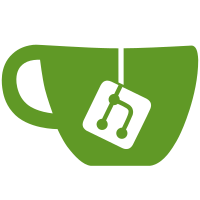
8 changed files with 260 additions and 0 deletions
@ -0,0 +1,24 @@ |
|||||
|
package com.yxt.supervise.cyf.api.finishproductinventory; |
||||
|
|
||||
|
import com.baomidou.mybatisplus.annotation.TableName; |
||||
|
import com.yxt.common.core.domain.BaseEntity; |
||||
|
import io.swagger.annotations.ApiModel; |
||||
|
import lombok.Data; |
||||
|
|
||||
|
/** |
||||
|
* @author feikefei |
||||
|
* @create 2023-05-22-16:07 |
||||
|
*/ |
||||
|
@Data |
||||
|
@ApiModel(value = "成品油 库存信息", description = "成品油 库存信息") |
||||
|
@TableName("finish_product_inventory") |
||||
|
public class FinishProductInventory extends BaseEntity { |
||||
|
//数量
|
||||
|
private Double amount; |
||||
|
//产品sid
|
||||
|
private String prodSid; |
||||
|
//编号
|
||||
|
private String number; |
||||
|
//单价
|
||||
|
private Double price; |
||||
|
} |
@ -0,0 +1,25 @@ |
|||||
|
package com.yxt.supervise.cyf.api.finishproductinventory; |
||||
|
|
||||
|
import com.yxt.common.core.dto.Dto; |
||||
|
import io.swagger.annotations.ApiModel; |
||||
|
import lombok.Data; |
||||
|
|
||||
|
/** |
||||
|
* @author feikefei |
||||
|
* @create 2023-05-22-16:12 |
||||
|
*/ |
||||
|
@Data |
||||
|
@ApiModel(value = "成品油 库存信息 传输对象数据", description = "成品油 库存信息 传输对象数据") |
||||
|
public class FinishProductInventoryDto implements Dto{ |
||||
|
private String id; |
||||
|
private String sid; |
||||
|
|
||||
|
//数量
|
||||
|
private Double amount; |
||||
|
//产品sid
|
||||
|
private String prodSid; |
||||
|
//编号
|
||||
|
private String number; |
||||
|
//单价
|
||||
|
private Double price; |
||||
|
} |
@ -0,0 +1,26 @@ |
|||||
|
package com.yxt.supervise.cyf.api.finishproductinventory; |
||||
|
|
||||
|
import com.yxt.common.core.query.Query; |
||||
|
import io.swagger.annotations.ApiModel; |
||||
|
import lombok.Data; |
||||
|
|
||||
|
/** |
||||
|
* @author feikefei |
||||
|
* @create 2023-05-23-9:36 |
||||
|
*/ |
||||
|
@Data |
||||
|
@ApiModel(value = "成品油 库存信息 分页传输对象", description = "成品油 库存信息 分页传输对象") |
||||
|
public class FinishProductInventoryQuery implements Query { |
||||
|
private String id; |
||||
|
private String sid; |
||||
|
//数量
|
||||
|
private Double amount; |
||||
|
//产品sid
|
||||
|
private String prodSid; |
||||
|
//编号
|
||||
|
private String number; |
||||
|
//单价
|
||||
|
private Double price; |
||||
|
|
||||
|
private String InformationNumber; |
||||
|
} |
@ -0,0 +1,29 @@ |
|||||
|
package com.yxt.supervise.cyf.api.finishproductinventory; |
||||
|
|
||||
|
import com.yxt.common.core.vo.Vo; |
||||
|
import io.swagger.annotations.ApiModel; |
||||
|
import lombok.Data; |
||||
|
|
||||
|
/** |
||||
|
* @author feikefei |
||||
|
* @create 2023-05-23-9:36 |
||||
|
*/ |
||||
|
@Data |
||||
|
@ApiModel(value = "成品油 库存信息 返回视图对象字段", description = "成品油 库存信息 返回视图对象字段") |
||||
|
public class FinishProductInventoryVo implements Vo { |
||||
|
private String id; |
||||
|
private String sid; |
||||
|
//数量
|
||||
|
private Double amount; |
||||
|
//产品sid
|
||||
|
private String prodSid; |
||||
|
//编号
|
||||
|
private String number; |
||||
|
//单价
|
||||
|
private Double price; |
||||
|
|
||||
|
//品牌名称
|
||||
|
private String name; |
||||
|
//品牌编号
|
||||
|
private String InformationNumber; |
||||
|
} |
@ -0,0 +1,26 @@ |
|||||
|
package com.yxt.supervise.cyf.biz.finishproductinventory; |
||||
|
|
||||
|
import com.baomidou.mybatisplus.core.conditions.Wrapper; |
||||
|
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||
|
import com.baomidou.mybatisplus.core.toolkit.Constants; |
||||
|
import com.yxt.supervise.cyf.api.finishproductinventory.FinishProductInventory; |
||||
|
import com.yxt.supervise.cyf.api.finishproductinventory.FinishProductInventoryVo; |
||||
|
import org.apache.ibatis.annotations.Mapper; |
||||
|
import org.apache.ibatis.annotations.Param; |
||||
|
import org.apache.ibatis.annotations.Select; |
||||
|
|
||||
|
/** |
||||
|
* @author feikefei |
||||
|
* @create 2023-05-23-10:45 |
||||
|
*/ |
||||
|
@Mapper |
||||
|
public interface FinishProductInventoryMapper extends BaseMapper<FinishProductInventory> { |
||||
|
|
||||
|
IPage<FinishProductInventoryVo> selectPageVo(IPage<FinishProductInventory> page, @Param(Constants.WRAPPER) Wrapper<FinishProductInventory> qw); |
||||
|
|
||||
|
FinishProductInventory selectPriceByProdSid(@Param("prodSid") String prodSid); |
||||
|
|
||||
|
@Select("select count(*) from finish_product_inventory where prodSid = #{prodSid)") |
||||
|
Integer selectByProdSid(@Param("prodSid") String prodSid); |
||||
|
} |
@ -0,0 +1,16 @@ |
|||||
|
<?xml version="1.0" encoding="UTF-8" ?> |
||||
|
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
||||
|
<mapper namespace="com.yxt.supervise.cyf.biz.finishproductinventory.FinishProductInventoryMapper"> |
||||
|
<select id="selectPageVo" resultType="com.yxt.supervise.cyf.api.finishproductinventory.FinishProductInventoryVo"> |
||||
|
select p.*,i.name,i.number InformationNumber |
||||
|
from finish_product_inventory p |
||||
|
inner join product_information i on p.prodSid = i.sid |
||||
|
<where> |
||||
|
${ew.sqlSegment} |
||||
|
</where> |
||||
|
</select> |
||||
|
|
||||
|
<select id="selectPriceByProdSid" resultType="com.yxt.supervise.cyf.api.finishproductinventory.FinishProductInventory"> |
||||
|
select * from finish_product_inventory where prodSid = #{prodSid} |
||||
|
</select> |
||||
|
</mapper> |
@ -0,0 +1,51 @@ |
|||||
|
package com.yxt.supervise.cyf.biz.finishproductinventory; |
||||
|
|
||||
|
import com.yxt.common.core.query.PagerQuery; |
||||
|
import com.yxt.common.core.result.ResultBean; |
||||
|
import com.yxt.common.core.vo.PagerVo; |
||||
|
import com.yxt.supervise.cyf.api.finishproductinventory.FinishProductInventoryDto; |
||||
|
import com.yxt.supervise.cyf.api.finishproductinventory.FinishProductInventoryQuery; |
||||
|
import com.yxt.supervise.cyf.api.finishproductinventory.FinishProductInventoryVo; |
||||
|
import io.swagger.annotations.Api; |
||||
|
import io.swagger.annotations.ApiOperation; |
||||
|
import org.springframework.beans.factory.annotation.Autowired; |
||||
|
import org.springframework.web.bind.annotation.*; |
||||
|
|
||||
|
/** |
||||
|
* @author feikefei |
||||
|
* @create 2023-05-23-10:42 |
||||
|
*/ |
||||
|
@Api(tags = "成品油 库存信息") |
||||
|
@RestController |
||||
|
@RequestMapping("cyf/inventory") |
||||
|
public class FinishProductInventoryRest { |
||||
|
|
||||
|
@Autowired |
||||
|
private FinishProductInventoryService finishProductInventoryService; |
||||
|
|
||||
|
@ApiOperation("增加") |
||||
|
@PostMapping("/save") |
||||
|
public ResultBean save(@RequestBody FinishProductInventoryDto dto){ |
||||
|
return finishProductInventoryService.save(dto); |
||||
|
} |
||||
|
|
||||
|
@ApiOperation("删除") |
||||
|
@GetMapping("/delete/{sid}") |
||||
|
public ResultBean delete(@PathVariable String sid){ |
||||
|
return finishProductInventoryService.delete(sid); |
||||
|
} |
||||
|
|
||||
|
@ApiOperation("修改") |
||||
|
@PostMapping("/update") |
||||
|
public ResultBean update(@RequestBody FinishProductInventoryDto dto){ |
||||
|
return finishProductInventoryService.update(dto); |
||||
|
} |
||||
|
|
||||
|
@ApiOperation("成品库记录 分页查询") |
||||
|
@PostMapping("/listPage") |
||||
|
public ResultBean<PagerVo<FinishProductInventoryVo>> listPage(PagerQuery<FinishProductInventoryQuery> pq){ |
||||
|
ResultBean rb = ResultBean.fireFail(); |
||||
|
PagerVo<FinishProductInventoryVo> listPage = finishProductInventoryService.listPage(pq); |
||||
|
return rb.success().setData(listPage); |
||||
|
} |
||||
|
} |
@ -0,0 +1,63 @@ |
|||||
|
package com.yxt.supervise.cyf.biz.finishproductinventory; |
||||
|
|
||||
|
import cn.hutool.core.bean.BeanUtil; |
||||
|
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||
|
import com.yxt.common.base.service.MybatisBaseService; |
||||
|
import com.yxt.common.base.utils.PagerUtil; |
||||
|
import com.yxt.common.core.query.PagerQuery; |
||||
|
import com.yxt.common.core.result.ResultBean; |
||||
|
import com.yxt.common.core.vo.PagerVo; |
||||
|
import com.yxt.supervise.cyf.api.finishproductinventory.FinishProductInventory; |
||||
|
import com.yxt.supervise.cyf.api.finishproductinventory.FinishProductInventoryDto; |
||||
|
import com.yxt.supervise.cyf.api.finishproductinventory.FinishProductInventoryQuery; |
||||
|
import com.yxt.supervise.cyf.api.finishproductinventory.FinishProductInventoryVo; |
||||
|
import org.springframework.stereotype.Service; |
||||
|
|
||||
|
/** |
||||
|
* @author feikefei |
||||
|
* @create 2023-05-23-10:45 |
||||
|
*/ |
||||
|
@Service |
||||
|
public class FinishProductInventoryService extends MybatisBaseService<FinishProductInventoryMapper, FinishProductInventory> { |
||||
|
|
||||
|
public ResultBean save(FinishProductInventoryDto dto){ |
||||
|
ResultBean rb = ResultBean.fireFail(); |
||||
|
Integer integer = baseMapper.selectByProdSid(dto.getProdSid()); |
||||
|
if (integer != 0){ |
||||
|
return rb.success().setMsg("该品牌已存在库存"); |
||||
|
} |
||||
|
FinishProductInventory record = new FinishProductInventory(); |
||||
|
BeanUtil.copyProperties(dto,record,"id","sid"); |
||||
|
baseMapper.insert(record); |
||||
|
return rb.success().setMsg("添加成功"); |
||||
|
} |
||||
|
|
||||
|
public ResultBean delete(String sid){ |
||||
|
ResultBean rb = ResultBean.fireFail(); |
||||
|
baseMapper.delete(new QueryWrapper<FinishProductInventory>().eq("sid",sid)); |
||||
|
return rb.success().setMsg("删除成功"); |
||||
|
} |
||||
|
|
||||
|
public ResultBean update(FinishProductInventoryDto dto){ |
||||
|
ResultBean rb = ResultBean.fireFail(); |
||||
|
FinishProductInventory finishProductInventory = fetchBySid(dto.getSid()); |
||||
|
BeanUtil.copyProperties(dto,finishProductInventory,"id","sid"); |
||||
|
baseMapper.updateById(finishProductInventory); |
||||
|
return rb.success().setMsg("修改成功"); |
||||
|
} |
||||
|
|
||||
|
public PagerVo<FinishProductInventoryVo> listPage(PagerQuery<FinishProductInventoryQuery> pq){ |
||||
|
FinishProductInventoryQuery params = pq.getParams(); |
||||
|
QueryWrapper<FinishProductInventory> wq = new QueryWrapper<>(); |
||||
|
if (params != null){ |
||||
|
if (!params.getInformationNumber().equals("")){ |
||||
|
wq.eq("i.number",params.getInformationNumber()); |
||||
|
} |
||||
|
} |
||||
|
IPage<FinishProductInventory> page = PagerUtil.queryToPage(pq); |
||||
|
IPage<FinishProductInventoryVo> pageVo = baseMapper.selectPageVo(page, wq); |
||||
|
PagerVo<FinishProductInventoryVo> pageToVo = PagerUtil.pageToVo(pageVo, null); |
||||
|
return pageToVo; |
||||
|
} |
||||
|
} |
Loading…
Reference in new issue