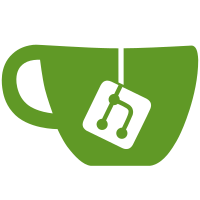
25 changed files with 1249 additions and 7 deletions
@ -0,0 +1,24 @@ |
|||
package com.yxt.anrui.portal.api.sysorganization; |
|||
|
|||
import lombok.Data; |
|||
|
|||
import java.util.Map; |
|||
|
|||
@Data |
|||
public class OrgDeptObjVo { |
|||
|
|||
|
|||
private String id; |
|||
private String dictValue; |
|||
|
|||
private Map<String, Object> extra; |
|||
|
|||
// 静态方法,直接通过方法创建对象并赋值
|
|||
public static OrgDeptObjVo of(String id, String dictValue) { |
|||
OrgDeptObjVo formCommon = new OrgDeptObjVo(); |
|||
formCommon.setId(id); |
|||
formCommon.setDictValue(dictValue); |
|||
return formCommon; |
|||
} |
|||
|
|||
} |
@ -0,0 +1,92 @@ |
|||
package com.yxt.anrui.oa.api; |
|||
|
|||
import com.yxt.anrui.oa.biz.adreimbursedassetapply.AdReimbursedAssetApplyDetailVo; |
|||
import com.yxt.anrui.oa.biz.adreimbursedassetapply.AdReimbursedAssetApplyDto; |
|||
import com.yxt.anrui.oa.biz.adreimbursedassetapply.AdReimbursedAssetApplyService; |
|||
import com.yxt.anrui.oa.biz.adreimbursedassetapply.AdReimbursedAssetApplyVo; |
|||
import com.yxt.anrui.oa.biz.oaform.flowable.CompleteDto; |
|||
import com.yxt.anrui.oa.biz.oaform.flowable.NodeQuery; |
|||
import com.yxt.anrui.oa.biz.oaform.flowable.TaskDto; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.cloud.openfeign.SpringQueryMap; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import javax.validation.Valid; |
|||
|
|||
@RestController |
|||
@RequestMapping("v1/AdReimbursedAssetApply") |
|||
public class AdReimbursedAssetApplyRest { |
|||
|
|||
|
|||
@Autowired |
|||
private AdReimbursedAssetApplyService adReimbursedAssetApplyService; |
|||
|
|||
@ApiOperation("初始化(新增或修改)") |
|||
@GetMapping({"/getInit", "/getInit/{sid}"}) |
|||
public ResultBean<AdReimbursedAssetApplyVo> getInit( |
|||
@PathVariable(value = "sid", required = false) String sid, |
|||
@RequestParam(value = "userSid", required = false) String userSid, |
|||
@RequestParam(value = "orgPath", required = false) String orgPath) { |
|||
ResultBean<AdReimbursedAssetApplyVo> rb = ResultBean.fireFail(); |
|||
if (sid == null || sid.isEmpty()) { |
|||
// 执行新增初始化
|
|||
if (userSid == null || orgPath == null) { |
|||
return rb.setMsg("userSid和orgPath不能为空"); |
|||
} |
|||
return adReimbursedAssetApplyService.getSaveInit(userSid, orgPath); |
|||
} else { |
|||
// 执行修改初始化
|
|||
return adReimbursedAssetApplyService.getUpdateInit(sid); |
|||
} |
|||
} |
|||
|
|||
@ApiOperation("新增或修改") |
|||
@PostMapping("/save") |
|||
public ResultBean save(@RequestBody AdReimbursedAssetApplyDto dto) { |
|||
return adReimbursedAssetApplyService.saveOrUpdateDto(dto); |
|||
} |
|||
|
|||
@ApiOperation("根据sid批量删除") |
|||
@DeleteMapping("/delBySids") |
|||
public ResultBean delBySids(@RequestBody String[] sids) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
adReimbursedAssetApplyService.delAll(sids); |
|||
return rb.success(); |
|||
} |
|||
|
|||
@ApiOperation("详情") |
|||
@GetMapping("/details/{sid}") |
|||
ResultBean<AdReimbursedAssetApplyDetailVo> details(@PathVariable("sid") String sid |
|||
, @RequestParam(value = "application", required = false) String application) { |
|||
return adReimbursedAssetApplyService.details(sid,application); |
|||
} |
|||
|
|||
@ApiOperation("提交审批流程") |
|||
@PostMapping("/submit") |
|||
public ResultBean submit(@RequestBody @Valid AdReimbursedAssetApplyDto dto) { |
|||
return adReimbursedAssetApplyService.submit(dto); |
|||
} |
|||
|
|||
@ApiOperation(value = "办理(同意)") |
|||
@PutMapping("/complete") |
|||
public ResultBean complete(@Valid @RequestBody CompleteDto dto) { |
|||
return adReimbursedAssetApplyService.complete(dto); |
|||
} |
|||
|
|||
@ApiOperation(value = "驳回任务") |
|||
@PutMapping(value = "/reject") |
|||
public ResultBean reject(@Valid @RequestBody TaskDto dto) { |
|||
return adReimbursedAssetApplyService.reject(dto); |
|||
} |
|||
|
|||
@ApiOperation("获取流程操作标题") |
|||
@GetMapping("/getFlowOperateTitle") |
|||
@ResponseBody |
|||
ResultBean<String> getFlowOperateTitle(@SpringQueryMap NodeQuery query) { |
|||
return adReimbursedAssetApplyService.getFlowOperateTitle(query); |
|||
} |
|||
|
|||
|
|||
} |
@ -0,0 +1,10 @@ |
|||
package com.yxt.anrui.oa.biz.adpurchaseassetapply; |
|||
|
|||
import lombok.Data; |
|||
|
|||
@Data |
|||
public class PurchaseAssetApplyVo { |
|||
|
|||
private String sid; |
|||
private String billNo; |
|||
} |
@ -0,0 +1,82 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.oa.biz.adreimbursedassetapply; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.math.BigDecimal; |
|||
|
|||
@Data |
|||
@ApiModel(value = "固定资产费用报销", description = "固定资产费用报销") |
|||
@TableName("ad_reimbursed_asset_apply") |
|||
public class AdReimbursedAssetApply extends BaseEntity { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty("基础表单sid") |
|||
private String formSid; // 基础表单sid
|
|||
@ApiModelProperty("关联审批sid列表,英文逗号分隔") |
|||
private String linkFormSids; // 关联审批sid列表,英文逗号分隔
|
|||
@ApiModelProperty("固定资产采购申请编号") |
|||
private String sourceBillNo; // 固定资产采购申请编号
|
|||
|
|||
@ApiModelProperty("报销人sid") |
|||
private String reimbursementSid; // 报销人sid
|
|||
@ApiModelProperty("报销人") |
|||
private String reimbursement; // 报销人
|
|||
@ApiModelProperty("费用所属部门sid") |
|||
private String feesDeptSid; // 费用所属部门sid
|
|||
@ApiModelProperty("费用所属部门") |
|||
private String feesDept; // 费用所属部门
|
|||
@ApiModelProperty("是否有固定资产采购申请(1是,0否)") |
|||
private String isPurchaseKey; // 是否有固定资产采购申请(1是,0否)
|
|||
@ApiModelProperty("是否有固定资产采购申请(1是,0否)") |
|||
private String isPurchaseValue; // 是否有固定资产采购申请(1是,0否)
|
|||
@ApiModelProperty("是否超固定资产采购申请范围(1是,0否)") |
|||
private String isBeyondKey; // 是否超固定资产采购申请范围(1是,0否)
|
|||
@ApiModelProperty("是否超固定资产采购申请范围(1是,0否)") |
|||
private String isBeyondValue; // 是否超固定资产采购申请范围(1是,0否)
|
|||
@ApiModelProperty("固定资产类别key") |
|||
private String assetTypeKey; // 固定资产类别key
|
|||
@ApiModelProperty("固定资产类别value") |
|||
private String assetTypeValue; // 固定资产类别value
|
|||
@ApiModelProperty("是否有服务站机器设备(1是,0否)") |
|||
private String isStationKey; // 是否有服务站机器设备(1是,0否)
|
|||
private String isStationValue; // 是否有服务站机器设备(1是,0否)
|
|||
|
|||
@ApiModelProperty("合计采购金额") |
|||
private BigDecimal totalAmount; // 合计采购金额
|
|||
@ApiModelProperty("报销金额") |
|||
private BigDecimal reimbursedAmount; // 报销金额
|
|||
@ApiModelProperty("现金、打卡或平借款") |
|||
private String reimbursedWay; // 现金、打卡或平借款
|
|||
@ApiModelProperty("打卡请输入(姓名、卡号、开户行)") |
|||
private String reimbursedInfo; // 打卡请输入(姓名、卡号、开户行)
|
|||
|
|||
} |
@ -0,0 +1,52 @@ |
|||
package com.yxt.anrui.oa.biz.adreimbursedassetapply; |
|||
|
|||
import com.yxt.anrui.oa.biz.adreimbursedassetdetails.AdReimbursedAssetListDetailsVo; |
|||
import com.yxt.anrui.oa.biz.oaform.OaFormCommonVo; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.ArrayList; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2025/1/21 |
|||
**/ |
|||
@Data |
|||
public class AdReimbursedAssetApplyDetailVo extends OaFormCommonVo { |
|||
|
|||
|
|||
|
|||
|
|||
@ApiModelProperty("备注") |
|||
private String remarks; |
|||
@ApiModelProperty("固定资产采购申请编号") |
|||
private String sourceBillNo; // 固定资产采购申请编号
|
|||
|
|||
@ApiModelProperty("报销人") |
|||
private String reimbursement; // 报销人
|
|||
@ApiModelProperty("费用所属部门") |
|||
private String feesDept; // 费用所属部门
|
|||
@ApiModelProperty("是否有固定资产采购申请(1是,0否)") |
|||
private String isPurchaseValue; // 是否有固定资产采购申请(1是,0否)
|
|||
@ApiModelProperty("是否超固定资产采购申请范围(1是,0否)") |
|||
private String isBeyondValue; // 是否超固定资产采购申请范围(1是,0否)
|
|||
@ApiModelProperty("固定资产类别value") |
|||
private String assetTypeValue; // 固定资产类别value
|
|||
private String isStationValue; // 是否有服务站机器设备(1是,0否)
|
|||
|
|||
@ApiModelProperty("合计采购金额") |
|||
private String totalAmount; // 合计采购金额
|
|||
@ApiModelProperty("报销金额") |
|||
private String reimbursedAmount; // 报销金额
|
|||
@ApiModelProperty("现金、打卡或平借款") |
|||
private String reimbursedWay; // 现金、打卡或平借款
|
|||
@ApiModelProperty("打卡请输入(姓名、卡号、开户行)") |
|||
private String reimbursedInfo; // 打卡请输入(姓名、卡号、开户行)
|
|||
|
|||
|
|||
|
|||
private List<AdReimbursedAssetListDetailsVo> list = new ArrayList<>(); |
|||
|
|||
} |
@ -0,0 +1,105 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.oa.biz.adreimbursedassetapply; |
|||
|
|||
|
|||
import com.yxt.anrui.oa.biz.adreimbursedassetdetails.AssetObjVo; |
|||
import com.yxt.anrui.oa.biz.oaform.FormCommon; |
|||
import com.yxt.anrui.oa.biz.oaform.OaFormDto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: oa(驻外人员认定申请) <br/> |
|||
* File: AdExpatriatesApplyDto.java <br/> |
|||
* Class: com.yxt.anrui.oa.api.adexpatriatesapply.AdExpatriatesApplyDto <br/> |
|||
* Description: 驻外人员认定申请 数据传输对象. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2025-01-16 15:22:53 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "固定资产费用报销 数据传输对象", description = "固定资产费用报销 数据传输对象") |
|||
public class AdReimbursedAssetApplyDto extends OaFormDto { |
|||
|
|||
|
|||
|
|||
|
|||
@ApiModelProperty("备注") |
|||
private String remarks; |
|||
|
|||
|
|||
@ApiModelProperty("报销人sid") |
|||
private String reimbursementSid; // 报销人sid
|
|||
@ApiModelProperty("报销人") |
|||
private String reimbursement; // 报销人
|
|||
|
|||
private FormCommon feesDeptObj; |
|||
@ApiModelProperty("费用所属部门sid") |
|||
private String feesDeptSid; // 费用所属部门sid
|
|||
@ApiModelProperty("费用所属部门") |
|||
private String feesDept; // 费用所属部门
|
|||
|
|||
private FormCommon isPurchaseObj; |
|||
@ApiModelProperty("是否有固定资产采购申请(1是,0否)") |
|||
private String isPurchaseKey; // 是否有固定资产采购申请(1是,0否)
|
|||
@ApiModelProperty("是否有固定资产采购申请(1是,0否)") |
|||
private String isPurchaseValue; // 是否有固定资产采购申请(1是,0否)
|
|||
|
|||
private FormCommon isBeyondObj; |
|||
@ApiModelProperty("是否超固定资产采购申请范围(1是,0否)") |
|||
private String isBeyondKey; // 是否超固定资产采购申请范围(1是,0否)
|
|||
@ApiModelProperty("是否超固定资产采购申请范围(1是,0否)") |
|||
private String isBeyondValue; // 是否超固定资产采购申请范围(1是,0否)
|
|||
|
|||
private FormCommon assetTypeObj; |
|||
@ApiModelProperty("固定资产类别key") |
|||
private String assetTypeKey; // 固定资产类别key
|
|||
@ApiModelProperty("固定资产类别value") |
|||
private String assetTypeValue; // 固定资产类别value
|
|||
|
|||
private FormCommon isStationObj; |
|||
@ApiModelProperty("是否有服务站机器设备(1是,0否)") |
|||
private String isStationKey; // 是否有服务站机器设备(1是,0否)
|
|||
private String isStationValue; // 是否有服务站机器设备(1是,0否)
|
|||
|
|||
@ApiModelProperty("合计采购金额") |
|||
private String totalAmount; // 合计采购金额
|
|||
@ApiModelProperty("报销金额") |
|||
private String reimbursedAmount; // 报销金额
|
|||
@ApiModelProperty("现金、打卡或平借款") |
|||
private String reimbursedWay; // 现金、打卡或平借款
|
|||
@ApiModelProperty("打卡请输入(姓名、卡号、开户行)") |
|||
private String reimbursedInfo; // 打卡请输入(姓名、卡号、开户行)
|
|||
|
|||
private AssetObjVo assetListObj; //固定资产采购编号及列表
|
|||
|
|||
} |
@ -0,0 +1,37 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.oa.biz.adreimbursedassetapply; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
|
|||
@Mapper |
|||
public interface AdReimbursedAssetApplyMapper extends BaseMapper<AdReimbursedAssetApply> { |
|||
|
|||
int selectBySid(String join); |
|||
|
|||
AdReimbursedAssetApplyDetailVo details(String sid); |
|||
} |
@ -0,0 +1,44 @@ |
|||
<?xml version="1.0" encoding="UTF-8" ?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.anrui.oa.biz.adreimbursedassetapply.AdReimbursedAssetApplyMapper"> |
|||
<!-- <where> ${ew.sqlSegment} </where>--> |
|||
<!-- ${ew.customSqlSegment} --> |
|||
|
|||
<select id="selectBySid" resultType="int"> |
|||
SELECT COUNT(*) |
|||
FROM ad_reimbursed_asset_apply ae |
|||
left join oa_form ofm on ae.formSid = ofm.sid |
|||
WHERE ofm.nodeState != '待提交' |
|||
and find_in_set(oa.sid, #{list}) |
|||
</select> |
|||
|
|||
<select id="details" resultMap="detailMap"> |
|||
select sourceBillNo,remarks, sid,assetTypeValue,isStationValue,totalAmount,reimbursement,feesDept,isPurchaseValue,isBeyondValue,reimbursedAmount,reimbursedWay,reimbursedInfo |
|||
from ad_reimbursed_asset_apply |
|||
where sid = #{sid} |
|||
</select> |
|||
|
|||
<resultMap id="detailMap" type="com.yxt.anrui.oa.biz.adreimbursedassetapply.AdReimbursedAssetApplyDetailVo"> |
|||
<result column="remarks" property="remarks"/> |
|||
<result column="sourceBillNo" property="sourceBillNo"/> |
|||
<result column="reimbursement" property="reimbursement"/> |
|||
<result column="feesDept" property="feesDept"/> |
|||
<result column="isPurchaseValue" property="isPurchaseValue"/> |
|||
<result column="isBeyondValue" property="isBeyondValue"/> |
|||
<result column="reimbursedAmount" property="reimbursedAmount"/> |
|||
<result column="reimbursedWay" property="reimbursedWay"/> |
|||
<result column="reimbursedInfo" property="reimbursedInfo"/> |
|||
<result column="assetTypeValue" property="assetTypeValue"/> |
|||
<result column="isStationValue" property="isStationValue"/> |
|||
<result column="totalAmount" property="totalAmount"/> |
|||
<collection property="list" ofType="com.yxt.anrui.oa.biz.adreimbursedassetdetails.AdReimbursedAssetListDetailsVo" |
|||
select="selectList" column="sid"> |
|||
</collection> |
|||
</resultMap> |
|||
|
|||
<select id="selectList" resultType="com.yxt.anrui.oa.biz.adreimbursedassetdetails.AdReimbursedAssetListDetailsVo"> |
|||
select * |
|||
from ad_reimbursed_asset_details |
|||
where mainSid = #{sid} |
|||
</select> |
|||
</mapper> |
@ -0,0 +1,346 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.oa.biz.adreimbursedassetapply; |
|||
|
|||
import cn.hutool.core.bean.BeanUtil; |
|||
import com.yxt.anrui.oa.biz.adreimbursedassetdetails.AdReimbursedAssetDetailsService; |
|||
import com.yxt.anrui.oa.biz.adreimbursedassetdetails.AdReimbursedAssetDetailsVo; |
|||
import com.yxt.anrui.oa.biz.adreimbursedassetdetails.AssetObjVo; |
|||
import com.yxt.anrui.oa.biz.oaappendix.OaAppendixService; |
|||
import com.yxt.anrui.oa.biz.oaform.*; |
|||
import com.yxt.anrui.oa.biz.oaform.flowable.*; |
|||
import com.yxt.anrui.oa.feign.flowable.flow.ProcDefEnum; |
|||
import com.yxt.anrui.oa.feign.portal.sysorganization.SysOrganizationFeign; |
|||
import com.yxt.anrui.oa.feign.portal.sysorganization.SysOrganizationVo; |
|||
import com.yxt.anrui.oa.feign.sysuser.SysUserFeign; |
|||
import com.yxt.anrui.oa.feign.sysuser.SysUserVo; |
|||
import com.yxt.common.base.service.MybatisBaseService; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import org.apache.commons.lang3.StringUtils; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
import java.util.Collections; |
|||
import java.util.HashMap; |
|||
import java.util.List; |
|||
import java.util.Map; |
|||
|
|||
@Service |
|||
public class AdReimbursedAssetApplyService extends MybatisBaseService<AdReimbursedAssetApplyMapper, AdReimbursedAssetApply> { |
|||
|
|||
@Autowired |
|||
private OaAppendixService oaAppendixService; |
|||
@Autowired |
|||
private OaFormService oaFormService; |
|||
@Autowired |
|||
private AdReimbursedAssetDetailsService adReimbursedAssetDetailsService; |
|||
@Autowired |
|||
private SysOrganizationFeign sysOrganizationFeign; |
|||
@Autowired |
|||
private SysUserFeign sysUserFeign; |
|||
|
|||
public ResultBean<AdReimbursedAssetApplyVo> getSaveInit(String userSid, String orgPath) { |
|||
ResultBean<AdReimbursedAssetApplyVo> rb = ResultBean.fireFail(); |
|||
AdReimbursedAssetApplyVo adReimbursedAssetApplyVo = new AdReimbursedAssetApplyVo(); |
|||
adReimbursedAssetApplyVo.setCreateBySid(userSid); |
|||
adReimbursedAssetApplyVo.setOrgSidPath(orgPath); |
|||
adReimbursedAssetApplyVo.setReimbursementSid(userSid); |
|||
SysUserVo userVo = sysUserFeign.fetchBySid(userSid).getData(); |
|||
if (null != userVo) { |
|||
adReimbursedAssetApplyVo.setReimbursement(userVo.getName()); |
|||
} |
|||
return rb.success().setData(adReimbursedAssetApplyVo); |
|||
} |
|||
|
|||
public ResultBean<AdReimbursedAssetApplyVo> getUpdateInit(String sid) { |
|||
ResultBean<AdReimbursedAssetApplyVo> rb = ResultBean.fireFail(); |
|||
AdReimbursedAssetApplyVo applyVo = new AdReimbursedAssetApplyVo(); |
|||
AdReimbursedAssetApply apply = fetchBySid(sid); |
|||
if (apply == null) { |
|||
return rb.setMsg("该申请不存在"); |
|||
} |
|||
OaForm oaForm = oaFormService.fetchBySid(sid); |
|||
applyVo.setTaskId(oaForm.getTaskId()); |
|||
applyVo.setProcInsId(oaForm.getProcInstId()); |
|||
//根据部门sid获取orgPath并赋值
|
|||
SysOrganizationVo organizationVo = sysOrganizationFeign.fetchBySid(oaForm.getDeptSid()).getData(); |
|||
String orgSidPath = organizationVo.getOrgSidPath(); |
|||
applyVo.setOrgSidPath(orgSidPath); |
|||
applyVo.setCreateBySid(oaForm.getCreateBySid()); |
|||
BeanUtil.copyProperties(apply, applyVo); |
|||
FormCommon assetTypeObj = FormCommon.of(apply.getAssetTypeKey(), apply.getAssetTypeValue()); |
|||
applyVo.setAssetTypeObj(assetTypeObj); |
|||
FormCommon isStationObj = FormCommon.of(apply.getIsStationKey(), apply.getIsStationValue()); |
|||
applyVo.setIsStationObj(isStationObj); |
|||
FormCommon obj1 = FormCommon.of(apply.getFeesDeptSid(), apply.getFeesDept()); |
|||
applyVo.setFeesDeptObj(obj1); |
|||
FormCommon obj2 = FormCommon.of(apply.getIsPurchaseKey(), apply.getIsPurchaseValue()); |
|||
applyVo.setIsPurchaseObj(obj2); |
|||
FormCommon obj3 = FormCommon.of(apply.getIsBeyondKey(), apply.getIsBeyondValue()); |
|||
applyVo.setIsBeyondObj(obj3); |
|||
if (StringUtils.isNotBlank(apply.getLinkFormSids())) { |
|||
AssetObjVo assetObj = new AssetObjVo(); |
|||
assetObj.setId(apply.getLinkFormSids()); |
|||
assetObj.setDictValue(apply.getSourceBillNo()); |
|||
List<AdReimbursedAssetDetailsVo> list = adReimbursedAssetDetailsService.getUpdateInit(sid); |
|||
list.removeAll(Collections.singleton(null)); |
|||
if (!list.isEmpty()) { |
|||
Map<String, List<AdReimbursedAssetDetailsVo>> extra = new HashMap<>(); |
|||
extra.put("list", list); |
|||
assetObj.setExtra(extra); |
|||
} |
|||
applyVo.setAssetListObj(assetObj); |
|||
} |
|||
applyVo.setSid(sid); |
|||
return rb.success().setData(applyVo); |
|||
} |
|||
|
|||
public ResultBean<String> saveOrUpdateDto(AdReimbursedAssetApplyDto dto) { |
|||
ResultBean<String> rb = ResultBean.fireFail(); |
|||
String sid = dto.getSid(); |
|||
AssetObjVo assetListObj = dto.getAssetListObj(); |
|||
String linkSid = ""; |
|||
String sourceBillNo = ""; |
|||
if (null != assetListObj) { |
|||
if (StringUtils.isNotBlank(assetListObj.getId())) { |
|||
linkSid = assetListObj.getId(); |
|||
} |
|||
if (StringUtils.isNotBlank(assetListObj.getDictValue())) { |
|||
sourceBillNo = assetListObj.getDictValue(); |
|||
} |
|||
} |
|||
Map<String, List<AdReimbursedAssetDetailsVo>> extra = assetListObj.getExtra(); |
|||
if (StringUtils.isBlank(sid)) { |
|||
// 新建操作
|
|||
AdReimbursedAssetApply entity = new AdReimbursedAssetApply(); |
|||
BeanUtil.copyProperties(dto, entity, "sid"); |
|||
entity.setLinkFormSids(linkSid); |
|||
entity.setSourceBillNo(sourceBillNo); |
|||
dto.setBillNo("GDZCFYBXSQ"); |
|||
dto.setSid(entity.getSid()); |
|||
ResultBean<String> resultBean = oaFormService.saveOaForm(dto); |
|||
|
|||
if (!resultBean.getSuccess()) { |
|||
return rb; |
|||
} |
|||
|
|||
entity.setFormSid(resultBean.getData()); |
|||
baseMapper.insert(entity); |
|||
sid = entity.getSid(); |
|||
} else { |
|||
// 更新操作
|
|||
AdReimbursedAssetApply entity = fetchBySid(sid); |
|||
BeanUtil.copyProperties(dto, entity, "id", "sid"); |
|||
entity.setLinkFormSids(linkSid); |
|||
entity.setSourceBillNo(sourceBillNo); |
|||
baseMapper.updateById(entity); |
|||
} |
|||
if (null != extra) { |
|||
List<AdReimbursedAssetDetailsVo> list = extra.get("list"); |
|||
if (!list.isEmpty()) { |
|||
adReimbursedAssetDetailsService.saveDetails(list, sid); |
|||
} |
|||
} |
|||
return rb.success().setData(sid); |
|||
} |
|||
|
|||
// 保存文件
|
|||
private void saveFiles(String sid, List<String> files, String attachType, String fileType) { |
|||
files.removeAll(Collections.singleton(null)); |
|||
oaAppendixService.saveFile(sid, files, attachType, fileType); |
|||
} |
|||
|
|||
public ResultBean delAll(String[] sids) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
//查询该sid中是否有流程不是待提交的
|
|||
int count = baseMapper.selectBySid(StringUtils.join(sids, ",")); |
|||
if (count > 0) { |
|||
return rb.setMsg("删除的数据中包含已提交或已办结审批的数据,删除失败"); |
|||
} |
|||
delBySids(sids); |
|||
return rb.success(); |
|||
} |
|||
|
|||
public ResultBean<AdReimbursedAssetApplyDetailVo> details(String sid, String application) { |
|||
ResultBean<AdReimbursedAssetApplyDetailVo> rb = ResultBean.fireFail(); |
|||
AdReimbursedAssetApplyDetailVo applyDetailVo = baseMapper.details(sid); |
|||
if (applyDetailVo == null) { |
|||
return rb.setMsg("该申请不存在"); |
|||
} |
|||
//基础字段赋值
|
|||
BeanUtil.copyProperties(oaFormService.getDetails(sid), applyDetailVo); |
|||
return rb.success().setData(applyDetailVo); |
|||
} |
|||
|
|||
/** |
|||
* 提交 |
|||
* |
|||
* @param dto |
|||
* @return |
|||
*/ |
|||
public ResultBean submit(AdReimbursedAssetApplyDto dto) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
ResultBean<String> stringResultBean = saveOrUpdateDto(dto); |
|||
if (!stringResultBean.getSuccess()) { |
|||
return rb.setMsg(stringResultBean.getData()); |
|||
} |
|||
String businessSid = stringResultBean.getData(); |
|||
|
|||
SubmitDto submitDto = new SubmitDto(); |
|||
submitDto.setUserSid(dto.getCreateBySid()); |
|||
submitDto.setBusinessSid(businessSid); |
|||
|
|||
Map<String, Object> formVariables = new HashMap<>(); |
|||
formVariables = getMap(formVariables, businessSid); |
|||
submitDto.setFormVariables(formVariables); |
|||
submitDto.setProcDefId(ProcDefEnum.HIHIREAPPLY.getProDefId()); |
|||
submitDto.setNextTaskId(dto.getTaskId()); |
|||
submitDto.setRule(OaFormRuleEnum.DIRECTLY_UNDER.getRule()); |
|||
return oaFormService.submit(submitDto); |
|||
} |
|||
|
|||
/** |
|||
* 办理(同意) |
|||
* |
|||
* @param dto |
|||
* @return |
|||
*/ |
|||
public ResultBean complete(CompleteDto dto) { |
|||
Map<String, Object> formVariables = dto.getFormVariables(); |
|||
formVariables = getMap(formVariables, dto.getBusinessSid()); |
|||
dto.setFormVariables(formVariables); |
|||
BusinessVariablesDto businessVariablesDto = new BusinessVariablesDto(); |
|||
BeanUtil.copyProperties(dto, businessVariablesDto); |
|||
return oaFormService.complete(businessVariablesDto); |
|||
} |
|||
|
|||
/** |
|||
* 驳回 |
|||
* |
|||
* @param dto |
|||
* @return |
|||
*/ |
|||
public ResultBean reject(TaskDto dto) { |
|||
Map<String, Object> formVariables = dto.getFormVariables(); |
|||
formVariables = getMap(formVariables, dto.getBusinessSid()); |
|||
dto.setFormVariables(formVariables); |
|||
return oaFormService.reject(dto); |
|||
} |
|||
|
|||
public ResultBean<String> getFlowOperateTitle(NodeQuery query) { |
|||
// 默认失败返回
|
|||
ResultBean<String> rb = ResultBean.fireFail(); |
|||
|
|||
// 获取next值和formVariables
|
|||
int next = query.getNext(); |
|||
|
|||
// 获取并更新formVariables
|
|||
Map<String, Object> formVariables = getMap(query.getFormVariables(), query.getBusinessSid()); |
|||
query.setFormVariables(formVariables); |
|||
|
|||
// 校验next参数是否有效(只允许0或1)
|
|||
if (next != 0 && next != 1) { |
|||
return rb.setMsg("参数错误:next"); // 如果next不是0或1,返回错误信息
|
|||
} |
|||
|
|||
// 获取节点名称
|
|||
String data = getNodeName(query, next); |
|||
|
|||
// 如果data为null,表示未获取到有效的节点信息
|
|||
if (data == null) { |
|||
return rb.setMsg("没有获取到节点信息"); // 返回错误消息
|
|||
} |
|||
|
|||
// 返回成功的结果和获取到的节点名称
|
|||
return rb.success().setData(data); |
|||
} |
|||
|
|||
/** |
|||
* 网关参数组成 |
|||
* |
|||
* @param formVariables |
|||
* @param sid |
|||
* @return |
|||
*/ |
|||
public Map<String, Object> getMap(Map<String, Object> formVariables, String sid) { |
|||
Map<String, Object> appMap = new HashMap<>(); |
|||
appMap.put("sid", sid); |
|||
/*appMap.put("editUrl", "approval/#/pages/EditOnboradingApplyActivity?sid=" + sid); |
|||
appMap.put("detailUrl", "approval/#/pages/DetailOnboradingApplyActivity?sid=" + sid); |
|||
appMap.put("flowOperateUrl", "oa/v1/HrHireApply/getFlowOperateTitle"); |
|||
appMap.put("agreeUrl", "oa/v1/HrHireApply/complete"); |
|||
appMap.put("stopUrl", "oa/v1/oaform/breakProcess"); |
|||
appMap.put("rejectUrl", "oa/v1/HrHireApply/reject"); |
|||
appMap.put("recallUrl", "oa/v1/oaform/revokeProcess"); |
|||
appMap.put("signUrl", "oa/v1/oaform/delegate"); |
|||
appMap.put("transferUrl", "oa/v1/oaform/assignTask");*/ |
|||
appMap.put(OaFormUrlEnum.HRHIREAPPLY_EDIT.getType(), OaFormUrlEnum.HRHIREAPPLY_EDIT.getUrl() + "?sid=" + sid); |
|||
appMap.put(OaFormUrlEnum.HRHIREAPPLY_DETAIL.getType(), OaFormUrlEnum.HRHIREAPPLY_DETAIL.getUrl() + "?sid=" + sid); |
|||
appMap.put(OaFormUrlEnum.HRHIREAPPLY_FLOWOPERATEURL.getType(), OaFormUrlEnum.HRHIREAPPLY_FLOWOPERATEURL.getUrl()); |
|||
appMap.put(OaFormUrlEnum.HRHIREAPPLY_AGREEURL.getType(), OaFormUrlEnum.HRHIREAPPLY_AGREEURL.getUrl()); |
|||
appMap.put(OaFormUrlEnum.STOPURL.getType(), OaFormUrlEnum.STOPURL.getUrl()); |
|||
appMap.put(OaFormUrlEnum.HRHIREAPPLY_REJECTURL.getType(), OaFormUrlEnum.HRHIREAPPLY_REJECTURL.getUrl()); |
|||
appMap.put(OaFormUrlEnum.RECALLURL.getType(), OaFormUrlEnum.RECALLURL.getUrl()); |
|||
appMap.put(OaFormUrlEnum.SIGNURL.getType(), OaFormUrlEnum.SIGNURL.getUrl()); |
|||
appMap.put(OaFormUrlEnum.TRANSFERURL.getType(), OaFormUrlEnum.TRANSFERURL.getUrl()); |
|||
formVariables.put("app", appMap); |
|||
//根据组织查询是否是分公司
|
|||
OaForm oaForm = oaFormService.fetchBySid(sid); |
|||
AdReimbursedAssetApply adExpatriatesApply = fetchBySid(sid); |
|||
SysOrganizationVo sysOrganization = sysOrganizationFeign.fetchBySid(oaForm.getUseOrgSid()).getData(); |
|||
//是否是分公司
|
|||
formVariables.put("isTrue", sysOrganization.getIsDept() == 0); |
|||
return formVariables; |
|||
} |
|||
|
|||
/** |
|||
* 根据next的值获取前一个节点或下一个节点的名称。 |
|||
* |
|||
* @param query 包含查询所需参数的NodeQuery对象 |
|||
* @param next 参数,0表示上一环节,1表示下一环节 |
|||
* @return 节点名称,如果失败则返回null |
|||
*/ |
|||
private String getNodeName(NodeQuery query, int next) { |
|||
// 根据next值选择相应的服务方法获取节点信息
|
|||
ResultBean<List<NodeVo>> resultBean = (next == 0) |
|||
? oaFormService.getPreviousNodesForReject(query) // 获取上一环节的节点
|
|||
: oaFormService.getNextNodesForSubmit(query); // 获取下一环节的节点
|
|||
// 如果服务调用成功
|
|||
if (resultBean.getSuccess()) { |
|||
// 清除结果列表中的null值,避免空节点
|
|||
resultBean.getData().removeAll(Collections.singleton(null)); |
|||
// 如果结果列表非空,返回第一个节点的名称
|
|||
if (!resultBean.getData().isEmpty()) { |
|||
return resultBean.getData().get(0).getName(); |
|||
} |
|||
} else { |
|||
// 如果服务调用失败,返回null
|
|||
return null; |
|||
} |
|||
// 如果结果为空,返回null
|
|||
return null; |
|||
} |
|||
} |
@ -0,0 +1,94 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.oa.biz.adreimbursedassetapply; |
|||
|
|||
import com.yxt.anrui.oa.biz.adreimbursedassetdetails.AssetObjVo; |
|||
import com.yxt.anrui.oa.biz.oaform.FormCommon; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
@Data |
|||
public class AdReimbursedAssetApplyVo { |
|||
|
|||
private String sid; |
|||
/* private String userSid; |
|||
private String orgPath;*/ |
|||
private String orgSidPath; |
|||
private String createBySid; |
|||
|
|||
@ApiModelProperty("备注") |
|||
private String remarks; |
|||
|
|||
@ApiModelProperty("报销人sid") |
|||
private String reimbursementSid; // 报销人sid
|
|||
@ApiModelProperty("报销人") |
|||
private String reimbursement; // 报销人
|
|||
|
|||
private FormCommon feesDeptObj; |
|||
@ApiModelProperty("费用所属部门sid") |
|||
private String feesDeptSid; // 费用所属部门sid
|
|||
@ApiModelProperty("费用所属部门") |
|||
private String feesDept; // 费用所属部门
|
|||
|
|||
private FormCommon isPurchaseObj; |
|||
@ApiModelProperty("是否有固定资产采购申请(1是,0否)") |
|||
private String isPurchaseKey; // 是否有固定资产采购申请(1是,0否)
|
|||
@ApiModelProperty("是否有固定资产采购申请(1是,0否)") |
|||
private String isPurchaseValue; // 是否有固定资产采购申请(1是,0否)
|
|||
|
|||
private FormCommon isBeyondObj; |
|||
@ApiModelProperty("是否超固定资产采购申请范围(1是,0否)") |
|||
private String isBeyondKey; // 是否超固定资产采购申请范围(1是,0否)
|
|||
@ApiModelProperty("是否超固定资产采购申请范围(1是,0否)") |
|||
private String isBeyondValue; // 是否超固定资产采购申请范围(1是,0否)
|
|||
|
|||
private FormCommon assetTypeObj; |
|||
@ApiModelProperty("固定资产类别key") |
|||
private String assetTypeKey; // 固定资产类别key
|
|||
@ApiModelProperty("固定资产类别value") |
|||
private String assetTypeValue; // 固定资产类别value
|
|||
|
|||
private FormCommon isStationObj; |
|||
@ApiModelProperty("是否有服务站机器设备(1是,0否)") |
|||
private String isStationKey; // 是否有服务站机器设备(1是,0否)
|
|||
private String isStationValue; // 是否有服务站机器设备(1是,0否)
|
|||
|
|||
@ApiModelProperty("合计采购金额") |
|||
private String totalAmount; // 合计采购金额
|
|||
@ApiModelProperty("报销金额") |
|||
private String reimbursedAmount; // 报销金额
|
|||
@ApiModelProperty("现金、打卡或平借款") |
|||
private String reimbursedWay; // 现金、打卡或平借款
|
|||
@ApiModelProperty("打卡请输入(姓名、卡号、开户行)") |
|||
private String reimbursedInfo; // 打卡请输入(姓名、卡号、开户行)
|
|||
|
|||
private AssetObjVo assetListObj; //固定资产采购编号及列表
|
|||
|
|||
private String taskId; |
|||
@ApiModelProperty("流程实例id") |
|||
private String procInsId; |
|||
|
|||
} |
@ -0,0 +1,60 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.oa.biz.adreimbursedassetdetails; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.math.BigDecimal; |
|||
|
|||
@Data |
|||
@ApiModel(value = "固定资产费用报销列表", description = "固定资产费用报销") |
|||
@TableName("ad_reimbursed_asset_details") |
|||
public class AdReimbursedAssetDetails extends BaseEntity { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty("申请sid") |
|||
private String mainSid; // 申请sid
|
|||
@ApiModelProperty("固定资产基础信息sid") |
|||
private String goodsSpuSid; // 固定资产基础信息sid
|
|||
@ApiModelProperty("固定资产编号") |
|||
private String goodsSkuCode; // 固定资产编号
|
|||
@ApiModelProperty("固定资产名称") |
|||
private String goodsSpuName; // 固定资产名称
|
|||
@ApiModelProperty("规格型号") |
|||
private String goodsSkuOwnSpec; // 规格型号
|
|||
@ApiModelProperty("单位") |
|||
private String unit; // 单位
|
|||
@ApiModelProperty("采购单价") |
|||
private BigDecimal cost; // 预计采购单价
|
|||
@ApiModelProperty("采购数量") |
|||
private BigDecimal estimateCount; // 预计采购数量
|
|||
@ApiModelProperty("采购金额") |
|||
private BigDecimal estimateMoney; // 预计采购金额
|
|||
} |
@ -0,0 +1,42 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.oa.biz.adreimbursedassetdetails; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
import org.apache.ibatis.annotations.Select; |
|||
|
|||
import java.util.List; |
|||
|
|||
@Mapper |
|||
public interface AdReimbursedAssetDetailsMapper extends BaseMapper<AdReimbursedAssetDetails> { |
|||
|
|||
@Select("select * from ad_reimbursed_asset_details where mainSid = #{mainSid}") |
|||
List<AdReimbursedAssetDetails> selectByMainSid(String mainSid); |
|||
|
|||
@Select("select * from ad_reimbursed_asset_details where mainSid = #{mainSid}") |
|||
List<AdReimbursedAssetDetailsVo> getUpdateInit(String mainSid); |
|||
} |
@ -0,0 +1,5 @@ |
|||
<?xml version="1.0" encoding="UTF-8" ?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.anrui.oa.biz.adreimbursedassetdetails.AdReimbursedAssetDetailsMapper"> |
|||
|
|||
</mapper> |
@ -0,0 +1,62 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.oa.biz.adreimbursedassetdetails; |
|||
|
|||
import cn.hutool.core.bean.BeanUtil; |
|||
import com.yxt.common.base.service.MybatisBaseService; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
import java.util.Collections; |
|||
import java.util.List; |
|||
|
|||
@Service |
|||
public class AdReimbursedAssetDetailsService extends MybatisBaseService<AdReimbursedAssetDetailsMapper, AdReimbursedAssetDetails> { |
|||
|
|||
public void saveDetails(List<AdReimbursedAssetDetailsVo> list, String sid) { |
|||
//根据sid查询明细并删除
|
|||
List<AdReimbursedAssetDetails> list2 = baseMapper.selectByMainSid(sid); |
|||
list2.removeAll(Collections.singleton(null)); |
|||
if (!list2.isEmpty()) { |
|||
list2.stream().forEach(v -> { |
|||
deleteBySid(v.getSid()); |
|||
}); |
|||
} |
|||
list.removeAll(Collections.singleton(null)); |
|||
if (!list.isEmpty()) { |
|||
list.stream().forEach(details -> { |
|||
AdReimbursedAssetDetails entity = new AdReimbursedAssetDetails(); |
|||
BeanUtil.copyProperties(details, entity); |
|||
entity.setMainSid(sid); |
|||
baseMapper.insert(entity); |
|||
}); |
|||
} |
|||
} |
|||
|
|||
public List<AdReimbursedAssetDetailsVo> getUpdateInit(String mainSid) { |
|||
return baseMapper.getUpdateInit(mainSid); |
|||
} |
|||
|
|||
} |
@ -0,0 +1,26 @@ |
|||
package com.yxt.anrui.oa.biz.adreimbursedassetdetails; |
|||
|
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
@Data |
|||
public class AdReimbursedAssetDetailsVo { |
|||
|
|||
@ApiModelProperty("固定资产基础信息sid") |
|||
private String goodsSpuSid; // 固定资产基础信息sid
|
|||
@ApiModelProperty("固定资产编号") |
|||
private String goodsSkuCode; // 固定资产编号
|
|||
@ApiModelProperty("固定资产名称") |
|||
private String goodsSpuName; // 固定资产名称
|
|||
@ApiModelProperty("规格型号") |
|||
private String goodsSkuOwnSpec; // 规格型号
|
|||
@ApiModelProperty("单位") |
|||
private String unit; // 单位
|
|||
@ApiModelProperty("采购单价") |
|||
private String cost; // 预计采购单价
|
|||
@ApiModelProperty("采购数量") |
|||
private String estimateCount; // 预计采购数量
|
|||
@ApiModelProperty("采购金额") |
|||
private String estimateMoney; // 预计采购金额
|
|||
|
|||
} |
@ -0,0 +1,69 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.oa.biz.adreimbursedassetdetails; |
|||
|
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: yxt-oa(认定人员) <br/> |
|||
* File: AdExpatriatesDetailVo.java <br/> |
|||
* Class: com.yxt.anrui.oa.api.adexpatriatesdetail.AdExpatriatesDetailVo <br/> |
|||
* Description: 驻外人员认定列表 视图数据对象. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2025-01-20 15:35:08 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "固定资产费用报销列表 视图数据对象", description = "固定资产费用报销列表 视图数据对象") |
|||
public class AdReimbursedAssetListDetailsVo implements Vo { |
|||
|
|||
private String sid; |
|||
|
|||
|
|||
@ApiModelProperty("固定资产编号") |
|||
private String goodsSkuCode; // 固定资产编号
|
|||
@ApiModelProperty("固定资产名称") |
|||
private String goodsSpuName; // 固定资产名称
|
|||
@ApiModelProperty("规格型号") |
|||
private String goodsSkuOwnSpec; // 规格型号
|
|||
@ApiModelProperty("单位") |
|||
private String unit; // 单位
|
|||
@ApiModelProperty("预计采购单价") |
|||
private String cost; // 预计采购单价
|
|||
@ApiModelProperty("预计采购数量") |
|||
private String estimateCount; // 预计采购数量
|
|||
@ApiModelProperty("预计采购金额") |
|||
private String estimateMoney; // 预计采购金额
|
|||
|
|||
} |
@ -0,0 +1,16 @@ |
|||
package com.yxt.anrui.oa.biz.adreimbursedassetdetails; |
|||
|
|||
import lombok.Data; |
|||
|
|||
import java.util.List; |
|||
import java.util.Map; |
|||
|
|||
@Data |
|||
public class AssetObjVo { |
|||
|
|||
private String id; |
|||
private String dictValue; |
|||
|
|||
private Map<String, List<AdReimbursedAssetDetailsVo>> extra; |
|||
|
|||
} |
Loading…
Reference in new issue