formobj.sid = sid |
|||
} |
|||
}) |
|||
req.initConSetUp(sid).then((res) => { |
|||
if (res.success) { |
|||
if (res.data === '003') { |
|||
this.contractDisabled = false |
|||
} else { |
|||
this.contractDisabled = true |
|||
this.contractKey = res.data |
|||
} |
|||
} |
|||
}) |
|||
}, |
|||
lenderJobChange(value) { |
|||
const choose = this.career_list.filter((item) => item.dictKey === value) |
|||
if (choose.length > 0 && choose !== null) { |
|||
this.formobj.lenderJob = choose[0].dictValue |
|||
} else { |
|||
this.formobj.lenderJob = '' |
|||
} |
|||
}, |
|||
marriageChange(value) { |
|||
const choose = this.marital_list.filter((item) => item.dictKey === value) |
|||
if (choose.length > 0 && choose !== null) { |
|||
this.formobj.marriageType = choose[0].dictValue |
|||
} else { |
|||
this.formobj.marriageType = '' |
|||
} |
|||
}, |
|||
childrenChange(val) { |
|||
if (val === '1') { |
|||
this.formobj.children = '有' |
|||
} else { |
|||
this.formobj.children = '无' |
|||
} |
|||
}, |
|||
spouseChange(value) { |
|||
const choose = this.career_list.filter((item) => item.dictKey === value) |
|||
if (choose.length > 0 && choose !== null) { |
|||
this.formobj.spouseJob = choose[0].dictValue |
|||
} else { |
|||
this.formobj.spouseJob = '' |
|||
} |
|||
}, |
|||
addPerson() { |
|||
this.formobj.otherPersonnel.push({ |
|||
address: '', |
|||
company: '', |
|||
idCard: '', |
|||
job: '', |
|||
mobile: '', |
|||
name: '', |
|||
personnelKey: '', |
|||
personnelType: '', |
|||
residentAddress: '', |
|||
sid: '' |
|||
}) |
|||
}, |
|||
deletePerson(index) { |
|||
this.formobj.otherPersonnel.splice(index, 1) |
|||
}, |
|||
identityChange(value, row) { |
|||
const choose = this.identity_list.filter((item) => item.dictValue === value) |
|||
if (choose.length > 0) { |
|||
row.personnelKey = choose[0].dictKey |
|||
} else { |
|||
row.personnelKey = '' |
|||
} |
|||
}, |
|||
changeBusiness(value) { |
|||
const choose = this.business_list.filter((item) => item.businessName === value) |
|||
if (choose.length > 0) { |
|||
this.formobj.accCompRegistNum = choose[0].taxpayerNo |
|||
this.formobj.accComphone = choose[0].phone |
|||
this.formobj.accCompxAddress = choose[0].address |
|||
} else { |
|||
this.formobj.accCompRegistNum = '' |
|||
this.formobj.accComphone = '' |
|||
this.formobj.accCompxAddress = '' |
|||
} |
|||
}, |
|||
saveOrUpdate() { |
|||
if (this.contractKey === '') { |
|||
this.$message({ showClose: true, type: 'error', message: '生成合同设置不能为空' }) |
|||
return |
|||
} |
|||
if (!this.formobj.actualBuyer) { |
|||
if (this.formobj.otherPersonnel.length === 0) { |
|||
this.$message({ showClose: true, type: 'error', message: '若借款人不是实际购车人,则其他人员表中必须录入实际购车人' }) |
|||
return |
|||
} else { |
|||
let tip = '0' |
|||
for (var i = 0; i < this.formobj.otherPersonnel.length; i++) { |
|||
if (this.formobj.otherPersonnel[i].personnelKey === '3') { |
|||
tip = '1' |
|||
break |
|||
} |
|||
} |
|||
if (tip === '0') { |
|||
this.$message({ showClose: true, type: 'error', message: '若借款人不是实际购车人,则其他人员表中必须录入实际购车人' }) |
|||
return |
|||
} |
|||
} |
|||
} |
|||
this.$refs['form_obj'].validate((valid) => { |
|||
if (valid) { |
|||
this.submitdisabled = true |
|||
req.saveOrUpdate(this.formobj).then((resp) => { |
|||
if (resp.success) { |
|||
const loading = this.$loading({ |
|||
lock: true, |
|||
text: '合同信息正在生成中', |
|||
spinner: 'el-icon-loading', |
|||
background: 'rgba(0, 0, 0, 0.7)' |
|||
}) |
|||
req.saveLoanCon({ sid: this.formobj.sid, conSetUpKey: this.contractKey }).then((res) => { |
|||
if (res.success) { |
|||
loading.close() |
|||
this.$message({ showClose: true, type: 'success', message: '操作成功' }) |
|||
this.handleReturn('true') |
|||
} |
|||
}) |
|||
} else { |
|||
this.submitdisabled = false |
|||
} |
|||
}).catch(() => { |
|||
this.submitdisabled = false |
|||
}) |
|||
} |
|||
}) |
|||
}, |
|||
handleReturn(isreload) { |
|||
if (isreload === 'true') this.$emit('reloadlist') |
|||
this.formobj = { |
|||
accCompAddress: '', |
|||
accCompContract: '', |
|||
accCompName: '', |
|||
accCompRegistNum: '', |
|||
accComphone: '', |
|||
accCompxAddress: '', |
|||
actualBuyer: false, |
|||
address: '', |
|||
bankCardImages: [], |
|||
bankNumber: '', |
|||
children: '', |
|||
childrenKey: '', |
|||
emergencyContactMobile: '', |
|||
emergencyContactName: '', |
|||
emergencyContactType: '', |
|||
emergencyContactTypeKey: '', |
|||
idNumber: '', |
|||
invoBank: '', |
|||
isCompBuy: '', |
|||
isSecurity: '', |
|||
koseki: '', |
|||
lenderJob: '', |
|||
lenderJobKey: '', |
|||
lenderNam: '', |
|||
lenderSid: '', |
|||
marriageType: '', |
|||
marriageTypeKey: '', |
|||
mobile: '', |
|||
otherPersonnel: [], |
|||
sid: '', |
|||
spouseAddress: '', |
|||
spouseIdCard: '', |
|||
spouseJob: '', |
|||
spouseJobKey: '', |
|||
spouseMobile: '', |
|||
spouseName: '', |
|||
spouseRegisterAddress: '', |
|||
vehRegis: '' |
|||
} |
|||
this.submitdisabled = false |
|||
this.contractKey = '' |
|||
this.contractDisabled = false |
|||
this.$refs['form_obj'].resetFields() |
|||
this.$emit('doback') |
|||
} |
|||
} |
|||
} |
|||
</script> |
|||
|
|||
<style scoped> |
|||
.span-sty { |
|||
width: 200px !important; |
|||
} |
|||
.addinputInfo { |
|||
margin-left: 190px !important; |
|||
} |
|||
/deep/ .el-form-item__error { |
|||
margin-left: 190px !important; |
|||
} |
|||
.titleOne { |
|||
padding: 7px; |
|||
display: flex; |
|||
flex-direction: row; |
|||
justify-content: space-between; |
|||
align-items: center; |
|||
} |
|||
</style> |
@ -0,0 +1,298 @@ |
|||
<template> |
|||
<div class="app-container"> |
|||
<!--列表页面--> |
|||
<div v-show="viewState == 1"> |
|||
<button-bar view-title="放款差额确认" ref="btnbar" :btndisabled="btndisabled" @btnhandle="btnHandle"/> |
|||
<!--Start查询列表部分--> |
|||
<div class="main-content"> |
|||
<div class="searchcon"> |
|||
<el-button size="small" class="searchbtn" @click="clicksearchShow">{{ searchxianshitit }}</el-button> |
|||
<div v-show="isSearchShow" class="search"> |
|||
<el-form ref="listQueryform" :inline="true" :model="listQuery" label-width="100px" class="tab-header"> |
|||
<el-form-item label="分公司"> |
|||
<el-input v-model="listQuery.params.useOrgName" placeholder="" clearable/> |
|||
</el-form-item> |
|||
<el-form-item label="申请部门"> |
|||
<el-input v-model="listQuery.params.createDept" placeholder="" clearable/> |
|||
</el-form-item> |
|||
<el-form-item label="申请人"> |
|||
<el-input v-model="listQuery.params.createByName" placeholder="" clearable/> |
|||
</el-form-item> |
|||
<el-form-item label="申请日期"> |
|||
<el-date-picker v-model="listQuery.params.createTimeStart" value-format="yyyy-MM-dd" format="yyyy-MM-dd" type="date" placeholder="选择日期"></el-date-picker> |
|||
<span style="padding: 0 8px">至</span> |
|||
<el-date-picker v-model="listQuery.params.createTimeEnd" value-format="yyyy-MM-dd" format="yyyy-MM-dd" type="date" placeholder="选择日期"></el-date-picker> |
|||
</el-form-item> |
|||
</el-form> |
|||
<div class="btn" style="text-align: center;"> |
|||
<el-button type="primary" icon="el-icon-search" size="small" @click="handleFilter">查询</el-button> |
|||
<el-button type="primary" icon="el-icon-refresh" size="small" @click="handleReset">重置</el-button> |
|||
</div> |
|||
</div> |
|||
</div> |
|||
<!--End查询列表部分--> |
|||
<div class="listtop"> |
|||
<div class="tit">放款差额确认申请列表</div> |
|||
<pageye v-show="list.length > 0" :total="listQuery.total" :page.sync="listQuery.current" :limit.sync="listQuery.size" class="pagination" @pagination="getList"/> |
|||
</div> |
|||
<!--Start 主页面主要部分 --> |
|||
<div class=""> |
|||
<el-table :key="tableKey" v-loading="listLoading" :data="list" :border="true" style="width: 100%;" @selection-change="handleSelectionChange"> |
|||
<el-table-column type="selection" align="center" width="50"/> |
|||
<el-table-column label="序号" type="index" width="80" :index="indexMethod" align="center"/> |
|||
<el-table-column label="操作" width="180px" align="center"> |
|||
<template slot-scope="scope"> |
|||
<el-button type="primary" size="mini" @click="toEdit(scope.row)" :disabled="scope.row.nodeState =='发起申请' ? false : scope.row.nodeState == '待提交' ? false : true">办理</el-button> |
|||
<el-button type="primary" size="mini" @click="toInfo(scope.row)">查看</el-button> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column label="状态" width="180px" header-align="center"> |
|||
<template slot-scope="scope"> |
|||
<span v-if="scope.row.nodeState=='待提交'" type="primary" size="mini">待提交</span> |
|||
<span v-else @click="flowRecord(scope.row)" class="bluezi">{{ scope.row.nodeState }}</span> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column label="分公司" align="center"> |
|||
<template slot-scope="scope"> |
|||
<span>{{ scope.row.useOrgName }}</span> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column label="申请部门" align="center"> |
|||
<template slot-scope="scope"> |
|||
<span>{{ scope.row.createDept }}</span> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column label="申请人" align="center" width="100"> |
|||
<template slot-scope="scope"> |
|||
<span>{{ scope.row.createByName }}</span> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column label="申请日期" align="center" width="100"> |
|||
<template slot-scope="scope"> |
|||
<span>{{ scope.row.createTime }}</span> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column label="申请编号" align="center" width="100"> |
|||
<template slot-scope="scope"> |
|||
<span>{{ scope.row.billNo }}</span> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column label="备注" align="center"> |
|||
<template slot-scope="scope"> |
|||
<span>{{ scope.row.remarks }}</span> |
|||
</template> |
|||
</el-table-column> |
|||
</el-table> |
|||
</div> |
|||
<!--End 主页面主要部分--> |
|||
<div class="pages"> |
|||
<div class="tit"/> |
|||
<!-- 翻页 --> |
|||
<pagination v-show="list.length > 0" :total="listQuery.total" :page.sync="listQuery.current" :limit.sync="listQuery.size" class="pagination" @pagination="getList"/> |
|||
</div> |
|||
<!--End查询列表部分--> |
|||
</div> |
|||
</div> |
|||
<!--款项结转新增及修改 --> |
|||
<loanbalancerecognitionAdd v-show="viewState == 2 || viewState == 3" ref="divAdd" @doback="resetState" @reloadlist="getList"/> |
|||
<!--款项结转申请详情--> |
|||
<loanbalancerecognitionInfo v-show="viewState == 4" ref="divInfo" @doback="resetState" /> |
|||
<!-- 流程审批记录 --> |
|||
<el-dialog title="" :visible.sync="centerDialogVisible" width="78%" height="1%" :before-close="closeIt" center> |
|||
<iframe frameborder="0" id="iframe" style="width:100%;" scrolling="no" :src="this.centerDialogVisible === true ? url :''"></iframe> |
|||
</el-dialog> |
|||
</div> |
|||
</template> |
|||
|
|||
<script> |
|||
import Pagination from '@/components/pagination' |
|||
import pageye from '@/components/pagination/pageye' |
|||
import ButtonBar from '@/components/ButtonBar' |
|||
import req from '@/api/loanbalancerecognition/loanbalancerecognition' |
|||
import { getStorage } from '@/utils/auth' |
|||
import loanbalancerecognitionAdd from './loanbalancerecognitionAdd' |
|||
import loanbalancerecognitionInfo from './loanbalancerecognitionInfo' |
|||
|
|||
export default { |
|||
name: 'LoanBalanceRecognition', |
|||
components: { |
|||
Pagination, |
|||
pageye, |
|||
ButtonBar, |
|||
loanbalancerecognitionAdd, |
|||
loanbalancerecognitionInfo |
|||
}, |
|||
data() { |
|||
return { |
|||
url: '', |
|||
dialogHeight: '80%', |
|||
centerDialogVisible: false, |
|||
btndisabled: false, |
|||
btnList: [ |
|||
{ |
|||
type: 'info', |
|||
size: 'small', |
|||
icon: 'cross', |
|||
btnKey: 'doClose', |
|||
btnLabel: '关闭' |
|||
} |
|||
], |
|||
isSearchShow: false, |
|||
searchxianshitit: '显示查询条件', |
|||
viewState: 1, // 1、列表 2、新增 3、编辑 4、查看 |
|||
tableKey: 0, |
|||
list: [], |
|||
sids: [], // 用于导出的时候保存已选择的SIDs |
|||
FormLoading: false, |
|||
listLoading: false, |
|||
// 翻页 |
|||
listQuery: { |
|||
current: 1, |
|||
size: 10, |
|||
total: 0, |
|||
params: { |
|||
createByName: '', |
|||
createDept: '', |
|||
createTimeEnd: '', |
|||
createTimeStart: '', |
|||
menuUrl: '', |
|||
orgPath: '', |
|||
useOrgName: '', |
|||
userSid: '' |
|||
} |
|||
} |
|||
} |
|||
}, |
|||
created() { |
|||
// 初始化变量 |
|||
this.getList() |
|||
}, |
|||
mounted() { |
|||
// 在外部vue的window上添加postMessage的监听,而且绑定处理函数handleMessage |
|||
window.addEventListener('message', this.handleMessage) |
|||
this.$refs['btnbar'].setButtonList(this.btnList) |
|||
}, |
|||
methods: { |
|||
async handleMessage(event) { |
|||
var code = '' |
|||
if (event.data.params !== null && event.data.params !== undefined) { |
|||
code = event.data.params.code |
|||
} |
|||
if (code === 1) { |
|||
this.centerDialogVisible = false |
|||
} else if (code === 2) { |
|||
this.dialogHeight = event.data.params.data |
|||
this.setIframeHeight(document.getElementById('iframe')) |
|||
} |
|||
}, |
|||
closeIt() { |
|||
this.url = '' |
|||
this.centerDialogVisible = false |
|||
}, |
|||
setIframeHeight(iframe) { |
|||
iframe.height = this.dialogHeight |
|||
}, |
|||
flowRecord(row) { |
|||
this.centerDialogVisible = true |
|||
var params = { |
|||
deployId: row.procDefId, |
|||
procInsId: row.procInstId, |
|||
token: getStorage() |
|||
} |
|||
this.url = '/#/flow/flowRecordForBusiness?data=' + encodeURI((JSON.stringify(params))) |
|||
}, |
|||
// 搜索条件效果 |
|||
clicksearchShow() { |
|||
this.isSearchShow = !this.isSearchShow |
|||
if (this.isSearchShow) { |
|||
this.searchxianshitit = '隐藏查询条件' |
|||
} else { |
|||
this.searchxianshitit = '显示查询条件' |
|||
} |
|||
}, |
|||
btnHandle(btnKey) { |
|||
console.log('XXXXXXXXXXXXXXX ' + btnKey) |
|||
switch (btnKey) { |
|||
case 'doClose': |
|||
this.doClose() |
|||
break |
|||
default: |
|||
break |
|||
} |
|||
}, |
|||
// 信息条数 获取点击时当前的sid |
|||
handleSelectionChange(row) { |
|||
const aa = [] |
|||
row.forEach(element => { |
|||
aa.push(element.sid) |
|||
}) |
|||
this.sids = aa |
|||
}, |
|||
// 表中序号 |
|||
indexMethod(index) { |
|||
var pagestart = (this.listQuery.current - 1) * this.listQuery.size |
|||
var pageindex = index + 1 + pagestart |
|||
return pageindex |
|||
}, |
|||
// 查询列表信息 |
|||
getList() { |
|||
this.listLoading = true |
|||
this.listQuery.params.userSid = window.sessionStorage.getItem('userSid') |
|||
this.listQuery.params.orgPath = window.sessionStorage.getItem('defaultOrgPath') |
|||
this.listQuery.params.menuUrl = this.$route.path |
|||
req.listPage(this.listQuery).then(response => { |
|||
this.listLoading = false |
|||
if (response.success) { |
|||
this.list = response.data.records |
|||
this.listQuery.total = response.data.total |
|||
} else { |
|||
this.list = [] |
|||
this.listQuery.total = 0 |
|||
} |
|||
}) |
|||
}, |
|||
// 查询按钮 |
|||
handleFilter() { |
|||
this.listQuery.current = 1 |
|||
this.getList() |
|||
}, |
|||
// 点击重置 |
|||
handleReset() { |
|||
this.listQuery = { |
|||
current: 1, |
|||
size: 10, |
|||
total: 0, |
|||
params: { |
|||
createByName: '', |
|||
createDept: '', |
|||
createTimeEnd: '', |
|||
createTimeStart: '', |
|||
menuUrl: '', |
|||
orgPath: '', |
|||
useOrgName: '', |
|||
userSid: '' |
|||
} |
|||
} |
|||
this.getList() |
|||
}, |
|||
toEdit(row) { |
|||
this.viewState = 2 |
|||
this.$refs['divAdd'].showEdit(row) |
|||
}, |
|||
toInfo(row) { |
|||
this.viewState = 4 |
|||
this.$refs['divInfo'].showInfo(row) |
|||
}, |
|||
// 修改、编辑、详情返回列表页面 |
|||
resetState() { |
|||
this.viewState = 1 |
|||
}, |
|||
doClose() { |
|||
this.$store.dispatch('tagsView/delView', this.$route) |
|||
this.$router.go(-1) |
|||
} |
|||
} |
|||
} |
|||
</script> |
|||
<style scoped> |
|||
</style> |
@ -0,0 +1,244 @@ |
|||
<template> |
|||
<div class="app-container"> |
|||
<div v-show="viewState == 1"> |
|||
<div class="tab-header webtop"> |
|||
<div>{{ viewTitle }}</div> |
|||
<div> |
|||
<el-button type="primary" size="small" :disabled="submitdisabled" @click="saveOrUpdate()">保存</el-button> |
|||
<el-button type="primary" size="small" :disabled="submitdisabled" @click="submit()">提交</el-button> |
|||
<el-button type="info" size="small" @click="handleReturn()">关闭</el-button> |
|||
</div> |
|||
</div> |
|||
<div class="listconadd"> |
|||
<el-form ref="form_obj" :model="formobj" :rules="rules" class="formaddcopy02"> |
|||
<el-row style="border-top: 1px solid #e0e3eb"> |
|||
<el-col :span="8"> |
|||
<div class="span-sty">申请部门</div> |
|||
<el-form-item><span class="addinputInfo">{{ formobj.createDept }}</span></el-form-item> |
|||
</el-col> |
|||
<el-col :span="8"> |
|||
<div class="span-sty">申请人</div> |
|||
<el-form-item><span class="addinputInfo">{{ formobj.createByName }}</span></el-form-item> |
|||
</el-col> |
|||
<el-col :span="8"> |
|||
<div class="span-sty">申请日期</div> |
|||
<el-form-item><span class="addinputInfo">{{ formobj.createDate }}</span></el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<el-row> |
|||
<el-col :span="24"> |
|||
<div class="span-sty">备注</div> |
|||
<el-form-item><el-input v-model="formobj.remarks" clearable placeholder="" class="addinputInfo addinputw" /></el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<el-row> |
|||
<el-col :span="24"> |
|||
<div class="span-sty">附件</div> |
|||
<el-form-item> |
|||
<upload-img ref="uploadImg" class="addinputInfo" v-model="formobj.filesList" :limit="50" bucket="map" :upload-data="{ type: '0001' }"/> |
|||
</el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<div class="title"> |
|||
<div>金融产品政策列表</div> |
|||
<div>金额单位:元</div> |
|||
</div> |
|||
<el-table :key="tableKey" :data="formobj.loanDiffDetails" :index="index" border style="width: 100%"> |
|||
<el-table-column fixed width="80" label="序号" type="index" :index="index + 1" align="center"/> |
|||
<el-table-column prop="bankName" label="资方" align="center" width="100" /> |
|||
<el-table-column prop="borrowName" label="贷款人" align="center" /> |
|||
<el-table-column prop="vinNo" label="车架号" align="center" width="100" /> |
|||
<el-table-column label="放款金额" align="center"> |
|||
<el-table-column prop="makeLoan" label="应放" align="center" /> |
|||
<el-table-column prop="realityLoan" label="实放" align="center" width="100" /> |
|||
<el-table-column prop="diffLoan" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
<el-table-column label="主产品厂家贴息" align="center"> |
|||
<el-table-column prop="makeDiscount" label="预计" align="center" /> |
|||
<el-table-column label="实际" align="center" width="150"> |
|||
<template slot-scope="scope"> |
|||
<el-input v-model="scope.row.realityDiscount" clearable @keyup.native="UpNumber" placeholder="" @input="realityDiscountInput(scope.row)" /> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column prop="diffDiscount" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
<el-table-column label="其它融厂家贴息" align="center"> |
|||
<el-table-column prop="makeOtherDiscount" label="预计" align="center" /> |
|||
<el-table-column label="实际" align="center" width="150"> |
|||
<template slot-scope="scope"> |
|||
<el-input v-model="scope.row.realityOtherDiscount" clearable @keyup.native="UpNumber" placeholder="" @input="realityOtherDiscountInput(scope.row)" /> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column prop="diffOtherDiscount" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
<el-table-column label="固定贷款保证金" align="center"> |
|||
<el-table-column prop="makeLoanMargin" label="预计" align="center" /> |
|||
<el-table-column label="实际" align="center" width="150"> |
|||
<template slot-scope="scope"> |
|||
<el-input v-model="scope.row.realityLoanMargin" clearable @keyup.native="UpNumber" placeholder="" @input="realityLoanMarginInput(scope.row)" /> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column prop="diffLoanMargin" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
<el-table-column label="意外险" align="center"> |
|||
<el-table-column prop="receivedPremium" label="已收" align="center" /> |
|||
<el-table-column label="实际" align="center" width="150"> |
|||
<template slot-scope="scope"> |
|||
<el-input v-model="scope.row.realityPremium" clearable @keyup.native="UpNumber" placeholder="" @input="realityPremiumInput(scope.row)" /> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column prop="diffPremium" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
</el-table> |
|||
</el-form> |
|||
</div> |
|||
</div> |
|||
</div> |
|||
</template> |
|||
|
|||
<script> |
|||
import req from '@/api/loanbalancerecognition/loanbalancerecognition' |
|||
import uploadImg from '@/components/uploadFile/uploadImg' |
|||
|
|||
export default { |
|||
name: 'LoanBalanceRecognitionAdd', |
|||
components: { |
|||
uploadImg |
|||
}, |
|||
data() { |
|||
return { |
|||
viewTitle: '', |
|||
viewState: 1, |
|||
submitdisabled: false, |
|||
tableKey: 0, |
|||
index: 0, |
|||
formobj: { |
|||
createByName: '', |
|||
createDate: '', |
|||
createDept: '', |
|||
filesList: [], |
|||
loanDiffDetails: [], |
|||
remarks: '', |
|||
sid: '' |
|||
}, |
|||
rules: {} |
|||
} |
|||
}, |
|||
methods: { |
|||
showEdit(row) { |
|||
this.viewTitle = '【编辑】放款差额确认' |
|||
this.$nextTick(() => { |
|||
this.$refs['form_obj'].clearValidate() |
|||
}) |
|||
req.fetchBySid({ sid: row.sid }).then((res) => { |
|||
if (res.success) { |
|||
this.formobj = res.data |
|||
} |
|||
}) |
|||
}, |
|||
UpNumber(e) { |
|||
e.target.value = e.target.value.replace(/[^0-9.]/g, '') // 清除“数字”和“.”以外的字符 |
|||
e.target.value = e.target.value.replace(/^00/, '0.') // 开头不能有两个0 |
|||
e.target.value = e.target.value.replace(/\.{2,}/g, '.') // 只保留第一个. 清除多余的 |
|||
e.target.value = e.target.value.replace('.', '$#$').replace(/\./g, '').replace('$#$', '.') |
|||
e.target.value = e.target.value.replace(/^(\-)*(\d+)\.(\d\d).*$/, '$1$2.$3') // 只能输入两个小数 |
|||
if (e.target.value.indexOf('.') < 0 && e.target.value !== '' && e.target.value !== '-') { |
|||
// 以上已经过滤,此处控制的是如果没有小数点,首位不能为类似于 01、02的金额 |
|||
e.target.value = parseFloat(e.target.value) |
|||
} |
|||
}, |
|||
// 计算主产品厂家贴息--差额 |
|||
realityDiscountInput(row) { |
|||
if (row.makeDiscount !== '' && row.realityDiscount !== '' && row.realityDiscount !== null) { |
|||
row.diffDiscount = parseFloat(row.makeDiscount) - parseFloat(row.realityDiscount) |
|||
} else { |
|||
row.diffDiscount = '' |
|||
} |
|||
}, |
|||
// 计算其它融厂家铁屑--差额 |
|||
realityOtherDiscountInput(row) { |
|||
if (row.makeOtherDiscount !== '' && row.realityOtherDiscount !== '' && row.realityOtherDiscount !== null) { |
|||
row.diffOtherDiscount = parseFloat(row.makeOtherDiscount) - parseFloat(row.realityOtherDiscount) |
|||
} else { |
|||
row.diffOtherDiscount = '' |
|||
} |
|||
}, |
|||
// 计算固定保贷款保证金--差额 |
|||
realityLoanMarginInput(row) { |
|||
if (row.makeLoanMargin !== '' && row.realityLoanMargin !== '' && row.realityLoanMargin !== null) { |
|||
row.diffLoanMargin = parseFloat(row.makeLoanMargin) - parseFloat(row.realityLoanMargin) |
|||
} else { |
|||
row.diffLoanMargin = '' |
|||
} |
|||
}, |
|||
// 计算意外险--差额 |
|||
realityPremiumInput(row) { |
|||
if (row.receivedPremium !== '' && row.realityPremium !== '' && row.realityPremium !== null) { |
|||
row.diffPremium = parseFloat(row.receivedPremium) - parseFloat(row.realityPremium) |
|||
} else { |
|||
row.diffPremium = '' |
|||
} |
|||
}, |
|||
saveOrUpdate() { |
|||
this.$refs['form_obj'].validate((valid) => { |
|||
if (valid) { |
|||
this.submitdisabled = true |
|||
req.saveOrUpdate(this.formobj).then((res) => { |
|||
if (res.success) { |
|||
this.$message({ showClose: true, type: 'success', message: '保存成功' }) |
|||
this.handleReturn('true') |
|||
} else { |
|||
this.submitdisabled = false |
|||
} |
|||
}).catch(() => { |
|||
this.submitdisabled = false |
|||
}) |
|||
} |
|||
}) |
|||
}, |
|||
submit() { |
|||
this.$refs['form_obj'].validate((valid) => { |
|||
if (valid) { |
|||
this.submitdisabled = true |
|||
req.submit(this.formobj).then((res) => { |
|||
if (res.success) { |
|||
this.$message({ showClose: true, type: 'success', message: '操作成功' }) |
|||
this.handleReturn('true') |
|||
} else { |
|||
this.submitdisabled = false |
|||
} |
|||
}).catch(() => { |
|||
this.submitdisabled = false |
|||
}) |
|||
} |
|||
}) |
|||
}, |
|||
handleReturn(isreload) { |
|||
if (isreload === 'true') this.$emit('reloadlist') |
|||
this.formobj = { |
|||
createByName: '', |
|||
createDate: '', |
|||
createDept: '', |
|||
filesList: [], |
|||
loanDiffDetails: [], |
|||
remarks: '', |
|||
sid: '' |
|||
} |
|||
this.submitdisabled = false |
|||
this.$emit('doback') |
|||
} |
|||
} |
|||
} |
|||
</script> |
|||
|
|||
<style scoped> |
|||
.addinputInfo { |
|||
margin-left: 60px !important; |
|||
} |
|||
.title { |
|||
display: flex; |
|||
flex-direction: row; |
|||
justify-content: space-between; |
|||
align-items: center; |
|||
} |
|||
</style> |
@ -0,0 +1,147 @@ |
|||
<template> |
|||
<div class="app-container"> |
|||
<div v-show="viewState == 1"> |
|||
<div class="tab-header webtop"> |
|||
<div>{{ viewTitle }}</div> |
|||
<div> |
|||
<el-button type="info" size="small" @click="handleReturn()">关闭</el-button> |
|||
</div> |
|||
</div> |
|||
<div class="listconadd"> |
|||
<el-form ref="form_obj" :model="formobj" :rules="rules" class="formaddcopy02"> |
|||
<el-row style="border-top: 1px solid #e0e3eb"> |
|||
<el-col :span="8"> |
|||
<div class="span-sty">申请部门</div> |
|||
<el-form-item><span class="addinputInfo">{{ formobj.createDept }}</span></el-form-item> |
|||
</el-col> |
|||
<el-col :span="8"> |
|||
<div class="span-sty">申请人</div> |
|||
<el-form-item><span class="addinputInfo">{{ formobj.createByName }}</span></el-form-item> |
|||
</el-col> |
|||
<el-col :span="8"> |
|||
<div class="span-sty">申请日期</div> |
|||
<el-form-item><span class="addinputInfo">{{ formobj.createDate }}</span></el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<el-row> |
|||
<el-col :span="24"> |
|||
<div class="span-sty">备注</div> |
|||
<el-form-item><span class="addinputInfo">{{ formobj.remarks }}</span></el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<el-row> |
|||
<el-col :span="24"> |
|||
<div class="span-sty">附件</div> |
|||
<el-form-item> |
|||
<el-image class="addinputInfo" style="width: 150px; height: 150px" v-for="(item, index) in formobj.filesList" :key="index" :src="item" :preview-src-list="formobj.filesList"/> |
|||
</el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<div class="title"> |
|||
<div>金融产品政策列表</div> |
|||
<div>金额单位:元</div> |
|||
</div> |
|||
<el-table :key="tableKey" :data="formobj.loanDiffDetails" :index="index" border style="width: 100%"> |
|||
<el-table-column fixed width="80" label="序号" type="index" :index="index + 1" align="center"/> |
|||
<el-table-column prop="bankName" label="资方" align="center" width="100" /> |
|||
<el-table-column prop="borrowName" label="贷款人" align="center" /> |
|||
<el-table-column prop="vinNo" label="车架号" align="center" width="100" /> |
|||
<el-table-column label="放款金额" align="center"> |
|||
<el-table-column prop="makeLoan" label="应放" align="center" /> |
|||
<el-table-column prop="realityLoan" label="实放" align="center" width="100" /> |
|||
<el-table-column prop="diffLoan" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
<el-table-column label="主产品厂家贴息" align="center"> |
|||
<el-table-column prop="makeDiscount" label="预计" align="center" /> |
|||
<el-table-column prop="realityDiscount" label="实际" align="center" width="100" /> |
|||
<el-table-column prop="diffDiscount" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
<el-table-column label="其它融厂家贴息" align="center"> |
|||
<el-table-column prop="makeOtherDiscount" label="预计" align="center" /> |
|||
<el-table-column prop="realityOtherDiscount" label="实际" align="center" width="100" /> |
|||
<el-table-column prop="diffOtherDiscount" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
<el-table-column label="固定贷款保证金" align="center"> |
|||
<el-table-column prop="makeLoanMargin" label="预计" align="center" /> |
|||
<el-table-column prop="realityLoanMargin" label="实际" align="center" width="100" /> |
|||
<el-table-column prop="diffLoanMargin" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
<el-table-column label="意外险" align="center"> |
|||
<el-table-column prop="receivedPremium" label="已收" align="center" /> |
|||
<el-table-column prop="realityPremium" label="实际" align="center" width="100" /> |
|||
<el-table-column prop="diffPremium" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
</el-table> |
|||
</el-form> |
|||
</div> |
|||
</div> |
|||
</div> |
|||
</template> |
|||
|
|||
<script> |
|||
import req from '@/api/loanbalancerecognition/loanbalancerecognition' |
|||
|
|||
export default { |
|||
name: 'LoanBalanceRecognitionInfo', |
|||
data() { |
|||
return { |
|||
viewTitle: '', |
|||
viewState: 1, |
|||
submitdisabled: false, |
|||
tableKey: 0, |
|||
index: 0, |
|||
formobj: { |
|||
createByName: '', |
|||
createDate: '', |
|||
createDept: '', |
|||
filesList: [], |
|||
loanDiffDetails: [], |
|||
remarks: '', |
|||
sid: '' |
|||
}, |
|||
rules: {} |
|||
} |
|||
}, |
|||
methods: { |
|||
showInfo(row) { |
|||
this.viewTitle = '放款差额确认' |
|||
req.fetchBySid({ sid: row.sid }).then((res) => { |
|||
if (res.success) { |
|||
this.formobj = res.data |
|||
if (this.formobj.filesList.length > 0) { |
|||
const aa = [] |
|||
this.formobj.filesList.forEach((e) => { |
|||
aa.push(e.url) |
|||
}) |
|||
this.formobj.filesList = aa |
|||
} |
|||
} |
|||
}) |
|||
}, |
|||
handleReturn() { |
|||
this.formobj = { |
|||
createByName: '', |
|||
createDate: '', |
|||
createDept: '', |
|||
filesList: [], |
|||
loanDiffDetails: [], |
|||
remarks: '', |
|||
sid: '' |
|||
} |
|||
this.$emit('doback') |
|||
} |
|||
} |
|||
} |
|||
</script> |
|||
|
|||
<style scoped> |
|||
.addinputInfo { |
|||
margin-left: 60px !important; |
|||
} |
|||
.title { |
|||
display: flex; |
|||
flex-direction: row; |
|||
justify-content: space-between; |
|||
align-items: center; |
|||
} |
|||
</style> |
@ -0,0 +1,415 @@ |
|||
<template> |
|||
<div class="app-container"> |
|||
<div v-show="viewState == 1"> |
|||
<div class="tab-header webtop"> |
|||
<div>{{ viewTitle }}</div> |
|||
<div> |
|||
<el-button type="primary" size="small" @click="openCountersign('加签')">加 签</el-button> |
|||
<el-button type="primary" size="small" @click="openAgree('同意')">同 意</el-button> |
|||
<el-button type="danger" size="small" @click="openReject('驳回')">驳 回</el-button> |
|||
<el-button type="danger" size="small" @click="openStop('终止')">终 止</el-button> |
|||
</div> |
|||
</div> |
|||
<div class=""> |
|||
<el-form ref="form_obj" :model="formobj" :rules="rules" class="formaddcopy02"> |
|||
<el-row style="border-top: 1px solid #e0e3eb"> |
|||
<el-col :span="8"> |
|||
<div class="span-sty">申请部门</div> |
|||
<el-form-item><span class="addinputInfo">{{ formobj.createDept }}</span></el-form-item> |
|||
</el-col> |
|||
<el-col :span="8"> |
|||
<div class="span-sty">申请人</div> |
|||
<el-form-item><span class="addinputInfo">{{ formobj.createByName }}</span></el-form-item> |
|||
</el-col> |
|||
<el-col :span="8"> |
|||
<div class="span-sty">申请日期</div> |
|||
<el-form-item><span class="addinputInfo">{{ formobj.createDate }}</span></el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<el-row> |
|||
<el-col :span="24"> |
|||
<div class="span-sty">备注</div> |
|||
<el-form-item><span class="addinputInfo">{{ formobj.remarks }}</span></el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<el-row> |
|||
<el-col :span="24"> |
|||
<div class="span-sty">附件</div> |
|||
<el-form-item> |
|||
<el-image class="addinputInfo" style="width: 150px; height: 150px" v-for="(item, index) in formobj.filesList" :key="index" :src="item" :preview-src-list="formobj.filesList"/> |
|||
</el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<div class="title"> |
|||
<div>金融产品政策列表</div> |
|||
<div>金额单位:元</div> |
|||
</div> |
|||
<el-table :key="tableKey" :data="formobj.loanDiffDetails" :index="index" border style="width: 100%"> |
|||
<el-table-column fixed width="80" label="序号" type="index" :index="index + 1" align="center"/> |
|||
<el-table-column prop="bankName" label="资方" align="center" width="100" /> |
|||
<el-table-column prop="borrowName" label="贷款人" align="center" /> |
|||
<el-table-column prop="vinNo" label="车架号" align="center" width="100" /> |
|||
<el-table-column label="放款金额" align="center"> |
|||
<el-table-column prop="makeLoan" label="应放" align="center" /> |
|||
<el-table-column prop="realityLoan" label="实放" align="center" width="100" /> |
|||
<el-table-column prop="diffLoan" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
<el-table-column label="主产品厂家贴息" align="center"> |
|||
<el-table-column prop="makeDiscount" label="预计" align="center" /> |
|||
<el-table-column prop="realityDiscount" label="实际" align="center" width="100" /> |
|||
<el-table-column prop="diffDiscount" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
<el-table-column label="其它融厂家贴息" align="center"> |
|||
<el-table-column prop="makeOtherDiscount" label="预计" align="center" /> |
|||
<el-table-column prop="realityOtherDiscount" label="实际" align="center" width="100" /> |
|||
<el-table-column prop="diffOtherDiscount" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
<el-table-column label="固定贷款保证金" align="center"> |
|||
<el-table-column prop="makeLoanMargin" label="预计" align="center" /> |
|||
<el-table-column prop="realityLoanMargin" label="实际" align="center" width="100" /> |
|||
<el-table-column prop="diffLoanMargin" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
<el-table-column label="意外险" align="center"> |
|||
<el-table-column prop="receivedPremium" label="已收" align="center" /> |
|||
<el-table-column prop="realityPremium" label="实际" align="center" width="100" /> |
|||
<el-table-column prop="diffPremium" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
</el-table> |
|||
</el-form> |
|||
</div> |
|||
</div> |
|||
<!-- 选择待办人 的弹出框--> |
|||
<el-dialog title="填写审批意见" :visible.sync="nodeDialogVisible" width="80%"> |
|||
<el-form class="formadd" > |
|||
<el-row v-show="countersignLink" style="border-top: 1px solid #e0e3eb"> |
|||
<el-col :span="4" class="tleftb"> |
|||
<span><span class="icon">*</span>加签人员:</span> |
|||
</el-col> |
|||
<el-col :span="20"> |
|||
<el-form-item> |
|||
<el-select v-model="countersign.assignee" placeholder="请选择" filterable> |
|||
<el-option v-for="item in options" :key="item.userSid" :label="item.staffName" :value="item.userSid"> |
|||
</el-option> |
|||
</el-select> |
|||
</el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<el-row v-show="currentLink" style="border-top: 1px solid #e0e3eb"> |
|||
<el-col :span="4" class="tleftb"> |
|||
<span>当前环节:</span> |
|||
</el-col> |
|||
<el-col :span="20"> |
|||
<el-form-item><span>{{ current.taskName }}->{{ nextNode.name }}</span></el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<el-row :class="{rowClass:!currentLink}"> |
|||
<el-col :span="4" class="tleftb"> |
|||
<span>意见:</span> |
|||
</el-col> |
|||
<el-col :span="20"> |
|||
<el-form-item><el-input size="small" v-model="dialogList.comment" placeholder="审批意见" class="addinputw" type="textarea" :autosize="{ minRows: 1, maxRows: 10}" clearable ></el-input></el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<div style="text-align:center;margin-top: 20px;"> |
|||
<el-button type="primary" size="mini" @click="reject">确 定</el-button> |
|||
<el-button type="info " size="mini" @click="nodeDialogVisible = false">取 消</el-button> |
|||
</div> |
|||
</el-form> |
|||
</el-dialog> |
|||
</div> |
|||
</template> |
|||
|
|||
<script> |
|||
import req from '@/api/loanbalancerecognition/loanbalancerecognition' |
|||
|
|||
export default { |
|||
name: 'LoanBalanceRecognitionInfo', |
|||
data() { |
|||
return { |
|||
viewTitle: '', |
|||
viewState: 1, |
|||
submitdisabled: false, |
|||
tableKey: 0, |
|||
index: 0, |
|||
formobj: { |
|||
createByName: '', |
|||
createDate: '', |
|||
createDept: '', |
|||
filesList: [], |
|||
loanDiffDetails: [], |
|||
remarks: '', |
|||
sid: '' |
|||
}, |
|||
rules: {}, |
|||
options: [], |
|||
operation: '', // 点击操作按钮 |
|||
dialogList: { |
|||
comment: '' |
|||
}, |
|||
startTask: true, |
|||
current: { |
|||
taskDefKey: '', |
|||
taskName: '' // 当前环节名称 |
|||
}, |
|||
nextNode: {}, // 下一环节 |
|||
nodeDialogVisible: false, |
|||
currentLink: true, |
|||
countersignLink: false, |
|||
// 环节所需参数 |
|||
linkByParameter: { |
|||
businessSid: '', |
|||
comment: '', |
|||
instanceId: '', |
|||
taskId: '', |
|||
orgSidPath: '', |
|||
taskDefKey: '', |
|||
userSid: '' |
|||
}, |
|||
// 加签按钮所需参数 |
|||
countersign: { |
|||
taskId: '', |
|||
assignee: '', |
|||
userSid: '', |
|||
instanceId: '', |
|||
views: '' |
|||
} |
|||
} |
|||
}, |
|||
created() { |
|||
console.log('url:' + window.location.href) |
|||
var one = window.location.href.indexOf('&data') + 6 |
|||
const data = window.location.href.substr(one) // url解码unescape()已从web中移除,尽量不使用 |
|||
const obj = JSON.parse(decodeURIComponent(data)) |
|||
console.log('iframe页面获取的obj:', obj) |
|||
// 点击(同意、终止、驳回、驳回)操作时所需的参数 |
|||
this.linkByParameter.businessSid = obj.businessSid |
|||
this.linkByParameter.instanceId = obj.instanceId |
|||
this.linkByParameter.taskId = obj.taskId |
|||
this.linkByParameter.taskDefKey = obj.taskDefKey |
|||
// this.linkByParameter.orgSidPath = window.sessionStorage.getItem('orgSidPath') |
|||
this.linkByParameter.userSid = window.sessionStorage.getItem('userSid') |
|||
this.current.taskDefKey = obj.taskDefKey |
|||
this.current.taskName = obj.taskName |
|||
// 加签参数 |
|||
this.countersign.taskId = obj.taskId |
|||
this.countersign.userSid = window.sessionStorage.getItem('userSid') |
|||
this.countersign.instanceId = obj.instanceId |
|||
// 加载表单数据 |
|||
this.showInfo(obj.businessSid) |
|||
}, |
|||
mounted() { |
|||
window.parent.postMessage({ |
|||
cmd: 'returnHeight', |
|||
params: { |
|||
// 告诉父级页面,子页面的弹框高度。 |
|||
code: 2, |
|||
data: 500 + 'px' |
|||
} |
|||
}, '*') |
|||
}, |
|||
methods: { |
|||
showInfo(sid) { |
|||
this.viewTitle = '放款差额确认' |
|||
req.fetchBySid({ sid: sid }).then((res) => { |
|||
if (res.success) { |
|||
this.formobj = res.data |
|||
if (this.formobj.filesList.length > 0) { |
|||
const aa = [] |
|||
this.formobj.filesList.forEach((e) => { |
|||
aa.push(e.url) |
|||
}) |
|||
this.formobj.filesList = aa |
|||
} |
|||
} |
|||
}) |
|||
}, |
|||
// 加签 |
|||
openCountersign(val) { |
|||
this.operation = val |
|||
this.currentLink = true |
|||
this.countersignLink = true |
|||
this.dialogList.comment = '' |
|||
req.getNextNodesForSubmit({ taskDefKey: this.current.taskDefKey, businessSid: this.linkByParameter.businessSid }).then((resp) => { |
|||
if (resp.success) { |
|||
var arr = resp.data |
|||
this.nextNode = arr[0] |
|||
this.nodeDialogVisible = true |
|||
} |
|||
this.submitdisabled = false |
|||
}) |
|||
}, |
|||
// 同意 |
|||
openAgree(val) { |
|||
this.operation = val |
|||
this.currentLink = true |
|||
this.countersignLink = false |
|||
this.dialogList.comment = '同意' |
|||
req.getNextNodesForSubmit({ taskDefKey: this.current.taskDefKey, businessSid: this.linkByParameter.businessSid }).then((resp) => { |
|||
if (resp.success) { |
|||
var arr = resp.data |
|||
this.nextNode = arr[0] |
|||
this.nodeDialogVisible = true |
|||
} |
|||
this.submitdisabled = false |
|||
}) |
|||
}, |
|||
// 驳回 |
|||
openReject(val) { |
|||
this.operation = val |
|||
this.currentLink = true |
|||
this.countersignLink = false |
|||
this.dialogList.comment = '' |
|||
req.getPreviousNodesForReject({ taskDefKey: this.current.taskDefKey, businessSid: this.linkByParameter.businessSid }).then((resp) => { |
|||
if (resp.success) { |
|||
var arr = resp.data |
|||
this.nextNode = arr[0] |
|||
this.nodeDialogVisible = true |
|||
} |
|||
this.submitdisabled = false |
|||
}) |
|||
}, |
|||
// 终止 |
|||
openStop(val) { |
|||
this.operation = val |
|||
this.currentLink = false |
|||
this.countersignLink = false |
|||
this.dialogList.comment = '' |
|||
this.nodeDialogVisible = true |
|||
}, |
|||
reject() { |
|||
if (this.operation === '同意') { |
|||
this.handleAgree() |
|||
} else if (this.operation === '驳回') { |
|||
if (this.dialogList.comment === '') { |
|||
this.$message({ showClose: true, type: 'error', message: '请填写审批意见' }) |
|||
} else { |
|||
this.handleReject() |
|||
} |
|||
} else if (this.operation === '终止') { |
|||
if (this.dialogList.comment === '') { |
|||
this.$message({ showClose: true, type: 'error', message: '请填写审批意见' }) |
|||
} else { |
|||
this.handleStop() |
|||
} |
|||
} else if (this.operation === '加签') { |
|||
this.handleCountersign() |
|||
} |
|||
}, |
|||
/** 加签 */ |
|||
handleCountersign() { |
|||
if (this.countersign.assignee === '') { |
|||
this.$message({ showClose: true, type: 'error', message: '请选择加签人员' }) |
|||
return |
|||
} |
|||
if (this.dialogList.comment === '') { |
|||
this.$message({ showClose: true, type: 'error', message: '请填写审批意见' }) |
|||
return |
|||
} |
|||
this.countersign.views = this.dialogList.comment |
|||
req.delegate(this.countersign).then((response) => { |
|||
if (response.success) { |
|||
this.$notify({ |
|||
title: '提示', |
|||
message: '执行成功', |
|||
type: 'success', |
|||
duration: 2000 |
|||
}) |
|||
this.nodeDialogVisible = false |
|||
// 子页面向父级页面传递值 |
|||
window.parent.postMessage({ |
|||
cmd: 'returnHeight', |
|||
params: { |
|||
// 操作成功,告诉父级页面关闭弹框 |
|||
code: 1 |
|||
} |
|||
}, '*') |
|||
} |
|||
}) |
|||
}, |
|||
/** 同意任务 */ |
|||
handleAgree() { |
|||
this.linkByParameter.comment = this.dialogList.comment |
|||
req.complete(this.linkByParameter).then((response) => { |
|||
if (response.success) { |
|||
this.$notify({ |
|||
title: '提示', |
|||
message: '执行成功', |
|||
type: 'success', |
|||
duration: 2000 |
|||
}) |
|||
this.nodeDialogVisible = false |
|||
// 子页面向父级页面传递值 |
|||
window.parent.postMessage({ |
|||
cmd: 'returnHeight', |
|||
params: { |
|||
// 操作成功,告诉父级页面关闭弹框 |
|||
code: 1 |
|||
} |
|||
}, '*') |
|||
} |
|||
}) |
|||
}, |
|||
/** 驳回任务 */ |
|||
handleReject() { |
|||
this.linkByParameter.comment = this.dialogList.comment |
|||
req.reject(this.linkByParameter).then((response) => { |
|||
if (response.success) { |
|||
this.$notify({ |
|||
title: '提示', |
|||
message: '执行成功', |
|||
type: 'success', |
|||
duration: 2000 |
|||
}) |
|||
this.nodeDialogVisible = false |
|||
// 子页面向父级页面传递值 |
|||
window.parent.postMessage({ |
|||
cmd: 'returnHeight', |
|||
params: { |
|||
// 操作成功,告诉父级页面关闭弹框 |
|||
code: 1 |
|||
} |
|||
}, '*') |
|||
} |
|||
}) |
|||
}, |
|||
/** 终止任务 */ |
|||
handleStop() { |
|||
this.linkByParameter.comment = this.dialogList.comment |
|||
req.breakProcess(this.linkByParameter).then((response) => { |
|||
if (response.success) { |
|||
this.$notify({ |
|||
title: '提示', |
|||
message: '执行成功', |
|||
type: 'success', |
|||
duration: 2000 |
|||
}) |
|||
this.nodeDialogVisible = false |
|||
// 子页面向父级页面传递值 |
|||
window.parent.postMessage({ |
|||
cmd: 'returnHeight', |
|||
params: { |
|||
// 操作成功,告诉父级页面关闭弹框 |
|||
code: 1 |
|||
} |
|||
}, '*') |
|||
} |
|||
}) |
|||
} |
|||
} |
|||
} |
|||
</script> |
|||
|
|||
<style scoped> |
|||
.addinputInfo { |
|||
margin-left: 60px !important; |
|||
} |
|||
.title { |
|||
display: flex; |
|||
flex-direction: row; |
|||
justify-content: space-between; |
|||
align-items: center; |
|||
} |
|||
.rowClass{ |
|||
border-top: 1px solid #E0E3EB; |
|||
} |
|||
</style> |
@ -0,0 +1,260 @@ |
|||
<template> |
|||
<div class="app-container"> |
|||
<div v-show="viewState == 1"> |
|||
<div class="tab-header webtop"> |
|||
<div>{{ viewTitle }}</div> |
|||
<div> |
|||
<el-button type="primary" size="small" :disabled="submitdisabled" @click="saveOrUpdate()">保存</el-button> |
|||
<el-button type="primary" size="small" :disabled="submitdisabled" @click="submit()">提交</el-button> |
|||
</div> |
|||
</div> |
|||
<div class=""> |
|||
<el-form ref="form_obj" :model="formobj" :rules="rules" class="formaddcopy02"> |
|||
<el-row style="border-top: 1px solid #e0e3eb"> |
|||
<el-col :span="8"> |
|||
<div class="span-sty">申请部门</div> |
|||
<el-form-item><span class="addinputInfo">{{ formobj.createDept }}</span></el-form-item> |
|||
</el-col> |
|||
<el-col :span="8"> |
|||
<div class="span-sty">申请人</div> |
|||
<el-form-item><span class="addinputInfo">{{ formobj.createByName }}</span></el-form-item> |
|||
</el-col> |
|||
<el-col :span="8"> |
|||
<div class="span-sty">申请日期</div> |
|||
<el-form-item><span class="addinputInfo">{{ formobj.createDate }}</span></el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<el-row> |
|||
<el-col :span="24"> |
|||
<div class="span-sty">备注</div> |
|||
<el-form-item><el-input v-model="formobj.remarks" clearable placeholder="" class="addinputInfo addinputw" /></el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<el-row> |
|||
<el-col :span="24"> |
|||
<div class="span-sty">附件</div> |
|||
<el-form-item> |
|||
<upload-img ref="uploadImg" class="addinputInfo" v-model="formobj.filesList" :limit="50" bucket="map" :upload-data="{ type: '0001' }"/> |
|||
</el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<div class="title"> |
|||
<div>金融产品政策列表</div> |
|||
<div>金额单位:元</div> |
|||
</div> |
|||
<el-table :key="tableKey" :data="formobj.loanDiffDetails" :index="index" border style="width: 100%"> |
|||
<el-table-column fixed width="80" label="序号" type="index" :index="index + 1" align="center"/> |
|||
<el-table-column prop="bankName" label="资方" align="center" width="100" /> |
|||
<el-table-column prop="borrowName" label="贷款人" align="center" /> |
|||
<el-table-column prop="vinNo" label="车架号" align="center" width="100" /> |
|||
<el-table-column label="放款金额" align="center"> |
|||
<el-table-column prop="makeLoan" label="应放" align="center" /> |
|||
<el-table-column prop="realityLoan" label="实放" align="center" width="100" /> |
|||
<el-table-column prop="diffLoan" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
<el-table-column label="主产品厂家贴息" align="center"> |
|||
<el-table-column prop="makeDiscount" label="预计" align="center" /> |
|||
<el-table-column label="实际" align="center" width="150"> |
|||
<template slot-scope="scope"> |
|||
<el-input v-model="scope.row.realityDiscount" clearable @keyup.native="UpNumber" placeholder="" @input="realityDiscountInput(scope.row)" /> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column prop="diffDiscount" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
<el-table-column label="其它融厂家贴息" align="center"> |
|||
<el-table-column prop="makeOtherDiscount" label="预计" align="center" /> |
|||
<el-table-column label="实际" align="center" width="150"> |
|||
<template slot-scope="scope"> |
|||
<el-input v-model="scope.row.realityOtherDiscount" clearable @keyup.native="UpNumber" placeholder="" @input="realityOtherDiscountInput(scope.row)" /> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column prop="diffOtherDiscount" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
<el-table-column label="固定贷款保证金" align="center"> |
|||
<el-table-column prop="makeLoanMargin" label="预计" align="center" /> |
|||
<el-table-column label="实际" align="center" width="150"> |
|||
<template slot-scope="scope"> |
|||
<el-input v-model="scope.row.realityLoanMargin" clearable @keyup.native="UpNumber" placeholder="" @input="realityLoanMarginInput(scope.row)" /> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column prop="diffLoanMargin" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
<el-table-column label="意外险" align="center"> |
|||
<el-table-column prop="receivedPremium" label="已收" align="center" /> |
|||
<el-table-column label="实际" align="center" width="150"> |
|||
<template slot-scope="scope"> |
|||
<el-input v-model="scope.row.realityPremium" clearable @keyup.native="UpNumber" placeholder="" @input="realityPremiumInput(scope.row)" /> |
|||
</template> |
|||
</el-table-column> |
|||
<el-table-column prop="diffPremium" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
</el-table> |
|||
</el-form> |
|||
</div> |
|||
</div> |
|||
</div> |
|||
</template> |
|||
|
|||
<script> |
|||
import req from '@/api/loanbalancerecognition/loanbalancerecognition' |
|||
import uploadImg from '@/components/uploadFile/uploadImg' |
|||
|
|||
export default { |
|||
name: 'LoanBalanceRecognitionAdd', |
|||
components: { |
|||
uploadImg |
|||
}, |
|||
data() { |
|||
return { |
|||
viewTitle: '', |
|||
viewState: 1, |
|||
submitdisabled: false, |
|||
tableKey: 0, |
|||
index: 0, |
|||
formobj: { |
|||
createByName: '', |
|||
createDate: '', |
|||
createDept: '', |
|||
filesList: [], |
|||
loanDiffDetails: [], |
|||
remarks: '', |
|||
sid: '' |
|||
}, |
|||
rules: {} |
|||
} |
|||
}, |
|||
created() { |
|||
console.log('url:' + window.location.href) |
|||
var one = window.location.href.indexOf('&data') + 6 |
|||
const data = window.location.href.substr(one) // url解码unescape()已从web中移除,尽量不使用 |
|||
const obj = JSON.parse(decodeURIComponent(data)) |
|||
this.showInfo(obj.businessSid) |
|||
}, |
|||
mounted() { |
|||
window.parent.postMessage({ |
|||
cmd: 'returnHeight', |
|||
params: { |
|||
// 告诉父级页面,子页面的弹框高度。 |
|||
code: 2, |
|||
data: 500 + 'px' |
|||
} |
|||
}, '*') |
|||
}, |
|||
methods: { |
|||
showInfo(sid) { |
|||
this.viewTitle = '【编辑】放款差额确认' |
|||
this.$nextTick(() => { |
|||
this.$refs['form_obj'].clearValidate() |
|||
}) |
|||
req.fetchBySid({ sid: sid }).then((res) => { |
|||
if (res.success) { |
|||
this.formobj = res.data |
|||
} |
|||
}) |
|||
}, |
|||
UpNumber(e) { |
|||
e.target.value = e.target.value.replace(/[^0-9.]/g, '') // 清除“数字”和“.”以外的字符 |
|||
e.target.value = e.target.value.replace(/^00/, '0.') // 开头不能有两个0 |
|||
e.target.value = e.target.value.replace(/\.{2,}/g, '.') // 只保留第一个. 清除多余的 |
|||
e.target.value = e.target.value.replace('.', '$#$').replace(/\./g, '').replace('$#$', '.') |
|||
e.target.value = e.target.value.replace(/^(\-)*(\d+)\.(\d\d).*$/, '$1$2.$3') // 只能输入两个小数 |
|||
if (e.target.value.indexOf('.') < 0 && e.target.value !== '' && e.target.value !== '-') { |
|||
// 以上已经过滤,此处控制的是如果没有小数点,首位不能为类似于 01、02的金额 |
|||
e.target.value = parseFloat(e.target.value) |
|||
} |
|||
}, |
|||
// 计算主产品厂家贴息--差额 |
|||
realityDiscountInput(row) { |
|||
if (row.makeDiscount !== '' && row.realityDiscount !== '' && row.realityDiscount !== null) { |
|||
row.diffDiscount = parseFloat(row.makeDiscount) - parseFloat(row.realityDiscount) |
|||
} else { |
|||
row.diffDiscount = '' |
|||
} |
|||
}, |
|||
// 计算其它融厂家铁屑--差额 |
|||
realityOtherDiscountInput(row) { |
|||
if (row.makeOtherDiscount !== '' && row.realityOtherDiscount !== '' && row.realityOtherDiscount !== null) { |
|||
row.diffOtherDiscount = parseFloat(row.makeOtherDiscount) - parseFloat(row.realityOtherDiscount) |
|||
} else { |
|||
row.diffOtherDiscount = '' |
|||
} |
|||
}, |
|||
// 计算固定保贷款保证金--差额 |
|||
realityLoanMarginInput(row) { |
|||
if (row.makeLoanMargin !== '' && row.realityLoanMargin !== '' && row.realityLoanMargin !== null) { |
|||
row.diffLoanMargin = parseFloat(row.makeLoanMargin) - parseFloat(row.realityLoanMargin) |
|||
} else { |
|||
row.diffLoanMargin = '' |
|||
} |
|||
}, |
|||
// 计算意外险--差额 |
|||
realityPremiumInput(row) { |
|||
if (row.receivedPremium !== '' && row.realityPremium !== '' && row.realityPremium !== null) { |
|||
row.diffPremium = parseFloat(row.receivedPremium) - parseFloat(row.realityPremium) |
|||
} else { |
|||
row.diffPremium = '' |
|||
} |
|||
}, |
|||
saveOrUpdate() { |
|||
this.$refs['form_obj'].validate((valid) => { |
|||
if (valid) { |
|||
this.submitdisabled = true |
|||
req.saveOrUpdate(this.formobj).then((res) => { |
|||
if (res.success) { |
|||
this.$message({ showClose: true, type: 'success', message: '保存成功' }) |
|||
// 子页面向父级页面传递值(关闭弹框) |
|||
window.parent.postMessage({ |
|||
cmd: 'returnHeight', |
|||
params: { |
|||
// 操作成功,告诉父级页面关闭弹框 |
|||
code: 1 |
|||
} |
|||
}, '*') |
|||
} else { |
|||
this.submitdisabled = false |
|||
} |
|||
}).catch(() => { |
|||
this.submitdisabled = false |
|||
}) |
|||
} |
|||
}) |
|||
}, |
|||
submit() { |
|||
this.$refs['form_obj'].validate((valid) => { |
|||
if (valid) { |
|||
this.submitdisabled = true |
|||
req.submit(this.formobj).then((res) => { |
|||
if (res.success) { |
|||
this.$message({ showClose: true, type: 'success', message: '操作成功' }) |
|||
// 子页面向父级页面传递值(关闭弹框) |
|||
window.parent.postMessage({ |
|||
cmd: 'returnHeight', |
|||
params: { |
|||
// 操作成功,告诉父级页面关闭弹框 |
|||
code: 1 |
|||
} |
|||
}, '*') |
|||
} else { |
|||
this.submitdisabled = false |
|||
} |
|||
}).catch(() => { |
|||
this.submitdisabled = false |
|||
}) |
|||
} |
|||
}) |
|||
} |
|||
} |
|||
} |
|||
</script> |
|||
|
|||
<style scoped> |
|||
.addinputInfo { |
|||
margin-left: 60px !important; |
|||
} |
|||
.title { |
|||
display: flex; |
|||
flex-direction: row; |
|||
justify-content: space-between; |
|||
align-items: center; |
|||
} |
|||
</style> |
@ -0,0 +1,207 @@ |
|||
<template> |
|||
<div class="app-container"> |
|||
<div v-show="viewState == 1"> |
|||
<div class="tab-header webtop"> |
|||
<div>{{ viewTitle }}</div> |
|||
<div> |
|||
<el-button type="danger" size="small" @click="openRevoke()">撤回</el-button> |
|||
</div> |
|||
</div> |
|||
<div class=""> |
|||
<el-form ref="form_obj" :model="formobj" :rules="rules" class="formaddcopy02"> |
|||
<el-row style="border-top: 1px solid #e0e3eb"> |
|||
<el-col :span="8"> |
|||
<div class="span-sty">申请部门</div> |
|||
<el-form-item><span class="addinputInfo">{{ formobj.createDept }}</span></el-form-item> |
|||
</el-col> |
|||
<el-col :span="8"> |
|||
<div class="span-sty">申请人</div> |
|||
<el-form-item><span class="addinputInfo">{{ formobj.createByName }}</span></el-form-item> |
|||
</el-col> |
|||
<el-col :span="8"> |
|||
<div class="span-sty">申请日期</div> |
|||
<el-form-item><span class="addinputInfo">{{ formobj.createDate }}</span></el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<el-row> |
|||
<el-col :span="24"> |
|||
<div class="span-sty">备注</div> |
|||
<el-form-item><span class="addinputInfo">{{ formobj.remarks }}</span></el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<el-row> |
|||
<el-col :span="24"> |
|||
<div class="span-sty">附件</div> |
|||
<el-form-item> |
|||
<el-image class="addinputInfo" style="width: 150px; height: 150px" v-for="(item, index) in formobj.filesList" :key="index" :src="item" :preview-src-list="formobj.filesList"/> |
|||
</el-form-item> |
|||
</el-col> |
|||
</el-row> |
|||
<div class="title"> |
|||
<div>金融产品政策列表</div> |
|||
<div>金额单位:元</div> |
|||
</div> |
|||
<el-table :key="tableKey" :data="formobj.loanDiffDetails" :index="index" border style="width: 100%"> |
|||
<el-table-column fixed width="80" label="序号" type="index" :index="index + 1" align="center"/> |
|||
<el-table-column prop="bankName" label="资方" align="center" width="100" /> |
|||
<el-table-column prop="borrowName" label="贷款人" align="center" /> |
|||
<el-table-column prop="vinNo" label="车架号" align="center" width="100" /> |
|||
<el-table-column label="放款金额" align="center"> |
|||
<el-table-column prop="makeLoan" label="应放" align="center" /> |
|||
<el-table-column prop="realityLoan" label="实放" align="center" width="100" /> |
|||
<el-table-column prop="diffLoan" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
<el-table-column label="主产品厂家贴息" align="center"> |
|||
<el-table-column prop="makeDiscount" label="预计" align="center" /> |
|||
<el-table-column prop="realityDiscount" label="实际" align="center" width="100" /> |
|||
<el-table-column prop="diffDiscount" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
<el-table-column label="其它融厂家贴息" align="center"> |
|||
<el-table-column prop="makeOtherDiscount" label="预计" align="center" /> |
|||
<el-table-column prop="realityOtherDiscount" label="实际" align="center" width="100" /> |
|||
<el-table-column prop="diffOtherDiscount" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
<el-table-column label="固定贷款保证金" align="center"> |
|||
<el-table-column prop="makeLoanMargin" label="预计" align="center" /> |
|||
<el-table-column prop="realityLoanMargin" label="实际" align="center" width="100" /> |
|||
<el-table-column prop="diffLoanMargin" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
<el-table-column label="意外险" align="center"> |
|||
<el-table-column prop="receivedPremium" label="已收" align="center" /> |
|||
<el-table-column prop="realityPremium" label="实际" align="center" width="100" /> |
|||
<el-table-column prop="diffPremium" label="差额" align="center" width="100" /> |
|||
</el-table-column> |
|||
</el-table> |
|||
</el-form> |
|||
</div> |
|||
</div> |
|||
</div> |
|||
</template> |
|||
|
|||
<script> |
|||
import req from '@/api/loanbalancerecognition/loanbalancerecognition' |
|||
|
|||
export default { |
|||
name: 'LoanBalanceRecognitionInfo', |
|||
data() { |
|||
return { |
|||
viewTitle: '', |
|||
viewState: 1, |
|||
submitdisabled: false, |
|||
tableKey: 0, |
|||
index: 0, |
|||
formobj: { |
|||
createByName: '', |
|||
createDate: '', |
|||
createDept: '', |
|||
filesList: [], |
|||
loanDiffDetails: [], |
|||
remarks: '', |
|||
sid: '' |
|||
}, |
|||
// 环节所需参数 |
|||
linkByParameter: { |
|||
businessSid: '', |
|||
comment: '', |
|||
instanceId: '', |
|||
taskId: '', |
|||
orgSidPath: '', |
|||
taskDefKey: '', |
|||
userSid: '' |
|||
}, |
|||
rules: {} |
|||
} |
|||
}, |
|||
created() { |
|||
console.log('url:' + window.location.href) |
|||
var one = window.location.href.indexOf('&data') + 6 |
|||
const data = window.location.href.substr(one) // url解码unescape()已从web中移除,尽量不使用 |
|||
const obj = JSON.parse(decodeURIComponent(data)) |
|||
console.log('iframe页面获取的obj:', obj) |
|||
// 点击(同意、终止、驳回、驳回)操作时所需的参数 |
|||
this.linkByParameter.businessSid = obj.businessSid |
|||
this.linkByParameter.instanceId = obj.instanceId |
|||
this.linkByParameter.taskId = obj.taskId |
|||
this.linkByParameter.taskDefKey = obj.taskDefKey |
|||
this.linkByParameter.userSid = window.sessionStorage.getItem('userSid') |
|||
// 加载表单数据 |
|||
this.showInfo(obj.businessSid) |
|||
}, |
|||
mounted() { |
|||
window.parent.postMessage({ |
|||
cmd: 'returnHeight', |
|||
params: { |
|||
// 告诉父级页面,子页面的弹框高度。 |
|||
code: 2, |
|||
data: 500 + 'px' |
|||
} |
|||
}, '*') |
|||
}, |
|||
methods: { |
|||
showInfo(sid) { |
|||
this.viewTitle = '放款差额确认' |
|||
req.fetchBySid({ sid: sid }).then((res) => { |
|||
if (res.success) { |
|||
this.formobj = res.data |
|||
if (this.formobj.filesList.length > 0) { |
|||
const aa = [] |
|||
this.formobj.filesList.forEach((e) => { |
|||
aa.push(e.url) |
|||
}) |
|||
this.formobj.filesList = aa |
|||
} |
|||
} |
|||
}) |
|||
}, |
|||
/** 确认撤回任务 */ |
|||
openRevoke() { |
|||
this.$confirm('是否确认执行撤回操作', '提示', { |
|||
confirmButtonText: '确定', |
|||
cancelButtonText: '取消', |
|||
type: 'warning' |
|||
}).then(() => { |
|||
this.handleRevoke() |
|||
}).catch(() => { |
|||
this.$message({ |
|||
type: 'info', |
|||
message: '已取消撤回' |
|||
}) |
|||
}) |
|||
}, |
|||
/** 撤回任务 */ |
|||
handleRevoke() { |
|||
req.revokeProcess(this.linkByParameter).then((response) => { |
|||
if (response.success) { |
|||
this.$notify({ |
|||
title: '提示', |
|||
message: '执行成功', |
|||
type: 'success', |
|||
duration: 2000 |
|||
}) |
|||
this.nodeDialogVisible = false |
|||
// 子页面向父级页面传递值 |
|||
window.parent.postMessage({ |
|||
cmd: 'returnHeight', |
|||
params: { |
|||
// 操作成功,告诉父级页面关闭弹框 |
|||
code: 1 |
|||
} |
|||
}, '*') |
|||
} |
|||
}) |
|||
} |
|||
} |
|||
} |
|||
</script> |
|||
|
|||
<style scoped> |
|||
.addinputInfo { |
|||
margin-left: 60px !important; |
|||
} |
|||
.title { |
|||
display: flex; |
|||
flex-direction: row; |
|||
justify-content: space-between; |
|||
align-items: center; |
|||
} |
|||
</style> |
@ -0,0 +1,28 @@ |
|||
package com.yxt.anrui.riskcenter.api.loanbuckleapply; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.ArrayList; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author Administrator |
|||
* @description |
|||
* @date 2023/11/13 14:11 |
|||
*/ |
|||
@Data |
|||
public class LoanBuckleApplyDto implements Dto { |
|||
|
|||
private String sid; |
|||
private String userSid; |
|||
private String dept; |
|||
private String deptSid; |
|||
private String applyName; |
|||
private String applyDate; |
|||
private String remarks; |
|||
private String orgPath; |
|||
private List<String> files = new ArrayList<>(); |
|||
private List<LoanBuckleHistoryRecord> records = new ArrayList<>(); |
|||
} |
@ -0,0 +1,33 @@ |
|||
package com.yxt.anrui.riskcenter.api.loanbuckleapply; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.ArrayList; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author Administrator |
|||
* @description |
|||
* @date 2023/11/13 13:43 |
|||
*/ |
|||
@Data |
|||
public class LoanBuckleApplyInit implements Vo { |
|||
|
|||
private String sid; |
|||
private String userSid; |
|||
private String dept; |
|||
private String deptSid; |
|||
private String applyName; |
|||
private String applyDate; |
|||
private String remarks; |
|||
private String orgPath; |
|||
@ApiModelProperty("任务id") |
|||
private String taskId; |
|||
@ApiModelProperty("实例id") |
|||
private String instanceId; |
|||
private List<String> files = new ArrayList<>(); |
|||
private List<LoanBuckleHistoryRecord> records = new ArrayList<>(); |
|||
|
|||
} |
@ -0,0 +1,31 @@ |
|||
package com.yxt.anrui.riskcenter.api.loanbuckleapply; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author Administrator |
|||
* @description |
|||
* @date 2023/11/13 13:32 |
|||
*/ |
|||
@Data |
|||
public class LoanBuckleApplyQuery implements Query { |
|||
|
|||
@ApiModelProperty("组织全路径") |
|||
private String orgPath; |
|||
@ApiModelProperty("菜单sid") |
|||
private String menuSid; |
|||
@ApiModelProperty("菜单url") |
|||
private String menuUrl; |
|||
@ApiModelProperty("用户sid") |
|||
private String userSid; |
|||
@ApiModelProperty("分公司") |
|||
private String company; // 使用组织名称
|
|||
@ApiModelProperty("申请人") |
|||
private String applyName; // 申请人
|
|||
private String billNo; |
|||
private String applyStartDate; |
|||
private String applyEndDate; |
|||
|
|||
} |
@ -0,0 +1,36 @@ |
|||
package com.yxt.anrui.riskcenter.api.loanbuckleapply; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author Administrator |
|||
* @description |
|||
* @date 2023/11/13 13:32 |
|||
*/ |
|||
@Data |
|||
public class LoanBuckleApplyVo implements Vo { |
|||
|
|||
private String sid; |
|||
@ApiModelProperty("流程状态") |
|||
private String nodeState; // 流程状态
|
|||
@ApiModelProperty("分公司") |
|||
private String company; // 使用组织名称
|
|||
@ApiModelProperty("申请人") |
|||
private String applyName; // 申请人
|
|||
@ApiModelProperty("申请部门") |
|||
private String dept; |
|||
@ApiModelProperty("申请日期") |
|||
private String applyDate; // 申请日期
|
|||
@ApiModelProperty("申请编号") |
|||
private String billNo; |
|||
@ApiModelProperty("流程定义的id") |
|||
private String procDefId; // 流程定义的id
|
|||
@ApiModelProperty("流程实例的sid") |
|||
private String procInstId; // 流程实例的sid
|
|||
@ApiModelProperty("备注") |
|||
private String remarks; // 备注
|
|||
|
|||
|
|||
} |
@ -0,0 +1,44 @@ |
|||
package com.yxt.anrui.riskcenter.api.loanbuckleapply; |
|||
|
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.math.BigDecimal; |
|||
import java.util.Date; |
|||
|
|||
/** |
|||
* @author Administrator |
|||
* @description |
|||
* @date 2023/11/13 13:45 |
|||
*/ |
|||
@Data |
|||
public class LoanBuckleHistoryRecord { |
|||
|
|||
private String recordSid; |
|||
private String loanContractNo; |
|||
private String vinNo; |
|||
@ApiModelProperty("资方名称") |
|||
private String bankName; |
|||
@ApiModelProperty("资方合同") |
|||
private String bankContractNo; |
|||
@ApiModelProperty("客户") |
|||
private String customer; |
|||
@ApiModelProperty("借款人名称") |
|||
private String borrowerName; |
|||
@ApiModelProperty("还款方式") |
|||
private String returnWay; |
|||
@ApiModelProperty("期数") |
|||
private String period; |
|||
@ApiModelProperty("应还日期") |
|||
private String dueDate; |
|||
@ApiModelProperty("应还金额") |
|||
private String dueMoney; |
|||
@ApiModelProperty("实还金额") |
|||
private String actualMoney; |
|||
@ApiModelProperty("本期未还金额") |
|||
private String outstandingMoney; |
|||
@ApiModelProperty("实还日期") |
|||
private String actualDate; |
|||
|
|||
} |
|||
|
@ -0,0 +1,23 @@ |
|||
package com.yxt.anrui.riskcenter.api.loanbuckleapply.app; |
|||
|
|||
import lombok.Data; |
|||
|
|||
import java.util.ArrayList; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author Administrator |
|||
* @description |
|||
* @date 2023/11/13 15:49 |
|||
*/ |
|||
@Data |
|||
public class AppBuckleDetailsVo { |
|||
private String sid; |
|||
private String publishInfo; |
|||
private String time; |
|||
private String remarks; |
|||
private List<String> files = new ArrayList<>(); |
|||
private String taskId; |
|||
private String procInsId; |
|||
private List<AppRecordVo> records = new ArrayList<>(); |
|||
} |
@ -0,0 +1,23 @@ |
|||
package com.yxt.anrui.riskcenter.api.loanbuckleapply.app; |
|||
|
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author Administrator |
|||
* @description |
|||
* @date 2023/11/13 15:49 |
|||
*/ |
|||
@Data |
|||
public class AppRecordVo { |
|||
private String contractId; |
|||
private String instalments; |
|||
private String vin; |
|||
private String type; |
|||
private String zf; |
|||
private String zfNo; |
|||
private String cusName; |
|||
private String loanName; |
|||
private String repaymentDate; |
|||
private String info1; |
|||
private String info2; |
|||
} |
@ -0,0 +1,41 @@ |
|||
package com.yxt.anrui.riskcenter.api.loanbuckleapply.flow; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import javax.validation.constraints.NotBlank; |
|||
import java.util.Map; |
|||
|
|||
/** |
|||
* @Author dimengzhe |
|||
* @Date 2022/6/28 9:01 |
|||
* @Description |
|||
*/ |
|||
@Data |
|||
public class BuckleCompleteDto implements Dto { |
|||
private static final long serialVersionUID = 3240453987322803352L; |
|||
@ApiModelProperty(value = "用户sid") |
|||
@NotBlank(message = "参数错误:userSid") |
|||
private String userSid; |
|||
@ApiModelProperty(value = "用户全路径sid") |
|||
private String orgSidPath; |
|||
@ApiModelProperty(value = "节点id") |
|||
@NotBlank(message = "参数错误:taskDefKey") |
|||
private String taskDefKey; |
|||
@ApiModelProperty(value = "任务id") |
|||
@NotBlank(message = "参数错误:taskId") |
|||
private String taskId; |
|||
@ApiModelProperty(value = "流程id") |
|||
@NotBlank(message = "参数错误:instanceId") |
|||
private String instanceId; |
|||
@ApiModelProperty(value = "意见") |
|||
@NotBlank(message = "参数错误:comment") |
|||
private String comment; |
|||
@ApiModelProperty(value = "业务sid") |
|||
@NotBlank(message = "参数错误:businessSid") |
|||
private String businessSid; |
|||
@ApiModelProperty(value = "分支字段及业务字段") |
|||
private Map<String, Object> formVariables; |
|||
|
|||
} |
@ -0,0 +1,26 @@ |
|||
package com.yxt.anrui.riskcenter.api.loanbuckleapply.flow; |
|||
|
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author Administrator |
|||
* @description |
|||
* @date 2023/9/28 9:28 |
|||
*/ |
|||
@Data |
|||
public class BuckleDelegateQuery { |
|||
@ApiModelProperty |
|||
private String userSid; |
|||
@ApiModelProperty("流程实例id") |
|||
// @JsonProperty("procInsId")
|
|||
private String instanceId; |
|||
@ApiModelProperty("任务Id") |
|||
private String taskId; |
|||
@ApiModelProperty("审批人sid") |
|||
private String assignee; |
|||
@ApiModelProperty("填写意见") |
|||
private String views; |
|||
|
|||
|
|||
} |
@ -0,0 +1,26 @@ |
|||
package com.yxt.anrui.riskcenter.api.loanbuckleapply.flow; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Map; |
|||
|
|||
/** |
|||
* @Author dimengzhe |
|||
* @Date 2022/6/28 10:42 |
|||
* @Description |
|||
*/ |
|||
@Data |
|||
public class BuckleGetNodeQuery implements Query { |
|||
private static final long serialVersionUID = -5674867230708197611L; |
|||
|
|||
@ApiModelProperty(value = "环节定义id") |
|||
private String taskDefKey; |
|||
@ApiModelProperty(value = "业务sid") |
|||
private String businessSid; |
|||
|
|||
@ApiModelProperty(value = "分支字段及业务字段") |
|||
private Map<String, Object> formVariables; |
|||
|
|||
} |
@ -0,0 +1,25 @@ |
|||
package com.yxt.anrui.riskcenter.api.loanbuckleapply.flow; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @Author dimengzhe |
|||
* @Date 2022/6/28 11:09 |
|||
* @Description |
|||
*/ |
|||
@Data |
|||
public class BuckleGetNodeVo implements Vo { |
|||
private static final long serialVersionUID = 8802774014747063504L; |
|||
@ApiModelProperty(value = "节点名称") |
|||
private String name; |
|||
@ApiModelProperty(value = "节点id") |
|||
private String id; |
|||
@ApiModelProperty(value = "审批组") |
|||
private List<String> candidateGroups; |
|||
@ApiModelProperty(value = "是否是最后环节") |
|||
private String endTask; |
|||
} |
@ -0,0 +1,56 @@ |
|||
package com.yxt.anrui.riskcenter.api.loanbuckleapply.flow; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import javax.validation.constraints.NotBlank; |
|||
|
|||
/** |
|||
* @Author dimengzhe |
|||
* @Date 2022/6/28 17:29 |
|||
* @Description 终止、撤回、驳回查询参数 |
|||
*/ |
|||
@Data |
|||
public class BuckleTaskQuery implements Query { |
|||
private static final long serialVersionUID = -4006020771892400451L; |
|||
/** |
|||
* 终止、驳回、撤回 |
|||
*/ |
|||
@ApiModelProperty("任务Id") |
|||
@NotBlank(message = "参数错误:taskId") |
|||
private String taskId; |
|||
/** |
|||
* 终止、驳回、撤回 |
|||
*/ |
|||
@ApiModelProperty("业务sid") |
|||
@NotBlank(message = "参数错误:businessSid") |
|||
private String businessSid; |
|||
/** |
|||
* 终止、驳回 |
|||
*/ |
|||
@ApiModelProperty("任务意见") |
|||
private String comment; |
|||
/** |
|||
* 终止、撤回、驳回 |
|||
*/ |
|||
@ApiModelProperty("用户Sid") |
|||
private String userSid; |
|||
/** |
|||
* 终止 |
|||
*/ |
|||
@ApiModelProperty("流程实例Id") |
|||
private String instanceId; |
|||
/*@ApiModelProperty("用户Id") |
|||
private String userId; |
|||
@ApiModelProperty("节点") |
|||
private String targetKey; |
|||
@ApiModelProperty("流程变量信息") |
|||
private Map<String, Object> values = new HashMap<>(); |
|||
@ApiModelProperty("审批人") |
|||
private String assignee; |
|||
@ApiModelProperty("候选人") |
|||
private List<String> candidateUsers = new ArrayList<>(); |
|||
@ApiModelProperty("审批组") |
|||
private List<String> candidateGroups = new ArrayList<>();*/ |
|||
} |
@ -0,0 +1,21 @@ |
|||
package com.yxt.anrui.riskcenter.api.loanbuckleapply.flow; |
|||
|
|||
import com.yxt.anrui.riskcenter.api.loanbuckleapply.LoanBuckleApplyDto; |
|||
import com.yxt.anrui.riskcenter.api.loancustomerrecord.LoanCustomerRecordDto; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @Author dimengzhe |
|||
* @Date 2022/6/27 13:38 |
|||
* @Description |
|||
*/ |
|||
@Data |
|||
public class SubmitBuckleDto extends LoanBuckleApplyDto { |
|||
private static final long serialVersionUID = 378585162071125756L; |
|||
@ApiModelProperty("流程实例id") |
|||
private String instanceId; |
|||
@ApiModelProperty("任务id") |
|||
private String taskId; |
|||
|
|||
} |
@ -0,0 +1,14 @@ |
|||
package com.yxt.anrui.riskcenter.api.loandiff; |
|||
|
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/15 |
|||
**/ |
|||
@Data |
|||
public class DiffFile { |
|||
|
|||
private String url; |
|||
} |
@ -0,0 +1,41 @@ |
|||
package com.yxt.anrui.riskcenter.api.loandiff; |
|||
|
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/13 |
|||
**/ |
|||
@Data |
|||
public class LoanDiff extends BaseEntity { |
|||
private static final long serialVersionUID = 5447301533409285441L; |
|||
|
|||
private String createByName; |
|||
@ApiModelProperty("申请部门sid") |
|||
private String createDeptSid; |
|||
@ApiModelProperty("申请部门") |
|||
private String createDept; |
|||
@ApiModelProperty("编号") |
|||
private String billNo; |
|||
@ApiModelProperty("附件") |
|||
private String files; |
|||
@ApiModelProperty("组织全路径") |
|||
private String orgSidPath; |
|||
@ApiModelProperty("流程状态") |
|||
private String nodeState; |
|||
@ApiModelProperty("环节key") |
|||
private String nodeId; |
|||
@ApiModelProperty("流程实例id") |
|||
private String procDefId; |
|||
@ApiModelProperty("实例id") |
|||
private String procInstId; |
|||
@ApiModelProperty("任务id") |
|||
private String taskId; |
|||
@ApiModelProperty("分公司sid") |
|||
private String useOrgSid; |
|||
@ApiModelProperty("分公司名称") |
|||
private String useOrgName; |
|||
} |
@ -0,0 +1,33 @@ |
|||
package com.yxt.anrui.riskcenter.api.loandiff; |
|||
|
|||
import com.yxt.anrui.riskcenter.api.loandiffdetails.AppRecords; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.ArrayList; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/14 |
|||
**/ |
|||
@Data |
|||
public class LoanDiffDetailsssApp { |
|||
|
|||
private String sid; |
|||
@ApiModelProperty("申请部门-申请人") |
|||
private String publishInfo; |
|||
@ApiModelProperty("申请时间") |
|||
private String time; |
|||
@ApiModelProperty("备注") |
|||
private String remarks; |
|||
|
|||
private List<String> files = new ArrayList<>(); |
|||
|
|||
private String taskId; |
|||
|
|||
private String procInsId; |
|||
|
|||
private List<AppRecords> records = new ArrayList<>(); |
|||
} |
@ -0,0 +1,26 @@ |
|||
package com.yxt.anrui.riskcenter.api.loandiff; |
|||
|
|||
import com.yxt.anrui.riskcenter.api.loandiffdetails.LoanDiffDetailsDto; |
|||
import com.yxt.common.core.dto.Dto; |
|||
import lombok.Data; |
|||
|
|||
import java.util.ArrayList; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/14 |
|||
**/ |
|||
@Data |
|||
public class LoanDiffDto implements Dto { |
|||
private static final long serialVersionUID = 3046052875256156498L; |
|||
private String sid; |
|||
private List<LoanDiffDetailsDto> loanDiffDetails; |
|||
|
|||
// private List<String> filesList;
|
|||
|
|||
private List<DiffFile> filesList = new ArrayList<>(); |
|||
|
|||
private String remarks; |
|||
} |
@ -0,0 +1,81 @@ |
|||
package com.yxt.anrui.riskcenter.api.loandiff; |
|||
|
|||
import com.yxt.anrui.riskcenter.api.loandiff.flowable.*; |
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
import org.springframework.cloud.openfeign.SpringQueryMap; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import javax.validation.Valid; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/13 |
|||
**/ |
|||
@Api(tags = "放款确认申请") |
|||
@FeignClient( |
|||
contextId = "anrui-riskcenter-LoanDiff", |
|||
name = "anrui-riskcenter", |
|||
path = "v1/LoanDiff", |
|||
fallback = LoanDiffFeignFallback.class) |
|||
public interface LoanDiffFeign { |
|||
@ApiOperation("融资放款确认推送数据") |
|||
@PostMapping("/save") |
|||
ResultBean save(@RequestBody LoanDiffsDto dto); |
|||
|
|||
@ApiOperation("分页列表") |
|||
@PostMapping("/listPage") |
|||
public ResultBean<PagerVo<LoanDiffVo>> listPage(@RequestBody PagerQuery<LoanDiffQuery> pq); |
|||
|
|||
@ApiOperation("保存") |
|||
@PostMapping("/update") |
|||
public ResultBean update(@RequestBody LoanDiffDto dto); |
|||
|
|||
@ApiOperation("详情") |
|||
@GetMapping("/details") |
|||
ResultBean<LoanDiffInitDetails> details(@RequestParam("sid")String sid); |
|||
|
|||
@ApiOperation("提交") |
|||
@PostMapping("/submitDiffApply") |
|||
public ResultBean submitDiffApply(@Valid @RequestBody SubmitDiffDto dto); |
|||
|
|||
@ApiOperation(value = "办理(同意)") |
|||
@PostMapping("/complete") |
|||
public ResultBean complete(@Valid @RequestBody DiffCompleteDto query); |
|||
|
|||
@ApiOperation(value = "获取上一个环节") |
|||
@GetMapping(value = "/getPreviousNodesForReject") |
|||
ResultBean<List<DiffApplyNodeVo>> getPreviousNodesForReject(@Valid @SpringQueryMap DiffApplyNodeQuery query); |
|||
|
|||
@ApiOperation(value = "获取下一个环节") |
|||
@GetMapping(value = "/getNextNodesForSubmit") |
|||
ResultBean<List<DiffApplyNodeVo>> getNextNodesForSubmit(@Valid @SpringQueryMap DiffApplyNodeQuery query); |
|||
|
|||
@ApiOperation(value = "驳回任务") |
|||
@PostMapping(value = "/reject") |
|||
public ResultBean taskReject(@Valid @RequestBody DiffApplyTaskQuery query); |
|||
|
|||
@ApiOperation(value = "撤回流程") |
|||
@PostMapping(value = "/revokeProcess") |
|||
public ResultBean revokeProcess(@Valid @RequestBody DiffApplyTaskQuery query); |
|||
|
|||
@ApiOperation(value = "终止任务") |
|||
@PostMapping(value = "/breakProcess") |
|||
public ResultBean breakProcess(@Valid @RequestBody DiffApplyTaskQuery query); |
|||
|
|||
@ApiOperation(value = "app详情") |
|||
@PostMapping(value = "/appDetails/{sid}") |
|||
ResultBean<LoanDiffDetailsssApp> appDetails(@PathVariable("sid")String sid); |
|||
|
|||
@ApiOperation(value = "加签") |
|||
@PostMapping(value = "/delegate") |
|||
@ResponseBody |
|||
public ResultBean delegate(@RequestBody DelegateQuery query); |
|||
|
|||
} |
@ -0,0 +1,9 @@ |
|||
package com.yxt.anrui.riskcenter.api.loandiff; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/14 |
|||
**/ |
|||
public class LoanDiffFeignFallback { |
|||
} |
@ -0,0 +1,37 @@ |
|||
package com.yxt.anrui.riskcenter.api.loandiff; |
|||
|
|||
import com.yxt.anrui.riskcenter.api.loandiffdetails.LoanDiffDetailssVo; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.ArrayList; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/14 |
|||
**/ |
|||
@Data |
|||
public class LoanDiffInitDetails { |
|||
|
|||
private String sid; |
|||
@ApiModelProperty("申请部门") |
|||
private String createDept; |
|||
@ApiModelProperty("申请人") |
|||
private String createByName; |
|||
@ApiModelProperty("申请日期") |
|||
private String createDate; |
|||
@ApiModelProperty("备注") |
|||
private String remarks; |
|||
@ApiModelProperty("附件") |
|||
// private List<String> files = new ArrayList<>();
|
|||
private List<DiffFile> filesList = new ArrayList<>(); |
|||
|
|||
private List<LoanDiffDetailssVo> loanDiffDetails = new ArrayList<>(); |
|||
|
|||
@ApiModelProperty("流程实例id") |
|||
private String instanceId; |
|||
@ApiModelProperty("任务id") |
|||
private String taskId; |
|||
} |
@ -0,0 +1,33 @@ |
|||
package com.yxt.anrui.riskcenter.api.loandiff; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/14 |
|||
**/ |
|||
@Data |
|||
public class LoanDiffQuery implements Query { |
|||
private static final long serialVersionUID = 7002305390491310057L; |
|||
@ApiModelProperty("分公司") |
|||
private String useOrgName; |
|||
@ApiModelProperty("申请部门") |
|||
private String createDept; |
|||
@ApiModelProperty("申请人") |
|||
private String createByName; |
|||
@ApiModelProperty("申请日期开始时间") |
|||
private String createTimeStart; |
|||
@ApiModelProperty("申请日期结束时间") |
|||
private String createTimeEnd; |
|||
@ApiModelProperty("机构全路径sid") |
|||
private String orgPath; |
|||
@ApiModelProperty("菜单url") |
|||
private String menuUrl; |
|||
@ApiModelProperty("用户sid") |
|||
private String userSid; |
|||
|
|||
|
|||
} |
@ -0,0 +1,35 @@ |
|||
package com.yxt.anrui.riskcenter.api.loandiff; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/14 |
|||
**/ |
|||
@Data |
|||
public class LoanDiffVo implements Vo { |
|||
private static final long serialVersionUID = -7935993141002359582L; |
|||
|
|||
private String sid; |
|||
@ApiModelProperty("流程状态") |
|||
private String nodeState; |
|||
@ApiModelProperty("分公司") |
|||
private String useOrgName; |
|||
@ApiModelProperty("申请部门") |
|||
private String createDept; |
|||
@ApiModelProperty("申请人") |
|||
private String createByName; |
|||
@ApiModelProperty("申请日期") |
|||
private String createTime; |
|||
@ApiModelProperty("申请编号") |
|||
private String billNo; |
|||
@ApiModelProperty("备注") |
|||
private String remarks; |
|||
@ApiModelProperty("流程定义id") |
|||
private String procDefId; |
|||
@ApiModelProperty("流程实例id") |
|||
private String procInstId; |
|||
} |
@ -0,0 +1,34 @@ |
|||
package com.yxt.anrui.riskcenter.api.loandiff; |
|||
|
|||
import com.yxt.anrui.riskcenter.api.loandiffdetails.LoanDiffDetails; |
|||
import com.yxt.anrui.riskcenter.api.loandiffdetails.LoanDiffDetailssDto; |
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/14 |
|||
**/ |
|||
@Data |
|||
public class LoanDiffsDto implements Dto { |
|||
private static final long serialVersionUID = 7160584186871507179L; |
|||
@ApiModelProperty("申请人") |
|||
private String createBySid; |
|||
@ApiModelProperty("分公司sid") |
|||
private String useOrgSid; |
|||
@ApiModelProperty("分公司名称") |
|||
private String useOrgName; |
|||
private String createByName; |
|||
@ApiModelProperty("申请部门") |
|||
private String createDept; |
|||
@ApiModelProperty("申请部门sid") |
|||
private String createDeptSid; |
|||
|
|||
private List<LoanDiffDetailssDto> loanDiffDetailssDtos; |
|||
|
|||
|
|||
} |
@ -0,0 +1,24 @@ |
|||
package com.yxt.anrui.riskcenter.api.loandiff.flowable; |
|||
|
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/15 |
|||
**/ |
|||
@Data |
|||
public class DelegateQuery { |
|||
|
|||
@ApiModelProperty |
|||
private String userSid; |
|||
@ApiModelProperty("流程实例id") |
|||
private String instanceId; |
|||
@ApiModelProperty("任务Id") |
|||
private String taskId; |
|||
@ApiModelProperty("审批人sid") |
|||
private String assignee; |
|||
@ApiModelProperty("填写意见") |
|||
private String views; |
|||
} |
@ -0,0 +1,18 @@ |
|||
package com.yxt.anrui.riskcenter.api.loandiff.flowable; |
|||
|
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/14 |
|||
**/ |
|||
@Data |
|||
public class DiffApplyNodeQuery { |
|||
|
|||
@ApiModelProperty(value = "环节定义id") |
|||
private String taskDefKey; |
|||
@ApiModelProperty(value = "业务sid") |
|||
private String businessSid; |
|||
} |
@ -0,0 +1,24 @@ |
|||
package com.yxt.anrui.riskcenter.api.loandiff.flowable; |
|||
|
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/14 |
|||
**/ |
|||
@Data |
|||
public class DiffApplyNodeVo { |
|||
|
|||
@ApiModelProperty(value = "节点名称") |
|||
private String name; |
|||
@ApiModelProperty(value = "节点id") |
|||
private String id; |
|||
@ApiModelProperty(value = "审批组") |
|||
private List<String> candidateGroups; |
|||
@ApiModelProperty(value = "是否是最后环节") |
|||
private String endTask; |
|||
} |
@ -0,0 +1,43 @@ |
|||
package com.yxt.anrui.riskcenter.api.loandiff.flowable; |
|||
|
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import javax.validation.constraints.NotBlank; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/14 |
|||
**/ |
|||
@Data |
|||
public class DiffApplyTaskQuery { |
|||
|
|||
/** |
|||
* 终止、驳回、撤回 |
|||
*/ |
|||
@ApiModelProperty("任务Id") |
|||
@NotBlank(message = "参数错误:taskId") |
|||
private String taskId; |
|||
/** |
|||
* 终止、驳回、撤回 |
|||
*/ |
|||
@ApiModelProperty("业务sid") |
|||
@NotBlank(message = "参数错误:businessSid") |
|||
private String businessSid; |
|||
/** |
|||
* 终止、驳回 |
|||
*/ |
|||
@ApiModelProperty("任务意见") |
|||
private String comment; |
|||
/** |
|||
* 终止、撤回、驳回 |
|||
*/ |
|||
@ApiModelProperty("用户Sid") |
|||
private String userSid; |
|||
/** |
|||
* 终止 |
|||
*/ |
|||
@ApiModelProperty("流程实例Id") |
|||
private String instanceId; |
|||
} |
@ -0,0 +1,38 @@ |
|||
package com.yxt.anrui.riskcenter.api.loandiff.flowable; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import javax.validation.constraints.NotBlank; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/14 |
|||
**/ |
|||
@Data |
|||
public class DiffCompleteDto implements Dto { |
|||
|
|||
private static final long serialVersionUID = -1137187204046560845L; |
|||
@ApiModelProperty(value = "用户sid") |
|||
@NotBlank(message = "参数错误:userSid") |
|||
private String userSid; |
|||
@ApiModelProperty(value = "用户全路径sid") |
|||
private String orgSidPath; |
|||
@ApiModelProperty(value = "节点id") |
|||
@NotBlank(message = "参数错误:taskDefKey") |
|||
private String taskDefKey; |
|||
@ApiModelProperty(value = "任务id") |
|||
@NotBlank(message = "参数错误:taskId") |
|||
private String taskId; |
|||
@ApiModelProperty(value = "流程id") |
|||
@NotBlank(message = "参数错误:instanceId") |
|||
private String instanceId; |
|||
@ApiModelProperty(value = "意见") |
|||
private String comment; |
|||
@ApiModelProperty(value = "业务sid") |
|||
@NotBlank(message = "参数错误:businessSid") |
|||
private String businessSid; |
|||
|
|||
} |
@ -0,0 +1,19 @@ |
|||
package com.yxt.anrui.riskcenter.api.loandiff.flowable; |
|||
|
|||
import com.yxt.anrui.riskcenter.api.loandiff.LoanDiffDto; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/14 |
|||
**/ |
|||
@Data |
|||
public class SubmitDiffDto extends LoanDiffDto { |
|||
|
|||
@ApiModelProperty("流程实例id") |
|||
private String instanceId; |
|||
@ApiModelProperty("任务id") |
|||
private String taskId; |
|||
} |
@ -0,0 +1,50 @@ |
|||
package com.yxt.anrui.riskcenter.api.loandiffdetails; |
|||
|
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/14 |
|||
**/ |
|||
@Data |
|||
public class AppRecords { |
|||
@ApiModelProperty("车架号") |
|||
private String vin; |
|||
@ApiModelProperty("资方") |
|||
private String zf; |
|||
@ApiModelProperty("贷款人") |
|||
private String loanName; |
|||
@ApiModelProperty("应放") |
|||
private String fk1; |
|||
@ApiModelProperty("实放") |
|||
private String fk2; |
|||
|
|||
@ApiModelProperty("主产品贴息:预计") |
|||
private String mainTx1; |
|||
@ApiModelProperty("主产品信息:实际") |
|||
private String mainTx2; |
|||
@ApiModelProperty("保证金预计") |
|||
private String dkbzj1; |
|||
@ApiModelProperty("保证金实际") |
|||
private String dkbzj2; |
|||
|
|||
private String otherTx1; |
|||
|
|||
private String otherTx2; |
|||
private String diffOtherTx; |
|||
|
|||
@ApiModelProperty("意外险:预计") |
|||
private String ywx1; |
|||
@ApiModelProperty("意外险:实扣") |
|||
private String ywx2; |
|||
@ApiModelProperty("放款金额:差额") |
|||
private String diffFk; |
|||
@ApiModelProperty("意外险:差额") |
|||
private String diffYwx; |
|||
@ApiModelProperty("主产品厂家贴息:差额") |
|||
private String diffMainTx; |
|||
@ApiModelProperty("贷款保证金:差额") |
|||
private String diffDkbzj; |
|||
} |
@ -0,0 +1,65 @@ |
|||
package com.yxt.anrui.riskcenter.api.loandiffdetails; |
|||
|
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.math.BigDecimal; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/13 |
|||
**/ |
|||
@Data |
|||
public class LoanDiffDetails extends BaseEntity { |
|||
private static final long serialVersionUID = 2569023626756288954L; |
|||
@ApiModelProperty("主申请sid") |
|||
private String mainSid; |
|||
@ApiModelProperty("资方sid") |
|||
private String bankSid; |
|||
@ApiModelProperty("资方") |
|||
private String bankName; |
|||
@ApiModelProperty("销售订单车辆sid") |
|||
private String busVinSid; |
|||
@ApiModelProperty("车架号") |
|||
private String vinNo; |
|||
@ApiModelProperty("车辆sid") |
|||
private String vinSid; |
|||
@ApiModelProperty("贷款人sid") |
|||
private String borrowSid; |
|||
@ApiModelProperty("贷款人") |
|||
private String borrowName; |
|||
@ApiModelProperty("放款金额:应放") |
|||
private BigDecimal makeLoan; |
|||
@ApiModelProperty("放款金额:实放") |
|||
private BigDecimal realityLoan; |
|||
private BigDecimal diffLoan; |
|||
@ApiModelProperty("主产品厂家贴息:预计") |
|||
private BigDecimal makeDiscount; |
|||
|
|||
@ApiModelProperty("主产品厂家贴息:实际") |
|||
private BigDecimal realityDiscount; |
|||
private BigDecimal diffDiscount; |
|||
|
|||
@ApiModelProperty("其他融厂家贴息:预计") |
|||
private BigDecimal makeOtherDiscount; |
|||
|
|||
@ApiModelProperty("其他融厂家贴息:实际") |
|||
private BigDecimal realityOtherDiscount; |
|||
private BigDecimal diffOtherDiscount; |
|||
|
|||
@ApiModelProperty("固定贷款保证金:预计") |
|||
private BigDecimal makeLoanMargin; |
|||
|
|||
@ApiModelProperty("固定贷款保证金:实际") |
|||
private BigDecimal realityLoanMargin; |
|||
private BigDecimal diffLoanMargin; |
|||
|
|||
@ApiModelProperty("意外险:已收") |
|||
private BigDecimal receivedPremium; |
|||
|
|||
@ApiModelProperty("意外险:实扣") |
|||
private BigDecimal realityPremium; |
|||
private BigDecimal diffPremium; |
|||
} |
@ -0,0 +1,30 @@ |
|||
package com.yxt.anrui.riskcenter.api.loandiffdetails; |
|||
|
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/14 |
|||
**/ |
|||
@Data |
|||
public class LoanDiffDetailsDto { |
|||
private String sid; |
|||
@ApiModelProperty("主产品厂家贴息:实际") |
|||
private String realityDiscount; |
|||
@ApiModelProperty("主产品厂家贴息:差额") |
|||
private String diffDiscount; |
|||
@ApiModelProperty("其他融厂家贴息:实际") |
|||
private String realityOtherDiscount; |
|||
@ApiModelProperty("其他融厂家贴息:差额") |
|||
private String diffOtherDiscount; |
|||
@ApiModelProperty("固定贷款保证金:实际") |
|||
private String realityLoanMargin; |
|||
@ApiModelProperty("固定贷款保证金:差额") |
|||
private String diffLoanMargin; |
|||
@ApiModelProperty("实扣意外险") |
|||
private String realityPremium; |
|||
@ApiModelProperty("意外险:差额") |
|||
private String diffPremium; |
|||
} |
@ -0,0 +1,49 @@ |
|||
package com.yxt.anrui.riskcenter.api.loandiffdetails; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.math.BigDecimal; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/14 |
|||
**/ |
|||
@Data |
|||
public class LoanDiffDetailssDto implements Dto { |
|||
private static final long serialVersionUID = -2530442143507437830L; |
|||
|
|||
@ApiModelProperty("销售订单车辆sid") |
|||
private String busVinSid; |
|||
@ApiModelProperty("车辆sid") |
|||
private String vinSid; |
|||
@ApiModelProperty("车架号") |
|||
private String vinNo; |
|||
@ApiModelProperty("资方sid") |
|||
private String bankSid; |
|||
@ApiModelProperty("资方名称") |
|||
private String bankName; |
|||
@ApiModelProperty("贷款人sid") |
|||
private String borrowSid; |
|||
@ApiModelProperty("贷款人") |
|||
private String borrowName; |
|||
|
|||
@ApiModelProperty("放款金额:应放") |
|||
private BigDecimal makeLoan; |
|||
@ApiModelProperty("放款金额:实放") |
|||
private BigDecimal realityLoan; |
|||
@ApiModelProperty("放款金额:差额") |
|||
private BigDecimal diffLoan; |
|||
@ApiModelProperty("主产品厂家贴息:预计") |
|||
private BigDecimal makeDiscount; |
|||
@ApiModelProperty("其他融厂家贴息:预计") |
|||
private BigDecimal makeOtherDiscount; |
|||
@ApiModelProperty("固定贷款保证金:预计") |
|||
private BigDecimal makeLoanMargin; |
|||
@ApiModelProperty("意外险:已收") |
|||
private BigDecimal receivedPremium; |
|||
|
|||
|
|||
} |
@ -0,0 +1,65 @@ |
|||
package com.yxt.anrui.riskcenter.api.loandiffdetails; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.math.BigDecimal; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/14 |
|||
**/ |
|||
@Data |
|||
public class LoanDiffDetailssVo implements Vo { |
|||
private static final long serialVersionUID = 8471596886220398279L; |
|||
|
|||
private String sid; |
|||
@ApiModelProperty("资方") |
|||
private String bankName; |
|||
|
|||
@ApiModelProperty("车架号") |
|||
private String vinNo; |
|||
|
|||
|
|||
@ApiModelProperty("贷款人") |
|||
private String borrowName; |
|||
@ApiModelProperty("放款金额:应放") |
|||
private BigDecimal makeLoan; |
|||
@ApiModelProperty("放款金额:实放") |
|||
private BigDecimal realityLoan; |
|||
@ApiModelProperty("放款金额:差额") |
|||
private BigDecimal diffLoan; |
|||
@ApiModelProperty("主产品厂家贴息:预计") |
|||
private BigDecimal makeDiscount; |
|||
|
|||
@ApiModelProperty("主产品厂家贴息:实际") |
|||
private BigDecimal realityDiscount; |
|||
@ApiModelProperty("主产品厂家贴息:差额") |
|||
private BigDecimal diffDiscount; |
|||
|
|||
@ApiModelProperty("其他融厂家贴息:预计") |
|||
private BigDecimal makeOtherDiscount; |
|||
|
|||
@ApiModelProperty("其他融厂家贴息:实际") |
|||
private BigDecimal realityOtherDiscount; |
|||
@ApiModelProperty("其他融厂家贴息:差额") |
|||
private BigDecimal diffOtherDiscount; |
|||
|
|||
@ApiModelProperty("固定贷款保证金:预计") |
|||
private BigDecimal makeLoanMargin; |
|||
|
|||
@ApiModelProperty("固定贷款保证金:实际") |
|||
private BigDecimal realityLoanMargin; |
|||
@ApiModelProperty("固定贷款保证金:差额") |
|||
private BigDecimal diffLoanMargin; |
|||
|
|||
@ApiModelProperty("意外险:已收") |
|||
private BigDecimal receivedPremium; |
|||
|
|||
@ApiModelProperty("意外险:实扣") |
|||
private BigDecimal realityPremium; |
|||
@ApiModelProperty("意外险:差额") |
|||
private BigDecimal diffPremium; |
|||
} |
@ -0,0 +1,38 @@ |
|||
package com.yxt.anrui.riskcenter.api.loanrepaymenthistory; |
|||
|
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author Administrator |
|||
* @description |
|||
* @date 2023/11/13 14:02 |
|||
*/ |
|||
@Data |
|||
public class LoanRepaymentHistoryRecordVo { |
|||
private String recordSid; |
|||
private String loanContractNo; |
|||
private String vinNo; |
|||
@ApiModelProperty("资方名称") |
|||
private String bankName; |
|||
@ApiModelProperty("资方合同") |
|||
private String bankContractNo; |
|||
@ApiModelProperty("客户") |
|||
private String customer; |
|||
@ApiModelProperty("借款人名称") |
|||
private String borrowerName; |
|||
@ApiModelProperty("还款方式") |
|||
private String returnWay; |
|||
@ApiModelProperty("期数") |
|||
private String period; |
|||
@ApiModelProperty("应还日期") |
|||
private String dueDate; |
|||
@ApiModelProperty("应还金额") |
|||
private String dueMoney; |
|||
@ApiModelProperty("实还金额") |
|||
private String actualMoney; |
|||
@ApiModelProperty("本期未还金额") |
|||
private String outstandingMoney; |
|||
@ApiModelProperty("实还日期") |
|||
private String actualDate; |
|||
} |
@ -1,5 +1,42 @@ |
|||
<?xml version="1.0" encoding="UTF-8" ?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.anrui.riskcenter.biz.loanbuckleapply.LoanBuckleApplyMapper"> |
|||
<update id="updateFlowFiled"> |
|||
UPDATE loan_buckle_apply |
|||
SET nodeState=#{nodeState} |
|||
, nodeSid=#{taskDefKey} |
|||
<if test="procDefId != null and procDefId != ''"> |
|||
, procDefId=#{procDefId} |
|||
</if> |
|||
<if test="procInsId != null and procInsId != ''"> |
|||
, procInstId=#{procInsId} |
|||
</if> |
|||
<if test="taskId != null and taskId != ''"> |
|||
, taskId=#{taskId} |
|||
</if> |
|||
WHERE sid = #{sid} |
|||
</update> |
|||
|
|||
<select id="listPage" resultType="com.yxt.anrui.riskcenter.api.loanbuckleapply.LoanBuckleApplyVo"> |
|||
SELECT |
|||
sid, |
|||
nodeState, |
|||
useOrgName as company, |
|||
dept, |
|||
applyName, |
|||
date_format(applyDate, '%Y-%m-%d') as applyDate, |
|||
remarks, |
|||
billNo, |
|||
procDefId, |
|||
procInstId |
|||
FROM loan_buckle_apply |
|||
<where> |
|||
${ew.sqlSegment} |
|||
</where> |
|||
</select> |
|||
<select id="selectNum" resultType="java.lang.Integer"> |
|||
select IFNULL(CAST(REPLACE(MAX(billNo), #{bill}, '') AS SIGNED), 0) as code |
|||
from loan_buckle_apply |
|||
where billNo LIKE concat(#{bill}, '%') |
|||
</select> |
|||
</mapper> |
@ -0,0 +1,30 @@ |
|||
package com.yxt.anrui.riskcenter.biz.loandiff; |
|||
|
|||
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import com.baomidou.mybatisplus.core.toolkit.Constants; |
|||
import com.yxt.anrui.buscenter.api.bussalesorder.BusSalesOrder; |
|||
import com.yxt.anrui.buscenter.api.bussalesordervehicle.BusSalesOrderVehicle; |
|||
import com.yxt.anrui.riskcenter.api.loandiff.LoanDiff; |
|||
import com.yxt.anrui.riskcenter.api.loandiff.LoanDiffVo; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
import org.apache.ibatis.annotations.Param; |
|||
|
|||
import java.util.Map; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/13 |
|||
**/ |
|||
@Mapper |
|||
public interface LoanDiffMapper extends BaseMapper<LoanDiff> { |
|||
int updateFlowFiled(Map<String, Object> map); |
|||
|
|||
IPage<LoanDiffVo> listPageVo(IPage<LoanDiff> page, @Param(Constants.WRAPPER)QueryWrapper<LoanDiff> qw); |
|||
|
|||
BusSalesOrderVehicle selectByBusVinSid(String busVinSid); |
|||
|
|||
BusSalesOrder selectByOrderSid(String salesOrderSid); |
|||
} |
@ -0,0 +1,47 @@ |
|||
<?xml version="1.0" encoding="UTF-8" ?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.anrui.riskcenter.biz.loandiff.LoanDiffMapper"> |
|||
<update id="updateFlowFiled"> |
|||
UPDATE loan_diff |
|||
SET nodeState=#{nodeState} |
|||
<if test="taskDefKey != null and taskDefKey != ''"> |
|||
, nodeId=#{taskDefKey} |
|||
</if> |
|||
<if test="procDefId != null and procDefId != ''"> |
|||
, procDefId=#{procDefId} |
|||
</if> |
|||
<if test="procInsId != null and procInsId != ''"> |
|||
, procInstId=#{procInsId} |
|||
</if> |
|||
<if test="taskId != null and taskId != ''"> |
|||
, taskId=#{taskId} |
|||
</if> |
|||
WHERE sid = #{sid} |
|||
</update> |
|||
|
|||
<select id="listPageVo" resultType="com.yxt.anrui.riskcenter.api.loandiff.LoanDiffVo"> |
|||
select ld.sid, |
|||
ld.createByName, |
|||
ld.useOrgName, |
|||
ld.createDept, |
|||
DATE_FORMAT(ld.createTime, '%Y-%m-%d') as createTime, |
|||
ld.billNo, |
|||
ld.remarks, |
|||
if(length(ld.nodeState) > 0, nodeState, '待提交') as nodeState, |
|||
procDefId, |
|||
procInstId |
|||
from loan_diff ld |
|||
<where> |
|||
${ew.sqlSegment} |
|||
</where> |
|||
order by ld.id desc |
|||
</select> |
|||
|
|||
<select id="selectByBusVinSid" resultType="com.yxt.anrui.buscenter.api.bussalesordervehicle.BusSalesOrderVehicle"> |
|||
select * from anrui_buscenter.bus_sales_order_vehicle where sid = #{busVinSid} |
|||
</select> |
|||
|
|||
<select id="selectByOrderSid" resultType="com.yxt.anrui.buscenter.api.bussalesorder.BusSalesOrder"> |
|||
select * from anrui_buscenter.bus_sales_order where sid = #{salesOrderSid} |
|||
</select> |
|||
</mapper> |
@ -0,0 +1,98 @@ |
|||
package com.yxt.anrui.riskcenter.biz.loandiff; |
|||
|
|||
import cn.hutool.core.bean.BeanUtil; |
|||
import com.yxt.anrui.flowable.sqloperationsymbol.BusinessVariables; |
|||
import com.yxt.anrui.riskcenter.api.loandiff.*; |
|||
import com.yxt.anrui.riskcenter.api.loandiff.flowable.*; |
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.web.bind.annotation.RequestMapping; |
|||
import org.springframework.web.bind.annotation.RestController; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/13 |
|||
**/ |
|||
@RestController |
|||
@RequestMapping("v1/LoanDiff") |
|||
public class LoanDiffRest implements LoanDiffFeign { |
|||
|
|||
@Autowired |
|||
private LoanDiffService loanDiffService; |
|||
|
|||
|
|||
@Override |
|||
public ResultBean save(LoanDiffsDto dto) { |
|||
return loanDiffService.saveDiff(dto); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<PagerVo<LoanDiffVo>> listPage(PagerQuery<LoanDiffQuery> pq) { |
|||
ResultBean<PagerVo<LoanDiffVo>> rb = ResultBean.fireFail(); |
|||
PagerVo<LoanDiffVo> pv = loanDiffService.listPageVo(pq); |
|||
return rb.success().setData(pv); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean update(LoanDiffDto dto) { |
|||
return loanDiffService.updateDiff(dto); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<LoanDiffInitDetails> details(String sid) { |
|||
return loanDiffService.details(sid); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean submitDiffApply(SubmitDiffDto dto) { |
|||
return loanDiffService.submitDiffApply(dto); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean complete(DiffCompleteDto query) { |
|||
BusinessVariables bv = new BusinessVariables(); |
|||
BeanUtil.copyProperties(query, bv); |
|||
bv.setModelId(""); |
|||
return loanDiffService.complete(bv); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<DiffApplyNodeVo>> getPreviousNodesForReject(DiffApplyNodeQuery query) { |
|||
return loanDiffService.getPreviousNodesForReject(query); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<DiffApplyNodeVo>> getNextNodesForSubmit(DiffApplyNodeQuery query) { |
|||
return loanDiffService.getNextNodesForSubmit(query); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean taskReject(DiffApplyTaskQuery query) { |
|||
return loanDiffService.taskReject(query); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean revokeProcess(DiffApplyTaskQuery query) { |
|||
return loanDiffService.revokeProcess(query); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean breakProcess(DiffApplyTaskQuery query) { |
|||
return loanDiffService.breakProcess(query); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<LoanDiffDetailsssApp> appDetails(String sid) { |
|||
return loanDiffService.appDetails(sid); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean delegate(DelegateQuery query) { |
|||
return loanDiffService.delegate(query); |
|||
} |
|||
} |
@ -0,0 +1,676 @@ |
|||
package com.yxt.anrui.riskcenter.biz.loandiff; |
|||
|
|||
import cn.hutool.core.bean.BeanUtil; |
|||
import cn.hutool.core.date.DateUtil; |
|||
import com.alibaba.fastjson.JSON; |
|||
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import com.google.common.util.concurrent.ThreadFactoryBuilder; |
|||
import com.yxt.anrui.base.api.basepurchasesystem.BasePurchaseSystemDetailsVo; |
|||
import com.yxt.anrui.base.api.basepurchasesystem.BasePurchaseSystemFeign; |
|||
import com.yxt.anrui.base.api.busvehicleapply.BusVehicleApplyVo; |
|||
import com.yxt.anrui.buscenter.api.bussalesorder.BusSalesOrder; |
|||
import com.yxt.anrui.buscenter.api.bussalesordervehicle.BusSalesOrderVehicle; |
|||
import com.yxt.anrui.fin.api.kingdee.FinKingDeeFeign; |
|||
import com.yxt.anrui.fin.api.kingdee.bdcustomer.BdCustomer; |
|||
import com.yxt.anrui.fin.api.kingdee.capitalcreditresult.CapitalCreditResult; |
|||
import com.yxt.anrui.fin.api.kingdee.capitalcreditresult.CwSystemYT; |
|||
import com.yxt.anrui.flowable.api.flow.FlowableFeign; |
|||
import com.yxt.anrui.flowable.api.flow.UpdateFlowFieldVo; |
|||
import com.yxt.anrui.flowable.api.flow2.FlowDelegateQuery; |
|||
import com.yxt.anrui.flowable.api.flow2.FlowFeign; |
|||
import com.yxt.anrui.flowable.api.flowtask.FlowTaskFeign; |
|||
import com.yxt.anrui.flowable.api.flowtask.FlowTaskVo; |
|||
import com.yxt.anrui.flowable.api.flowtask.LatestTaskVo; |
|||
import com.yxt.anrui.flowable.api.utils.ProcDefEnum; |
|||
import com.yxt.anrui.flowable.sqloperationsymbol.BusinessVariables; |
|||
import com.yxt.anrui.portal.api.sysorganization.SysOrganizationFeign; |
|||
import com.yxt.anrui.portal.api.sysorganization.SysOrganizationVo; |
|||
import com.yxt.anrui.portal.api.sysuser.PrivilegeQuery; |
|||
import com.yxt.anrui.portal.api.sysuser.SysUserFeign; |
|||
import com.yxt.anrui.riskcenter.api.loandiff.*; |
|||
import com.yxt.anrui.riskcenter.api.loandiff.flowable.*; |
|||
import com.yxt.anrui.riskcenter.api.loandiffdetails.*; |
|||
import com.yxt.anrui.riskcenter.api.loansolutions.PushVo; |
|||
import com.yxt.anrui.riskcenter.api.loansolutions.app.SolutionsDetailsVo; |
|||
import com.yxt.anrui.riskcenter.api.loantemplate.LoanTemplate; |
|||
import com.yxt.anrui.riskcenter.api.loantemplate.LoanTemplateQuery; |
|||
import com.yxt.anrui.riskcenter.api.loantemplate.LoanTemplateVo; |
|||
import com.yxt.anrui.riskcenter.api.loantemplate.flowable.SubmitTemplateDto; |
|||
import com.yxt.anrui.riskcenter.api.loantemplate.flowable.TemplateApplyNodeVo; |
|||
import com.yxt.anrui.riskcenter.biz.loandiffdetails.LoanDiffDetailsService; |
|||
import com.yxt.anrui.riskcenter.biz.loantemplate.LoanTemplateMapper; |
|||
import com.yxt.common.base.config.component.FileUploadComponent; |
|||
import com.yxt.common.base.service.MybatisBaseService; |
|||
import com.yxt.common.base.utils.PagerUtil; |
|||
import com.yxt.common.base.utils.StringUtils; |
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import com.yxt.messagecenter.api.message.MessageFeign; |
|||
import com.yxt.messagecenter.api.message.MessageFlowVo; |
|||
import com.yxt.messagecenter.api.message.MessageFlowableQuery; |
|||
import org.apache.tomcat.util.threads.ThreadPoolExecutor; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
import java.math.BigDecimal; |
|||
import java.util.*; |
|||
import java.util.concurrent.*; |
|||
import java.util.stream.Collectors; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/13 |
|||
**/ |
|||
@Service |
|||
public class LoanDiffService extends MybatisBaseService<LoanDiffMapper, LoanDiff> { |
|||
|
|||
@Autowired |
|||
private FlowableFeign flowableFeign; |
|||
|
|||
@Autowired |
|||
private FlowFeign flowFeign; |
|||
|
|||
@Autowired |
|||
private FlowTaskFeign flowTaskFeign; |
|||
@Autowired |
|||
private MessageFeign messageFeign; |
|||
@Autowired |
|||
private LoanDiffDetailsService loanDiffDetailsService; |
|||
@Autowired |
|||
private FileUploadComponent fileUploadComponent; |
|||
@Autowired |
|||
private SysUserFeign sysUserFeign; |
|||
@Autowired |
|||
private FinKingDeeFeign finKingDeeFeign; |
|||
@Autowired |
|||
private BasePurchaseSystemFeign basePurchaseSystemFeign; |
|||
@Autowired |
|||
private SysOrganizationFeign sysOrganizationFeign; |
|||
|
|||
/** |
|||
* 判断提交的流程是否被允许 |
|||
* |
|||
* @param dto |
|||
* @return |
|||
*/ |
|||
private synchronized int submitBusinessData(SubmitDiffDto dto, LoanDiff loanDiff) { |
|||
int r = 0; |
|||
if (StringUtils.isBlank(dto.getSid())) { |
|||
r = 1; |
|||
} else { |
|||
if (loanDiff != null) { |
|||
String businessTaskId = loanDiff.getTaskId(); |
|||
if (StringUtils.isBlank(businessTaskId) && StringUtils.isBlank(dto.getTaskId())) { |
|||
//新提交
|
|||
r = 1; |
|||
} else if (StringUtils.isNotBlank(businessTaskId) && businessTaskId.equals(dto.getTaskId())) { |
|||
//二次提交//只有数据一致的时候才能进行下一步
|
|||
r = 2; |
|||
} |
|||
} else { |
|||
r = 3; |
|||
} |
|||
|
|||
} |
|||
return r; |
|||
} |
|||
|
|||
public ResultBean submitDiffApply(SubmitDiffDto dto) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
LoanDiff loanDiff = fetchBySid(dto.getSid()); |
|||
int r = submitBusinessData(dto, loanDiff); |
|||
if (r == 3) { |
|||
return rb.setMsg("该申请不存在"); |
|||
} |
|||
if (r == 0) { |
|||
return rb.setMsg("操作失败!提交的数据不一致"); |
|||
} |
|||
ResultBean<String> resultBean = updateDiff(dto); |
|||
if (!resultBean.getSuccess()) { |
|||
return rb.setMsg(resultBean.getMsg()); |
|||
} |
|||
|
|||
String businessSid = dto.getSid(); |
|||
loanDiff = fetchBySid(businessSid); |
|||
//创建BusinessVariables实体对象
|
|||
BusinessVariables bv = new BusinessVariables(); |
|||
//流程中的参数赋值、若有网关,则赋值网关中判断的字段。
|
|||
Map<String, Object> variables = new HashMap<>(); |
|||
Map<String, Object> appMap = new HashMap<>(); |
|||
appMap.put("sid", businessSid); |
|||
variables.put("app", appMap); |
|||
//用户的部门全路径sid
|
|||
bv.setOrgSidPath(loanDiff.getOrgSidPath()); |
|||
bv.setBusinessSid(businessSid); |
|||
bv.setUserSid(loanDiff.getCreateBySid()); |
|||
bv.setFormVariables(variables); |
|||
if (r == 1) { |
|||
//ToDo:流程定义id
|
|||
bv.setModelId(ProcDefEnum.LOANDIFFAPPLY.getProDefId()); |
|||
ResultBean<UpdateFlowFieldVo> voResultBean = flowFeign.startProcess(bv); |
|||
if (!voResultBean.getSuccess()) { |
|||
return rb.setMsg(voResultBean.getMsg()); |
|||
} |
|||
UpdateFlowFieldVo ufVo = voResultBean.getData(); |
|||
updateFlowFiled(BeanUtil.beanToMap(ufVo)); |
|||
loanDiff = fetchBySid(businessSid); |
|||
//==================================添加线程
|
|||
try { |
|||
ThreadFactory namedThreadFactory = new ThreadFactoryBuilder() |
|||
.setNameFormat("demo-pool-%d").build(); |
|||
ExecutorService pool = new ThreadPoolExecutor(2, 100, |
|||
0L, TimeUnit.MILLISECONDS, |
|||
new LinkedBlockingQueue<Runnable>(1024), namedThreadFactory, new ThreadPoolExecutor.AbortPolicy()); |
|||
LoanDiff finalLoanDiff = loanDiff; |
|||
Future future1 = pool.submit(() -> { |
|||
//极光推送
|
|||
MessageFlowableQuery messageFlowableQuery = new MessageFlowableQuery(); |
|||
MessageFlowVo messageFlowVo = new MessageFlowVo(); |
|||
BeanUtil.copyProperties(ufVo, messageFlowVo); |
|||
messageFlowableQuery.setUfVo(messageFlowVo); |
|||
messageFlowableQuery.setAppMap(appMap); |
|||
messageFlowableQuery.setBusinessSid(businessSid); |
|||
messageFlowableQuery.setModuleName("放款差额确认申请"); |
|||
messageFlowableQuery.setMsgContent(finalLoanDiff.getCreateByName() + "提交的" + messageFlowableQuery.getModuleName() + ",请审批"); |
|||
messageFlowableQuery.setMsgTitle("放款差额确认"); |
|||
ResultBean<String> stringResultBean = messageFeign.pushMessage(messageFlowableQuery); |
|||
}); |
|||
} catch (Exception e) { |
|||
e.printStackTrace(); |
|||
} |
|||
//==================================添加线程
|
|||
return voResultBean; |
|||
} |
|||
if (r == 2) { |
|||
// ToDo:驳回到发起人后再次提交
|
|||
if (StringUtils.isBlank(dto.getInstanceId())) { |
|||
return rb.setMsg("参数错误:instanceId"); |
|||
} |
|||
bv.setTaskId(loanDiff.getTaskId()); |
|||
bv.setTaskDefKey(loanDiff.getNodeId()); |
|||
bv.setComment("重新提交"); |
|||
bv.setInstanceId(dto.getInstanceId()); |
|||
return complete(bv); |
|||
} |
|||
return rb; |
|||
} |
|||
|
|||
public ResultBean complete(BusinessVariables bv) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
String businessSid = bv.getBusinessSid(); |
|||
LoanDiff loanDiff = fetchBySid(businessSid); |
|||
Map<String, Object> variables = new HashMap<>(); |
|||
Map<String, Object> appMap = new HashMap<>(); |
|||
appMap.put("sid", businessSid); |
|||
variables.put("app", appMap); |
|||
bv.setFormVariables(variables); |
|||
bv.setOrgSidPath(loanDiff.getOrgSidPath()); |
|||
bv.setModelId(loanDiff.getProcDefId()); |
|||
if (bv.getTaskId().equals(loanDiff.getTaskId())) { |
|||
ResultBean<UpdateFlowFieldVo> resultBean = flowFeign.handleProsess(bv); |
|||
if (!resultBean.getSuccess()) { |
|||
return rb.setMsg(resultBean.getMsg()); |
|||
} |
|||
UpdateFlowFieldVo ufVo = resultBean.getData(); |
|||
updateFlowFiled(BeanUtil.beanToMap(resultBean.getData())); |
|||
if ("Event_end".equals(resultBean.getData().getTaskDefKey())) { |
|||
//推送财务其他应收单
|
|||
pushFinOther(businessSid); |
|||
} else { |
|||
//极光推送
|
|||
loanDiff = fetchBySid(businessSid); |
|||
MessageFlowableQuery messageFlowableQuery = new MessageFlowableQuery(); |
|||
MessageFlowVo messageFlowVo = new MessageFlowVo(); |
|||
BeanUtil.copyProperties(ufVo, messageFlowVo); |
|||
messageFlowVo.setProcDefId(loanDiff.getProcDefId()); |
|||
messageFlowVo.setProcInsId(loanDiff.getProcInstId()); |
|||
messageFlowableQuery.setUfVo(messageFlowVo); |
|||
messageFlowableQuery.setAppMap(appMap); |
|||
messageFlowableQuery.setBusinessSid(businessSid); |
|||
messageFlowableQuery.setModuleName("放款差额确认申请"); |
|||
messageFlowableQuery.setMsgContent(loanDiff.getCreateByName() + "提交的" + messageFlowableQuery.getModuleName() + ",请审批"); |
|||
messageFlowableQuery.setMsgTitle("放款差额确认"); |
|||
ResultBean<String> stringResultBean = messageFeign.pushMessage(messageFlowableQuery); |
|||
} |
|||
return rb.success().setData(resultBean.getData()); |
|||
} else { |
|||
return rb.setMsg("操作失败!提交的数据不一致"); |
|||
} |
|||
} |
|||
|
|||
public void pushFinOther(String sid) { |
|||
List<LoanDiffDetails> list = loanDiffDetailsService.selectDetailsByMainSid(sid); |
|||
list.removeAll(Collections.singleton(null)); |
|||
if (!list.isEmpty()) { |
|||
for (int i = 0; i < list.size(); i++) { |
|||
CapitalCreditResult creditResult = new CapitalCreditResult(); |
|||
LoanDiffDetails loanDiffDetails = list.get(i); |
|||
String busVinSid = loanDiffDetails.getBusVinSid(); |
|||
BusSalesOrderVehicle busSalesOrderVehicle = baseMapper.selectByBusVinSid(busVinSid); |
|||
BusSalesOrder busSalesOrder = baseMapper.selectByOrderSid(busSalesOrderVehicle.getSalesOrderSid()); |
|||
String customerNumber = ""; |
|||
//判断财务系统是否有客户
|
|||
Boolean aBoolean = finKingDeeFeign.customerExistState(busSalesOrderVehicle.getTemporaryNo()).getData(); |
|||
String linkNo = ""; |
|||
if (!aBoolean) { |
|||
BdCustomer bdCustomer = new BdCustomer(); |
|||
bdCustomer.setFNumber(busSalesOrderVehicle.getTemporaryNo()); |
|||
bdCustomer.setFShortName(busSalesOrder.getContractNo()); |
|||
BasePurchaseSystemDetailsVo data = basePurchaseSystemFeign.fetchDetailsByDeptSid(busSalesOrder.getPurchaseSystemSid()).getData(); |
|||
bdCustomer.setTOrgIds(data.getOrgCode()); |
|||
if (StringUtils.isNotBlank(busSalesOrderVehicle.getLinkNo())) { |
|||
String vinNo = busSalesOrderVehicle.getLinkNo(); |
|||
if (vinNo.length() > 8) { |
|||
linkNo = vinNo.substring(vinNo.length() - 8); |
|||
} else { |
|||
linkNo = busSalesOrderVehicle.getLinkNo(); |
|||
} |
|||
bdCustomer.setFName(busSalesOrderVehicle.getBorrowName() + linkNo); |
|||
} else { |
|||
bdCustomer.setFName(busSalesOrderVehicle.getBorrowName() + busSalesOrderVehicle.getTemporaryNo()); |
|||
} |
|||
ResultBean<String> resultBean = finKingDeeFeign.draftBdCustomer(bdCustomer); |
|||
if (resultBean.getSuccess()) { |
|||
customerNumber = bdCustomer.getFNumber(); |
|||
} |
|||
} else { |
|||
customerNumber = busSalesOrderVehicle.getTemporaryNo(); |
|||
} |
|||
creditResult.setCommUnit(customerNumber); |
|||
SysOrganizationVo sysOrganizationVo1 = sysOrganizationFeign.fetchBySid(busSalesOrder.getUseOrgSid()).getData(); |
|||
creditResult.setCollectionOrg(sysOrganizationVo1.getOrgCode()); |
|||
creditResult.setBussDate(DateUtil.formatDate(new Date())); |
|||
List<CapitalCreditResult.CapitalCreditResultDetailDto> collectionDetailDtoListOne = new ArrayList<>(); |
|||
SysOrganizationVo sysOrganizationVo2 = sysOrganizationFeign.fetchBySid(busSalesOrder.getOrgSid()).getData(); |
|||
if (loanDiffDetails.getRealityLoan() != null) {//实扣贷款保证金
|
|||
CapitalCreditResult.CapitalCreditResultDetailDto creditResultDetailDto = new CapitalCreditResult.CapitalCreditResultDetailDto(); |
|||
creditResultDetailDto.setDearDept(sysOrganizationVo2.getOrgCode()); |
|||
creditResultDetailDto.setExTaxMoney("-" + loanDiffDetails.getRealityLoanMargin().toString()); |
|||
creditResultDetailDto.setUseTo(CwSystemYT.BOND_AMOUNTS.getType()); |
|||
collectionDetailDtoListOne.add(creditResultDetailDto); |
|||
} |
|||
if (loanDiffDetails.getRealityDiscount() != null) {//实扣主产品厂家贴息
|
|||
CapitalCreditResult.CapitalCreditResultDetailDto creditResultDetailDto = new CapitalCreditResult.CapitalCreditResultDetailDto(); |
|||
creditResultDetailDto.setDearDept(sysOrganizationVo2.getOrgCode()); |
|||
creditResultDetailDto.setExTaxMoney("-" + loanDiffDetails.getRealityDiscount().toString()); |
|||
creditResultDetailDto.setUseTo(CwSystemYT.SANFANG_TIEXI_DIECHEKUAN.getType()); |
|||
collectionDetailDtoListOne.add(creditResultDetailDto); |
|||
} |
|||
if (loanDiffDetails.getRealityOtherDiscount() != null) {//实扣其他融厂家贴息
|
|||
CapitalCreditResult.CapitalCreditResultDetailDto creditResultDetailDto = new CapitalCreditResult.CapitalCreditResultDetailDto(); |
|||
creditResultDetailDto.setDearDept(sysOrganizationVo2.getOrgCode()); |
|||
creditResultDetailDto.setExTaxMoney("-" + loanDiffDetails.getRealityOtherDiscount().toString()); |
|||
creditResultDetailDto.setUseTo(CwSystemYT.SANFANG_TIEXI_DIECHEKUAN.getType()); |
|||
collectionDetailDtoListOne.add(creditResultDetailDto); |
|||
} |
|||
if (loanDiffDetails.getReceivedPremium() != null) {//已收意外险
|
|||
CapitalCreditResult.CapitalCreditResultDetailDto creditResultDetailDto = new CapitalCreditResult.CapitalCreditResultDetailDto(); |
|||
creditResultDetailDto.setDearDept(sysOrganizationVo2.getOrgCode()); |
|||
creditResultDetailDto.setExTaxMoney("-" + loanDiffDetails.getReceivedPremium().toString()); |
|||
creditResultDetailDto.setUseTo(CwSystemYT.PROXY_ACCIDENT_PREMIUM.getType()); |
|||
collectionDetailDtoListOne.add(creditResultDetailDto); |
|||
} |
|||
if (loanDiffDetails.getDiffPremium() != null) {//意外险差额
|
|||
CapitalCreditResult.CapitalCreditResultDetailDto creditResultDetailDto = new CapitalCreditResult.CapitalCreditResultDetailDto(); |
|||
creditResultDetailDto.setDearDept(sysOrganizationVo2.getOrgCode()); |
|||
creditResultDetailDto.setExTaxMoney(loanDiffDetails.getDiffPremium().toString()); |
|||
creditResultDetailDto.setUseTo(CwSystemYT.PROXY_ACCIDENT_PREMIUM.getType()); |
|||
collectionDetailDtoListOne.add(creditResultDetailDto); |
|||
} |
|||
creditResult.setResultDetails(collectionDetailDtoListOne); |
|||
finKingDeeFeign.pushOtherReceivableBill(creditResult); |
|||
} |
|||
} |
|||
} |
|||
|
|||
public ResultBean<List<DiffApplyNodeVo>> getPreviousNodesForReject(DiffApplyNodeQuery query) { |
|||
ResultBean<List<DiffApplyNodeVo>> rb = ResultBean.fireFail(); |
|||
BusinessVariables bv = new BusinessVariables(); |
|||
BeanUtil.copyProperties(query, bv); |
|||
LoanDiff loanDiff = fetchBySid(query.getBusinessSid()); |
|||
bv.setModelId(loanDiff.getProcDefId()); |
|||
ResultBean<List<Map<String, Object>>> resultBean = flowTaskFeign.getPreviousNodesForReject(bv); |
|||
//判断数组是否为空,若为空则赋值,若不为空,则遍历循环将map中的数据赋值给TemplateApplyNodeVo
|
|||
List<DiffApplyNodeVo> voList = Optional.ofNullable(resultBean.getData()).orElse(new ArrayList<>()).stream().map(m -> JSON.parseObject(JSON.toJSONString(m), DiffApplyNodeVo.class)).collect(Collectors.toList()); |
|||
return rb.success().setData(voList); |
|||
} |
|||
|
|||
public ResultBean<List<DiffApplyNodeVo>> getNextNodesForSubmit(DiffApplyNodeQuery query) { |
|||
ResultBean<List<DiffApplyNodeVo>> rb = ResultBean.fireFail(); |
|||
BusinessVariables bv = new BusinessVariables(); |
|||
BeanUtil.copyProperties(query, bv); |
|||
LoanDiff loanDiff = fetchBySid(query.getBusinessSid()); |
|||
bv.setModelId(loanDiff.getProcDefId()); |
|||
ResultBean<List<Map<String, Object>>> resultBean = flowTaskFeign.getNextNodesForSubmit(bv); |
|||
//判断数组是否为空,若为空则赋值,若不为空,则遍历循环将map中的数据赋值给TemplateApplyNodeVo
|
|||
List<DiffApplyNodeVo> voList = Optional.ofNullable(resultBean.getData()).orElse(new ArrayList<>()).stream().map(m -> JSON.parseObject(JSON.toJSONString(m), DiffApplyNodeVo.class)).collect(Collectors.toList()); |
|||
return rb.success().setData(voList); |
|||
} |
|||
|
|||
public ResultBean taskReject(DiffApplyTaskQuery query) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
String businessSid = query.getBusinessSid(); |
|||
LoanDiff loanDiff = fetchBySid(businessSid); |
|||
if (loanDiff == null) { |
|||
return rb.setMsg("该申请不存在"); |
|||
} |
|||
String businessTaskId = loanDiff.getTaskId(); |
|||
if (StringUtils.isNotBlank(businessTaskId)) { |
|||
if (businessTaskId.equals(query.getTaskId())) { |
|||
if (StringUtils.isBlank(query.getComment())) { |
|||
return rb.setMsg("请填写意见"); |
|||
} |
|||
if (StringUtils.isBlank(query.getUserSid())) { |
|||
return rb.setMsg("参数错误:userSid"); |
|||
} |
|||
FlowTaskVo flowTaskVo = new FlowTaskVo(); |
|||
BeanUtil.copyProperties(query, flowTaskVo); |
|||
Map<String, Object> variables = new HashMap<>(); |
|||
Map<String, Object> appMap = new HashMap<>(); |
|||
appMap.put("sid", businessSid); |
|||
variables.put("app", appMap); |
|||
ResultBean<UpdateFlowFieldVo> resultBean = flowableFeign.taskReject(flowTaskVo); |
|||
if (!resultBean.getSuccess()) { |
|||
return rb.setMsg(resultBean.getMsg()); |
|||
} |
|||
UpdateFlowFieldVo ufVo = resultBean.getData(); |
|||
Map<String, Object> map = BeanUtil.beanToMap(ufVo); |
|||
//更新业务中的流程相关的参数
|
|||
updateFlowFiled(map); |
|||
//极光推送
|
|||
loanDiff = fetchBySid(businessSid); |
|||
MessageFlowableQuery messageFlowableQuery = new MessageFlowableQuery(); |
|||
MessageFlowVo messageFlowVo = new MessageFlowVo(); |
|||
BeanUtil.copyProperties(ufVo, messageFlowVo); |
|||
String procId = loanDiff.getProcInstId(); |
|||
messageFlowVo.setProcInsId(procId); |
|||
messageFlowVo.setProcDefId(loanDiff.getProcDefId()); |
|||
messageFlowableQuery.setUfVo(messageFlowVo); |
|||
messageFlowableQuery.setAppMap(appMap); |
|||
messageFlowableQuery.setBusinessSid(businessSid); |
|||
messageFlowableQuery.setModuleName("放款差额确认申请"); |
|||
ResultBean<List<LatestTaskVo>> listResultBean = flowTaskFeign.getLatestTasks(procId); |
|||
String nextName = listResultBean.getData().get(0).getName_(); |
|||
String nextNodeUserSids = listResultBean.getData().get(0).getASSIGNEE_(); |
|||
if ("发起申请".equals(nextName)) { |
|||
messageFlowableQuery.setMsgContent("您提交的" + messageFlowableQuery.getModuleName() + "已被驳回,请重新提交"); |
|||
} else { |
|||
messageFlowableQuery.setMsgContent(loanDiff.getCreateByName() + "提交的" + messageFlowableQuery.getModuleName() + ",请审批"); |
|||
} |
|||
|
|||
messageFlowableQuery.setMsgTitle("放款差额确认"); |
|||
ResultBean<String> stringResultBean = messageFeign.pushMessage(messageFlowableQuery); |
|||
return rb.success(); |
|||
} |
|||
} |
|||
return rb.setMsg("操作失败!提交的数据不一致!"); |
|||
} |
|||
|
|||
public ResultBean revokeProcess(DiffApplyTaskQuery query) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
if (StringUtils.isBlank(query.getUserSid())) { |
|||
return rb.setMsg("参数错误:userSid"); |
|||
} |
|||
LoanDiff loanDiff = fetchBySid(query.getBusinessSid()); |
|||
String businessTaskId = loanDiff.getTaskId(); |
|||
if (StringUtils.isNotBlank(businessTaskId)) { |
|||
if (businessTaskId.equals(query.getTaskId())) { |
|||
FlowTaskVo flowTaskVo = new FlowTaskVo(); |
|||
BeanUtil.copyProperties(query, flowTaskVo); |
|||
ResultBean<UpdateFlowFieldVo> resultBean = flowableFeign.revokeProcess(flowTaskVo); |
|||
if (!resultBean.getSuccess()) { |
|||
return rb.setMsg(resultBean.getMsg()); |
|||
} |
|||
updateFlowFiled(BeanUtil.beanToMap(resultBean.getData())); |
|||
return rb.success().setData(resultBean.getData()); |
|||
} |
|||
} |
|||
return rb.setMsg("操作失败,提交的数据不一致!"); |
|||
} |
|||
|
|||
public ResultBean breakProcess(DiffApplyTaskQuery query) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
if (StringUtils.isBlank(query.getInstanceId())) { |
|||
return rb.setMsg("参数错误:instanceId"); |
|||
} |
|||
if (StringUtils.isBlank(query.getUserSid())) { |
|||
return rb.setMsg("参数错误:userSid"); |
|||
} |
|||
if (StringUtils.isBlank(query.getComment())) { |
|||
return rb.setMsg("请填写意见"); |
|||
} |
|||
LoanDiff loanDiff = fetchBySid(query.getBusinessSid()); |
|||
String businessTaskId = loanDiff.getTaskId(); |
|||
if (StringUtils.isNotBlank(businessTaskId)) { |
|||
if (query.getUserSid().equals(loanDiff.getCreateBySid())) { |
|||
FlowTaskVo flowTaskVo = new FlowTaskVo(); |
|||
BeanUtil.copyProperties(query, flowTaskVo); |
|||
ResultBean<UpdateFlowFieldVo> resultBean = flowableFeign.breakProcess(flowTaskVo); |
|||
if (!resultBean.getSuccess()) { |
|||
return rb.setMsg(resultBean.getMsg()); |
|||
} |
|||
Map<String, Object> map = BeanUtil.beanToMap(resultBean.getData()); |
|||
updateFlowFiled(map); |
|||
return rb.success().setData(resultBean.getData()); |
|||
} else { |
|||
if (businessTaskId.equals(query.getTaskId())) { |
|||
FlowTaskVo flowTaskVo = new FlowTaskVo(); |
|||
BeanUtil.copyProperties(query, flowTaskVo); |
|||
ResultBean<UpdateFlowFieldVo> resultBean = flowableFeign.breakProcess(flowTaskVo); |
|||
if (!resultBean.getSuccess()) { |
|||
return rb.setMsg(resultBean.getMsg()); |
|||
} |
|||
Map<String, Object> map = BeanUtil.beanToMap(resultBean.getData()); |
|||
updateFlowFiled(map); |
|||
return rb.success().setData(resultBean.getData()); |
|||
} |
|||
} |
|||
|
|||
} |
|||
return rb.setMsg("操作失败!提交的数据不一致!"); |
|||
} |
|||
|
|||
/** |
|||
* 更新流程的状态 |
|||
* |
|||
* @param map |
|||
* @return |
|||
*/ |
|||
private int updateFlowFiled(Map<String, Object> map) { |
|||
return baseMapper.updateFlowFiled(map); |
|||
} |
|||
|
|||
public PagerVo<LoanDiffVo> listPageVo(PagerQuery<LoanDiffQuery> pq) { |
|||
LoanDiffQuery query = pq.getParams(); |
|||
QueryWrapper<LoanDiff> qw = new QueryWrapper<>(); |
|||
if (query != null) { |
|||
//分公司
|
|||
if (StringUtils.isNotBlank(query.getUseOrgName())) { |
|||
qw.like("ld.useOrgName", query.getUseOrgName()); |
|||
} |
|||
//申请部门
|
|||
if (StringUtils.isNotBlank(query.getCreateDept())) { |
|||
qw.like("ld.createDept", query.getCreateDept()); |
|||
} |
|||
if (StringUtils.isNotBlank(query.getCreateByName())) { |
|||
qw.like("ld.createByName", query.getCreateByName()); |
|||
} |
|||
//========================================数据授权开始
|
|||
if (StringUtils.isNotBlank(query.getMenuUrl())) { |
|||
//=======================
|
|||
PrivilegeQuery privilegeQuery = new PrivilegeQuery(); |
|||
privilegeQuery.setOrgPath(query.getOrgPath()); |
|||
privilegeQuery.setMenuUrl(query.getMenuUrl()); |
|||
privilegeQuery.setUserSid(query.getUserSid()); |
|||
ResultBean<String> defaultIdReltBean = sysUserFeign.selectPrivilegeLevel(privilegeQuery); |
|||
if (org.apache.commons.lang3.StringUtils.isNotBlank(defaultIdReltBean.getData())) { |
|||
//数据权限ID(1集团、2事业部、3分公司、4部门、5个人)
|
|||
String orgSidPath = query.getOrgPath(); |
|||
orgSidPath = orgSidPath + "/"; |
|||
int i1 = orgSidPath.indexOf("/"); |
|||
int i2 = orgSidPath.indexOf("/", i1 + 1); |
|||
int i3 = orgSidPath.indexOf("/", i2 + 1); |
|||
int i4 = orgSidPath.indexOf("/", i3 + 1); |
|||
String orgLevelKey = defaultIdReltBean.getData(); |
|||
if ("1".equals(orgLevelKey)) { |
|||
orgSidPath = orgSidPath.substring(0, i1); |
|||
qw.like("ld.orgSidPath", orgSidPath); |
|||
} else if ("2".equals(orgLevelKey)) { |
|||
orgSidPath = orgSidPath.substring(0, i2); |
|||
qw.like("ld.orgSidPath", orgSidPath); |
|||
} else if ("3".equals(orgLevelKey)) { |
|||
orgSidPath = orgSidPath.substring(0, i3); |
|||
qw.like("ld.orgSidPath", orgSidPath); |
|||
} else if ("4".equals(orgLevelKey)) { |
|||
orgSidPath = orgSidPath.substring(0, i4); |
|||
qw.like("ld.orgSidPath", orgSidPath); |
|||
} else if ("5".equals(orgLevelKey)) { |
|||
qw.eq("ld.createBySid", query.getUserSid()); |
|||
} else { |
|||
PagerVo<LoanDiffVo> p = new PagerVo<>(); |
|||
return p; |
|||
} |
|||
} else { |
|||
PagerVo<LoanDiffVo> p = new PagerVo<>(); |
|||
return p; |
|||
} |
|||
} else { |
|||
PagerVo<LoanDiffVo> p = new PagerVo<>(); |
|||
return p; |
|||
|
|||
} |
|||
//申请日期开始时间
|
|||
String createTimeStart = query.getCreateTimeStart(); |
|||
//申请日期结束时间
|
|||
String createTimeEnd = query.getCreateTimeEnd(); |
|||
qw.apply(StringUtils.isNotBlank(createTimeStart), "date_format (ld.createTime,'%Y-%m-%d') >= date_format('" + createTimeStart + "','%Y-%m-%d')"). |
|||
apply(StringUtils.isNotBlank(createTimeEnd), "date_format (ld.createTime,'%Y-%m-%d') <= date_format('" + createTimeEnd + "','%Y-%m-%d')" |
|||
); |
|||
|
|||
} |
|||
IPage<LoanDiff> page = PagerUtil.queryToPage(pq); |
|||
IPage<LoanDiffVo> pagging = baseMapper.listPageVo(page, qw); |
|||
PagerVo<LoanDiffVo> p = PagerUtil.pageToVo(pagging, null); |
|||
return p; |
|||
} |
|||
|
|||
public ResultBean saveDiff(LoanDiffsDto dto) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
LoanDiff loanDiff = new LoanDiff(); |
|||
BeanUtil.copyProperties(dto, loanDiff); |
|||
List<LoanDiffDetailssDto> loanDiffDetailssDtos = dto.getLoanDiffDetailssDtos(); |
|||
loanDiffDetailssDtos.removeAll(Collections.singleton(null)); |
|||
if (!loanDiffDetailssDtos.isEmpty()) { |
|||
for (int i = 0; i < loanDiffDetailssDtos.size(); i++) { |
|||
LoanDiffDetailssDto loanDiffDetailssDto = loanDiffDetailssDtos.get(i); |
|||
LoanDiffDetails loanDiffDetails = new LoanDiffDetails(); |
|||
BeanUtil.copyProperties(loanDiffDetailssDto, loanDiffDetails); |
|||
loanDiffDetails.setMainSid(loanDiff.getSid()); |
|||
loanDiffDetailsService.insert(loanDiffDetails); |
|||
} |
|||
} |
|||
baseMapper.insert(loanDiff); |
|||
return rb.success(); |
|||
} |
|||
|
|||
public ResultBean updateDiff(LoanDiffDto dto) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
String sid = dto.getSid(); |
|||
LoanDiff loanDiff = fetchBySid(sid); |
|||
if (loanDiff == null) { |
|||
return rb.setMsg("该申请不存在"); |
|||
} |
|||
BeanUtil.copyProperties(dto, loanDiff, "sid"); |
|||
List<LoanDiffDetailsDto> loanDiffDetailsList = dto.getLoanDiffDetails(); |
|||
loanDiffDetailsList.removeAll(Collections.singleton(null)); |
|||
if (!loanDiffDetailsList.isEmpty()) { |
|||
for (int i = 0; i < loanDiffDetailsList.size(); i++) { |
|||
LoanDiffDetailsDto loanDiffDetailsDto = loanDiffDetailsList.get(i); |
|||
LoanDiffDetails loanDiffDetails1 = loanDiffDetailsService.fetchBySid(loanDiffDetailsDto.getSid()); |
|||
BeanUtil.copyProperties(loanDiffDetailsDto, loanDiffDetails1, "sid"); |
|||
loanDiffDetailsService.updateById(loanDiffDetails1); |
|||
} |
|||
} |
|||
// List<String> filesList = dto.getFilesList();
|
|||
List<DiffFile> fileLists = dto.getFilesList(); |
|||
fileLists.removeAll(Collections.singleton(null)); |
|||
if (!fileLists.isEmpty()) { |
|||
List<String> files = fileLists.stream().map(v -> v.getUrl()).collect(Collectors.toList()); |
|||
files.removeAll(Collections.singleton(null)); |
|||
if (!files.isEmpty()) { |
|||
String filss = String.join(",", files).replaceAll(fileUploadComponent.getUrlPrefix(), ""); |
|||
loanDiff.setFiles(filss); |
|||
baseMapper.updateById(loanDiff); |
|||
} |
|||
|
|||
} |
|||
return rb.success(); |
|||
} |
|||
|
|||
public ResultBean<LoanDiffInitDetails> details(String sid) { |
|||
ResultBean<LoanDiffInitDetails> rb = ResultBean.fireFail(); |
|||
LoanDiffInitDetails details = new LoanDiffInitDetails(); |
|||
LoanDiff loanDiff = fetchBySid(sid); |
|||
if (loanDiff == null) { |
|||
return rb.setMsg("该申请不存在"); |
|||
} |
|||
details.setCreateDept(loanDiff.getCreateDept()); |
|||
details.setCreateByName(loanDiff.getCreateByName()); |
|||
details.setRemarks(loanDiff.getRemarks()); |
|||
details.setSid(sid); |
|||
details.setCreateDate(DateUtil.format(loanDiff.getCreateTime(), "yyyy-MM-dd")); |
|||
details.setInstanceId(loanDiff.getProcInstId()); |
|||
details.setTaskId(loanDiff.getTaskId()); |
|||
String files = loanDiff.getFiles(); |
|||
if (StringUtils.isNotBlank(files)) { |
|||
List<String> stringList = Arrays.asList(files.split(",")).stream().map(c -> fileUploadComponent.getUrlPrefix() + c).collect(Collectors.toList()); |
|||
List<DiffFile> fileList = new ArrayList<>(); |
|||
for (int i = 0; i < stringList.size(); i++) { |
|||
DiffFile diffFile = new DiffFile(); |
|||
diffFile.setUrl(stringList.get(i)); |
|||
fileList.add(diffFile); |
|||
} |
|||
details.setFilesList(fileList); |
|||
} |
|||
List<LoanDiffDetailssVo> detailssVoList = loanDiffDetailsService.selectByMainSid(sid); |
|||
detailssVoList.removeAll(Collections.singleton(null)); |
|||
if (!detailssVoList.isEmpty()) { |
|||
details.setLoanDiffDetails(detailssVoList); |
|||
} |
|||
return rb.success().setData(details); |
|||
} |
|||
|
|||
public ResultBean<LoanDiffDetailsssApp> appDetails(String sid) { |
|||
ResultBean<LoanDiffDetailsssApp> rb = ResultBean.fireFail(); |
|||
LoanDiff loanDiff = fetchBySid(sid); |
|||
LoanDiffDetailsssApp loanDiffDetailsssApp = new LoanDiffDetailsssApp(); |
|||
loanDiffDetailsssApp.setSid(sid); |
|||
loanDiffDetailsssApp.setPublishInfo(loanDiff.getCreateDept() + "-" + loanDiff.getCreateByName()); |
|||
loanDiffDetailsssApp.setRemarks(loanDiff.getRemarks()); |
|||
loanDiffDetailsssApp.setTime(DateUtil.format(loanDiff.getCreateTime(), "yyyy-MM-dd")); |
|||
loanDiffDetailsssApp.setProcInsId(loanDiff.getProcInstId()); |
|||
loanDiffDetailsssApp.setTaskId(loanDiff.getTaskId()); |
|||
String files = loanDiff.getFiles(); |
|||
if (StringUtils.isNotBlank(files)) { |
|||
List<String> stringList = Arrays.asList(files.split(",")).stream().map(c -> fileUploadComponent.getUrlPrefix() + c).collect(Collectors.toList()); |
|||
loanDiffDetailsssApp.setFiles(stringList); |
|||
} |
|||
List<AppRecords> detailssVoList = loanDiffDetailsService.selectByMainSid2(sid); |
|||
detailssVoList.removeAll(Collections.singleton(null)); |
|||
if (!detailssVoList.isEmpty()) { |
|||
loanDiffDetailsssApp.setRecords(detailssVoList); |
|||
} |
|||
return rb.success().setData(loanDiffDetailsssApp); |
|||
} |
|||
|
|||
public ResultBean delegate(DelegateQuery query) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
FlowDelegateQuery delegateQuery = new FlowDelegateQuery(); |
|||
BeanUtil.copyProperties(query, delegateQuery); |
|||
flowFeign.delegate(delegateQuery); |
|||
return rb.success(); |
|||
} |
|||
} |
@ -0,0 +1,23 @@ |
|||
package com.yxt.anrui.riskcenter.biz.loandiffdetails; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.yxt.anrui.riskcenter.api.loandiffdetails.AppRecords; |
|||
import com.yxt.anrui.riskcenter.api.loandiffdetails.LoanDiffDetails; |
|||
import com.yxt.anrui.riskcenter.api.loandiffdetails.LoanDiffDetailssVo; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/14 |
|||
**/ |
|||
@Mapper |
|||
public interface LoanDiffDetailsMapper extends BaseMapper<LoanDiffDetails> { |
|||
List<LoanDiffDetailssVo> selectByMainSid(String sid); |
|||
|
|||
List<AppRecords> selectByMainSid2(String sid); |
|||
|
|||
List<LoanDiffDetails> selectDetailsByMainSid(String sid); |
|||
} |
@ -0,0 +1,36 @@ |
|||
<?xml version="1.0" encoding="UTF-8" ?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.anrui.riskcenter.biz.loandiffdetails.LoanDiffDetailsMapper"> |
|||
<select id="selectByMainSid" resultType="com.yxt.anrui.riskcenter.api.loandiffdetails.LoanDiffDetailssVo"> |
|||
select * |
|||
from anrui_riskcenter.loan_diff_details |
|||
where mainSid = #{sid} |
|||
</select> |
|||
|
|||
<select id="selectByMainSid2" resultType="com.yxt.anrui.riskcenter.api.loandiffdetails.AppRecords"> |
|||
select vinNo vin, |
|||
bankName zf, |
|||
borrowName loanName, |
|||
makeLoan fk1, |
|||
realityLoan fk2, |
|||
diffLoan diffFk, |
|||
makeDiscount mainTx1, |
|||
realityDiscount mainTx2, |
|||
diffDiscount diffMainTx, |
|||
makeLoanMargin dkbzj1, |
|||
realityLoanMargin dkbzj2, |
|||
diffLoanMargin diffDkbzj, |
|||
makeOtherDiscount otherTx1, |
|||
realityOtherDiscount otherTx2, |
|||
diffOtherDiscount diffOtherTx, |
|||
receivedPremium ywx1, |
|||
realityPremium ywx2, |
|||
diffPremium diffYwx |
|||
from anrui_riskcenter.loan_diff_details |
|||
where mainSid = #{sid} |
|||
</select> |
|||
|
|||
<select id="selectDetailsByMainSid" resultType="com.yxt.anrui.riskcenter.api.loandiffdetails.LoanDiffDetails"> |
|||
select * from anrui_riskcenter.loan_diff_details where mainSid = #{sid} |
|||
</select> |
|||
</mapper> |
@ -0,0 +1,9 @@ |
|||
package com.yxt.anrui.riskcenter.biz.loandiffdetails; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/14 |
|||
**/ |
|||
public class LoanDiffDetailsRest { |
|||
} |
@ -0,0 +1,31 @@ |
|||
package com.yxt.anrui.riskcenter.biz.loandiffdetails; |
|||
|
|||
import com.yxt.anrui.riskcenter.api.loandiff.LoanDiff; |
|||
import com.yxt.anrui.riskcenter.api.loandiffdetails.AppRecords; |
|||
import com.yxt.anrui.riskcenter.api.loandiffdetails.LoanDiffDetails; |
|||
import com.yxt.anrui.riskcenter.api.loandiffdetails.LoanDiffDetailssVo; |
|||
import com.yxt.anrui.riskcenter.biz.loandiff.LoanDiffMapper; |
|||
import com.yxt.common.base.service.MybatisBaseService; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2023/11/14 |
|||
**/ |
|||
@Service |
|||
public class LoanDiffDetailsService extends MybatisBaseService<LoanDiffDetailsMapper, LoanDiffDetails> { |
|||
public List<LoanDiffDetailssVo> selectByMainSid(String sid) { |
|||
return baseMapper.selectByMainSid(sid); |
|||
} |
|||
|
|||
public List<AppRecords> selectByMainSid2(String sid) { |
|||
return baseMapper.selectByMainSid2(sid); |
|||
} |
|||
|
|||
public List<LoanDiffDetails> selectDetailsByMainSid(String sid) { |
|||
return baseMapper.selectDetailsByMainSid(sid); |
|||
} |
|||
} |
Some files were not shown because too many files changed in this diff
Loading…
Reference in new issue