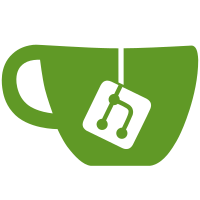
123 changed files with 5560 additions and 10 deletions
@ -0,0 +1,25 @@ |
|||
package com.yxt.supervise.crm.api.bankmanager; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/12 9:40 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "银行联系人信息", description = "银行联系人信息") |
|||
@TableName("bank_manager") |
|||
public class BankManager extends BaseEntity { |
|||
@ApiModelProperty("联系人") |
|||
private String name; |
|||
@ApiModelProperty("电话") |
|||
private String telephone; |
|||
@ApiModelProperty("职务") |
|||
private String post; |
|||
@ApiModelProperty("银行sid") |
|||
private String bankSid; |
|||
} |
@ -0,0 +1,34 @@ |
|||
package com.yxt.supervise.crm.api.bankmanager; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/12 9:40 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "银行联系人信息 视图数据详情", description = "银行联系人信息 视图数据详情") |
|||
public class BankManagerDetailsVo implements Vo { |
|||
private String id; |
|||
private String sid; |
|||
private String lockVersion; |
|||
private String createTime; |
|||
private String modifyTime; |
|||
private String isEnable; |
|||
private String state; |
|||
private String isDeletec; |
|||
private String remarks; |
|||
private String createBySid; |
|||
private String updateBySid; |
|||
@ApiModelProperty("联系人") |
|||
private String name; |
|||
@ApiModelProperty("电话") |
|||
private String telephone; |
|||
@ApiModelProperty("职务") |
|||
private String post; |
|||
@ApiModelProperty("银行sid") |
|||
private String bankSid; |
|||
} |
@ -0,0 +1,34 @@ |
|||
package com.yxt.supervise.crm.api.bankmanager; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/12 9:41 |
|||
*/ |
|||
@ApiModel(value = "银行联系人信息 数据传输对象", description = "银行联系人信息 数据传输对象") |
|||
@Data |
|||
public class BankManagerDto implements Dto { |
|||
private String id; |
|||
private String sid; |
|||
private String lockVersion; |
|||
private String createTime; |
|||
private String modifyTime; |
|||
private String isEnable; |
|||
private String state; |
|||
private String isDeletec; |
|||
private String remarks; |
|||
private String createBySid; |
|||
private String updateBySid; |
|||
@ApiModelProperty("联系人") |
|||
private String name; |
|||
@ApiModelProperty("电话") |
|||
private String telephone; |
|||
@ApiModelProperty("职务") |
|||
private String post; |
|||
@ApiModelProperty("银行sid") |
|||
private String bankSid; |
|||
} |
@ -0,0 +1,18 @@ |
|||
package com.yxt.supervise.crm.api.bankmanager; |
|||
|
|||
import com.yxt.supervise.crm.api.loanbankinformation.LoanBankInformationFeignFallback; |
|||
import io.swagger.annotations.Api; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/12 9:41 |
|||
*/ |
|||
@Api(tags = "银行联系人信息") |
|||
@FeignClient( |
|||
contextId = "supervise-crm-BankManager", |
|||
name = "supervise-crm", |
|||
path = "v1/bankmanager", |
|||
fallback = LoanBankInformationFeignFallback.class) |
|||
public interface BankManagerFeign { |
|||
} |
@ -0,0 +1,11 @@ |
|||
package com.yxt.supervise.crm.api.bankmanager; |
|||
|
|||
import org.springframework.stereotype.Component; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/12 9:41 |
|||
*/ |
|||
@Component |
|||
public class BankManagerFeignFallback implements BankManagerFeign{ |
|||
} |
@ -0,0 +1,34 @@ |
|||
package com.yxt.supervise.crm.api.bankmanager; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/12 9:41 |
|||
*/ |
|||
@ApiModel(value = "银行联系人信息 查询条件", description = "银行联系人信息 查询条件") |
|||
@Data |
|||
public class BankManagerQuery implements Query { |
|||
private String id; |
|||
private String sid; |
|||
private String lockVersion; |
|||
private String createTime; |
|||
private String modifyTime; |
|||
private String isEnable; |
|||
private String state; |
|||
private String isDeletec; |
|||
private String remarks; |
|||
private String createBySid; |
|||
private String updateBySid; |
|||
@ApiModelProperty("联系人") |
|||
private String name; |
|||
@ApiModelProperty("电话") |
|||
private String telephone; |
|||
@ApiModelProperty("职务") |
|||
private String post; |
|||
@ApiModelProperty("银行sid") |
|||
private String bankSid; |
|||
} |
@ -0,0 +1,34 @@ |
|||
package com.yxt.supervise.crm.api.bankmanager; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/12 9:41 |
|||
*/ |
|||
@ApiModel(value = "银行联系人信息 视图数据对象", description = "银行联系人信息 视图数据对象") |
|||
@Data |
|||
public class BankManagerVo implements Vo { |
|||
private String id; |
|||
private String sid; |
|||
private String lockVersion; |
|||
private String createTime; |
|||
private String modifyTime; |
|||
private String isEnable; |
|||
private String state; |
|||
private String isDeletec; |
|||
private String remarks; |
|||
private String createBySid; |
|||
private String updateBySid; |
|||
@ApiModelProperty("联系人") |
|||
private String name; |
|||
@ApiModelProperty("电话") |
|||
private String telephone; |
|||
@ApiModelProperty("职务") |
|||
private String post; |
|||
@ApiModelProperty("银行sid") |
|||
private String bankSid; |
|||
} |
@ -0,0 +1,22 @@ |
|||
package com.yxt.supervise.crm.api.crmcustomertemp; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import lombok.Data; |
|||
|
|||
import java.util.ArrayList; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @Author dimengzhe |
|||
* @Date 2022/11/16 11:56 |
|||
* @Description |
|||
*/ |
|||
@Data |
|||
public class CrmCustomerFileDDto implements Dto { |
|||
private static final long serialVersionUID = -5399631895644837616L; |
|||
|
|||
private String userSid; |
|||
private String customerSid; |
|||
|
|||
private List<String> idImages = new ArrayList<>(); |
|||
} |
@ -0,0 +1,144 @@ |
|||
package com.yxt.supervise.crm.api.crmcustomertemp; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
@ApiModel(value = "潜在客户信息", description = "潜在客户信息") |
|||
@TableName("crm_customer_temp") |
|||
@Data |
|||
public class CrmCustomerTemp extends BaseEntity { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
|
|||
@ApiModelProperty("客户编号(部门编码+客户类型(1位,0个人,1企业)+部门内部流水号(6位))") |
|||
private String customerNo; |
|||
|
|||
@ApiModelProperty("客户类型(自然人/法人)") |
|||
private String customerType; |
|||
|
|||
@ApiModelProperty("客户类型key") |
|||
private String customerTypeKey; |
|||
|
|||
@ApiModelProperty("客户分类(个人:司机/个体老板/其他。企业:企业型客户/一级经销商/二级经销商/终端物流客户)") |
|||
private String customerClass; |
|||
|
|||
@ApiModelProperty("客户分类key") |
|||
private String customerClassKey; |
|||
|
|||
@ApiModelProperty("客户来源(公司资源/自主开发/交接客户/转介绍客户/集团内销)") |
|||
private String source; |
|||
|
|||
@ApiModelProperty("客户来源key") |
|||
private String sourceKey; |
|||
|
|||
@ApiModelProperty("客户级别(意向客户/准客户/成交客户/集团内销/黑名单客户)") |
|||
private String level; |
|||
|
|||
@ApiModelProperty("客户级别key") |
|||
private String levelKey; |
|||
|
|||
@ApiModelProperty("客户名称") |
|||
private String name; |
|||
|
|||
@ApiModelProperty("证件类型key") |
|||
private String certificateTypeKey; |
|||
|
|||
@ApiModelProperty("证件类型(个人为身份证/企业为营业执照)") |
|||
private String certificateType; |
|||
|
|||
@ApiModelProperty("证件号码(个人为身份证号/企业为统一社会信用代码)") |
|||
private String IDNumber; |
|||
|
|||
@ApiModelProperty("证件有效期") |
|||
private String endDate; |
|||
|
|||
@ApiModelProperty("行政区划代码(省)") |
|||
private String address_province; |
|||
|
|||
@ApiModelProperty("行政区划代码(市)") |
|||
private String address_city; |
|||
|
|||
@ApiModelProperty("行政区划代码(县)") |
|||
private String address_county; |
|||
|
|||
@ApiModelProperty("省") |
|||
private String province; |
|||
|
|||
@ApiModelProperty("市") |
|||
private String city; |
|||
|
|||
@ApiModelProperty("县") |
|||
private String county; |
|||
|
|||
@ApiModelProperty("详细地址") |
|||
private String address; |
|||
|
|||
@ApiModelProperty("邮编") |
|||
private String zipCode; |
|||
|
|||
@ApiModelProperty("电子邮箱") |
|||
private String e_mail; |
|||
|
|||
@ApiModelProperty("联系人") |
|||
private String contacts; |
|||
|
|||
@ApiModelProperty("联系电话") |
|||
private String mobile; |
|||
|
|||
@ApiModelProperty("紧急联系电话") |
|||
private String emergencyContact; |
|||
|
|||
@ApiModelProperty("紧急联系电话") |
|||
private String emergencyMobile; |
|||
|
|||
@ApiModelProperty("微信号码") |
|||
private String weixin; |
|||
|
|||
@ApiModelProperty("业务人员sid") |
|||
private String staffSid; |
|||
|
|||
@ApiModelProperty("创建组织sid") |
|||
private String createOrgSid; |
|||
|
|||
@ApiModelProperty("性别") |
|||
private String sex; |
|||
|
|||
@ApiModelProperty("性别key") |
|||
private String sexKey; |
|||
|
|||
@ApiModelProperty("生日") |
|||
private String birthday; |
|||
|
|||
@ApiModelProperty("所在公司名称") |
|||
private String companyName; |
|||
|
|||
@ApiModelProperty("跟进状态key") |
|||
private String follow_state_key; |
|||
|
|||
@ApiModelProperty("跟进状态") |
|||
private String follow_state; |
|||
|
|||
@ApiModelProperty("是否开启提醒key(1开启,0不开启)") |
|||
private String isOnRemindkey; |
|||
|
|||
@ApiModelProperty("是否开启提醒(1开启,0不开启)") |
|||
private String isOnRemind; |
|||
|
|||
@ApiModelProperty("提醒日期") |
|||
private String remind_day; |
|||
|
|||
@ApiModelProperty("提醒备注") |
|||
private String remind_remark; |
|||
|
|||
@ApiModelProperty("客户头像") |
|||
private String customerPhoto; |
|||
|
|||
@ApiModelProperty("见面方式key") |
|||
private String visitWayKey; |
|||
|
|||
@ApiModelProperty("见面方式(到店/电话/拜访)") |
|||
private String visitWay; |
|||
} |
@ -0,0 +1,117 @@ |
|||
package com.yxt.supervise.crm.api.crmcustomertemp; |
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import javax.validation.constraints.NotBlank; |
|||
import java.util.List; |
|||
|
|||
@ApiModel(value = "潜在客户信息 数据传输对象", description = "潜在客户信息 数据传输对象") |
|||
@Data |
|||
public class CrmCustomerTempDto implements Dto { |
|||
|
|||
private static final long serialVersionUID = 793474187074718535L; |
|||
|
|||
@ApiModelProperty(value = "当前登录用户的sid") |
|||
private String userSid; |
|||
|
|||
@ApiModelProperty(value = "客户类型(1个人/2企业)", required = true) |
|||
@NotBlank(message = "客户类型为必选项") |
|||
private String customerType; |
|||
|
|||
@ApiModelProperty(value = "客户类型key", required = true) |
|||
@NotBlank(message = "客户类型为必选项") |
|||
private String customerTypeKey; |
|||
|
|||
@ApiModelProperty(value = "见面方式key", required = true) |
|||
@NotBlank(message = "见面方式为必选项") |
|||
private String visitWayKey; |
|||
|
|||
@ApiModelProperty(value = "见面方式(到店/电话/拜访)", required = true) |
|||
@NotBlank(message = "见面方式为必选项") |
|||
private String visitWay; |
|||
|
|||
@ApiModelProperty(value = "客户名称", required = true) |
|||
@NotBlank(message = "客户名称为必填项") |
|||
private String name; |
|||
|
|||
@ApiModelProperty("联系电话") |
|||
@NotBlank(message = "联系电话为必填项") |
|||
private String mobile; |
|||
|
|||
@ApiModelProperty("微信号码") |
|||
private String weixin; |
|||
|
|||
@ApiModelProperty("公司名称:个人客户时显示") |
|||
private String companyName; |
|||
|
|||
@ApiModelProperty("行政区划代码(省)") |
|||
private String address_province; |
|||
|
|||
@ApiModelProperty("行政区划代码(市)") |
|||
private String address_city; |
|||
@ApiModelProperty("行政区划代码(县)") |
|||
private String address_county; |
|||
@ApiModelProperty("客户地址:省") |
|||
private String province; |
|||
@ApiModelProperty("客户地址:市") |
|||
private String city; |
|||
@ApiModelProperty("客户地址:县") |
|||
private String county; |
|||
@ApiModelProperty("客户地址:详细地址") |
|||
private String address; |
|||
|
|||
@ApiModelProperty("客户级别(意向客户/准客户/成交客户/集团内销/黑名单客户)") |
|||
private String level; |
|||
@ApiModelProperty("客户级别key") |
|||
private String levelKey; |
|||
@ApiModelProperty("是否开启提醒(1开启,0不开启)") |
|||
private String isOnRemindkey; |
|||
@ApiModelProperty("是否开启提醒(1开启,0不开启)") |
|||
private String isOnRemind; |
|||
@ApiModelProperty("提醒日期") |
|||
private String remind_day; |
|||
@ApiModelProperty("提醒备注") |
|||
private String remind_remark; |
|||
//更多信息
|
|||
@ApiModelProperty("客户生日:个人客户时显示") |
|||
private String birthday; |
|||
@ApiModelProperty("性别:个人客户时显示") |
|||
private String sex; |
|||
@ApiModelProperty("性别key:个人客户时") |
|||
private String sexKey; |
|||
@ApiModelProperty("证件类型key") |
|||
private String certificateTypeKey; |
|||
@ApiModelProperty("证件类型(个人为身份证/企业为营业执照)") |
|||
private String certificateType; |
|||
@ApiModelProperty("证件号码(个人为身份证号/企业为统一社会信用代码)") |
|||
private String IDNumber; |
|||
@ApiModelProperty("证件有效期") |
|||
private String endDate; |
|||
@ApiModelProperty("电子邮箱") |
|||
private String e_mail; |
|||
@ApiModelProperty(value = "联系人") |
|||
private String contacts; |
|||
@ApiModelProperty("紧急联系人") |
|||
private String emergencyContact; |
|||
|
|||
@ApiModelProperty("紧急联系电话") |
|||
private String emergencyMobile; |
|||
|
|||
@ApiModelProperty("客户来源(公司资源/自主开发/交接客户/转介绍客户/集团内销)") |
|||
private String source; |
|||
|
|||
@ApiModelProperty("客户来源key") |
|||
private String sourceKey; |
|||
@ApiModelProperty("客户分类(个人:司机/个体老板/其他。企业:企业型客户/一级经销商/二级经销商/终端物流客户)") |
|||
private String customerClass; |
|||
@ApiModelProperty("客户分类key") |
|||
private String customerClassKey; |
|||
@ApiModelProperty(value = "备注") |
|||
private String remarks; |
|||
@ApiModelProperty("业务人员sid") |
|||
private String staffSid; |
|||
|
|||
|
|||
} |
@ -0,0 +1,86 @@ |
|||
package com.yxt.supervise.crm.api.crmcustomertemp; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import javax.validation.Valid; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* Project: supervise-crm(客户管理) <br/> |
|||
* File: CrmCustomerTempFeign.java <br/> |
|||
* Class: com.yxt.supervise.crm.api.crmcustomertemp.CrmCustomerTempFeign <br/> |
|||
* Description: 潜在客户信息. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2022-01-12 11:21:16 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Api(tags = "潜在客户信息") |
|||
@FeignClient( |
|||
contextId = "supervise-crm-CrmCustomerTemp", |
|||
name = "supervise-crm", |
|||
path = "v1/crmcustomertemp", |
|||
fallback = CrmCustomerTempFeignFallback.class) |
|||
public interface CrmCustomerTempFeign { |
|||
|
|||
/** |
|||
* 潜在客户管理的分页查询 |
|||
* |
|||
* @param pq |
|||
* @return |
|||
*/ |
|||
@ApiOperation("根据条件分页查询数据的列表") |
|||
@PostMapping("/listPage") |
|||
public ResultBean<PagerVo<CrmCustomerTempVo>> listPage(@RequestBody PagerQuery<CrmCustomerTempQuery> pq); |
|||
|
|||
/** |
|||
* pc端潜在客户新增保存 |
|||
* |
|||
* @param dto 客户信息及运行信息数据传输对象 |
|||
* @return |
|||
*/ |
|||
@ApiOperation("新增保存") |
|||
@PostMapping("/save") |
|||
public ResultBean save(@Valid @RequestBody CrmCustomerTempDto dto); |
|||
|
|||
/** |
|||
* pc端潜在客户编辑保存 |
|||
* |
|||
* @param dto 数据传输对象 |
|||
* @param sid 潜在客户sid |
|||
* @return |
|||
*/ |
|||
@ApiOperation("修改保存") |
|||
@PostMapping("/update/{sid}") |
|||
public ResultBean update(@RequestBody CrmCustomerTempUpdateDto dto, @PathVariable("sid") String sid); |
|||
|
|||
/** |
|||
* pc潜在客户的批量删除 |
|||
* |
|||
* @param sid |
|||
* @return |
|||
*/ |
|||
@ApiOperation("删除记录") |
|||
@DeleteMapping("/del") |
|||
public ResultBean del(@RequestBody String[] sid); |
|||
|
|||
/** |
|||
* pc潜在客户的编辑回显 |
|||
* |
|||
* @param sid |
|||
* @return |
|||
*/ |
|||
@ApiOperation("获取一条记录") |
|||
@GetMapping("/fetchSid/{sid}") |
|||
public ResultBean<CrmCustomerTempVo> fetchSid(@PathVariable("sid") String sid); |
|||
|
|||
} |
@ -0,0 +1,51 @@ |
|||
package com.yxt.supervise.crm.api.crmcustomertemp; |
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import org.springframework.stereotype.Component; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* Project: supervise-crm(客户管理) <br/> |
|||
* File: CrmCustomerTempFeignFallback.java <br/> |
|||
* Class: com.yxt.supervise.crm.api.crmcustomertemp.CrmCustomerTempFeignFallback <br/> |
|||
* Description: 潜在客户信息. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2022-01-12 11:21:16 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Component |
|||
public class CrmCustomerTempFeignFallback implements CrmCustomerTempFeign { |
|||
|
|||
|
|||
@Override |
|||
public ResultBean<PagerVo<CrmCustomerTempVo>> listPage(PagerQuery<CrmCustomerTempQuery> pq) { |
|||
return null; |
|||
} |
|||
|
|||
|
|||
@Override |
|||
public ResultBean save(CrmCustomerTempDto dto) { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean update(CrmCustomerTempUpdateDto dto, String sid) { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean del(String[] sid) { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<CrmCustomerTempVo> fetchSid(String sid) { |
|||
return null; |
|||
} |
|||
} |
@ -0,0 +1,20 @@ |
|||
package com.yxt.supervise.crm.api.crmcustomertemp; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @Author dimengzhe |
|||
* @Date 2022/9/26 14:30 |
|||
* @Description |
|||
*/ |
|||
@Data |
|||
public class CrmCustomerTempListQuery implements Query { |
|||
private static final long serialVersionUID = -8235453055415557265L; |
|||
|
|||
private String userSid; |
|||
private String staffSid; |
|||
@ApiModelProperty("组织机构全路径sid") |
|||
private String orgPath; |
|||
} |
@ -0,0 +1,22 @@ |
|||
package com.yxt.supervise.crm.api.crmcustomertemp; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @Author dimengzhe |
|||
* @Date 2022/9/26 14:28 |
|||
* @Description |
|||
*/ |
|||
@Data |
|||
public class CrmCustomerTempListVo implements Vo { |
|||
private static final long serialVersionUID = 5338688085476236821L; |
|||
|
|||
private String name; |
|||
private String sid; |
|||
private String mobile; |
|||
@ApiModelProperty("客户编码") |
|||
private String customerNo; |
|||
|
|||
} |
@ -0,0 +1,53 @@ |
|||
package com.yxt.supervise.crm.api.crmcustomertemp; |
|||
|
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
|
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* Project: supervise-crm(客户管理) <br/> |
|||
* File: CrmCustomerTempQuery.java <br/> |
|||
* Class: com.yxt.supervise.crm.api.crmcustomertemp.CrmCustomerTempQuery <br/> |
|||
* Description: 潜在客户信息 查询条件. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2022-01-12 11:21:16 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@ApiModel(value = "潜在客户信息 查询条件", description = "潜在客户信息 查询条件") |
|||
@Data |
|||
public class CrmCustomerTempQuery implements Query { |
|||
|
|||
@ApiModelProperty("客户类型key(自然人/法人)") |
|||
private String customerTypeKey; |
|||
|
|||
@ApiModelProperty("客户级别(意向客户/准客户/成交客户/集团内销/黑名单客户)") |
|||
private String levelKey; |
|||
|
|||
@ApiModelProperty("客户名称") |
|||
private String name; |
|||
|
|||
@ApiModelProperty("联系电话") |
|||
private String mobile; |
|||
|
|||
@ApiModelProperty("提醒开始日期") |
|||
private String remindStartDay; |
|||
|
|||
@ApiModelProperty("提醒结束日期") |
|||
private String remindEndDay; |
|||
|
|||
@ApiModelProperty("业务员sid") |
|||
private String staffSid; |
|||
|
|||
@ApiModelProperty("客户sid") |
|||
private List<String> customerSidList; |
|||
|
|||
} |
@ -0,0 +1,32 @@ |
|||
package com.yxt.supervise.crm.api.crmcustomertemp; |
|||
|
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
|
|||
/** |
|||
* Project: supervise-crm(客户管理) <br/> |
|||
* File: CrmCustomerTempVo.java <br/> |
|||
* Class: com.yxt.supervise.crm.api.crmcustomertemp.CrmCustomerTempVo <br/> |
|||
* Description: 潜在客户信息 视图数据对象. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2022-01-12 11:21:16 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
public class CrmCustomerTempToFin implements Vo { |
|||
|
|||
@ApiModelProperty("潜在客户sid") |
|||
private String sid; |
|||
@ApiModelProperty("客户名称") |
|||
private String name; |
|||
@ApiModelProperty("车架号") |
|||
private String vinNo; |
|||
} |
@ -0,0 +1,109 @@ |
|||
package com.yxt.supervise.crm.api.crmcustomertemp; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import javax.validation.constraints.NotBlank; |
|||
import javax.validation.constraints.Pattern; |
|||
|
|||
/** |
|||
* @Author dimengzhe |
|||
* @Date 2022/7/12 14:04 |
|||
* @Description |
|||
*/ |
|||
@Data |
|||
public class CrmCustomerTempUpdateDto implements Dto { |
|||
private static final long serialVersionUID = -1744083007571476652L; |
|||
|
|||
@ApiModelProperty(value = "当前登录用户的sid") |
|||
private String userSid; |
|||
|
|||
@ApiModelProperty(value = "客户类型(个人/企业)", required = true) |
|||
@NotBlank(message = "客户类型为必选项") |
|||
private String customerType; |
|||
|
|||
@ApiModelProperty(value = "客户类型key", required = true) |
|||
@NotBlank(message = "客户类型为必选项") |
|||
private String customerTypeKey; |
|||
|
|||
@ApiModelProperty(value = "见面方式key", required = true) |
|||
@NotBlank(message = "见面方式为必选项") |
|||
private String visitWayKey; |
|||
|
|||
@ApiModelProperty(value = "见面方式(到店/电话/拜访)", required = true) |
|||
@NotBlank(message = "见面方式为必选项") |
|||
private String visitWay; |
|||
|
|||
@ApiModelProperty(value = "客户名称", required = true) |
|||
@NotBlank(message = "客户名称为必填项") |
|||
private String name; |
|||
|
|||
@ApiModelProperty("联系电话") |
|||
@NotBlank(message = "联系电话为必填项") |
|||
@Pattern(regexp = "^((13[0-9])|(14[5,7])|(15[0-3,5-9])|(17[0,3,5-8])|(18[0-9])|166|198|199|(147))\\d{8}$", message = "手机号码格式不正确") |
|||
private String mobile; |
|||
|
|||
@ApiModelProperty("微信号码") |
|||
private String weixin; |
|||
|
|||
@ApiModelProperty("公司名称:个人客户时显示") |
|||
private String companyName; |
|||
|
|||
@ApiModelProperty("行政区划代码(省)") |
|||
private String address_province; |
|||
|
|||
@ApiModelProperty("行政区划代码(市)") |
|||
private String address_city; |
|||
@ApiModelProperty("行政区划代码(县)") |
|||
private String address_county; |
|||
@ApiModelProperty("客户地址:省") |
|||
private String province; |
|||
@ApiModelProperty("客户地址:市") |
|||
private String city; |
|||
@ApiModelProperty("客户地址:县") |
|||
private String county; |
|||
@ApiModelProperty("客户地址:详细地址") |
|||
private String address; |
|||
|
|||
@ApiModelProperty("客户级别(意向客户/准客户/成交客户/集团内销/黑名单客户)") |
|||
private String level; |
|||
@ApiModelProperty("客户级别key") |
|||
private String levelKey; |
|||
//更多信息
|
|||
@ApiModelProperty("客户生日:个人客户时显示") |
|||
private String birthday; |
|||
@ApiModelProperty("性别:个人客户时显示") |
|||
private String sex; |
|||
@ApiModelProperty("性别key:个人客户时") |
|||
private String sexKey; |
|||
@ApiModelProperty("证件类型key") |
|||
private String certificateTypeKey; |
|||
@ApiModelProperty("证件类型(个人为身份证/企业为营业执照)") |
|||
private String certificateType; |
|||
@ApiModelProperty("证件号码(个人为身份证号/企业为统一社会信用代码)") |
|||
private String IDNumber; |
|||
@ApiModelProperty("证件有效期") |
|||
private String endDate; |
|||
@ApiModelProperty("电子邮箱") |
|||
private String e_mail; |
|||
@ApiModelProperty(value = "联系人") |
|||
private String contacts; |
|||
@ApiModelProperty("紧急联系人") |
|||
private String emergencyContact; |
|||
|
|||
@ApiModelProperty("紧急联系电话") |
|||
private String emergencyMobile; |
|||
|
|||
@ApiModelProperty("客户来源(公司资源/自主开发/交接客户/转介绍客户/集团内销)") |
|||
private String source; |
|||
|
|||
@ApiModelProperty("客户来源key") |
|||
private String sourceKey; |
|||
@ApiModelProperty("客户分类(个人:司机/个体老板/其他。企业:企业型客户/一级经销商/二级经销商/终端物流客户)") |
|||
private String customerClass; |
|||
@ApiModelProperty("客户分类key") |
|||
private String customerClassKey; |
|||
@ApiModelProperty(value = "备注") |
|||
private String remarks; |
|||
} |
@ -0,0 +1,181 @@ |
|||
package com.yxt.supervise.crm.api.crmcustomertemp; |
|||
|
|||
|
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import com.yxt.common.core.vo.Vo; |
|||
|
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* Project: supervise-crm(客户管理) <br/> |
|||
* File: CrmCustomerTempVo.java <br/> |
|||
* Class: com.yxt.supervise.crm.api.crmcustomertemp.CrmCustomerTempVo <br/> |
|||
* Description: 潜在客户信息 视图数据对象. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2022-01-12 11:21:16 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@ApiModel(value = "潜在客户信息 视图数据对象", description = "潜在客户信息 视图数据对象") |
|||
@Data |
|||
public class CrmCustomerTempVo implements Vo { |
|||
|
|||
@ApiModelProperty("潜在客户sid") |
|||
private String sid; |
|||
|
|||
@ApiModelProperty("分公司名称") |
|||
private String createOrgName; |
|||
|
|||
@ApiModelProperty("业务员名称") |
|||
private String staffName; |
|||
|
|||
@ApiModelProperty("部门名称") |
|||
private String deptName; |
|||
|
|||
@ApiModelProperty("创建人sid") |
|||
private String createBySid; |
|||
|
|||
@ApiModelProperty("登记日期") |
|||
@JsonFormat(pattern = "yyyy-MM-dd", timezone = "GMT+8") |
|||
private Date createTime; |
|||
|
|||
@ApiModelProperty("客户编号(部门编码+客户类型(1位,0个人,1企业)+部门内部流水号(6位))") |
|||
private String customerNo; |
|||
|
|||
@ApiModelProperty("客户类型(自然人/法人)") |
|||
private String customerType; |
|||
|
|||
@ApiModelProperty("客户类型key") |
|||
private String customerTypeKey; |
|||
|
|||
@ApiModelProperty("客户分类(个人:司机/个体老板/其他。企业:企业型客户/一级经销商/二级经销商/终端物流客户)") |
|||
private String customerClass; |
|||
|
|||
@ApiModelProperty("客户分类key") |
|||
private String customerClassKey; |
|||
|
|||
@ApiModelProperty("客户来源(公司资源/自主开发/交接客户/转介绍客户/集团内销)") |
|||
private String source; |
|||
|
|||
@ApiModelProperty("客户来源key") |
|||
private String sourceKey; |
|||
|
|||
@ApiModelProperty("客户级别(意向客户/准客户/成交客户/集团内销/黑名单客户)") |
|||
private String level; |
|||
|
|||
@ApiModelProperty("客户级别key") |
|||
private String levelKey; |
|||
|
|||
@ApiModelProperty("客户名称") |
|||
private String name; |
|||
|
|||
@ApiModelProperty("证件类型key") |
|||
private String certificateTypeKey; |
|||
|
|||
@ApiModelProperty("证件类型(个人为身份证/企业为营业执照)") |
|||
private String certificateType; |
|||
|
|||
@ApiModelProperty("证件号码(个人为身份证号/企业为统一社会信用代码)") |
|||
private String IDNumber; |
|||
|
|||
@ApiModelProperty("证件有效期") |
|||
private String endDate; |
|||
|
|||
@ApiModelProperty("行政区划代码(省)") |
|||
private String address_province; |
|||
|
|||
@ApiModelProperty("行政区划代码(市)") |
|||
private String address_city; |
|||
|
|||
@ApiModelProperty("行政区划代码(县)") |
|||
private String address_county; |
|||
|
|||
@ApiModelProperty("省") |
|||
private String province; |
|||
|
|||
@ApiModelProperty("市") |
|||
private String city; |
|||
|
|||
@ApiModelProperty("县") |
|||
private String county; |
|||
|
|||
@ApiModelProperty("客户地址") |
|||
private String address; |
|||
|
|||
@ApiModelProperty("邮编") |
|||
private String zipCode; |
|||
|
|||
@ApiModelProperty("电子邮箱") |
|||
private String e_mail; |
|||
|
|||
@ApiModelProperty("联系人") |
|||
private String contacts; |
|||
|
|||
@ApiModelProperty("联系电话") |
|||
private String mobile; |
|||
|
|||
@ApiModelProperty("紧急联系电话") |
|||
private String emergencyContact; |
|||
|
|||
@ApiModelProperty("紧急联系电话") |
|||
private String emergencyMobile; |
|||
|
|||
@ApiModelProperty("微信号码") |
|||
private String weixin; |
|||
|
|||
@ApiModelProperty("业务人员sid") |
|||
private String staffSid; |
|||
|
|||
@ApiModelProperty("创建组织sid") |
|||
private String createOrgSid; |
|||
|
|||
@ApiModelProperty("性别") |
|||
private String sex; |
|||
|
|||
@ApiModelProperty("性别key") |
|||
private String sexKey; |
|||
|
|||
@ApiModelProperty("客户生日") |
|||
private String birthday; |
|||
|
|||
@ApiModelProperty("所在公司名称") |
|||
private String companyName; |
|||
|
|||
@ApiModelProperty("跟进状态key") |
|||
private String follow_state_key; |
|||
|
|||
@ApiModelProperty("跟进状态") |
|||
private String follow_state; |
|||
|
|||
@ApiModelProperty("是否开启提醒key(1开启,0不开启)") |
|||
private String isOnRemindkey; |
|||
|
|||
@ApiModelProperty("是否开启提醒(1开启,0不开启)") |
|||
private String isOnRemind; |
|||
|
|||
@ApiModelProperty("提醒日期") |
|||
private String remind_day; |
|||
|
|||
@ApiModelProperty("提醒备注") |
|||
private String remind_remark; |
|||
|
|||
@ApiModelProperty("客户头像") |
|||
private String customerPhoto; |
|||
|
|||
@ApiModelProperty("见面方式key") |
|||
private String visitWayKey; |
|||
|
|||
@ApiModelProperty("见面方式(到店/电话/拜访)") |
|||
private String visitWay; |
|||
|
|||
@ApiModelProperty("备注") |
|||
private String remarks; |
|||
} |
@ -0,0 +1,44 @@ |
|||
package com.yxt.supervise.crm.api.enterpriseinformation; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/12 11:43 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "企业信息", description = "企业信息") |
|||
@TableName("enterprise_information") |
|||
public class EnterpriseInformation extends BaseEntity { |
|||
@ApiModelProperty("企业名称") |
|||
private String enterpriseName; |
|||
@ApiModelProperty("企业简称") |
|||
private String enterpriseAbbreviation; |
|||
@ApiModelProperty("银行账户") |
|||
private String bankAccount; |
|||
@ApiModelProperty("账号") |
|||
private String accountNumber; |
|||
@ApiModelProperty("开户行") |
|||
private String openingBankName; |
|||
@ApiModelProperty("法人") |
|||
private String juridicalPerson; |
|||
@ApiModelProperty("营业执照号") |
|||
private String businessLicenseNumber; |
|||
@ApiModelProperty("地址") |
|||
private String address; |
|||
@ApiModelProperty("省") |
|||
private String province; |
|||
@ApiModelProperty("市") |
|||
private String city; |
|||
@ApiModelProperty("区") |
|||
private String county; |
|||
@ApiModelProperty("联系人") |
|||
private String contacts; |
|||
@ApiModelProperty("电话") |
|||
private String telephone; |
|||
|
|||
} |
@ -0,0 +1,43 @@ |
|||
package com.yxt.supervise.crm.api.enterpriseinformation; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/12 11:43 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "企业信息 视图数据详情", description = "企业信息 视图数据详情") |
|||
public class EnterpriseInformationDetailsVo implements Vo { |
|||
private String id; |
|||
private String sid; |
|||
@ApiModelProperty("企业名称") |
|||
private String enterpriseName; |
|||
@ApiModelProperty("企业简称") |
|||
private String enterpriseAbbreviation; |
|||
@ApiModelProperty("银行账户") |
|||
private String bankAccount; |
|||
@ApiModelProperty("账号") |
|||
private String accountNumber; |
|||
@ApiModelProperty("开户行") |
|||
private String openingBankName; |
|||
@ApiModelProperty("法人") |
|||
private String juridicalPerson; |
|||
@ApiModelProperty("营业执照号") |
|||
private String businessLicenseNumber; |
|||
@ApiModelProperty("地址") |
|||
private String address; |
|||
@ApiModelProperty("省") |
|||
private String province; |
|||
@ApiModelProperty("市") |
|||
private String city; |
|||
@ApiModelProperty("区") |
|||
private String county; |
|||
@ApiModelProperty("联系人") |
|||
private String contacts; |
|||
@ApiModelProperty("电话") |
|||
private String telephone; |
|||
} |
@ -0,0 +1,43 @@ |
|||
package com.yxt.supervise.crm.api.enterpriseinformation; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/12 11:43 |
|||
*/ |
|||
@ApiModel(value = "企业信息 数据传输对象", description = "企业信息 数据传输对象") |
|||
@Data |
|||
public class EnterpriseInformationDto implements Dto { |
|||
private String id; |
|||
private String sid; |
|||
@ApiModelProperty("企业名称") |
|||
private String enterpriseName; |
|||
@ApiModelProperty("企业简称") |
|||
private String enterpriseAbbreviation; |
|||
@ApiModelProperty("银行账户") |
|||
private String bankAccount; |
|||
@ApiModelProperty("账号") |
|||
private String accountNumber; |
|||
@ApiModelProperty("开户行") |
|||
private String openingBankName; |
|||
@ApiModelProperty("法人") |
|||
private String juridicalPerson; |
|||
@ApiModelProperty("营业执照号") |
|||
private String businessLicenseNumber; |
|||
@ApiModelProperty("地址") |
|||
private String address; |
|||
@ApiModelProperty("省") |
|||
private String province; |
|||
@ApiModelProperty("市") |
|||
private String city; |
|||
@ApiModelProperty("区") |
|||
private String county; |
|||
@ApiModelProperty("联系人") |
|||
private String contacts; |
|||
@ApiModelProperty("电话") |
|||
private String telephone; |
|||
} |
@ -0,0 +1,18 @@ |
|||
package com.yxt.supervise.crm.api.enterpriseinformation; |
|||
|
|||
import com.yxt.supervise.crm.api.loanbankinformation.LoanBankInformationFeignFallback; |
|||
import io.swagger.annotations.Api; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/12 11:44 |
|||
*/ |
|||
@Api(tags = "企业信息") |
|||
@FeignClient( |
|||
contextId = "supervise-crm-EnterpriseInformation", |
|||
name = "supervise-crm", |
|||
path = "v1/enterpriseinformation", |
|||
fallback = EnterpriseInformationFeignFallback.class) |
|||
public interface EnterpriseInformationFeign { |
|||
} |
@ -0,0 +1,11 @@ |
|||
package com.yxt.supervise.crm.api.enterpriseinformation; |
|||
|
|||
import org.springframework.stereotype.Component; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/12 11:44 |
|||
*/ |
|||
@Component |
|||
public class EnterpriseInformationFeignFallback implements EnterpriseInformationFeign{ |
|||
} |
@ -0,0 +1,43 @@ |
|||
package com.yxt.supervise.crm.api.enterpriseinformation; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/12 11:44 |
|||
*/ |
|||
@ApiModel(value = "企业信息 查询条件", description = "企业信息 查询条件") |
|||
@Data |
|||
public class EnterpriseInformationQuery implements Query { |
|||
private String id; |
|||
private String sid; |
|||
@ApiModelProperty("企业名称") |
|||
private String enterpriseName; |
|||
@ApiModelProperty("企业简称") |
|||
private String enterpriseAbbreviation; |
|||
@ApiModelProperty("银行账户") |
|||
private String bankAccount; |
|||
@ApiModelProperty("账号") |
|||
private String accountNumber; |
|||
@ApiModelProperty("开户行") |
|||
private String openingBankName; |
|||
@ApiModelProperty("法人") |
|||
private String juridicalPerson; |
|||
@ApiModelProperty("营业执照号") |
|||
private String businessLicenseNumber; |
|||
@ApiModelProperty("地址") |
|||
private String address; |
|||
@ApiModelProperty("省") |
|||
private String province; |
|||
@ApiModelProperty("市") |
|||
private String city; |
|||
@ApiModelProperty("区") |
|||
private String county; |
|||
@ApiModelProperty("联系人") |
|||
private String contacts; |
|||
@ApiModelProperty("电话") |
|||
private String telephone; |
|||
} |
@ -0,0 +1,43 @@ |
|||
package com.yxt.supervise.crm.api.enterpriseinformation; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/12 11:44 |
|||
*/ |
|||
@ApiModel(value = "企业信息 视图数据对象", description = "企业信息 视图数据对象") |
|||
@Data |
|||
public class EnterpriseInformationVo implements Vo { |
|||
private String id; |
|||
private String sid; |
|||
@ApiModelProperty("企业名称") |
|||
private String enterpriseName; |
|||
@ApiModelProperty("企业简称") |
|||
private String enterpriseAbbreviation; |
|||
@ApiModelProperty("银行账户") |
|||
private String bankAccount; |
|||
@ApiModelProperty("账号") |
|||
private String accountNumber; |
|||
@ApiModelProperty("开户行") |
|||
private String openingBankName; |
|||
@ApiModelProperty("法人") |
|||
private String juridicalPerson; |
|||
@ApiModelProperty("营业执照号") |
|||
private String businessLicenseNumber; |
|||
@ApiModelProperty("地址") |
|||
private String address; |
|||
@ApiModelProperty("省") |
|||
private String province; |
|||
@ApiModelProperty("市") |
|||
private String city; |
|||
@ApiModelProperty("区") |
|||
private String county; |
|||
@ApiModelProperty("联系人") |
|||
private String contacts; |
|||
@ApiModelProperty("电话") |
|||
private String telephone; |
|||
} |
@ -0,0 +1,18 @@ |
|||
package com.yxt.supervise.crm.api.enterpriseproject; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/7/24 10:45 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "企业项目关联", description = "企业项目关联") |
|||
@TableName("enterprise_project") |
|||
public class EnterpriseProject extends BaseEntity { |
|||
private String projectSid; |
|||
private String enterpriseSid; |
|||
} |
@ -0,0 +1,21 @@ |
|||
package com.yxt.supervise.crm.api.enterpriseproject; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/7/24 10:46 |
|||
*/ |
|||
@ApiModel(value = "企业信息 数据传输对象", description = "企业信息 数据传输对象") |
|||
@Data |
|||
public class EnterpriseProjectDto implements Dto { |
|||
private String id; |
|||
private String sid; |
|||
private String projectSid; |
|||
private String enterpriseSid; |
|||
private List<String> enterpriseSids; |
|||
} |
@ -0,0 +1,16 @@ |
|||
package com.yxt.supervise.crm.api.enterpriseproject; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/7/24 10:47 |
|||
*/ |
|||
@Data |
|||
public class EnterpriseProjectQuery implements Query { |
|||
private String id; |
|||
private String ids; |
|||
private String projectSid; |
|||
private String enterpriseSid; |
|||
} |
@ -0,0 +1,23 @@ |
|||
package com.yxt.supervise.crm.api.enterpriseproject; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/7/24 10:45 |
|||
*/ |
|||
@Data |
|||
|
|||
public class EnterpriseProjectVo implements Vo { |
|||
private String id; |
|||
private String ids; |
|||
private String projectSid; |
|||
private String enterpriseName; |
|||
private String enterpriseSid; |
|||
private String type="担保企业"; |
|||
private String telephone; |
|||
private String contacts; |
|||
|
|||
|
|||
} |
@ -0,0 +1,17 @@ |
|||
package com.yxt.supervise.crm.api.industrydictionary; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/25 9:34 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "所属行业字典", description = "所属行业字典") |
|||
@TableName("industry_dictionary") |
|||
public class IndustryDictionary extends BaseEntity { |
|||
private String industryName; |
|||
} |
@ -0,0 +1,17 @@ |
|||
package com.yxt.supervise.crm.api.industrydictionary; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/25 9:36 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "所属行业字典 视图数据详情", description = "所属行业字典 视图数据详情") |
|||
public class IndustryDictionaryDetailsVo implements Vo { |
|||
private String industryName; |
|||
private String id; |
|||
private String sid; |
|||
} |
@ -0,0 +1,18 @@ |
|||
package com.yxt.supervise.crm.api.industrydictionary; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/25 9:36 |
|||
*/ |
|||
@ApiModel(value = "所属行业字典 数据传输对象", description = "所属行业字典 数据传输对象") |
|||
@Data |
|||
public class IndustryDictionaryDto implements Dto { |
|||
private String industryName; |
|||
private String id; |
|||
private String sid; |
|||
private String remarks; |
|||
} |
@ -0,0 +1,18 @@ |
|||
package com.yxt.supervise.crm.api.industrydictionary; |
|||
|
|||
import com.yxt.supervise.crm.api.warehouselocation.WarehouseLocationFeignFallback; |
|||
import io.swagger.annotations.Api; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/25 9:36 |
|||
*/ |
|||
@Api(tags = "所属行业字典") |
|||
@FeignClient( |
|||
contextId = "supervise-crm-industrydictionary", |
|||
name = "supervise-crm", |
|||
path = "v1/industrydictionary", |
|||
fallback = IndustryDictionaryFeignFallback.class) |
|||
public interface IndustryDictionaryFeign { |
|||
} |
@ -0,0 +1,11 @@ |
|||
package com.yxt.supervise.crm.api.industrydictionary; |
|||
|
|||
import org.springframework.stereotype.Component; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/25 9:37 |
|||
*/ |
|||
@Component |
|||
public class IndustryDictionaryFeignFallback { |
|||
} |
@ -0,0 +1,17 @@ |
|||
package com.yxt.supervise.crm.api.industrydictionary; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/25 9:37 |
|||
*/ |
|||
@ApiModel(value = "所属行业字典 查询条件", description = "所属行业字典 查询条件") |
|||
@Data |
|||
public class IndustryDictionaryQuery implements Query { |
|||
private String industryName; |
|||
private String id; |
|||
private String sid; |
|||
} |
@ -0,0 +1,18 @@ |
|||
package com.yxt.supervise.crm.api.industrydictionary; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/25 9:37 |
|||
*/ |
|||
@ApiModel(value = "所属行业字典 视图数据对象", description = "所属行业字典 视图数据对象") |
|||
@Data |
|||
public class IndustryDictionaryVo implements Vo { |
|||
private String industryName; |
|||
private String id; |
|||
private String sid; |
|||
private String remarks; |
|||
} |
@ -0,0 +1,35 @@ |
|||
package com.yxt.supervise.crm.api.loanbankinformation; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/11 16:04 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "贷款银行信息", description = "贷款银行信息") |
|||
@TableName("loan_bank_information") |
|||
public class LoanBankInformation extends BaseEntity { |
|||
@ApiModelProperty("银行名称") |
|||
private String bankName; |
|||
@ApiModelProperty("银行简称") |
|||
private String bankAbbreviation; |
|||
@ApiModelProperty("地址") |
|||
private String address; |
|||
@ApiModelProperty("省") |
|||
private String province; |
|||
@ApiModelProperty("市") |
|||
private String city; |
|||
@ApiModelProperty("区") |
|||
private String county; |
|||
@ApiModelProperty("联系人") |
|||
private String contacts; |
|||
@ApiModelProperty("电话") |
|||
private String telephone; |
|||
@ApiModelProperty("上级sid") |
|||
private String pSid; |
|||
} |
@ -0,0 +1,37 @@ |
|||
package com.yxt.supervise.crm.api.loanbankinformation; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/11 16:07 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "贷款银行信息 视图数据详情", description = "贷款银行信息 视图数据详情") |
|||
public class LoanBankInformationDetailsVo implements Vo { |
|||
@ApiModelProperty("id") |
|||
private Integer id; |
|||
@ApiModelProperty("sid") |
|||
private String sid; |
|||
@ApiModelProperty("银行名称") |
|||
private String bankName; |
|||
@ApiModelProperty("银行简称") |
|||
private String bankAbbreviation; |
|||
@ApiModelProperty("地址") |
|||
private String address; |
|||
@ApiModelProperty("省") |
|||
private String province; |
|||
@ApiModelProperty("市") |
|||
private String city; |
|||
@ApiModelProperty("区") |
|||
private String county; |
|||
@ApiModelProperty("联系人") |
|||
private String contacts; |
|||
@ApiModelProperty("电话") |
|||
private String telephone; |
|||
@ApiModelProperty("上级sid") |
|||
private String pSid; |
|||
} |
@ -0,0 +1,37 @@ |
|||
package com.yxt.supervise.crm.api.loanbankinformation; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/11 16:07 |
|||
*/ |
|||
@ApiModel(value = "贷款银行信息 数据传输对象", description = "贷款银行信息 数据传输对象") |
|||
@Data |
|||
public class LoanBankInformationDto implements Dto { |
|||
@ApiModelProperty("id") |
|||
private String id; |
|||
@ApiModelProperty("sid") |
|||
private String sid; |
|||
@ApiModelProperty("银行名称") |
|||
private String bankName; |
|||
@ApiModelProperty("银行简称") |
|||
private String bankAbbreviation; |
|||
@ApiModelProperty("地址") |
|||
private String address; |
|||
@ApiModelProperty("省") |
|||
private String province; |
|||
@ApiModelProperty("市") |
|||
private String city; |
|||
@ApiModelProperty("区") |
|||
private String county; |
|||
@ApiModelProperty("联系人") |
|||
private String contacts; |
|||
@ApiModelProperty("电话") |
|||
private String telephone; |
|||
@ApiModelProperty("上级sid") |
|||
private String pSid; |
|||
} |
@ -0,0 +1,29 @@ |
|||
package com.yxt.supervise.crm.api.loanbankinformation; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import com.yxt.supervise.crm.api.loanbankinformation.LoanBankInformationFeignFallback; |
|||
import com.yxt.supervise.crm.api.loanbankinformation.LoanBankInformationQuery; |
|||
import com.yxt.supervise.crm.api.loanbankinformation.LoanBankInformationVo; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
import org.springframework.web.bind.annotation.PostMapping; |
|||
import org.springframework.web.bind.annotation.RequestBody; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/11 16:08 |
|||
*/ |
|||
@Api(tags = "贷款银行信息") |
|||
@FeignClient( |
|||
contextId = "supervise-crm-LoanBankInformation", |
|||
name = "supervise-crm", |
|||
path = "v1/loanbankinformation", |
|||
fallback = LoanBankInformationFeignFallback.class) |
|||
public interface LoanBankInformationFeign { |
|||
@ApiOperation("根据条件分页查询数据的列表") |
|||
@PostMapping("/listPage") |
|||
public ResultBean<PagerVo<LoanBankInformationVo>> listPage(@RequestBody PagerQuery<LoanBankInformationQuery> pq); |
|||
} |
@ -0,0 +1,20 @@ |
|||
package com.yxt.supervise.crm.api.loanbankinformation; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import com.yxt.supervise.crm.api.loanbankinformation.LoanBankInformationQuery; |
|||
import com.yxt.supervise.crm.api.loanbankinformation.LoanBankInformationVo; |
|||
import org.springframework.stereotype.Component; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/11 16:08 |
|||
*/ |
|||
@Component |
|||
public class LoanBankInformationFeignFallback implements LoanBankInformationFeign{ |
|||
@Override |
|||
public ResultBean<PagerVo<LoanBankInformationVo>> listPage(PagerQuery<LoanBankInformationQuery> pq) { |
|||
return null; |
|||
} |
|||
} |
@ -0,0 +1,37 @@ |
|||
package com.yxt.supervise.crm.api.loanbankinformation; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/11 16:08 |
|||
*/ |
|||
@ApiModel(value = "贷款银行信息 查询条件", description = "贷款银行信息 查询条件") |
|||
@Data |
|||
public class LoanBankInformationQuery implements Query { |
|||
@ApiModelProperty("id") |
|||
private Integer id; |
|||
@ApiModelProperty("sid") |
|||
private String sid; |
|||
@ApiModelProperty("银行名称") |
|||
private String bankName; |
|||
@ApiModelProperty("银行简称") |
|||
private String bankAbbreviation; |
|||
@ApiModelProperty("地址") |
|||
private String address; |
|||
@ApiModelProperty("省") |
|||
private String province; |
|||
@ApiModelProperty("市") |
|||
private String city; |
|||
@ApiModelProperty("区") |
|||
private String county; |
|||
@ApiModelProperty("联系人") |
|||
private String contacts; |
|||
@ApiModelProperty("电话") |
|||
private String telephone; |
|||
@ApiModelProperty("上级sid") |
|||
private String pSid; |
|||
} |
@ -0,0 +1,44 @@ |
|||
package com.yxt.supervise.crm.api.loanbankinformation; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import com.yxt.supervise.crm.api.bankmanager.BankManager; |
|||
import com.yxt.supervise.crm.api.bankmanager.BankManagerVo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/11 16:08 |
|||
*/ |
|||
@ApiModel(value = "贷款银行信息 视图数据对象", description = "贷款银行信息 视图数据对象") |
|||
@Data |
|||
public class LoanBankInformationVo implements Vo { |
|||
@ApiModelProperty("id") |
|||
private Integer id; |
|||
@ApiModelProperty("sid") |
|||
private String sid; |
|||
@ApiModelProperty("银行名称") |
|||
private String bankName; |
|||
@ApiModelProperty("银行简称") |
|||
private String bankAbbreviation; |
|||
@ApiModelProperty("地址") |
|||
private String address; |
|||
@ApiModelProperty("省") |
|||
private String province; |
|||
@ApiModelProperty("市") |
|||
private String city; |
|||
@ApiModelProperty("区") |
|||
private String county; |
|||
@ApiModelProperty("联系人") |
|||
private String contacts; |
|||
@ApiModelProperty("电话") |
|||
private String telephone; |
|||
@ApiModelProperty("上级sid") |
|||
private String pSid; |
|||
//下级银行
|
|||
private List<LoanBankInformationVo> pBank; |
|||
private List<BankManagerVo> managerList; |
|||
} |
@ -0,0 +1,26 @@ |
|||
package com.yxt.supervise.crm.api.projectinformation; |
|||
|
|||
import com.yxt.common.core.result.ResultBean; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
import org.springframework.web.bind.annotation.PostMapping; |
|||
import org.springframework.web.bind.annotation.RequestParam; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/25 9:28 |
|||
*/ |
|||
@Api(tags = "项目信息") |
|||
@FeignClient( |
|||
contextId = "supervise-crm-projectinformation", |
|||
name = "supervise-crm", |
|||
path = "v1/projectinformation", |
|||
fallback = ProjectInformationFeignFallback.class) |
|||
public interface ProjectInformationFeign { |
|||
@ApiOperation("根据userSid查询该人的项目") |
|||
@PostMapping("/getProjectSidByUserSid") |
|||
ResultBean<List<ProjectInformationVo>> getProjectSidByUserSid(@RequestParam("userSid") String userSid); |
|||
} |
@ -0,0 +1,11 @@ |
|||
package com.yxt.supervise.crm.api.projectinformation; |
|||
|
|||
import org.springframework.stereotype.Component; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/25 9:29 |
|||
*/ |
|||
@Component |
|||
public class ProjectInformationFeignFallback { |
|||
} |
@ -0,0 +1,61 @@ |
|||
package com.yxt.supervise.crm.api.projectinformation; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import com.yxt.supervise.crm.api.enterpriseproject.EnterpriseProjectVo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/25 9:29 |
|||
*/ |
|||
@ApiModel(value = "项目信息 视图数据对象", description = "项目信息 视图数据对象") |
|||
@Data |
|||
public class ProjectInformationVo implements Vo { |
|||
private String id ; |
|||
private String sid; |
|||
//项目名称
|
|||
private String entryName; |
|||
//所属行业
|
|||
private String industryName; |
|||
//项目类型
|
|||
private String typeName; |
|||
//授信额度
|
|||
private String creditLimit; |
|||
//贷款行
|
|||
private String bankName; |
|||
//客户经理
|
|||
private String bManagerName; |
|||
//签约日期
|
|||
private String signingDate; |
|||
//结束日期
|
|||
private String endDate; |
|||
//企业名称
|
|||
private String enterpriseName; |
|||
//联系人
|
|||
private String eContacts; |
|||
//监管负责人
|
|||
private String regulatoryLeader; |
|||
|
|||
private String generalManager; |
|||
private String fillInDate; |
|||
private String bankSid; |
|||
private String managerSid; |
|||
private String enterpriseSid; |
|||
private String industrySid; |
|||
private String typeSid; |
|||
private String remarks; |
|||
private String engaDate; |
|||
private String url; |
|||
private List<EnterpriseProjectVo> enterpriseProjectVoList; |
|||
private String useLimit; |
|||
private String loanDate; |
|||
private String useDate; |
|||
private String bankPledgeRate; |
|||
//状态名称
|
|||
private String stateName; |
|||
//状态说明
|
|||
private String stateRemarks; |
|||
} |
@ -0,0 +1,19 @@ |
|||
package com.yxt.supervise.crm.api.projecttemplate; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/9/27 15:43 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "模版消息发送的员工", description = "模版消息发送的员工") |
|||
@TableName("project_template") |
|||
public class ProjectTemplate { |
|||
private String id; |
|||
private String sid; |
|||
private String userProjectSid;//用户项目关联sid
|
|||
private String templateSid;//微信模版消息sid
|
|||
} |
@ -0,0 +1,18 @@ |
|||
package com.yxt.supervise.crm.api.projecttemplate; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import lombok.Data; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/9/27 15:45 |
|||
*/ |
|||
@Data |
|||
public class ProjectTemplateDto implements Dto { |
|||
private String id; |
|||
private String sid; |
|||
private String userProjectSid;//用户项目关联sid
|
|||
private List<String> templateSid;//微信模版消息sid
|
|||
} |
@ -0,0 +1,16 @@ |
|||
package com.yxt.supervise.crm.api.projecttemplate; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/9/27 15:46 |
|||
*/ |
|||
@Data |
|||
public class ProjectTemplateQuery implements Query { |
|||
private String id; |
|||
private String ids; |
|||
private String userProjectSid;//用户项目关联sid
|
|||
private String templateSid;//微信模版消息sid
|
|||
} |
@ -0,0 +1,17 @@ |
|||
package com.yxt.supervise.crm.api.projecttemplate; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/9/27 15:46 |
|||
*/ |
|||
@Data |
|||
public class ProjectTemplateVo implements Vo { |
|||
private String id; |
|||
private String ids; |
|||
private String userProjectSid;//用户项目关联sid
|
|||
private String templateSid;//微信模版消息sid
|
|||
private String userSid; |
|||
} |
@ -0,0 +1,17 @@ |
|||
package com.yxt.supervise.crm.api.projecttypedictionary; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/25 9:27 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "项目类型字典", description = "项目类型字典") |
|||
@TableName("project_type_dictionary") |
|||
public class ProjectTypeDictionary extends BaseEntity { |
|||
private String projectType; |
|||
} |
@ -0,0 +1,17 @@ |
|||
package com.yxt.supervise.crm.api.projecttypedictionary; |
|||
|
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/25 9:28 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "项目类型字典 视图数据详情", description = "项目类型字典 视图数据详情") |
|||
public class ProjectTypeDictionaryDetailsVo { |
|||
private String typeName; |
|||
private String id; |
|||
private String sid; |
|||
|
|||
} |
@ -0,0 +1,19 @@ |
|||
package com.yxt.supervise.crm.api.projecttypedictionary; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/25 9:28 |
|||
*/ |
|||
@ApiModel(value = "项目类型字典 数据传输对象", description = "项目类型字典 数据传输对象") |
|||
@Data |
|||
public class ProjectTypeDictionaryDto implements Dto { |
|||
private String typeName; |
|||
private String id; |
|||
private String sid; |
|||
private String remarks; |
|||
private String projectType; |
|||
} |
@ -0,0 +1,17 @@ |
|||
package com.yxt.supervise.crm.api.projecttypedictionary; |
|||
|
|||
import io.swagger.annotations.Api; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/25 9:28 |
|||
*/ |
|||
@Api(tags = "项目类型字典") |
|||
@FeignClient( |
|||
contextId = "supervise-crm-projecttypedictionary", |
|||
name = "supervise-crm", |
|||
path = "v1/projecttypedictionary", |
|||
fallback = ProjectTypeDictionaryFeignFallback.class) |
|||
public interface ProjectTypeDictionaryFeign { |
|||
} |
@ -0,0 +1,11 @@ |
|||
package com.yxt.supervise.crm.api.projecttypedictionary; |
|||
|
|||
import org.springframework.stereotype.Component; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/25 9:29 |
|||
*/ |
|||
@Component |
|||
public class ProjectTypeDictionaryFeignFallback { |
|||
} |
@ -0,0 +1,18 @@ |
|||
package com.yxt.supervise.crm.api.projecttypedictionary; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/25 9:29 |
|||
*/ |
|||
@ApiModel(value = "项目类型字典 查询条件", description = "项目类型字典 查询条件") |
|||
@Data |
|||
public class ProjectTypeDictionaryQuery implements Query { |
|||
private String typeName; |
|||
private String id; |
|||
private String sid; |
|||
|
|||
} |
@ -0,0 +1,19 @@ |
|||
package com.yxt.supervise.crm.api.projecttypedictionary; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/25 9:29 |
|||
*/ |
|||
@ApiModel(value = "项目类型字典 视图数据对象", description = "项目类型字典 视图数据对象") |
|||
@Data |
|||
public class ProjectTypeDictionaryVo implements Vo { |
|||
private String typeName; |
|||
private String id; |
|||
private String sid; |
|||
private String remarks; |
|||
private String projectType; |
|||
} |
@ -0,0 +1,18 @@ |
|||
package com.yxt.supervise.crm.api.storehouseproject; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/7/19 15:37 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "仓库 项目关联表", description = "仓库项目关联表") |
|||
@TableName("storehouse_project") |
|||
public class StoreHouseProject extends BaseEntity { |
|||
private String shSid; |
|||
private String projectSid; |
|||
} |
@ -0,0 +1,19 @@ |
|||
package com.yxt.supervise.crm.api.storehouseproject; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import lombok.Data; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/7/19 15:37 |
|||
*/ |
|||
@Data |
|||
public class StoreHouseProjectDto implements Dto { |
|||
private String id; |
|||
private String sid; |
|||
private String shSid;//仓库sid
|
|||
private String projectSid;//项目sid
|
|||
private List<String> shSids; |
|||
} |
@ -0,0 +1,16 @@ |
|||
package com.yxt.supervise.crm.api.storehouseproject; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/7/19 15:37 |
|||
*/ |
|||
@Data |
|||
public class StoreHouseProjectQuery implements Query { |
|||
private String id; |
|||
private String sid; |
|||
private String shSid; |
|||
private String projectSid; |
|||
} |
@ -0,0 +1,23 @@ |
|||
package com.yxt.supervise.crm.api.storehouseproject; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/7/19 15:37 |
|||
*/ |
|||
@Data |
|||
public class StoreHouseProjectVo implements Vo { |
|||
private String id; |
|||
private String sid; |
|||
private String shSid; |
|||
private String projectSid; |
|||
//仓库名称
|
|||
private String name; |
|||
private String address; |
|||
private String linkerName; // 联系人姓名
|
|||
private String linkerPhone; // 联系人电话
|
|||
private String picUrl; // 图片访问url
|
|||
} |
@ -0,0 +1,18 @@ |
|||
package com.yxt.supervise.crm.api.templatemessage; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/9/27 15:43 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "模版消息", description = "模版消息") |
|||
@TableName("template_message") |
|||
public class TemplateMessage extends BaseEntity { |
|||
private String typeSid; |
|||
private String name;//
|
|||
} |
@ -0,0 +1,18 @@ |
|||
package com.yxt.supervise.crm.api.templatemessage; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import lombok.Data; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/9/27 15:45 |
|||
*/ |
|||
@Data |
|||
public class TemplateMessageDto implements Dto { |
|||
private String id; |
|||
private String sid; |
|||
private String typeSid; |
|||
private String name;//
|
|||
} |
@ -0,0 +1,14 @@ |
|||
package com.yxt.supervise.crm.api.templatemessage; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/9/27 15:46 |
|||
*/ |
|||
@Data |
|||
public class TemplateMessageQuery implements Query { |
|||
private String typeSid; |
|||
private String name;//
|
|||
} |
@ -0,0 +1,18 @@ |
|||
package com.yxt.supervise.crm.api.templatemessage; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/9/27 15:46 |
|||
*/ |
|||
@Data |
|||
public class TemplateMessageVo implements Vo { |
|||
private String id; |
|||
private String sid; |
|||
private String typeSid; |
|||
private String name;//
|
|||
//1 未选中 2选中
|
|||
private String state; |
|||
} |
@ -0,0 +1,17 @@ |
|||
package com.yxt.supervise.crm.api.templatemessagetype; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/9/27 15:43 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "模版消息类型", description = "模版消息类型") |
|||
@TableName("template_message_message") |
|||
public class TemplateMessageType extends BaseEntity { |
|||
private String name;//
|
|||
} |
@ -0,0 +1,16 @@ |
|||
package com.yxt.supervise.crm.api.templatemessagetype; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/9/27 15:45 |
|||
*/ |
|||
@Data |
|||
public class TemplateMessageTypeDto implements Dto { |
|||
private String id; |
|||
private String sid; |
|||
private String typeSid; |
|||
private String name;//
|
|||
} |
@ -0,0 +1,14 @@ |
|||
package com.yxt.supervise.crm.api.templatemessagetype; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/9/27 15:46 |
|||
*/ |
|||
@Data |
|||
public class TemplateMessageTypeQuery implements Query { |
|||
private String typeSid; |
|||
private String name;//
|
|||
} |
@ -0,0 +1,22 @@ |
|||
package com.yxt.supervise.crm.api.templatemessagetype; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import com.yxt.supervise.crm.api.templatemessage.TemplateMessageVo; |
|||
import lombok.Data; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/9/27 15:46 |
|||
*/ |
|||
@Data |
|||
public class TemplateMessageTypeVo implements Vo { |
|||
private String id; |
|||
private String sid; |
|||
private String name;//
|
|||
private List<TemplateMessageVo> list; |
|||
//1 未选中 2选中
|
|||
|
|||
private String state; |
|||
} |
@ -0,0 +1,20 @@ |
|||
package com.yxt.supervise.crm.api.userproject; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.EntityWithId; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/8/6 11:34 |
|||
*/ |
|||
@Data |
|||
@TableName("user_project") |
|||
public class UserProject extends EntityWithId { |
|||
|
|||
private String sid; |
|||
private String projectSid; |
|||
private String userSid; |
|||
private String userType; |
|||
|
|||
} |
@ -0,0 +1,22 @@ |
|||
package com.yxt.supervise.crm.api.userproject; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import lombok.Data; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/8/6 11:34 |
|||
*/ |
|||
@Data |
|||
public class UserProjectDto implements Dto { |
|||
private String id; |
|||
private String sid; |
|||
private String projectSid; |
|||
private String userSid; |
|||
private String userType; |
|||
private List<String> bankUsers; |
|||
private List<String> users; |
|||
|
|||
} |
@ -0,0 +1,46 @@ |
|||
package com.yxt.supervise.crm.api.userproject; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/8/6 11:35 |
|||
*/ |
|||
public class UserProjectQuery implements Query { |
|||
private String id; |
|||
private String sid; |
|||
private String projectSid; |
|||
private String userSid; |
|||
|
|||
public String getId() { |
|||
return id; |
|||
} |
|||
|
|||
public void setId(String id) { |
|||
this.id = id; |
|||
} |
|||
|
|||
public String getSid() { |
|||
return sid; |
|||
} |
|||
|
|||
public void setSid(String sid) { |
|||
this.sid = sid; |
|||
} |
|||
|
|||
public String getProjectSid() { |
|||
return projectSid; |
|||
} |
|||
|
|||
public void setProjectSid(String projectSid) { |
|||
this.projectSid = projectSid; |
|||
} |
|||
|
|||
public String getUserSid() { |
|||
return userSid; |
|||
} |
|||
|
|||
public void setUserSid(String userSid) { |
|||
this.userSid = userSid; |
|||
} |
|||
} |
@ -0,0 +1,74 @@ |
|||
package com.yxt.supervise.crm.api.userproject; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/8/6 11:35 |
|||
*/ |
|||
public class UserProjectVo implements Query { |
|||
private String id; |
|||
private String sid; |
|||
private String projectSid; |
|||
private String userSid; |
|||
private String name; |
|||
private String orgName; |
|||
//是否绑定openid;1 未绑定 2绑定
|
|||
private String isOpenId; |
|||
|
|||
public String getIsOpenId() { |
|||
return isOpenId; |
|||
} |
|||
|
|||
public void setIsOpenId(String isOpenId) { |
|||
this.isOpenId = isOpenId; |
|||
} |
|||
|
|||
public String getName() { |
|||
return name; |
|||
} |
|||
|
|||
public void setName(String name) { |
|||
this.name = name; |
|||
} |
|||
|
|||
public String getOrgName() { |
|||
return orgName; |
|||
} |
|||
|
|||
public void setOrgName(String orgName) { |
|||
this.orgName = orgName; |
|||
} |
|||
|
|||
public String getId() { |
|||
return id; |
|||
} |
|||
|
|||
public void setId(String id) { |
|||
this.id = id; |
|||
} |
|||
|
|||
public String getSid() { |
|||
return sid; |
|||
} |
|||
|
|||
public void setSid(String sid) { |
|||
this.sid = sid; |
|||
} |
|||
|
|||
public String getProjectSid() { |
|||
return projectSid; |
|||
} |
|||
|
|||
public void setProjectSid(String projectSid) { |
|||
this.projectSid = projectSid; |
|||
} |
|||
|
|||
public String getUserSid() { |
|||
return userSid; |
|||
} |
|||
|
|||
public void setUserSid(String userSid) { |
|||
this.userSid = userSid; |
|||
} |
|||
} |
@ -0,0 +1,39 @@ |
|||
package com.yxt.supervise.crm.api.warehouselocation; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/12 16:48 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "仓库位置信息", description = "仓库位置信息") |
|||
@TableName("warehouse_location") |
|||
public class WarehouseLocation extends BaseEntity { |
|||
@ApiModelProperty("位置") |
|||
private String address; |
|||
@ApiModelProperty("省") |
|||
private String province; |
|||
@ApiModelProperty("市") |
|||
private String city; |
|||
@ApiModelProperty("区") |
|||
private String county; |
|||
@ApiModelProperty("面积") |
|||
private String squareMeasure; |
|||
@ApiModelProperty("层高") |
|||
private String floorHeight; |
|||
@ApiModelProperty("性质") |
|||
private String properties; |
|||
@ApiModelProperty("联系人") |
|||
private String contacts; |
|||
@ApiModelProperty("电话") |
|||
private String telephone; |
|||
@ApiModelProperty("价格") |
|||
private String price; |
|||
@ApiModelProperty("房源") |
|||
private String housingResources; |
|||
} |
@ -0,0 +1,39 @@ |
|||
package com.yxt.supervise.crm.api.warehouselocation; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/12 16:48 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "仓库位置信息 视图数据详情", description = "仓库位置信息 视图数据详情") |
|||
public class WarehouseLocationDetailsVo implements Vo { |
|||
private String sid; |
|||
private String id; |
|||
@ApiModelProperty("位置") |
|||
private String address; |
|||
@ApiModelProperty("省") |
|||
private String province; |
|||
@ApiModelProperty("市") |
|||
private String city; |
|||
@ApiModelProperty("区") |
|||
private String county; |
|||
@ApiModelProperty("面积") |
|||
private String squareMeasure; |
|||
@ApiModelProperty("层高") |
|||
private String floorHeight; |
|||
@ApiModelProperty("性质") |
|||
private String properties; |
|||
@ApiModelProperty("联系人") |
|||
private String contacts; |
|||
@ApiModelProperty("电话") |
|||
private String telephone; |
|||
@ApiModelProperty("价格") |
|||
private String price; |
|||
@ApiModelProperty("房源") |
|||
private String housingResources; |
|||
} |
@ -0,0 +1,46 @@ |
|||
package com.yxt.supervise.crm.api.warehouselocation; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.HashMap; |
|||
import java.util.Map; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/12 16:48 |
|||
*/ |
|||
@ApiModel(value = "仓库位置信息 数据传输对象", description = "仓库位置信息 数据传输对象") |
|||
@Data |
|||
public class WarehouseLocationDto implements Dto { |
|||
private String sid; |
|||
private String id; |
|||
@ApiModelProperty("位置") |
|||
private String address; |
|||
@ApiModelProperty("省") |
|||
private String province; |
|||
@ApiModelProperty("市") |
|||
private String city; |
|||
@ApiModelProperty("区") |
|||
private String county; |
|||
@ApiModelProperty("面积") |
|||
private String squareMeasure; |
|||
@ApiModelProperty("层高") |
|||
private String floorHeight; |
|||
@ApiModelProperty("性质") |
|||
private String properties; |
|||
@ApiModelProperty("联系人") |
|||
private String contacts; |
|||
@ApiModelProperty("电话") |
|||
private String telephone; |
|||
@ApiModelProperty("价格") |
|||
private String price; |
|||
@ApiModelProperty("房源") |
|||
private String housingResources; |
|||
@ApiModelProperty("备注") |
|||
private String remarks; |
|||
private String [] imageFiles; |
|||
|
|||
} |
@ -0,0 +1,18 @@ |
|||
package com.yxt.supervise.crm.api.warehouselocation; |
|||
|
|||
import com.yxt.supervise.crm.api.loanbankinformation.LoanBankInformationFeignFallback; |
|||
import io.swagger.annotations.Api; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/12 16:48 |
|||
*/ |
|||
@Api(tags = "贷款银行信息") |
|||
@FeignClient( |
|||
contextId = "supervise-crm-WarehouseLocation", |
|||
name = "supervise-crm", |
|||
path = "v1/warehouselocation", |
|||
fallback = WarehouseLocationFeignFallback.class) |
|||
public interface WarehouseLocationFeign { |
|||
} |
@ -0,0 +1,11 @@ |
|||
package com.yxt.supervise.crm.api.warehouselocation; |
|||
|
|||
import org.springframework.stereotype.Component; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/12 16:49 |
|||
*/ |
|||
@Component |
|||
public class WarehouseLocationFeignFallback { |
|||
} |
@ -0,0 +1,39 @@ |
|||
package com.yxt.supervise.crm.api.warehouselocation; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/12 16:49 |
|||
*/ |
|||
@ApiModel(value = "仓库位置信息 查询条件", description = "仓库位置信息 查询条件") |
|||
@Data |
|||
public class WarehouseLocationQuery implements Query { |
|||
private String sid; |
|||
private String id; |
|||
@ApiModelProperty("位置") |
|||
private String address; |
|||
@ApiModelProperty("省") |
|||
private String province; |
|||
@ApiModelProperty("市") |
|||
private String city; |
|||
@ApiModelProperty("区") |
|||
private String county; |
|||
@ApiModelProperty("面积") |
|||
private String squareMeasure; |
|||
@ApiModelProperty("层高") |
|||
private String floorHeight; |
|||
@ApiModelProperty("性质") |
|||
private String properties; |
|||
@ApiModelProperty("联系人") |
|||
private String contacts; |
|||
@ApiModelProperty("电话") |
|||
private String telephone; |
|||
@ApiModelProperty("价格") |
|||
private String price; |
|||
@ApiModelProperty("房源") |
|||
private String housingResources; |
|||
} |
@ -0,0 +1,42 @@ |
|||
package com.yxt.supervise.crm.api.warehouselocation; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/4/12 16:49 |
|||
*/ |
|||
@ApiModel(value = "仓库位置信息 视图数据对象", description = "仓库位置信息 视图数据对象") |
|||
@Data |
|||
public class WarehouseLocationVo implements Vo { |
|||
private String sid; |
|||
private String id; |
|||
@ApiModelProperty("位置") |
|||
private String address; |
|||
@ApiModelProperty("省") |
|||
private String province; |
|||
@ApiModelProperty("市") |
|||
private String city; |
|||
@ApiModelProperty("区") |
|||
private String county; |
|||
@ApiModelProperty("面积") |
|||
private String squareMeasure; |
|||
@ApiModelProperty("层高") |
|||
private String floorHeight; |
|||
@ApiModelProperty("性质") |
|||
private String properties; |
|||
@ApiModelProperty("联系人") |
|||
private String contacts; |
|||
@ApiModelProperty("电话") |
|||
private String telephone; |
|||
@ApiModelProperty("价格") |
|||
private String price; |
|||
@ApiModelProperty("房源") |
|||
private String housingResources; |
|||
@ApiModelProperty("备注") |
|||
private String remarks; |
|||
private String url; |
|||
} |
@ -0,0 +1,267 @@ |
|||
package com.yxt.supervise.system.config; |
|||
|
|||
import io.swagger.annotations.ApiModelProperty; |
|||
|
|||
/** |
|||
* @author dimengzhe |
|||
* @date 2021/7/1 10:23 |
|||
* @description 常量值管理 |
|||
*/ |
|||
public class DictCommonType { |
|||
|
|||
//客户端获取手机验证码redis前缀定义
|
|||
public static final String WX_REGIST = "wx-regist-";//注册获取验证码
|
|||
public static final String WX_LOGIN = "wx-login-";//登录获取验证码
|
|||
public static final String WX_FORGET = "wx-forget-";//忘记密码获取验证码
|
|||
public static final String WX_UPDATE = "wx-update-";//忘记密码获取验证码
|
|||
public static final String WX_NEW = "wx-new-";//新手机号获取验证码
|
|||
|
|||
/*供应厂商相关*/ |
|||
@ApiModelProperty(value = "供应商分类") |
|||
public static final String SUPPLIER_TYPE = "supplierType";//数据字典已添加
|
|||
@ApiModelProperty(value = "供应类别") |
|||
public static final String SUPPLY_TYPE = "supplyType";//数据字典已添加
|
|||
@ApiModelProperty(value = "供应商分组") |
|||
public static final String SUPPLIE_RGROUP = "supplierGroup";//数据字典已添加
|
|||
|
|||
/*供应厂商财务信息相关*/ |
|||
@ApiModelProperty(value = "结算币种") |
|||
public static final String SETTLEMENT_CURRENCY = "settlementCurrency";//数据字典已添加
|
|||
@ApiModelProperty(value = "结算方式") |
|||
public static final String SETTLEMENT_WAY = "settlementWay";//数据字典已添加
|
|||
@ApiModelProperty(value = "税分类") |
|||
public static final String TAX_CLASSIFICATION = "taxClassification";//数据字典已添加
|
|||
/*基础信息品牌相关*/ |
|||
@ApiModelProperty(value = "品牌类型") |
|||
public static final String BRAND_TYPE = "brandType";//数据字典已添加
|
|||
@ApiModelProperty(value = "职级(岗位)") |
|||
public static final String POSTLEVEL = "postLevel"; |
|||
|
|||
/*车型、车型配置、车辆信息相关*/ |
|||
@ApiModelProperty(value = "车辆类型") |
|||
public static final String VEHICLE_TYPE = "vehicleType";//数据字典已添加
|
|||
@ApiModelProperty(value = "排放标准") |
|||
public static final String EMISSION_STANDARD = "emissionStandard";//数据字典已添加
|
|||
@ApiModelProperty(value = "产品线") |
|||
public static final String PRODUCT_LINE = "productLine";//数据字典已添加
|
|||
@ApiModelProperty(value = "后桥") |
|||
public static final String REAR_AXLE = "rearAxle";//数据字典已添加
|
|||
@ApiModelProperty(value = "速比") |
|||
public static final String SPEED_RATIO = "speedRatio";//数据字典已添加
|
|||
@ApiModelProperty(value = "系别") |
|||
private static final String SERIES = "series";//数据字典已添加
|
|||
@ApiModelProperty(value = "车辆功能") |
|||
private static final String VEHICLE_FUNCTION = "vehicleFunction";//数据字典已添加
|
|||
@ApiModelProperty(value = "细分市场") |
|||
private static final String MARKETSEGMENTS = "marketSegments";//数据字典已添加
|
|||
|
|||
@ApiModelProperty(value = "车身颜色") |
|||
public static final String BODYCOLOR = "bodyColor"; |
|||
@ApiModelProperty(value = "缓速器") |
|||
public static final String SLOWMACHINE = "slowMachine"; |
|||
@ApiModelProperty(value = "后视镜") |
|||
public static final String REARVIEWMIRROR = "rearViewMirror"; |
|||
@ApiModelProperty(value = "轮胎") |
|||
public static final String TIRESIZE = "tireSize"; |
|||
@ApiModelProperty(value = "驾驶室") |
|||
public static final String SPECIFICATION = "specification"; |
|||
@ApiModelProperty(value = "后桥速比") |
|||
public static final String REARAXLERATIO = "rearAxleRatio"; |
|||
@ApiModelProperty(value = "轮毂材质") |
|||
public static final String HUBMATERIAL = "hubMaterial"; |
|||
@ApiModelProperty(value = "悬架") |
|||
public static final String SUSPENSION = "suspension"; |
|||
@ApiModelProperty(value = "座椅") |
|||
public static final String SEAT = "seat"; |
|||
@ApiModelProperty(value = "鞍座") |
|||
public static final String SADDLE = "saddle"; |
|||
@ApiModelProperty(value = "轴距") |
|||
public static final String WHEELBASE = "wheelbase"; |
|||
@ApiModelProperty(value = "保险杠") |
|||
public static final String BUMPER = "bumper"; |
|||
@ApiModelProperty(value = "配置包") |
|||
public static final String CONFIGURINGBAO = "configuringBao"; |
|||
@ApiModelProperty(value = "独立热源") |
|||
public static final String INDEPENDENTSOURCES = "independentSources"; |
|||
@ApiModelProperty(value = "燃料箱") |
|||
public static final String FUELTANK = "fuelTank"; |
|||
@ApiModelProperty(value = "有无:1有0无") |
|||
public static final String WHETHER = "whether"; |
|||
|
|||
@ApiModelProperty(value = "车辆状态") |
|||
public static final String VEHICLE_STATE = "vehicleState"; |
|||
@ApiModelProperty(value = "车辆状态(车辆台账)") |
|||
public static final String VEHICLE_LEDGER_STATE = "vehicleLedgerState"; |
|||
@ApiModelProperty(value = "驱动") |
|||
public static final String DRIVER = "driver"; |
|||
@ApiModelProperty(value = "马力") |
|||
public static final String HORSEPOWER = "horsepower"; |
|||
@ApiModelProperty(value = "锁定状态") |
|||
public static final String LOCKED_STATE = "lockedState"; |
|||
@ApiModelProperty(value = "预计订金日期") |
|||
public static final String RESERVE_DEPOSIT_DATE = "reserveDepositDate"; |
|||
|
|||
|
|||
@ApiModelProperty(value = "贷款主体类型") |
|||
public static final String LOANTYPE = "loanType"; |
|||
@ApiModelProperty(value = "打包项目") |
|||
public static final String PACKAGINGPROJECT = "packagingProject"; |
|||
@ApiModelProperty(value = "融资项目(比打包项目多一个“配件”)") |
|||
public static final String PACKAGINGPROJECTFIN = "packagingProjectFin"; |
|||
@ApiModelProperty(value = "变速箱") |
|||
public static final String GEARBOX = "gearbox"; |
|||
@ApiModelProperty(value = "燃料种类") |
|||
public static final String FUELTYPE = "fuelType"; |
|||
@ApiModelProperty(value = "版本(车辆需求)") |
|||
public static final String VEHICLEVERSION = "vehicleVersion"; |
|||
@ApiModelProperty(value = "采购形式") |
|||
public static final String PURCHASINGFORM = "purchasingForm"; |
|||
/*经销商相关*/ |
|||
@ApiModelProperty(value = "经销商分类") |
|||
public static final String DISTRIBUTOR_CLASSIFICATION = "distributorClassification";//数据字典已添加
|
|||
@ApiModelProperty(value = "经销商类型") |
|||
public static final String DISTRIBUTOR_TYPE = "distributorType";//数据字典已添加
|
|||
@ApiModelProperty(value = "经销商等级") |
|||
public static final String DISTRIBUTOR_LEVEL = "distributorLevel";//数据字典已添加
|
|||
@ApiModelProperty(value = "企业性质") |
|||
public static final String ENTERPRISE_NATURE = "enterpriseNature";//数据字典已添加
|
|||
@ApiModelProperty(value = "登记状态") |
|||
public static final String REGIST_STATE = "registState"; |
|||
@ApiModelProperty(value = "注册资本单位") |
|||
public static final String REGISTEREDCAPITAL_ORG = "registeredCapitalOrg"; |
|||
@ApiModelProperty(value = "项目类型") |
|||
public static final String PROJECT_TYPE = "projectType"; |
|||
/*经销商相关结束*/ |
|||
|
|||
|
|||
@ApiModelProperty(value = "合格证情况:0001虚拟 ,002正式") |
|||
public static final String CERTIFICATE_SITUATION = "certificateSituation"; |
|||
@ApiModelProperty(value = "客户类型:1个人2企业") |
|||
public static final String CUSTOMER_TYPE = "customerType"; |
|||
@ApiModelProperty(value = "登记注册号类型") |
|||
public static final String REGIST_NUM_TYPE = "registNumType"; |
|||
@ApiModelProperty(value = "现居住状况") |
|||
public static final String CURRENT_LIVE_STATE = "currentlivestate"; |
|||
@ApiModelProperty(value = "教育程度") |
|||
public static final String EDUCATION_DEGREE = "educationdegree"; |
|||
@ApiModelProperty(value = "雇员类型") |
|||
public static final String EMPLOYEE_TYPE = "employeetype"; |
|||
@ApiModelProperty(value = "证件类型") |
|||
public static final String DOCUMENT_TYPE = "documenttype"; |
|||
@ApiModelProperty(value = "行业类别") |
|||
public static final String INDUSTRY_TYPE = "industrytype"; |
|||
@ApiModelProperty(value = "经济类型") |
|||
public static final String ECONOMIC_TYPE = "economictype"; |
|||
@ApiModelProperty(value = "组织机构类别") |
|||
public static final String ORGANIZATION_TYPE = "organizationtype"; |
|||
@ApiModelProperty(value = "组织机构类别细分") |
|||
public static final String ORGANIZATION_TYPE_DETAILS = "organizationtypedetails"; |
|||
|
|||
@ApiModelProperty(value = "是或否") |
|||
public static final String IS_TRUE = "isTrue"; |
|||
@ApiModelProperty(value = "准驾车型") |
|||
public static final String CAR_TYPE = "carType"; |
|||
|
|||
@ApiModelProperty(value = "与客户关系") |
|||
public static final String RELATION_SHIP = "relationship"; |
|||
@ApiModelProperty(value = "现工作单位性质") |
|||
public static final String ORG_NATURE = "orgNature"; |
|||
@ApiModelProperty(value = "主要收入来源") |
|||
public static final String INCOME_SOURCE = "incomeSource"; |
|||
@ApiModelProperty(value = "职位") |
|||
public static final String POSITION = "position"; |
|||
@ApiModelProperty(value = "经销商类型") |
|||
public static final String DEALERS_TYPE = "dealersType"; |
|||
@ApiModelProperty(value = "附件类型") |
|||
public static final String ATTACH_TYPE = "attachType"; |
|||
@ApiModelProperty(value = "信用记录") |
|||
public static final String CREDIT_RECORD = "creditRecord"; |
|||
@ApiModelProperty(value = "拟租赁形式l") |
|||
public static final String PLANS_TO_LEASE = "plansToLease"; |
|||
@ApiModelProperty(value = "去返程货物") |
|||
public static final String GO_GOODS = "goGoods"; |
|||
@ApiModelProperty(value = "经营业务范围") |
|||
public static final String BUSINESSSCOPE = "businessScope"; |
|||
/* 客户信息相关*/ |
|||
@ApiModelProperty(value = "客户分类") |
|||
public static final String CUSTOMER_CLASS = "customerClass"; |
|||
@ApiModelProperty(value = "客户来源") |
|||
public static final String CUSTOMER_SOURCE = "customerSource"; |
|||
@ApiModelProperty(value = "客户等级") |
|||
public static final String CUSTOMER_LEVEL = "customerLevel"; |
|||
@ApiModelProperty(value = "来访方式") |
|||
public static final String VISIT_WAY = "visitWay"; |
|||
@ApiModelProperty(value = "跟进状态") |
|||
public static final String FOLLOW_STATE = "followState"; |
|||
@ApiModelProperty(value = "提醒日期(天)") |
|||
public static final String REMIND_DAY = "remindDay"; |
|||
@ApiModelProperty(value = "承运货物类型") |
|||
public static final String CONSIGNMENT_TYPE = "consignmentType"; |
|||
@ApiModelProperty(value = "承运货物(暂为假数据)") |
|||
public static final String CONSIGNMENT = "consignment"; |
|||
@ApiModelProperty(value = "购车方式") |
|||
public static final String PURCHASETYPE = "purchaseType"; |
|||
@ApiModelProperty(value = "提车方式") |
|||
public static final String SALETYPE = "saleType"; |
|||
/* 车辆订单 */ |
|||
@ApiModelProperty(value = "单据类型") |
|||
public static final String BILLSTYPE = "billsType"; |
|||
@ApiModelProperty(value = "合同类型") |
|||
public static final String CONTRACTTYPE = "contractType"; |
|||
|
|||
@ApiModelProperty(value = "主车优惠类型") |
|||
public static final String DISCOUNTTYPE = "discountType"; |
|||
@ApiModelProperty(value = "还款方式") |
|||
public static final String MODEOFREPAY = "modeOfRePay"; |
|||
@ApiModelProperty(value = "保证金方式") |
|||
public static final String BONDMETHOD = "bondMethod"; |
|||
@ApiModelProperty(value = "业务类型") |
|||
public static final String BUSINESSTYPE = "businessType"; |
|||
@ApiModelProperty(value = "开户银行") |
|||
public static final String BANK = "bank"; |
|||
@ApiModelProperty(value = "账户类型") |
|||
public static final String ACCOUNTTYPE = "accountType"; |
|||
@ApiModelProperty(value = "运输货物") |
|||
public static final String TRANSPORTCARGO = "transportCargo"; |
|||
@ApiModelProperty(value = "结账周期") |
|||
public static final String CHECKOUTCYCLE = "checkoutCycle"; |
|||
@ApiModelProperty(value = "虚拟订单类型") |
|||
public static final String DEPOSITBILLTYPE = "depositBillType"; |
|||
@ApiModelProperty(value = "付款方式") |
|||
public static final String PAYMENTTYPE = "paymentType"; |
|||
|
|||
/* 物料相关 */ |
|||
@ApiModelProperty(value = "物料分组") |
|||
public static final String MATERIAL_GROUP = "materialGroup"; |
|||
@ApiModelProperty(value = "物料属性") |
|||
public static final String MATERIAL_PROPERTIES = "materialProperties"; |
|||
@ApiModelProperty(value = "存货类别") |
|||
public static final String STOCK_TYPE = "stockType"; |
|||
@ApiModelProperty(value = "基本单位") |
|||
public static final String BASIC_UNIT = "basicUnit"; |
|||
|
|||
/* 合同相关 */ |
|||
@ApiModelProperty(value = "人员类型") |
|||
public static final String PERSONNEL_TYPE = "personnelType"; |
|||
|
|||
/* 开票申请单相关 */ |
|||
@ApiModelProperty(value = "开票性质") |
|||
public static final String INVOICING_NATURE = "invoicingNature"; |
|||
|
|||
/*员工信息管理相关*/ |
|||
@ApiModelProperty(value = "婚姻状况") |
|||
public static final String MARITAL_STATUS = "maritalstatus"; |
|||
@ApiModelProperty(value = "民族") |
|||
public static final String NATIONAL = "national"; |
|||
@ApiModelProperty(value = "性别") |
|||
public static final String SEX = "sex"; |
|||
@ApiModelProperty(value = "政治面貌") |
|||
public static final String POLITICAL = "political"; |
|||
|
|||
|
|||
/*资料清单相关*/ |
|||
@ApiModelProperty(value = "资料类别") |
|||
public static final String DATA_TYPE = "dataType"; |
|||
@ApiModelProperty(value = "文件格式") |
|||
public static final String FILE_TYPE = "fileType"; |
|||
} |
@ -0,0 +1,66 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.supervise.system.dictcommon; |
|||
|
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: yxt_supervise(宇信通监管) <br/> |
|||
* File: DictCommonVo.java <br/> |
|||
* Class: com.supervise.api.dictcommon.DictCommonVo <br/> |
|||
* Description: 数据字典——数据项 视图数据对象. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2022-11-11 11:40:29 <br/> |
|||
* |
|||
* @author dongjianzhao |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "数据字典——数据项 视图数据详情", description = "数据字典——数据项 视图数据详情") |
|||
public class DictCommonDetailsVo implements Vo { |
|||
|
|||
private String sid; // sid
|
|||
|
|||
@ApiModelProperty("类型code") |
|||
private String dictType; // 类型code
|
|||
@ApiModelProperty("key值") |
|||
private String dictKey; // key值
|
|||
@ApiModelProperty("value值") |
|||
private String dictValue; // value值
|
|||
@ApiModelProperty("路径") |
|||
private String sidPath; // 路径
|
|||
@ApiModelProperty("父级sid") |
|||
private String parentSid; // 父级sid
|
|||
@ApiModelProperty("分组名称") |
|||
private String groupName; // 分组名称
|
|||
|
|||
} |
@ -0,0 +1,66 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.supervise.system.dictcommon; |
|||
|
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: yxt_supervise(宇信通监管) <br/> |
|||
* File: DictCommonDto.java <br/> |
|||
* Class: com.supervise.api.dictcommon.DictCommonDto <br/> |
|||
* Description: 数据字典——数据项 数据传输对象. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2022-11-11 11:40:28 <br/> |
|||
* |
|||
* @author dongjianzhao |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "数据字典——数据项 数据传输对象", description = "数据字典——数据项 数据传输对象") |
|||
public class DictCommonDto implements Dto { |
|||
|
|||
private String sid; // sid
|
|||
|
|||
@ApiModelProperty("类型code") |
|||
private String dictType; // 类型code
|
|||
@ApiModelProperty("key值") |
|||
private String dictKey; // key值
|
|||
@ApiModelProperty("value值") |
|||
private String dictValue; // value值
|
|||
@ApiModelProperty("路径") |
|||
private String sidPath; // 路径
|
|||
@ApiModelProperty("父级sid") |
|||
private String parentSid; // 父级sid
|
|||
@ApiModelProperty("分组名称") |
|||
private String groupName; // 分组名称
|
|||
|
|||
} |
@ -0,0 +1,94 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.supervise.system.dictcommon; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import io.swagger.annotations.ApiParam; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* Project: yxt_supervise(宇信通监管) <br/> |
|||
* File: DictCommonFeign.java <br/> |
|||
* Class: com.supervise.api.dictcommon.DictCommonFeign <br/> |
|||
* Description: 数据字典——数据项. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2022-11-11 11:40:28 <br/> |
|||
* |
|||
* @author dongjianzhao |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Api(tags = "数据字典——数据项") |
|||
@FeignClient( |
|||
contextId = "supervise-system-DictCommon", |
|||
name = "supervise-system", |
|||
path = "v1/dictcommon", |
|||
fallback = DictCommonFeignFallback.class) |
|||
public interface DictCommonFeign { |
|||
|
|||
@ApiOperation("根据条件分页查询数据的列表") |
|||
@PostMapping("/listPage") |
|||
@ResponseBody |
|||
public ResultBean<PagerVo<DictCommonVo>> listPage(@RequestBody PagerQuery<DictCommonQuery> pq); |
|||
|
|||
@ApiOperation("新增或修改") |
|||
@PostMapping("/save") |
|||
@ResponseBody |
|||
public ResultBean save(@RequestBody DictCommonDto dto); |
|||
|
|||
@ApiOperation("根据sid删除记录") |
|||
@DeleteMapping("/delBySids") |
|||
@ResponseBody |
|||
public ResultBean delBySids(@RequestBody String[] sids); |
|||
|
|||
@ApiOperation("根据SID获取一条记录") |
|||
@GetMapping("/fetchDetailsBySid/{sid}") |
|||
@ResponseBody |
|||
public ResultBean<DictCommonDetailsVo> fetchDetailsBySid(@PathVariable("sid") String sid); |
|||
|
|||
@GetMapping("/typeValues") |
|||
@ResponseBody |
|||
@ApiOperation("下拉框的获取") |
|||
ResultBean<List<DictCommonVo>> getTypeValues(@RequestParam("type") String type, @RequestParam(value = "psid", defaultValue = "0") String psid); |
|||
|
|||
@GetMapping("/getFirstDictKeyByType") |
|||
@ResponseBody |
|||
@ApiOperation("根据数据字典的key获取第一个值(默认值)") |
|||
ResultBean getFirstDictKeyByType(@RequestParam("dictType") String dictType, @RequestParam("psid") String psid); |
|||
|
|||
@ResponseBody |
|||
@GetMapping("/selectBykey/{key}/{type}") |
|||
@ApiOperation(value = "数据字典信息修改时的初始化信息") |
|||
public ResultBean<DictCommonVo> selectBykey(@ApiParam(value = "数据字典key", required = true) @PathVariable("key") String key, @ApiParam(value = "数据字典type", required = true) @PathVariable("type") String type); |
|||
} |
@ -0,0 +1,88 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.supervise.system.dictcommon; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import org.springframework.stereotype.Component; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* Project: yxt_supervise(宇信通监管) <br/> |
|||
* File: DictCommonFeignFallback.java <br/> |
|||
* Class: com.supervise.api.dictcommon.DictCommonFeignFallback <br/> |
|||
* Description: 数据字典——数据项. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2022-11-11 11:40:29 <br/> |
|||
* |
|||
* @author dongjianzhao |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Component |
|||
public class DictCommonFeignFallback implements DictCommonFeign { |
|||
|
|||
@Override |
|||
public ResultBean<PagerVo<DictCommonVo>> listPage(PagerQuery<DictCommonQuery> pq){ |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
return rb.setMsg("接口yxt_supervise/dictcommon/listPage无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean save(DictCommonDto dto){ |
|||
return ResultBean.fireFail().setMsg("接口yxt_supervise/dictcommon/save无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean delBySids( String[] sids){ |
|||
return ResultBean.fireFail().setMsg("接口yxt_supervise/dictcommon/delBySids无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<DictCommonDetailsVo> fetchDetailsBySid(String sid){ |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
return rb.setMsg("接口yxt_supervise/dictcommon/fetchDetailsBySid无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<DictCommonVo>> getTypeValues(String type, String psid) { |
|||
ResultBean<List<DictCommonVo>> rb = ResultBean.fireFail(); |
|||
return rb.setMsg("接口yxt_supervise/dictcommon/getTypeValues无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean getFirstDictKeyByType(String dictType, String psid) { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<DictCommonVo> selectBykey(String key, String type) { |
|||
return null; |
|||
} |
|||
} |
@ -0,0 +1,64 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.supervise.system.dictcommon; |
|||
|
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: yxt_supervise(宇信通监管) <br/> |
|||
* File: DictCommonQuery.java <br/> |
|||
* Class: com.supervise.api.dictcommon.DictCommonQuery <br/> |
|||
* Description: 数据字典——数据项 查询条件. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2022-11-11 11:40:28 <br/> |
|||
* |
|||
* @author dongjianzhao |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "数据字典——数据项 查询条件", description = "数据字典——数据项 查询条件") |
|||
public class DictCommonQuery implements Query { |
|||
|
|||
@ApiModelProperty("类型code") |
|||
private String dictType; // 类型code
|
|||
@ApiModelProperty("key值") |
|||
private String dictKey; // key值
|
|||
@ApiModelProperty("value值") |
|||
private String dictValue; // value值
|
|||
@ApiModelProperty("路径") |
|||
private String sidPath; // 路径
|
|||
@ApiModelProperty("父级sid") |
|||
private String parentSid; // 父级sid
|
|||
@ApiModelProperty("分组名称") |
|||
private String groupName; // 分组名称
|
|||
|
|||
} |
@ -0,0 +1,66 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.supervise.system.dictcommon; |
|||
|
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: yxt_supervise(宇信通监管) <br/> |
|||
* File: DictCommonVo.java <br/> |
|||
* Class: com.supervise.api.dictcommon.DictCommonVo <br/> |
|||
* Description: 数据字典——数据项 视图数据对象. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2022-11-11 11:40:28 <br/> |
|||
* |
|||
* @author dongjianzhao |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "数据字典——数据项 视图数据对象", description = "数据字典——数据项 视图数据对象") |
|||
public class DictCommonVo implements Vo { |
|||
|
|||
private String sid; // sid
|
|||
|
|||
@ApiModelProperty("类型code") |
|||
private String dictType; // 类型code
|
|||
@ApiModelProperty("key值") |
|||
private String dictKey; // key值
|
|||
@ApiModelProperty("value值") |
|||
private String dictValue; // value值
|
|||
@ApiModelProperty("路径") |
|||
private String sidPath; // 路径
|
|||
@ApiModelProperty("父级sid") |
|||
private String parentSid; // 父级sid
|
|||
@ApiModelProperty("分组名称") |
|||
private String groupName; // 分组名称
|
|||
|
|||
} |
@ -0,0 +1,23 @@ |
|||
package com.yxt.supervise.system.sysorganization; |
|||
|
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author dimengzhe |
|||
* @date 2020/11/3 16:19 |
|||
* @description |
|||
*/ |
|||
@Data |
|||
public class QrCodeVo { |
|||
@ApiModelProperty("部门名称") |
|||
private String departmentName; |
|||
@ApiModelProperty("单位名称") |
|||
private String organizationName; |
|||
@ApiModelProperty("部门地址") |
|||
private String address; |
|||
@ApiModelProperty("二维码图片地址") |
|||
private String qrFilePath; |
|||
@ApiModelProperty(value = "部门sid") |
|||
private String sid; |
|||
} |
@ -0,0 +1,29 @@ |
|||
package com.yxt.supervise.system.sysorganization; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @Author dimengzhe |
|||
* @Date 2022/2/14 11:45 |
|||
* @Description 员工所在部门下拉列表 |
|||
*/ |
|||
@Data |
|||
public class SysOrgListVo implements Vo { |
|||
private static final long serialVersionUID = -3558876605554852892L; |
|||
|
|||
@ApiModelProperty(value = "组织名称") |
|||
private String name; |
|||
@ApiModelProperty(value = "部门编码") |
|||
private String orgCode; |
|||
@ApiModelProperty(value = "sid") |
|||
private String sid; |
|||
@ApiModelProperty(value = "上级sid") |
|||
private String psid; |
|||
@ApiModelProperty(value = "orgSidPath") |
|||
private String orgSidPath; |
|||
private List<SysOrgVo> children; |
|||
} |
@ -0,0 +1,17 @@ |
|||
package com.yxt.supervise.system.sysorganization; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @Author dimengzhe |
|||
* @Date 2022/9/26 11:48 |
|||
* @Description |
|||
*/ |
|||
@Data |
|||
public class SysOrgStaffVo implements Vo { |
|||
private static final long serialVersionUID = -4311553944345419092L; |
|||
|
|||
private String staffName; |
|||
private String staffSid; |
|||
} |
@ -0,0 +1,31 @@ |
|||
package com.yxt.supervise.system.sysorganization; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author dimengzhe |
|||
* @date 2021/10/12 20:55 |
|||
* @description |
|||
*/ |
|||
@Data |
|||
public class SysOrgVo implements Vo { |
|||
private static final long serialVersionUID = 2983637708030419942L; |
|||
@ApiModelProperty(value = "组织名称") |
|||
private String name; |
|||
@ApiModelProperty(value = "sid") |
|||
private String sid; |
|||
@ApiModelProperty(value = "psid") |
|||
private String psid; |
|||
@ApiModelProperty(value = "id") |
|||
private String id; |
|||
@ApiModelProperty(value = "orgSidPath") |
|||
private String orgSidPath; |
|||
@ApiModelProperty(value = "部门编码") |
|||
private String orgCode; |
|||
|
|||
private List<SysOrgVo> children; |
|||
} |
@ -0,0 +1,87 @@ |
|||
package com.yxt.supervise.system.sysorganization; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: anrui_portal(门户建设) <br/> |
|||
* File: SysOrganization.java <br/> |
|||
* Class: SysOrganization <br/> |
|||
* Description: 组织机构表. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2021-08-03 00:24:28 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@ApiModel(value = "组织机构表", description = "组织机构表") |
|||
@TableName("sys_organization") |
|||
@Data |
|||
public class SysOrganization extends BaseEntity { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
|
|||
@ApiModelProperty("部门/组织名称") |
|||
private String name; |
|||
|
|||
@ApiModelProperty("父(部门/组织) sid") |
|||
private String psid; |
|||
|
|||
@ApiModelProperty("联系电话") |
|||
private String linkPhone; |
|||
@ApiModelProperty("联系人") |
|||
private String linkPerson; |
|||
|
|||
@ApiModelProperty("部门sid全路径") |
|||
private String orgSidPath; |
|||
|
|||
@ApiModelProperty("排序") |
|||
private Integer sort; |
|||
|
|||
@ApiModelProperty("地址") |
|||
private String addrs; |
|||
|
|||
@ApiModelProperty("地理位置经纬度") |
|||
private String jwd; |
|||
|
|||
@ApiModelProperty("二维码") |
|||
private String qrText; |
|||
|
|||
@ApiModelProperty("限制本部门成员查看通讯录:限制开启后,本部门成员只能看到限定范围内的通讯录不能看到所有通讯录,仅可见自己") |
|||
private Integer limitOrgMember; |
|||
|
|||
@ApiModelProperty("部门编码") |
|||
private String orgCode; |
|||
|
|||
@ApiModelProperty("部门简称(地区简称+门店名称首字母(遇到首字母重复时用2个字母))") |
|||
private String orgShortName; |
|||
|
|||
@ApiModelProperty("销售区域划分(本店终端销售:0,门店对应业务区域划分销售:1至9,具体编号划分各门店报备确定)") |
|||
private String regionDivision; |
|||
|
|||
@ApiModelProperty("是否是部门(0否,1是)") |
|||
private Integer isDept; |
|||
|
|||
@ApiModelProperty("组织简称") |
|||
private String orgAbbre; |
|||
|
|||
@ApiModelProperty("组织属性key") |
|||
private String orgAttributeKey; |
|||
|
|||
@ApiModelProperty("组织属性value") |
|||
private String orgAttributeValue; |
|||
|
|||
@ApiModelProperty("管理层级key") |
|||
private String orgLevelKey; |
|||
|
|||
@ApiModelProperty("管理层级value") |
|||
private String orgLevelValue; |
|||
|
|||
@ApiModelProperty("其他编码") |
|||
private String otherCode; |
|||
} |
@ -0,0 +1,91 @@ |
|||
package com.yxt.supervise.system.sysorganization; |
|||
|
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: anrui_portal(门户建设) <br/> |
|||
* File: SysOrganizationDto.java <br/> |
|||
* Class: SysOrganizationDto <br/> |
|||
* Description: 组织机构表 数据传输对象. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2021-08-03 00:24:28 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@ApiModel(value = "组织机构表 数据传输对象", description = "组织机构表 数据传输对象") |
|||
@Data |
|||
public class SysOrganizationDto implements Dto { |
|||
|
|||
|
|||
@ApiModelProperty("部门/组织名称") |
|||
private String name; |
|||
|
|||
@ApiModelProperty("父(部门/组织) sid") |
|||
private String psid; |
|||
|
|||
@ApiModelProperty("联系电话") |
|||
private String linkPhone; |
|||
@ApiModelProperty("联系人") |
|||
private String linkPerson; |
|||
|
|||
@ApiModelProperty("部门sid全路径") |
|||
private String orgSidPath; |
|||
|
|||
@ApiModelProperty("排序") |
|||
private Integer sort; |
|||
|
|||
@ApiModelProperty("地址") |
|||
private String addrs; |
|||
|
|||
@ApiModelProperty("地理位置经纬度") |
|||
private String jwd; |
|||
|
|||
@ApiModelProperty("二维码") |
|||
private String qrText; |
|||
|
|||
@ApiModelProperty("限制本部门成员查看通讯录:限制开启后,本部门成员只能看到限定范围内的通讯录不能看到所有通讯录,仅可见自己") |
|||
private Integer limitOrgMember; |
|||
|
|||
@ApiModelProperty("部门编码") |
|||
private String orgCode; |
|||
|
|||
@ApiModelProperty("部门简称(地区简称+门店名称首字母(遇到首字母重复时用2个字母))") |
|||
private String orgShortName; |
|||
|
|||
@ApiModelProperty("销售区域划分(本店终端销售:0,门店对应业务区域划分销售:1至9,具体编号划分各门店报备确定)") |
|||
private String regionDivision; |
|||
|
|||
@ApiModelProperty("主管人员sid") |
|||
private String zgStaffSid; |
|||
|
|||
@ApiModelProperty("分管人员sid") |
|||
private String fgStaffSid; |
|||
|
|||
@ApiModelProperty("组织简称") |
|||
private String orgAbbre; |
|||
|
|||
@ApiModelProperty("是否是部门(0否,1是)") |
|||
private Integer isDept; |
|||
|
|||
@ApiModelProperty("组织属性key") |
|||
private String orgAttributeKey; |
|||
|
|||
@ApiModelProperty("组织属性value") |
|||
private String orgAttributeValue; |
|||
|
|||
@ApiModelProperty("管理层级key") |
|||
private String orgLevelKey; |
|||
|
|||
@ApiModelProperty("管理层级value") |
|||
private String orgLevelValue; |
|||
|
|||
@ApiModelProperty("其他编码") |
|||
private String otherCode; |
|||
} |
@ -0,0 +1,182 @@ |
|||
package com.yxt.supervise.system.sysorganization; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import io.swagger.annotations.ApiParam; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
import org.springframework.cloud.openfeign.SpringQueryMap; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import java.util.List; |
|||
import java.util.Map; |
|||
|
|||
/** |
|||
* Project: anrui_portal(门户建设) <br/> |
|||
* File: SysOrganizationFeign.java <br/> |
|||
* Class: SysOrganizationFeign <br/> |
|||
* Description: 组织机构表. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2021-08-03 00:24:28 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Api(tags = "组织机构表") |
|||
@FeignClient( |
|||
contextId = "supervise-system-SysOrganization", |
|||
name = "supervise-system", |
|||
path = "v1/sysorganization", |
|||
fallback = SysOrganizationFeignFallback.class) |
|||
public interface SysOrganizationFeign { |
|||
|
|||
@ApiOperation("根据条件分页查询数据的列表") |
|||
@PostMapping("/listPage") |
|||
public ResultBean<PagerVo<SysOrganizationVo>> listPage(@RequestBody PagerQuery<SysOrganizationQuery> pq); |
|||
|
|||
@ApiOperation("根据条件查询所有数据列表") |
|||
@PostMapping("/listAll") |
|||
public ResultBean<List<SysOrganizationVo>> listAll(@RequestBody SysOrganizationQuery query); |
|||
|
|||
@ApiOperation("所有数据列表") |
|||
@GetMapping("/list") |
|||
public ResultBean<List<SysOrganizationVo>> list(); |
|||
|
|||
@ApiOperation("一级组织机构列表") |
|||
@GetMapping("/selectFirstOrgList") |
|||
public ResultBean<List<SysOrganizationVo>> selectFirstOrgList(); |
|||
|
|||
@ApiOperation("根据父级sid查询子集列表") |
|||
@GetMapping("/selectChildrenListBySid/{sid}") |
|||
public ResultBean<List<SysOrganizationVo>> selectChildrenListBySid(@PathVariable("sid") String sid); |
|||
|
|||
@ApiOperation("新增保存") |
|||
@PostMapping("/save") |
|||
public ResultBean save(@RequestBody SysOrganizationDto dto); |
|||
|
|||
@ApiOperation("修改保存") |
|||
@PostMapping("/update/{sid}") |
|||
public ResultBean update(@RequestBody SysOrganizationDto dto, @PathVariable("sid") String sid); |
|||
|
|||
@ApiOperation("删除记录") |
|||
@GetMapping("/del/{ids}") |
|||
public ResultBean del(@PathVariable("ids") String ids); |
|||
|
|||
@ApiOperation("删除记录 根据sid") |
|||
@GetMapping("/delBySid/{sid}") |
|||
public ResultBean delBySid(@PathVariable("sid") String sid); |
|||
|
|||
@ApiOperation("获取一条记录") |
|||
@GetMapping("/fetch/{id}") |
|||
public ResultBean<SysOrganizationVo> fetch(@PathVariable("id") String id); |
|||
|
|||
@ApiOperation("获取一条记录 根据sid") |
|||
@ResponseBody |
|||
@GetMapping("/fetchBySid/{sid}") |
|||
public ResultBean<SysOrganizationVo> fetchBySid(@PathVariable("sid") String sid); |
|||
|
|||
|
|||
@ApiOperation("手机获取组织架构") |
|||
@ResponseBody |
|||
@GetMapping("/selectAppOrganization") |
|||
public ResultBean<Map<String, Object>> selectAppOrganization(@RequestParam(value = "sid", required = false) String sid); |
|||
|
|||
@ApiOperation("组织架构") |
|||
@ResponseBody |
|||
@GetMapping("/selectListOrg") |
|||
ResultBean<List<SysOrgVo>> selectListOrg(); |
|||
|
|||
@ApiOperation("获取到分公司") |
|||
@ResponseBody |
|||
@GetMapping("/getListOrg") |
|||
ResultBean<List<SysOrgVo>> getListOrg(); |
|||
|
|||
@ApiOperation("获取同品牌下分公司") |
|||
@ResponseBody |
|||
@GetMapping("/getListOrgByBrand/{orgSid}") |
|||
ResultBean<List<SysOrgVo>> getListOrgByBrand(@PathVariable(value = "orgSid") String orgSid); |
|||
|
|||
@ApiOperation("获取指定品牌下分公司(已筛选本分公司)") |
|||
@ResponseBody |
|||
@GetMapping("/getListOrgByBrandSid/{orgSid}/{brandSid}") |
|||
ResultBean<List<SysOrgVo>> getListOrgByBrandSid(@PathVariable(value = "orgSid") String orgSid,@PathVariable(value = "brandSid") String brandSid); |
|||
|
|||
@ApiOperation("获取指定品牌下分公司(未筛选本分公司)") |
|||
@ResponseBody |
|||
@GetMapping("/getListOrgByBrandSidNo/{orgSid}/{brandSid}") |
|||
ResultBean<List<SysOrgVo>> getListOrgByBrandSidNo(@PathVariable(value = "orgSid") String orgSid,@PathVariable(value = "brandSid") String brandSid); |
|||
|
|||
@ApiOperation("获取指定分公司下部门信息") |
|||
@ResponseBody |
|||
@GetMapping("/getListDeptByOrgSid/{orgSid}") |
|||
ResultBean<List<SysOrgVo>> getListDeptByOrgSid(@PathVariable(value = "orgSid") String orgSid); |
|||
|
|||
@ApiOperation("根据业务员sid获取到分公司") |
|||
@ResponseBody |
|||
@GetMapping("/getUseOrgByUserSid") |
|||
ResultBean<SysOrganizationVo> getUseOrgByUserSid(@RequestParam("userSid") String userSid); |
|||
|
|||
@ApiOperation("根据部门sid查询组织信息") |
|||
@ResponseBody |
|||
@GetMapping("/selectBySid") |
|||
ResultBean<SysOrganization> selectBySid(@RequestParam("sid") String sid); |
|||
|
|||
@ApiOperation("根据组织架构sid查询组织下所有分公司") |
|||
@ResponseBody |
|||
@GetMapping("/selectUseOrgSidBySid") |
|||
ResultBean<List<Map<String, String>>> selectUseOrgSidBySid(@RequestParam("sid") String sid); |
|||
|
|||
/** |
|||
* 查询公司所有的部门 |
|||
* |
|||
* @return |
|||
*/ |
|||
@ApiOperation("所在部门下拉") |
|||
@ResponseBody |
|||
@GetMapping("/selectListOne") |
|||
ResultBean<List<SysOrgListVo>> selectListOne(); |
|||
|
|||
@PostMapping("/getQrCode/{sid}") |
|||
@ResponseBody |
|||
@ApiOperation("查看二维码") |
|||
public ResultBean<QrCodeVo> getQrCode(@ApiParam(value = "sid", required = true) @PathVariable("sid") String sid); |
|||
|
|||
@GetMapping("/getOrgSid/{sid}") |
|||
@ResponseBody |
|||
@ApiOperation("根据当前用户的所在组织获取当前用户所在的分公司") |
|||
ResultBean<String> getOrgSid(@PathVariable(value = "sid") String sid); |
|||
|
|||
@GetMapping("/selectOrgList") |
|||
@ResponseBody |
|||
@ApiOperation("当前分公司销售部门及部门人员") |
|||
ResultBean<List<SysOrganizationListVo>> selectOrgList(@SpringQueryMap SysOrganizationListQuery query); |
|||
|
|||
@GetMapping("/selectOrgLists") |
|||
@ResponseBody |
|||
@ApiOperation("当前分公司下所有部门") |
|||
ResultBean<List<SysOrganizationListsVo>> selectOrgLists(@SpringQueryMap SysOrganizationListQuery query); |
|||
|
|||
@GetMapping("/selectByPSid") |
|||
@ResponseBody |
|||
@ApiOperation("查询分公司下的销售支持部") |
|||
ResultBean<SysOrganizationVo> selectByPSid(@RequestParam("sid") String sid, @RequestParam("name") String name); |
|||
|
|||
@GetMapping("/selectOrgSidList") |
|||
@ResponseBody |
|||
@ApiOperation("所有分公司sid") |
|||
ResultBean<List<SysOrganizationVo>> selectOrgSidList(); |
|||
|
|||
@GetMapping("/selectOrgByPSid") |
|||
@ResponseBody |
|||
@ApiOperation("查询事业部下所有分公司") |
|||
ResultBean<List<SysOrganizationVo>> selectOrgByPSid(@RequestParam("psid") String psid); |
|||
|
|||
@GetMapping("/selectByOrgSidPath") |
|||
@ResponseBody |
|||
@ApiOperation("组织层级分页查询") |
|||
ResultBean<SysOrganizationVo> selectByOrgSidPath(@RequestParam("orgSidPath") String orgSidPath); |
|||
} |
@ -0,0 +1,184 @@ |
|||
package com.yxt.supervise.system.sysorganization; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import org.springframework.stereotype.Component; |
|||
|
|||
import java.util.List; |
|||
import java.util.Map; |
|||
|
|||
/** |
|||
* Project: anrui_portal(门户建设) <br/> |
|||
* File: SysOrganizationFeignFallback.java <br/> |
|||
* Class: SysOrganizationFeignFallback <br/> |
|||
* Description: 组织机构表. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2021-08-03 00:24:28 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Component |
|||
public class SysOrganizationFeignFallback implements SysOrganizationFeign { |
|||
|
|||
@Override |
|||
public ResultBean<PagerVo<SysOrganizationVo>> listPage(PagerQuery<SysOrganizationQuery> pq) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
return rb.setMsg("接口anrui_portal/sysorganization/listPage无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<SysOrganizationVo>> listAll(SysOrganizationQuery query) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
return rb.setMsg("接口anrui_portal/sysorganization/listAll无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<SysOrganizationVo>> list() { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
return rb.setMsg("接口anrui_portal/sysorganization/list无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean selectFirstOrgList() { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
return rb.setMsg("接口anrui_portal/sysorganization/selectFirstOrgList无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean selectChildrenListBySid(String sid) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
return rb.setMsg("接口anrui_portal/sysorganization/selectChildrenListBySid无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean save(SysOrganizationDto dto) { |
|||
return ResultBean.fireFail().setMsg("接口anrui_portal/sysorganization/save无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean update(SysOrganizationDto dto, String sid) { |
|||
return ResultBean.fireFail().setMsg("接口anrui_portal/sysorganization/update无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean del(String ids) { |
|||
return ResultBean.fireFail().setMsg("接口anrui_portal/sysorganization/del无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean delBySid(String sid) { |
|||
return ResultBean.fireFail().setMsg("接口anrui_portal/sysorganization/del无法访问"); |
|||
|
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<SysOrganizationVo> fetch(String id) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
return rb.setMsg("接口anrui_portal/sysorganization/fetch无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<SysOrganizationVo> fetchBySid(String sid) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
return rb.setMsg("接口anrui_portal/sysorganization/fetchBySid无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean selectAppOrganization(String sid) { |
|||
return ResultBean.fireFail().setMsg("接口anrui_portal/sysorganization/selectAppOrganization无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<SysOrgVo>> selectListOrg() { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<SysOrgVo>> getListOrg() { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<SysOrgVo>> getListOrgByBrand(String orgSid) { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<SysOrgVo>> getListOrgByBrandSid(String orgSid, String brandSid) { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<SysOrgVo>> getListOrgByBrandSidNo(String orgSid, String brandSid) { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<SysOrgVo>> getListDeptByOrgSid(String orgSid) { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<SysOrganizationVo> getUseOrgByUserSid(String staffSid) { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<SysOrganization> selectBySid(String sid) { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<Map<String, String>>> selectUseOrgSidBySid(String sid) { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<SysOrgListVo>> selectListOne() { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<QrCodeVo> getQrCode(String sid) { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<String> getOrgSid(String sid) { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<SysOrganizationListVo>> selectOrgList(SysOrganizationListQuery query) { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<SysOrganizationListsVo>> selectOrgLists(SysOrganizationListQuery query) { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<SysOrganizationVo> selectByPSid(String sid, String name) { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<SysOrganizationVo>> selectOrgSidList() { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<SysOrganizationVo>> selectOrgByPSid(String psid) { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<SysOrganizationVo> selectByOrgSidPath(String orgSidPath) { |
|||
return null; |
|||
} |
|||
} |
@ -0,0 +1,16 @@ |
|||
package com.yxt.supervise.system.sysorganization; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @Author dimengzhe |
|||
* @Date 2022/9/26 11:49 |
|||
* @Description |
|||
*/ |
|||
@Data |
|||
public class SysOrganizationListQuery implements Query { |
|||
private static final long serialVersionUID = 8657016527420117948L; |
|||
|
|||
private String userSid; |
|||
} |
@ -0,0 +1,23 @@ |
|||
package com.yxt.supervise.system.sysorganization; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import lombok.Data; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @Author dimengzhe |
|||
* @Date 2022/9/26 11:42 |
|||
* @Description |
|||
*/ |
|||
@Data |
|||
public class SysOrganizationListVo implements Vo { |
|||
private static final long serialVersionUID = -3709239161608411765L; |
|||
|
|||
private String orgDeptSid; |
|||
private String orgDeptName; |
|||
|
|||
private List<SysOrgStaffVo> staffinfoVoList; |
|||
|
|||
|
|||
} |
@ -0,0 +1,17 @@ |
|||
package com.yxt.supervise.system.sysorganization; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @Author dimengzhe |
|||
* @Date 2022/9/30 11:28 |
|||
* @Description |
|||
*/ |
|||
@Data |
|||
public class SysOrganizationListsVo implements Vo { |
|||
private static final long serialVersionUID = -7021974833444298712L; |
|||
|
|||
private String orgDeptSid; |
|||
private String orgDeptName; |
|||
} |
@ -0,0 +1,58 @@ |
|||
package com.yxt.supervise.system.sysorganization; |
|||
|
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: anrui_portal(门户建设) <br/> |
|||
* File: SysOrganizationQuery.java <br/> |
|||
* Class: SysOrganizationQuery <br/> |
|||
* Description: 组织机构表 查询条件. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2021-08-03 00:24:28 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@ApiModel(value = "组织机构表 查询条件", description = "组织机构表 查询条件") |
|||
@Data |
|||
public class SysOrganizationQuery implements Query { |
|||
|
|||
|
|||
@ApiModelProperty("部门/组织名称") |
|||
private String name; |
|||
|
|||
@ApiModelProperty("父(部门/组织) sid") |
|||
private String psid; |
|||
|
|||
@ApiModelProperty("联系电话") |
|||
private String linkPhone; |
|||
@ApiModelProperty("联系人") |
|||
private String linkPerson; |
|||
|
|||
@ApiModelProperty("部门sid全路径") |
|||
private String orgSidPath; |
|||
|
|||
@ApiModelProperty("排序") |
|||
private Integer sort; |
|||
|
|||
@ApiModelProperty("地址") |
|||
private String addrs; |
|||
|
|||
@ApiModelProperty("地理位置经纬度") |
|||
private String jwd; |
|||
|
|||
@ApiModelProperty("二维码") |
|||
private String qrText; |
|||
|
|||
@ApiModelProperty("限制本部门成员查看通讯录:限制开启后,本部门成员只能看到限定范围内的通讯录不能看到所有通讯录,仅可见自己") |
|||
private Integer limitOrgMember; |
|||
|
|||
@ApiModelProperty("部门编码") |
|||
private String orgCode; |
|||
} |
Some files were not shown because too many files changed in this diff
Loading…
Reference in new issue