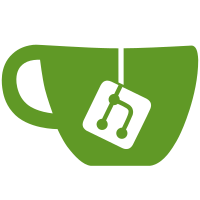
25 changed files with 894 additions and 3 deletions
@ -0,0 +1,33 @@ |
|||
package com.yxt.yythmall.mallplus.mbg.oms.vo; |
|||
|
|||
|
|||
import com.yxt.yythmall.mallplus.mbg.pms.entity.PmsProduct; |
|||
import com.yxt.yythmall.mallplus.mbg.pms.entity.PmsProductAttribute; |
|||
import com.yxt.yythmall.mallplus.mbg.pms.entity.PmsSkuStock; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 购物车中选择规格的商品信息 |
|||
* https://github.com/shenzhuan/mallplus on 2018/8/2.
|
|||
*/ |
|||
public class CartProduct extends PmsProduct { |
|||
private List<PmsProductAttribute> productAttributeList; |
|||
private List<PmsSkuStock> skuStockList; |
|||
|
|||
public List<PmsProductAttribute> getProductAttributeList() { |
|||
return productAttributeList; |
|||
} |
|||
|
|||
public void setProductAttributeList(List<PmsProductAttribute> productAttributeList) { |
|||
this.productAttributeList = productAttributeList; |
|||
} |
|||
|
|||
public List<PmsSkuStock> getSkuStockList() { |
|||
return skuStockList; |
|||
} |
|||
|
|||
public void setSkuStockList(List<PmsSkuStock> skuStockList) { |
|||
this.skuStockList = skuStockList; |
|||
} |
|||
} |
@ -0,0 +1,17 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.CmsPrefrenceAreaProductRelationMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.CmsPrefrenceAreaProductRelation"> |
|||
<id column="id" property="id"/> |
|||
<result column="prefrence_area_id" property="prefrenceAreaId"/> |
|||
<result column="product_id" property="productId"/> |
|||
</resultMap> |
|||
|
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, prefrence_area_id, product_id |
|||
</sql> |
|||
|
|||
</mapper> |
@ -0,0 +1,17 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.CmsSubjectProductRelationMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.CmsSubjectProductRelation"> |
|||
<id column="id" property="id"/> |
|||
<result column="subject_id" property="subjectId"/> |
|||
<result column="product_id" property="productId"/> |
|||
</resultMap> |
|||
|
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, subject_id, product_id |
|||
</sql> |
|||
|
|||
</mapper> |
@ -0,0 +1,17 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsAlbumMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsAlbum"> |
|||
<id column="id" property="id"/> |
|||
<result column="name" property="name"/> |
|||
<result column="pic" property="pic"/> |
|||
<result column="type" property="type"/> |
|||
<result column="sort" property="sort"/> |
|||
<result column="description" property="description"/> |
|||
<result column="store_id" property="storeId"/> |
|||
</resultMap> |
|||
|
|||
|
|||
</mapper> |
@ -0,0 +1,17 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsAlbumPicMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsAlbumPic"> |
|||
<id column="id" property="id"/> |
|||
<result column="album_id" property="albumId"/> |
|||
<result column="pic" property="pic"/> |
|||
</resultMap> |
|||
|
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, album_id, pic |
|||
</sql> |
|||
|
|||
</mapper> |
@ -0,0 +1,26 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsBrandMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsBrand"> |
|||
<id column="id" property="id"/> |
|||
<result column="name" property="name"/> |
|||
<result column="first_letter" property="firstLetter"/> |
|||
<result column="sort" property="sort"/> |
|||
<result column="factory_status" property="factoryStatus"/> |
|||
<result column="show_status" property="showStatus"/> |
|||
<result column="product_count" property="productCount"/> |
|||
<result column="product_comment_count" property="productCommentCount"/> |
|||
<result column="logo" property="logo"/> |
|||
<result column="big_pic" property="bigPic"/> |
|||
<result column="brand_story" property="brandStory"/> |
|||
</resultMap> |
|||
|
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, name, first_letter, sort, factory_status, show_status, product_count, product_comment_count, logo, big_pic, |
|||
brand_story |
|||
</sql> |
|||
|
|||
</mapper> |
@ -0,0 +1,35 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsCommentMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsComment"> |
|||
<id column="id" property="id"/> |
|||
<result column="product_id" property="productId"/> |
|||
<result column="member_nick_name" property="memberNickName"/> |
|||
<result column="product_name" property="productName"/> |
|||
<result column="star" property="star"/> |
|||
<result column="member_ip" property="memberIp"/> |
|||
<result column="create_time" property="createTime"/> |
|||
<result column="show_status" property="showStatus"/> |
|||
<result column="product_attribute" property="productAttribute"/> |
|||
<result column="collect_couont" property="collectCouont"/> |
|||
<result column="read_count" property="readCount"/> |
|||
<result column="content" property="content"/> |
|||
<result column="pics" property="pics"/> |
|||
<result column="member_icon" property="memberIcon"/> |
|||
<result column="replay_count" property="replayCount"/> |
|||
</resultMap> |
|||
|
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, product_id, member_nick_name, product_name, star, member_ip, create_time, show_status, product_attribute, |
|||
collect_couont, read_count, content, pics, member_icon, replay_count |
|||
</sql> |
|||
<select id="getByProductId" resultType="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsComment"> |
|||
select id, product_id, member_nick_name, product_name, star, member_ip, create_time, show_status, |
|||
product_attribute, collect_couont, read_count, content, pics, member_icon, replay_count |
|||
from pms_comment where product_id = #{productId} |
|||
</select> |
|||
|
|||
</mapper> |
@ -0,0 +1,21 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsCommentReplayMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsCommentReplay"> |
|||
<id column="id" property="id"/> |
|||
<result column="comment_id" property="commentId"/> |
|||
<result column="member_nick_name" property="memberNickName"/> |
|||
<result column="member_icon" property="memberIcon"/> |
|||
<result column="content" property="content"/> |
|||
<result column="create_time" property="createTime"/> |
|||
<result column="type" property="type"/> |
|||
</resultMap> |
|||
|
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, comment_id, member_nick_name, member_icon, content, create_time, type |
|||
</sql> |
|||
|
|||
</mapper> |
@ -0,0 +1,24 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsFavoriteMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsFavorite"> |
|||
<id column="id" property="id"/> |
|||
<result column="addTime" property="addTime"/> |
|||
<result column="type" property="type"/> |
|||
<result column="obj_id" property="objId"/> |
|||
<result column="store_id" property="storeId"/> |
|||
<result column="user_id" property="userId"/> |
|||
<result column="name" property="name"/> |
|||
<result column="meno1" property="meno1"/> |
|||
<result column="meno2" property="meno2"/> |
|||
<result column="meno3" property="meno3"/> |
|||
</resultMap> |
|||
|
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, addTime, type, obj_id, store_id, user_id, name, meno1, meno2, meno3 |
|||
</sql> |
|||
|
|||
</mapper> |
@ -0,0 +1,22 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsFeightTemplateMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsFeightTemplate"> |
|||
<id column="id" property="id"/> |
|||
<result column="name" property="name"/> |
|||
<result column="charge_type" property="chargeType"/> |
|||
<result column="first_weight" property="firstWeight"/> |
|||
<result column="first_fee" property="firstFee"/> |
|||
<result column="continue_weight" property="continueWeight"/> |
|||
<result column="continme_fee" property="continmeFee"/> |
|||
<result column="dest" property="dest"/> |
|||
</resultMap> |
|||
|
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, name, charge_type, first_weight, first_fee, continue_weight, continme_fee, dest |
|||
</sql> |
|||
|
|||
</mapper> |
@ -0,0 +1,19 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsMemberPriceMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsMemberPrice"> |
|||
<id column="id" property="id"/> |
|||
<result column="product_id" property="productId"/> |
|||
<result column="member_level_id" property="memberLevelId"/> |
|||
<result column="member_price" property="memberPrice"/> |
|||
<result column="member_level_name" property="memberLevelName"/> |
|||
</resultMap> |
|||
|
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, product_id, member_level_id, member_price, member_level_name |
|||
</sql> |
|||
|
|||
</mapper> |
@ -0,0 +1,35 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsProductAttributeCategoryMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsProductAttributeCategory"> |
|||
<id column="id" property="id"/> |
|||
<result column="name" property="name"/> |
|||
<result column="attribute_count" property="attributeCount"/> |
|||
<result column="param_count" property="paramCount"/> |
|||
</resultMap> |
|||
|
|||
<resultMap id="getListWithAttrMap" type="com.yxt.yythmall.mallplus.mbg.pms.vo.PmsProductAttributeCategoryItem" |
|||
extends="BaseResultMap"> |
|||
<collection property="productAttributeList" columnPrefix="attr_" |
|||
resultMap="BaseResultMap"> |
|||
</collection> |
|||
</resultMap> |
|||
<select id="getListWithAttr" resultMap="getListWithAttrMap"> |
|||
SELECT |
|||
pac.id, |
|||
pac.name, |
|||
pa.id attr_id, |
|||
pa.name attr_name |
|||
FROM |
|||
pms_product_attribute_category pac |
|||
LEFT JOIN pms_product_attribute pa ON pac.id = pa.product_attribute_category_id |
|||
AND pa.type=1; |
|||
</select> |
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, name, attribute_count, param_count |
|||
</sql> |
|||
|
|||
</mapper> |
@ -0,0 +1,44 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsProductAttributeMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsProductAttribute"> |
|||
<id column="id" property="id"/> |
|||
<result column="product_attribute_category_id" property="productAttributeCategoryId"/> |
|||
<result column="name" property="name"/> |
|||
<result column="select_type" property="selectType"/> |
|||
<result column="input_type" property="inputType"/> |
|||
<result column="input_list" property="inputList"/> |
|||
<result column="sort" property="sort"/> |
|||
<result column="filter_type" property="filterType"/> |
|||
<result column="search_type" property="searchType"/> |
|||
<result column="related_status" property="relatedStatus"/> |
|||
<result column="hand_add_status" property="handAddStatus"/> |
|||
<result column="type" property="type"/> |
|||
</resultMap> |
|||
|
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, product_attribute_category_id, name, select_type, input_type, input_list, sort, filter_type, search_type, |
|||
related_status, hand_add_status, type |
|||
</sql> |
|||
|
|||
<select id="getProductAttrInfo" resultType="com.yxt.yythmall.mallplus.mbg.pms.vo.ProductAttrInfo"> |
|||
SELECT |
|||
pa.id attributeId, |
|||
pac.id attributeCategoryId |
|||
FROM |
|||
pms_product_category_attribute_relation pcar |
|||
LEFT JOIN pms_product_attribute pa ON pa.id = pcar.product_attribute_id |
|||
LEFT JOIN pms_product_attribute_category pac ON pa.product_attribute_category_id = pac.id |
|||
WHERE |
|||
pcar.product_category_id = #{productCategoryId} |
|||
</select> |
|||
|
|||
<select id="getProductAttrById" resultType="com.yxt.yythmall.mallplus.mbg.pms.vo.PmsProductAttr"> |
|||
select pa.* from pms_product_attribute_value pv |
|||
LEFT JOIN pms_product_attribute pa ON pv.product_attribute_id = pa.id |
|||
WHERE pv.product_id = #{productId} and pa.type = #{type} |
|||
</select> |
|||
</mapper> |
@ -0,0 +1,18 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsProductAttributeValueMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsProductAttributeValue"> |
|||
<id column="id" property="id"/> |
|||
<result column="product_id" property="productId"/> |
|||
<result column="product_attribute_id" property="productAttributeId"/> |
|||
<result column="value" property="value"/> |
|||
</resultMap> |
|||
|
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, product_id, product_attribute_id, value |
|||
</sql> |
|||
|
|||
</mapper> |
@ -0,0 +1,17 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsProductCategoryAttributeRelationMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsProductCategoryAttributeRelation"> |
|||
<id column="id" property="id"/> |
|||
<result column="product_category_id" property="productCategoryId"/> |
|||
<result column="product_attribute_id" property="productAttributeId"/> |
|||
</resultMap> |
|||
|
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, product_category_id, product_attribute_id |
|||
</sql> |
|||
|
|||
</mapper> |
@ -0,0 +1,50 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsProductCategoryMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsProductCategory"> |
|||
<id column="id" property="id"/> |
|||
<result column="parent_id" property="parentId"/> |
|||
<result column="name" property="name"/> |
|||
<result column="level" property="level"/> |
|||
<result column="product_count" property="productCount"/> |
|||
<result column="product_unit" property="productUnit"/> |
|||
<result column="nav_status" property="navStatus"/> |
|||
<result column="show_status" property="showStatus"/> |
|||
<result column="index_status" property="indexStatus"/> |
|||
<result column="sort" property="sort"/> |
|||
<result column="icon" property="icon"/> |
|||
<result column="keywords" property="keywords"/> |
|||
<result column="description" property="description"/> |
|||
</resultMap> |
|||
|
|||
<resultMap id="listWithChildrenMap" type="com.yxt.yythmall.mallplus.mbg.pms.vo.PmsProductCategoryWithChildrenItem" |
|||
extends="BaseResultMap"> |
|||
<collection property="children" resultMap="BaseResultMap" |
|||
columnPrefix="child_"></collection> |
|||
</resultMap> |
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, parent_id, name, level, product_count, product_unit, nav_status, show_status, index_status,sort, icon, |
|||
keywords, description |
|||
</sql> |
|||
|
|||
<select id="listWithChildren" resultMap="listWithChildrenMap"> |
|||
select |
|||
c1.id, |
|||
c1.name, |
|||
c2.id child_id, |
|||
c2.name child_name |
|||
from pms_product_category c1 left join pms_product_category c2 on c1.id = c2.parent_id |
|||
where c1.parent_id = 0 |
|||
</select> |
|||
|
|||
<select id="selectCountByNameAndLevel" resultType="int"> |
|||
select count(*) from pms_product_category where parent_id = #{parentId} and name = #{name} |
|||
</select> |
|||
|
|||
<select id="selectCountByNameAndLevelId" resultType="int"> |
|||
select count(*) from pms_product_category where parent_id = #{parentId} and name = #{name} and id <> #{id} |
|||
</select> |
|||
</mapper> |
@ -0,0 +1,28 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsProductConsultMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsProductConsult"> |
|||
<id column="id" property="id"/> |
|||
<result column="goods_id" property="goodsId"/> |
|||
<result column="goods_name" property="goodsName"/> |
|||
<result column="member_id" property="memberId"/> |
|||
<result column="member_name" property="memberName"/> |
|||
<result column="store_id" property="storeId"/> |
|||
<result column="email" property="email"/> |
|||
<result column="consult_content" property="consultContent"/> |
|||
<result column="consult_addtime" property="consultAddtime"/> |
|||
<result column="consult_reply" property="consultReply"/> |
|||
<result column="consult_reply_time" property="consultReplyTime"/> |
|||
<result column="isanonymous" property="isanonymous"/> |
|||
<result column="is_del" property="isDel"/> |
|||
</resultMap> |
|||
|
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, goods_id, goods_name, member_id, member_name, store_id, email, consult_content, consult_addtime, |
|||
consult_reply, consult_reply_time, isanonymous, is_del |
|||
</sql> |
|||
|
|||
</mapper> |
@ -0,0 +1,18 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsProductFullReductionMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsProductFullReduction"> |
|||
<id column="id" property="id"/> |
|||
<result column="product_id" property="productId"/> |
|||
<result column="full_price" property="fullPrice"/> |
|||
<result column="reduce_price" property="reducePrice"/> |
|||
</resultMap> |
|||
|
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, product_id, full_price, reduce_price |
|||
</sql> |
|||
|
|||
</mapper> |
@ -0,0 +1,19 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsProductLadderMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsProductLadder"> |
|||
<id column="id" property="id"/> |
|||
<result column="product_id" property="productId"/> |
|||
<result column="count" property="count"/> |
|||
<result column="discount" property="discount"/> |
|||
<result column="price" property="price"/> |
|||
</resultMap> |
|||
|
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, product_id, count, discount, price |
|||
</sql> |
|||
|
|||
</mapper> |
@ -0,0 +1,229 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsProductMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsProduct"> |
|||
<id column="id" property="id"/> |
|||
<result column="brand_id" property="brandId"/> |
|||
<result column="product_category_id" property="productCategoryId"/> |
|||
<result column="feight_template_id" property="feightTemplateId"/> |
|||
<result column="product_attribute_category_id" property="productAttributeCategoryId"/> |
|||
<result column="name" property="name"/> |
|||
<result column="pic" property="pic"/> |
|||
<result column="product_sn" property="productSn"/> |
|||
<result column="delete_status" property="deleteStatus"/> |
|||
<result column="publish_status" property="publishStatus"/> |
|||
<result column="new_status" property="newStatus"/> |
|||
<result column="recommand_status" property="recommandStatus"/> |
|||
<result column="verify_status" property="verifyStatus"/> |
|||
<result column="sort" property="sort"/> |
|||
<result column="sale" property="sale"/> |
|||
<result column="price" property="price"/> |
|||
<result column="promotion_price" property="promotionPrice"/> |
|||
<result column="gift_growth" property="giftGrowth"/> |
|||
<result column="gift_point" property="giftPoint"/> |
|||
<result column="use_point_limit" property="usePointLimit"/> |
|||
<result column="sub_title" property="subTitle"/> |
|||
<result column="description" property="description"/> |
|||
<result column="original_price" property="originalPrice"/> |
|||
<result column="stock" property="stock"/> |
|||
<result column="low_stock" property="lowStock"/> |
|||
<result column="unit" property="unit"/> |
|||
<result column="weight" property="weight"/> |
|||
<result column="preview_status" property="previewStatus"/> |
|||
<result column="service_ids" property="serviceIds"/> |
|||
<result column="keywords" property="keywords"/> |
|||
<result column="note" property="note"/> |
|||
<result column="album_pics" property="albumPics"/> |
|||
<result column="detail_title" property="detailTitle"/> |
|||
<result column="detail_desc" property="detailDesc"/> |
|||
<result column="detail_html" property="detailHtml"/> |
|||
<result column="detail_mobile_html" property="detailMobileHtml"/> |
|||
<result column="promotion_start_time" property="promotionStartTime"/> |
|||
<result column="promotion_end_time" property="promotionEndTime"/> |
|||
<result column="promotion_per_limit" property="promotionPerLimit"/> |
|||
<result column="promotion_type" property="promotionType"/> |
|||
<result column="brand_name" property="brandName"/> |
|||
<result column="product_category_name" property="productCategoryName"/> |
|||
<result column="supply_id" property="supplyId"/> |
|||
<result column="create_time" property="createTime"/> |
|||
<result column="school_name" property="schoolName"/> |
|||
<result column="area_id" property="areaId"/> |
|||
<result column="area_name" property="areaName"/> |
|||
<result column="school_id" property="schoolId"/> |
|||
<result column="transfee" property="transfee"/> |
|||
<result column="type" property="type"/> |
|||
<result column="store_name" property="storeName"/> |
|||
<result column="tags" property="tags"/> |
|||
<result column="store_class_id" property="storeClassId"/> |
|||
|
|||
</resultMap> |
|||
|
|||
<resultMap id="cartProductMap" type="com.yxt.yythmall.mallplus.mbg.oms.vo.CartProduct" autoMapping="true"> |
|||
<id column="id" jdbcType="BIGINT" property="id"/> |
|||
<collection property="productAttributeList" columnPrefix="attr_" |
|||
resultMap="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsProductAttributeMapper.BaseResultMap"> |
|||
</collection> |
|||
<collection property="skuStockList" columnPrefix="sku_" |
|||
resultMap="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsSkuStockMapper.BaseResultMap"> |
|||
</collection> |
|||
</resultMap> |
|||
<resultMap id="promotionProductMap" type="com.yxt.yythmall.mallplus.mbg.pms.vo.PromotionProduct" |
|||
extends="BaseResultMap"> |
|||
<id column="id" jdbcType="BIGINT" property="id"/> |
|||
<collection property="skuStockList" columnPrefix="sku_" |
|||
resultMap="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsSkuStockMapper.BaseResultMap"> |
|||
</collection> |
|||
<collection property="productLadderList" columnPrefix="ladder_" |
|||
resultMap="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsProductLadderMapper.BaseResultMap"> |
|||
</collection> |
|||
<collection property="productFullReductionList" columnPrefix="full_" |
|||
resultMap="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsProductFullReductionMapper.BaseResultMap"> |
|||
</collection> |
|||
</resultMap> |
|||
|
|||
<resultMap id="updateInfoMap" type="com.yxt.yythmall.mallplus.mbg.pms.vo.PmsProductResult" |
|||
extends="BaseResultMap"> |
|||
<result column="cateParentId" jdbcType="BIGINT" property="cateParentId"/> |
|||
<collection property="productLadderList" columnPrefix="ladder_" |
|||
resultMap="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsProductLadderMapper.BaseResultMap"> |
|||
</collection> |
|||
<collection property="productFullReductionList" columnPrefix="full_" |
|||
resultMap="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsProductFullReductionMapper.BaseResultMap"> |
|||
</collection> |
|||
<collection property="memberPriceList" columnPrefix="member_" |
|||
resultMap="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsMemberPriceMapper.BaseResultMap"> |
|||
</collection> |
|||
<collection property="skuStockList" columnPrefix="sku_" |
|||
resultMap="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsSkuStockMapper.BaseResultMap"> |
|||
</collection> |
|||
<collection property="productAttributeValueList" columnPrefix="attribute_" |
|||
resultMap="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsProductAttributeValueMapper.BaseResultMap"> |
|||
</collection> |
|||
<collection property="subjectProductRelationList" column="{productId=id}" |
|||
select="selectSubjectProductRelationByProductId"/> |
|||
<collection property="prefrenceAreaProductRelationList" column="{productId=id}" |
|||
select="selectPrefrenceAreaProductRelationByProductId"/> |
|||
|
|||
</resultMap> |
|||
|
|||
<select id="getUpdateInfo" resultMap="updateInfoMap"> |
|||
SELECT *, |
|||
pc.parent_id cateParentId, |
|||
l.id ladder_id,l.product_id ladder_product_id,l.discount ladder_discount,l.count ladder_count,l.price |
|||
ladder_price, |
|||
pf.id full_id,pf.product_id full_product_id,pf.full_price full_full_price,pf.reduce_price full_reduce_price, |
|||
m.id member_id,m.product_id member_product_id,m.member_level_id member_member_level_id,m.member_price |
|||
member_member_price,m.member_level_name member_member_level_name, |
|||
s.id sku_id,s.product_id sku_product_id,s.price sku_price,s.low_stock sku_low_stock,s.pic sku_pic,s.sale |
|||
sku_sale,s.sku_code sku_sku_code,s.sp1 sku_sp1,s.sp2 sku_sp2,s.sp3 sku_sp3,s.stock sku_stock,s.lock_stock |
|||
sku_lock_stock, |
|||
a.id attribute_id,a.product_id attribute_product_id,a.product_attribute_id |
|||
attribute_product_attribute_id,a.value attribute_value |
|||
FROM pms_product p |
|||
LEFT JOIN pms_product_category pc on pc.id = p.product_category_id |
|||
LEFT JOIN pms_product_ladder l ON p.id = l.product_id |
|||
LEFT JOIN pms_product_full_reduction pf ON pf.product_id=p.id |
|||
LEFT JOIN pms_member_price m ON m.product_id = p.id |
|||
LEFT JOIN pms_sku_stock s ON s.product_id = p.id |
|||
LEFT JOIN pms_product_attribute_value a ON a.product_id=p.id |
|||
WHERE p.id=#{id} |
|||
</select> |
|||
<select id="selectSubjectProductRelationByProductId" |
|||
resultMap="com.yxt.yythmall.mallplus.mbg.pms.mapper.CmsSubjectProductRelationMapper.BaseResultMap"> |
|||
select * from cms_subject_product_relation where product_id=#{productId} |
|||
</select> |
|||
<select id="selectPrefrenceAreaProductRelationByProductId" |
|||
resultMap="com.yxt.yythmall.mallplus.mbg.pms.mapper.CmsPrefrenceAreaProductRelationMapper.BaseResultMap"> |
|||
select * from cms_prefrence_area_product_relation where product_id=#{productId} |
|||
</select> |
|||
|
|||
<select id="listByDate" resultMap="BaseResultMap"> |
|||
select publish_status,stock from pms_product where |
|||
<if test="type==1"> |
|||
date_format(create_time,'%y%m%d') = date_format(#{date},'%y%m%d') |
|||
</if> |
|||
<if test="type==2"> |
|||
date_format(create_time,'%y%m') = date_format(#{date},'%y%m') |
|||
</if> |
|||
</select> |
|||
|
|||
<select id="getCartProduct" resultMap="cartProductMap"> |
|||
SELECT |
|||
p.id id, |
|||
p.`name` name, |
|||
p.sub_title subTitle, |
|||
p.price price, |
|||
p.pic pic, |
|||
p.product_attribute_category_id productAttributeCategoryId, |
|||
p.stock stock, |
|||
pa.id attr_id, |
|||
pa.`name` attr_name, |
|||
ps.id sku_id, |
|||
ps.sku_code sku_code, |
|||
ps.price sku_price, |
|||
ps.sp1 sku_sp1, |
|||
ps.sp2 sku_sp2, |
|||
ps.sp3 sku_sp3, |
|||
ps.stock sku_stock, |
|||
ps.pic sku_pic |
|||
FROM |
|||
pms_product p |
|||
LEFT JOIN pms_product_attribute pa ON p.product_attribute_category_id = pa.product_attribute_category_id |
|||
LEFT JOIN pms_sku_stock ps ON p.id=ps.product_id |
|||
WHERE |
|||
p.id = #{id} |
|||
AND pa.type = 0 |
|||
ORDER BY pa.sort desc |
|||
</select> |
|||
<select id="getPromotionProductList" resultMap="promotionProductMap"> |
|||
SELECT |
|||
p.id, |
|||
p.`name`, |
|||
p.promotion_type, |
|||
p.gift_growth, |
|||
p.gift_point, |
|||
sku.id sku_id, |
|||
sku.price sku_price, |
|||
sku.sku_code sku_sku_code, |
|||
sku.promotion_price sku_promotion_price, |
|||
sku.stock sku_stock, |
|||
sku.lock_stock sku_lock_stock, |
|||
ladder.id ladder_id, |
|||
ladder.count ladder_count, |
|||
ladder.discount ladder_discount, |
|||
full_re.id full_id, |
|||
full_re.full_price full_full_price, |
|||
full_re.reduce_price full_reduce_price |
|||
FROM |
|||
pms_product p |
|||
LEFT JOIN pms_sku_stock sku ON p.id = sku.product_id |
|||
LEFT JOIN pms_product_ladder ladder ON p.id = ladder.product_id |
|||
LEFT JOIN pms_product_full_reduction full_re ON p.id = full_re.product_id |
|||
WHERE |
|||
p.id IN |
|||
<foreach collection="ids" open="(" close=")" item="id" separator=","> |
|||
#{id} |
|||
</foreach> |
|||
</select> |
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, brand_id, product_category_id, feight_template_id, product_attribute_category_id, name, pic, product_sn, |
|||
delete_status, publish_status, new_status, recommand_status, verify_status, sort, sale, price, promotion_price, |
|||
gift_growth, gift_point, use_point_limit, sub_title, description, original_price, stock, low_stock, unit, |
|||
weight, preview_status, service_ids, keywords, note, album_pics, detail_title, detail_desc, detail_html, |
|||
detail_mobile_html, promotion_start_time, promotion_end_time, promotion_per_limit, promotion_type, brand_name, |
|||
product_category_name, supply_id, create_time, school_id |
|||
</sql> |
|||
|
|||
<select id="countGoodsByToday" resultType="java.lang.Integer"> |
|||
select count(*) from pms_product where member_id = #{id} |
|||
and DATE_FORMAT(create_time,'%Y-%m-%d') = DATE_FORMAT(now(),'%Y-%m-%d') |
|||
|
|||
</select> |
|||
<select id="selectByTags" resultMap="BaseResultMap"> |
|||
select id, brand_id, product_category_id, product_attribute_category_id, name,area_id, pic , sale, price, original_price , |
|||
weight from pms_product where find_in_set(#{tags}, tags) and publish_status=1 and verify_status=1 |
|||
</select> |
|||
</mapper> |
@ -0,0 +1,27 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsProductOperateLogMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsProductOperateLog"> |
|||
<id column="id" property="id"/> |
|||
<result column="product_id" property="productId"/> |
|||
<result column="price_old" property="priceOld"/> |
|||
<result column="price_new" property="priceNew"/> |
|||
<result column="sale_price_old" property="salePriceOld"/> |
|||
<result column="sale_price_new" property="salePriceNew"/> |
|||
<result column="gift_point_old" property="giftPointOld"/> |
|||
<result column="gift_point_new" property="giftPointNew"/> |
|||
<result column="use_point_limit_old" property="usePointLimitOld"/> |
|||
<result column="use_point_limit_new" property="usePointLimitNew"/> |
|||
<result column="operate_man" property="operateMan"/> |
|||
<result column="create_time" property="createTime"/> |
|||
</resultMap> |
|||
|
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, product_id, price_old, price_new, sale_price_old, sale_price_new, gift_point_old, gift_point_new, |
|||
use_point_limit_old, use_point_limit_new, operate_man, create_time |
|||
</sql> |
|||
|
|||
</mapper> |
@ -0,0 +1,20 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsProductVertifyRecordMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsProductVertifyRecord"> |
|||
<id column="id" property="id"/> |
|||
<result column="product_id" property="productId"/> |
|||
<result column="create_time" property="createTime"/> |
|||
<result column="vertify_man" property="vertifyMan"/> |
|||
<result column="status" property="status"/> |
|||
<result column="detail" property="detail"/> |
|||
</resultMap> |
|||
|
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, product_id, create_time, vertify_man, status, detail |
|||
</sql> |
|||
|
|||
</mapper> |
@ -0,0 +1,43 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsSkuStockMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsSkuStock"> |
|||
<id column="id" property="id"/> |
|||
<result column="product_id" property="productId"/> |
|||
<result column="sku_code" property="skuCode"/> |
|||
<result column="price" property="price"/> |
|||
<result column="stock" property="stock"/> |
|||
<result column="low_stock" property="lowStock"/> |
|||
<result column="sp1" property="sp1"/> |
|||
<result column="sp2" property="sp2"/> |
|||
<result column="sp3" property="sp3"/> |
|||
<result column="pic" property="pic"/> |
|||
<result column="sale" property="sale"/> |
|||
<result column="promotion_price" property="promotionPrice"/> |
|||
<result column="lock_stock" property="lockStock"/> |
|||
</resultMap> |
|||
|
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, product_id, sku_code, price, stock, low_stock, sp1, sp2, sp3, pic, sale, promotion_price, lock_stock |
|||
</sql> |
|||
|
|||
<insert id="replaceList"> |
|||
REPLACE INTO pms_sku_stock (id,product_id, sku_code, price, stock, low_stock, sp1, sp2, sp3, pic, sale) VALUES |
|||
<foreach collection="list" item="item" index="index" separator=","> |
|||
(#{item.id,jdbcType=BIGINT}, |
|||
#{item.productId,jdbcType=BIGINT}, |
|||
#{item.skuCode,jdbcType=VARCHAR}, |
|||
#{item.price,jdbcType=DECIMAL}, |
|||
#{item.stock,jdbcType=INTEGER}, |
|||
#{item.lowStock,jdbcType=INTEGER}, |
|||
#{item.sp1,jdbcType=VARCHAR}, |
|||
#{item.sp2,jdbcType=VARCHAR}, |
|||
#{item.sp3,jdbcType=VARCHAR}, |
|||
#{item.pic,jdbcType=VARCHAR}, |
|||
#{item.sale,jdbcType=INTEGER}) |
|||
</foreach> |
|||
</insert> |
|||
</mapper> |
@ -0,0 +1,93 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
|
|||
<mapper namespace="com.yxt.yythmall.mallplus.mbg.pms.mapper.PmsSmallNaviconCategoryMapper"> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsSmallNaviconCategory"> |
|||
<id column="id" jdbcType="BIGINT" property="id"/> |
|||
<result column="title" property="title"/> |
|||
<result column="icon" property="icon"/> |
|||
<result column="summary" property="summary"/> |
|||
<result column="content" property="content"/> |
|||
<result column="sort" property="sort"/> |
|||
</resultMap> |
|||
|
|||
<sql id="Base_Column_List"> |
|||
`id`,`title`,`icon`,`summary`,`content`,`sort` |
|||
</sql> |
|||
|
|||
<select id="get" resultMap="BaseResultMap"> |
|||
select |
|||
<include refid="Base_Column_List"/> |
|||
from pms_small_navicon_category where id = #{value} |
|||
</select> |
|||
|
|||
<select id="list" resultMap="BaseResultMap"> |
|||
select |
|||
<include refid="Base_Column_List"/> |
|||
from pms_small_navicon_category |
|||
<where> |
|||
<if test="id != null and id != ''">and id = #{id}</if> |
|||
<if test="title != null and title != ''">and title = #{title}</if> |
|||
<if test="icon != null and icon != ''">and icon = #{icon}</if> |
|||
<if test="summary != null and summary != ''">and summary = #{summary}</if> |
|||
<if test="content != null and content != ''">and content = #{content}</if> |
|||
<if test="sort != null and sort != ''">and sort = #{sort}</if> |
|||
</where> |
|||
|
|||
</select> |
|||
<select id="count" resultType="int"> |
|||
select count(*) from pms_small_navicon_category |
|||
<where> |
|||
<if test="id != null and id != ''">and id = #{id}</if> |
|||
<if test="title != null and title != ''">and title = #{title}</if> |
|||
<if test="icon != null and icon != ''">and icon = #{icon}</if> |
|||
<if test="summary != null and summary != ''">and summary = #{summary}</if> |
|||
<if test="content != null and content != ''">and content = #{content}</if> |
|||
<if test="sort != null and sort != ''">and sort = #{sort}</if> |
|||
</where> |
|||
</select> |
|||
|
|||
<insert id="save" parameterType="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsSmallNaviconCategory" useGeneratedKeys="true" |
|||
keyProperty="id"> |
|||
insert into pms_small_navicon_category |
|||
( |
|||
`title`, |
|||
`icon`, |
|||
`summary`, |
|||
`content`, |
|||
`sort` |
|||
) |
|||
values |
|||
( |
|||
#{title}, |
|||
#{icon}, |
|||
#{summary}, |
|||
#{content}, |
|||
#{sort} |
|||
) |
|||
</insert> |
|||
|
|||
<update id="update" parameterType="com.yxt.yythmall.mallplus.mbg.pms.entity.PmsSmallNaviconCategory"> |
|||
update pms_small_navicon_category |
|||
<set> |
|||
<if test="title != null">`title` = #{title},</if> |
|||
<if test="icon != null">`icon` = #{icon},</if> |
|||
<if test="summary != null">`summary` = #{summary},</if> |
|||
<if test="content != null">`content` = #{content},</if> |
|||
<if test="sort != null">`sort` = #{sort}</if> |
|||
</set> |
|||
where id = #{id} |
|||
</update> |
|||
|
|||
<delete id="remove"> |
|||
delete from pms_small_navicon_category where id = #{value} |
|||
</delete> |
|||
|
|||
<delete id="batchRemove"> |
|||
delete from pms_small_navicon_category where id in |
|||
<foreach item="id" collection="array" open="(" separator="," close=")"> |
|||
#{id} |
|||
</foreach> |
|||
</delete> |
|||
|
|||
</mapper> |
Loading…
Reference in new issue