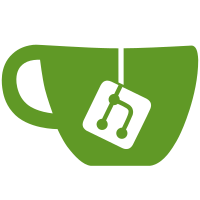
39 changed files with 894 additions and 51 deletions
@ -0,0 +1,35 @@ |
|||||
|
package com.yxt.anrui.riskcenter.api.loanrepaymenthistory; |
||||
|
|
||||
|
import com.yxt.common.core.query.Query; |
||||
|
import io.swagger.annotations.ApiModelProperty; |
||||
|
import lombok.Data; |
||||
|
|
||||
|
/** |
||||
|
* @author Fan |
||||
|
* @description |
||||
|
* @date 2023/12/5 8:41 |
||||
|
*/ |
||||
|
@Data |
||||
|
public class TransferPaymentQuery implements Query { |
||||
|
|
||||
|
private String vinNo; //车架号
|
||||
|
private String bankName; //资方
|
||||
|
private String bankContractNo; //资方合同号
|
||||
|
@ApiModelProperty("应还开始日期") |
||||
|
private String dueStartDate; |
||||
|
@ApiModelProperty("应还结束日期") |
||||
|
private String dueEndDate; |
||||
|
private String accountTypeKey; //转付账户类型key
|
||||
|
private String prepareStartDate; //预转付开始日期
|
||||
|
private String prepareEndDate; //预转付结束日期
|
||||
|
private String overdueState; //转付状态
|
||||
|
@ApiModelProperty("组织全路径") |
||||
|
private String orgPath; |
||||
|
@ApiModelProperty("菜单sid") |
||||
|
private String menuSid; |
||||
|
@ApiModelProperty("菜单url") |
||||
|
private String menuUrl; |
||||
|
@ApiModelProperty("用户sid") |
||||
|
private String userSid; |
||||
|
private String type; //0待转付 1已转付
|
||||
|
} |
@ -0,0 +1,32 @@ |
|||||
|
package com.yxt.anrui.riskcenter.api.loanrepaymenthistory; |
||||
|
|
||||
|
import com.yxt.common.core.vo.Vo; |
||||
|
import lombok.Data; |
||||
|
|
||||
|
/** |
||||
|
* @author Fan |
||||
|
* @description |
||||
|
* @date 2023/12/5 8:40 |
||||
|
*/ |
||||
|
@Data |
||||
|
public class TransferPaymentVo implements Vo { |
||||
|
|
||||
|
private String paymentState; //转付状态
|
||||
|
private String vinNo; //车架号
|
||||
|
private String loanContractNo; //贷款合同号
|
||||
|
private String bankName; //资方
|
||||
|
private String bankContractNo; //资方合同号
|
||||
|
private String borrowerName; //贷款人
|
||||
|
private String period; //期数
|
||||
|
private String dueDate; //应还日期
|
||||
|
private String dueMoney; //实还金额
|
||||
|
private String prepareDate; //预转付日期
|
||||
|
private String spread; //息差
|
||||
|
private String transferPrincipal; //转付本金
|
||||
|
private String defaultInterest; //转付罚息
|
||||
|
private String accountType; //转付账户类型
|
||||
|
private String account; //转付账户
|
||||
|
private String accountNumber; //转付账户账号
|
||||
|
|
||||
|
|
||||
|
} |
@ -0,0 +1,49 @@ |
|||||
|
package com.yxt.anrui.riskcenter.api.loantransferpaymentapply; |
||||
|
|
||||
|
import com.baomidou.mybatisplus.annotation.TableName; |
||||
|
import com.yxt.common.core.domain.BaseEntity; |
||||
|
import io.swagger.annotations.ApiModel; |
||||
|
import io.swagger.annotations.ApiModelProperty; |
||||
|
import lombok.Data; |
||||
|
|
||||
|
import java.util.Date; |
||||
|
|
||||
|
/** |
||||
|
* @author liupopo |
||||
|
* @version 1.0 |
||||
|
* @since 1.0 |
||||
|
*/ |
||||
|
@ApiModel(value = "代收代付申请", description = "代收代付申请") |
||||
|
@TableName("loan_transfer_payment_apply") |
||||
|
@Data |
||||
|
public class LoanTransferPaymentApply extends BaseEntity { |
||||
|
private static final long serialVersionUID = 1L; |
||||
|
|
||||
|
@ApiModelProperty("申请人") |
||||
|
private String applyName; // 申请人
|
||||
|
@ApiModelProperty("申请日期") |
||||
|
private Date applyDate; // 申请日期
|
||||
|
@ApiModelProperty("申请编号") |
||||
|
private String billNo; |
||||
|
@ApiModelProperty("申请部门") |
||||
|
private String dept; |
||||
|
@ApiModelProperty("申请部门sid") |
||||
|
private String deptSid; |
||||
|
@ApiModelProperty("使用组织sid") |
||||
|
private String useOrgSid; // 使用组织sid
|
||||
|
@ApiModelProperty("使用组织名称") |
||||
|
private String useOrgName; // 使用组织名称
|
||||
|
@ApiModelProperty("流程定义的id") |
||||
|
private String procDefId; // 流程定义的id
|
||||
|
@ApiModelProperty("环节定义的sid") |
||||
|
private String nodeSid; // 环节定义的sid
|
||||
|
@ApiModelProperty("流程实例的sid") |
||||
|
private String procInstId; // 流程实例的sid
|
||||
|
@ApiModelProperty("流程状态") |
||||
|
private String nodeState; // 流程状态
|
||||
|
@ApiModelProperty("taskId") |
||||
|
private String taskId; // taskId
|
||||
|
@ApiModelProperty("申请人组织路径") |
||||
|
private String orgSidPath; // 申请人组织路径
|
||||
|
|
||||
|
} |
@ -0,0 +1,27 @@ |
|||||
|
package com.yxt.anrui.riskcenter.api.loantransferpaymentapply; |
||||
|
|
||||
|
import com.yxt.common.core.dto.Dto; |
||||
|
import lombok.Data; |
||||
|
|
||||
|
import java.util.ArrayList; |
||||
|
import java.util.List; |
||||
|
|
||||
|
/** |
||||
|
* @author Administrator |
||||
|
* @description |
||||
|
* @date 2023/11/13 14:11 |
||||
|
*/ |
||||
|
@Data |
||||
|
public class LoanTransferPaymentApplyDto implements Dto { |
||||
|
|
||||
|
private String sid; |
||||
|
private String userSid; |
||||
|
private String dept; |
||||
|
private String deptSid; |
||||
|
private String applyName; |
||||
|
private String applyDate; |
||||
|
private String remarks; |
||||
|
private String orgPath; |
||||
|
private List<LoanTransferPaymentApplyFile> files = new ArrayList<>(); |
||||
|
private List<LoanTransferPaymentRecordVo> records = new ArrayList<>(); |
||||
|
} |
@ -0,0 +1,35 @@ |
|||||
|
package com.yxt.anrui.riskcenter.api.loantransferpaymentapply; |
||||
|
|
||||
|
import com.yxt.anrui.riskcenter.api.loanbuckleapply.app.AppBuckleDetailsVo; |
||||
|
import com.yxt.anrui.riskcenter.api.loanbuckleapply.flow.*; |
||||
|
import com.yxt.common.core.query.PagerQuery; |
||||
|
import com.yxt.common.core.result.ResultBean; |
||||
|
import com.yxt.common.core.vo.PagerVo; |
||||
|
import io.swagger.annotations.Api; |
||||
|
import io.swagger.annotations.ApiOperation; |
||||
|
import io.swagger.annotations.ApiParam; |
||||
|
import org.springframework.cloud.openfeign.FeignClient; |
||||
|
import org.springframework.cloud.openfeign.SpringQueryMap; |
||||
|
import org.springframework.web.bind.annotation.*; |
||||
|
|
||||
|
import javax.validation.Valid; |
||||
|
import java.util.List; |
||||
|
|
||||
|
|
||||
|
/** |
||||
|
* @author liupopo |
||||
|
* @version 1.0 |
||||
|
* @since 1.0 |
||||
|
*/ |
||||
|
@Api(tags = "代收代付申请") |
||||
|
@FeignClient( |
||||
|
contextId = "anrui-riskcenter-LoanTransferPaymentApply", |
||||
|
name = "anrui-riskcenter", |
||||
|
path = "v1/loantransferpaymentapply", |
||||
|
fallback = LoanTransferPaymentApplyFeignFallback.class) |
||||
|
public interface LoanTransferPaymentApplyFeign { |
||||
|
|
||||
|
|
||||
|
|
||||
|
|
||||
|
} |
@ -0,0 +1,17 @@ |
|||||
|
package com.yxt.anrui.riskcenter.api.loantransferpaymentapply; |
||||
|
|
||||
|
|
||||
|
import com.yxt.anrui.riskcenter.api.loanbuckleapply.app.AppBuckleDetailsVo; |
||||
|
import com.yxt.anrui.riskcenter.api.loanbuckleapply.flow.*; |
||||
|
import com.yxt.common.core.query.PagerQuery; |
||||
|
import com.yxt.common.core.result.ResultBean; |
||||
|
import com.yxt.common.core.vo.PagerVo; |
||||
|
import org.springframework.stereotype.Component; |
||||
|
|
||||
|
import java.util.List; |
||||
|
|
||||
|
|
||||
|
@Component |
||||
|
public class LoanTransferPaymentApplyFeignFallback implements LoanTransferPaymentApplyFeign { |
||||
|
|
||||
|
} |
@ -0,0 +1,18 @@ |
|||||
|
package com.yxt.anrui.riskcenter.api.loantransferpaymentapply; |
||||
|
|
||||
|
import lombok.Data; |
||||
|
|
||||
|
/** |
||||
|
* @author Administrator |
||||
|
* @description |
||||
|
* @date 2023/11/17 9:18 |
||||
|
*/ |
||||
|
@Data |
||||
|
public class LoanTransferPaymentApplyFile { |
||||
|
private String filePath; |
||||
|
private String name; |
||||
|
private String size; |
||||
|
private String status; |
||||
|
private String uid; |
||||
|
private String url; |
||||
|
} |
@ -0,0 +1,34 @@ |
|||||
|
package com.yxt.anrui.riskcenter.api.loantransferpaymentapply; |
||||
|
|
||||
|
import com.yxt.anrui.riskcenter.api.loanbuckleapply.LoanBuckleHistoryRecord; |
||||
|
import com.yxt.common.core.vo.Vo; |
||||
|
import io.swagger.annotations.ApiModelProperty; |
||||
|
import lombok.Data; |
||||
|
|
||||
|
import java.util.ArrayList; |
||||
|
import java.util.List; |
||||
|
|
||||
|
/** |
||||
|
* @author Administrator |
||||
|
* @description |
||||
|
* @date 2023/11/13 13:43 |
||||
|
*/ |
||||
|
@Data |
||||
|
public class LoanTransferPaymentApplyInit implements Vo { |
||||
|
|
||||
|
private String sid; |
||||
|
private String userSid; |
||||
|
private String dept; |
||||
|
private String deptSid; |
||||
|
private String applyName; |
||||
|
private String applyDate; |
||||
|
private String remarks; |
||||
|
private String orgPath; |
||||
|
@ApiModelProperty("任务id") |
||||
|
private String taskId; |
||||
|
@ApiModelProperty("实例id") |
||||
|
private String instanceId; |
||||
|
private List<LoanTransferPaymentApplyFile> files = new ArrayList<>(); |
||||
|
private List<LoanTransferPaymentRecordVo> records = new ArrayList<>(); |
||||
|
|
||||
|
} |
@ -0,0 +1,31 @@ |
|||||
|
package com.yxt.anrui.riskcenter.api.loantransferpaymentapply; |
||||
|
|
||||
|
import com.yxt.common.core.query.Query; |
||||
|
import io.swagger.annotations.ApiModelProperty; |
||||
|
import lombok.Data; |
||||
|
|
||||
|
/** |
||||
|
* @author Administrator |
||||
|
* @description |
||||
|
* @date 2023/11/13 13:32 |
||||
|
*/ |
||||
|
@Data |
||||
|
public class LoanTransferPaymentApplyQuery implements Query { |
||||
|
|
||||
|
@ApiModelProperty("组织全路径") |
||||
|
private String orgPath; |
||||
|
@ApiModelProperty("菜单sid") |
||||
|
private String menuSid; |
||||
|
@ApiModelProperty("菜单url") |
||||
|
private String menuUrl; |
||||
|
@ApiModelProperty("用户sid") |
||||
|
private String userSid; |
||||
|
@ApiModelProperty("分公司") |
||||
|
private String company; // 使用组织名称
|
||||
|
@ApiModelProperty("申请人") |
||||
|
private String applyName; // 申请人
|
||||
|
private String billNo; |
||||
|
private String applyStartDate; |
||||
|
private String applyEndDate; |
||||
|
|
||||
|
} |
@ -0,0 +1,36 @@ |
|||||
|
package com.yxt.anrui.riskcenter.api.loantransferpaymentapply; |
||||
|
|
||||
|
import com.yxt.common.core.vo.Vo; |
||||
|
import io.swagger.annotations.ApiModelProperty; |
||||
|
import lombok.Data; |
||||
|
|
||||
|
/** |
||||
|
* @author Administrator |
||||
|
* @description |
||||
|
* @date 2023/11/13 13:32 |
||||
|
*/ |
||||
|
@Data |
||||
|
public class LoanTransferPaymentApplyVo implements Vo { |
||||
|
|
||||
|
private String sid; |
||||
|
@ApiModelProperty("流程状态") |
||||
|
private String nodeState; // 流程状态
|
||||
|
@ApiModelProperty("分公司") |
||||
|
private String company; // 使用组织名称
|
||||
|
@ApiModelProperty("申请人") |
||||
|
private String applyName; // 申请人
|
||||
|
@ApiModelProperty("申请部门") |
||||
|
private String dept; |
||||
|
@ApiModelProperty("申请日期") |
||||
|
private String applyDate; // 申请日期
|
||||
|
@ApiModelProperty("申请编号") |
||||
|
private String billNo; |
||||
|
@ApiModelProperty("流程定义的id") |
||||
|
private String procDefId; // 流程定义的id
|
||||
|
@ApiModelProperty("流程实例的sid") |
||||
|
private String procInstId; // 流程实例的sid
|
||||
|
@ApiModelProperty("备注") |
||||
|
private String remarks; // 备注
|
||||
|
|
||||
|
|
||||
|
} |
@ -0,0 +1,13 @@ |
|||||
|
package com.yxt.anrui.riskcenter.api.loantransferpaymentapply; |
||||
|
|
||||
|
import lombok.Data; |
||||
|
|
||||
|
/** |
||||
|
* @author Fan |
||||
|
* @description |
||||
|
* @date 2023/12/6 10:21 |
||||
|
*/ |
||||
|
@Data |
||||
|
public class LoanTransferPaymentRecordVo { |
||||
|
|
||||
|
} |
@ -0,0 +1,25 @@ |
|||||
|
package com.yxt.anrui.riskcenter.api.loantransferpaymentrecord; |
||||
|
|
||||
|
import com.baomidou.mybatisplus.annotation.TableName; |
||||
|
import com.yxt.common.core.domain.BaseEntity; |
||||
|
import io.swagger.annotations.ApiModel; |
||||
|
import io.swagger.annotations.ApiModelProperty; |
||||
|
import lombok.Data; |
||||
|
|
||||
|
/** |
||||
|
* @author liupopo |
||||
|
* @version 1.0 |
||||
|
* @since 1.0 |
||||
|
*/ |
||||
|
@ApiModel(value = "代收代付申请记录", description = "代收代付申请记录") |
||||
|
@TableName("loan_transfer_payment_record") |
||||
|
@Data |
||||
|
public class LoanTransferPaymentRecord extends BaseEntity { |
||||
|
private static final long serialVersionUID = 1L; |
||||
|
|
||||
|
@ApiModelProperty("申请表sid") |
||||
|
private String mainSid; |
||||
|
@ApiModelProperty("还款记录sid") |
||||
|
private String repaymentHistorySid; |
||||
|
|
||||
|
} |
@ -0,0 +1,22 @@ |
|||||
|
package com.yxt.anrui.riskcenter.api.loantransferpaymentrecord; |
||||
|
|
||||
|
import io.swagger.annotations.Api; |
||||
|
import org.springframework.cloud.openfeign.FeignClient; |
||||
|
|
||||
|
|
||||
|
/** |
||||
|
* @author liupopo |
||||
|
* @version 1.0 |
||||
|
* @since 1.0 |
||||
|
*/ |
||||
|
@Api(tags = "代收代付记录") |
||||
|
@FeignClient( |
||||
|
contextId = "anrui-riskcenter-LoanTransferPaymentRecord", |
||||
|
name = "anrui-riskcenter", |
||||
|
path = "v1/loantransferpaymentrecord", |
||||
|
fallback = LoanTransferPaymentRecordFeignFallback.class) |
||||
|
public interface LoanTransferPaymentRecordFeign { |
||||
|
|
||||
|
|
||||
|
|
||||
|
} |
@ -0,0 +1,11 @@ |
|||||
|
package com.yxt.anrui.riskcenter.api.loantransferpaymentrecord; |
||||
|
|
||||
|
|
||||
|
import org.springframework.stereotype.Component; |
||||
|
|
||||
|
|
||||
|
@Component |
||||
|
public class LoanTransferPaymentRecordFeignFallback implements LoanTransferPaymentRecordFeign { |
||||
|
|
||||
|
|
||||
|
} |
@ -0,0 +1,20 @@ |
|||||
|
package com.yxt.anrui.riskcenter.biz.loantransferpaymentapply; |
||||
|
|
||||
|
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
||||
|
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||
|
import com.baomidou.mybatisplus.core.toolkit.Constants; |
||||
|
import com.yxt.anrui.riskcenter.api.loanbuckleapply.LoanBuckleApply; |
||||
|
import com.yxt.anrui.riskcenter.api.loanbuckleapply.LoanBuckleApplyVo; |
||||
|
import com.yxt.anrui.riskcenter.api.loantransferpaymentapply.LoanTransferPaymentApply; |
||||
|
import org.apache.ibatis.annotations.Mapper; |
||||
|
import org.apache.ibatis.annotations.Param; |
||||
|
|
||||
|
import java.util.Map; |
||||
|
|
||||
|
|
||||
|
@Mapper |
||||
|
public interface LoanTransferPaymentApplyMapper extends BaseMapper<LoanTransferPaymentApply> { |
||||
|
|
||||
|
|
||||
|
} |
@ -0,0 +1,5 @@ |
|||||
|
<?xml version="1.0" encoding="UTF-8" ?> |
||||
|
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
||||
|
<mapper namespace="com.yxt.anrui.riskcenter.biz.loantransferpaymentapply.LoanTransferPaymentApplyMapper"> |
||||
|
|
||||
|
</mapper> |
@ -0,0 +1,28 @@ |
|||||
|
package com.yxt.anrui.riskcenter.biz.loantransferpaymentapply; |
||||
|
|
||||
|
import cn.hutool.core.bean.BeanUtil; |
||||
|
import com.yxt.anrui.flowable.api.utils.ProcDefEnum; |
||||
|
import com.yxt.anrui.flowable.sqloperationsymbol.BusinessVariables; |
||||
|
import com.yxt.anrui.riskcenter.api.loanbuckleapply.*; |
||||
|
import com.yxt.anrui.riskcenter.api.loanbuckleapply.app.AppBuckleDetailsVo; |
||||
|
import com.yxt.anrui.riskcenter.api.loanbuckleapply.flow.*; |
||||
|
import com.yxt.anrui.riskcenter.api.loantransferpaymentapply.LoanTransferPaymentApplyFeign; |
||||
|
import com.yxt.common.core.query.PagerQuery; |
||||
|
import com.yxt.common.core.result.ResultBean; |
||||
|
import com.yxt.common.core.vo.PagerVo; |
||||
|
import io.swagger.annotations.Api; |
||||
|
import org.springframework.beans.factory.annotation.Autowired; |
||||
|
import org.springframework.web.bind.annotation.RequestMapping; |
||||
|
import org.springframework.web.bind.annotation.RestController; |
||||
|
|
||||
|
import java.util.List; |
||||
|
|
||||
|
|
||||
|
@Api(tags = "代收代付申请") |
||||
|
@RestController |
||||
|
@RequestMapping("v1/loantransferpaymentapply") |
||||
|
public class LoanTransferPaymentApplyRest implements LoanTransferPaymentApplyFeign { |
||||
|
|
||||
|
|
||||
|
|
||||
|
} |
@ -0,0 +1,70 @@ |
|||||
|
package com.yxt.anrui.riskcenter.biz.loantransferpaymentapply; |
||||
|
|
||||
|
import cn.hutool.core.bean.BeanUtil; |
||||
|
import cn.hutool.core.date.DateTime; |
||||
|
import com.alibaba.fastjson.JSON; |
||||
|
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||
|
import com.google.common.util.concurrent.ThreadFactoryBuilder; |
||||
|
import com.yxt.anrui.base.common.enums.BillTypeEnum; |
||||
|
import com.yxt.anrui.base.common.utils.Rule; |
||||
|
import com.yxt.anrui.base.common.utils.domain.BillNo; |
||||
|
import com.yxt.anrui.flowable.api.flow.FlowableFeign; |
||||
|
import com.yxt.anrui.flowable.api.flow.UpdateFlowFieldVo; |
||||
|
import com.yxt.anrui.flowable.api.flow2.FlowDelegateQuery; |
||||
|
import com.yxt.anrui.flowable.api.flow2.FlowFeign; |
||||
|
import com.yxt.anrui.flowable.api.flowtask.FlowTaskFeign; |
||||
|
import com.yxt.anrui.flowable.api.flowtask.FlowTaskVo; |
||||
|
import com.yxt.anrui.flowable.api.flowtask.LatestTaskVo; |
||||
|
import com.yxt.anrui.flowable.api.utils.ProcDefEnum; |
||||
|
import com.yxt.anrui.flowable.sqloperationsymbol.BusinessVariables; |
||||
|
import com.yxt.anrui.portal.api.sysorganization.SysOrganizationFeign; |
||||
|
import com.yxt.anrui.portal.api.sysorganization.SysOrganizationVo; |
||||
|
import com.yxt.anrui.portal.api.sysstafforg.SysStaffOrg; |
||||
|
import com.yxt.anrui.portal.api.sysstafforg.SysStaffOrgFeign; |
||||
|
import com.yxt.anrui.portal.api.sysuser.PrivilegeQuery; |
||||
|
import com.yxt.anrui.portal.api.sysuser.SysUserFeign; |
||||
|
import com.yxt.anrui.portal.api.sysuser.SysUserVo; |
||||
|
import com.yxt.anrui.riskcenter.api.loanbuckleapply.*; |
||||
|
import com.yxt.anrui.riskcenter.api.loanbuckleapply.app.AppBuckleDetailsVo; |
||||
|
import com.yxt.anrui.riskcenter.api.loanbuckleapply.app.AppRecordVo; |
||||
|
import com.yxt.anrui.riskcenter.api.loanbuckleapply.flow.*; |
||||
|
import com.yxt.anrui.riskcenter.api.loanbuckleapplyrecord.LoanBuckleApplyRecord; |
||||
|
import com.yxt.anrui.riskcenter.api.loanfile.LoanFile; |
||||
|
import com.yxt.anrui.riskcenter.api.loanfile.LoanFileEnum; |
||||
|
import com.yxt.anrui.riskcenter.api.loanrepaymenthistory.LoanRepaymentHistory; |
||||
|
import com.yxt.anrui.riskcenter.api.loanrepaymenthistory.LoanRepaymentHistoryRecordVo; |
||||
|
import com.yxt.anrui.riskcenter.api.loantransferpaymentapply.LoanTransferPaymentApply; |
||||
|
import com.yxt.anrui.riskcenter.biz.loanbuckleapplyrecord.LoanBuckleApplyRecordService; |
||||
|
import com.yxt.anrui.riskcenter.biz.loanfile.LoanFileService; |
||||
|
import com.yxt.anrui.riskcenter.biz.loanrepaymenthistory.LoanRepaymentHistoryService; |
||||
|
import com.yxt.common.base.config.component.FileUploadComponent; |
||||
|
import com.yxt.common.base.service.MybatisBaseService; |
||||
|
import com.yxt.common.base.utils.PagerUtil; |
||||
|
import com.yxt.common.core.query.PagerQuery; |
||||
|
import com.yxt.common.core.result.ResultBean; |
||||
|
import com.yxt.common.core.vo.PagerVo; |
||||
|
import com.yxt.messagecenter.api.message.MessageFeign; |
||||
|
import com.yxt.messagecenter.api.message.MessageFlowVo; |
||||
|
import com.yxt.messagecenter.api.message.MessageFlowableQuery; |
||||
|
import org.apache.commons.lang3.StringUtils; |
||||
|
import org.springframework.beans.factory.annotation.Autowired; |
||||
|
import org.springframework.stereotype.Service; |
||||
|
import org.springframework.transaction.annotation.Transactional; |
||||
|
|
||||
|
import java.text.SimpleDateFormat; |
||||
|
import java.util.*; |
||||
|
import java.util.concurrent.*; |
||||
|
import java.util.stream.Collectors; |
||||
|
|
||||
|
/** |
||||
|
* @description: |
||||
|
* @author: fzz |
||||
|
* @date: 2023/7/6 |
||||
|
**/ |
||||
|
@Service |
||||
|
public class LoanTransferPaymentApplyService extends MybatisBaseService<LoanTransferPaymentApplyMapper, LoanTransferPaymentApply> { |
||||
|
|
||||
|
|
||||
|
|
||||
|
} |
@ -0,0 +1,16 @@ |
|||||
|
package com.yxt.anrui.riskcenter.biz.loantransferpaymentrecord; |
||||
|
|
||||
|
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
||||
|
import com.yxt.anrui.riskcenter.api.loanbuckleapplyrecord.LoanBuckleApplyRecord; |
||||
|
import com.yxt.anrui.riskcenter.api.loantransferpaymentrecord.LoanTransferPaymentRecord; |
||||
|
import org.apache.ibatis.annotations.Mapper; |
||||
|
import org.apache.ibatis.annotations.Param; |
||||
|
|
||||
|
import java.util.List; |
||||
|
|
||||
|
|
||||
|
@Mapper |
||||
|
public interface LoanTransferPaymentRecordMapper extends BaseMapper<LoanTransferPaymentRecord> { |
||||
|
|
||||
|
|
||||
|
} |
@ -0,0 +1,6 @@ |
|||||
|
<?xml version="1.0" encoding="UTF-8" ?> |
||||
|
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
||||
|
<mapper namespace="com.yxt.anrui.riskcenter.biz.loantransferpaymentrecord.LoanTransferPaymentRecordMapper"> |
||||
|
|
||||
|
|
||||
|
</mapper> |
@ -0,0 +1,16 @@ |
|||||
|
package com.yxt.anrui.riskcenter.biz.loantransferpaymentrecord; |
||||
|
|
||||
|
import com.yxt.anrui.riskcenter.api.loanbuckleapplyrecord.LoanBuckleApplyRecordFeign; |
||||
|
import com.yxt.anrui.riskcenter.api.loantransferpaymentrecord.LoanTransferPaymentRecordFeign; |
||||
|
import io.swagger.annotations.Api; |
||||
|
import org.springframework.web.bind.annotation.RequestMapping; |
||||
|
import org.springframework.web.bind.annotation.RestController; |
||||
|
|
||||
|
|
||||
|
@Api(tags = "代收代付记录") |
||||
|
@RestController |
||||
|
@RequestMapping("v1/loantransferpaymentrecord") |
||||
|
public class LoanTransferPaymentRecordRest implements LoanTransferPaymentRecordFeign { |
||||
|
|
||||
|
|
||||
|
} |
@ -0,0 +1,19 @@ |
|||||
|
package com.yxt.anrui.riskcenter.biz.loantransferpaymentrecord; |
||||
|
|
||||
|
|
||||
|
import com.yxt.anrui.riskcenter.api.loantransferpaymentrecord.LoanTransferPaymentRecord; |
||||
|
import com.yxt.common.base.service.MybatisBaseService; |
||||
|
import org.springframework.stereotype.Service; |
||||
|
|
||||
|
import java.util.List; |
||||
|
|
||||
|
/** |
||||
|
* @description: |
||||
|
* @author: fzz |
||||
|
* @date: 2023/7/6 |
||||
|
**/ |
||||
|
@Service |
||||
|
public class LoanTransferPaymentRecordService extends MybatisBaseService<LoanTransferPaymentRecordMapper, LoanTransferPaymentRecord> { |
||||
|
|
||||
|
|
||||
|
} |
Loading…
Reference in new issue