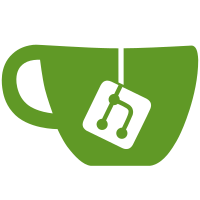
160 changed files with 8803 additions and 21 deletions
@ -0,0 +1,72 @@ |
|||
package com.yxt.supervise.customer.api.gdinstoragegd; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import com.yxt.common.core.domain.EntityWithId; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/29 13:34 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "入库数据", description = "入库数据") |
|||
@TableName("gd_instorage_gd") |
|||
public class GdInstorageGd extends EntityWithId { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String cola0; // 企业组织机构代码证
|
|||
@ApiModelProperty("仓库/门店编号") |
|||
private String colb1; // 仓库/门店编号
|
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String colc2; // 仓库/门店名称
|
|||
@ApiModelProperty("入库时间") |
|||
private String cold3; // 入库时间
|
|||
@ApiModelProperty("供应商编号") |
|||
private String colex; // 供应商编号
|
|||
@ApiModelProperty("供应商名称") |
|||
private String cole4; // 供应商名称
|
|||
@ApiModelProperty("单据号") |
|||
private String colf5; // 单据号
|
|||
@ApiModelProperty("单据类型") |
|||
private String colg6; // 单据类型
|
|||
@ApiModelProperty("货号") |
|||
private String colh7; // 货号
|
|||
@ApiModelProperty("商品名称") |
|||
private String coli8; // 商品名称
|
|||
@ApiModelProperty("商品生产日期") |
|||
private String colj9; // 商品生产日期
|
|||
@ApiModelProperty("商品保质期") |
|||
private String colk10; // 商品保质期
|
|||
@ApiModelProperty("商品规格") |
|||
private String coll11; // 商品规格
|
|||
@ApiModelProperty("类别编号") |
|||
private String colm12; // 类别编号
|
|||
@ApiModelProperty("类别名称") |
|||
private String coln13; // 类别名称
|
|||
@ApiModelProperty("一级类别名称") |
|||
private String colo14; // 一级类别名称
|
|||
@ApiModelProperty("二级类别名称") |
|||
private String colp15; // 二级类别名称
|
|||
@ApiModelProperty("入库金额") |
|||
private String colq16; // 入库金额
|
|||
@ApiModelProperty("入库数量") |
|||
private String colr17; // 入库数量
|
|||
@ApiModelProperty("成本金额") |
|||
private String cols18; // 成本金额
|
|||
@ApiModelProperty("成本税额") |
|||
private String colt19; // 成本税额
|
|||
@ApiModelProperty("不含税成本") |
|||
private String colu20; // 不含税成本
|
|||
@ApiModelProperty("进项税率") |
|||
private String colv21; // 进项税率
|
|||
@ApiModelProperty("单据日期") |
|||
private String orderDate; // 单据日期
|
|||
@ApiModelProperty("供货商编码统一") |
|||
private String supplierCodeUnified; // 供货商编码统一
|
|||
@ApiModelProperty("入库定单号") |
|||
private String inOrderNo; // 入库定单号
|
|||
} |
@ -0,0 +1,67 @@ |
|||
package com.yxt.supervise.customer.api.gdinstoragegd; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/29 13:40 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "入库数据 视图数据详情", description = "入库数据 视图数据详情") |
|||
public class GdInstorageGdDetailsVo implements Vo { |
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String cola0; // 企业组织机构代码证
|
|||
@ApiModelProperty("仓库/门店编号") |
|||
private String colb1; // 仓库/门店编号
|
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String colc2; // 仓库/门店名称
|
|||
@ApiModelProperty("入库时间") |
|||
private String cold3; // 入库时间
|
|||
@ApiModelProperty("供应商编号") |
|||
private String colex; // 供应商编号
|
|||
@ApiModelProperty("供应商名称") |
|||
private String cole4; // 供应商名称
|
|||
@ApiModelProperty("单据号") |
|||
private String colf5; // 单据号
|
|||
@ApiModelProperty("单据类型") |
|||
private String colg6; // 单据类型
|
|||
@ApiModelProperty("货号") |
|||
private String colh7; // 货号
|
|||
@ApiModelProperty("商品名称") |
|||
private String coli8; // 商品名称
|
|||
@ApiModelProperty("商品生产日期") |
|||
private String colj9; // 商品生产日期
|
|||
@ApiModelProperty("商品保质期") |
|||
private String colk10; // 商品保质期
|
|||
@ApiModelProperty("商品规格") |
|||
private String coll11; // 商品规格
|
|||
@ApiModelProperty("类别编号") |
|||
private String colm12; // 类别编号
|
|||
@ApiModelProperty("类别名称") |
|||
private String coln13; // 类别名称
|
|||
@ApiModelProperty("一级类别名称") |
|||
private String colo14; // 一级类别名称
|
|||
@ApiModelProperty("二级类别名称") |
|||
private String colp15; // 二级类别名称
|
|||
@ApiModelProperty("入库金额") |
|||
private String colq16; // 入库金额
|
|||
@ApiModelProperty("入库数量") |
|||
private String colr17; // 入库数量
|
|||
@ApiModelProperty("成本金额") |
|||
private String cols18; // 成本金额
|
|||
@ApiModelProperty("成本税额") |
|||
private String colt19; // 成本税额
|
|||
@ApiModelProperty("不含税成本") |
|||
private String colu20; // 不含税成本
|
|||
@ApiModelProperty("进项税率") |
|||
private String colv21; // 进项税率
|
|||
@ApiModelProperty("单据日期") |
|||
private String orderDate; // 单据日期
|
|||
@ApiModelProperty("供货商编码统一") |
|||
private String supplierCodeUnified; // 供货商编码统一
|
|||
@ApiModelProperty("入库定单号") |
|||
private String inOrderNo; // 入库定单号
|
|||
} |
@ -0,0 +1,67 @@ |
|||
package com.yxt.supervise.customer.api.gdinstoragegd; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/29 13:46 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "入库数据 数据传输对象", description = "入库数据 数据传输对象") |
|||
public class GdInstorageGdDto implements Dto { |
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String cola0; // 企业组织机构代码证
|
|||
@ApiModelProperty("仓库/门店编号") |
|||
private String colb1; // 仓库/门店编号
|
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String colc2; // 仓库/门店名称
|
|||
@ApiModelProperty("入库时间") |
|||
private String cold3; // 入库时间
|
|||
@ApiModelProperty("供应商编号") |
|||
private String colex; // 供应商编号
|
|||
@ApiModelProperty("供应商名称") |
|||
private String cole4; // 供应商名称
|
|||
@ApiModelProperty("单据号") |
|||
private String colf5; // 单据号
|
|||
@ApiModelProperty("单据类型") |
|||
private String colg6; // 单据类型
|
|||
@ApiModelProperty("货号") |
|||
private String colh7; // 货号
|
|||
@ApiModelProperty("商品名称") |
|||
private String coli8; // 商品名称
|
|||
@ApiModelProperty("商品生产日期") |
|||
private String colj9; // 商品生产日期
|
|||
@ApiModelProperty("商品保质期") |
|||
private String colk10; // 商品保质期
|
|||
@ApiModelProperty("商品规格") |
|||
private String coll11; // 商品规格
|
|||
@ApiModelProperty("类别编号") |
|||
private String colm12; // 类别编号
|
|||
@ApiModelProperty("类别名称") |
|||
private String coln13; // 类别名称
|
|||
@ApiModelProperty("一级类别名称") |
|||
private String colo14; // 一级类别名称
|
|||
@ApiModelProperty("二级类别名称") |
|||
private String colp15; // 二级类别名称
|
|||
@ApiModelProperty("入库金额") |
|||
private String colq16; // 入库金额
|
|||
@ApiModelProperty("入库数量") |
|||
private String colr17; // 入库数量
|
|||
@ApiModelProperty("成本金额") |
|||
private String cols18; // 成本金额
|
|||
@ApiModelProperty("成本税额") |
|||
private String colt19; // 成本税额
|
|||
@ApiModelProperty("不含税成本") |
|||
private String colu20; // 不含税成本
|
|||
@ApiModelProperty("进项税率") |
|||
private String colv21; // 进项税率
|
|||
@ApiModelProperty("单据日期") |
|||
private String orderDate; // 单据日期
|
|||
@ApiModelProperty("供货商编码统一") |
|||
private String supplierCodeUnified; // 供货商编码统一
|
|||
@ApiModelProperty("入库定单号") |
|||
private String inOrderNo; // 入库定单号
|
|||
} |
@ -0,0 +1,37 @@ |
|||
package com.yxt.supervise.customer.api.gdinstoragegd; |
|||
|
|||
import com.alibaba.excel.annotation.ExcelProperty; |
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/29 14:56 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "入库数据 Excel视图数据对象", description = "入库数据 Excel视图数据对象") |
|||
public class GdInstorageGdExcelVo implements Vo { |
|||
@ExcelProperty(value = "仓库/门店名称") |
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String colc2; // 仓库/门店名称
|
|||
@ApiModelProperty("入库时间") |
|||
@ExcelProperty(value = "入库时间") |
|||
private String cold3; // 入库时间
|
|||
@ApiModelProperty("商品名称") |
|||
@ExcelProperty(value = "商品名称") |
|||
private String coli8; // 商品名称
|
|||
@ExcelProperty(value = "商品规格") |
|||
@ApiModelProperty("商品规格") |
|||
private String coll11; // 商品规格
|
|||
@ExcelProperty(value = "类别编号") |
|||
@ApiModelProperty("类别编号") |
|||
private String colm12; // 类别编号
|
|||
@ExcelProperty(value = "类别名称") |
|||
@ApiModelProperty("类别名称") |
|||
private String coln13; // 类别名称
|
|||
@ExcelProperty(value = "入库数量") |
|||
@ApiModelProperty("入库数量") |
|||
private String colr17; // 入库数量
|
|||
} |
@ -0,0 +1,29 @@ |
|||
package com.yxt.supervise.customer.api.gdinstoragegd; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
import org.springframework.web.bind.annotation.PostMapping; |
|||
import org.springframework.web.bind.annotation.RequestBody; |
|||
import org.springframework.web.bind.annotation.ResponseBody; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/29 13:47 |
|||
*/ |
|||
@Api(tags = "入库数据") |
|||
@FeignClient( |
|||
contextId = "yxt-supervise-GdInstorageGd", |
|||
name = "yxt-supervise", |
|||
path = "v1/gdinstoragegd", |
|||
fallback = GdInstorageGdFeignFallback.class) |
|||
public interface GdInstorageGdFeign { |
|||
@ApiOperation("根据条件分页查询数据的列表") |
|||
@PostMapping("/listPage") |
|||
@ResponseBody |
|||
public ResultBean<PagerVo<GdInstorageGd>> listPage(@RequestBody PagerQuery<GdInstorageGdQuery> pq); |
|||
|
|||
} |
@ -0,0 +1,21 @@ |
|||
package com.yxt.supervise.customer.api.gdinstoragegd; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import org.springframework.stereotype.Component; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/29 13:48 |
|||
*/ |
|||
@Component |
|||
public class GdInstorageGdFeignFallback implements GdInstorageGdFeign{ |
|||
|
|||
@Override |
|||
public ResultBean<PagerVo<GdInstorageGd>> listPage(PagerQuery<GdInstorageGdQuery> pq) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
return rb.setMsg("接口yxt-supervise/gdinstoragegd/listPage无法访问"); |
|||
|
|||
} |
|||
} |
@ -0,0 +1,71 @@ |
|||
package com.yxt.supervise.customer.api.gdinstoragegd; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/29 13:50 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "入库数据 查询条件", description = "入库数据 查询条件") |
|||
public class GdInstorageGdQuery implements Query { |
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String cola0; // 企业组织机构代码证
|
|||
@ApiModelProperty("仓库/门店编号") |
|||
private String colb1; // 仓库/门店编号
|
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String colc2; // 仓库/门店名称
|
|||
@ApiModelProperty("入库时间") |
|||
private String cold3; // 入库时间
|
|||
@ApiModelProperty("供应商编号") |
|||
private String colex; // 供应商编号
|
|||
@ApiModelProperty("供应商名称") |
|||
private String cole4; // 供应商名称
|
|||
@ApiModelProperty("单据号") |
|||
private String colf5; // 单据号
|
|||
@ApiModelProperty("单据类型") |
|||
private String colg6; // 单据类型
|
|||
@ApiModelProperty("货号") |
|||
private String colh7; // 货号
|
|||
@ApiModelProperty("商品名称") |
|||
private String coli8; // 商品名称
|
|||
@ApiModelProperty("商品生产日期") |
|||
private String colj9; // 商品生产日期
|
|||
@ApiModelProperty("商品保质期") |
|||
private String colk10; // 商品保质期
|
|||
@ApiModelProperty("商品规格") |
|||
private String coll11; // 商品规格
|
|||
@ApiModelProperty("类别编号") |
|||
private String colm12; // 类别编号
|
|||
@ApiModelProperty("类别名称") |
|||
private String coln13; // 类别名称
|
|||
@ApiModelProperty("一级类别名称") |
|||
private String colo14; // 一级类别名称
|
|||
@ApiModelProperty("二级类别名称") |
|||
private String colp15; // 二级类别名称
|
|||
@ApiModelProperty("入库金额") |
|||
private String colq16; // 入库金额
|
|||
@ApiModelProperty("入库数量") |
|||
private String colr17; // 入库数量
|
|||
@ApiModelProperty("成本金额") |
|||
private String cols18; // 成本金额
|
|||
@ApiModelProperty("成本税额") |
|||
private String colt19; // 成本税额
|
|||
@ApiModelProperty("不含税成本") |
|||
private String colu20; // 不含税成本
|
|||
@ApiModelProperty("进项税率") |
|||
private String colv21; // 进项税率
|
|||
@ApiModelProperty("单据日期") |
|||
private String orderDate; // 单据日期
|
|||
@ApiModelProperty("供货商编码统一") |
|||
private String supplierCodeUnified; // 供货商编码统一
|
|||
@ApiModelProperty("入库定单号") |
|||
private String inOrderNo; // 入库定单号
|
|||
@ApiModelProperty("开始时间") |
|||
private String startTime; |
|||
@ApiModelProperty("结束时间") |
|||
private String endTime; |
|||
} |
@ -0,0 +1,67 @@ |
|||
package com.yxt.supervise.customer.api.gdinstoragegd; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/29 13:51 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "入库数据 视图数据对象", description = "入库数据 视图数据对象") |
|||
public class GdInstorageGdVo implements Vo { |
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String cola0; // 企业组织机构代码证
|
|||
@ApiModelProperty("仓库/门店编号") |
|||
private String colb1; // 仓库/门店编号
|
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String colc2; // 仓库/门店名称
|
|||
@ApiModelProperty("入库时间") |
|||
private String cold3; // 入库时间
|
|||
@ApiModelProperty("供应商编号") |
|||
private String colex; // 供应商编号
|
|||
@ApiModelProperty("供应商名称") |
|||
private String cole4; // 供应商名称
|
|||
@ApiModelProperty("单据号") |
|||
private String colf5; // 单据号
|
|||
@ApiModelProperty("单据类型") |
|||
private String colg6; // 单据类型
|
|||
@ApiModelProperty("货号") |
|||
private String colh7; // 货号
|
|||
@ApiModelProperty("商品名称") |
|||
private String coli8; // 商品名称
|
|||
@ApiModelProperty("商品生产日期") |
|||
private String colj9; // 商品生产日期
|
|||
@ApiModelProperty("商品保质期") |
|||
private String colk10; // 商品保质期
|
|||
@ApiModelProperty("商品规格") |
|||
private String coll11; // 商品规格
|
|||
@ApiModelProperty("类别编号") |
|||
private String colm12; // 类别编号
|
|||
@ApiModelProperty("类别名称") |
|||
private String coln13; // 类别名称
|
|||
@ApiModelProperty("一级类别名称") |
|||
private String colo14; // 一级类别名称
|
|||
@ApiModelProperty("二级类别名称") |
|||
private String colp15; // 二级类别名称
|
|||
@ApiModelProperty("入库金额") |
|||
private String colq16; // 入库金额
|
|||
@ApiModelProperty("入库数量") |
|||
private String colr17; // 入库数量
|
|||
@ApiModelProperty("成本金额") |
|||
private String cols18; // 成本金额
|
|||
@ApiModelProperty("成本税额") |
|||
private String colt19; // 成本税额
|
|||
@ApiModelProperty("不含税成本") |
|||
private String colu20; // 不含税成本
|
|||
@ApiModelProperty("进项税率") |
|||
private String colv21; // 进项税率
|
|||
@ApiModelProperty("单据日期") |
|||
private String orderDate; // 单据日期
|
|||
@ApiModelProperty("供货商编码统一") |
|||
private String supplierCodeUnified; // 供货商编码统一
|
|||
@ApiModelProperty("入库定单号") |
|||
private String inOrderNo; // 入库定单号
|
|||
} |
@ -0,0 +1,74 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.supervise.customer.api.gdinstoragejmd; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.EntityWithId; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: yxt_supervise <br/> |
|||
* File: GdInstorageJmd.java <br/> |
|||
* Class: com.yxt.supervise.portal.biz.gdinstorage.GdInstorageJmd <br/> |
|||
* Description: <描述类的功能>. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2023/1/5 10:45 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@TableName("gd_instorage_jmd") |
|||
public class GdInstorageJmd extends EntityWithId { |
|||
private String cola0; // 企业组织机构代码证
|
|||
private String colb1; // 仓库/门店编号
|
|||
private String colc2; // 仓库/门店名称
|
|||
private String cold3; // 入库时间
|
|||
private String colex; // 供应商编号
|
|||
private String cole4; // 供应商名称
|
|||
private String colf5; // 单据号
|
|||
private String colg6; // 单据类型
|
|||
private String colh7; // 货号
|
|||
private String coli8; // 商品名称
|
|||
private String colj9; // 商品生产日期
|
|||
private String colk10; // 商品保质期
|
|||
private String coll11; // 商品规格
|
|||
private String colm12; // 类别编号
|
|||
private String coln13; // 类别名称
|
|||
private String colo14; // 一级类别名称
|
|||
private String colp15; // 二级类别名称
|
|||
private String colq16; // 入库金额
|
|||
private String colr17; // 入库数量
|
|||
private String cols18; // 成本金额
|
|||
private String colt19; // 成本税额
|
|||
private String colu20; // 不含税成本
|
|||
private String colv21; // 进项税率
|
|||
private String orderDate; // 单据日期
|
|||
private String supplierCodeUnified; // 供货商编码统一
|
|||
private String inOrderNo; // 入库定单号
|
|||
} |
@ -0,0 +1,33 @@ |
|||
package com.yxt.supervise.customer.api.gdinstoragelog; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import com.yxt.common.core.domain.EntityWithId; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
|
|||
@Data |
|||
@TableName("gd_instorage_log") |
|||
public class GdInstorageLog extends EntityWithId { |
|||
|
|||
public GdInstorageLog() { |
|||
} |
|||
|
|||
public GdInstorageLog(String fileFullPath) { |
|||
this.fileFullPath = fileFullPath; |
|||
} |
|||
|
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss", timezone = "GMT+8") |
|||
private Date createTime = new Date(); // 记录创建时间/
|
|||
private String remarks; // 备注说明/
|
|||
private String fileFullPath; // 文件完整路径/
|
|||
private String fileUrl; //文件下载地址
|
|||
private String outFilePath; // 汇总文件路径
|
|||
// private String outfile; //输出文件名
|
|||
private int allNum; // 总记录数/
|
|||
private int validNum; // 有效记录数/
|
|||
private long durations; // 程序运行时长/
|
|||
private int errRowNum; // 出错的条数/
|
|||
private String orderDate; // 单据日期
|
|||
} |
@ -0,0 +1,25 @@ |
|||
package com.yxt.supervise.customer.api.gdinstoragelogerr; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.EntityWithId; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
|
|||
@Data |
|||
@TableName("gd_instorage_log_err") |
|||
public class GdInstorageLogErr extends EntityWithId { |
|||
private Date createTime = new Date(); // 记录创建时间',
|
|||
private String remarks; // 备注说明',
|
|||
private String fileFullPath; // 文件完整路径',
|
|||
private String errInfo; // 异常信息',
|
|||
private String rowContent; // 原记录内容',
|
|||
private int rowNum; // 出错行数',
|
|||
private String prodCode; //商品编码
|
|||
private String prodName; //商品名称
|
|||
private String prodNum; //'商品数量
|
|||
private String prodValue; //商品货值
|
|||
private String supplierCode; //供货商编码
|
|||
private String supplierName; //供货商名称
|
|||
private String orderDate; // 单据日期
|
|||
} |
@ -0,0 +1,72 @@ |
|||
package com.yxt.supervise.customer.api.gdinstorageyc; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.EntityWithId; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 10:00 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "入库数据(烟草)", description = "入库数据(烟草)") |
|||
@TableName("gd_instorage_yc") |
|||
public class GdInstorageYc extends EntityWithId { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String cola0; // 企业组织机构代码证
|
|||
@ApiModelProperty("仓库/门店编号") |
|||
private String colb1; // 仓库/门店编号
|
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String colc2; // 仓库/门店名称
|
|||
@ApiModelProperty("入库时间") |
|||
private String cold3; // 入库时间
|
|||
@ApiModelProperty("供应商编号") |
|||
private String colex; // 供应商编号
|
|||
@ApiModelProperty("供应商名称") |
|||
private String cole4; // 供应商名称
|
|||
@ApiModelProperty("单据号") |
|||
private String colf5; // 单据号
|
|||
@ApiModelProperty("单据类型") |
|||
private String colg6; // 单据类型
|
|||
@ApiModelProperty("货号") |
|||
private String colh7; // 货号
|
|||
@ApiModelProperty("商品名称") |
|||
private String coli8; // 商品名称
|
|||
@ApiModelProperty("商品生产日期") |
|||
private String colj9; // 商品生产日期
|
|||
@ApiModelProperty("商品保质期") |
|||
private String colk10; // 商品保质期
|
|||
@ApiModelProperty("商品规格") |
|||
private String coll11; // 商品规格
|
|||
@ApiModelProperty("类别编号") |
|||
private String colm12; // 类别编号
|
|||
@ApiModelProperty("类别名称") |
|||
private String coln13; // 类别名称
|
|||
@ApiModelProperty("一级类别名称") |
|||
private String colo14; // 一级类别名称
|
|||
@ApiModelProperty("二级类别名称") |
|||
private String colp15; // 二级类别名称
|
|||
@ApiModelProperty("入库金额") |
|||
private String colq16; // 入库金额
|
|||
@ApiModelProperty("入库数量") |
|||
private String colr17; // 入库数量
|
|||
@ApiModelProperty("成本金额") |
|||
private String cols18; // 成本金额
|
|||
@ApiModelProperty("成本税额") |
|||
private String colt19; // 成本税额
|
|||
@ApiModelProperty("不含税成本") |
|||
private String colu20; // 不含税成本
|
|||
@ApiModelProperty("进项税率") |
|||
private String colv21; // 进项税率
|
|||
@ApiModelProperty("单据日期") |
|||
private String orderDate; // 单据日期
|
|||
@ApiModelProperty("供货商编码统一") |
|||
private String supplierCodeUnified; // 供货商编码统一
|
|||
@ApiModelProperty("入库定单号") |
|||
private String inOrderNo; // 入库定单号
|
|||
private String purchaseDate; // 采购日期
|
|||
} |
@ -0,0 +1,67 @@ |
|||
package com.yxt.supervise.customer.api.gdinstorageyc; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 10:01 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "入库数据(烟草) 视图数据详情", description = "入库数据(烟草) 视图数据详情") |
|||
public class GdInstorageYcDetailsVo implements Vo { |
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String cola0; // 企业组织机构代码证
|
|||
@ApiModelProperty("仓库/门店编号") |
|||
private String colb1; // 仓库/门店编号
|
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String colc2; // 仓库/门店名称
|
|||
@ApiModelProperty("入库时间") |
|||
private String cold3; // 入库时间
|
|||
@ApiModelProperty("供应商编号") |
|||
private String colex; // 供应商编号
|
|||
@ApiModelProperty("供应商名称") |
|||
private String cole4; // 供应商名称
|
|||
@ApiModelProperty("单据号") |
|||
private String colf5; // 单据号
|
|||
@ApiModelProperty("单据类型") |
|||
private String colg6; // 单据类型
|
|||
@ApiModelProperty("货号") |
|||
private String colh7; // 货号
|
|||
@ApiModelProperty("商品名称") |
|||
private String coli8; // 商品名称
|
|||
@ApiModelProperty("商品生产日期") |
|||
private String colj9; // 商品生产日期
|
|||
@ApiModelProperty("商品保质期") |
|||
private String colk10; // 商品保质期
|
|||
@ApiModelProperty("商品规格") |
|||
private String coll11; // 商品规格
|
|||
@ApiModelProperty("类别编号") |
|||
private String colm12; // 类别编号
|
|||
@ApiModelProperty("类别名称") |
|||
private String coln13; // 类别名称
|
|||
@ApiModelProperty("一级类别名称") |
|||
private String colo14; // 一级类别名称
|
|||
@ApiModelProperty("二级类别名称") |
|||
private String colp15; // 二级类别名称
|
|||
@ApiModelProperty("入库金额") |
|||
private String colq16; // 入库金额
|
|||
@ApiModelProperty("入库数量") |
|||
private String colr17; // 入库数量
|
|||
@ApiModelProperty("成本金额") |
|||
private String cols18; // 成本金额
|
|||
@ApiModelProperty("成本税额") |
|||
private String colt19; // 成本税额
|
|||
@ApiModelProperty("不含税成本") |
|||
private String colu20; // 不含税成本
|
|||
@ApiModelProperty("进项税率") |
|||
private String colv21; // 进项税率
|
|||
@ApiModelProperty("单据日期") |
|||
private String orderDate; // 单据日期
|
|||
@ApiModelProperty("供货商编码统一") |
|||
private String supplierCodeUnified; // 供货商编码统一
|
|||
@ApiModelProperty("入库定单号") |
|||
private String inOrderNo; // 入库定单号
|
|||
} |
@ -0,0 +1,67 @@ |
|||
package com.yxt.supervise.customer.api.gdinstorageyc; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 10:01 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "入库数据(烟草) 数据传输对象", description = "入库数据(烟草) 数据传输对象") |
|||
public class GdInstorageYcDto implements Dto { |
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String cola0; // 企业组织机构代码证
|
|||
@ApiModelProperty("仓库/门店编号") |
|||
private String colb1; // 仓库/门店编号
|
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String colc2; // 仓库/门店名称
|
|||
@ApiModelProperty("入库时间") |
|||
private String cold3; // 入库时间
|
|||
@ApiModelProperty("供应商编号") |
|||
private String colex; // 供应商编号
|
|||
@ApiModelProperty("供应商名称") |
|||
private String cole4; // 供应商名称
|
|||
@ApiModelProperty("单据号") |
|||
private String colf5; // 单据号
|
|||
@ApiModelProperty("单据类型") |
|||
private String colg6; // 单据类型
|
|||
@ApiModelProperty("货号") |
|||
private String colh7; // 货号
|
|||
@ApiModelProperty("商品名称") |
|||
private String coli8; // 商品名称
|
|||
@ApiModelProperty("商品生产日期") |
|||
private String colj9; // 商品生产日期
|
|||
@ApiModelProperty("商品保质期") |
|||
private String colk10; // 商品保质期
|
|||
@ApiModelProperty("商品规格") |
|||
private String coll11; // 商品规格
|
|||
@ApiModelProperty("类别编号") |
|||
private String colm12; // 类别编号
|
|||
@ApiModelProperty("类别名称") |
|||
private String coln13; // 类别名称
|
|||
@ApiModelProperty("一级类别名称") |
|||
private String colo14; // 一级类别名称
|
|||
@ApiModelProperty("二级类别名称") |
|||
private String colp15; // 二级类别名称
|
|||
@ApiModelProperty("入库金额") |
|||
private String colq16; // 入库金额
|
|||
@ApiModelProperty("入库数量") |
|||
private String colr17; // 入库数量
|
|||
@ApiModelProperty("成本金额") |
|||
private String cols18; // 成本金额
|
|||
@ApiModelProperty("成本税额") |
|||
private String colt19; // 成本税额
|
|||
@ApiModelProperty("不含税成本") |
|||
private String colu20; // 不含税成本
|
|||
@ApiModelProperty("进项税率") |
|||
private String colv21; // 进项税率
|
|||
@ApiModelProperty("单据日期") |
|||
private String orderDate; // 单据日期
|
|||
@ApiModelProperty("供货商编码统一") |
|||
private String supplierCodeUnified; // 供货商编码统一
|
|||
@ApiModelProperty("入库定单号") |
|||
private String inOrderNo; // 入库定单号
|
|||
} |
@ -0,0 +1,37 @@ |
|||
package com.yxt.supervise.customer.api.gdinstorageyc; |
|||
|
|||
import com.alibaba.excel.annotation.ExcelProperty; |
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 10:01 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "入库数据(烟草) Excel视图数据对象", description = "入库数据(烟草) Excel视图数据对象") |
|||
public class GdInstorageYcExcelVo implements Vo { |
|||
@ExcelProperty(value = "仓库/门店名称") |
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String colc2; // 仓库/门店名称
|
|||
@ApiModelProperty("入库时间") |
|||
@ExcelProperty(value = "入库时间") |
|||
private String cold3; // 入库时间
|
|||
@ApiModelProperty("商品名称") |
|||
@ExcelProperty(value = "商品名称") |
|||
private String coli8; // 商品名称
|
|||
@ExcelProperty(value = "商品规格") |
|||
@ApiModelProperty("商品规格") |
|||
private String coll11; // 商品规格
|
|||
@ExcelProperty(value = "类别编号") |
|||
@ApiModelProperty("类别编号") |
|||
private String colm12; // 类别编号
|
|||
@ExcelProperty(value = "类别名称") |
|||
@ApiModelProperty("类别名称") |
|||
private String coln13; // 类别名称
|
|||
@ExcelProperty(value = "入库数量") |
|||
@ApiModelProperty("入库数量") |
|||
private String colr17; // 入库数量
|
|||
} |
@ -0,0 +1,27 @@ |
|||
package com.yxt.supervise.customer.api.gdinstorageyc; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import com.yxt.supervise.customer.api.gdinstoragegd.GdInstorageGd; |
|||
import com.yxt.supervise.customer.api.gdinstoragegd.GdInstorageGdFeignFallback; |
|||
import com.yxt.supervise.customer.api.gdinstoragegd.GdInstorageGdQuery; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
import org.springframework.web.bind.annotation.PostMapping; |
|||
import org.springframework.web.bind.annotation.RequestBody; |
|||
import org.springframework.web.bind.annotation.ResponseBody; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 10:01 |
|||
*/ |
|||
@Api(tags = "入库数据(烟草)") |
|||
@FeignClient( |
|||
contextId = "yxt-supervise-GdInstorageYc", |
|||
name = "yxt-supervise", |
|||
path = "v1/gdinstorageyc", |
|||
fallback = GdInstorageYcFeignFallback.class) |
|||
public interface GdInstorageYcFeign { |
|||
} |
@ -0,0 +1,11 @@ |
|||
package com.yxt.supervise.customer.api.gdinstorageyc; |
|||
|
|||
import org.springframework.stereotype.Component; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 10:01 |
|||
*/ |
|||
@Component |
|||
public class GdInstorageYcFeignFallback implements GdInstorageYcFeign{ |
|||
} |
@ -0,0 +1,71 @@ |
|||
package com.yxt.supervise.customer.api.gdinstorageyc; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 10:02 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "入库数据(烟草) 查询条件", description = "入库数据(烟草) 查询条件") |
|||
public class GdInstorageYcQuery implements Query { |
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String cola0; // 企业组织机构代码证
|
|||
@ApiModelProperty("仓库/门店编号") |
|||
private String colb1; // 仓库/门店编号
|
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String colc2; // 仓库/门店名称
|
|||
@ApiModelProperty("入库时间") |
|||
private String cold3; // 入库时间
|
|||
@ApiModelProperty("供应商编号") |
|||
private String colex; // 供应商编号
|
|||
@ApiModelProperty("供应商名称") |
|||
private String cole4; // 供应商名称
|
|||
@ApiModelProperty("单据号") |
|||
private String colf5; // 单据号
|
|||
@ApiModelProperty("单据类型") |
|||
private String colg6; // 单据类型
|
|||
@ApiModelProperty("货号") |
|||
private String colh7; // 货号
|
|||
@ApiModelProperty("商品名称") |
|||
private String coli8; // 商品名称
|
|||
@ApiModelProperty("商品生产日期") |
|||
private String colj9; // 商品生产日期
|
|||
@ApiModelProperty("商品保质期") |
|||
private String colk10; // 商品保质期
|
|||
@ApiModelProperty("商品规格") |
|||
private String coll11; // 商品规格
|
|||
@ApiModelProperty("类别编号") |
|||
private String colm12; // 类别编号
|
|||
@ApiModelProperty("类别名称") |
|||
private String coln13; // 类别名称
|
|||
@ApiModelProperty("一级类别名称") |
|||
private String colo14; // 一级类别名称
|
|||
@ApiModelProperty("二级类别名称") |
|||
private String colp15; // 二级类别名称
|
|||
@ApiModelProperty("入库金额") |
|||
private String colq16; // 入库金额
|
|||
@ApiModelProperty("入库数量") |
|||
private String colr17; // 入库数量
|
|||
@ApiModelProperty("成本金额") |
|||
private String cols18; // 成本金额
|
|||
@ApiModelProperty("成本税额") |
|||
private String colt19; // 成本税额
|
|||
@ApiModelProperty("不含税成本") |
|||
private String colu20; // 不含税成本
|
|||
@ApiModelProperty("进项税率") |
|||
private String colv21; // 进项税率
|
|||
@ApiModelProperty("单据日期") |
|||
private String orderDate; // 单据日期
|
|||
@ApiModelProperty("供货商编码统一") |
|||
private String supplierCodeUnified; // 供货商编码统一
|
|||
@ApiModelProperty("入库定单号") |
|||
private String inOrderNo; // 入库定单号
|
|||
@ApiModelProperty("开始时间") |
|||
private String startTime; |
|||
@ApiModelProperty("结束时间") |
|||
private String endTime; |
|||
} |
@ -0,0 +1,67 @@ |
|||
package com.yxt.supervise.customer.api.gdinstorageyc; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 10:02 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "入库数据(烟草) 视图数据对象", description = "入库数据(烟草) 视图数据对象") |
|||
public class GdInstorageYcVo implements Vo { |
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String cola0; // 企业组织机构代码证
|
|||
@ApiModelProperty("仓库/门店编号") |
|||
private String colb1; // 仓库/门店编号
|
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String colc2; // 仓库/门店名称
|
|||
@ApiModelProperty("入库时间") |
|||
private String cold3; // 入库时间
|
|||
@ApiModelProperty("供应商编号") |
|||
private String colex; // 供应商编号
|
|||
@ApiModelProperty("供应商名称") |
|||
private String cole4; // 供应商名称
|
|||
@ApiModelProperty("单据号") |
|||
private String colf5; // 单据号
|
|||
@ApiModelProperty("单据类型") |
|||
private String colg6; // 单据类型
|
|||
@ApiModelProperty("货号") |
|||
private String colh7; // 货号
|
|||
@ApiModelProperty("商品名称") |
|||
private String coli8; // 商品名称
|
|||
@ApiModelProperty("商品生产日期") |
|||
private String colj9; // 商品生产日期
|
|||
@ApiModelProperty("商品保质期") |
|||
private String colk10; // 商品保质期
|
|||
@ApiModelProperty("商品规格") |
|||
private String coll11; // 商品规格
|
|||
@ApiModelProperty("类别编号") |
|||
private String colm12; // 类别编号
|
|||
@ApiModelProperty("类别名称") |
|||
private String coln13; // 类别名称
|
|||
@ApiModelProperty("一级类别名称") |
|||
private String colo14; // 一级类别名称
|
|||
@ApiModelProperty("二级类别名称") |
|||
private String colp15; // 二级类别名称
|
|||
@ApiModelProperty("入库金额") |
|||
private String colq16; // 入库金额
|
|||
@ApiModelProperty("入库数量") |
|||
private String colr17; // 入库数量
|
|||
@ApiModelProperty("成本金额") |
|||
private String cols18; // 成本金额
|
|||
@ApiModelProperty("成本税额") |
|||
private String colt19; // 成本税额
|
|||
@ApiModelProperty("不含税成本") |
|||
private String colu20; // 不含税成本
|
|||
@ApiModelProperty("进项税率") |
|||
private String colv21; // 进项税率
|
|||
@ApiModelProperty("单据日期") |
|||
private String orderDate; // 单据日期
|
|||
@ApiModelProperty("供货商编码统一") |
|||
private String supplierCodeUnified; // 供货商编码统一
|
|||
@ApiModelProperty("入库定单号") |
|||
private String inOrderNo; // 入库定单号
|
|||
} |
@ -0,0 +1,42 @@ |
|||
package com.yxt.supervise.customer.api.gdinventorylog; |
|||
|
|||
import cn.hutool.core.lang.UUID; |
|||
import cn.hutool.core.util.IdUtil; |
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import com.yxt.common.core.domain.EntityWithId; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/24 13:45 |
|||
*/ |
|||
@Data |
|||
@TableName("gd_inventory_log") |
|||
public class GdInventoryLog extends EntityWithId { |
|||
|
|||
public GdInventoryLog() { |
|||
} |
|||
|
|||
public GdInventoryLog(String fileFullPath) { |
|||
this.fileFullPath = fileFullPath; |
|||
} |
|||
|
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss", timezone = "GMT+8") |
|||
private Date createTime = new Date(); // 记录创建时间/
|
|||
private String remarks; // 备注说明/
|
|||
private String fileFullPath; // 文件完整路径/
|
|||
private String outFilePath; // 汇总文件路径
|
|||
// private String outfile; //输出文件名
|
|||
private String fileUrl; //文件下载地址
|
|||
private int allNum; // 总记录数/
|
|||
private int validNum; // 有效记录数/
|
|||
private long durations; // 程序运行时长/
|
|||
private int errRowNum; // 出错的条数/
|
|||
private String orderDate; // 单据日期
|
|||
|
|||
private String sid = IdUtil.fastSimpleUUID(); |
|||
private int state = 0; |
|||
} |
@ -0,0 +1,24 @@ |
|||
package com.yxt.supervise.customer.api.gdinventorylog; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
|
|||
public class GdInventoryLogQuery implements Query { |
|||
private String dataDateStart; // 数据日期
|
|||
private String dataDateEnd; // 数据日期
|
|||
|
|||
public String getDataDateStart() { |
|||
return dataDateStart; |
|||
} |
|||
|
|||
public void setDataDateStart(String dataDateStart) { |
|||
this.dataDateStart = dataDateStart; |
|||
} |
|||
|
|||
public String getDataDateEnd() { |
|||
return dataDateEnd; |
|||
} |
|||
|
|||
public void setDataDateEnd(String dataDateEnd) { |
|||
this.dataDateEnd = dataDateEnd; |
|||
} |
|||
} |
@ -0,0 +1,8 @@ |
|||
package com.yxt.supervise.customer.api.gdinventorylog; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/24 13:46 |
|||
*/ |
|||
public class GdInventoryLogVo { |
|||
} |
@ -0,0 +1,29 @@ |
|||
package com.yxt.supervise.customer.api.gdinventorylogerr; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.EntityWithId; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/24 13:57 |
|||
*/ |
|||
@Data |
|||
@TableName("gd_inventory_log_err") |
|||
public class GdInventoryLogErr extends EntityWithId { |
|||
private Date createTime = new Date(); // 记录创建时间',
|
|||
private String remarks; // 备注说明',
|
|||
private String fileFullPath; // 文件完整路径',
|
|||
private String errInfo; // 异常信息',
|
|||
private String rowContent; // 原记录内容',
|
|||
private int rowNum; // 出错行数',
|
|||
private String prodCode; //商品编码
|
|||
private String prodName; //商品名称
|
|||
private String prodNum; //'商品数量
|
|||
private String prodValue; //商品货值
|
|||
private String supplierCode; //供货商编码
|
|||
private String supplierName; //供货商名称
|
|||
private String orderDate; // 单据日期
|
|||
} |
@ -0,0 +1,51 @@ |
|||
package com.yxt.supervise.customer.api.gdinventoryok; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import com.yxt.common.core.domain.EntityWithId; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 10:37 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "国大库存数据", description = "国大库存数据") |
|||
@TableName("gd_inventory_ok") |
|||
public class GdInventoryOk extends EntityWithId { |
|||
// 企业组织机构代码证 仓库编号 仓库名称 类别编号 类别名称 一级类别名称 二级类别名称 商品编码 商品条码 商品名称 仓库类型 商品数量 商品货值 供应商 数据日期
|
|||
|
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String orgCode;//企业组织机构代码证
|
|||
@ApiModelProperty("仓库/门店编号") |
|||
private String warehouseCode;//仓库/门店编号
|
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String warehouseName;//仓库/门店名称
|
|||
@ApiModelProperty("类别编号") |
|||
private String typeCode;//类别编号
|
|||
@ApiModelProperty("类别名称") |
|||
private String typeName;//类别名称
|
|||
@ApiModelProperty("一级类别名称") |
|||
private String typeOne;//一级类别名称
|
|||
@ApiModelProperty("二级类别名称") |
|||
private String typeTwo;//二级类别名称
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode;//商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode;//商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName;//商品名称
|
|||
@ApiModelProperty("仓库类型") |
|||
private String warehouseType;//仓库类型
|
|||
@ApiModelProperty("商品数量") |
|||
private String prodNum;//商品数量
|
|||
@ApiModelProperty("商品货值") |
|||
private String prodValue;//商品货值
|
|||
@ApiModelProperty("供应商") |
|||
private String suppliderName;//供应商
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate;//数据日期
|
|||
|
|||
} |
@ -0,0 +1,47 @@ |
|||
package com.yxt.supervise.customer.api.gdinventoryok; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 10:57 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "国大库存数据 视图数据详情", description = "国大库存数据 视图数据详情") |
|||
public class GdInventoryOkDetailsVo implements Vo { |
|||
@ApiModelProperty("ID,唯一编号") |
|||
private String id; |
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String orgCode;//企业组织机构代码证
|
|||
@ApiModelProperty("仓库/门店编号") |
|||
private String warehouseCode;//仓库/门店编号
|
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String warehouseName;//仓库/门店名称
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode;//商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode;//商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName;//商品名称
|
|||
@ApiModelProperty("仓库类型") |
|||
private String warehouseType;//仓库类型
|
|||
@ApiModelProperty("商品数量") |
|||
private String prodNum;//商品数量
|
|||
@ApiModelProperty("商品货值") |
|||
private String prodValue;//商品货值
|
|||
@ApiModelProperty("供应商") |
|||
private String suppliderName;//供应商
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate;//数据日期
|
|||
@ApiModelProperty("类别编号") |
|||
private String typeCode;//类别编号
|
|||
@ApiModelProperty("类别名称") |
|||
private String typeName;//类别名称
|
|||
@ApiModelProperty("一级类别名称") |
|||
private String typeOne;//一级类别名称
|
|||
@ApiModelProperty("二级类别名称") |
|||
private String typeTwo;//二级类别名称
|
|||
} |
@ -0,0 +1,47 @@ |
|||
package com.yxt.supervise.customer.api.gdinventoryok; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 10:58 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "国大库存数据 数据传输对象", description = "国大库存数据 数据传输对象") |
|||
public class GdInventoryOkDto implements Dto { |
|||
@ApiModelProperty("ID,唯一编号") |
|||
private String id; |
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String orgCode;//企业组织机构代码证
|
|||
@ApiModelProperty("仓库/门店编号") |
|||
private String warehouseCode;//仓库/门店编号
|
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String warehouseName;//仓库/门店名称
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode;//商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode;//商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName;//商品名称
|
|||
@ApiModelProperty("仓库类型") |
|||
private String warehouseType;//仓库类型
|
|||
@ApiModelProperty("商品数量") |
|||
private String prodNum;//商品数量
|
|||
@ApiModelProperty("商品货值") |
|||
private String prodValue;//商品货值
|
|||
@ApiModelProperty("供应商") |
|||
private String suppliderName;//供应商
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate;//数据日期
|
|||
@ApiModelProperty("类别编号") |
|||
private String typeCode;//类别编号
|
|||
@ApiModelProperty("类别名称") |
|||
private String typeName;//类别名称
|
|||
@ApiModelProperty("一级类别名称") |
|||
private String typeOne;//一级类别名称
|
|||
@ApiModelProperty("二级类别名称") |
|||
private String typeTwo;//二级类别名称
|
|||
} |
@ -0,0 +1,41 @@ |
|||
package com.yxt.supervise.customer.api.gdinventoryok; |
|||
|
|||
import com.alibaba.excel.annotation.ExcelProperty; |
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 11:55 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "国大库存数据 Excel视图数据对象", description = "国大库存数据 Excel视图数据对象") |
|||
public class GdInventoryOkExcelVo implements Vo { |
|||
@ExcelProperty(value = "仓库/门店编号") |
|||
@ApiModelProperty("仓库/门店编号") |
|||
private String warehouseCode;//仓库/门店编号
|
|||
@ExcelProperty(value = "仓库/门店名称") |
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String warehouseName;//仓库/门店名称
|
|||
@ExcelProperty(value = "商品编码") |
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode;//商品编码
|
|||
@ExcelProperty(value = "商品名称") |
|||
@ApiModelProperty("商品名称") |
|||
private String prodName;//商品名称
|
|||
@ExcelProperty(value = "商品数量") |
|||
@ApiModelProperty("商品数量") |
|||
private String prodNum;//商品数量
|
|||
@ExcelProperty(value = "商品货值") |
|||
@ApiModelProperty("商品货值") |
|||
private String prodValue;//商品货值
|
|||
@ExcelProperty(value = "供应商") |
|||
@ApiModelProperty("供应商") |
|||
private String suppliderName;//供应商
|
|||
@ExcelProperty(value = "数据日期") |
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate;//数据日期
|
|||
|
|||
} |
@ -0,0 +1,18 @@ |
|||
package com.yxt.supervise.customer.api.gdinventoryok; |
|||
|
|||
import com.yxt.supervise.customer.api.gdinstoragegd.GdInstorageGdFeignFallback; |
|||
import io.swagger.annotations.Api; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 11:02 |
|||
*/ |
|||
@Api(tags = "国大库存数据") |
|||
@FeignClient( |
|||
contextId = "yxt-supervise-GdInventoryOk", |
|||
name = "yxt-supervise", |
|||
path = "v1/gdinventoryok", |
|||
fallback = GdInstorageGdFeignFallback.class) |
|||
public interface GdInventoryOkFeign { |
|||
} |
@ -0,0 +1,11 @@ |
|||
package com.yxt.supervise.customer.api.gdinventoryok; |
|||
|
|||
import org.springframework.stereotype.Component; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 11:04 |
|||
*/ |
|||
@Component |
|||
public class GdInventoryOkFeignFallback implements GdInventoryOkFeign{ |
|||
} |
@ -0,0 +1,52 @@ |
|||
package com.yxt.supervise.customer.api.gdinventoryok; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 11:05 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "国大库存数据 查询条件", description = "国大库存数据 查询条件") |
|||
public class GdInventoryOkQuery implements Query { |
|||
@ApiModelProperty("ID,唯一编号") |
|||
private String id; |
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String orgCode;//企业组织机构代码证
|
|||
@ApiModelProperty("仓库/门店编号") |
|||
private String warehouseCode;//仓库/门店编号
|
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String warehouseName;//仓库/门店名称
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode;//商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode;//商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName;//商品名称
|
|||
@ApiModelProperty("仓库类型") |
|||
private String warehouseType;//仓库类型
|
|||
@ApiModelProperty("商品数量") |
|||
private String prodNum;//商品数量
|
|||
@ApiModelProperty("商品货值") |
|||
private String prodValue;//商品货值
|
|||
@ApiModelProperty("供应商") |
|||
private String suppliderName;//供应商
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate;//数据日期
|
|||
@ApiModelProperty("类别编号") |
|||
private String typeCode;//类别编号
|
|||
@ApiModelProperty("类别名称") |
|||
private String typeName;//类别名称
|
|||
@ApiModelProperty("一级类别名称") |
|||
private String typeOne;//一级类别名称
|
|||
@ApiModelProperty("二级类别名称") |
|||
private String typeTwo;//二级类别名称
|
|||
|
|||
@ApiModelProperty("开始时间") |
|||
private String startDate; |
|||
@ApiModelProperty("结束时间") |
|||
private String endDate; |
|||
} |
@ -0,0 +1,47 @@ |
|||
package com.yxt.supervise.customer.api.gdinventoryok; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 11:07 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "国大库存数据 视图数据对象", description = "国大库存数据 视图数据对象") |
|||
public class GdInventoryOkVo implements Vo { |
|||
@ApiModelProperty("ID,唯一编号") |
|||
private String id; |
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String orgCode;//企业组织机构代码证
|
|||
@ApiModelProperty("仓库/门店编号") |
|||
private String warehouseCode;//仓库/门店编号
|
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String warehouseName;//仓库/门店名称
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode;//商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode;//商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName;//商品名称
|
|||
@ApiModelProperty("仓库类型") |
|||
private String warehouseType;//仓库类型
|
|||
@ApiModelProperty("商品数量") |
|||
private String prodNum;//商品数量
|
|||
@ApiModelProperty("商品货值") |
|||
private String prodValue;//商品货值
|
|||
@ApiModelProperty("供应商") |
|||
private String suppliderName;//供应商
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate;//数据日期
|
|||
@ApiModelProperty("类别编号") |
|||
private String typeCode;//类别编号
|
|||
@ApiModelProperty("类别名称") |
|||
private String typeName;//类别名称
|
|||
@ApiModelProperty("一级类别名称") |
|||
private String typeOne;//一级类别名称
|
|||
@ApiModelProperty("二级类别名称") |
|||
private String typeTwo;//二级类别名称
|
|||
} |
@ -0,0 +1,49 @@ |
|||
package com.yxt.supervise.customer.api.gdinventoryyc; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.EntityWithId; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 9:31 |
|||
*/ |
|||
|
|||
@Data |
|||
@ApiModel(value = "国大库存数据(烟草)", description = "国大库存数据") |
|||
@TableName("gd_inventory_yc") |
|||
public class GdInventoryYc extends EntityWithId { |
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String orgCode;//企业组织机构代码证
|
|||
@ApiModelProperty("仓库/门店编号") |
|||
private String warehouseCode;//仓库/门店编号
|
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String warehouseName;//仓库/门店名称
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode;//商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode;//商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName;//商品名称
|
|||
@ApiModelProperty("仓库类型") |
|||
private String warehouseType;//仓库类型
|
|||
@ApiModelProperty("商品数量") |
|||
private String prodNum;//商品数量
|
|||
@ApiModelProperty("商品货值") |
|||
private String prodValue;//商品货值
|
|||
@ApiModelProperty("供应商") |
|||
private String suppliderName;//供应商
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate;//数据日期
|
|||
@ApiModelProperty("类别编号") |
|||
private String typeCode;//类别编号
|
|||
@ApiModelProperty("类别名称") |
|||
private String typeName;//类别名称
|
|||
@ApiModelProperty("一级类别名称") |
|||
private String typeOne;//一级类别名称
|
|||
@ApiModelProperty("二级类别名称") |
|||
private String typeTwo;//二级类别名称
|
|||
|
|||
} |
@ -0,0 +1,47 @@ |
|||
package com.yxt.supervise.customer.api.gdinventoryyc; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 9:31 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "国大库存数据(烟草) 视图数据详情", description = "国大库存数据(烟草) 视图数据详情") |
|||
public class GdInventoryYcDetailsVo implements Vo { |
|||
@ApiModelProperty("ID,唯一编号") |
|||
private String id; |
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String orgCode;//企业组织机构代码证
|
|||
@ApiModelProperty("仓库/门店编号") |
|||
private String warehouseCode;//仓库/门店编号
|
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String warehouseName;//仓库/门店名称
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode;//商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode;//商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName;//商品名称
|
|||
@ApiModelProperty("仓库类型") |
|||
private String warehouseType;//仓库类型
|
|||
@ApiModelProperty("商品数量") |
|||
private String prodNum;//商品数量
|
|||
@ApiModelProperty("商品货值") |
|||
private String prodValue;//商品货值
|
|||
@ApiModelProperty("供应商") |
|||
private String suppliderName;//供应商
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate;//数据日期
|
|||
@ApiModelProperty("类别编号") |
|||
private String typeCode;//类别编号
|
|||
@ApiModelProperty("类别名称") |
|||
private String typeName;//类别名称
|
|||
@ApiModelProperty("一级类别名称") |
|||
private String typeOne;//一级类别名称
|
|||
@ApiModelProperty("二级类别名称") |
|||
private String typeTwo;//二级类别名称
|
|||
} |
@ -0,0 +1,47 @@ |
|||
package com.yxt.supervise.customer.api.gdinventoryyc; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 9:31 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "国大库存数据(烟草) 数据传输对象", description = "国大库存数据(烟草) 数据传输对象") |
|||
public class GdInventoryYcDto implements Dto { |
|||
@ApiModelProperty("ID,唯一编号") |
|||
private String id; |
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String orgCode;//企业组织机构代码证
|
|||
@ApiModelProperty("仓库/门店编号") |
|||
private String warehouseCode;//仓库/门店编号
|
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String warehouseName;//仓库/门店名称
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode;//商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode;//商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName;//商品名称
|
|||
@ApiModelProperty("仓库类型") |
|||
private String warehouseType;//仓库类型
|
|||
@ApiModelProperty("商品数量") |
|||
private String prodNum;//商品数量
|
|||
@ApiModelProperty("商品货值") |
|||
private String prodValue;//商品货值
|
|||
@ApiModelProperty("供应商") |
|||
private String suppliderName;//供应商
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate;//数据日期
|
|||
@ApiModelProperty("类别编号") |
|||
private String typeCode;//类别编号
|
|||
@ApiModelProperty("类别名称") |
|||
private String typeName;//类别名称
|
|||
@ApiModelProperty("一级类别名称") |
|||
private String typeOne;//一级类别名称
|
|||
@ApiModelProperty("二级类别名称") |
|||
private String typeTwo;//二级类别名称
|
|||
} |
@ -0,0 +1,41 @@ |
|||
package com.yxt.supervise.customer.api.gdinventoryyc; |
|||
|
|||
import com.alibaba.excel.annotation.ExcelProperty; |
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 9:32 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "国大库存数据(烟草) Excel视图数据对象", description = "国大库存数据(烟草) Excel视图数据对象") |
|||
public class GdInventoryYcExcelVo implements Vo { |
|||
@ExcelProperty(value = "仓库/门店编号") |
|||
@ApiModelProperty("仓库/门店编号") |
|||
private String warehouseCode;//仓库/门店编号
|
|||
@ExcelProperty(value = "仓库/门店名称") |
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String warehouseName;//仓库/门店名称
|
|||
@ExcelProperty(value = "商品编码") |
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode;//商品编码
|
|||
@ExcelProperty(value = "商品名称") |
|||
@ApiModelProperty("商品名称") |
|||
private String prodName;//商品名称
|
|||
@ExcelProperty(value = "商品数量") |
|||
@ApiModelProperty("商品数量") |
|||
private String prodNum;//商品数量
|
|||
@ExcelProperty(value = "商品货值") |
|||
@ApiModelProperty("商品货值") |
|||
private String prodValue;//商品货值
|
|||
@ExcelProperty(value = "供应商") |
|||
@ApiModelProperty("供应商") |
|||
private String suppliderName;//供应商
|
|||
@ExcelProperty(value = "数据日期") |
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate;//数据日期
|
|||
|
|||
} |
@ -0,0 +1,18 @@ |
|||
package com.yxt.supervise.customer.api.gdinventoryyc; |
|||
|
|||
import com.yxt.supervise.customer.api.gdinstoragegd.GdInstorageGdFeignFallback; |
|||
import io.swagger.annotations.Api; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 9:32 |
|||
*/ |
|||
@Api(tags = "国大库存数据(烟草)") |
|||
@FeignClient( |
|||
contextId = "yxt-supervise-GdInventoryYc", |
|||
name = "yxt-supervise", |
|||
path = "v1/gdinventoryyc", |
|||
fallback = GdInventoryYcFeignFallback.class) |
|||
public interface GdInventoryYcFeign { |
|||
} |
@ -0,0 +1,12 @@ |
|||
package com.yxt.supervise.customer.api.gdinventoryyc; |
|||
|
|||
import com.yxt.supervise.customer.api.gdinventoryok.GdInventoryOkFeign; |
|||
import org.springframework.stereotype.Component; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 9:32 |
|||
*/ |
|||
@Component |
|||
public class GdInventoryYcFeignFallback implements GdInventoryYcFeign { |
|||
} |
@ -0,0 +1,52 @@ |
|||
package com.yxt.supervise.customer.api.gdinventoryyc; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 9:32 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "国大库存数据(烟草) 查询条件", description = "国大库存数据(烟草) 查询条件") |
|||
public class GdInventoryYcQuery implements Query { |
|||
@ApiModelProperty("ID,唯一编号") |
|||
private String id; |
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String orgCode;//企业组织机构代码证
|
|||
@ApiModelProperty("仓库/门店编号") |
|||
private String warehouseCode;//仓库/门店编号
|
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String warehouseName;//仓库/门店名称
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode;//商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode;//商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName;//商品名称
|
|||
@ApiModelProperty("仓库类型") |
|||
private String warehouseType;//仓库类型
|
|||
@ApiModelProperty("商品数量") |
|||
private String prodNum;//商品数量
|
|||
@ApiModelProperty("商品货值") |
|||
private String prodValue;//商品货值
|
|||
@ApiModelProperty("供应商") |
|||
private String suppliderName;//供应商
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate;//数据日期
|
|||
@ApiModelProperty("类别编号") |
|||
private String typeCode;//类别编号
|
|||
@ApiModelProperty("类别名称") |
|||
private String typeName;//类别名称
|
|||
@ApiModelProperty("一级类别名称") |
|||
private String typeOne;//一级类别名称
|
|||
@ApiModelProperty("二级类别名称") |
|||
private String typeTwo;//二级类别名称
|
|||
|
|||
@ApiModelProperty("开始时间") |
|||
private String startDate; |
|||
@ApiModelProperty("结束时间") |
|||
private String endDate; |
|||
} |
@ -0,0 +1,47 @@ |
|||
package com.yxt.supervise.customer.api.gdinventoryyc; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 9:32 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "国大库存数据(烟草) 视图数据对象", description = "国大库存数据(烟草) 视图数据对象") |
|||
public class GdInventoryYcVo implements Vo { |
|||
@ApiModelProperty("ID,唯一编号") |
|||
private String id; |
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String orgCode;//企业组织机构代码证
|
|||
@ApiModelProperty("仓库/门店编号") |
|||
private String warehouseCode;//仓库/门店编号
|
|||
@ApiModelProperty("仓库/门店名称") |
|||
private String warehouseName;//仓库/门店名称
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode;//商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode;//商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName;//商品名称
|
|||
@ApiModelProperty("仓库类型") |
|||
private String warehouseType;//仓库类型
|
|||
@ApiModelProperty("商品数量") |
|||
private String prodNum;//商品数量
|
|||
@ApiModelProperty("商品货值") |
|||
private String prodValue;//商品货值
|
|||
@ApiModelProperty("供应商") |
|||
private String suppliderName;//供应商
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate;//数据日期
|
|||
@ApiModelProperty("类别编号") |
|||
private String typeCode;//类别编号
|
|||
@ApiModelProperty("类别名称") |
|||
private String typeName;//类别名称
|
|||
@ApiModelProperty("一级类别名称") |
|||
private String typeOne;//一级类别名称
|
|||
@ApiModelProperty("二级类别名称") |
|||
private String typeTwo;//二级类别名称
|
|||
} |
@ -0,0 +1,16 @@ |
|||
package com.yxt.supervise.customer.api.gdrescategoryprod; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.EntityWithId; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/24 14:04 |
|||
*/ |
|||
@Data |
|||
@TableName("gd_rescategory_prod") |
|||
public class GdRescategoryProd extends EntityWithId { |
|||
private String prodCode; |
|||
} |
|||
|
@ -0,0 +1,58 @@ |
|||
package com.yxt.supervise.customer.api.gdsales; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import com.yxt.common.core.domain.EntityWithId; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/28 15:05 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "销售数据", description = "销售数据") |
|||
@TableName("gd_sales") |
|||
public class GdSales extends EntityWithId { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String orgCode; // 企业组织机构代码证
|
|||
@ApiModelProperty("订单类型") |
|||
private String orderType; // 订单类型
|
|||
@ApiModelProperty("销售订单号") |
|||
private String orderNo; // 销售订单号
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode; // 商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode; // 商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName; // 商品名称
|
|||
@ApiModelProperty("品牌代码") |
|||
private String brandCode; // 品牌代码
|
|||
@ApiModelProperty("品牌名称") |
|||
private String brandName; // 品牌名称
|
|||
@ApiModelProperty("商品大类") |
|||
private String categoryb; // 商品大类
|
|||
@ApiModelProperty("商品中类") |
|||
private String categorym; // 商品中类
|
|||
@ApiModelProperty("商品小类") |
|||
private String categorys; // 商品小类
|
|||
@ApiModelProperty("客户代码") |
|||
private String customerCode; // 客户代码
|
|||
@ApiModelProperty("客户名称") |
|||
private String customerName; // 客户名称
|
|||
@ApiModelProperty("销售数量") |
|||
private String saleNum; // 销售数量
|
|||
@ApiModelProperty("销售渠道") |
|||
private String storeCode; // 销售渠道
|
|||
@ApiModelProperty("销售价格") |
|||
private String salePrice; // 销售价格
|
|||
@ApiModelProperty("销售成本") |
|||
private String saleCost; // 销售成本
|
|||
@ApiModelProperty("利润") |
|||
private String profit; // 利润
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate; // 数据日期
|
|||
} |
@ -0,0 +1,59 @@ |
|||
package com.yxt.supervise.customer.api.gdsales; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/28 15:16 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "销售数据 视图数据详情", description = "销售数据 视图数据详情") |
|||
public class GdSalesDetailsVo implements Vo { |
|||
private String sid; // sid
|
|||
|
|||
@ApiModelProperty("创建者") |
|||
private String createSid; // 创建者
|
|||
@ApiModelProperty("更新者") |
|||
private String modifySid; // 更新者
|
|||
@ApiModelProperty("销售订单号") |
|||
private String code; // 销售订单号
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode; // 商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode; // 商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName; // 商品名称
|
|||
@ApiModelProperty("销售数量") |
|||
private String saleNum; // 销售数量
|
|||
@ApiModelProperty("销售渠道") |
|||
private String storeCode; // 销售渠道
|
|||
@ApiModelProperty("销售渠道名称") |
|||
private String storeName; // 销售渠道名称
|
|||
@ApiModelProperty("销售价格") |
|||
private String salePrice; // 销售价格
|
|||
@ApiModelProperty("销售成本") |
|||
private String saleCost; // 销售成本
|
|||
@ApiModelProperty("利润") |
|||
private String profit; // 利润
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate; // 数据日期
|
|||
@ApiModelProperty("类型") |
|||
private String orderType; // 类型
|
|||
@ApiModelProperty("客户代码") |
|||
private String customerCode; // 客户代码
|
|||
@ApiModelProperty("客户名称") |
|||
private String customerName; // 客户名称
|
|||
@ApiModelProperty("品牌代码") |
|||
private String brandCode; // 品牌代码
|
|||
@ApiModelProperty("品牌名称") |
|||
private String brandName; // 品牌名称
|
|||
@ApiModelProperty("大类") |
|||
private String categoryb; // 大类
|
|||
@ApiModelProperty("种类") |
|||
private String categorym; // 种类
|
|||
@ApiModelProperty("小类") |
|||
private String categorys; // 小类
|
|||
} |
@ -0,0 +1,61 @@ |
|||
package com.yxt.supervise.customer.api.gdsales; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/28 15:15 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "销售数据 数据传输对象", description = "销售数据 数据传输对象") |
|||
public class GdSalesDto implements Dto { |
|||
private String sid; // sid
|
|||
|
|||
@ApiModelProperty("创建者") |
|||
private String createSid; // 创建者
|
|||
@ApiModelProperty("更新者") |
|||
private String modifySid; // 更新者
|
|||
@ApiModelProperty("销售订单号") |
|||
private String code; // 销售订单号
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode; // 商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode; // 商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName; // 商品名称
|
|||
@ApiModelProperty("销售数量") |
|||
private String saleNum; // 销售数量
|
|||
@ApiModelProperty("销售渠道") |
|||
private String storeCode; // 销售渠道
|
|||
@ApiModelProperty("销售渠道名称") |
|||
private String storeName; // 销售渠道名称
|
|||
@ApiModelProperty("销售价格") |
|||
private String salePrice; // 销售价格
|
|||
@ApiModelProperty("销售成本") |
|||
private String saleCost; // 销售成本
|
|||
@ApiModelProperty("利润") |
|||
private String profit; // 利润
|
|||
@ApiModelProperty("数据日期") |
|||
private Date dataDate; // 数据日期
|
|||
@ApiModelProperty("类型") |
|||
private String orderType; // 类型
|
|||
@ApiModelProperty("客户代码") |
|||
private String customerCode; // 客户代码
|
|||
@ApiModelProperty("客户名称") |
|||
private String customerName; // 客户名称
|
|||
@ApiModelProperty("品牌代码") |
|||
private String brandCode; // 品牌代码
|
|||
@ApiModelProperty("品牌名称") |
|||
private String brandName; // 品牌名称
|
|||
@ApiModelProperty("大类") |
|||
private String categoryb; // 大类
|
|||
@ApiModelProperty("种类") |
|||
private String categorym; // 种类
|
|||
@ApiModelProperty("小类") |
|||
private String categorys; // 小类
|
|||
} |
@ -0,0 +1,83 @@ |
|||
package com.yxt.supervise.customer.api.gdsales; |
|||
|
|||
import com.alibaba.excel.annotation.ExcelProperty; |
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
import lombok.experimental.Accessors; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/29 10:43 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "销售数据 导出excel视图数据对象", description = "销售数据 导出excel视图数据对象") |
|||
public class GdSalesExcelVo implements Vo { |
|||
// private String sid; // sid
|
|||
//
|
|||
// @ApiModelProperty("创建者")
|
|||
// private String createSid; // 创建者
|
|||
// @ApiModelProperty("更新者")
|
|||
// private String modifySid; // 更新者
|
|||
@ExcelProperty(value = "销售订单号") |
|||
@ApiModelProperty("销售订单号") |
|||
private String code; // 销售订单号
|
|||
@ExcelProperty(value = "商品编码") |
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode; // 商品编码
|
|||
// @ApiModelProperty("商品条码")
|
|||
// private String prodBarCode; // 商品条码
|
|||
@ExcelProperty(value = "商品名称") |
|||
@ApiModelProperty("商品名称") |
|||
private String prodName; // 商品名称
|
|||
@ExcelProperty(value = "销售数量") |
|||
@ApiModelProperty("销售数量") |
|||
private String saleNum; // 销售数量
|
|||
// @ApiModelProperty("销售渠道")
|
|||
// private String storeCode; // 销售渠道
|
|||
@ExcelProperty(value = "销售渠道") |
|||
@ApiModelProperty("销售渠道名称") |
|||
private String storeName; // 销售渠道名称
|
|||
// @ApiModelProperty("销售价格")
|
|||
// private String salePrice; // 销售价格
|
|||
// @ApiModelProperty("销售成本")
|
|||
// private String saleCost; // 销售成本
|
|||
// @ApiModelProperty("利润")
|
|||
// private String profit; // 利润
|
|||
@ExcelProperty(value = "数据日期") |
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate; // 数据日期
|
|||
@ExcelProperty(value = "类型") |
|||
@ApiModelProperty("类型") |
|||
private String orderType; // 类型
|
|||
// @ApiModelProperty("客户代码")
|
|||
// private String customerCode; // 客户代码
|
|||
// @ApiModelProperty("客户名称")
|
|||
// private String customerName; // 客户名称
|
|||
@ExcelProperty(value = "品牌编码") |
|||
@ApiModelProperty("品牌代码") |
|||
private String brandCode; // 品牌代码
|
|||
@ExcelProperty(value = "品牌名称") |
|||
@ApiModelProperty("品牌名称") |
|||
private String brandName; // 品牌名称
|
|||
// @ApiModelProperty("大类")
|
|||
// private String categoryb; // 大类
|
|||
// @ApiModelProperty("种类")
|
|||
// private String categorym; // 种类
|
|||
// @ApiModelProperty("小类")
|
|||
// private String categorys; // 小类
|
|||
@ExcelProperty(value = "总价") |
|||
@ApiModelProperty("总价") |
|||
private String total; |
|||
|
|||
public void setSaleNum(String saleNum){ |
|||
int i=saleNum.indexOf("."); |
|||
if(i!=-1){ |
|||
String num=saleNum.substring(0,i); |
|||
this.saleNum=num; |
|||
}else{ |
|||
this.saleNum=saleNum; |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,31 @@ |
|||
package com.yxt.supervise.customer.api.gdsales; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
import org.springframework.web.bind.annotation.PostMapping; |
|||
import org.springframework.web.bind.annotation.RequestBody; |
|||
import org.springframework.web.bind.annotation.ResponseBody; |
|||
|
|||
import javax.servlet.http.HttpServletResponse; |
|||
import java.io.IOException; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/28 15:19 |
|||
*/ |
|||
@Api(tags = "销售数据") |
|||
@FeignClient( |
|||
contextId = "yxt-supervise-SalesData", |
|||
name = "yxt-supervise", |
|||
path = "v1/gdsales", |
|||
fallback = GdSalesFeignFallback.class) |
|||
public interface GdSalesFeign { |
|||
@ApiOperation("根据条件分页查询数据的列表") |
|||
@PostMapping("/listPage") |
|||
@ResponseBody |
|||
public ResultBean<PagerVo<GdSales>> listPage(@RequestBody PagerQuery<GdSalesQuery> pq); |
|||
} |
@ -0,0 +1,21 @@ |
|||
package com.yxt.supervise.customer.api.gdsales; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import org.springframework.stereotype.Component; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/28 15:20 |
|||
*/ |
|||
@Component |
|||
public class GdSalesFeignFallback implements GdSalesFeign{ |
|||
|
|||
@Override |
|||
public ResultBean<PagerVo<GdSales>> listPage(PagerQuery<GdSalesQuery> pq) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
return rb.setMsg("接口yxt-supervise/gdsales/listPage无法访问"); |
|||
|
|||
} |
|||
} |
@ -0,0 +1,72 @@ |
|||
package com.yxt.supervise.customer.api.gdsales; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/28 15:21 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "销售数据 查询条件", description = "销售数据 查询条件") |
|||
public class GdSalesQuery implements Query { |
|||
|
|||
@ApiModelProperty("创建者") |
|||
private String createSid; // 创建者
|
|||
@ApiModelProperty("更新者") |
|||
private String modifySid; // 更新者
|
|||
@ApiModelProperty("销售订单号") |
|||
private String code; // 销售订单号
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode; // 商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode; // 商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName; // 商品名称
|
|||
@ApiModelProperty("销售数量") |
|||
private String saleNum; // 销售数量
|
|||
@ApiModelProperty("销售渠道") |
|||
private String storeCode; // 销售渠道
|
|||
@ApiModelProperty("销售价格") |
|||
private String salePrice; // 销售价格
|
|||
@ApiModelProperty("销售渠道名称") |
|||
private String storeName; // 销售渠道名称
|
|||
@ApiModelProperty("销售成本") |
|||
private String saleCost; // 销售成本
|
|||
@ApiModelProperty("利润") |
|||
private String profit; // 利润
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate; // 数据日期
|
|||
@ApiModelProperty("类型") |
|||
private String orderType; // 类型
|
|||
@ApiModelProperty("客户代码") |
|||
private String customerCode; // 客户代码
|
|||
@ApiModelProperty("客户名称") |
|||
private String customerName; // 客户名称
|
|||
@ApiModelProperty("品牌代码") |
|||
private String brandCode; // 品牌代码
|
|||
@ApiModelProperty("品牌名称") |
|||
private String brandName; // 品牌名称
|
|||
@ApiModelProperty("大类") |
|||
private String categoryb; // 大类
|
|||
@ApiModelProperty("种类") |
|||
private String categorym; // 种类
|
|||
@ApiModelProperty("小类") |
|||
private String categorys; // 小类
|
|||
//开始时间
|
|||
private String startTime; |
|||
//结束时间
|
|||
private String endTime; |
|||
public void setSaleNum(String saleNum){ |
|||
int i=saleNum.indexOf("."); |
|||
if(i!=-1){ |
|||
String num=saleNum.substring(0,i); |
|||
this.saleNum=num; |
|||
}else{ |
|||
this.saleNum=saleNum; |
|||
} |
|||
} |
|||
|
|||
} |
@ -0,0 +1,82 @@ |
|||
package com.yxt.supervise.customer.api.gdsales; |
|||
|
|||
import com.alibaba.excel.annotation.ExcelProperty; |
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/28 15:21 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "销售数据 视图数据对象", description = "销售数据 视图数据对象") |
|||
public class GdSalesVo implements Vo { |
|||
// private String sid; // sid
|
|||
//
|
|||
// @ApiModelProperty("创建者")
|
|||
// private String createSid; // 创建者
|
|||
// @ApiModelProperty("更新者")
|
|||
// private String modifySid; // 更新者
|
|||
@ExcelProperty(value = "销售订单号") |
|||
@ApiModelProperty("销售订单号") |
|||
private String code; // 销售订单号
|
|||
@ExcelProperty(value = "商品编码") |
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode; // 商品编码
|
|||
// @ApiModelProperty("商品条码")
|
|||
// private String prodBarCode; // 商品条码
|
|||
@ExcelProperty(value = "商品名称") |
|||
@ApiModelProperty("商品名称") |
|||
private String prodName; // 商品名称
|
|||
@ExcelProperty(value = "销售数量") |
|||
@ApiModelProperty("销售数量") |
|||
private String saleNum; // 销售数量
|
|||
// @ApiModelProperty("销售渠道")
|
|||
// private String storeCode; // 销售渠道
|
|||
@ExcelProperty(value = "销售渠道") |
|||
@ApiModelProperty("销售渠道名称") |
|||
private String storeName; // 销售渠道名称
|
|||
// @ApiModelProperty("销售价格")
|
|||
// private String salePrice; // 销售价格
|
|||
// @ApiModelProperty("销售成本")
|
|||
// private String saleCost; // 销售成本
|
|||
// @ApiModelProperty("利润")
|
|||
// private String profit; // 利润
|
|||
@ExcelProperty(value = "数据日期") |
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate; // 数据日期
|
|||
@ExcelProperty(value = "数据日期") |
|||
@ApiModelProperty("类型") |
|||
private String orderType; // 类型
|
|||
// @ApiModelProperty("客户代码")
|
|||
// private String customerCode; // 客户代码
|
|||
// @ApiModelProperty("客户名称")
|
|||
// private String customerName; // 客户名称
|
|||
@ExcelProperty(value = "品牌编码") |
|||
@ApiModelProperty("品牌代码") |
|||
private String brandCode; // 品牌代码
|
|||
@ExcelProperty(value = "品牌名称") |
|||
@ApiModelProperty("品牌名称") |
|||
private String brandName; // 品牌名称
|
|||
// @ApiModelProperty("大类")
|
|||
// private String categoryb; // 大类
|
|||
// @ApiModelProperty("种类")
|
|||
// private String categorym; // 种类
|
|||
// @ApiModelProperty("小类")
|
|||
// private String categorys; // 小类
|
|||
@ExcelProperty(value = "总价") |
|||
@ApiModelProperty("总价") |
|||
private String total; |
|||
|
|||
public void setSaleNum(String saleNum){ |
|||
int i=saleNum.indexOf("."); |
|||
if(i!=-1){ |
|||
String num=saleNum.substring(0,i); |
|||
this.saleNum=num; |
|||
}else{ |
|||
this.saleNum=saleNum; |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,68 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.supervise.customer.api.gdsalesgd; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.EntityWithId; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: yxt_supervise <br/> |
|||
* File: GdSalesGd.java <br/> |
|||
* Class: com.yxt.supervise.portal.biz.gdsales.GdSalesGd <br/> |
|||
* Description: <描述类的功能>. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2023/1/7 16:09 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@TableName("gd_sales_gd") |
|||
public class GdSalesGd extends EntityWithId { |
|||
|
|||
private String orgCode; // 企业组织机构代码证
|
|||
private String orderType; // 订单类型
|
|||
private String orderNo; // 销售订单号
|
|||
private String prodCode; // 商品编码
|
|||
private String prodBarCode; // 商品条码
|
|||
private String prodName; // 商品名称
|
|||
private String brandCode; // 品牌代码
|
|||
private String brandName; // 品牌名称
|
|||
private String categoryb; // 商品大类
|
|||
private String categorym; // 商品中类
|
|||
private String categorys; // 商品小类
|
|||
private String customerCode; // 客户代码
|
|||
private String customerName; // 客户名称
|
|||
private String saleNum; // 销售数量
|
|||
private String storeCode; // 销售渠道
|
|||
private String salePrice; // 销售价格
|
|||
private String saleCost; // 销售成本
|
|||
private String profit; // 利润
|
|||
private String dataDate; // 数据日期
|
|||
} |
@ -0,0 +1,69 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.supervise.customer.api.gdsaleslog; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import com.yxt.common.core.domain.EntityWithId; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
|
|||
/** |
|||
* Project: yxt_supervise <br/> |
|||
* File: GdSalesLog.java <br/> |
|||
* Class: com.yxt.supervise.portal.biz.gdsales.GdSalesLog <br/> |
|||
* Description: <描述类的功能>. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2023/1/7 16:10 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@TableName("gd_sales_log") |
|||
public class GdSalesLog extends EntityWithId { |
|||
public GdSalesLog() { |
|||
} |
|||
|
|||
public GdSalesLog(String fileFullPath) { |
|||
this.fileFullPath = fileFullPath; |
|||
} |
|||
|
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss", timezone = "GMT+8") |
|||
private Date createTime = new Date(); // 记录创建时间/
|
|||
private String remarks; // 备注说明',
|
|||
private String fileFullPath; // 文件完整路径',
|
|||
private String outFilePath; // 汇总文件路径',
|
|||
private String fileUrl; // 文件下载地址',
|
|||
private int allNum = 0; // 总记录数',
|
|||
private int validNum = 0; // 有效记录数',
|
|||
private long durations = 0; // 程序运行时长',
|
|||
private int errRowNum = 0; // 出错的条数',
|
|||
private String orderDate; // 单据日期',
|
|||
} |
@ -0,0 +1,38 @@ |
|||
|
|||
package com.yxt.supervise.customer.api.gdsaleslogerr; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import com.yxt.common.core.domain.EntityWithId; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
|
|||
/** |
|||
* Project: yxt_supervise <br/> |
|||
* File: GdSalesLogErr.java <br/> |
|||
* Class: com.yxt.supervise.portal.biz.gdsales.GdSalesLogErr <br/> |
|||
* Description: <描述类的功能>. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2023/1/7 16:11 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@TableName("gd_sales_log_err") |
|||
public class GdSalesLogErr extends EntityWithId { |
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss", timezone = "GMT+8") |
|||
private Date createTime = new Date(); // 记录创建时间',
|
|||
private String remarks; // 备注说明',
|
|||
private String fileFullPath; // 文件完整路径',
|
|||
private String errInfo; // 异常信息',
|
|||
private String rowContent; // 原记录内容',
|
|||
private int rowNum; // 出错行数',
|
|||
private String orderDate; // 单据日期
|
|||
private String orderNo; // 销售订单号
|
|||
private String prodCode; //商品编码
|
|||
private String prodName; //商品名称
|
|||
} |
@ -0,0 +1,57 @@ |
|||
package com.yxt.supervise.customer.api.gdsalesyc; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.EntityWithId; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 8:48 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "销售数据(烟草)", description = "销售数据(烟草)") |
|||
@TableName("gd_sales_yc") |
|||
public class GdSalesYc extends EntityWithId { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String orgCode; // 企业组织机构代码证
|
|||
@ApiModelProperty("订单类型") |
|||
private String orderType; // 订单类型
|
|||
@ApiModelProperty("销售订单号") |
|||
private String orderNo; // 销售订单号
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode; // 商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode; // 商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName; // 商品名称
|
|||
@ApiModelProperty("品牌代码") |
|||
private String brandCode; // 品牌代码
|
|||
@ApiModelProperty("品牌名称") |
|||
private String brandName; // 品牌名称
|
|||
@ApiModelProperty("商品大类") |
|||
private String categoryb; // 商品大类
|
|||
@ApiModelProperty("商品中类") |
|||
private String categorym; // 商品中类
|
|||
@ApiModelProperty("商品小类") |
|||
private String categorys; // 商品小类
|
|||
@ApiModelProperty("客户代码") |
|||
private String customerCode; // 客户代码
|
|||
@ApiModelProperty("客户名称") |
|||
private String customerName; // 客户名称
|
|||
@ApiModelProperty("销售数量") |
|||
private String saleNum; // 销售数量
|
|||
@ApiModelProperty("销售渠道") |
|||
private String storeCode; // 销售渠道
|
|||
@ApiModelProperty("销售价格") |
|||
private String salePrice; // 销售价格
|
|||
@ApiModelProperty("销售成本") |
|||
private String saleCost; // 销售成本
|
|||
@ApiModelProperty("利润") |
|||
private String profit; // 利润
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate; // 数据日期
|
|||
} |
@ -0,0 +1,59 @@ |
|||
package com.yxt.supervise.customer.api.gdsalesyc; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 8:48 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "销售数据(烟草) 视图数据详情", description = "销售数据(烟草) 视图数据详情") |
|||
public class GdSalesYcDetailsVo implements Vo { |
|||
private String sid; // sid
|
|||
|
|||
@ApiModelProperty("创建者") |
|||
private String createSid; // 创建者
|
|||
@ApiModelProperty("更新者") |
|||
private String modifySid; // 更新者
|
|||
@ApiModelProperty("销售订单号") |
|||
private String code; // 销售订单号
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode; // 商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode; // 商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName; // 商品名称
|
|||
@ApiModelProperty("销售数量") |
|||
private String saleNum; // 销售数量
|
|||
@ApiModelProperty("销售渠道") |
|||
private String storeCode; // 销售渠道
|
|||
@ApiModelProperty("销售渠道名称") |
|||
private String storeName; // 销售渠道名称
|
|||
@ApiModelProperty("销售价格") |
|||
private String salePrice; // 销售价格
|
|||
@ApiModelProperty("销售成本") |
|||
private String saleCost; // 销售成本
|
|||
@ApiModelProperty("利润") |
|||
private String profit; // 利润
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate; // 数据日期
|
|||
@ApiModelProperty("类型") |
|||
private String orderType; // 类型
|
|||
@ApiModelProperty("客户代码") |
|||
private String customerCode; // 客户代码
|
|||
@ApiModelProperty("客户名称") |
|||
private String customerName; // 客户名称
|
|||
@ApiModelProperty("品牌代码") |
|||
private String brandCode; // 品牌代码
|
|||
@ApiModelProperty("品牌名称") |
|||
private String brandName; // 品牌名称
|
|||
@ApiModelProperty("大类") |
|||
private String categoryb; // 大类
|
|||
@ApiModelProperty("种类") |
|||
private String categorym; // 种类
|
|||
@ApiModelProperty("小类") |
|||
private String categorys; // 小类
|
|||
} |
@ -0,0 +1,59 @@ |
|||
package com.yxt.supervise.customer.api.gdsalesyc; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 8:49 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "销售数据 数据传输对象", description = "销售数据 数据传输对象") |
|||
public class GdSalesYcDto implements Dto { |
|||
private String sid; // sid
|
|||
|
|||
@ApiModelProperty("创建者") |
|||
private String createSid; // 创建者
|
|||
@ApiModelProperty("更新者") |
|||
private String modifySid; // 更新者
|
|||
@ApiModelProperty("销售订单号") |
|||
private String code; // 销售订单号
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode; // 商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode; // 商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName; // 商品名称
|
|||
@ApiModelProperty("销售数量") |
|||
private String saleNum; // 销售数量
|
|||
@ApiModelProperty("销售渠道") |
|||
private String storeCode; // 销售渠道
|
|||
@ApiModelProperty("销售渠道名称") |
|||
private String storeName; // 销售渠道名称
|
|||
@ApiModelProperty("销售价格") |
|||
private String salePrice; // 销售价格
|
|||
@ApiModelProperty("销售成本") |
|||
private String saleCost; // 销售成本
|
|||
@ApiModelProperty("利润") |
|||
private String profit; // 利润
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate; // 数据日期
|
|||
@ApiModelProperty("类型") |
|||
private String orderType; // 类型
|
|||
@ApiModelProperty("客户代码") |
|||
private String customerCode; // 客户代码
|
|||
@ApiModelProperty("客户名称") |
|||
private String customerName; // 客户名称
|
|||
@ApiModelProperty("品牌代码") |
|||
private String brandCode; // 品牌代码
|
|||
@ApiModelProperty("品牌名称") |
|||
private String brandName; // 品牌名称
|
|||
@ApiModelProperty("大类") |
|||
private String categoryb; // 大类
|
|||
@ApiModelProperty("种类") |
|||
private String categorym; // 种类
|
|||
@ApiModelProperty("小类") |
|||
private String categorys; // 小类
|
|||
} |
@ -0,0 +1,72 @@ |
|||
package com.yxt.supervise.customer.api.gdsalesyc; |
|||
|
|||
import com.alibaba.excel.annotation.ExcelProperty; |
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 8:49 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "销售数据 导出excel视图数据对象", description = "销售数据 导出excel视图数据对象") |
|||
public class GdSalesYcExcelVo implements Vo { |
|||
// private String sid; // sid
|
|||
//
|
|||
// @ApiModelProperty("创建者")
|
|||
// private String createSid; // 创建者
|
|||
// @ApiModelProperty("更新者")
|
|||
// private String modifySid; // 更新者
|
|||
@ExcelProperty(value = "销售订单号") |
|||
@ApiModelProperty("销售订单号") |
|||
private String code; // 销售订单号
|
|||
@ExcelProperty(value = "商品编码") |
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode; // 商品编码
|
|||
// @ApiModelProperty("商品条码")
|
|||
// private String prodBarCode; // 商品条码
|
|||
@ExcelProperty(value = "商品名称") |
|||
@ApiModelProperty("商品名称") |
|||
private String prodName; // 商品名称
|
|||
@ExcelProperty(value = "销售数量") |
|||
@ApiModelProperty("销售数量") |
|||
private String saleNum; // 销售数量
|
|||
// @ApiModelProperty("销售渠道")
|
|||
// private String storeCode; // 销售渠道
|
|||
@ExcelProperty(value = "销售渠道") |
|||
@ApiModelProperty("销售渠道名称") |
|||
private String storeName; // 销售渠道名称
|
|||
// @ApiModelProperty("销售价格")
|
|||
// private String salePrice; // 销售价格
|
|||
// @ApiModelProperty("销售成本")
|
|||
// private String saleCost; // 销售成本
|
|||
// @ApiModelProperty("利润")
|
|||
// private String profit; // 利润
|
|||
@ExcelProperty(value = "数据日期") |
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate; // 数据日期
|
|||
@ExcelProperty(value = "类型") |
|||
@ApiModelProperty("类型") |
|||
private String orderType; // 类型
|
|||
// @ApiModelProperty("客户代码")
|
|||
// private String customerCode; // 客户代码
|
|||
// @ApiModelProperty("客户名称")
|
|||
// private String customerName; // 客户名称
|
|||
@ExcelProperty(value = "品牌编码") |
|||
@ApiModelProperty("品牌代码") |
|||
private String brandCode; // 品牌代码
|
|||
@ExcelProperty(value = "品牌名称") |
|||
@ApiModelProperty("品牌名称") |
|||
private String brandName; // 品牌名称
|
|||
// @ApiModelProperty("大类")
|
|||
// private String categoryb; // 大类
|
|||
// @ApiModelProperty("种类")
|
|||
// private String categorym; // 种类
|
|||
// @ApiModelProperty("小类")
|
|||
// private String categorys; // 小类
|
|||
@ExcelProperty(value = "总价") |
|||
@ApiModelProperty("总价") |
|||
private String total; |
|||
} |
@ -0,0 +1,18 @@ |
|||
package com.yxt.supervise.customer.api.gdsalesyc; |
|||
|
|||
import com.yxt.supervise.customer.api.gdsales.GdSalesFeignFallback; |
|||
import io.swagger.annotations.Api; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 8:49 |
|||
*/ |
|||
@Api(tags = "销售数据(烟草)") |
|||
@FeignClient( |
|||
contextId = "yxt-supervise-SalesYcData", |
|||
name = "yxt-supervise", |
|||
path = "v1/gdsalesyc", |
|||
fallback = GdSalesYcFeignFallback.class) |
|||
public interface GdSalesYcFeign { |
|||
} |
@ -0,0 +1,11 @@ |
|||
package com.yxt.supervise.customer.api.gdsalesyc; |
|||
|
|||
import org.springframework.stereotype.Component; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 8:49 |
|||
*/ |
|||
@Component |
|||
public class GdSalesYcFeignFallback implements GdSalesYcFeign{ |
|||
} |
@ -0,0 +1,62 @@ |
|||
package com.yxt.supervise.customer.api.gdsalesyc; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 8:50 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "销售数据(烟草) 查询条件", description = "销售数据(烟草) 查询条件") |
|||
public class GdSalesYcQuery implements Query { |
|||
|
|||
@ApiModelProperty("创建者") |
|||
private String createSid; // 创建者
|
|||
@ApiModelProperty("更新者") |
|||
private String modifySid; // 更新者
|
|||
@ApiModelProperty("销售订单号") |
|||
private String code; // 销售订单号
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode; // 商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode; // 商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName; // 商品名称
|
|||
@ApiModelProperty("销售数量") |
|||
private String saleNum; // 销售数量
|
|||
@ApiModelProperty("销售渠道") |
|||
private String storeCode; // 销售渠道
|
|||
@ApiModelProperty("销售价格") |
|||
private String salePrice; // 销售价格
|
|||
@ApiModelProperty("销售渠道名称") |
|||
private String storeName; // 销售渠道名称
|
|||
@ApiModelProperty("销售成本") |
|||
private String saleCost; // 销售成本
|
|||
@ApiModelProperty("利润") |
|||
private String profit; // 利润
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate; // 数据日期
|
|||
@ApiModelProperty("类型") |
|||
private String orderType; // 类型
|
|||
@ApiModelProperty("客户代码") |
|||
private String customerCode; // 客户代码
|
|||
@ApiModelProperty("客户名称") |
|||
private String customerName; // 客户名称
|
|||
@ApiModelProperty("品牌代码") |
|||
private String brandCode; // 品牌代码
|
|||
@ApiModelProperty("品牌名称") |
|||
private String brandName; // 品牌名称
|
|||
@ApiModelProperty("大类") |
|||
private String categoryb; // 大类
|
|||
@ApiModelProperty("种类") |
|||
private String categorym; // 种类
|
|||
@ApiModelProperty("小类") |
|||
private String categorys; // 小类
|
|||
//开始时间
|
|||
private String startTime; |
|||
//结束时间
|
|||
private String endTime; |
|||
} |
@ -0,0 +1,72 @@ |
|||
package com.yxt.supervise.customer.api.gdsalesyc; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/31 8:50 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "销售数据(烟草)", description = "销售数据(烟草)") |
|||
public class GdSalesYcVo implements Vo { |
|||
private String sid; // sid
|
|||
|
|||
@ApiModelProperty("创建者") |
|||
private String createSid; // 创建者
|
|||
@ApiModelProperty("更新者") |
|||
private String modifySid; // 更新者
|
|||
|
|||
@ApiModelProperty("销售订单号") |
|||
private String code; // 销售订单号
|
|||
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode; // 商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode; // 商品条码
|
|||
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName; // 商品名称
|
|||
|
|||
@ApiModelProperty("销售数量") |
|||
private String saleNum; // 销售数量
|
|||
@ApiModelProperty("销售渠道") |
|||
private String storeCode; // 销售渠道
|
|||
|
|||
@ApiModelProperty("销售渠道名称") |
|||
private String storeName; // 销售渠道名称
|
|||
@ApiModelProperty("销售价格") |
|||
private String salePrice; // 销售价格
|
|||
@ApiModelProperty("销售成本") |
|||
private String saleCost; // 销售成本
|
|||
@ApiModelProperty("利润") |
|||
private String profit; // 利润
|
|||
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate; // 数据日期
|
|||
|
|||
@ApiModelProperty("类型") |
|||
private String orderType; // 类型
|
|||
@ApiModelProperty("客户代码") |
|||
private String customerCode; // 客户代码
|
|||
@ApiModelProperty("客户名称") |
|||
private String customerName; // 客户名称
|
|||
|
|||
@ApiModelProperty("品牌代码") |
|||
private String brandCode; // 品牌代码
|
|||
|
|||
@ApiModelProperty("品牌名称") |
|||
private String brandName; // 品牌名称
|
|||
@ApiModelProperty("大类") |
|||
private String categoryb; // 大类
|
|||
@ApiModelProperty("种类") |
|||
private String categorym; // 种类
|
|||
@ApiModelProperty("小类") |
|||
private String categorys; // 小类
|
|||
|
|||
@ApiModelProperty("总价") |
|||
private String total; |
|||
} |
@ -0,0 +1,55 @@ |
|||
package com.yxt.supervise.customer.api.gdwholesalegd; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.EntityWithId; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 8:55 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "配送中心批发数据", description = "配送中心批发数据") |
|||
@TableName("gd_wholesale_gd") |
|||
public class GdWholesaleGd extends EntityWithId { |
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String orgCode; // 企业组织机构代码证
|
|||
@ApiModelProperty("订单类型") |
|||
private String orderType; // 订单类型
|
|||
@ApiModelProperty("销售订单号") |
|||
private String orderNo; // 销售订单号
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode; // 商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode; // 商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName; // 商品名称
|
|||
@ApiModelProperty("品牌代码") |
|||
private String brandCode; // 品牌代码
|
|||
@ApiModelProperty("品牌名称") |
|||
private String brandName; // 品牌名称
|
|||
@ApiModelProperty("商品大类") |
|||
private String categoryb; // 商品大类
|
|||
@ApiModelProperty("商品中类") |
|||
private String categorym; // 商品中类
|
|||
@ApiModelProperty("商品小类") |
|||
private String categorys; // 商品小类
|
|||
@ApiModelProperty("客户代码") |
|||
private String customerCode; // 客户代码
|
|||
@ApiModelProperty("客户名称") |
|||
private String customerName; // 客户名称
|
|||
@ApiModelProperty("销售数量") |
|||
private String saleNum; // 销售数量
|
|||
@ApiModelProperty("销售渠道") |
|||
private String storeCode; // 销售渠道
|
|||
@ApiModelProperty("销售价格") |
|||
private String salePrice; // 销售价格
|
|||
@ApiModelProperty("销售成本") |
|||
private String saleCost; // 销售成本
|
|||
@ApiModelProperty("利润") |
|||
private String profit; // 利润
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate; // 数据日期
|
|||
} |
@ -0,0 +1,53 @@ |
|||
package com.yxt.supervise.customer.api.gdwholesalegd; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 8:59 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "配送中心批发数据", description = "配送中心批发数据") |
|||
public class GdWholesaleGdDetailsVo implements Vo { |
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String orgCode; // 企业组织机构代码证
|
|||
@ApiModelProperty("订单类型") |
|||
private String orderType; // 订单类型
|
|||
@ApiModelProperty("销售订单号") |
|||
private String orderNo; // 销售订单号
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode; // 商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode; // 商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName; // 商品名称
|
|||
@ApiModelProperty("品牌代码") |
|||
private String brandCode; // 品牌代码
|
|||
@ApiModelProperty("品牌名称") |
|||
private String brandName; // 品牌名称
|
|||
@ApiModelProperty("商品大类") |
|||
private String categoryb; // 商品大类
|
|||
@ApiModelProperty("商品中类") |
|||
private String categorym; // 商品中类
|
|||
@ApiModelProperty("商品小类") |
|||
private String categorys; // 商品小类
|
|||
@ApiModelProperty("客户代码") |
|||
private String customerCode; // 客户代码
|
|||
@ApiModelProperty("客户名称") |
|||
private String customerName; // 客户名称
|
|||
@ApiModelProperty("销售数量") |
|||
private String saleNum; // 销售数量
|
|||
@ApiModelProperty("销售渠道") |
|||
private String storeCode; // 销售渠道
|
|||
@ApiModelProperty("销售价格") |
|||
private String salePrice; // 销售价格
|
|||
@ApiModelProperty("销售成本") |
|||
private String saleCost; // 销售成本
|
|||
@ApiModelProperty("利润") |
|||
private String profit; // 利润
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate; // 数据日期
|
|||
} |
@ -0,0 +1,53 @@ |
|||
package com.yxt.supervise.customer.api.gdwholesalegd; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 9:01 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "配送中心批发数据", description = "配送中心批发数据") |
|||
public class GdWholesaleGdDto implements Dto { |
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String orgCode; // 企业组织机构代码证
|
|||
@ApiModelProperty("订单类型") |
|||
private String orderType; // 订单类型
|
|||
@ApiModelProperty("销售订单号") |
|||
private String orderNo; // 销售订单号
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode; // 商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode; // 商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName; // 商品名称
|
|||
@ApiModelProperty("品牌代码") |
|||
private String brandCode; // 品牌代码
|
|||
@ApiModelProperty("品牌名称") |
|||
private String brandName; // 品牌名称
|
|||
@ApiModelProperty("商品大类") |
|||
private String categoryb; // 商品大类
|
|||
@ApiModelProperty("商品中类") |
|||
private String categorym; // 商品中类
|
|||
@ApiModelProperty("商品小类") |
|||
private String categorys; // 商品小类
|
|||
@ApiModelProperty("客户代码") |
|||
private String customerCode; // 客户代码
|
|||
@ApiModelProperty("客户名称") |
|||
private String customerName; // 客户名称
|
|||
@ApiModelProperty("销售数量") |
|||
private String saleNum; // 销售数量
|
|||
@ApiModelProperty("销售渠道") |
|||
private String storeCode; // 销售渠道
|
|||
@ApiModelProperty("销售价格") |
|||
private String salePrice; // 销售价格
|
|||
@ApiModelProperty("销售成本") |
|||
private String saleCost; // 销售成本
|
|||
@ApiModelProperty("利润") |
|||
private String profit; // 利润
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate; // 数据日期
|
|||
} |
@ -0,0 +1,47 @@ |
|||
package com.yxt.supervise.customer.api.gdwholesalegd; |
|||
|
|||
import com.alibaba.excel.annotation.ExcelProperty; |
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 9:44 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "配送中心批发数据 导出excel视图数据对象", description = "配送中心批发数据 导出excel视图数据对象") |
|||
public class GdWholesaleGdExcelVo implements Vo { |
|||
@ExcelProperty(value = "销售订单号") |
|||
@ApiModelProperty("销售订单号") |
|||
private String code; // 销售订单号
|
|||
@ExcelProperty(value = "商品编码") |
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode; // 商品编码
|
|||
@ExcelProperty(value = "商品名称") |
|||
@ApiModelProperty("商品名称") |
|||
private String prodName; // 商品名称
|
|||
@ExcelProperty(value = "品牌代码") |
|||
@ApiModelProperty("品牌代码") |
|||
private String brandCode; // 品牌代码
|
|||
@ExcelProperty(value = "品牌名称") |
|||
@ApiModelProperty("品牌名称") |
|||
private String brandName; // 品牌名称
|
|||
@ExcelProperty(value = "销售数量") |
|||
@ApiModelProperty("销售数量") |
|||
private String saleNum; // 销售数量
|
|||
@ExcelProperty(value = "类型") |
|||
@ApiModelProperty("类型") |
|||
private String orderType; // 类型
|
|||
@ExcelProperty(value = "销售渠道") |
|||
@ApiModelProperty("销售渠道") |
|||
private String storeName; //
|
|||
@ExcelProperty(value = "数据日期") |
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate; // 数据日期
|
|||
@ExcelProperty(value = "价格") |
|||
@ApiModelProperty("总价") |
|||
private String total; //
|
|||
|
|||
} |
@ -0,0 +1,18 @@ |
|||
package com.yxt.supervise.customer.api.gdwholesalegd; |
|||
|
|||
import com.yxt.supervise.customer.api.gdsales.GdSalesFeignFallback; |
|||
import io.swagger.annotations.Api; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 9:05 |
|||
*/ |
|||
@Api(tags = "配送中心批发数据") |
|||
@FeignClient( |
|||
contextId = "yxt-supervise-GdWholesaleGd", |
|||
name = "yxt-supervise", |
|||
path = "v1/gdwholesalegd", |
|||
fallback = GdSalesFeignFallback.class) |
|||
public interface GdWholesaleGdFeign { |
|||
} |
@ -0,0 +1,11 @@ |
|||
package com.yxt.supervise.customer.api.gdwholesalegd; |
|||
|
|||
import org.springframework.stereotype.Component; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 9:05 |
|||
*/ |
|||
@Component |
|||
public class GdWholesaleGdFeignFallback implements GdWholesaleGdFeign{ |
|||
} |
@ -0,0 +1,57 @@ |
|||
package com.yxt.supervise.customer.api.gdwholesalegd; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 9:05 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "配送中心批发数据 查询条件", description = "配送中心批发数据 查询条件") |
|||
public class GdWholesaleGdQuery implements Query { |
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String orgCode; // 企业组织机构代码证
|
|||
@ApiModelProperty("订单类型") |
|||
private String orderType; // 订单类型
|
|||
@ApiModelProperty("销售订单号") |
|||
private String orderNo; // 销售订单号
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode; // 商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode; // 商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName; // 商品名称
|
|||
@ApiModelProperty("品牌代码") |
|||
private String brandCode; // 品牌代码
|
|||
@ApiModelProperty("品牌名称") |
|||
private String brandName; // 品牌名称
|
|||
@ApiModelProperty("商品大类") |
|||
private String categoryb; // 商品大类
|
|||
@ApiModelProperty("商品中类") |
|||
private String categorym; // 商品中类
|
|||
@ApiModelProperty("商品小类") |
|||
private String categorys; // 商品小类
|
|||
@ApiModelProperty("客户代码") |
|||
private String customerCode; // 客户代码
|
|||
@ApiModelProperty("客户名称") |
|||
private String customerName; // 客户名称
|
|||
@ApiModelProperty("销售数量") |
|||
private String saleNum; // 销售数量
|
|||
@ApiModelProperty("销售渠道") |
|||
private String storeCode; // 销售渠道
|
|||
@ApiModelProperty("销售价格") |
|||
private String salePrice; // 销售价格
|
|||
@ApiModelProperty("销售成本") |
|||
private String saleCost; // 销售成本
|
|||
@ApiModelProperty("利润") |
|||
private String profit; // 利润
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate; // 数据日期
|
|||
@ApiModelProperty("开始日期") |
|||
private String startDate; |
|||
@ApiModelProperty("结束日期") |
|||
private String endDate; |
|||
} |
@ -0,0 +1,57 @@ |
|||
package com.yxt.supervise.customer.api.gdwholesalegd; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 9:06 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "配送中心批发数据 视图数据对象", description = "配送中心批发数据 视图数据对象") |
|||
public class GdWholesaleGdVo implements Vo { |
|||
@ApiModelProperty("企业组织机构代码证") |
|||
private String orgCode; // 企业组织机构代码证
|
|||
@ApiModelProperty("订单类型") |
|||
private String orderType; // 订单类型
|
|||
@ApiModelProperty("销售订单号") |
|||
private String orderNo; // 销售订单号
|
|||
@ApiModelProperty("商品编码") |
|||
private String prodCode; // 商品编码
|
|||
@ApiModelProperty("商品条码") |
|||
private String prodBarCode; // 商品条码
|
|||
@ApiModelProperty("商品名称") |
|||
private String prodName; // 商品名称
|
|||
@ApiModelProperty("品牌代码") |
|||
private String brandCode; // 品牌代码
|
|||
@ApiModelProperty("品牌名称") |
|||
private String brandName; // 品牌名称
|
|||
@ApiModelProperty("商品大类") |
|||
private String categoryb; // 商品大类
|
|||
@ApiModelProperty("商品中类") |
|||
private String categorym; // 商品中类
|
|||
@ApiModelProperty("商品小类") |
|||
private String categorys; // 商品小类
|
|||
@ApiModelProperty("客户代码") |
|||
private String customerCode; // 客户代码
|
|||
@ApiModelProperty("客户名称") |
|||
private String customerName; // 客户名称
|
|||
@ApiModelProperty("销售数量") |
|||
private String saleNum; // 销售数量
|
|||
@ApiModelProperty("销售渠道") |
|||
private String storeCode; // 销售渠道
|
|||
@ApiModelProperty("渠道名称") |
|||
private String storeName; |
|||
@ApiModelProperty("销售价格") |
|||
private String salePrice; // 销售价格
|
|||
@ApiModelProperty("销售成本") |
|||
private String saleCost; // 销售成本
|
|||
@ApiModelProperty("利润") |
|||
private String profit; // 利润
|
|||
@ApiModelProperty("数据日期") |
|||
private String dataDate; // 数据日期
|
|||
@ApiModelProperty("总价") |
|||
private String total; |
|||
} |
@ -0,0 +1,156 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.supervise.customer.api.productinformation; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
|
|||
/** |
|||
* Project: supervise-customer(客户中心) <br/> |
|||
* File: ProductInformation.java <br/> |
|||
* Class: com.supervise.customer.api.productinformation.ProductInformation <br/> |
|||
* Description: 商品档案信息. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2022-11-11 11:40:29 <br/> |
|||
* |
|||
* @author dongjianzhao |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "商品档案信息", description = "商品档案信息") |
|||
@TableName("product_information") |
|||
public class ProductInformation extends BaseEntity { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty("代码") |
|||
private String code; |
|||
@ApiModelProperty("名称") |
|||
private String name; |
|||
@ApiModelProperty("含量") |
|||
private String content; |
|||
@ApiModelProperty("类别") |
|||
private String category; |
|||
@ApiModelProperty("类别key") |
|||
private String categoryKey; |
|||
@ApiModelProperty("规格单位") |
|||
private String unit; |
|||
@ApiModelProperty("制造厂") |
|||
private String manufacturer; |
|||
@ApiModelProperty("制造厂sid") |
|||
private String manufacturerSid; |
|||
@ApiModelProperty("品牌") |
|||
private String brand; |
|||
@ApiModelProperty("品牌sid") |
|||
private String brandSid; |
|||
@ApiModelProperty("产地") |
|||
private String placeOfOrigin; |
|||
@ApiModelProperty("等级") |
|||
private String grade; |
|||
@ApiModelProperty("等级key") |
|||
private String gradeKey; |
|||
@ApiModelProperty("保质期") |
|||
private String qualityGuaranteePeriod; |
|||
@ApiModelProperty("部门") |
|||
private String deptartment; |
|||
@ApiModelProperty("货架") |
|||
private String goodsShelves; |
|||
@ApiModelProperty("货架code") |
|||
private String goodsShelvesCode; |
|||
@ApiModelProperty("第二代码") |
|||
private String secondCode; |
|||
@ApiModelProperty("毛利率") |
|||
private String grossProfitMargin; |
|||
@ApiModelProperty("采购员") |
|||
private String buyer; |
|||
@ApiModelProperty("核算售价") |
|||
private String accountingSalesPrice; |
|||
@ApiModelProperty("最新进价(系统中导出的)") |
|||
private String latestPurchasePrice; |
|||
@ApiModelProperty("库存价") |
|||
private String inventoryPrice; |
|||
@ApiModelProperty("合同进价") |
|||
private String contractPurchasePrice; |
|||
@ApiModelProperty("会员价") |
|||
private String membershipPrice; |
|||
@ApiModelProperty("进项税率") |
|||
private String inputTaxRate; |
|||
@ApiModelProperty("销项税率") |
|||
private String outputTaxRate; |
|||
@ApiModelProperty("仓位") |
|||
private String position; |
|||
@ApiModelProperty("仓位code") |
|||
private String positionCode; |
|||
@ApiModelProperty("结算供应商") |
|||
private String supplier; |
|||
@ApiModelProperty("结算供应商Sid") |
|||
private String supplierSid; |
|||
@ApiModelProperty("配货方式") |
|||
private String distributionMethod; |
|||
@ApiModelProperty("配货方式Key") |
|||
private String distributionMethodKey; |
|||
@ApiModelProperty("来源单位") |
|||
private String sourceUnit; |
|||
@ApiModelProperty("管理到效期") |
|||
private String managementExpirationDate; |
|||
@ApiModelProperty("配货价") |
|||
private String rationingPrice; |
|||
@ApiModelProperty("最低售价") |
|||
private String lowestSellingPrice; |
|||
@ApiModelProperty("缺省进价") |
|||
private String defaultPurchasePrice; |
|||
@ApiModelProperty("批发价") |
|||
private String tradePrice; |
|||
@ApiModelProperty("规格说明") |
|||
private String specifications; |
|||
@ApiModelProperty("规格长宽高") |
|||
private String description; |
|||
@ApiModelProperty("规格重量") |
|||
private String weight; |
|||
@ApiModelProperty("最后调价时间") |
|||
private Date priceAdjustmentTime; |
|||
@ApiModelProperty("对方系统代码") |
|||
private String systemCode; |
|||
@ApiModelProperty("去税最新进价") |
|||
private String priceWithoutTax; |
|||
@ApiModelProperty("去税库存价") |
|||
private String taxableInventoryPrice; |
|||
@ApiModelProperty("去税合同进价") |
|||
private String ContractPriceExcludingTax; |
|||
@ApiModelProperty("商品状态") |
|||
private String productStatus; |
|||
@ApiModelProperty("商品状态key") |
|||
private String productStatusKey; |
|||
@ApiModelProperty("最新进价(需要每次导入采购订单更新的)") |
|||
private String newestPurchasePrice; |
|||
} |
@ -0,0 +1,155 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.supervise.customer.api.productinformation; |
|||
|
|||
|
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
|
|||
/** |
|||
* Project: supervise-customer(客户中心) <br/> |
|||
* File: ProductInformationVo.java <br/> |
|||
* Class: com.supervise.customer.api.productinformation.ProductInformationVo <br/> |
|||
* Description: 商品档案信息 视图数据对象. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2022-11-11 11:40:29 <br/> |
|||
* |
|||
* @author dongjianzhao |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "商品档案信息 视图数据详情", description = "商品档案信息 视图数据详情") |
|||
public class ProductInformationDetailsVo implements Vo { |
|||
|
|||
private static final long serialVersionUID = -7305436694722608899L; |
|||
private String sid; |
|||
@ApiModelProperty("代码") |
|||
private String code; |
|||
@ApiModelProperty("名称") |
|||
private String name; |
|||
@ApiModelProperty("类别") |
|||
private String category; |
|||
@ApiModelProperty("类别key") |
|||
private String categoryKey; |
|||
@ApiModelProperty("规格单位") |
|||
private String unit; |
|||
@ApiModelProperty("制造厂") |
|||
private String manufacturer; |
|||
@ApiModelProperty("制造厂sid") |
|||
private String manufacturerSid; |
|||
@ApiModelProperty("品牌") |
|||
private String brand; |
|||
@ApiModelProperty("品牌sid") |
|||
private String brandSid; |
|||
@ApiModelProperty("产地") |
|||
private String placeOfOrigin; |
|||
@ApiModelProperty("等级") |
|||
private String grade; |
|||
@ApiModelProperty("等级key") |
|||
private String gradeKey; |
|||
@ApiModelProperty("保质期") |
|||
private String qualityGuaranteePeriod; |
|||
|
|||
@ApiModelProperty("货架") |
|||
private String goodsShelves; |
|||
@ApiModelProperty("货架key") |
|||
private String goodsShelvesCode; |
|||
@ApiModelProperty("第二代码") |
|||
private String secondCode; |
|||
@ApiModelProperty("毛利率") |
|||
private String grossProfitMargin; |
|||
|
|||
@ApiModelProperty("核算售价") |
|||
private String accountingSalesPrice; |
|||
@ApiModelProperty("最新进价") |
|||
private String latestPurchasePrice; |
|||
@ApiModelProperty("库存价") |
|||
private String inventoryPrice; |
|||
@ApiModelProperty("合同进价") |
|||
private String contractPurchasePrice; |
|||
|
|||
@ApiModelProperty("会员价") |
|||
private String membershipPrice; |
|||
|
|||
@ApiModelProperty("进项利率") |
|||
private String inputTaxRate; |
|||
@ApiModelProperty("销项利率") |
|||
private String outputTaxRate; |
|||
@ApiModelProperty("仓位") |
|||
private String position; |
|||
@ApiModelProperty("仓位code") |
|||
private String positionCode; |
|||
@ApiModelProperty("结算供应商") |
|||
private String supplier; |
|||
@ApiModelProperty("结算供应商sid") |
|||
private String supplierSid; |
|||
@ApiModelProperty("配货方式") |
|||
private String distributionMethod; |
|||
@ApiModelProperty("配货方式key") |
|||
private String distributionMethodKey; |
|||
@ApiModelProperty("来源单位") |
|||
private String sourceUnit; |
|||
@ApiModelProperty("管理到货期") |
|||
private String managementExpirationDate; |
|||
|
|||
@ApiModelProperty("配货价") |
|||
private String rationingPrice; |
|||
@ApiModelProperty("最低售价") |
|||
private String lowestSellingPrice; |
|||
@ApiModelProperty("缺省进价") |
|||
private String defaultPurchasePrice; |
|||
@ApiModelProperty("批发价") |
|||
private String tradePrice; |
|||
@ApiModelProperty("规格说明") |
|||
private String specifications; |
|||
@ApiModelProperty("规格长宽高") |
|||
private String description; |
|||
@ApiModelProperty("规格重量") |
|||
private String weight; |
|||
@ApiModelProperty("最后调价时间") |
|||
private Date priceAdjustmentTime; |
|||
@ApiModelProperty("对方系统代码") |
|||
private String systemCode; |
|||
@ApiModelProperty("去税最新进价") |
|||
private String priceWithoutTax; |
|||
@ApiModelProperty("去税库存价") |
|||
private String taxableInventoryPrice; |
|||
@ApiModelProperty("去税合同进价") |
|||
private String ContractPriceExcludingTax; |
|||
@ApiModelProperty("商品状态") |
|||
private String productStatus; |
|||
@ApiModelProperty("商品状态key") |
|||
private String productStatusKey; |
|||
@ApiModelProperty("最新进价(需要每次导入采购订单更新的)") |
|||
private String newestPurchasePrice; |
|||
} |
@ -0,0 +1,155 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.supervise.customer.api.productinformation; |
|||
|
|||
|
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
|
|||
/** |
|||
* Project: supervise-customer(客户中心) <br/> |
|||
* File: ProductInformationDto.java <br/> |
|||
* Class: com.supervise.customer.api.productinformation.ProductInformationDto <br/> |
|||
* Description: 商品档案信息 数据传输对象. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2022-11-11 11:40:29 <br/> |
|||
* |
|||
* @author dongjianzhao |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "商品档案信息 数据传输对象", description = "商品档案信息 数据传输对象") |
|||
public class ProductInformationDto implements Dto { |
|||
|
|||
private static final long serialVersionUID = 2628182250934148420L; |
|||
private String sid; |
|||
@ApiModelProperty("代码") |
|||
private String code; |
|||
@ApiModelProperty("名称") |
|||
private String name; |
|||
@ApiModelProperty("类别") |
|||
private String category; |
|||
@ApiModelProperty("类别key") |
|||
private String categoryKey; |
|||
@ApiModelProperty("规格单位") |
|||
private String unit; |
|||
@ApiModelProperty("制造厂") |
|||
private String manufacturer; |
|||
@ApiModelProperty("制造厂sid") |
|||
private String manufacturerSid; |
|||
@ApiModelProperty("品牌") |
|||
private String brand; |
|||
@ApiModelProperty("品牌sid") |
|||
private String brandSid; |
|||
@ApiModelProperty("产地") |
|||
private String placeOfOrigin; |
|||
@ApiModelProperty("等级") |
|||
private String grade; |
|||
@ApiModelProperty("等级key") |
|||
private String gradeKey; |
|||
@ApiModelProperty("保质期") |
|||
private String qualityGuaranteePeriod; |
|||
|
|||
@ApiModelProperty("货架") |
|||
private String goodsShelves; |
|||
@ApiModelProperty("货架key") |
|||
private String goodsShelvesCode; |
|||
@ApiModelProperty("第二代码") |
|||
private String secondCode; |
|||
@ApiModelProperty("毛利率") |
|||
private String grossProfitMargin; |
|||
|
|||
@ApiModelProperty("核算售价") |
|||
private String accountingSalesPrice; |
|||
@ApiModelProperty("最新进价") |
|||
private String latestPurchasePrice; |
|||
@ApiModelProperty("库存价") |
|||
private String inventoryPrice; |
|||
@ApiModelProperty("合同进价") |
|||
private String contractPurchasePrice; |
|||
|
|||
@ApiModelProperty("会员价") |
|||
private String membershipPrice; |
|||
|
|||
@ApiModelProperty("进项利率") |
|||
private String inputTaxRate; |
|||
@ApiModelProperty("销项利率") |
|||
private String outputTaxRate; |
|||
@ApiModelProperty("仓位") |
|||
private String position; |
|||
@ApiModelProperty("仓位code") |
|||
private String positionCode; |
|||
@ApiModelProperty("结算供应商") |
|||
private String supplier; |
|||
@ApiModelProperty("结算供应商sid") |
|||
private String supplierSid; |
|||
@ApiModelProperty("配货方式") |
|||
private String distributionMethod; |
|||
@ApiModelProperty("配货方式key") |
|||
private String distributionMethodKey; |
|||
@ApiModelProperty("来源单位") |
|||
private String sourceUnit; |
|||
@ApiModelProperty("管理到货期") |
|||
private String managementExpirationDate; |
|||
|
|||
@ApiModelProperty("配货价") |
|||
private String rationingPrice; |
|||
@ApiModelProperty("最低售价") |
|||
private String lowestSellingPrice; |
|||
@ApiModelProperty("缺省进价") |
|||
private String defaultPurchasePrice; |
|||
@ApiModelProperty("批发价") |
|||
private String tradePrice; |
|||
@ApiModelProperty("规格说明") |
|||
private String specifications; |
|||
@ApiModelProperty("规格长宽高") |
|||
private String description; |
|||
@ApiModelProperty("规格重量") |
|||
private String weight; |
|||
@ApiModelProperty("最后调价时间") |
|||
private Date priceAdjustmentTime; |
|||
@ApiModelProperty("对方系统代码") |
|||
private String systemCode; |
|||
@ApiModelProperty("去税最新进价") |
|||
private String priceWithoutTax; |
|||
@ApiModelProperty("去税库存价") |
|||
private String taxableInventoryPrice; |
|||
@ApiModelProperty("去税合同进价") |
|||
private String ContractPriceExcludingTax; |
|||
@ApiModelProperty("商品状态") |
|||
private String productStatus; |
|||
@ApiModelProperty("商品状态key") |
|||
private String productStatusKey; |
|||
@ApiModelProperty("最新进价(需要每次导入采购订单更新的)") |
|||
private String newestPurchasePrice; |
|||
} |
@ -0,0 +1,76 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.supervise.customer.api.productinformation; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
/** |
|||
* Project: yxt_supervise(宇信通监管) <br/> |
|||
* File: ProductInformationFeign.java <br/> |
|||
* Class: com.supervise.api.productinformation.ProductInformationFeign <br/> |
|||
* Description: 商品档案信息. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2022-11-11 11:40:29 <br/> |
|||
* |
|||
* @author dongjianzhao |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Api(tags = "商品档案信息") |
|||
@FeignClient( |
|||
contextId = "supervise-customer-ProductInformation", |
|||
name = "supervise-customer", |
|||
path = "v1/productinformation", |
|||
fallback = ProductInformationFeignFallback.class) |
|||
public interface ProductInformationFeign { |
|||
|
|||
@ApiOperation("根据条件分页查询数据的列表") |
|||
@PostMapping("/listPage") |
|||
@ResponseBody |
|||
public ResultBean<PagerVo<ProductInformationVo>> listPage(@RequestBody PagerQuery<ProductInformationQuery> pq); |
|||
|
|||
@ApiOperation("新增或修改") |
|||
@PostMapping("/save") |
|||
@ResponseBody |
|||
public ResultBean save(@RequestBody ProductInformationDto dto); |
|||
|
|||
@ApiOperation("根据sid删除记录") |
|||
@DeleteMapping("/delBySid/{sid}") |
|||
@ResponseBody |
|||
public ResultBean delBySid(@PathVariable("sid")String sid); |
|||
|
|||
@ApiOperation("根据SID获取一条记录") |
|||
@GetMapping("/fetchDetailsBySid/{sid}") |
|||
@ResponseBody |
|||
public ResultBean<ProductInformationDetailsVo> fetchDetailsBySid(@PathVariable("sid") String sid); |
|||
} |
@ -0,0 +1,70 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.supervise.customer.api.productinformation; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import org.springframework.stereotype.Component; |
|||
|
|||
/** |
|||
* Project: supervise-customer(客户中心) <br/> |
|||
* File: ProductInformationFeignFallback.java <br/> |
|||
* Class: com.supervise.customer.api.productinformation.ProductInformationFeignFallback <br/> |
|||
* Description: 商品档案信息. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2022-11-11 11:40:29 <br/> |
|||
* |
|||
* @author dongjianzhao |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Component |
|||
public class ProductInformationFeignFallback implements ProductInformationFeign { |
|||
|
|||
@Override |
|||
public ResultBean<PagerVo<ProductInformationVo>> listPage(PagerQuery<ProductInformationQuery> pq) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
return rb.setMsg("接口yxt_supervise/productinformation/listPage无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean save(ProductInformationDto dto) { |
|||
return ResultBean.fireFail().setMsg("接口yxt_supervise/productinformation/save无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean delBySid(String sid) { |
|||
return ResultBean.fireFail().setMsg("接口yxt_supervise/productinformation/delBySids无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<ProductInformationDetailsVo> fetchDetailsBySid(String sid) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
return rb.setMsg("接口yxt_supervise/productinformation/fetchDetailsBySid无法访问"); |
|||
} |
|||
} |
@ -0,0 +1,60 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.supervise.customer.api.productinformation; |
|||
|
|||
|
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
|
|||
/** |
|||
* Project: supervise-customer(客户中心) <br/> |
|||
* File: ProductInformationQuery.java <br/> |
|||
* Class: com.supervise.customer.api.productinformation.ProductInformationQuery <br/> |
|||
* Description: 商品档案信息 查询条件. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2022-11-11 11:40:29 <br/> |
|||
* |
|||
* @author dongjianzhao |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "商品档案信息 查询条件", description = "商品档案信息 查询条件") |
|||
public class ProductInformationQuery implements Query { |
|||
|
|||
private static final long serialVersionUID = -2156227778094595808L; |
|||
|
|||
|
|||
@ApiModelProperty("名称") |
|||
private String name; |
|||
|
|||
} |
@ -0,0 +1,80 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.supervise.customer.api.productinformation; |
|||
|
|||
|
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
|
|||
/** |
|||
* Project: supervise-customer(客户中心) <br/> |
|||
* File: ProductInformationVo.java <br/> |
|||
* Class: com.supervise.customer.api.productinformation.ProductInformationVo <br/> |
|||
* Description: 商品档案信息 视图数据对象. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2022-11-11 11:40:29 <br/> |
|||
* |
|||
* @author dongjianzhao |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "商品档案信息 视图数据对象", description = "商品档案信息 视图数据对象") |
|||
public class ProductInformationVo implements Vo { |
|||
|
|||
private static final long serialVersionUID = 8544481348404723979L; |
|||
private String sid; |
|||
@ApiModelProperty("代码") |
|||
private String code; |
|||
@ApiModelProperty("名称") |
|||
private String name; |
|||
@ApiModelProperty("类别") |
|||
private String category; |
|||
@ApiModelProperty("规格单位") |
|||
private String unit; |
|||
@ApiModelProperty("制造厂") |
|||
private String manufacturer; |
|||
|
|||
@ApiModelProperty("品牌") |
|||
private String brand; |
|||
|
|||
@ApiModelProperty("产地") |
|||
private String placeOfOrigin; |
|||
|
|||
@ApiModelProperty("第二代码") |
|||
private String secondCode; |
|||
@ApiModelProperty("规格长宽高") |
|||
private String description; |
|||
@ApiModelProperty("最新进价(需要每次导入采购订单更新的)") |
|||
private String newestPurchasePrice; |
|||
|
|||
} |
@ -0,0 +1,74 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisition; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 13:42 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "订单采购信息", description = "订单采购信息") |
|||
@TableName("purchase_requisition") |
|||
public class PurchaseRequisition extends BaseEntity { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty("单号") |
|||
private String code; // 单号
|
|||
@ApiModelProperty("采购员姓名") |
|||
private String buyerName; // 采购员姓名
|
|||
@ApiModelProperty("采购员编码") |
|||
private String buyerCode; // 采购员编码
|
|||
@ApiModelProperty("采购日期") |
|||
private String purchaseDate; // 采购日期
|
|||
@ApiModelProperty("到货日期") |
|||
private String arrivalDate; // 到货日期
|
|||
@ApiModelProperty("采购组织名称") |
|||
private String purchasingOrgName; // 采购组织名称
|
|||
@ApiModelProperty("采购组织编码") |
|||
private String purchasingOrgCode; // 采购组织编码
|
|||
@ApiModelProperty("采购部门名称") |
|||
private String purchasingDeptCode; // 采购部门名称
|
|||
@ApiModelProperty("采购部门编码") |
|||
private String purchasingDeptName; // 采购部门编码
|
|||
@ApiModelProperty("供应商名称") |
|||
private String supplierName; // 供应商名称
|
|||
@ApiModelProperty("供应商编码") |
|||
private String supplierCode; // 供应商编码
|
|||
@ApiModelProperty("供应商唯一编码") |
|||
private String supplierOnlyCode; // 供应商编码
|
|||
@ApiModelProperty("物流公司名称") |
|||
private String logisticsCompanyName; // 物流公司名称
|
|||
@ApiModelProperty("物流公司编码") |
|||
private String logisticsCompanyCode; // 物流公司编码
|
|||
@ApiModelProperty("单据到效期") |
|||
private String expiryDate; // 单据到效期
|
|||
@ApiModelProperty("仓位") |
|||
private String warehousePosition;//仓位
|
|||
@ApiModelProperty("仓位code") |
|||
private String warehousePositionCode;//
|
|||
@ApiModelProperty("新订单编号") |
|||
private String newCode;//
|
|||
@ApiModelProperty("旧订单编号") |
|||
private String oldCode;//
|
|||
@ApiModelProperty("完成状态") |
|||
private String completionStatus;//完成状态
|
|||
@ApiModelProperty("完成状态") |
|||
private String completionStatusKey;//完成状态
|
|||
|
|||
|
|||
@ApiModelProperty("监管0未审核,1审核通过,2审核不通过") |
|||
private String purchaseState = "0"; |
|||
|
|||
@ApiModelProperty("监管审批意见") |
|||
private String purchaseRemarks; |
|||
|
|||
@ApiModelProperty("银行0未审核,1审核通过,2审核不通过") |
|||
private String bankState = "0"; // 银行0未审核,1审核通过,2审核不通过',
|
|||
|
|||
@ApiModelProperty("银行审批意见") |
|||
private String bankRemarks; // 银行审批意见',
|
|||
} |
@ -0,0 +1,63 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisition; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.ArrayList; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 13:46 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "采购订单表 视图数据详情", description = "采购订单表 视图数据详情") |
|||
public class PurchaseRequisitionDetailsVo implements Vo { |
|||
private String sid; // sid
|
|||
@ApiModelProperty("单号") |
|||
private String code; // 单号
|
|||
@ApiModelProperty("采购员姓名") |
|||
private String buyerName; // 采购员姓名
|
|||
@ApiModelProperty("采购员编码") |
|||
private String buyerCode; // 采购员编码
|
|||
@ApiModelProperty("采购日期") |
|||
private String purchaseDate; // 采购日期
|
|||
@ApiModelProperty("到货日期") |
|||
private String arrivalDate; // 到货日期
|
|||
@ApiModelProperty("创建日期") |
|||
private String createTime; |
|||
@ApiModelProperty("采购组织名称") |
|||
private String purchasingOrgName; // 采购组织名称
|
|||
@ApiModelProperty("采购组织编码") |
|||
private String purchasingOrgCode; // 采购组织编码
|
|||
@ApiModelProperty("采购部门名称") |
|||
private String purchasingDeptCode; // 采购部门名称
|
|||
@ApiModelProperty("采购部门编码") |
|||
private String purchasingDeptName; // 采购部门编码
|
|||
@ApiModelProperty("供应商名称") |
|||
private String supplierName; // 供应商名称
|
|||
@ApiModelProperty("供应商编码") |
|||
private String supplierCode; // 供应商编码
|
|||
@ApiModelProperty("供应商唯一编码") |
|||
private String supplierOnlyCode; // 供应商编码
|
|||
@ApiModelProperty("物流公司名称") |
|||
private String logisticsCompanyName; // 物流公司名称
|
|||
@ApiModelProperty("物流公司编码") |
|||
private String logisticsCompanyCode; // 物流公司编码
|
|||
@ApiModelProperty("单据到效期") |
|||
private String expiryDate; // 单据到效期
|
|||
@ApiModelProperty("仓位") |
|||
private String warehousePosition;//仓位
|
|||
@ApiModelProperty("仓位code") |
|||
private String warehousePositionCode;//
|
|||
@ApiModelProperty("新订单编号") |
|||
private String newCode;//
|
|||
@ApiModelProperty("旧订单编号") |
|||
private String oldCode;//
|
|||
@ApiModelProperty("完成状态") |
|||
private String completionStatus;//完成状态
|
|||
@ApiModelProperty("完成状态") |
|||
private String completionStatusKey;//完成状态
|
|||
} |
@ -0,0 +1,56 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisition; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 13:46 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "采购订单表 数据传输对象", description = "采购订单表 数据传输对象") |
|||
public class PurchaseRequisitionDto implements Dto { |
|||
private String sid; // sid
|
|||
@ApiModelProperty("单号") |
|||
private String code; // 单号
|
|||
@ApiModelProperty("采购员姓名") |
|||
private String buyerName; // 采购员姓名
|
|||
@ApiModelProperty("采购员编码") |
|||
private String buyerCode; // 采购员编码
|
|||
@ApiModelProperty("采购日期") |
|||
private String purchaseDate; // 采购日期
|
|||
@ApiModelProperty("到货日期") |
|||
private String arrivalDate; // 到货日期
|
|||
@ApiModelProperty("采购组织名称") |
|||
private String purchasingOrgName; // 采购组织名称
|
|||
@ApiModelProperty("采购组织编码") |
|||
private String purchasingOrgCode; // 采购组织编码
|
|||
@ApiModelProperty("采购部门名称") |
|||
private String purchasingDeptCode; // 采购部门名称
|
|||
@ApiModelProperty("采购部门编码") |
|||
private String purchasingDeptName; // 采购部门编码
|
|||
@ApiModelProperty("供应商名称") |
|||
private String supplierName; // 供应商名称
|
|||
@ApiModelProperty("供应商编码") |
|||
private String supplierCode; // 供应商编码
|
|||
@ApiModelProperty("物流公司名称") |
|||
private String logisticsCompanyName; // 物流公司名称
|
|||
@ApiModelProperty("物流公司编码") |
|||
private String logisticsCompanyCode; // 物流公司编码
|
|||
@ApiModelProperty("单据到效期") |
|||
private String expiryDate; // 单据到效期
|
|||
@ApiModelProperty("仓位") |
|||
private String warehousePosition;//仓位
|
|||
@ApiModelProperty("仓位code") |
|||
private String warehousePositionCode;//
|
|||
@ApiModelProperty("新订单编号") |
|||
private String newCode;//
|
|||
@ApiModelProperty("旧订单编号") |
|||
private String oldCode;//
|
|||
@ApiModelProperty("完成状态") |
|||
private String completionStatus;//完成状态
|
|||
@ApiModelProperty("完成状态") |
|||
private String completionStatusKey;//完成状态
|
|||
} |
@ -0,0 +1,41 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisition; |
|||
|
|||
import com.alibaba.excel.annotation.ExcelProperty; |
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 15:00 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "采购订单表 导出excel视图数据对象", description = "采购订单表 导出excel视图数据对象") |
|||
public class PurchaseRequisitionExcelVo implements Vo { |
|||
@ExcelProperty(value = "订单号") |
|||
@ApiModelProperty("订单号") |
|||
private String code; // 订单号
|
|||
@ExcelProperty(value = "采购日期") |
|||
@ApiModelProperty("采购日期") |
|||
private String purchaseDate; // 采购日期
|
|||
@ExcelProperty(value = "采购日期") |
|||
@ApiModelProperty("建单日期") |
|||
private String createTime; // 采购日期
|
|||
@ExcelProperty(value = "到货日期") |
|||
@ApiModelProperty("到货日期") |
|||
private String arrivalDate; // 到货日期
|
|||
@ExcelProperty(value = "供应商名称") |
|||
@ApiModelProperty("供应商名称") |
|||
private String supplierName; // 供应商名称
|
|||
@ExcelProperty(value = "供应商编码") |
|||
@ApiModelProperty("供应商编码") |
|||
private String supplierCode; // 供应商编码
|
|||
@ExcelProperty(value = "供应商唯一编码") |
|||
@ApiModelProperty("供应商唯一编码") |
|||
private String supplierOnlyCode; |
|||
|
|||
|
|||
|
|||
|
|||
} |
@ -0,0 +1,17 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisition; |
|||
|
|||
import io.swagger.annotations.Api; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 13:47 |
|||
*/ |
|||
@Api(tags = "采购订单表") |
|||
@FeignClient( |
|||
contextId = "PurchaseRequisition", |
|||
name = "yxt-supervise", |
|||
path = "v1/purchaserequisition", |
|||
fallback = PurchaseRequisitionFeignFallback.class) |
|||
public interface PurchaseRequisitionFeign { |
|||
} |
@ -0,0 +1,11 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisition; |
|||
|
|||
import org.springframework.stereotype.Component; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 13:47 |
|||
*/ |
|||
@Component |
|||
public class PurchaseRequisitionFeignFallback implements PurchaseRequisitionFeign{ |
|||
} |
@ -0,0 +1,65 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisition; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 13:47 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "采购订单表 查询条件", description = "采购订单表 查询条件") |
|||
public class PurchaseRequisitionQuery implements Query { |
|||
@ApiModelProperty("单号") |
|||
private String code; // 单号
|
|||
@ApiModelProperty("采购员姓名") |
|||
private String buyerName; // 采购员姓名
|
|||
@ApiModelProperty("采购员编码") |
|||
private String buyerCode; // 采购员编码
|
|||
@ApiModelProperty("采购日期开始") |
|||
private String purchaseDateStart; // 采购日期
|
|||
@ApiModelProperty("采购日期结束") |
|||
private String purchaseDateEnd; // 采购日期
|
|||
@ApiModelProperty("到货日期开始") |
|||
private String arrivalDateStart; // 到货日期
|
|||
@ApiModelProperty("到货日期结束") |
|||
private String arrivalDateEnd; // 到货日期
|
|||
@ApiModelProperty("采购组织名称") |
|||
private String purchasingOrgName; // 采购组织名称
|
|||
@ApiModelProperty("采购组织编码") |
|||
private String purchasingOrgCode; // 采购组织编码
|
|||
@ApiModelProperty("采购部门名称") |
|||
private String purchasingDeptCode; // 采购部门名称
|
|||
@ApiModelProperty("采购部门编码") |
|||
private String purchasingDeptName; // 采购部门编码
|
|||
@ApiModelProperty("供应商名称") |
|||
private String supplierName; // 供应商名称
|
|||
@ApiModelProperty("供应商编码") |
|||
private String supplierCode; // 供应商编码
|
|||
@ApiModelProperty("供应商唯一编码") |
|||
private String supplierOnlyCode; // 供应商编码
|
|||
@ApiModelProperty("物流公司名称") |
|||
private String logisticsCompanyName; // 物流公司名称
|
|||
@ApiModelProperty("物流公司编码") |
|||
private String logisticsCompanyCode; // 物流公司编码
|
|||
@ApiModelProperty("单据到效期") |
|||
private String expiryDate; // 单据到效期
|
|||
@ApiModelProperty("仓位") |
|||
private String warehousePosition;//仓位
|
|||
@ApiModelProperty("仓位code") |
|||
private String warehousePositionCode;//
|
|||
@ApiModelProperty("新订单编号") |
|||
private String newCode;//
|
|||
@ApiModelProperty("旧订单编号") |
|||
private String oldCode;//
|
|||
@ApiModelProperty("完成状态") |
|||
private String completionStatus;//完成状态
|
|||
@ApiModelProperty("完成状态") |
|||
private String completionStatusKey;//完成状态
|
|||
@ApiModelProperty("开始时间") |
|||
private String startDate; |
|||
@ApiModelProperty("结束时间") |
|||
private String endDate; |
|||
} |
@ -0,0 +1,59 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisition; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 13:48 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "采购订单表 视图数据对象", description = "采购订单表 视图数据对象") |
|||
public class PurchaseRequisitionVo implements Vo { |
|||
@ApiModelProperty("单号") |
|||
private String code; // 单号
|
|||
@ApiModelProperty("采购员姓名") |
|||
private String buyerName; // 采购员姓名
|
|||
@ApiModelProperty("采购员编码") |
|||
private String buyerCode; // 采购员编码
|
|||
@ApiModelProperty("采购日期") |
|||
private String purchaseDate; // 采购日期
|
|||
@ApiModelProperty("到货日期") |
|||
private String arrivalDate; // 到货日期
|
|||
@ApiModelProperty("创建时间") |
|||
private String createTime; |
|||
@ApiModelProperty("采购组织名称") |
|||
private String purchasingOrgName; // 采购组织名称
|
|||
@ApiModelProperty("采购组织编码") |
|||
private String purchasingOrgCode; // 采购组织编码
|
|||
@ApiModelProperty("采购部门名称") |
|||
private String purchasingDeptCode; // 采购部门名称
|
|||
@ApiModelProperty("采购部门编码") |
|||
private String purchasingDeptName; // 采购部门编码
|
|||
@ApiModelProperty("供应商名称") |
|||
private String supplierName; // 供应商名称
|
|||
@ApiModelProperty("供应商编码") |
|||
private String supplierCode; // 供应商编码
|
|||
@ApiModelProperty("供应商唯一编码") |
|||
private String supplierOnlyCode; |
|||
@ApiModelProperty("物流公司名称") |
|||
private String logisticsCompanyName; // 物流公司名称
|
|||
@ApiModelProperty("物流公司编码") |
|||
private String logisticsCompanyCode; // 物流公司编码
|
|||
@ApiModelProperty("单据到效期") |
|||
private String expiryDate; // 单据到效期
|
|||
@ApiModelProperty("仓位") |
|||
private String warehousePosition;//仓位
|
|||
@ApiModelProperty("仓位code") |
|||
private String warehousePositionCode;//
|
|||
@ApiModelProperty("新订单编号") |
|||
private String newCode;//
|
|||
@ApiModelProperty("旧订单编号") |
|||
private String oldCode;//
|
|||
@ApiModelProperty("完成状态") |
|||
private String completionStatus;//完成状态
|
|||
@ApiModelProperty("完成状态") |
|||
private String completionStatusKey;//完成状态
|
|||
} |
@ -0,0 +1,57 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisitionpro; |
|||
|
|||
public class InTransitCheckVo { |
|||
|
|||
public InTransitCheckVo() { |
|||
} |
|||
|
|||
public InTransitCheckVo(String supplierOnlyCode) { |
|||
this.supplierOnlyCode = supplierOnlyCode; |
|||
// this.supplierName = supplierName;
|
|||
} |
|||
|
|||
private String supplierOnlyCode; |
|||
// private String supplierName;
|
|||
private double orderAmount = 0.0; |
|||
private double instorageAmount = 0.0; |
|||
|
|||
public String getSupplierOnlyCode() { |
|||
return supplierOnlyCode; |
|||
} |
|||
|
|||
public void setSupplierOnlyCode(String supplierOnlyCode) { |
|||
this.supplierOnlyCode = supplierOnlyCode; |
|||
} |
|||
|
|||
// public String getSupplierName() {
|
|||
// return supplierName;
|
|||
// }
|
|||
//
|
|||
// public void setSupplierName(String supplierName) {
|
|||
// this.supplierName = supplierName;
|
|||
// }
|
|||
|
|||
public double getOrderAmount() { |
|||
return orderAmount; |
|||
} |
|||
|
|||
public void setOrderAmount(double orderAmount) { |
|||
this.orderAmount = orderAmount; |
|||
} |
|||
|
|||
public double getInstorageAmount() { |
|||
return instorageAmount; |
|||
} |
|||
|
|||
public void setInstorageAmount(double instorageAmount) { |
|||
this.instorageAmount = instorageAmount; |
|||
} |
|||
|
|||
public boolean getHasInTransit() { |
|||
return this.getOrderAmount() > this.getInstorageAmount(); |
|||
} |
|||
|
|||
public double getAmountOfInTransit() { |
|||
return this.getOrderAmount() - this.getInstorageAmount(); |
|||
} |
|||
} |
@ -0,0 +1,67 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisitionpro; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 15:39 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "采购订单商品信息", description = "采购订单商品信息") |
|||
@TableName("purchase_requisition_pro") |
|||
public class PurchaseRequisitionPro extends BaseEntity { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty("主表sid") |
|||
private String mainSid; // 主表sid
|
|||
@ApiModelProperty("商品代码") |
|||
private String proCode; // 商品代码
|
|||
@ApiModelProperty("商品名称") |
|||
private String proName; // 商品名称
|
|||
@ApiModelProperty("规格型号") |
|||
private String content; // 规格型号
|
|||
@ApiModelProperty("数量") |
|||
private String number; // 数量
|
|||
@ApiModelProperty("包装数量") |
|||
private String packageNumber; // 包装数量
|
|||
@ApiModelProperty("包内数量") |
|||
private String packageInsideNumber; // 包内数量
|
|||
@ApiModelProperty("包装规格") |
|||
private String packageSpec; // 包装规格
|
|||
@ApiModelProperty("单位") |
|||
private String unit; // 单位
|
|||
@ApiModelProperty("包装价格(元)") |
|||
private String packagePrice; // 包装价格(元)
|
|||
@ApiModelProperty("包合计(元)") |
|||
private String packageTotalPrice; // 包合计(元)
|
|||
@ApiModelProperty("单价(元)") |
|||
private String unitPrice; // 单价(元)
|
|||
@ApiModelProperty("含税单价(元)") |
|||
private String unitPriceTax; // 含税单价(元)
|
|||
@ApiModelProperty("合计(元)") |
|||
private String totalPrice; // 合计(元)
|
|||
@ApiModelProperty("商品品类名称") |
|||
private String category; // 商品品类名称
|
|||
@ApiModelProperty("商品品类编码") |
|||
private String categoryKey; // 商品品类编码
|
|||
@ApiModelProperty("品牌名称") |
|||
private String brand; // 品牌名称
|
|||
@ApiModelProperty("品牌编码") |
|||
private String brandCode; // 品牌编码
|
|||
@ApiModelProperty("发货日期") |
|||
private String issuanceDate; // 发货日期
|
|||
@ApiModelProperty("第二代码") |
|||
private String secondCode;// 第二代码
|
|||
@ApiModelProperty("零售金额") |
|||
private String retailAmount;// 零售金额
|
|||
@ApiModelProperty("含税金额") |
|||
private Double taxInclusiveAmount;// 含税金额
|
|||
@ApiModelProperty("配货金额") |
|||
private Double distributionAmount;// 配货金额
|
|||
@ApiModelProperty("订单编号") |
|||
private String preqCode;// 订单编号
|
|||
} |
@ -0,0 +1,111 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisitionpro; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
|
|||
public class PurchaseRequisitionProCheckVo implements Vo { |
|||
public PurchaseRequisitionProCheckVo() { |
|||
} |
|||
|
|||
public PurchaseRequisitionProCheckVo(int resState) { |
|||
this.resState = resState; |
|||
} |
|||
|
|||
public PurchaseRequisitionProCheckVo(String prSid, String prCode, String productCode, String productName, int resState) { |
|||
this.prSid = prSid; |
|||
this.prCode = prCode; |
|||
this.productCode = productCode; |
|||
this.productName = productName; |
|||
this.resState = resState; |
|||
} |
|||
|
|||
private String prSid; |
|||
private String prCode; |
|||
private String productCode; |
|||
private String productName; |
|||
private String brandName; |
|||
private String brandCode; |
|||
private String categoryName; |
|||
private String categoryCode; |
|||
private int resState = 0; // 0=正常;1=商品不存在;2=品牌不符;3=品类不符
|
|||
private String msg; |
|||
|
|||
public String getPrSid() { |
|||
return prSid; |
|||
} |
|||
|
|||
public void setPrSid(String prSid) { |
|||
this.prSid = prSid; |
|||
} |
|||
|
|||
public String getPrCode() { |
|||
return prCode; |
|||
} |
|||
|
|||
public void setPrCode(String prCode) { |
|||
this.prCode = prCode; |
|||
} |
|||
|
|||
public String getProductCode() { |
|||
return productCode; |
|||
} |
|||
|
|||
public void setProductCode(String productCode) { |
|||
this.productCode = productCode; |
|||
} |
|||
|
|||
public String getProductName() { |
|||
return productName; |
|||
} |
|||
|
|||
public void setProductName(String productName) { |
|||
this.productName = productName; |
|||
} |
|||
|
|||
public String getBrandName() { |
|||
return brandName; |
|||
} |
|||
|
|||
public void setBrandName(String brandName) { |
|||
this.brandName = brandName; |
|||
} |
|||
|
|||
public String getBrandCode() { |
|||
return brandCode; |
|||
} |
|||
|
|||
public void setBrandCode(String brandCode) { |
|||
this.brandCode = brandCode; |
|||
} |
|||
|
|||
public String getCategoryName() { |
|||
return categoryName; |
|||
} |
|||
|
|||
public void setCategoryName(String categoryName) { |
|||
this.categoryName = categoryName; |
|||
} |
|||
|
|||
public String getCategoryCode() { |
|||
return categoryCode; |
|||
} |
|||
|
|||
public void setCategoryCode(String categoryCode) { |
|||
this.categoryCode = categoryCode; |
|||
} |
|||
|
|||
public int getResState() { |
|||
return resState; |
|||
} |
|||
|
|||
public void setResState(int resState) { |
|||
this.resState = resState; |
|||
} |
|||
|
|||
public String getMsg() { |
|||
return msg; |
|||
} |
|||
|
|||
public void setMsg(String msg) { |
|||
this.msg = msg; |
|||
} |
|||
} |
@ -0,0 +1,57 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisitionpro; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 15:40 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "采购订单商品信息 视图数据详情", description = "采购订单商品信息 视图数据详情") |
|||
public class PurchaseRequisitionProDetailsVo implements Vo { |
|||
@ApiModelProperty("主表sid") |
|||
private String mainSid; // 主表sid
|
|||
@ApiModelProperty("商品代码") |
|||
private String proCode; // 商品代码
|
|||
@ApiModelProperty("商品名称") |
|||
private String proName; // 商品名称
|
|||
@ApiModelProperty("规格型号") |
|||
private String content; // 规格型号
|
|||
@ApiModelProperty("数量") |
|||
private String number; // 数量
|
|||
@ApiModelProperty("包装数量") |
|||
private String packageNumber; // 包装数量
|
|||
@ApiModelProperty("包内数量") |
|||
private String packageInsideNumber; // 包内数量
|
|||
@ApiModelProperty("包装规格") |
|||
private String packageSpec; // 包装规格
|
|||
@ApiModelProperty("单位") |
|||
private String unit; // 单位
|
|||
@ApiModelProperty("包装价格(元)") |
|||
private String packagePrice; // 包装价格(元)
|
|||
@ApiModelProperty("包合计(元)") |
|||
private String packageTotalPrice; // 包合计(元)
|
|||
@ApiModelProperty("单价(元)") |
|||
private String unitPrice; // 单价(元)
|
|||
@ApiModelProperty("含税单价(元)") |
|||
private String unitPriceTax; // 含税单价(元)
|
|||
@ApiModelProperty("合计(元)") |
|||
private String totalPrice; // 合计(元)
|
|||
@ApiModelProperty("商品品类名称") |
|||
private String category; // 商品品类名称
|
|||
@ApiModelProperty("商品品类编码") |
|||
private String categoryKey; // 商品品类编码
|
|||
@ApiModelProperty("品牌名称") |
|||
private String brand; // 品牌名称
|
|||
@ApiModelProperty("品牌编码") |
|||
private String brandCode; // 品牌编码
|
|||
@ApiModelProperty("发货日期") |
|||
private String issuanceDate; // 发货日期
|
|||
@ApiModelProperty("第二代码") |
|||
private String secondCode;// 第二代码
|
|||
@ApiModelProperty("零售金额") |
|||
private String retailAmount;// 零售金额
|
|||
} |
@ -0,0 +1,58 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisitionpro; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 15:40 |
|||
*/ |
|||
|
|||
@Data |
|||
@ApiModel(value = "采购订单商品信息 数据传输对象", description = "采购订单商品信息 数据传输对象") |
|||
public class PurchaseRequisitionProDto implements Dto { |
|||
@ApiModelProperty("主表sid") |
|||
private String mainSid; // 主表sid
|
|||
@ApiModelProperty("商品代码") |
|||
private String proCode; // 商品代码
|
|||
@ApiModelProperty("商品名称") |
|||
private String proName; // 商品名称
|
|||
@ApiModelProperty("规格型号") |
|||
private String content; // 规格型号
|
|||
@ApiModelProperty("数量") |
|||
private String number; // 数量
|
|||
@ApiModelProperty("包装数量") |
|||
private String packageNumber; // 包装数量
|
|||
@ApiModelProperty("包内数量") |
|||
private String packageInsideNumber; // 包内数量
|
|||
@ApiModelProperty("包装规格") |
|||
private String packageSpec; // 包装规格
|
|||
@ApiModelProperty("单位") |
|||
private String unit; // 单位
|
|||
@ApiModelProperty("包装价格(元)") |
|||
private String packagePrice; // 包装价格(元)
|
|||
@ApiModelProperty("包合计(元)") |
|||
private String packageTotalPrice; // 包合计(元)
|
|||
@ApiModelProperty("单价(元)") |
|||
private String unitPrice; // 单价(元)
|
|||
@ApiModelProperty("含税单价(元)") |
|||
private String unitPriceTax; // 含税单价(元)
|
|||
@ApiModelProperty("合计(元)") |
|||
private String totalPrice; // 合计(元)
|
|||
@ApiModelProperty("商品品类名称") |
|||
private String category; // 商品品类名称
|
|||
@ApiModelProperty("商品品类编码") |
|||
private String categoryKey; // 商品品类编码
|
|||
@ApiModelProperty("品牌名称") |
|||
private String brand; // 品牌名称
|
|||
@ApiModelProperty("品牌编码") |
|||
private String brandCode; // 品牌编码
|
|||
@ApiModelProperty("发货日期") |
|||
private String issuanceDate; // 发货日期
|
|||
@ApiModelProperty("第二代码") |
|||
private String secondCode;// 第二代码
|
|||
@ApiModelProperty("零售金额") |
|||
private String retailAmount;// 零售金额
|
|||
} |
@ -0,0 +1,42 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisitionpro; |
|||
|
|||
import com.alibaba.excel.annotation.ExcelProperty; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 15:41 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "采购订单商品信息 导出excel视图数据对象", description = "采购订单商品信息 导出excel视图数据对象") |
|||
public class PurchaseRequisitionProExcelVo { |
|||
@ExcelProperty(value = "商品代码") |
|||
@ApiModelProperty("商品代码") |
|||
private String proCode; // 商品代码
|
|||
@ExcelProperty(value = "商品名称") |
|||
@ApiModelProperty("商品名称") |
|||
private String proName; // 商品名称
|
|||
@ExcelProperty(value = "数量") |
|||
@ApiModelProperty("数量") |
|||
private String number; // 数量
|
|||
@ExcelProperty(value = "合计(元)") |
|||
@ApiModelProperty("合计(元)") |
|||
private String totalPrice; // 合计(元)
|
|||
@ExcelProperty(value = "商品品类名称") |
|||
@ApiModelProperty("商品品类名称") |
|||
private String category; // 商品品类名称
|
|||
@ExcelProperty(value = "商品品类编码") |
|||
@ApiModelProperty("商品品类编码") |
|||
private String categoryKey; // 商品品类编码
|
|||
@ExcelProperty(value = "品牌名称") |
|||
@ApiModelProperty("品牌名称") |
|||
private String brand; // 品牌名称
|
|||
@ExcelProperty(value = "品牌编码") |
|||
@ApiModelProperty("品牌编码") |
|||
private String brandCode; // 品牌编码
|
|||
@ExcelProperty(value = "订单时间") |
|||
@ApiModelProperty("订单时间") |
|||
private String createTime; |
|||
} |
@ -0,0 +1,17 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisitionpro; |
|||
|
|||
import io.swagger.annotations.Api; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 15:41 |
|||
*/ |
|||
@Api(tags = "采购订单商品信息") |
|||
@FeignClient( |
|||
contextId = "PurchaseRequisitionPro", |
|||
name = "yxt-supervise", |
|||
path = "v1/purchaserequisitionpro", |
|||
fallback = PurchaseRequisitionProFeignFallback.class) |
|||
public interface PurchaseRequisitionProFeign { |
|||
} |
@ -0,0 +1,11 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisitionpro; |
|||
|
|||
import org.springframework.stereotype.Component; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 15:41 |
|||
*/ |
|||
@Component |
|||
public class PurchaseRequisitionProFeignFallback implements PurchaseRequisitionProFeign{ |
|||
} |
@ -0,0 +1,63 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisitionpro; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 15:41 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "采购订单商品信息 查询条件", description = "采购订单商品信息 查询条件") |
|||
public class PurchaseRequisitionProQuery implements Query { |
|||
@ApiModelProperty("主表sid") |
|||
private String mainSid; // 主表sid
|
|||
@ApiModelProperty("商品代码") |
|||
private String proCode; // 商品代码
|
|||
@ApiModelProperty("商品名称") |
|||
private String proName; // 商品名称
|
|||
@ApiModelProperty("规格型号") |
|||
private String content; // 规格型号
|
|||
@ApiModelProperty("数量") |
|||
private String number; // 数量
|
|||
@ApiModelProperty("包装数量") |
|||
private String packageNumber; // 包装数量
|
|||
@ApiModelProperty("包内数量") |
|||
private String packageInsideNumber; // 包内数量
|
|||
@ApiModelProperty("包装规格") |
|||
private String packageSpec; // 包装规格
|
|||
@ApiModelProperty("单位") |
|||
private String unit; // 单位
|
|||
@ApiModelProperty("包装价格(元)") |
|||
private String packagePrice; // 包装价格(元)
|
|||
@ApiModelProperty("包合计(元)") |
|||
private String packageTotalPrice; // 包合计(元)
|
|||
@ApiModelProperty("单价(元)") |
|||
private String unitPrice; // 单价(元)
|
|||
@ApiModelProperty("含税单价(元)") |
|||
private String unitPriceTax; // 含税单价(元)
|
|||
@ApiModelProperty("合计(元)") |
|||
private String totalPrice; // 合计(元)
|
|||
@ApiModelProperty("商品品类名称") |
|||
private String category; // 商品品类名称
|
|||
@ApiModelProperty("商品品类编码") |
|||
private String categoryKey; // 商品品类编码
|
|||
@ApiModelProperty("品牌名称") |
|||
private String brand; // 品牌名称
|
|||
@ApiModelProperty("品牌编码") |
|||
private String brandCode; // 品牌编码
|
|||
@ApiModelProperty("发货日期") |
|||
private String issuanceDate; // 发货日期
|
|||
@ApiModelProperty("第二代码") |
|||
private String secondCode;// 第二代码
|
|||
@ApiModelProperty("零售金额") |
|||
private String retailAmount;// 零售金额
|
|||
@ApiModelProperty("开始时间") |
|||
private String startDate; |
|||
@ApiModelProperty("结束时间") |
|||
private String endDate; |
|||
@ApiModelProperty("订单编号") |
|||
private String code; |
|||
} |
@ -0,0 +1,59 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisitionpro; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/3/30 15:42 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "采购订单商品信息 视图数据对象", description = "采购订单商品信息 视图数据对象") |
|||
public class PurchaseRequisitionProVo implements Vo { |
|||
@ApiModelProperty("主表sid") |
|||
private String mainSid; // 主表sid
|
|||
@ApiModelProperty("商品代码") |
|||
private String proCode; // 商品代码
|
|||
@ApiModelProperty("商品名称") |
|||
private String proName; // 商品名称
|
|||
@ApiModelProperty("规格型号") |
|||
private String content; // 规格型号
|
|||
@ApiModelProperty("数量") |
|||
private String number; // 数量
|
|||
@ApiModelProperty("包装数量") |
|||
private String packageNumber; // 包装数量
|
|||
@ApiModelProperty("包内数量") |
|||
private String packageInsideNumber; // 包内数量
|
|||
@ApiModelProperty("包装规格") |
|||
private String packageSpec; // 包装规格
|
|||
@ApiModelProperty("单位") |
|||
private String unit; // 单位
|
|||
@ApiModelProperty("包装价格(元)") |
|||
private String packagePrice; // 包装价格(元)
|
|||
@ApiModelProperty("包合计(元)") |
|||
private String packageTotalPrice; // 包合计(元)
|
|||
@ApiModelProperty("单价(元)") |
|||
private String unitPrice; // 单价(元)
|
|||
@ApiModelProperty("含税单价(元)") |
|||
private String unitPriceTax; // 含税单价(元)
|
|||
@ApiModelProperty("合计(元)") |
|||
private String totalPrice; // 合计(元)
|
|||
@ApiModelProperty("商品品类名称") |
|||
private String category; // 商品品类名称
|
|||
@ApiModelProperty("商品品类编码") |
|||
private String categoryKey; // 商品品类编码
|
|||
@ApiModelProperty("品牌名称") |
|||
private String brand; // 品牌名称
|
|||
@ApiModelProperty("品牌编码") |
|||
private String brandCode; // 品牌编码
|
|||
@ApiModelProperty("发货日期") |
|||
private String issuanceDate; // 发货日期
|
|||
@ApiModelProperty("第二代码") |
|||
private String secondCode;// 第二代码
|
|||
@ApiModelProperty("零售金额") |
|||
private String retailAmount;// 零售金额
|
|||
@ApiModelProperty("订单编号") |
|||
private String code; |
|||
} |
@ -0,0 +1,48 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisitionstore; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/3 16:45 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "采购订单商品信息(烟草)", description = "采购订单商品信息(烟草)") |
|||
@TableName("purchase_requisition_store") |
|||
public class PurchaseRequisitionStore extends BaseEntity { |
|||
|
|||
@ApiModelProperty("创建者") |
|||
private String createSid; // 创建者
|
|||
@ApiModelProperty("更新者") |
|||
private String modifySid; // 更新者
|
|||
@ApiModelProperty("创建时间") |
|||
private Date createTime; |
|||
@ApiModelProperty("记录修改时间") |
|||
private Date modifyTime; |
|||
@ApiModelProperty("关联表sid") |
|||
private String mainSid; // 关联表sid
|
|||
@ApiModelProperty("烟草证编码") |
|||
private String yCode; // 烟草证编码
|
|||
@ApiModelProperty("门店代码") |
|||
private String storeCode; // 门店代码
|
|||
@ApiModelProperty("门店名称") |
|||
private String storeName; // 门店名称
|
|||
@ApiModelProperty("金额") |
|||
private String price; // 金额
|
|||
@ApiModelProperty("批次") |
|||
private String pc; // 批次
|
|||
@ApiModelProperty("明细总金额") |
|||
private String detailPrice; // 明细总金额
|
|||
@ApiModelProperty("创建者") |
|||
private String createBySid; |
|||
@ApiModelProperty("更新者") |
|||
private String updateBySid; |
|||
@ApiModelProperty("备注") |
|||
private String remarks; |
|||
} |
@ -0,0 +1,45 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisitionstore; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/3 16:45 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "采购订单商品信息(烟草) 视图数据详情", description = "采购订单商品信息(烟草)视图数据详情") |
|||
public class PurchaseRequisitionStoreDetailsVo implements Vo { |
|||
@ApiModelProperty("创建者") |
|||
private String createSid; // 创建者
|
|||
@ApiModelProperty("更新者") |
|||
private String modifySid; // 更新者
|
|||
@ApiModelProperty("创建时间") |
|||
private Date createTime; |
|||
@ApiModelProperty("记录修改时间") |
|||
private Date modifyTime; |
|||
@ApiModelProperty("关联表sid") |
|||
private String mainSid; // 关联表sid
|
|||
@ApiModelProperty("烟草证编码") |
|||
private String yCode; // 烟草证编码
|
|||
@ApiModelProperty("门店代码") |
|||
private String storeCode; // 门店代码
|
|||
@ApiModelProperty("门店名称") |
|||
private String storeName; // 门店名称
|
|||
@ApiModelProperty("金额") |
|||
private String price; // 金额
|
|||
@ApiModelProperty("批次") |
|||
private String pc; // 批次
|
|||
@ApiModelProperty("明细总金额") |
|||
private String detailPrice; // 明细总金额
|
|||
@ApiModelProperty("创建者") |
|||
private String createBySid; |
|||
@ApiModelProperty("更新者") |
|||
private String updateBySid; |
|||
@ApiModelProperty("备注") |
|||
private String remarks; |
|||
} |
@ -0,0 +1,45 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisitionstore; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/3 16:46 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "采购订单商品信息(烟草) 数据传输对象", description = "采购订单商品信息(烟草) 数据传输对象") |
|||
public class PurchaseRequisitionStoreDto implements Dto { |
|||
@ApiModelProperty("创建者") |
|||
private String createSid; // 创建者
|
|||
@ApiModelProperty("更新者") |
|||
private String modifySid; // 更新者
|
|||
@ApiModelProperty("创建时间") |
|||
private Date createTime; |
|||
@ApiModelProperty("记录修改时间") |
|||
private Date modifyTime; |
|||
@ApiModelProperty("关联表sid") |
|||
private String mainSid; // 关联表sid
|
|||
@ApiModelProperty("烟草证编码") |
|||
private String yCode; // 烟草证编码
|
|||
@ApiModelProperty("门店代码") |
|||
private String storeCode; // 门店代码
|
|||
@ApiModelProperty("门店名称") |
|||
private String storeName; // 门店名称
|
|||
@ApiModelProperty("金额") |
|||
private String price; // 金额
|
|||
@ApiModelProperty("批次") |
|||
private String pc; // 批次
|
|||
@ApiModelProperty("明细总金额") |
|||
private String detailPrice; // 明细总金额
|
|||
@ApiModelProperty("创建者") |
|||
private String createBySid; |
|||
@ApiModelProperty("更新者") |
|||
private String updateBySid; |
|||
@ApiModelProperty("备注") |
|||
private String remarks; |
|||
} |
@ -0,0 +1,34 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisitionstore; |
|||
|
|||
import com.alibaba.excel.annotation.ExcelProperty; |
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/3 16:46 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "采购订单商品信息(烟草) Excel视图数据对象", description = "采购订单商品信息(烟草) Excel视图数据对象") |
|||
public class PurchaseRequisitionStoreExcelVo implements Vo { |
|||
@ExcelProperty(value = "创建时间") |
|||
@ApiModelProperty("创建时间") |
|||
private Date createTime; |
|||
@ExcelProperty(value = "烟草证编码") |
|||
@ApiModelProperty("烟草证编码") |
|||
private String yCode; // 烟草证编码
|
|||
@ExcelProperty(value = "门店代码") |
|||
@ApiModelProperty("门店代码") |
|||
private String storeCode; // 门店代码
|
|||
@ExcelProperty(value = "门店名称") |
|||
@ApiModelProperty("门店名称") |
|||
private String storeName; // 门店名称
|
|||
@ExcelProperty(value = "金额") |
|||
@ApiModelProperty("金额") |
|||
private String price; // 金额
|
|||
|
|||
} |
@ -0,0 +1,17 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisitionstore; |
|||
|
|||
import io.swagger.annotations.Api; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/3 16:46 |
|||
*/ |
|||
@Api(tags = "采购订单商品信息") |
|||
@FeignClient( |
|||
contextId = "yxt-supervise-PurchaseRequisitionStore", |
|||
name = "yxt-supervise", |
|||
path = "v1/purchaserequisitionstore", |
|||
fallback = PurchaseRequisitionStoreFeignFallback.class) |
|||
public interface PurchaseRequisitionStoreFeign { |
|||
} |
@ -0,0 +1,11 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisitionstore; |
|||
|
|||
import org.springframework.stereotype.Component; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/3 16:46 |
|||
*/ |
|||
@Component |
|||
public class PurchaseRequisitionStoreFeignFallback implements PurchaseRequisitionStoreFeign{ |
|||
} |
@ -0,0 +1,49 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisitionstore; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/3 16:46 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "采购订单商品信息(烟草) 查询条件", description = "采购订单商品信息(烟草) 查询条件") |
|||
public class PurchaseRequisitionStoreQuery implements Query { |
|||
@ApiModelProperty("创建者") |
|||
private String createSid; // 创建者
|
|||
@ApiModelProperty("更新者") |
|||
private String modifySid; // 更新者
|
|||
@ApiModelProperty("创建时间") |
|||
private Date createTime; |
|||
@ApiModelProperty("记录修改时间") |
|||
private Date modifyTime; |
|||
@ApiModelProperty("关联表sid") |
|||
private String mainSid; // 关联表sid
|
|||
@ApiModelProperty("烟草证编码") |
|||
private String yCode; // 烟草证编码
|
|||
@ApiModelProperty("门店代码") |
|||
private String storeCode; // 门店代码
|
|||
@ApiModelProperty("门店名称") |
|||
private String storeName; // 门店名称
|
|||
@ApiModelProperty("金额") |
|||
private String price; // 金额
|
|||
@ApiModelProperty("批次") |
|||
private String pc; // 批次
|
|||
@ApiModelProperty("明细总金额") |
|||
private String detailPrice; // 明细总金额
|
|||
@ApiModelProperty("创建者") |
|||
private String createBySid; |
|||
@ApiModelProperty("更新者") |
|||
private String updateBySid; |
|||
@ApiModelProperty("备注") |
|||
private String remarks; |
|||
@ApiModelProperty("开始时间") |
|||
private String startDate; |
|||
@ApiModelProperty("结束时间") |
|||
private String endDate; |
|||
} |
Some files were not shown because too many files changed in this diff
Loading…
Reference in new issue