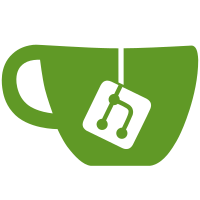
40 changed files with 715 additions and 0 deletions
@ -0,0 +1,34 @@ |
|||
package com.yxt.yyth.api.lpkgiftbag; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableField; |
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
import java.util.UUID; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:06 |
|||
*/ |
|||
@ApiModel(value = "礼包信息", description = "礼包信息") |
|||
@TableName("lpk_giftbag") |
|||
@Data |
|||
public class LpkGiftBag { |
|||
private String id; |
|||
private String sid = UUID.randomUUID().toString(); |
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss", timezone = "GMT+8") |
|||
private Date createTime; |
|||
private String remarks; |
|||
private String isEnable; |
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss", timezone = "GMT+8") |
|||
private Date dateStart; |
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss", timezone = "GMT+8") |
|||
private Date dateEnd; |
|||
private String name; |
|||
private String boundary; |
|||
private String boundaryPrice; |
|||
private String iconUrl; |
|||
} |
@ -0,0 +1,14 @@ |
|||
package com.yxt.yyth.api.lpkgiftbag; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:13 |
|||
*/ |
|||
@ApiModel(value = "礼包信息 数据传输对象", description = "礼包信息 数据传输对象") |
|||
@Data |
|||
public class LpkGiftBagDto implements Dto { |
|||
} |
@ -0,0 +1,15 @@ |
|||
package com.yxt.yyth.api.lpkgiftbag; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:31 |
|||
*/ |
|||
@ApiModel(value = "礼包信息 查询条件", description = "礼包信息 查询条件") |
|||
@Data |
|||
public class LpkGiftBagQuery implements Query { |
|||
|
|||
} |
@ -0,0 +1,17 @@ |
|||
package com.yxt.yyth.api.lpkgiftbag; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
import lombok.NoArgsConstructor; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:12 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "礼包信息 视图数据对象", description = "礼包信息 视图数据对象") |
|||
@NoArgsConstructor |
|||
public class LpkGiftBagVo implements Vo { |
|||
|
|||
} |
@ -0,0 +1,27 @@ |
|||
package com.yxt.yyth.api.lpkgiftbaggoods; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
import java.util.UUID; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:06 |
|||
*/ |
|||
@ApiModel(value = "礼包包含商品信息", description = "礼包包含商品信息") |
|||
@TableName("lpk_giftbag_goods") |
|||
@Data |
|||
public class LpkGiftBagGoods { |
|||
private String id; |
|||
private String sid = UUID.randomUUID().toString(); |
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss", timezone = "GMT+8") |
|||
private Date createTime; |
|||
private String remarks; |
|||
private String giftbagSid; |
|||
private String goodsSid; |
|||
private String goodsNumber; |
|||
} |
@ -0,0 +1,14 @@ |
|||
package com.yxt.yyth.api.lpkgiftbaggoods; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:13 |
|||
*/ |
|||
@ApiModel(value = "礼包包含商品信息 数据传输对象", description = "礼包包含商品信息 数据传输对象") |
|||
@Data |
|||
public class LpkGiftBagGoodsDto implements Dto { |
|||
} |
@ -0,0 +1,15 @@ |
|||
package com.yxt.yyth.api.lpkgiftbaggoods; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:31 |
|||
*/ |
|||
@ApiModel(value = "礼包包含商品信息 查询条件", description = "礼包包含商品信息 查询条件") |
|||
@Data |
|||
public class LpkGiftBagGoodsQuery implements Query { |
|||
|
|||
} |
@ -0,0 +1,17 @@ |
|||
package com.yxt.yyth.api.lpkgiftbaggoods; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
import lombok.NoArgsConstructor; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:12 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "礼包包含商品信息 视图数据对象", description = "礼包包含商品信息 视图数据对象") |
|||
@NoArgsConstructor |
|||
public class LpkGiftBagGoodsVo implements Vo { |
|||
|
|||
} |
@ -0,0 +1,37 @@ |
|||
package com.yxt.yyth.api.lpkgiftcard; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
import java.util.UUID; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:06 |
|||
*/ |
|||
@ApiModel(value = "礼品卡信息", description = "礼品卡信息") |
|||
@TableName("lpk_giftcard") |
|||
@Data |
|||
public class LpkGiftCard { |
|||
private String id; |
|||
private String sid = UUID.randomUUID().toString(); |
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss", timezone = "GMT+8") |
|||
private Date createTime; |
|||
private String remarks; |
|||
private String isEnable; |
|||
private String giftbagSid; |
|||
private String code; |
|||
private String codeKey; |
|||
private String state; |
|||
private String grantName; |
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss", timezone = "GMT+8") |
|||
private Date grantDate; |
|||
private String customerMobile; |
|||
private String customerSid; |
|||
|
|||
|
|||
|
|||
} |
@ -0,0 +1,14 @@ |
|||
package com.yxt.yyth.api.lpkgiftcard; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:13 |
|||
*/ |
|||
@ApiModel(value = "礼品卡信息 数据传输对象", description = "礼品卡信息 数据传输对象") |
|||
@Data |
|||
public class LpkGiftCardDto implements Dto { |
|||
} |
@ -0,0 +1,15 @@ |
|||
package com.yxt.yyth.api.lpkgiftcard; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:31 |
|||
*/ |
|||
@ApiModel(value = "礼品卡信息 查询条件", description = "礼品卡信息 查询条件") |
|||
@Data |
|||
public class LpkGiftCardQuery implements Query { |
|||
|
|||
} |
@ -0,0 +1,17 @@ |
|||
package com.yxt.yyth.api.lpkgiftcard; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
import lombok.NoArgsConstructor; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:12 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "礼品卡信息 视图数据对象", description = "礼品卡信息 视图数据对象") |
|||
@NoArgsConstructor |
|||
public class LpkGiftCardVo implements Vo { |
|||
|
|||
} |
@ -0,0 +1,33 @@ |
|||
package com.yxt.yyth.api.lpkgoods; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
import java.util.UUID; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:06 |
|||
*/ |
|||
@ApiModel(value = "商品信息", description = "商品信息") |
|||
@TableName("lpk_goods") |
|||
@Data |
|||
public class LpkGoods { |
|||
private String id; |
|||
private String sid = UUID.randomUUID().toString(); |
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss", timezone = "GMT+8") |
|||
private Date createTime; |
|||
private String remarks; |
|||
private String isEnable; |
|||
private String code; |
|||
private String barcode; |
|||
private String name; |
|||
private String unitName; |
|||
private String typeCode; |
|||
private String price; |
|||
private String picUrl; |
|||
|
|||
} |
@ -0,0 +1,14 @@ |
|||
package com.yxt.yyth.api.lpkgoods; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:13 |
|||
*/ |
|||
@ApiModel(value = "商品信息 数据传输对象", description = "商品信息 数据传输对象") |
|||
@Data |
|||
public class LpkGoodsDto implements Dto { |
|||
} |
@ -0,0 +1,15 @@ |
|||
package com.yxt.yyth.api.lpkgoods; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:31 |
|||
*/ |
|||
@ApiModel(value = "商品信息 查询条件", description = "商品信息 查询条件") |
|||
@Data |
|||
public class LpkGoodsQuery implements Query { |
|||
|
|||
} |
@ -0,0 +1,17 @@ |
|||
package com.yxt.yyth.api.lpkgoods; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
import lombok.NoArgsConstructor; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:12 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "商品信息 视图数据对象", description = "商品信息 视图数据对象") |
|||
@NoArgsConstructor |
|||
public class LpkGoodsVo implements Vo { |
|||
|
|||
} |
@ -0,0 +1,33 @@ |
|||
package com.yxt.yyth.api.lpkstore; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
import java.util.UUID; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:06 |
|||
*/ |
|||
@ApiModel(value = "取货点(门店)信息", description = "取货点(门店)信息") |
|||
@TableName("lpk_store") |
|||
@Data |
|||
public class LpkStore { |
|||
private String id; |
|||
private String sid = UUID.randomUUID().toString(); |
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss", timezone = "GMT+8") |
|||
private Date createTime; |
|||
private String remarks; |
|||
private String isEnable; |
|||
private String code; |
|||
private String barcode; |
|||
private String name; |
|||
private String unitName; |
|||
private String typeCode; |
|||
private String price; |
|||
private String picUrl; |
|||
|
|||
} |
@ -0,0 +1,14 @@ |
|||
package com.yxt.yyth.api.lpkstore; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:13 |
|||
*/ |
|||
@ApiModel(value = "取货点(门店)信息 数据传输对象", description = "取货点(门店)信息 数据传输对象") |
|||
@Data |
|||
public class LpkStoreDto implements Dto { |
|||
} |
@ -0,0 +1,15 @@ |
|||
package com.yxt.yyth.api.lpkstore; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:31 |
|||
*/ |
|||
@ApiModel(value = "取货点(门店)信息 查询条件", description = "取货点(门店)信息 查询条件") |
|||
@Data |
|||
public class LpkStoreQuery implements Query { |
|||
|
|||
} |
@ -0,0 +1,17 @@ |
|||
package com.yxt.yyth.api.lpkstore; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
import lombok.NoArgsConstructor; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:12 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "取货点(门店)信息 视图数据对象", description = "取货点(门店)信息 视图数据对象") |
|||
@NoArgsConstructor |
|||
public class LpkStoreVo implements Vo { |
|||
|
|||
} |
@ -0,0 +1,21 @@ |
|||
package com.yxt.yyth.biz.lpkgiftbag; |
|||
|
|||
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import com.baomidou.mybatisplus.core.toolkit.Constants; |
|||
import com.yxt.yyth.api.lpkcustomer.LpkCustomer; |
|||
import com.yxt.yyth.api.lpkcustomer.LpkCustomerVo; |
|||
import com.yxt.yyth.api.lpkgiftbag.LpkGiftBag; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
import org.apache.ibatis.annotations.Param; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:03 |
|||
*/ |
|||
@Mapper |
|||
public interface LpkGiftBagMapper extends BaseMapper<LpkGiftBag> { |
|||
|
|||
|
|||
} |
@ -0,0 +1,7 @@ |
|||
<?xml version="1.0" encoding="UTF-8" ?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yyth.biz.lpkgiftbag.LpkGiftBagMapper"> |
|||
<!-- <where> ${ew.sqlSegment} </where>--> |
|||
<!-- ${ew.customSqlSegment} --> |
|||
|
|||
</mapper> |
@ -0,0 +1,30 @@ |
|||
package com.yxt.yyth.biz.lpkgiftbag; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import com.yxt.yyth.api.lpkcustomer.LpkCustomerQuery; |
|||
import com.yxt.yyth.api.lpkcustomer.LpkCustomerVo; |
|||
import com.yxt.yyth.api.lpkcustomer.WxBindMobileDto; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:03 |
|||
*/ |
|||
|
|||
@Api(tags = "礼包信息") |
|||
@RestController |
|||
@RequestMapping("lpkgiftbag") |
|||
public class LpkGiftBagRest { |
|||
|
|||
@Autowired |
|||
LpkGiftBagService lpkGiftBagService; |
|||
|
|||
|
|||
|
|||
|
|||
} |
@ -0,0 +1,15 @@ |
|||
package com.yxt.yyth.biz.lpkgiftbag; |
|||
|
|||
import com.yxt.common.base.service.MybatisBaseService; |
|||
import com.yxt.yyth.api.lpkcustomer.LpkCustomer; |
|||
import com.yxt.yyth.api.lpkgiftbag.LpkGiftBag; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:03 |
|||
*/ |
|||
@Service |
|||
public class LpkGiftBagService extends MybatisBaseService<LpkGiftBagMapper, LpkGiftBag> { |
|||
|
|||
} |
@ -0,0 +1,16 @@ |
|||
package com.yxt.yyth.biz.lpkgiftbaggoods; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.yxt.yyth.api.lpkgiftbag.LpkGiftBag; |
|||
import com.yxt.yyth.api.lpkgiftbaggoods.LpkGiftBagGoods; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:03 |
|||
*/ |
|||
@Mapper |
|||
public interface LpkGiftBagGoodsMapper extends BaseMapper<LpkGiftBagGoods> { |
|||
|
|||
|
|||
} |
@ -0,0 +1,7 @@ |
|||
<?xml version="1.0" encoding="UTF-8" ?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yyth.biz.lpkgiftbaggoods.LpkGiftBagGoodsMapper"> |
|||
<!-- <where> ${ew.sqlSegment} </where>--> |
|||
<!-- ${ew.customSqlSegment} --> |
|||
|
|||
</mapper> |
@ -0,0 +1,24 @@ |
|||
package com.yxt.yyth.biz.lpkgiftbaggoods; |
|||
|
|||
import io.swagger.annotations.Api; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.web.bind.annotation.RequestMapping; |
|||
import org.springframework.web.bind.annotation.RestController; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:03 |
|||
*/ |
|||
|
|||
@Api(tags = "礼包包含商品信息") |
|||
@RestController |
|||
@RequestMapping("lpkgiftbaggoods") |
|||
public class LpkGiftBagGoodsRest { |
|||
|
|||
@Autowired |
|||
LpkGiftBagGoodsService lpkGiftBagGoodsService; |
|||
|
|||
|
|||
|
|||
|
|||
} |
@ -0,0 +1,15 @@ |
|||
package com.yxt.yyth.biz.lpkgiftbaggoods; |
|||
|
|||
import com.yxt.common.base.service.MybatisBaseService; |
|||
import com.yxt.yyth.api.lpkgiftbag.LpkGiftBag; |
|||
import com.yxt.yyth.api.lpkgiftbaggoods.LpkGiftBagGoods; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:03 |
|||
*/ |
|||
@Service |
|||
public class LpkGiftBagGoodsService extends MybatisBaseService<LpkGiftBagGoodsMapper, LpkGiftBagGoods> { |
|||
|
|||
} |
@ -0,0 +1,16 @@ |
|||
package com.yxt.yyth.biz.lpkgiftcard; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.yxt.yyth.api.lpkgiftbag.LpkGiftBag; |
|||
import com.yxt.yyth.api.lpkgiftcard.LpkGiftCard; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:03 |
|||
*/ |
|||
@Mapper |
|||
public interface LpkGiftCardMapper extends BaseMapper<LpkGiftCard> { |
|||
|
|||
|
|||
} |
@ -0,0 +1,7 @@ |
|||
<?xml version="1.0" encoding="UTF-8" ?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yyth.biz.lpkgiftcard.LpkGiftCardMapper"> |
|||
<!-- <where> ${ew.sqlSegment} </where>--> |
|||
<!-- ${ew.customSqlSegment} --> |
|||
|
|||
</mapper> |
@ -0,0 +1,24 @@ |
|||
package com.yxt.yyth.biz.lpkgiftcard; |
|||
|
|||
import io.swagger.annotations.Api; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.web.bind.annotation.RequestMapping; |
|||
import org.springframework.web.bind.annotation.RestController; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:03 |
|||
*/ |
|||
|
|||
@Api(tags = "礼品卡信息") |
|||
@RestController |
|||
@RequestMapping("lpkgiftcard") |
|||
public class LpkGiftCardRest { |
|||
|
|||
@Autowired |
|||
LpkGiftCardService lpkGiftCardService; |
|||
|
|||
|
|||
|
|||
|
|||
} |
@ -0,0 +1,15 @@ |
|||
package com.yxt.yyth.biz.lpkgiftcard; |
|||
|
|||
import com.yxt.common.base.service.MybatisBaseService; |
|||
import com.yxt.yyth.api.lpkgiftbag.LpkGiftBag; |
|||
import com.yxt.yyth.api.lpkgiftcard.LpkGiftCard; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:03 |
|||
*/ |
|||
@Service |
|||
public class LpkGiftCardService extends MybatisBaseService<LpkGiftCardMapper, LpkGiftCard> { |
|||
|
|||
} |
@ -0,0 +1,16 @@ |
|||
package com.yxt.yyth.biz.lpkgoods; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.yxt.yyth.api.lpkgiftbag.LpkGiftBag; |
|||
import com.yxt.yyth.api.lpkgoods.LpkGoods; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:03 |
|||
*/ |
|||
@Mapper |
|||
public interface LpkGoodsMapper extends BaseMapper<LpkGoods> { |
|||
|
|||
|
|||
} |
@ -0,0 +1,7 @@ |
|||
<?xml version="1.0" encoding="UTF-8" ?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yyth.biz.lpkgoods.LpkGoodsMapper"> |
|||
<!-- <where> ${ew.sqlSegment} </where>--> |
|||
<!-- ${ew.customSqlSegment} --> |
|||
|
|||
</mapper> |
@ -0,0 +1,24 @@ |
|||
package com.yxt.yyth.biz.lpkgoods; |
|||
|
|||
import io.swagger.annotations.Api; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.web.bind.annotation.RequestMapping; |
|||
import org.springframework.web.bind.annotation.RestController; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:03 |
|||
*/ |
|||
|
|||
@Api(tags = "商品信息") |
|||
@RestController |
|||
@RequestMapping("lpkgoods") |
|||
public class LpkGoodsRest { |
|||
|
|||
@Autowired |
|||
LpkGoodsService lpkGoodsService; |
|||
|
|||
|
|||
|
|||
|
|||
} |
@ -0,0 +1,15 @@ |
|||
package com.yxt.yyth.biz.lpkgoods; |
|||
|
|||
import com.yxt.common.base.service.MybatisBaseService; |
|||
import com.yxt.yyth.api.lpkgiftbag.LpkGiftBag; |
|||
import com.yxt.yyth.api.lpkgoods.LpkGoods; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:03 |
|||
*/ |
|||
@Service |
|||
public class LpkGoodsService extends MybatisBaseService<LpkGoodsMapper, LpkGoods> { |
|||
|
|||
} |
@ -0,0 +1,16 @@ |
|||
package com.yxt.yyth.biz.lpkstore; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.yxt.yyth.api.lpkgoods.LpkGoods; |
|||
import com.yxt.yyth.api.lpkstore.LpkStore; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:03 |
|||
*/ |
|||
@Mapper |
|||
public interface LpkStoreMapper extends BaseMapper<LpkStore> { |
|||
|
|||
|
|||
} |
@ -0,0 +1,7 @@ |
|||
<?xml version="1.0" encoding="UTF-8" ?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yyth.biz.lpkstore.LpkStoreMapper"> |
|||
<!-- <where> ${ew.sqlSegment} </where>--> |
|||
<!-- ${ew.customSqlSegment} --> |
|||
|
|||
</mapper> |
@ -0,0 +1,24 @@ |
|||
package com.yxt.yyth.biz.lpkstore; |
|||
|
|||
import io.swagger.annotations.Api; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.web.bind.annotation.RequestMapping; |
|||
import org.springframework.web.bind.annotation.RestController; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:03 |
|||
*/ |
|||
|
|||
@Api(tags = "取货点(门店)信息") |
|||
@RestController |
|||
@RequestMapping("lpkstore") |
|||
public class LpkStoreRest { |
|||
|
|||
@Autowired |
|||
LpkStoreService lpkStoreService; |
|||
|
|||
|
|||
|
|||
|
|||
} |
@ -0,0 +1,15 @@ |
|||
package com.yxt.yyth.biz.lpkstore; |
|||
|
|||
import com.yxt.common.base.service.MybatisBaseService; |
|||
import com.yxt.yyth.api.lpkgoods.LpkGoods; |
|||
import com.yxt.yyth.api.lpkstore.LpkStore; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/21 15:03 |
|||
*/ |
|||
@Service |
|||
public class LpkStoreService extends MybatisBaseService<LpkStoreMapper, LpkStore> { |
|||
|
|||
} |
Loading…
Reference in new issue