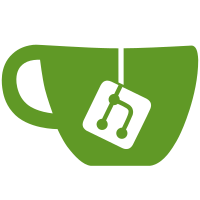
8 changed files with 315 additions and 0 deletions
@ -0,0 +1,24 @@ |
|||
package com.yxt.supervise.cyf.api.producttype; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author feikefei |
|||
* @create 2023-05-22-15:48 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "商品类型信息", description = "商品类型信息") |
|||
@TableName("product_type") |
|||
public class ProductType extends BaseEntity { |
|||
//商品类型名称
|
|||
private String name; |
|||
//商品类型编号
|
|||
private String number; |
|||
//上级sid
|
|||
private String pSid; |
|||
//产品sid
|
|||
private String prodSid; |
|||
} |
@ -0,0 +1,27 @@ |
|||
package com.yxt.supervise.cyf.api.producttype; |
|||
|
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author feikefei |
|||
* @create 2023-05-22-15:53 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "产品类型信息 数据传输对象", description = "产品类型信息 数据传输对象") |
|||
public class ProductTypeDto { |
|||
private String id; |
|||
private String sid; |
|||
|
|||
//商品类型名称
|
|||
private String name; |
|||
//商品类型编号
|
|||
private String number; |
|||
//上级sid
|
|||
private String pSid; |
|||
//产品sid
|
|||
private String prodSid; |
|||
private List<ProductTypeDto> typeList; |
|||
} |
@ -0,0 +1,25 @@ |
|||
package com.yxt.supervise.cyf.api.producttype; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author feikefei |
|||
* @create 2023-05-23-9:21 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "商品类型信息 分页传输对象数据", description = "商品类型信息 分页传输对象数据") |
|||
public class ProductTypeQuery implements Query { |
|||
private String id; |
|||
private String sid; |
|||
|
|||
//商品类型名称
|
|||
private String name; |
|||
//商品类型编号
|
|||
private String number; |
|||
//上级sid
|
|||
private String pSid; |
|||
//产品sid
|
|||
private String prodSid; |
|||
} |
@ -0,0 +1,29 @@ |
|||
package com.yxt.supervise.cyf.api.producttype; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author feikefei |
|||
* @create 2023-05-23-9:23 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "商品类型信息 视图返回字段", description = "商品类型信息 视图返回字段") |
|||
public class ProductTypeVo implements Vo { |
|||
private String id; |
|||
private String sid; |
|||
//商品类型名称
|
|||
private String name; |
|||
//商品类型编号
|
|||
private String number; |
|||
//上级sid
|
|||
private String pSid; |
|||
//产品sid
|
|||
private String prodSid; |
|||
|
|||
//品牌名称
|
|||
private String informationName; |
|||
//品牌编号
|
|||
private String informationNumber; |
|||
} |
@ -0,0 +1,28 @@ |
|||
package com.yxt.supervise.cyf.biz.producttype; |
|||
|
|||
import com.baomidou.mybatisplus.core.conditions.Wrapper; |
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import com.baomidou.mybatisplus.core.toolkit.Constants; |
|||
import com.yxt.supervise.cyf.api.producttype.ProductType; |
|||
import com.yxt.supervise.cyf.api.producttype.ProductTypeVo; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
import org.apache.ibatis.annotations.Param; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author feikefei |
|||
* @create 2023-05-23-9:04 |
|||
*/ |
|||
@Mapper |
|||
public interface ProductTypeMapper extends BaseMapper<ProductType> { |
|||
|
|||
IPage<ProductTypeVo> selectPageVo(IPage<ProductType> page, @Param(Constants.WRAPPER) Wrapper<ProductType> qw); |
|||
|
|||
List<ProductType> selectProductType(@Param("sid") String sid); |
|||
|
|||
Integer selectProductTypeByName(@Param("name") String name); |
|||
|
|||
Integer selectProductTypeByNumber(@Param("number") String number); |
|||
} |
@ -0,0 +1,23 @@ |
|||
<?xml version="1.0" encoding="UTF-8" ?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.supervise.cyf.biz.producttype.ProductTypeMapper"> |
|||
<select id="selectPageVo" resultType="com.yxt.supervise.cyf.api.producttype.ProductTypeVo"> |
|||
select p.id,p.sid,p.name,p.number,p.pSid,p.prodSid,i.name informationName,i.number informationNumber from product_type p |
|||
right join product_information i on p.prodSid = i.sid |
|||
<where> |
|||
${ew.sqlSegment} |
|||
</where> |
|||
</select> |
|||
|
|||
<select id="selectProductType" resultType="com.yxt.supervise.cyf.api.producttype.ProductType"> |
|||
select * from product_type where pSid = #{sid} |
|||
</select> |
|||
|
|||
<select id="selectProductTypeByName" resultType="int"> |
|||
select count(*) from product_type where name = #{name} |
|||
</select> |
|||
|
|||
<select id="selectProductTypeByNumber" resultType="int"> |
|||
select count(*) from product_type where number = #{number} |
|||
</select> |
|||
</mapper> |
@ -0,0 +1,58 @@ |
|||
package com.yxt.supervise.cyf.biz.producttype; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import com.yxt.supervise.cyf.api.producttype.ProductTypeDto; |
|||
import com.yxt.supervise.cyf.api.producttype.ProductTypeQuery; |
|||
import com.yxt.supervise.cyf.api.producttype.ProductTypeVo; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
|
|||
/** |
|||
* @author feikefei |
|||
* @create 2023-05-23-9:03 |
|||
*/ |
|||
@Api(tags = "商品类型信息") |
|||
@RestController |
|||
@RequestMapping("cyf/productType") |
|||
public class ProductTypeRest { |
|||
|
|||
@Autowired |
|||
private ProductTypeService productTypeService; |
|||
|
|||
@ApiOperation("增加") |
|||
@PostMapping("/save") |
|||
public ResultBean save(@RequestBody ProductTypeDto dto){ |
|||
return productTypeService.save(dto); |
|||
} |
|||
|
|||
@ApiOperation("删除") |
|||
@DeleteMapping("/delete/{sid}") |
|||
public ResultBean del(@PathVariable String sid){ |
|||
return productTypeService.del(sid); |
|||
} |
|||
|
|||
@ApiOperation("修改") |
|||
@PostMapping("/update") |
|||
public ResultBean update(@RequestBody ProductTypeDto dto){ |
|||
return productTypeService.update(dto); |
|||
} |
|||
|
|||
@ApiOperation("商品类型信息分页查询") |
|||
@PostMapping("/listPage") |
|||
public ResultBean<PagerVo<ProductTypeDto>> listPage(@RequestBody PagerQuery<ProductTypeQuery> pq){ |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
PagerVo<ProductTypeVo> vo = productTypeService.listPage(pq); |
|||
return rb.success().setData(vo); |
|||
} |
|||
|
|||
@ApiOperation("查询当前商品类别") |
|||
@GetMapping("/selectProductType/{sid}") |
|||
public ResultBean selectProductType(@PathVariable String sid){ |
|||
return productTypeService.selectProductType(sid); |
|||
} |
|||
} |
@ -0,0 +1,101 @@ |
|||
package com.yxt.supervise.cyf.biz.producttype; |
|||
|
|||
import cn.hutool.core.bean.BeanUtil; |
|||
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import com.yxt.common.base.service.MybatisBaseService; |
|||
import com.yxt.common.base.utils.PagerUtil; |
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import com.yxt.supervise.cyf.api.producttype.ProductType; |
|||
import com.yxt.supervise.cyf.api.producttype.ProductTypeDto; |
|||
import com.yxt.supervise.cyf.api.producttype.ProductTypeQuery; |
|||
import com.yxt.supervise.cyf.api.producttype.ProductTypeVo; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author feikefei |
|||
* @create 2023-05-23-9:04 |
|||
*/ |
|||
@Service |
|||
public class ProductTypeService extends MybatisBaseService<ProductTypeMapper, ProductType> { |
|||
|
|||
public ResultBean save(ProductTypeDto dto){ |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
//拿到该品牌商品下的类别
|
|||
List<ProductTypeDto> typeList = dto.getTypeList(); |
|||
if (dto.getSid() != null){ |
|||
for (ProductTypeDto productTypeDto : typeList){ |
|||
Integer integer = baseMapper.selectProductTypeByName(productTypeDto.getName()); |
|||
if (integer != 0) { |
|||
return rb.success().setMsg("该品牌商品已有此类型名称"); |
|||
} |
|||
Integer integer1 = baseMapper.selectProductTypeByNumber(productTypeDto.getNumber()); |
|||
if (integer1 != 0){ |
|||
return rb.success().setMsg("该编码已有品牌商品类型占用"); |
|||
} |
|||
ProductType type1 = new ProductType(); |
|||
BeanUtil.copyProperties(productTypeDto,type1,"id","sid"); |
|||
type1.setPSid(dto.getSid()); |
|||
baseMapper.insert(type1); |
|||
} |
|||
return rb.success().setMsg("添加成功"); |
|||
}else { |
|||
Integer integer = baseMapper.selectProductTypeByName(dto.getName()); |
|||
if (integer != 0) { |
|||
return rb.success().setMsg("该品牌已有该商品"); |
|||
} |
|||
Integer integer1 = baseMapper.selectProductTypeByNumber(dto.getNumber()); |
|||
if (integer1 != 0){ |
|||
return rb.success().setMsg("该编码已有品牌商品占用"); |
|||
} |
|||
ProductType type = new ProductType(); |
|||
BeanUtil.copyProperties(dto,type,"id","sid","typeList"); |
|||
baseMapper.insert(type); |
|||
for (ProductTypeDto productTypeDto : typeList) { |
|||
ProductType type1 = new ProductType(); |
|||
BeanUtil.copyProperties(productTypeDto,type1,"id","sid"); |
|||
type1.setPSid(type.getSid()); |
|||
baseMapper.insert(type1); |
|||
} |
|||
return rb.success().setMsg("添加成功"); |
|||
} |
|||
} |
|||
|
|||
public ResultBean del(String sid){ |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
baseMapper.delete(new QueryWrapper<ProductType>().eq("sid",sid)); |
|||
return rb.success().setMsg("删除成功"); |
|||
} |
|||
|
|||
public ResultBean update(ProductTypeDto dto){ |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
ProductType productType = fetchBySid(dto.getSid()); |
|||
BeanUtil.copyProperties(dto,productType,"id","sid"); |
|||
baseMapper.updateById(productType); |
|||
return rb.success().setMsg("修改成功"); |
|||
} |
|||
|
|||
public PagerVo<ProductTypeVo> listPage(PagerQuery<ProductTypeQuery> pq){ |
|||
ProductTypeQuery params = pq.getParams(); |
|||
QueryWrapper<ProductType> wq = new QueryWrapper<>(); |
|||
if (params != null){ |
|||
if (!params.getName().equals("")){ |
|||
wq.eq("p.name",params.getName()); |
|||
} |
|||
} |
|||
IPage<ProductType> page = PagerUtil.queryToPage(pq); |
|||
IPage<ProductTypeVo> pageVo = baseMapper.selectPageVo(page, wq); |
|||
PagerVo<ProductTypeVo> vo = PagerUtil.pageToVo(pageVo, null); |
|||
return vo; |
|||
} |
|||
|
|||
public ResultBean selectProductType(String sid){ |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
List<ProductType> productTypes = baseMapper.selectProductType(sid); |
|||
return rb.success().setData(productTypes); |
|||
} |
|||
} |
Loading…
Reference in new issue