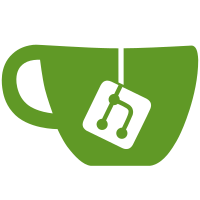
13 changed files with 352 additions and 33 deletions
@ -0,0 +1,111 @@ |
|||
package com.yxt.supervise.customer.api.purchaserequisitionpro; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
|
|||
public class PurchaseRequisitionProCheckVo implements Vo { |
|||
public PurchaseRequisitionProCheckVo() { |
|||
} |
|||
|
|||
public PurchaseRequisitionProCheckVo(int resState) { |
|||
this.resState = resState; |
|||
} |
|||
|
|||
public PurchaseRequisitionProCheckVo(String prSid, String prCode, String productCode, String productName, int resState) { |
|||
this.prSid = prSid; |
|||
this.prCode = prCode; |
|||
this.productCode = productCode; |
|||
this.productName = productName; |
|||
this.resState = resState; |
|||
} |
|||
|
|||
private String prSid; |
|||
private String prCode; |
|||
private String productCode; |
|||
private String productName; |
|||
private String brandName; |
|||
private String brandCode; |
|||
private String categoryName; |
|||
private String categoryCode; |
|||
private int resState = 0; // 0=正常;1=商品不存在;2=品牌不符;3=品类不符
|
|||
private String msg; |
|||
|
|||
public String getPrSid() { |
|||
return prSid; |
|||
} |
|||
|
|||
public void setPrSid(String prSid) { |
|||
this.prSid = prSid; |
|||
} |
|||
|
|||
public String getPrCode() { |
|||
return prCode; |
|||
} |
|||
|
|||
public void setPrCode(String prCode) { |
|||
this.prCode = prCode; |
|||
} |
|||
|
|||
public String getProductCode() { |
|||
return productCode; |
|||
} |
|||
|
|||
public void setProductCode(String productCode) { |
|||
this.productCode = productCode; |
|||
} |
|||
|
|||
public String getProductName() { |
|||
return productName; |
|||
} |
|||
|
|||
public void setProductName(String productName) { |
|||
this.productName = productName; |
|||
} |
|||
|
|||
public String getBrandName() { |
|||
return brandName; |
|||
} |
|||
|
|||
public void setBrandName(String brandName) { |
|||
this.brandName = brandName; |
|||
} |
|||
|
|||
public String getBrandCode() { |
|||
return brandCode; |
|||
} |
|||
|
|||
public void setBrandCode(String brandCode) { |
|||
this.brandCode = brandCode; |
|||
} |
|||
|
|||
public String getCategoryName() { |
|||
return categoryName; |
|||
} |
|||
|
|||
public void setCategoryName(String categoryName) { |
|||
this.categoryName = categoryName; |
|||
} |
|||
|
|||
public String getCategoryCode() { |
|||
return categoryCode; |
|||
} |
|||
|
|||
public void setCategoryCode(String categoryCode) { |
|||
this.categoryCode = categoryCode; |
|||
} |
|||
|
|||
public int getResState() { |
|||
return resState; |
|||
} |
|||
|
|||
public void setResState(int resState) { |
|||
this.resState = resState; |
|||
} |
|||
|
|||
public String getMsg() { |
|||
return msg; |
|||
} |
|||
|
|||
public void setMsg(String msg) { |
|||
this.msg = msg; |
|||
} |
|||
} |
@ -0,0 +1,77 @@ |
|||
package com.yxt.supervise.customer.api.supplierbankinfo; |
|||
|
|||
public class PurchaseRequisitionCheckVo { |
|||
public PurchaseRequisitionCheckVo() { |
|||
} |
|||
|
|||
public PurchaseRequisitionCheckVo(String prSid, String prCode, int resState) { |
|||
this.prSid = prSid; |
|||
this.prCode = prCode; |
|||
this.resState = resState; |
|||
} |
|||
|
|||
private String prSid; |
|||
private String prCode; |
|||
private String supplierCode; |
|||
private String supplierName; |
|||
private String supplierCodeUnified; |
|||
|
|||
private int resState = 0; // 0=正常;1=无供应商信息;2=供应商编码不符;3=有在途
|
|||
private String msg; |
|||
|
|||
public String getPrSid() { |
|||
return prSid; |
|||
} |
|||
|
|||
public void setPrSid(String prSid) { |
|||
this.prSid = prSid; |
|||
} |
|||
|
|||
public String getPrCode() { |
|||
return prCode; |
|||
} |
|||
|
|||
public void setPrCode(String prCode) { |
|||
this.prCode = prCode; |
|||
} |
|||
|
|||
public String getSupplierCode() { |
|||
return supplierCode; |
|||
} |
|||
|
|||
public void setSupplierCode(String supplierCode) { |
|||
this.supplierCode = supplierCode; |
|||
} |
|||
|
|||
public String getSupplierName() { |
|||
return supplierName; |
|||
} |
|||
|
|||
public void setSupplierName(String supplierName) { |
|||
this.supplierName = supplierName; |
|||
} |
|||
|
|||
public String getSupplierCodeUnified() { |
|||
return supplierCodeUnified; |
|||
} |
|||
|
|||
public void setSupplierCodeUnified(String supplierCodeUnified) { |
|||
this.supplierCodeUnified = supplierCodeUnified; |
|||
} |
|||
|
|||
public int getResState() { |
|||
return resState; |
|||
} |
|||
|
|||
public void setResState(int resState) { |
|||
this.resState = resState; |
|||
} |
|||
|
|||
public String getMsg() { |
|||
return msg; |
|||
} |
|||
|
|||
public void setMsg(String msg) { |
|||
this.msg = msg; |
|||
} |
|||
} |
Loading…
Reference in new issue