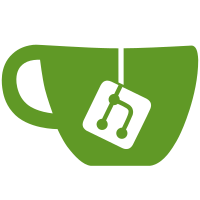
10 changed files with 713 additions and 0 deletions
@ -0,0 +1,152 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.reportcenter.api.asmonthfittingsinventoryreport; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.math.BigDecimal; |
|||
|
|||
/** |
|||
* Project: baobiao(baobiao) <br/> |
|||
* File: AsMonthFittingsInventoryReport.java <br/> |
|||
* Class: com.yxt.anrui.reportcenter.api.asmonthfittingsinventoryreport.AsMonthFittingsInventoryReport <br/> |
|||
* Description: 月度配件库存管理指标完成情况报表. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2025-03-05 14:43:41 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "月度配件库存管理指标完成情况报表", description = "月度配件库存管理指标完成情况报表") |
|||
@TableName("as_month_fittings_inventory_report") |
|||
public class AsMonthFittingsInventoryReport extends BaseEntity { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty("生成年月(yyyy-MM)") |
|||
private String createDate; // 生成年月(yyyy-MM)
|
|||
@ApiModelProperty("事业部sid") |
|||
private String busOrgSid; // 事业部sid
|
|||
@ApiModelProperty("事业部名称") |
|||
private String busOrgName; // 事业部名称
|
|||
@ApiModelProperty("分公司sid") |
|||
private String createOrgSid; // 分公司sid
|
|||
@ApiModelProperty("分公司名称") |
|||
private String createOrgName; // 分公司名称
|
|||
@ApiModelProperty("部门(服务站)sid") |
|||
private String useOrgSid; // 部门(服务站)sid
|
|||
@ApiModelProperty("部门(服务站)") |
|||
private String useOrgName; // 部门(服务站)
|
|||
@ApiModelProperty("品牌sid") |
|||
private String brandSid; // 品牌sid
|
|||
@ApiModelProperty("品牌名") |
|||
private String brandName; // 品牌名
|
|||
@ApiModelProperty("品名(区域)") |
|||
private String zoneName; // 品名(区域)
|
|||
@ApiModelProperty("项目(仓库)") |
|||
private String houseName; // 项目(仓库)
|
|||
@ApiModelProperty("在修车辆") |
|||
private BigDecimal repairVehAmount; // 在修车辆
|
|||
@ApiModelProperty("品种(个)-库存总计") |
|||
private BigDecimal fittingsVarietyTotal; // 品种(个)-库存总计
|
|||
@ApiModelProperty("金额(元)-库存总计") |
|||
private BigDecimal fittingsAmountTotal; // 金额(元)-库存总计
|
|||
@ApiModelProperty("品种(个)-库龄1-60天") |
|||
private BigDecimal fittingsVarietyOne; // 品种(个)-库龄1-60天
|
|||
@ApiModelProperty("金额(元)-库龄1-60天") |
|||
private BigDecimal fittingsAmountOne; // 金额(元)-库龄1-60天
|
|||
@ApiModelProperty("占比-库龄1-60天") |
|||
private BigDecimal fittingsPercentOne; // 占比-库龄1-60天
|
|||
@ApiModelProperty("品种(个)-库龄61-90天") |
|||
private BigDecimal fittingsVarietyTwo; // 品种(个)-库龄61-90天
|
|||
@ApiModelProperty("金额(元)-库龄61-90天") |
|||
private BigDecimal fittingsAmountTwo; // 金额(元)-库龄61-90天
|
|||
@ApiModelProperty("占比-库龄61-90天") |
|||
private BigDecimal fittingsPercentTwo; // 占比-库龄61-90天
|
|||
@ApiModelProperty("品种(个)-库龄91-180天") |
|||
private BigDecimal fittingsVarietyThree; // 品种(个)-库龄91-180天
|
|||
@ApiModelProperty("金额(元)-库龄91-180天") |
|||
private BigDecimal fittingsAmountThree; // 金额(元)-库龄91-180天
|
|||
@ApiModelProperty("占比-库龄91-180天") |
|||
private BigDecimal fittingsPercentThree; // 占比-库龄91-180天
|
|||
@ApiModelProperty("品种(个)-库龄150-180天") |
|||
private BigDecimal fittingsVarietyFour; // 品种(个)-库龄150-180天
|
|||
@ApiModelProperty("金额(元)-库龄150-180天") |
|||
private BigDecimal fittingsAmountFour; // 金额(元)-库龄150-180天
|
|||
@ApiModelProperty("占比-库龄150-180天") |
|||
private BigDecimal fittingsPercentFour; // 占比-库龄150-180天
|
|||
@ApiModelProperty("品种(个)-库龄181-270天") |
|||
private BigDecimal fittingsVarietyFive; // 品种(个)-库龄181-270天
|
|||
@ApiModelProperty("金额(元)-库龄181-270天") |
|||
private BigDecimal fittingsAmountFive; // 金额(元)-库龄181-270天
|
|||
@ApiModelProperty("占比-库龄181-270天") |
|||
private BigDecimal fittingsPercentFive; // 占比-库龄181-270天
|
|||
@ApiModelProperty("品种(个)-库龄240-270天") |
|||
private BigDecimal fittingsVarietySix; // 品种(个)-库龄240-270天
|
|||
@ApiModelProperty("金额(元)-库龄240-270天") |
|||
private BigDecimal fittingsAmountSix; // 金额(元)-库龄240-270天
|
|||
@ApiModelProperty("占比-库龄240-270天") |
|||
private BigDecimal fittingsPercentSix; // 占比-库龄240-270天
|
|||
@ApiModelProperty("品种(个)-库龄271-360天") |
|||
private BigDecimal fittingsVarietySeven; // 品种(个)-库龄271-360天
|
|||
@ApiModelProperty("金额(元)-库龄271-360天") |
|||
private BigDecimal fittingsAmountSeven; // 金额(元)-库龄271-360天
|
|||
@ApiModelProperty("占比-库龄271-360天") |
|||
private BigDecimal fittingsPercentSeven; // 占比-库龄271-360天
|
|||
@ApiModelProperty("品种(个)-库龄330-360天") |
|||
private BigDecimal fittingsVarietyEight; // 品种(个)-库龄330-360天
|
|||
@ApiModelProperty("金额(元)-库龄330-360天") |
|||
private BigDecimal fittingsAmountEight; // 金额(元)-库龄330-360天
|
|||
@ApiModelProperty("占比-库龄330-360天") |
|||
private BigDecimal fittingsPercentEight; // 占比-库龄330-360天
|
|||
@ApiModelProperty("品种(个)-库龄361-720天") |
|||
private BigDecimal fittingsVarietyNine; // 品种(个)-库龄361-720天
|
|||
@ApiModelProperty("金额(元)-库龄361-720天") |
|||
private BigDecimal fittingsAmountNine; // 金额(元)-库龄361-720天
|
|||
@ApiModelProperty("占比-库龄361-720天") |
|||
private BigDecimal fittingsPercentNine; // 占比-库龄361-720天
|
|||
@ApiModelProperty("品种(个)-库龄720天以上") |
|||
private BigDecimal fittingsVarietyTen; // 品种(个)-库龄720天以上
|
|||
@ApiModelProperty("金额(元)-库龄720天以上") |
|||
private BigDecimal fittingsAmountTen; // 金额(元)-库龄720天以上
|
|||
@ApiModelProperty("占比-库龄720天以上") |
|||
private BigDecimal fittingsPercentTen; // 占比-库龄720天以上
|
|||
@ApiModelProperty("当月出库配件成本-金额") |
|||
private BigDecimal fittingsOutAmount; // 当月出库配件成本-金额
|
|||
@ApiModelProperty("当月出库配件成本-占比") |
|||
private BigDecimal fittingsOutPercent; // 当月出库配件成本-占比
|
|||
@ApiModelProperty("当月入库配件成本-金额") |
|||
private BigDecimal fittingsInAmount; // 当月入库配件成本-金额
|
|||
@ApiModelProperty("当月入库配件成本-占比") |
|||
private BigDecimal fittingsInPercent; // 当月入库配件成本-占比
|
|||
|
|||
} |
@ -0,0 +1,74 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.reportcenter.api.asmonthfittingsinventoryreport; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* Project: baobiao(baobiao) <br/> |
|||
* File: AsMonthFittingsInventoryReportFeign.java <br/> |
|||
* Class: com.yxt.anrui.reportcenter.api.asmonthfittingsinventoryreport.AsMonthFittingsInventoryReportFeign <br/> |
|||
* Description: 月度配件库存管理指标完成情况报表. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2025-03-05 14:43:41 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Api(tags = "月度配件库存管理指标完成情况报表") |
|||
@FeignClient( |
|||
contextId = "baobiao-AsMonthFittingsInventoryReport", |
|||
name = "baobiao", |
|||
path = "v1/MonthFittingsReport", |
|||
fallback = AsMonthFittingsInventoryReportFeignFallback.class) |
|||
public interface AsMonthFittingsInventoryReportFeign { |
|||
|
|||
|
|||
@ApiOperation("月度配件库存管理指标完成情况列表") |
|||
@PostMapping("/monthFittingsList") |
|||
@ResponseBody |
|||
public ResultBean<PagerVo<MonthFittingsReportListVo>> monthFittingsList(@RequestBody PagerQuery<MonthFittingsReportListQuery> pq); |
|||
|
|||
@ApiOperation("月度配件库存管理指标完成情况列表明细") |
|||
@PostMapping("/monthFittingsListDetails") |
|||
@ResponseBody |
|||
public ResultBean<List<AsMonthFittingsInventoryReportVo>> monthFittingsListDetails(@RequestParam("createDate") String createDate, |
|||
@RequestParam("useOrgSid") String useOrgSid |
|||
); |
|||
|
|||
|
|||
|
|||
} |
@ -0,0 +1,64 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.reportcenter.api.asmonthfittingsinventoryreport; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import org.springframework.stereotype.Component; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* Project: baobiao(baobiao) <br/> |
|||
* File: AsMonthFittingsInventoryReportFeignFallback.java <br/> |
|||
* Class: com.yxt.anrui.reportcenter.api.asmonthfittingsinventoryreport.AsMonthFittingsInventoryReportFeignFallback <br/> |
|||
* Description: 月度配件库存管理指标完成情况报表. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2025-03-05 14:43:41 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Component |
|||
public class AsMonthFittingsInventoryReportFeignFallback implements AsMonthFittingsInventoryReportFeign { |
|||
|
|||
|
|||
|
|||
@Override |
|||
public ResultBean<PagerVo<MonthFittingsReportListVo>> monthFittingsList(PagerQuery<MonthFittingsReportListQuery> pq) { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<AsMonthFittingsInventoryReportVo>> monthFittingsListDetails(String createDate, String useOrgSid) { |
|||
return null; |
|||
} |
|||
|
|||
|
|||
} |
@ -0,0 +1,136 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.reportcenter.api.asmonthfittingsinventoryreport; |
|||
|
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.math.BigDecimal; |
|||
|
|||
/** |
|||
* Project: baobiao(baobiao) <br/> |
|||
* File: AsMonthFittingsInventoryReportVo.java <br/> |
|||
* Class: com.yxt.anrui.reportcenter.api.asmonthfittingsinventoryreport.AsMonthFittingsInventoryReportVo <br/> |
|||
* Description: 月度配件库存管理指标完成情况报表 视图数据对象. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2025-03-05 14:43:41 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "月度配件库存管理指标完成情况报表 视图数据对象", description = "月度配件库存管理指标完成情况报表 视图数据对象") |
|||
public class AsMonthFittingsInventoryReportVo implements Vo { |
|||
|
|||
@ApiModelProperty("生成年月(yyyy-MM)") |
|||
private String createDate; // 生成年月(yyyy-MM)
|
|||
@ApiModelProperty("品牌名") |
|||
private String brandName; // 品牌名
|
|||
@ApiModelProperty("品名(区域)") |
|||
private String zoneName; // 品名(区域)
|
|||
@ApiModelProperty("项目(仓库)") |
|||
private String houseName; // 项目(仓库)
|
|||
@ApiModelProperty("在修车辆") |
|||
private String repairVehAmount; // 在修车辆
|
|||
@ApiModelProperty("品种(个)-库存总计") |
|||
private String fittingsVarietyTotal; // 品种(个)-库存总计
|
|||
@ApiModelProperty("金额(元)-库存总计") |
|||
private String fittingsAmountTotal; // 金额(元)-库存总计
|
|||
@ApiModelProperty("品种(个)-库龄1-60天") |
|||
private String fittingsVarietyOne; // 品种(个)-库龄1-60天
|
|||
@ApiModelProperty("金额(元)-库龄1-60天") |
|||
private String fittingsAmountOne; // 金额(元)-库龄1-60天
|
|||
@ApiModelProperty("占比-库龄1-60天") |
|||
private String fittingsPercentOne; // 占比-库龄1-60天
|
|||
@ApiModelProperty("品种(个)-库龄61-90天") |
|||
private String fittingsVarietyTwo; // 品种(个)-库龄61-90天
|
|||
@ApiModelProperty("金额(元)-库龄61-90天") |
|||
private String fittingsAmountTwo; // 金额(元)-库龄61-90天
|
|||
@ApiModelProperty("占比-库龄61-90天") |
|||
private String fittingsPercentTwo; // 占比-库龄61-90天
|
|||
@ApiModelProperty("品种(个)-库龄91-180天") |
|||
private String fittingsVarietyThree; // 品种(个)-库龄91-180天
|
|||
@ApiModelProperty("金额(元)-库龄91-180天") |
|||
private String fittingsAmountThree; // 金额(元)-库龄91-180天
|
|||
@ApiModelProperty("占比-库龄91-180天") |
|||
private String fittingsPercentThree; // 占比-库龄91-180天
|
|||
@ApiModelProperty("品种(个)-库龄150-180天") |
|||
private String fittingsVarietyFour; // 品种(个)-库龄150-180天
|
|||
@ApiModelProperty("金额(元)-库龄150-180天") |
|||
private String fittingsAmountFour; // 金额(元)-库龄150-180天
|
|||
@ApiModelProperty("占比-库龄150-180天") |
|||
private String fittingsPercentFour; // 占比-库龄150-180天
|
|||
@ApiModelProperty("品种(个)-库龄181-270天") |
|||
private String fittingsVarietyFive; // 品种(个)-库龄181-270天
|
|||
@ApiModelProperty("金额(元)-库龄181-270天") |
|||
private String fittingsAmountFive; // 金额(元)-库龄181-270天
|
|||
@ApiModelProperty("占比-库龄181-270天") |
|||
private String fittingsPercentFive; // 占比-库龄181-270天
|
|||
@ApiModelProperty("品种(个)-库龄240-270天") |
|||
private String fittingsVarietySix; // 品种(个)-库龄240-270天
|
|||
@ApiModelProperty("金额(元)-库龄240-270天") |
|||
private String fittingsAmountSix; // 金额(元)-库龄240-270天
|
|||
@ApiModelProperty("占比-库龄240-270天") |
|||
private String fittingsPercentSix; // 占比-库龄240-270天
|
|||
@ApiModelProperty("品种(个)-库龄271-360天") |
|||
private String fittingsVarietySeven; // 品种(个)-库龄271-360天
|
|||
@ApiModelProperty("金额(元)-库龄271-360天") |
|||
private String fittingsAmountSeven; // 金额(元)-库龄271-360天
|
|||
@ApiModelProperty("占比-库龄271-360天") |
|||
private String fittingsPercentSeven; // 占比-库龄271-360天
|
|||
@ApiModelProperty("品种(个)-库龄330-360天") |
|||
private String fittingsVarietyEight; // 品种(个)-库龄330-360天
|
|||
@ApiModelProperty("金额(元)-库龄330-360天") |
|||
private String fittingsAmountEight; // 金额(元)-库龄330-360天
|
|||
@ApiModelProperty("占比-库龄330-360天") |
|||
private String fittingsPercentEight; // 占比-库龄330-360天
|
|||
@ApiModelProperty("品种(个)-库龄361-720天") |
|||
private String fittingsVarietyNine; // 品种(个)-库龄361-720天
|
|||
@ApiModelProperty("金额(元)-库龄361-720天") |
|||
private String fittingsAmountNine; // 金额(元)-库龄361-720天
|
|||
@ApiModelProperty("占比-库龄361-720天") |
|||
private String fittingsPercentNine; // 占比-库龄361-720天
|
|||
@ApiModelProperty("品种(个)-库龄720天以上") |
|||
private String fittingsVarietyTen; // 品种(个)-库龄720天以上
|
|||
@ApiModelProperty("金额(元)-库龄720天以上") |
|||
private String fittingsAmountTen; // 金额(元)-库龄720天以上
|
|||
@ApiModelProperty("占比-库龄720天以上") |
|||
private String fittingsPercentTen; // 占比-库龄720天以上
|
|||
@ApiModelProperty("当月出库配件成本-金额") |
|||
private String fittingsOutAmount; // 当月出库配件成本-金额
|
|||
@ApiModelProperty("当月出库配件成本-占比") |
|||
private String fittingsOutPercent; // 当月出库配件成本-占比
|
|||
@ApiModelProperty("当月入库配件成本-金额") |
|||
private String fittingsInAmount; // 当月入库配件成本-金额
|
|||
@ApiModelProperty("当月入库配件成本-占比") |
|||
private String fittingsInPercent; // 当月入库配件成本-占比
|
|||
|
|||
} |
@ -0,0 +1,14 @@ |
|||
package com.yxt.anrui.reportcenter.api.asmonthfittingsinventoryreport; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import lombok.Data; |
|||
|
|||
@Data |
|||
public class MonthFittingsReportListQuery implements Query { |
|||
|
|||
private String startDate; |
|||
private String endDate; |
|||
private String useOrgName; |
|||
|
|||
|
|||
} |
@ -0,0 +1,12 @@ |
|||
package com.yxt.anrui.reportcenter.api.asmonthfittingsinventoryreport; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import lombok.Data; |
|||
|
|||
@Data |
|||
public class MonthFittingsReportListVo implements Vo { |
|||
|
|||
private String createDate; |
|||
private String useOrgName; |
|||
private String useOrgSid; |
|||
} |
@ -0,0 +1,71 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.reportcenter.biz.asmonthfittingsinventoryreport; |
|||
|
|||
import com.baomidou.mybatisplus.core.conditions.Wrapper; |
|||
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.baomidou.mybatisplus.core.toolkit.Constants; |
|||
import com.yxt.anrui.reportcenter.api.asmonthfittingsinventoryreport.MonthFittingsReportListVo; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
import org.apache.ibatis.annotations.Param; |
|||
import org.apache.ibatis.annotations.Select; |
|||
import com.yxt.anrui.reportcenter.api.asmonthfittingsinventoryreport.AsMonthFittingsInventoryReport; |
|||
import com.yxt.anrui.reportcenter.api.asmonthfittingsinventoryreport.AsMonthFittingsInventoryReportVo; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* Project: baobiao(baobiao) <br/> |
|||
* File: AsMonthFittingsInventoryReportMapper.java <br/> |
|||
* Class: com.yxt.anrui.reportcenter.biz.asmonthfittingsinventoryreport.AsMonthFittingsInventoryReportMapper <br/> |
|||
* Description: 月度配件库存管理指标完成情况报表. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2025-03-05 14:43:41 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Mapper |
|||
public interface AsMonthFittingsInventoryReportMapper extends BaseMapper<AsMonthFittingsInventoryReport> { |
|||
|
|||
//@Update("update as_month_fittings_inventory_report set name=#{msg} where id=#{id}")
|
|||
//IPage<AsMonthFittingsInventoryReportVo> voPage(IPage<AsMonthFittingsInventoryReport> page, @Param(Constants.WRAPPER) QueryWrapper<AsMonthFittingsInventoryReport> qw);
|
|||
|
|||
IPage<AsMonthFittingsInventoryReportVo> selectPageVo(IPage<AsMonthFittingsInventoryReport> page, @Param(Constants.WRAPPER) Wrapper<AsMonthFittingsInventoryReport> qw); |
|||
|
|||
List<AsMonthFittingsInventoryReportVo> selectListAllVo(@Param(Constants.WRAPPER) Wrapper<AsMonthFittingsInventoryReport> qw); |
|||
|
|||
@Select("select * from as_month_fittings_inventory_report") |
|||
List<AsMonthFittingsInventoryReportVo> selectListVo(); |
|||
|
|||
IPage<MonthFittingsReportListVo> monthFittingsList(IPage<AsMonthFittingsInventoryReport> page, @Param(Constants.WRAPPER) QueryWrapper<AsMonthFittingsInventoryReport> qw); |
|||
|
|||
List<AsMonthFittingsInventoryReportVo> monthFittingsListDetails(@Param(Constants.WRAPPER)QueryWrapper<AsMonthFittingsInventoryReport> qw); |
|||
} |
@ -0,0 +1,28 @@ |
|||
<?xml version="1.0" encoding="UTF-8" ?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.anrui.reportcenter.biz.asmonthfittingsinventoryreport.AsMonthFittingsInventoryReportMapper"> |
|||
<!-- <where> ${ew.sqlSegment} </where>--> |
|||
<!-- ${ew.customSqlSegment} --> |
|||
<select id="selectPageVo" resultType="com.yxt.anrui.reportcenter.api.asmonthfittingsinventoryreport.AsMonthFittingsInventoryReportVo"> |
|||
SELECT * FROM as_month_fittings_inventory_report <where> ${ew.sqlSegment} </where> |
|||
</select> |
|||
|
|||
<select id="selectListAllVo" resultType="com.yxt.anrui.reportcenter.api.asmonthfittingsinventoryreport.AsMonthFittingsInventoryReportVo"> |
|||
SELECT * FROM as_month_fittings_inventory_report <where> ${ew.sqlSegment} </where> |
|||
</select> |
|||
<select id="monthFittingsList" |
|||
resultType="com.yxt.anrui.reportcenter.api.asmonthfittingsinventoryreport.MonthFittingsReportListVo"> |
|||
SELECT |
|||
createDate, |
|||
useOrgSid, |
|||
useOrgName |
|||
FROM |
|||
as_month_fittings_inventory_report AS mf |
|||
<where> ${ew.sqlSegment} </where> |
|||
GROUP BY mf.createDate,mf.useOrgSid |
|||
</select> |
|||
<select id="monthFittingsListDetails" |
|||
resultType="com.yxt.anrui.reportcenter.api.asmonthfittingsinventoryreport.AsMonthFittingsInventoryReportVo"> |
|||
SELECT * FROM as_month_fittings_inventory_report <where> ${ew.sqlSegment} </where> |
|||
</select> |
|||
</mapper> |
@ -0,0 +1,77 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.reportcenter.biz.asmonthfittingsinventoryreport; |
|||
|
|||
import com.yxt.anrui.reportcenter.api.asmonthfittingsinventoryreport.AsMonthFittingsInventoryReportFeign; |
|||
import com.yxt.anrui.reportcenter.api.asmonthfittingsinventoryreport.AsMonthFittingsInventoryReportVo; |
|||
import com.yxt.anrui.reportcenter.api.asmonthfittingsinventoryreport.MonthFittingsReportListQuery; |
|||
import com.yxt.anrui.reportcenter.api.asmonthfittingsinventoryreport.MonthFittingsReportListVo; |
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import io.swagger.annotations.Api; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.web.bind.annotation.RequestMapping; |
|||
import org.springframework.web.bind.annotation.RestController; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* Project: baobiao(baobiao) <br/> |
|||
* File: AsMonthFittingsInventoryReportFeignFallback.java <br/> |
|||
* Class: com.yxt.anrui.reportcenter.biz.asmonthfittingsinventoryreport.AsMonthFittingsInventoryReportRest <br/> |
|||
* Description: 月度配件库存管理指标完成情况报表. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2025-03-05 14:43:41 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Api(tags = "月度配件库存管理指标完成情况报表") |
|||
@RestController("com.yxt.anrui.reportcenter.biz.asmonthfittingsinventoryreport.AsMonthFittingsInventoryReportRest") |
|||
@RequestMapping("v1/MonthFittingsReport") |
|||
public class AsMonthFittingsInventoryReportRest implements AsMonthFittingsInventoryReportFeign { |
|||
|
|||
@Autowired |
|||
private AsMonthFittingsInventoryReportService asMonthFittingsInventoryReportService; |
|||
|
|||
@Override |
|||
public ResultBean<PagerVo<MonthFittingsReportListVo>> monthFittingsList(PagerQuery<MonthFittingsReportListQuery> pq) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
PagerVo<MonthFittingsReportListVo> pv = asMonthFittingsInventoryReportService.monthFittingsList(pq); |
|||
return rb.success().setData(pv); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<AsMonthFittingsInventoryReportVo>> monthFittingsListDetails(String createDate, String useOrgSid) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
List<AsMonthFittingsInventoryReportVo> pv = asMonthFittingsInventoryReportService.monthFittingsListDetails(createDate,useOrgSid); |
|||
return rb.success().setData(pv); |
|||
} |
|||
|
|||
} |
@ -0,0 +1,85 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.reportcenter.biz.asmonthfittingsinventoryreport; |
|||
|
|||
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import com.yxt.anrui.reportcenter.api.asmonthfittingsinventoryreport.*; |
|||
import com.yxt.common.base.service.MybatisBaseService; |
|||
import com.yxt.common.base.utils.PagerUtil; |
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import org.apache.commons.lang3.StringUtils; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* Project: baobiao(baobiao) <br/> |
|||
* File: AsMonthFittingsInventoryReportService.java <br/> |
|||
* Class: com.yxt.anrui.reportcenter.biz.asmonthfittingsinventoryreport.AsMonthFittingsInventoryReportService <br/> |
|||
* Description: 月度配件库存管理指标完成情况报表 业务逻辑. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2025-03-05 14:43:41 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Service |
|||
public class AsMonthFittingsInventoryReportService extends MybatisBaseService<AsMonthFittingsInventoryReportMapper, AsMonthFittingsInventoryReport> { |
|||
|
|||
|
|||
public PagerVo<MonthFittingsReportListVo> monthFittingsList(PagerQuery<MonthFittingsReportListQuery> pq) { |
|||
MonthFittingsReportListQuery query = pq.getParams(); |
|||
QueryWrapper<AsMonthFittingsInventoryReport> qw = new QueryWrapper<>(); |
|||
//生成日期开始时间
|
|||
String createTimeStart = query.getStartDate(); |
|||
//生成日期结束时间
|
|||
String createTimeEnd = query.getEndDate(); |
|||
qw.apply(StringUtils.isNotEmpty(createTimeStart), "date_format (mf.createDate,'%Y-%m') >= date_format('" + createTimeStart + "','%Y-%m')"). |
|||
apply(StringUtils.isNotEmpty(createTimeEnd), "date_format (mf.createDate,'%Y-%m') <= date_format('" + createTimeEnd + "','%Y-%m')" |
|||
); |
|||
if (StringUtils.isNotBlank(query.getUseOrgName())) { |
|||
qw.like("mf.useOrgName", query.getUseOrgName()); |
|||
} |
|||
IPage<AsMonthFittingsInventoryReport> page = PagerUtil.queryToPage(pq); |
|||
IPage<MonthFittingsReportListVo> pagging = baseMapper.monthFittingsList(page, qw); |
|||
PagerVo<MonthFittingsReportListVo> p = PagerUtil.pageToVo(pagging, null); |
|||
return p; |
|||
} |
|||
|
|||
public List<AsMonthFittingsInventoryReportVo> monthFittingsListDetails(String createDate, String useOrgSid) { |
|||
QueryWrapper<AsMonthFittingsInventoryReport> qw = new QueryWrapper<>(); |
|||
qw.eq("createDate", createDate); |
|||
qw.eq("useOrgSid", useOrgSid); |
|||
List<AsMonthFittingsInventoryReportVo> list = baseMapper.monthFittingsListDetails(qw); |
|||
return list; |
|||
} |
|||
|
|||
|
|||
} |
Loading…
Reference in new issue