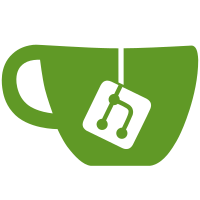
14 changed files with 278 additions and 8 deletions
@ -0,0 +1,36 @@ |
|||
package com.yxt.supervise.report.biz.stock; |
|||
|
|||
import cn.hutool.core.util.IdUtil; |
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import com.yxt.common.core.domain.EntityWithId; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
|
|||
@Data |
|||
@TableName("report_stock_day") |
|||
public class ReportStockDay extends EntityWithId { |
|||
private String sid = IdUtil.fastSimpleUUID(); // sid
|
|||
@JsonFormat( |
|||
pattern = "yyyy-MM-dd HH:mm:ss", |
|||
timezone = "GMT+8" |
|||
) |
|||
private Date createTime = new Date(); // 记录创建时间
|
|||
private String remarks; // 备注说明',
|
|||
private String orderDate; // 单据日期',
|
|||
|
|||
private String projectSid; // 项目Sid',
|
|||
private String projectName; // 项目名称',
|
|||
|
|||
private int stockNumber = 0; // 仓库数量',
|
|||
private String reportTime; // 汇总时间',
|
|||
private int countNumber = 0; // 合计货物数量',
|
|||
private double countAmount = 0; // 合计货值',
|
|||
|
|||
private String fileName; // 文件名',
|
|||
private String fileType; // 文件类型',
|
|||
private String fileSuffix; // 文件后缀',
|
|||
private String filePath; // 文件的相对路径',
|
|||
private String fullUrl; // 文件完整的访问URL',
|
|||
} |
@ -0,0 +1,8 @@ |
|||
package com.yxt.supervise.report.biz.stock; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
|
|||
@Mapper |
|||
public interface ReportStockDayMapper extends BaseMapper<ReportStockDay> { |
|||
} |
@ -0,0 +1,37 @@ |
|||
package com.yxt.supervise.report.biz.stock; |
|||
|
|||
import cn.hutool.core.util.IdUtil; |
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import com.yxt.common.core.domain.EntityWithId; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
|
|||
@Data |
|||
@TableName("report_stock_day_product") |
|||
public class ReportStockDayProduct extends EntityWithId { |
|||
private String sid = IdUtil.fastSimpleUUID(); // sid
|
|||
@JsonFormat( |
|||
pattern = "yyyy-MM-dd HH:mm:ss", |
|||
timezone = "GMT+8" |
|||
) |
|||
private Date createTime = new Date(); // 记录创建时间
|
|||
private String remarks; // 备注说明',
|
|||
private String orderDate; // 单据日期',
|
|||
|
|||
private String projectSid; // 项目Sid',
|
|||
private String projectName; // 项目名称',
|
|||
|
|||
private String reportSid; // 报表Sid',
|
|||
private String storeSid; // 仓库Sid',
|
|||
private String storeName; // 仓库名称',
|
|||
private String productSid; // 货物Sid',
|
|||
private String productName; // 货物名称',
|
|||
|
|||
private String reportStoreSid; // 报表仓库记录Sid',
|
|||
|
|||
private int prodNumber = 0; // 货物数量',
|
|||
private double prodPrice = 0; // 货物单价',
|
|||
private double prodAmount = 0; // 货物货值',
|
|||
} |
@ -0,0 +1,8 @@ |
|||
package com.yxt.supervise.report.biz.stock; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
|
|||
@Mapper |
|||
public interface ReportStockDayProductMapper extends BaseMapper<ReportStockDayProduct> { |
|||
} |
@ -0,0 +1,16 @@ |
|||
package com.yxt.supervise.report.biz.stock; |
|||
|
|||
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
|||
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
import java.util.List; |
|||
|
|||
@Service |
|||
public class ReportStockDayProductService extends ServiceImpl<ReportStockDayProductMapper, ReportStockDayProduct> { |
|||
public List<ReportStockDayProduct> listByReportStoreSid(String reportStoreSid) { |
|||
QueryWrapper<ReportStockDayProduct> qw = new QueryWrapper<>(); |
|||
qw.eq("reportStoreSid", reportStoreSid); |
|||
return baseMapper.selectList(qw); |
|||
} |
|||
} |
@ -0,0 +1,22 @@ |
|||
package com.yxt.supervise.report.biz.stock; |
|||
|
|||
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
|||
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
@Service |
|||
public class ReportStockDayService extends ServiceImpl<ReportStockDayMapper, ReportStockDay> { |
|||
public ReportStockDay fetchByProjectAndDay(String projectSid, String orderDate) { |
|||
|
|||
QueryWrapper<ReportStockDay> qw = new QueryWrapper<>(); |
|||
qw.eq("projectSid", projectSid); |
|||
qw.eq("orderDate", orderDate); |
|||
|
|||
return baseMapper.selectOne(qw); |
|||
} |
|||
|
|||
public ReportStockDay buildReportByProjectAndDay(String projectSid, String orderDate) { |
|||
// TODO: 根据项目Sid获取关联仓库,根据仓库Sid获取库存数据
|
|||
return null; |
|||
} |
|||
} |
@ -0,0 +1,32 @@ |
|||
package com.yxt.supervise.report.biz.stock; |
|||
|
|||
import cn.hutool.core.util.IdUtil; |
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import com.yxt.common.core.domain.EntityWithId; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
|
|||
@Data |
|||
@TableName("report_stock_day_store") |
|||
public class ReportStockDayStore extends EntityWithId { |
|||
private String sid = IdUtil.fastSimpleUUID(); // sid
|
|||
@JsonFormat( |
|||
pattern = "yyyy-MM-dd HH:mm:ss", |
|||
timezone = "GMT+8" |
|||
) |
|||
private Date createTime = new Date(); // 记录创建时间
|
|||
private String remarks; // 备注说明',
|
|||
private String orderDate; // 单据日期',
|
|||
|
|||
private String projectSid; // 项目Sid',
|
|||
private String projectName; // 项目名称',
|
|||
|
|||
private String reportSid; // 报表Sid',
|
|||
private String storeSid; // 仓库Sid',
|
|||
private String storeName; // 仓库名称',
|
|||
|
|||
private int productNumber = 0; // 货物数量',
|
|||
private double productAmount = 0; // 货物货值',
|
|||
} |
@ -0,0 +1,8 @@ |
|||
package com.yxt.supervise.report.biz.stock; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
|
|||
@Mapper |
|||
public interface ReportStockDayStoreMapper extends BaseMapper<ReportStockDayStore> { |
|||
} |
@ -0,0 +1,18 @@ |
|||
package com.yxt.supervise.report.biz.stock; |
|||
|
|||
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
|||
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
import java.util.List; |
|||
|
|||
@Service |
|||
public class ReportStockDayStoreService extends ServiceImpl<ReportStockDayStoreMapper, ReportStockDayStore> { |
|||
public List<ReportStockDayStore> listByProjectAndDay(String projectSid, String orderDate) { |
|||
|
|||
QueryWrapper<ReportStockDayStore> qw = new QueryWrapper<>(); |
|||
qw.eq("projectSid", projectSid); |
|||
qw.eq("orderDate", orderDate); |
|||
return baseMapper.selectList(qw); |
|||
} |
|||
} |
@ -0,0 +1,49 @@ |
|||
package com.yxt.supervise.report.biz.stock; |
|||
|
|||
import com.yxt.common.core.result.ResultBean; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.web.bind.annotation.GetMapping; |
|||
import org.springframework.web.bind.annotation.PathVariable; |
|||
import org.springframework.web.bind.annotation.RestController; |
|||
|
|||
import java.util.List; |
|||
|
|||
@RestController("/reportstock") |
|||
public class StockDayRest { |
|||
|
|||
@Autowired |
|||
private ReportStockDayService reportStockDayService; |
|||
@Autowired |
|||
private ReportStockDayStoreService reportStockDayStoreService; |
|||
@Autowired |
|||
private ReportStockDayProductService reportStockDayProductService; |
|||
|
|||
@GetMapping("/getDayGather/{projectSid}/{orderDate}") |
|||
public ResultBean<ReportStockDay> getDayGather(@PathVariable("projectSid") String projectSid, @PathVariable("orderDate") String orderDate) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
ReportStockDay pv = reportStockDayService.fetchByProjectAndDay(projectSid, orderDate); |
|||
return rb.success().setData(pv); |
|||
} |
|||
|
|||
@GetMapping("/getDayStore/{projectSid}/{orderDate}") |
|||
public ResultBean<List<ReportStockDayStore>> getDayStore(@PathVariable("projectSid") String projectSid, @PathVariable("orderDate") String orderDate) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
List<ReportStockDayStore> pv = reportStockDayStoreService.listByProjectAndDay(projectSid, orderDate); |
|||
return rb.success().setData(pv); |
|||
} |
|||
|
|||
@GetMapping("/getDayProduct/{reportStoreSid}") |
|||
public ResultBean<List<ReportStockDayProduct>> getDayProduct(@PathVariable("reportStoreSid") String reportStoreSid) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
List<ReportStockDayProduct> pv = reportStockDayProductService.listByReportStoreSid(reportStoreSid); |
|||
return rb.success().setData(pv); |
|||
} |
|||
|
|||
@GetMapping("/buildReport/{projectSid}/{orderDate}") |
|||
public ResultBean<ReportStockDay> buildReport(@PathVariable("projectSid") String projectSid, @PathVariable("orderDate") String orderDate) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
ReportStockDay pv = reportStockDayService.buildReportByProjectAndDay(projectSid, orderDate); |
|||
|
|||
return rb.success().setData(pv); |
|||
} |
|||
} |
Loading…
Reference in new issue