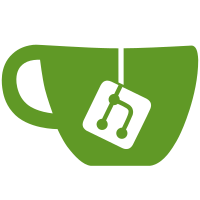
8 changed files with 252 additions and 0 deletions
@ -0,0 +1,20 @@ |
|||||
|
package com.yxt.supervise.cyf.api.productinformation; |
||||
|
|
||||
|
import com.baomidou.mybatisplus.annotation.TableName; |
||||
|
import com.yxt.common.core.domain.BaseEntity; |
||||
|
import io.swagger.annotations.ApiModel; |
||||
|
import lombok.Data; |
||||
|
|
||||
|
/** |
||||
|
* @author feikefei |
||||
|
* @create 2023-05-22-15:41 |
||||
|
*/ |
||||
|
@Data |
||||
|
@ApiModel(value = "产品信息", description = "产品信息") |
||||
|
@TableName("product_information") |
||||
|
public class ProductInformation extends BaseEntity { |
||||
|
//产品类型名称
|
||||
|
private String name; |
||||
|
//产品类型编号
|
||||
|
private String number; |
||||
|
} |
@ -0,0 +1,21 @@ |
|||||
|
package com.yxt.supervise.cyf.api.productinformation; |
||||
|
|
||||
|
import com.yxt.common.core.dto.Dto; |
||||
|
import io.swagger.annotations.ApiModel; |
||||
|
import lombok.Data; |
||||
|
|
||||
|
/** |
||||
|
* @author feikefei |
||||
|
* @create 2023-05-22-15:43 |
||||
|
*/ |
||||
|
@Data |
||||
|
@ApiModel(value = "产品信息 数据传输对象", description = "产品信息 数据传输对象") |
||||
|
public class ProductInformationDto implements Dto { |
||||
|
private String id; |
||||
|
private String sid; |
||||
|
|
||||
|
//产品类型名称
|
||||
|
private String name; |
||||
|
//产品类型编号
|
||||
|
private String number; |
||||
|
} |
@ -0,0 +1,23 @@ |
|||||
|
package com.yxt.supervise.cyf.api.productinformation; |
||||
|
|
||||
|
import com.baomidou.mybatisplus.annotation.TableName; |
||||
|
import com.yxt.common.core.query.Query; |
||||
|
import io.swagger.annotations.ApiModel; |
||||
|
import lombok.Data; |
||||
|
|
||||
|
/** |
||||
|
* @author feikefei |
||||
|
* @create 2023-05-22-17:57 |
||||
|
*/ |
||||
|
@Data |
||||
|
@ApiModel(value = "产品信息 查询条件", description = "产品信息 查询条件") |
||||
|
public class ProductInformationQuery implements Query { |
||||
|
|
||||
|
private String id; |
||||
|
private String sid; |
||||
|
|
||||
|
//产品类型名称
|
||||
|
private String name; |
||||
|
//产品类型编号
|
||||
|
private String number; |
||||
|
} |
@ -0,0 +1,22 @@ |
|||||
|
package com.yxt.supervise.cyf.api.productinformation; |
||||
|
|
||||
|
import com.yxt.common.core.vo.Vo; |
||||
|
import io.swagger.annotations.ApiModel; |
||||
|
import lombok.Data; |
||||
|
|
||||
|
/** |
||||
|
* @author feikefei |
||||
|
* @create 2023-05-22-17:58 |
||||
|
*/ |
||||
|
@Data |
||||
|
@ApiModel(value = "产品信息 视图数据对象", description = "产品信息 视图数据对象") |
||||
|
public class ProductInformationVo implements Vo { |
||||
|
|
||||
|
private String id; |
||||
|
private String sid; |
||||
|
|
||||
|
//产品类型名称
|
||||
|
private String name; |
||||
|
//产品类型编号
|
||||
|
private String number; |
||||
|
} |
@ -0,0 +1,27 @@ |
|||||
|
package com.yxt.supervise.cyf.biz.productinformation; |
||||
|
|
||||
|
import com.baomidou.mybatisplus.core.conditions.Wrapper; |
||||
|
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||
|
import com.baomidou.mybatisplus.core.toolkit.Constants; |
||||
|
import com.yxt.supervise.cyf.api.productinformation.ProductInformation; |
||||
|
import com.yxt.supervise.cyf.api.productinformation.ProductInformationVo; |
||||
|
import org.apache.ibatis.annotations.Mapper; |
||||
|
import org.apache.ibatis.annotations.Param; |
||||
|
import org.apache.ibatis.annotations.Select; |
||||
|
|
||||
|
import java.util.List; |
||||
|
|
||||
|
/** |
||||
|
* @author feikefei |
||||
|
* @create 2023-05-22-17:32 |
||||
|
*/ |
||||
|
@Mapper |
||||
|
public interface ProductInformationMapper extends BaseMapper<ProductInformation> { |
||||
|
|
||||
|
IPage<ProductInformationVo> selectPageVo(IPage<ProductInformation> page, @Param(Constants.WRAPPER) Wrapper<ProductInformation> qw); |
||||
|
|
||||
|
@Select("select * from product_information") |
||||
|
List<ProductInformation> selectList(); |
||||
|
|
||||
|
} |
@ -0,0 +1,16 @@ |
|||||
|
<?xml version="1.0" encoding="UTF-8" ?> |
||||
|
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
||||
|
<mapper namespace="com.yxt.supervise.cyf.biz.productinformation.ProductInformationMapper"> |
||||
|
<!-- <where> ${ew.sqlSegment} </where>--> |
||||
|
<!-- ${ew.customSqlSegment} --> |
||||
|
<select id="selectPageVo" resultType="com.yxt.supervise.cyf.api.productinformation.ProductInformationVo"> |
||||
|
SELECT |
||||
|
* |
||||
|
FROM |
||||
|
product_information |
||||
|
<where> |
||||
|
${ew.sqlSegment} |
||||
|
</where> |
||||
|
</select> |
||||
|
|
||||
|
</mapper> |
@ -0,0 +1,57 @@ |
|||||
|
package com.yxt.supervise.cyf.biz.productinformation; |
||||
|
|
||||
|
import com.yxt.common.core.query.PagerQuery; |
||||
|
import com.yxt.common.core.result.ResultBean; |
||||
|
import com.yxt.common.core.vo.PagerVo; |
||||
|
import com.yxt.supervise.cyf.api.productinformation.ProductInformationDto; |
||||
|
import com.yxt.supervise.cyf.api.productinformation.ProductInformationQuery; |
||||
|
import com.yxt.supervise.cyf.api.productinformation.ProductInformationVo; |
||||
|
import io.swagger.annotations.Api; |
||||
|
import io.swagger.annotations.ApiOperation; |
||||
|
import org.springframework.beans.factory.annotation.Autowired; |
||||
|
import org.springframework.web.bind.annotation.*; |
||||
|
|
||||
|
/** |
||||
|
* @author feikefei |
||||
|
* @create 2023-05-22-16:34 |
||||
|
*/ |
||||
|
@Api(tags = "产品信息") |
||||
|
@RestController |
||||
|
@RequestMapping("cyf/productInfo") |
||||
|
public class ProductInformationRest { |
||||
|
|
||||
|
@Autowired |
||||
|
private ProductInformationService productInformationService; |
||||
|
|
||||
|
@ApiOperation("添加产品信息") |
||||
|
@PostMapping("/save") |
||||
|
public ResultBean save(@RequestBody ProductInformationDto dto){ |
||||
|
return productInformationService.save(dto); |
||||
|
} |
||||
|
|
||||
|
@ApiOperation("删除") |
||||
|
@DeleteMapping("/delete/{sid}") |
||||
|
public ResultBean delete(@PathVariable String sid ){ |
||||
|
return productInformationService.del(sid); |
||||
|
} |
||||
|
|
||||
|
@ApiOperation("修改") |
||||
|
@PostMapping("/update") |
||||
|
public ResultBean update (@RequestBody ProductInformationDto dto){ |
||||
|
return productInformationService.update(dto); |
||||
|
} |
||||
|
|
||||
|
@ApiOperation("分页查询") |
||||
|
@PostMapping("/listPage") |
||||
|
public ResultBean<PagerVo<ProductInformationVo>> listPage(@RequestBody PagerQuery<ProductInformationQuery> pq){ |
||||
|
ResultBean rb = ResultBean.fireFail(); |
||||
|
PagerVo<ProductInformationVo> listPage = productInformationService.listPage(pq); |
||||
|
return rb.success().setData(listPage); |
||||
|
} |
||||
|
|
||||
|
@ApiOperation("查询") |
||||
|
@GetMapping("/selectList") |
||||
|
public ResultBean selectList(){ |
||||
|
return productInformationService.selectList(); |
||||
|
} |
||||
|
} |
@ -0,0 +1,66 @@ |
|||||
|
package com.yxt.supervise.cyf.biz.productinformation; |
||||
|
|
||||
|
import cn.hutool.core.bean.BeanUtil; |
||||
|
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||
|
import com.yxt.common.base.service.MybatisBaseService; |
||||
|
import com.yxt.common.base.utils.PagerUtil; |
||||
|
import com.yxt.common.core.query.PagerQuery; |
||||
|
import com.yxt.common.core.result.ResultBean; |
||||
|
import com.yxt.common.core.vo.PagerVo; |
||||
|
import com.yxt.supervise.cyf.api.productinformation.ProductInformation; |
||||
|
import com.yxt.supervise.cyf.api.productinformation.ProductInformationDto; |
||||
|
import com.yxt.supervise.cyf.api.productinformation.ProductInformationQuery; |
||||
|
import com.yxt.supervise.cyf.api.productinformation.ProductInformationVo; |
||||
|
import org.springframework.stereotype.Service; |
||||
|
|
||||
|
import java.util.List; |
||||
|
|
||||
|
/** |
||||
|
* @author feikefei |
||||
|
* @create 2023-05-22-17:14 |
||||
|
*/ |
||||
|
@Service |
||||
|
public class ProductInformationService extends MybatisBaseService<ProductInformationMapper, ProductInformation> { |
||||
|
|
||||
|
public ResultBean save(ProductInformationDto dto){ |
||||
|
ResultBean rb = ResultBean.fireFail(); |
||||
|
ProductInformation information = new ProductInformation(); |
||||
|
BeanUtil.copyProperties(dto,information,"id","sid"); |
||||
|
baseMapper.insert(information); |
||||
|
return rb.success().setMsg("增加成功"); |
||||
|
} |
||||
|
|
||||
|
public ResultBean del(String sid){ |
||||
|
ResultBean rb=new ResultBean(); |
||||
|
baseMapper.delete(new QueryWrapper<ProductInformation>().eq("sid",sid)); |
||||
|
return rb.success().setMsg("删除成功"); |
||||
|
} |
||||
|
|
||||
|
public ResultBean update (ProductInformationDto dto){ |
||||
|
ResultBean rb=new ResultBean(); |
||||
|
String dtoSid = dto.getSid(); |
||||
|
ProductInformation productInformation = fetchBySid(dtoSid); |
||||
|
BeanUtil.copyProperties(dto, productInformation, "id", "sid"); |
||||
|
baseMapper.updateById(productInformation); |
||||
|
return rb.success().setMsg("修改成功"); |
||||
|
} |
||||
|
|
||||
|
public PagerVo<ProductInformationVo> listPage(PagerQuery<ProductInformationQuery> pq){ |
||||
|
ProductInformationQuery params = pq.getParams(); |
||||
|
QueryWrapper<ProductInformation> qw = new QueryWrapper<>(); |
||||
|
if (params.getName() != null && !params.getName().equals("")){ |
||||
|
qw.eq("name",params.getName()); |
||||
|
} |
||||
|
IPage<ProductInformation> page = PagerUtil.queryToPage(pq); |
||||
|
IPage<ProductInformationVo> vo = baseMapper.selectPageVo(page, qw); |
||||
|
PagerVo<ProductInformationVo> objectPagerVo = PagerUtil.pageToVo(vo, null); |
||||
|
return objectPagerVo; |
||||
|
} |
||||
|
|
||||
|
public ResultBean selectList(){ |
||||
|
ResultBean rb = new ResultBean(); |
||||
|
List<ProductInformation> productInformations = baseMapper.selectList(); |
||||
|
return rb.success().setData(productInformations); |
||||
|
} |
||||
|
} |
Loading…
Reference in new issue