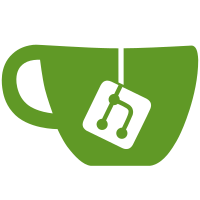
15 changed files with 415 additions and 21 deletions
@ -0,0 +1,122 @@ |
|||
package com.yxt.oms.apiadmin.aggregation; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
|
|||
import com.yxt.oms.biz.func.crmcustomer.*; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.apache.commons.lang3.StringUtils; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import javax.servlet.http.HttpServletResponse; |
|||
import javax.validation.Valid; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户管理) <br/> |
|||
* File: CrmCustomerTempFeignFallback.java <br/> |
|||
* Class: com.yxt.anrui.crm.biz.crmcustomertemp.CrmCustomerTempRest <br/> |
|||
* Description: 潜在客户信息. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2022-01-12 11:21:17 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Api(tags = "潜在客户信息") |
|||
@RestController |
|||
@RequestMapping("v1/crmcustomertemp") |
|||
public class CrmCustomerTempRest { |
|||
|
|||
@Autowired |
|||
private CrmCustomerTempService crmCustomerTempService; |
|||
|
|||
@ApiOperation("销售订单选择客户") |
|||
@PostMapping("/chooseCustomerList") |
|||
public ResultBean<PagerVo<SalesCustomerVo>> chooseCustomerList(@RequestBody PagerQuery<SalesCustomerQuery> pq) { |
|||
return crmCustomerTempService.chooseCustomerList(pq); |
|||
} |
|||
|
|||
//
|
|||
// /**
|
|||
// * 潜在客户管理的分页查询
|
|||
// *
|
|||
// * @param pq
|
|||
// * @return
|
|||
// */
|
|||
// @ApiOperation("根据条件分页查询数据的列表")
|
|||
// @PostMapping("/listPage")
|
|||
// public ResultBean<PagerVo<CrmCustomerTempVo>> listPage(@RequestBody PagerQuery<CrmCustomerTempQuery> pq) {
|
|||
// return crmCustomerTempService.listPageVo(pq);
|
|||
// }
|
|||
//
|
|||
//
|
|||
// /**
|
|||
// * 潜在客户新增保存
|
|||
// *
|
|||
// * @param dto 数据传输对象
|
|||
// * @return
|
|||
// */
|
|||
// @ApiOperation("新增保存")
|
|||
// @PostMapping("/save")
|
|||
// public ResultBean save(@Valid @RequestBody CrmCustomerTempDto dto) {
|
|||
// return crmCustomerTempService.saveCrmCustomer(dto);
|
|||
// }
|
|||
//
|
|||
// /**
|
|||
// * 潜在客户修改保存
|
|||
// *
|
|||
// * @param dto 数据传输对象
|
|||
// * @param sid 潜在客户sid
|
|||
// * @return
|
|||
// */
|
|||
// @ApiOperation("修改保存")
|
|||
// @PostMapping("/update/{sid}")
|
|||
// public ResultBean update(@RequestBody CrmCustomerTempUpdateDto dto, @PathVariable("sid") String sid) {
|
|||
// ResultBean rb = ResultBean.fireFail();
|
|||
// String weixin = dto.getWeixin();
|
|||
// if (StringUtils.isNotBlank(weixin)) {
|
|||
// if (!weixin.matches("^[a-zA-Z]{1}[-_a-zA-Z0-9]{5,19}$") && !weixin.matches("^((13[0-9])|(14[5,7])|(15[0-3,5-9])|(17[0,3,5-8])|(18[0-9])|166|198|199|(147))\\d{8}$")) {
|
|||
// return rb.setMsg("微信号格式不正确");
|
|||
// }
|
|||
// }
|
|||
// String email = dto.getE_mail();
|
|||
// if (StringUtils.isNotBlank(email)) {
|
|||
// if (!email.matches("^[0-9A-Za-z][\\.-_0-9A-Za-z]*@[0-9A-Za-z]+(?:\\.[0-9A-Za-z]+)+$")) {
|
|||
// return rb.setMsg("邮箱格式不正确");
|
|||
// }
|
|||
// }
|
|||
// ResultBean resultBean = crmCustomerTempService.updateCrmCustomer(dto, sid);
|
|||
// if (!resultBean.getSuccess()) {
|
|||
// return rb.setMsg(resultBean.getMsg());
|
|||
// }
|
|||
// return rb.success().setMsg(resultBean.getMsg());
|
|||
// }
|
|||
//
|
|||
// @ApiOperation("删除记录")
|
|||
// @DeleteMapping("/del")
|
|||
// public ResultBean del(@RequestBody String[] sid) {
|
|||
// ResultBean rb = ResultBean.fireFail();
|
|||
// crmCustomerTempService.delBySids(sid);
|
|||
// return rb.success();
|
|||
// }
|
|||
//
|
|||
// @ApiOperation("获取一条记录")
|
|||
// @GetMapping("/fetchSid/{sid}")
|
|||
// public ResultBean<CrmCustomerTempVo> fetchSid(@PathVariable("sid") String sid) {
|
|||
// ResultBean<CrmCustomerTempVo> rb = ResultBean.fireFail();
|
|||
// CrmCustomerTemp customerTemp = crmCustomerTempService.fetchBySid(sid);
|
|||
// if (customerTemp == null) {
|
|||
// return rb.setMsg("该客户不存在");
|
|||
// }
|
|||
// CrmCustomerTempVo vo = crmCustomerTempService.fetchAllBySid(sid);
|
|||
// return rb.success().setData(vo);
|
|||
// }
|
|||
|
|||
|
|||
|
|||
} |
@ -0,0 +1,67 @@ |
|||
package com.yxt.oms.biz.func.crmcustomer; |
|||
|
|||
import cn.hutool.core.bean.BeanUtil; |
|||
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
|
|||
import com.yxt.common.base.config.component.FileUploadComponent; |
|||
import com.yxt.common.base.service.MybatisBaseService; |
|||
import com.yxt.common.base.utils.HanZiConverterPinYin; |
|||
import com.yxt.common.base.utils.HttpStatusEnum; |
|||
import com.yxt.common.base.utils.PagerUtil; |
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import com.yxt.oms.biz.func.sysorganization.SysOrganizationVo; |
|||
import com.yxt.oms.feign.customer.customertemp.CrmCustomerTempFeign; |
|||
import com.yxt.oms.feign.portal.sysuser.SysUserFeign; |
|||
import com.yxt.oms.feign.portal.sysuser.SysUserVo; |
|||
import org.apache.commons.lang3.StringUtils; |
|||
import org.slf4j.Logger; |
|||
import org.slf4j.LoggerFactory; |
|||
import org.springframework.beans.BeanUtils; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.stereotype.Service; |
|||
import org.springframework.transaction.annotation.Transactional; |
|||
|
|||
import javax.annotation.Resource; |
|||
import java.util.*; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户管理) <br/> |
|||
* File: CrmCustomerTempService.java <br/> |
|||
* Class: com.yxt.anrui.crm.biz.crmcustomertemp.CrmCustomerTempService <br/> |
|||
* Description: 潜在客户信息 业务逻辑. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2022-01-12 11:21:17 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Service |
|||
public class CrmCustomerTempService { |
|||
public static final Logger logger = LoggerFactory.getLogger(CrmCustomerTempService.class); |
|||
|
|||
@Autowired |
|||
private FileUploadComponent fileUploadComponent; |
|||
@Resource |
|||
private SysUserFeign sysUserFeign; |
|||
@Autowired |
|||
private CrmCustomerTempFeign crmCustomerTempFeign; |
|||
|
|||
// public ResultBean<PagerVo<CrmCustomerTempVo>> listPageVo(PagerQuery<CrmCustomerTempQuery> pq) {
|
|||
// if (StringUtils.isNotBlank(pq.getParams().getUserSid())) {
|
|||
// String staffSid = sysUserFeign.selectBySid(pq.getParams().getUserSid()).getData().getStaffSid();
|
|||
// pq.getParams().setStaffSid(staffSid);
|
|||
// }
|
|||
// return crmCustomerTempFeign.listPage(pq);
|
|||
// }
|
|||
|
|||
|
|||
public ResultBean<PagerVo<SalesCustomerVo>> chooseCustomerList(PagerQuery<SalesCustomerQuery> pq) { |
|||
ResultBean<PagerVo<SalesCustomerVo>> pagerVoResultBean = crmCustomerTempFeign.chooseCustomerList(pq); |
|||
return pagerVoResultBean; |
|||
} |
|||
} |
@ -0,0 +1,28 @@ |
|||
package com.yxt.oms.biz.func.crmcustomer; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author Fan |
|||
* @description |
|||
* @date 2024/7/24 15:17 |
|||
*/ |
|||
@Data |
|||
public class SalesCustomerQuery implements Query { |
|||
|
|||
private String createOrgSid; |
|||
/** |
|||
* 客户编码 |
|||
*/ |
|||
private String customerNo; |
|||
/** |
|||
* 手机号 |
|||
*/ |
|||
private String mobile; |
|||
/** |
|||
* 客户名称 |
|||
*/ |
|||
private String name; |
|||
|
|||
} |
@ -0,0 +1,59 @@ |
|||
package com.yxt.oms.biz.func.crmcustomer; |
|||
|
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author Fan |
|||
* @description |
|||
* @date 2024/7/24 15:18 |
|||
*/ |
|||
@Data |
|||
public class SalesCustomerVo { |
|||
|
|||
/** |
|||
* 地址 |
|||
*/ |
|||
private String address; |
|||
/** |
|||
* 昵称 |
|||
*/ |
|||
private String buyserNickname; |
|||
/** |
|||
* 市 |
|||
*/ |
|||
private String city; |
|||
/** |
|||
* 县 |
|||
*/ |
|||
private String county; |
|||
/** |
|||
* 客户编码 |
|||
*/ |
|||
private String customerNo; |
|||
/** |
|||
* 手机 |
|||
*/ |
|||
private String mobile; |
|||
/** |
|||
* 客户名称 |
|||
*/ |
|||
private String name; |
|||
/** |
|||
* 平台 |
|||
*/ |
|||
private String originalPlat; |
|||
/** |
|||
* 固话 |
|||
*/ |
|||
private String phone; |
|||
/** |
|||
* 省 |
|||
*/ |
|||
private String province; |
|||
private String sid; |
|||
/** |
|||
* 街道 |
|||
*/ |
|||
private String street; |
|||
|
|||
} |
@ -0,0 +1,21 @@ |
|||
package com.yxt.oms.biz.func.smssalesbill; |
|||
|
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author Fan |
|||
* @description |
|||
* @date 2024/7/24 17:19 |
|||
*/ |
|||
@Data |
|||
public class AccountVo { |
|||
|
|||
@ApiModelProperty("结算账户") |
|||
private String bankAccount; // 结算账户
|
|||
@ApiModelProperty("结算账户") |
|||
private String bankAccountKey; // 结算账户
|
|||
@ApiModelProperty("结算金额") |
|||
private String amount; // 结算金额
|
|||
|
|||
} |
@ -0,0 +1,94 @@ |
|||
package com.yxt.oms.feign.customer.customertemp; |
|||
|
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import com.yxt.oms.biz.func.crmcustomer.*; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import javax.validation.Valid; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户管理) <br/> |
|||
* File: CrmCustomerTempFeign.java <br/> |
|||
* Class: com.yxt.anrui.crm.api.crmcustomertemp.CrmCustomerTempFeign <br/> |
|||
* Description: 潜在客户信息. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2022-01-12 11:21:16 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Api(tags = "潜在客户信息") |
|||
@FeignClient( |
|||
contextId = "ss-common-customer-CrmCustomerTemp", |
|||
name = "ss-common-customer", |
|||
path = "v1/crmcustomertemp" |
|||
) |
|||
public interface CrmCustomerTempFeign { |
|||
|
|||
@ApiOperation("销售订单选择客户") |
|||
@PostMapping("/chooseCustomerList") |
|||
public ResultBean<PagerVo<SalesCustomerVo>> chooseCustomerList(@RequestBody PagerQuery<SalesCustomerQuery> pq); |
|||
|
|||
// /**
|
|||
// * 潜在客户管理的分页查询
|
|||
// *
|
|||
// * @param pq
|
|||
// * @return
|
|||
// */
|
|||
// @ApiOperation("根据条件分页查询数据的列表")
|
|||
// @PostMapping("/listPage")
|
|||
// public ResultBean<PagerVo<CrmCustomerTempVo>> listPage(@RequestBody PagerQuery<CrmCustomerTempQuery> pq);
|
|||
//
|
|||
// /**
|
|||
// * pc端潜在客户新增保存
|
|||
// *
|
|||
// * @param dto 客户信息及运行信息数据传输对象
|
|||
// * @return
|
|||
// */
|
|||
// @ApiOperation("新增保存")
|
|||
// @PostMapping("/save")
|
|||
// public ResultBean save(@Valid @RequestBody CrmCustomerTempDto dto);
|
|||
//
|
|||
// /**
|
|||
// * pc端潜在客户编辑保存
|
|||
// *
|
|||
// * @param dto 数据传输对象
|
|||
// * @param sid 潜在客户sid
|
|||
// * @return
|
|||
// */
|
|||
// @ApiOperation("修改保存")
|
|||
// @PostMapping("/update/{sid}")
|
|||
// public ResultBean update(@RequestBody CrmCustomerTempUpdateDto dto, @PathVariable("sid") String sid);
|
|||
//
|
|||
// /**
|
|||
// * pc潜在客户的批量删除
|
|||
// *
|
|||
// * @param sid
|
|||
// * @return
|
|||
// */
|
|||
// @ApiOperation("删除记录")
|
|||
// @DeleteMapping("/del")
|
|||
// public ResultBean del(@RequestBody String[] sid);
|
|||
//
|
|||
// /**
|
|||
// * pc潜在客户的编辑回显
|
|||
// *
|
|||
// * @param sid
|
|||
// * @return
|
|||
// */
|
|||
// @ApiOperation("获取一条记录")
|
|||
// @GetMapping("/fetchSid/{sid}")
|
|||
// public ResultBean<CrmCustomerTempVo> fetchSid(@PathVariable("sid") String sid);
|
|||
|
|||
|
|||
|
|||
} |
Loading…
Reference in new issue