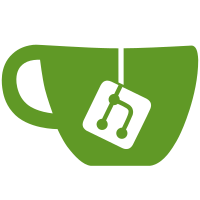
29 changed files with 1560 additions and 0 deletions
@ -0,0 +1,120 @@ |
|||
package com.yxt.yythmall.api.sms.entity; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.IdType; |
|||
import com.baomidou.mybatisplus.annotation.TableField; |
|||
import com.baomidou.mybatisplus.annotation.TableId; |
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.zscat.mallplus.utils.BaseEntity; |
|||
import lombok.Getter; |
|||
import lombok.Setter; |
|||
|
|||
import java.io.Serializable; |
|||
import java.math.BigDecimal; |
|||
import java.util.Date; |
|||
|
|||
/** |
|||
* <p> |
|||
* 优惠卷表 |
|||
* </p> |
|||
* |
|||
* @author zscat |
|||
* @since 2019-04-19 |
|||
*/ |
|||
@Setter |
|||
@Getter |
|||
@TableName("sms_coupon") |
|||
public class SmsCoupon extends BaseEntity implements Serializable { |
|||
|
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@TableId(value = "id", type = IdType.AUTO) |
|||
private Long id; |
|||
|
|||
/** |
|||
* 优惠卷类型;0->全场赠券;1->会员赠券;2->购物赠券;3->注册赠券 |
|||
*/ |
|||
private Integer type; |
|||
|
|||
private String name; |
|||
|
|||
/** |
|||
* 使用平台:0->全部;1->移动;2->PC |
|||
*/ |
|||
private Integer platform; |
|||
|
|||
/** |
|||
* 数量 |
|||
*/ |
|||
private Integer count; |
|||
|
|||
/** |
|||
* 金额 |
|||
*/ |
|||
private BigDecimal amount; |
|||
|
|||
/** |
|||
* 每人限领张数 |
|||
*/ |
|||
@TableField("per_limit") |
|||
private Integer perLimit; |
|||
|
|||
/** |
|||
* 使用门槛;0表示无门槛 |
|||
*/ |
|||
@TableField("min_point") |
|||
private BigDecimal minPoint; |
|||
|
|||
@TableField("start_time") |
|||
private Date startTime; |
|||
|
|||
@TableField("end_time") |
|||
private Date endTime; |
|||
|
|||
/** |
|||
* 使用类型:0->全场通用;1->指定分类;2->指定商品 |
|||
*/ |
|||
@TableField("use_type") |
|||
private Integer useType; |
|||
|
|||
/** |
|||
* 备注 |
|||
*/ |
|||
private String note; |
|||
|
|||
/** |
|||
* 发行数量 |
|||
*/ |
|||
@TableField("publish_count") |
|||
private Integer publishCount; |
|||
|
|||
/** |
|||
* 已使用数量 |
|||
*/ |
|||
@TableField("use_count") |
|||
private Integer useCount; |
|||
|
|||
/** |
|||
* 领取数量 |
|||
*/ |
|||
@TableField("receive_count") |
|||
private Integer receiveCount; |
|||
|
|||
/** |
|||
* 可以领取的日期 |
|||
*/ |
|||
@TableField("enable_time") |
|||
private Date enableTime; |
|||
|
|||
/** |
|||
* 优惠码 |
|||
*/ |
|||
private String code; |
|||
|
|||
/** |
|||
* 可领取的会员类型:0->无限时 |
|||
*/ |
|||
@TableField("member_level") |
|||
private Integer memberLevel; |
|||
|
|||
|
|||
} |
@ -0,0 +1,94 @@ |
|||
package com.yxt.yythmall.api.sms.entity; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.IdType; |
|||
import com.baomidou.mybatisplus.annotation.TableField; |
|||
import com.baomidou.mybatisplus.annotation.TableId; |
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.zscat.mallplus.utils.BaseEntity; |
|||
import lombok.Data; |
|||
|
|||
import java.io.Serializable; |
|||
import java.math.BigDecimal; |
|||
import java.util.Date; |
|||
|
|||
/** |
|||
* <p> |
|||
* 优惠券使用、领取历史表 |
|||
* </p> |
|||
* |
|||
* @author zscat |
|||
* @since 2019-04-19 |
|||
*/ |
|||
@Data |
|||
@TableName("sms_coupon_history") |
|||
public class SmsCouponHistory extends BaseEntity implements Serializable { |
|||
|
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@TableId(value = "id", type = IdType.AUTO) |
|||
private Long id; |
|||
|
|||
@TableField("coupon_id") |
|||
private Long couponId; |
|||
/** |
|||
* 使用门槛;0表示无门槛 |
|||
*/ |
|||
@TableField("min_point") |
|||
private BigDecimal minPoint; |
|||
|
|||
@TableField("member_id") |
|||
private Long memberId; |
|||
|
|||
@TableField("coupon_code") |
|||
private String couponCode; |
|||
|
|||
private BigDecimal amount; |
|||
/** |
|||
* 领取人昵称 |
|||
*/ |
|||
@TableField("member_nickname") |
|||
private String memberNickname; |
|||
|
|||
/** |
|||
* 获取类型:0->后台赠送;1->主动获取 |
|||
*/ |
|||
@TableField("get_type") |
|||
private Integer getType; |
|||
|
|||
@TableField("create_time") |
|||
private Date createTime; |
|||
|
|||
/** |
|||
* 使用状态:0->未使用;1->已使用;2->已过期 |
|||
*/ |
|||
@TableField("use_status") |
|||
private Integer useStatus; |
|||
|
|||
/** |
|||
* 使用时间 |
|||
*/ |
|||
@TableField("use_time") |
|||
private Date useTime; |
|||
|
|||
/** |
|||
* 订单编号 |
|||
*/ |
|||
@TableField("order_id") |
|||
private Long orderId; |
|||
|
|||
/** |
|||
* 订单号码 |
|||
*/ |
|||
@TableField("order_sn") |
|||
private String orderSn; |
|||
|
|||
@TableField("start_time") |
|||
private Date startTime; |
|||
|
|||
@TableField("end_time") |
|||
private Date endTime; |
|||
|
|||
private String note; |
|||
|
|||
|
|||
} |
@ -0,0 +1,96 @@ |
|||
package com.yxt.yythmall.api.sms.entity; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.IdType; |
|||
import com.baomidou.mybatisplus.annotation.TableField; |
|||
import com.baomidou.mybatisplus.annotation.TableId; |
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.zscat.mallplus.utils.BaseEntity; |
|||
|
|||
import java.io.Serializable; |
|||
|
|||
/** |
|||
* <p> |
|||
* 优惠券和产品分类关系表 |
|||
* </p> |
|||
* |
|||
* @author zscat |
|||
* @since 2019-04-19 |
|||
*/ |
|||
@TableName("sms_coupon_product_category_relation") |
|||
public class SmsCouponProductCategoryRelation extends BaseEntity implements Serializable { |
|||
|
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@TableId(value = "id", type = IdType.AUTO) |
|||
private Long id; |
|||
|
|||
@TableField("coupon_id") |
|||
private Long couponId; |
|||
|
|||
@TableField("product_category_id") |
|||
private Long productCategoryId; |
|||
|
|||
/** |
|||
* 产品分类名称 |
|||
*/ |
|||
@TableField("product_category_name") |
|||
private String productCategoryName; |
|||
|
|||
/** |
|||
* 父分类名称 |
|||
*/ |
|||
@TableField("parent_category_name") |
|||
private String parentCategoryName; |
|||
|
|||
|
|||
public Long getId() { |
|||
return id; |
|||
} |
|||
|
|||
public void setId(Long id) { |
|||
this.id = id; |
|||
} |
|||
|
|||
public Long getCouponId() { |
|||
return couponId; |
|||
} |
|||
|
|||
public void setCouponId(Long couponId) { |
|||
this.couponId = couponId; |
|||
} |
|||
|
|||
public Long getProductCategoryId() { |
|||
return productCategoryId; |
|||
} |
|||
|
|||
public void setProductCategoryId(Long productCategoryId) { |
|||
this.productCategoryId = productCategoryId; |
|||
} |
|||
|
|||
public String getProductCategoryName() { |
|||
return productCategoryName; |
|||
} |
|||
|
|||
public void setProductCategoryName(String productCategoryName) { |
|||
this.productCategoryName = productCategoryName; |
|||
} |
|||
|
|||
public String getParentCategoryName() { |
|||
return parentCategoryName; |
|||
} |
|||
|
|||
public void setParentCategoryName(String parentCategoryName) { |
|||
this.parentCategoryName = parentCategoryName; |
|||
} |
|||
|
|||
@Override |
|||
public String toString() { |
|||
return "SmsCouponProductCategoryRelation{" + |
|||
", id=" + id + |
|||
", couponId=" + couponId + |
|||
", productCategoryId=" + productCategoryId + |
|||
", productCategoryName=" + productCategoryName + |
|||
", parentCategoryName=" + parentCategoryName + |
|||
"}"; |
|||
} |
|||
} |
@ -0,0 +1,96 @@ |
|||
package com.yxt.yythmall.api.sms.entity; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.IdType; |
|||
import com.baomidou.mybatisplus.annotation.TableField; |
|||
import com.baomidou.mybatisplus.annotation.TableId; |
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.zscat.mallplus.utils.BaseEntity; |
|||
|
|||
import java.io.Serializable; |
|||
|
|||
/** |
|||
* <p> |
|||
* 优惠券和产品的关系表 |
|||
* </p> |
|||
* |
|||
* @author zscat |
|||
* @since 2019-04-19 |
|||
*/ |
|||
@TableName("sms_coupon_product_relation") |
|||
public class SmsCouponProductRelation extends BaseEntity implements Serializable { |
|||
|
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@TableId(value = "id", type = IdType.AUTO) |
|||
private Long id; |
|||
|
|||
@TableField("coupon_id") |
|||
private Long couponId; |
|||
|
|||
@TableField("product_id") |
|||
private Long productId; |
|||
|
|||
/** |
|||
* 商品名称 |
|||
*/ |
|||
@TableField("product_name") |
|||
private String productName; |
|||
|
|||
/** |
|||
* 商品编码 |
|||
*/ |
|||
@TableField("product_sn") |
|||
private String productSn; |
|||
|
|||
|
|||
public Long getId() { |
|||
return id; |
|||
} |
|||
|
|||
public void setId(Long id) { |
|||
this.id = id; |
|||
} |
|||
|
|||
public Long getCouponId() { |
|||
return couponId; |
|||
} |
|||
|
|||
public void setCouponId(Long couponId) { |
|||
this.couponId = couponId; |
|||
} |
|||
|
|||
public Long getProductId() { |
|||
return productId; |
|||
} |
|||
|
|||
public void setProductId(Long productId) { |
|||
this.productId = productId; |
|||
} |
|||
|
|||
public String getProductName() { |
|||
return productName; |
|||
} |
|||
|
|||
public void setProductName(String productName) { |
|||
this.productName = productName; |
|||
} |
|||
|
|||
public String getProductSn() { |
|||
return productSn; |
|||
} |
|||
|
|||
public void setProductSn(String productSn) { |
|||
this.productSn = productSn; |
|||
} |
|||
|
|||
@Override |
|||
public String toString() { |
|||
return "SmsCouponProductRelation{" + |
|||
", id=" + id + |
|||
", couponId=" + couponId + |
|||
", productId=" + productId + |
|||
", productName=" + productName + |
|||
", productSn=" + productSn + |
|||
"}"; |
|||
} |
|||
} |
@ -0,0 +1,46 @@ |
|||
package com.yxt.yythmall.api.sms.vo; |
|||
|
|||
|
|||
import com.yxt.yythmall.api.sms.entity.SmsCoupon; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCouponHistory; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCouponProductCategoryRelation; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCouponProductRelation; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 优惠券领取历史详情封装 |
|||
* https://github.com/shenzhuan/mallplus on 2018/8/29.
|
|||
*/ |
|||
public class SmsCouponHistoryDetail extends SmsCouponHistory { |
|||
//相关优惠券信息
|
|||
private SmsCoupon coupon; |
|||
//优惠券关联商品
|
|||
private List<SmsCouponProductRelation> productRelationList; |
|||
//优惠券关联商品分类
|
|||
private List<SmsCouponProductCategoryRelation> categoryRelationList; |
|||
|
|||
public SmsCoupon getCoupon() { |
|||
return coupon; |
|||
} |
|||
|
|||
public void setCoupon(SmsCoupon coupon) { |
|||
this.coupon = coupon; |
|||
} |
|||
|
|||
public List<SmsCouponProductRelation> getProductRelationList() { |
|||
return productRelationList; |
|||
} |
|||
|
|||
public void setProductRelationList(List<SmsCouponProductRelation> productRelationList) { |
|||
this.productRelationList = productRelationList; |
|||
} |
|||
|
|||
public List<SmsCouponProductCategoryRelation> getCategoryRelationList() { |
|||
return categoryRelationList; |
|||
} |
|||
|
|||
public void setCategoryRelationList(List<SmsCouponProductCategoryRelation> categoryRelationList) { |
|||
this.categoryRelationList = categoryRelationList; |
|||
} |
|||
} |
@ -0,0 +1,35 @@ |
|||
package com.yxt.yythmall.api.sms.vo; |
|||
|
|||
|
|||
import com.yxt.yythmall.api.sms.entity.SmsCoupon; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCouponProductCategoryRelation; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCouponProductRelation; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* 优惠券信息封装,包括绑定商品和绑定分类 |
|||
* https://github.com/shenzhuan/mallplus on 2018/8/28.
|
|||
*/ |
|||
public class SmsCouponParam extends SmsCoupon { |
|||
//优惠券绑定的商品
|
|||
private List<SmsCouponProductRelation> productRelationList; |
|||
//优惠券绑定的商品分类
|
|||
private List<SmsCouponProductCategoryRelation> productCategoryRelationList; |
|||
|
|||
public List<SmsCouponProductRelation> getProductRelationList() { |
|||
return productRelationList; |
|||
} |
|||
|
|||
public void setProductRelationList(List<SmsCouponProductRelation> productRelationList) { |
|||
this.productRelationList = productRelationList; |
|||
} |
|||
|
|||
public List<SmsCouponProductCategoryRelation> getProductCategoryRelationList() { |
|||
return productCategoryRelationList; |
|||
} |
|||
|
|||
public void setProductCategoryRelationList(List<SmsCouponProductCategoryRelation> productCategoryRelationList) { |
|||
this.productCategoryRelationList = productCategoryRelationList; |
|||
} |
|||
} |
@ -0,0 +1,16 @@ |
|||
package com.yxt.yythmall.biz.smscoupon; |
|||
|
|||
import com.baomidou.mybatisplus.extension.service.IService; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCouponHistory; |
|||
|
|||
/** |
|||
* <p> |
|||
* 优惠券使用、领取历史表 服务类 |
|||
* </p> |
|||
* |
|||
* @author zscat |
|||
* @since 2019-04-19 |
|||
*/ |
|||
public interface ISmsCouponHistoryService extends IService<SmsCouponHistory> { |
|||
|
|||
} |
@ -0,0 +1,16 @@ |
|||
package com.yxt.yythmall.biz.smscoupon; |
|||
|
|||
import com.baomidou.mybatisplus.extension.service.IService; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCouponProductCategoryRelation; |
|||
|
|||
/** |
|||
* <p> |
|||
* 优惠券和产品分类关系表 服务类 |
|||
* </p> |
|||
* |
|||
* @author zscat |
|||
* @since 2019-04-19 |
|||
*/ |
|||
public interface ISmsCouponProductCategoryRelationService extends IService<SmsCouponProductCategoryRelation> { |
|||
|
|||
} |
@ -0,0 +1,16 @@ |
|||
package com.yxt.yythmall.biz.smscoupon; |
|||
|
|||
import com.baomidou.mybatisplus.extension.service.IService; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCouponProductRelation; |
|||
|
|||
/** |
|||
* <p> |
|||
* 优惠券和产品的关系表 服务类 |
|||
* </p> |
|||
* |
|||
* @author zscat |
|||
* @since 2019-04-19 |
|||
*/ |
|||
public interface ISmsCouponProductRelationService extends IService<SmsCouponProductRelation> { |
|||
|
|||
} |
@ -0,0 +1,35 @@ |
|||
package com.yxt.yythmall.biz.smscoupon; |
|||
|
|||
import com.baomidou.mybatisplus.extension.service.IService; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCoupon; |
|||
import com.yxt.yythmall.api.sms.vo.SmsCouponParam; |
|||
import org.springframework.transaction.annotation.Transactional; |
|||
|
|||
/** |
|||
* <p> |
|||
* 优惠卷表 服务类 |
|||
* </p> |
|||
* |
|||
* @author zscat |
|||
* @since 2019-04-19 |
|||
*/ |
|||
public interface ISmsCouponService extends IService<SmsCoupon> { |
|||
|
|||
boolean saves(SmsCouponParam entity); |
|||
|
|||
boolean updateByIds(SmsCouponParam entity); |
|||
|
|||
/** |
|||
* 获取优惠券详情 |
|||
* |
|||
* @param id 优惠券表id |
|||
*/ |
|||
SmsCouponParam getItem(Long id); |
|||
|
|||
/** |
|||
* 根据优惠券id删除优惠券 |
|||
*/ |
|||
@Transactional |
|||
int delete(Long id); |
|||
|
|||
} |
@ -0,0 +1,119 @@ |
|||
package com.yxt.yythmall.biz.smscoupon; |
|||
|
|||
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
|||
import com.baomidou.mybatisplus.extension.plugins.pagination.Page; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCoupon; |
|||
import com.yxt.yythmall.api.sms.vo.SmsCouponParam; |
|||
import com.zscat.mallplus.utils.CommonResult; |
|||
import com.zscat.mallplus.utils.ValidatorUtils; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import io.swagger.annotations.ApiParam; |
|||
import lombok.extern.slf4j.Slf4j; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import javax.annotation.Resource; |
|||
|
|||
/** |
|||
* <p> |
|||
* 优惠卷表 |
|||
* </p> |
|||
* |
|||
* @author zscat |
|||
* @since 2019-04-19 |
|||
*/ |
|||
@Slf4j |
|||
@RestController |
|||
@Api(tags = "SmsCouponController", description = "优惠卷表管理") |
|||
@RequestMapping("/sms/SmsCoupon") |
|||
public class SmsCouponController { |
|||
@Resource |
|||
private com.yxt.yythmall.biz.smscoupon.ISmsCouponService ISmsCouponService; |
|||
|
|||
// @SysLog(MODULE = "sms", REMARK = "根据条件查询所有优惠卷表列表")
|
|||
@ApiOperation("根据条件查询所有优惠卷表列表") |
|||
@GetMapping(value = "/list") |
|||
// @PreAuthorize("hasAuthority('sms:SmsCoupon:read')")
|
|||
public Object getSmsCouponByPage(SmsCoupon entity, |
|||
@RequestParam(value = "pageNum", defaultValue = "1") Integer pageNum, |
|||
@RequestParam(value = "pageSize", defaultValue = "10") Integer pageSize |
|||
) { |
|||
try { |
|||
return new CommonResult().success(ISmsCouponService.page(new Page<SmsCoupon>(pageNum, pageSize), new QueryWrapper<>(entity))); |
|||
} catch (Exception e) { |
|||
log.error("根据条件查询所有优惠卷表列表:%s", e.getMessage(), e); |
|||
} |
|||
return new CommonResult().failed(); |
|||
} |
|||
|
|||
// @SysLog(MODULE = "sms", REMARK = "保存优惠卷表")
|
|||
@ApiOperation("保存优惠卷表") |
|||
@PostMapping(value = "/create") |
|||
// @PreAuthorize("hasAuthority('sms:SmsCoupon:create')")
|
|||
public Object saveSmsCoupon(@RequestBody SmsCouponParam entity) { |
|||
try { |
|||
if (ISmsCouponService.saves(entity)) { |
|||
return new CommonResult().success(); |
|||
} |
|||
} catch (Exception e) { |
|||
log.error("保存优惠卷表:%s", e.getMessage(), e); |
|||
return new CommonResult().failed(); |
|||
} |
|||
return new CommonResult().failed(); |
|||
} |
|||
|
|||
// @SysLog(MODULE = "sms", REMARK = "更新优惠卷表")
|
|||
@ApiOperation("更新优惠卷表") |
|||
@PostMapping(value = "/update/{id}") |
|||
// @PreAuthorize("hasAuthority('sms:SmsCoupon:update')")
|
|||
public Object updateSmsCoupon(@RequestBody SmsCouponParam entity) { |
|||
try { |
|||
if (ISmsCouponService.updateByIds(entity)) { |
|||
return new CommonResult().success(); |
|||
} |
|||
} catch (Exception e) { |
|||
log.error("更新优惠卷表:%s", e.getMessage(), e); |
|||
return new CommonResult().failed(); |
|||
} |
|||
return new CommonResult().failed(); |
|||
} |
|||
|
|||
// @SysLog(MODULE = "sms", REMARK = "删除优惠卷表")
|
|||
@ApiOperation("删除优惠卷表") |
|||
@GetMapping(value = "/delete/{id}") |
|||
// @PreAuthorize("hasAuthority('sms:SmsCoupon:delete')")
|
|||
public Object deleteSmsCoupon(@ApiParam("优惠卷表id") @PathVariable Long id) { |
|||
try { |
|||
if (ValidatorUtils.empty(id)) { |
|||
return new CommonResult().paramFailed("优惠卷表id"); |
|||
} |
|||
if (ISmsCouponService.delete(id) > 0) { |
|||
return new CommonResult().success(); |
|||
} |
|||
} catch (Exception e) { |
|||
log.error("删除优惠卷表:%s", e.getMessage(), e); |
|||
return new CommonResult().failed(); |
|||
} |
|||
return new CommonResult().failed(); |
|||
} |
|||
|
|||
// @SysLog(MODULE = "sms", REMARK = "给优惠卷表分配优惠卷表")
|
|||
@ApiOperation("查询优惠卷表明细") |
|||
@GetMapping(value = "/{id}") |
|||
// @PreAuthorize("hasAuthority('sms:SmsCoupon:read')")
|
|||
public Object getSmsCouponById(@ApiParam("优惠卷表id") @PathVariable Long id) { |
|||
try { |
|||
if (ValidatorUtils.empty(id)) { |
|||
return new CommonResult().paramFailed("优惠卷表id"); |
|||
} |
|||
SmsCouponParam coupon = ISmsCouponService.getItem(id); |
|||
return new CommonResult().success(coupon); |
|||
} catch (Exception e) { |
|||
log.error("查询优惠卷表明细:%s", e.getMessage(), e); |
|||
return new CommonResult().failed(); |
|||
} |
|||
|
|||
} |
|||
|
|||
|
|||
} |
@ -0,0 +1,132 @@ |
|||
package com.yxt.yythmall.biz.smscoupon; |
|||
|
|||
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
|||
import com.baomidou.mybatisplus.extension.plugins.pagination.Page; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCouponHistory; |
|||
import com.zscat.mallplus.utils.CommonResult; |
|||
import com.zscat.mallplus.utils.ValidatorUtils; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import io.swagger.annotations.ApiParam; |
|||
import lombok.extern.slf4j.Slf4j; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import javax.annotation.Resource; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* <p> |
|||
* 优惠券使用、领取历史表 |
|||
* </p> |
|||
* |
|||
* @author zscat |
|||
* @since 2019-04-19 |
|||
*/ |
|||
@Slf4j |
|||
@RestController |
|||
@Api(tags = "SmsCouponHistoryController", description = "优惠券使用、领取历史表管理") |
|||
@RequestMapping("/sms/SmsCouponHistory") |
|||
public class SmsCouponHistoryController { |
|||
@Resource |
|||
private com.yxt.yythmall.biz.smscoupon.ISmsCouponHistoryService ISmsCouponHistoryService; |
|||
|
|||
// @SysLog(MODULE = "sms", REMARK = "根据条件查询所有优惠券使用、领取历史表列表")
|
|||
@ApiOperation("根据条件查询所有优惠券使用、领取历史表列表") |
|||
@GetMapping(value = "/list") |
|||
// @PreAuthorize("hasAuthority('sms:SmsCouponHistory:read')")
|
|||
public Object getSmsCouponHistoryByPage(SmsCouponHistory entity, |
|||
@RequestParam(value = "pageNum", defaultValue = "1") Integer pageNum, |
|||
@RequestParam(value = "pageSize", defaultValue = "10") Integer pageSize |
|||
) { |
|||
try { |
|||
return new CommonResult().success(ISmsCouponHistoryService.page(new Page<SmsCouponHistory>(pageNum, pageSize), new QueryWrapper<>(entity))); |
|||
} catch (Exception e) { |
|||
log.error("根据条件查询所有优惠券使用、领取历史表列表:%s", e.getMessage(), e); |
|||
} |
|||
return new CommonResult().failed(); |
|||
} |
|||
|
|||
// @SysLog(MODULE = "sms", REMARK = "保存优惠券使用、领取历史表")
|
|||
@ApiOperation("保存优惠券使用、领取历史表") |
|||
@PostMapping(value = "/create") |
|||
// @PreAuthorize("hasAuthority('sms:SmsCouponHistory:create')")
|
|||
public Object saveSmsCouponHistory(@RequestBody SmsCouponHistory entity) { |
|||
try { |
|||
if (ISmsCouponHistoryService.save(entity)) { |
|||
return new CommonResult().success(); |
|||
} |
|||
} catch (Exception e) { |
|||
log.error("保存优惠券使用、领取历史表:%s", e.getMessage(), e); |
|||
return new CommonResult().failed(); |
|||
} |
|||
return new CommonResult().failed(); |
|||
} |
|||
|
|||
// @SysLog(MODULE = "sms", REMARK = "更新优惠券使用、领取历史表")
|
|||
@ApiOperation("更新优惠券使用、领取历史表") |
|||
@PostMapping(value = "/update/{id}") |
|||
// @PreAuthorize("hasAuthority('sms:SmsCouponHistory:update')")
|
|||
public Object updateSmsCouponHistory(@RequestBody SmsCouponHistory entity) { |
|||
try { |
|||
if (ISmsCouponHistoryService.updateById(entity)) { |
|||
return new CommonResult().success(); |
|||
} |
|||
} catch (Exception e) { |
|||
log.error("更新优惠券使用、领取历史表:%s", e.getMessage(), e); |
|||
return new CommonResult().failed(); |
|||
} |
|||
return new CommonResult().failed(); |
|||
} |
|||
|
|||
// @SysLog(MODULE = "sms", REMARK = "删除优惠券使用、领取历史表")
|
|||
@ApiOperation("删除优惠券使用、领取历史表") |
|||
@GetMapping(value = "/delete/{id}") |
|||
// @PreAuthorize("hasAuthority('sms:SmsCouponHistory:delete')")
|
|||
public Object deleteSmsCouponHistory(@ApiParam("优惠券使用、领取历史表id") @PathVariable Long id) { |
|||
try { |
|||
if (ValidatorUtils.empty(id)) { |
|||
return new CommonResult().paramFailed("优惠券使用、领取历史表id"); |
|||
} |
|||
if (ISmsCouponHistoryService.removeById(id)) { |
|||
return new CommonResult().success(); |
|||
} |
|||
} catch (Exception e) { |
|||
log.error("删除优惠券使用、领取历史表:%s", e.getMessage(), e); |
|||
return new CommonResult().failed(); |
|||
} |
|||
return new CommonResult().failed(); |
|||
} |
|||
|
|||
// @SysLog(MODULE = "sms", REMARK = "给优惠券使用、领取历史表分配优惠券使用、领取历史表")
|
|||
@ApiOperation("查询优惠券使用、领取历史表明细") |
|||
@GetMapping(value = "/{id}") |
|||
// @PreAuthorize("hasAuthority('sms:SmsCouponHistory:read')")
|
|||
public Object getSmsCouponHistoryById(@ApiParam("优惠券使用、领取历史表id") @PathVariable Long id) { |
|||
try { |
|||
if (ValidatorUtils.empty(id)) { |
|||
return new CommonResult().paramFailed("优惠券使用、领取历史表id"); |
|||
} |
|||
SmsCouponHistory coupon = ISmsCouponHistoryService.getById(id); |
|||
return new CommonResult().success(coupon); |
|||
} catch (Exception e) { |
|||
log.error("查询优惠券使用、领取历史表明细:%s", e.getMessage(), e); |
|||
return new CommonResult().failed(); |
|||
} |
|||
|
|||
} |
|||
|
|||
@ApiOperation(value = "批量删除优惠券使用、领取历史表") |
|||
@RequestMapping(value = "/delete/batch", method = RequestMethod.GET) |
|||
@ResponseBody |
|||
// @SysLog(MODULE = "pms", REMARK = "批量删除优惠券使用、领取历史表")
|
|||
// @PreAuthorize("hasAuthority('sms:SmsCouponHistory:delete')")
|
|||
public Object deleteBatch(@RequestParam("ids") List<Long> ids) { |
|||
boolean count = ISmsCouponHistoryService.removeByIds(ids); |
|||
if (count) { |
|||
return new CommonResult().success(count); |
|||
} else { |
|||
return new CommonResult().failed(); |
|||
} |
|||
} |
|||
|
|||
} |
@ -0,0 +1,26 @@ |
|||
package com.yxt.yythmall.biz.smscoupon; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCouponHistory; |
|||
import com.yxt.yythmall.api.sms.vo.SmsCouponHistoryDetail; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
import org.apache.ibatis.annotations.Param; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* <p> |
|||
* 优惠券使用、领取历史表 Mapper 接口 |
|||
* </p> |
|||
* |
|||
* @author zscat |
|||
* @since 2019-04-19 |
|||
*/@Mapper |
|||
public interface SmsCouponHistoryMapper extends BaseMapper<SmsCouponHistory> { |
|||
|
|||
List<SmsCouponHistoryDetail> getDetailList(Long memberId); |
|||
|
|||
int updateUseStatus(@Param("useStatus") int i, @Param("endTime") String tomorrow); |
|||
|
|||
int updateUseStatuss(@Param("useStatus")int i, @Param("memberId") Long memberId,@Param("couponId") Long couponId); |
|||
} |
@ -0,0 +1,72 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.biz.smscoupon.SmsCouponHistoryMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.api.sms.entity.SmsCouponHistory"> |
|||
<id column="id" property="id"/> |
|||
<result column="coupon_id" property="couponId"/> |
|||
<result column="member_id" property="memberId"/> |
|||
<result column="coupon_code" property="couponCode"/> |
|||
<result column="member_nickname" property="memberNickname"/> |
|||
<result column="get_type" property="getType"/> |
|||
<result column="create_time" property="createTime"/> |
|||
<result column="use_status" property="useStatus"/> |
|||
<result column="use_time" property="useTime"/> |
|||
<result column="order_id" property="orderId"/> |
|||
<result column="order_sn" property="orderSn"/> |
|||
<result column="start_time" property="startTime"/> |
|||
<result column="end_time" property="endTime"/> |
|||
<result column="note" property="note"/> |
|||
</resultMap> |
|||
|
|||
<resultMap id="couponHistoryDetailMap" type="com.yxt.yythmall.api.sms.vo.SmsCouponHistoryDetail" |
|||
extends="BaseResultMap"> |
|||
<association property="coupon" resultMap="com.yxt.yythmall.biz.smscoupon.SmsCouponMapper.BaseResultMap" |
|||
columnPrefix="c_"> |
|||
</association> |
|||
<collection property="productRelationList" columnPrefix="cpr_" |
|||
resultMap="com.yxt.yythmall.biz.smscoupon.SmsCouponProductRelationMapper.BaseResultMap"> |
|||
</collection> |
|||
<collection property="categoryRelationList" columnPrefix="cpcr_" |
|||
resultMap="com.yxt.yythmall.biz.smscoupon.SmsCouponProductCategoryRelationMapper.BaseResultMap"> |
|||
</collection> |
|||
</resultMap> |
|||
<select id="getDetailList" resultMap="couponHistoryDetailMap"> |
|||
SELECT |
|||
ch.*, |
|||
c.id c_id, |
|||
c.name c_name, |
|||
c.amount c_amount, |
|||
c.min_point c_min_point, |
|||
c.platform c_platform, |
|||
c.start_time c_start_time, |
|||
c.end_time c_end_time, |
|||
c.note c_note, |
|||
c.use_type c_use_type, |
|||
c.type c_type, |
|||
cpr.id cpr_id,cpr.product_id cpr_product_id, |
|||
cpcr.id cpcr_id,cpcr.product_category_id cpcr_product_category_id |
|||
FROM |
|||
sms_coupon_history ch |
|||
LEFT JOIN sms_coupon c ON ch.coupon_id = c.id |
|||
LEFT JOIN sms_coupon_product_relation cpr ON cpr.coupon_id = c.id |
|||
LEFT JOIN sms_coupon_product_category_relation cpcr ON cpcr.coupon_id = c.id |
|||
WHERE ch.member_id = #{memberId} |
|||
AND ch.use_status = 0 and now() >c.start_time and c.end_time>now() |
|||
order by ch.amount desc |
|||
</select> |
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, coupon_id, member_id, coupon_code, member_nickname, get_type, create_time, use_status, use_time, order_id, |
|||
order_sn, start_time, end_time, note |
|||
</sql> |
|||
|
|||
<update id="updateUseStatus"> |
|||
update sms_coupon_history set use_status = #{useStatus} where end_time <= #{endTime} and use_status = 0 |
|||
</update> |
|||
|
|||
<update id="updateUseStatuss"> |
|||
update sms_coupon_history set use_status = #{useStatus} where member_id = #{memberId} and coupon_id = #{couponId} |
|||
</update> |
|||
</mapper> |
@ -0,0 +1,18 @@ |
|||
package com.yxt.yythmall.biz.smscoupon; |
|||
|
|||
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCouponHistory; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
/** |
|||
* <p> |
|||
* 优惠券使用、领取历史表 服务实现类 |
|||
* </p> |
|||
* |
|||
* @author zscat |
|||
* @since 2019-04-19 |
|||
*/ |
|||
@Service |
|||
public class SmsCouponHistoryServiceImpl extends ServiceImpl<SmsCouponHistoryMapper, SmsCouponHistory> implements ISmsCouponHistoryService { |
|||
|
|||
} |
@ -0,0 +1,29 @@ |
|||
package com.yxt.yythmall.biz.smscoupon; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCoupon; |
|||
import com.yxt.yythmall.api.sms.vo.SmsCouponParam; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
import org.apache.ibatis.annotations.Param; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* <p> |
|||
* 优惠卷表 Mapper 接口 |
|||
* </p> |
|||
* |
|||
* @author zscat |
|||
* @since 2019-04-19 |
|||
*/ |
|||
@Mapper |
|||
public interface SmsCouponMapper extends BaseMapper<SmsCoupon> { |
|||
|
|||
List<SmsCoupon> selectNotRecive(@Param("memberId") Long memberId, @Param("limit") Integer limit); |
|||
|
|||
List<SmsCoupon> selectRecive(@Param("memberId") Long memberId, @Param("limit") Integer limit); |
|||
|
|||
SmsCouponParam getItem(@Param("id") Long id); |
|||
|
|||
List<SmsCoupon> selectAll(); |
|||
} |
@ -0,0 +1,76 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.biz.smscoupon.SmsCouponMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.api.sms.entity.SmsCoupon"> |
|||
<id column="id" property="id"/> |
|||
<result column="type" property="type"/> |
|||
<result column="name" property="name"/> |
|||
<result column="platform" property="platform"/> |
|||
<result column="count" property="count"/> |
|||
<result column="amount" property="amount"/> |
|||
<result column="per_limit" property="perLimit"/> |
|||
<result column="min_point" property="minPoint"/> |
|||
<result column="start_time" property="startTime"/> |
|||
<result column="end_time" property="endTime"/> |
|||
<result column="use_type" property="useType"/> |
|||
<result column="note" property="note"/> |
|||
<result column="publish_count" property="publishCount"/> |
|||
<result column="use_count" property="useCount"/> |
|||
<result column="receive_count" property="receiveCount"/> |
|||
<result column="enable_time" property="enableTime"/> |
|||
<result column="code" property="code"/> |
|||
<result column="member_level" property="memberLevel"/> |
|||
</resultMap> |
|||
|
|||
<resultMap id="couponItemParam" type="com.yxt.yythmall.api.sms.vo.SmsCouponParam" |
|||
extends="com.yxt.yythmall.biz.smscoupon.SmsCouponMapper.BaseResultMap"> |
|||
<collection property="productRelationList" columnPrefix="cpr_" |
|||
resultMap="com.yxt.yythmall.biz.smscoupon.SmsCouponProductRelationMapper.BaseResultMap"> |
|||
</collection> |
|||
<collection property="productCategoryRelationList" columnPrefix="cpcr_" |
|||
resultMap="com.yxt.yythmall.biz.smscoupon.SmsCouponProductCategoryRelationMapper.BaseResultMap"> |
|||
</collection> |
|||
</resultMap> |
|||
<select id="getItem" resultMap="couponItemParam"> |
|||
SELECT |
|||
c.*, |
|||
cpr.id cpr_id, |
|||
cpr.product_id cpr_product_id, |
|||
cpr.product_name cpr_product_name, |
|||
cpr.product_sn cpr_product_sn, |
|||
cpcr.id cpcr_id, |
|||
cpcr.product_category_id cpcr_product_category_id, |
|||
cpcr.product_category_name cpcr_product_category_name, |
|||
cpcr.parent_category_name cpcr_parent_category_name |
|||
FROM |
|||
sms_coupon c |
|||
LEFT JOIN sms_coupon_product_relation cpr ON c.id = cpr.coupon_id |
|||
LEFT JOIN sms_coupon_product_category_relation cpcr ON c.id = cpcr.coupon_id |
|||
WHERE |
|||
c.id = #{id} |
|||
</select> |
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, type, name, platform, count, amount, per_limit, min_point, start_time, end_time, use_type, note, |
|||
publish_count, use_count, receive_count, enable_time, code, member_level |
|||
</sql> |
|||
|
|||
<select id="selectNotRecive" resultMap="BaseResultMap"> |
|||
SELECT sms_coupon.* from sms_coupon where count>0 and now()>=start_time and end_time>=now() and (SELECT count(*) from sms_coupon_history where member_id = |
|||
#{memberId} and sms_coupon.id = coupon_id) < per_limit |
|||
<if test="limit != null and limit != ''"> |
|||
limit #{limit} |
|||
</if> |
|||
|
|||
</select> |
|||
<select id="selectRecive" resultMap="BaseResultMap"> |
|||
SELECT sms_coupon.* from sms_coupon where id in (SELECT coupon_id from sms_coupon_history where member_id = |
|||
#{memberId}) limit #{limit} |
|||
</select> |
|||
|
|||
<select id="selectAll" resultMap="BaseResultMap"> |
|||
SELECT sms_coupon.* from sms_coupon where count>0 and now()>=start_time and end_time>=now() |
|||
</select> |
|||
</mapper> |
@ -0,0 +1,132 @@ |
|||
package com.yxt.yythmall.biz.smscoupon; |
|||
|
|||
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
|||
import com.baomidou.mybatisplus.extension.plugins.pagination.Page; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCouponProductCategoryRelation; |
|||
import com.zscat.mallplus.utils.CommonResult; |
|||
import com.zscat.mallplus.utils.ValidatorUtils; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import io.swagger.annotations.ApiParam; |
|||
import lombok.extern.slf4j.Slf4j; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import javax.annotation.Resource; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* <p> |
|||
* 优惠券和产品分类关系表 |
|||
* </p> |
|||
* |
|||
* @author zscat |
|||
* @since 2019-04-19 |
|||
*/ |
|||
@Slf4j |
|||
@RestController |
|||
@Api(tags = "SmsCouponProductCategoryRelationController", description = "优惠券和产品分类关系表管理") |
|||
@RequestMapping("/sms/SmsCouponProductCategoryRelation") |
|||
public class SmsCouponProductCategoryRelationController { |
|||
@Resource |
|||
private com.yxt.yythmall.biz.smscoupon.ISmsCouponProductCategoryRelationService ISmsCouponProductCategoryRelationService; |
|||
|
|||
// @SysLog(MODULE = "sms", REMARK = "根据条件查询所有优惠券和产品分类关系表列表")
|
|||
@ApiOperation("根据条件查询所有优惠券和产品分类关系表列表") |
|||
@GetMapping(value = "/list") |
|||
// @PreAuthorize("hasAuthority('sms:SmsCouponProductCategoryRelation:read')")
|
|||
public Object getSmsCouponProductCategoryRelationByPage(SmsCouponProductCategoryRelation entity, |
|||
@RequestParam(value = "pageNum", defaultValue = "1") Integer pageNum, |
|||
@RequestParam(value = "pageSize", defaultValue = "10") Integer pageSize |
|||
) { |
|||
try { |
|||
return new CommonResult().success(ISmsCouponProductCategoryRelationService.page(new Page<SmsCouponProductCategoryRelation>(pageNum, pageSize), new QueryWrapper<>(entity))); |
|||
} catch (Exception e) { |
|||
log.error("根据条件查询所有优惠券和产品分类关系表列表:%s", e.getMessage(), e); |
|||
} |
|||
return new CommonResult().failed(); |
|||
} |
|||
|
|||
// @SysLog(MODULE = "sms", REMARK = "保存优惠券和产品分类关系表")
|
|||
@ApiOperation("保存优惠券和产品分类关系表") |
|||
@PostMapping(value = "/create") |
|||
// @PreAuthorize("hasAuthority('sms:SmsCouponProductCategoryRelation:create')")
|
|||
public Object saveSmsCouponProductCategoryRelation(@RequestBody SmsCouponProductCategoryRelation entity) { |
|||
try { |
|||
if (ISmsCouponProductCategoryRelationService.save(entity)) { |
|||
return new CommonResult().success(); |
|||
} |
|||
} catch (Exception e) { |
|||
log.error("保存优惠券和产品分类关系表:%s", e.getMessage(), e); |
|||
return new CommonResult().failed(); |
|||
} |
|||
return new CommonResult().failed(); |
|||
} |
|||
|
|||
// @SysLog(MODULE = "sms", REMARK = "更新优惠券和产品分类关系表")
|
|||
@ApiOperation("更新优惠券和产品分类关系表") |
|||
@PostMapping(value = "/update/{id}") |
|||
// @PreAuthorize("hasAuthority('sms:SmsCouponProductCategoryRelation:update')")
|
|||
public Object updateSmsCouponProductCategoryRelation(@RequestBody SmsCouponProductCategoryRelation entity) { |
|||
try { |
|||
if (ISmsCouponProductCategoryRelationService.updateById(entity)) { |
|||
return new CommonResult().success(); |
|||
} |
|||
} catch (Exception e) { |
|||
log.error("更新优惠券和产品分类关系表:%s", e.getMessage(), e); |
|||
return new CommonResult().failed(); |
|||
} |
|||
return new CommonResult().failed(); |
|||
} |
|||
|
|||
// @SysLog(MODULE = "sms", REMARK = "删除优惠券和产品分类关系表")
|
|||
@ApiOperation("删除优惠券和产品分类关系表") |
|||
@GetMapping(value = "/delete/{id}") |
|||
// @PreAuthorize("hasAuthority('sms:SmsCouponProductCategoryRelation:delete')")
|
|||
public Object deleteSmsCouponProductCategoryRelation(@ApiParam("优惠券和产品分类关系表id") @PathVariable Long id) { |
|||
try { |
|||
if (ValidatorUtils.empty(id)) { |
|||
return new CommonResult().paramFailed("优惠券和产品分类关系表id"); |
|||
} |
|||
if (ISmsCouponProductCategoryRelationService.removeById(id)) { |
|||
return new CommonResult().success(); |
|||
} |
|||
} catch (Exception e) { |
|||
log.error("删除优惠券和产品分类关系表:%s", e.getMessage(), e); |
|||
return new CommonResult().failed(); |
|||
} |
|||
return new CommonResult().failed(); |
|||
} |
|||
|
|||
// @SysLog(MODULE = "sms", REMARK = "给优惠券和产品分类关系表分配优惠券和产品分类关系表")
|
|||
@ApiOperation("查询优惠券和产品分类关系表明细") |
|||
@GetMapping(value = "/{id}") |
|||
// @PreAuthorize("hasAuthority('sms:SmsCouponProductCategoryRelation:read')")
|
|||
public Object getSmsCouponProductCategoryRelationById(@ApiParam("优惠券和产品分类关系表id") @PathVariable Long id) { |
|||
try { |
|||
if (ValidatorUtils.empty(id)) { |
|||
return new CommonResult().paramFailed("优惠券和产品分类关系表id"); |
|||
} |
|||
SmsCouponProductCategoryRelation coupon = ISmsCouponProductCategoryRelationService.getById(id); |
|||
return new CommonResult().success(coupon); |
|||
} catch (Exception e) { |
|||
log.error("查询优惠券和产品分类关系表明细:%s", e.getMessage(), e); |
|||
return new CommonResult().failed(); |
|||
} |
|||
|
|||
} |
|||
|
|||
@ApiOperation(value = "批量删除优惠券和产品分类关系表") |
|||
@RequestMapping(value = "/delete/batch", method = RequestMethod.GET) |
|||
@ResponseBody |
|||
// @SysLog(MODULE = "pms", REMARK = "批量删除优惠券和产品分类关系表")
|
|||
// @PreAuthorize("hasAuthority('sms:SmsCouponProductCategoryRelation:delete')")
|
|||
public Object deleteBatch(@RequestParam("ids") List<Long> ids) { |
|||
boolean count = ISmsCouponProductCategoryRelationService.removeByIds(ids); |
|||
if (count) { |
|||
return new CommonResult().success(count); |
|||
} else { |
|||
return new CommonResult().failed(); |
|||
} |
|||
} |
|||
|
|||
} |
@ -0,0 +1,17 @@ |
|||
package com.yxt.yythmall.biz.smscoupon; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCouponProductCategoryRelation; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
|
|||
/** |
|||
* <p> |
|||
* 优惠券和产品分类关系表 Mapper 接口 |
|||
* </p> |
|||
* |
|||
* @author zscat |
|||
* @since 2019-04-19 |
|||
*/@Mapper |
|||
public interface SmsCouponProductCategoryRelationMapper extends BaseMapper<SmsCouponProductCategoryRelation> { |
|||
|
|||
} |
@ -0,0 +1,19 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.biz.smscoupon.SmsCouponProductCategoryRelationMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.api.sms.entity.SmsCouponProductCategoryRelation"> |
|||
<id column="id" property="id"/> |
|||
<result column="coupon_id" property="couponId"/> |
|||
<result column="product_category_id" property="productCategoryId"/> |
|||
<result column="product_category_name" property="productCategoryName"/> |
|||
<result column="parent_category_name" property="parentCategoryName"/> |
|||
</resultMap> |
|||
|
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, coupon_id, product_category_id, product_category_name, parent_category_name |
|||
</sql> |
|||
|
|||
</mapper> |
@ -0,0 +1,18 @@ |
|||
package com.yxt.yythmall.biz.smscoupon; |
|||
|
|||
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCouponProductCategoryRelation; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
/** |
|||
* <p> |
|||
* 优惠券和产品分类关系表 服务实现类 |
|||
* </p> |
|||
* |
|||
* @author zscat |
|||
* @since 2019-04-19 |
|||
*/ |
|||
@Service |
|||
public class SmsCouponProductCategoryRelationServiceImpl extends ServiceImpl<SmsCouponProductCategoryRelationMapper, SmsCouponProductCategoryRelation> implements ISmsCouponProductCategoryRelationService { |
|||
|
|||
} |
@ -0,0 +1,132 @@ |
|||
package com.yxt.yythmall.biz.smscoupon; |
|||
|
|||
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
|||
import com.baomidou.mybatisplus.extension.plugins.pagination.Page; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCouponProductRelation; |
|||
import com.zscat.mallplus.utils.CommonResult; |
|||
import com.zscat.mallplus.utils.ValidatorUtils; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import io.swagger.annotations.ApiParam; |
|||
import lombok.extern.slf4j.Slf4j; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import javax.annotation.Resource; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* <p> |
|||
* 优惠券和产品的关系表 |
|||
* </p> |
|||
* |
|||
* @author zscat |
|||
* @since 2019-04-19 |
|||
*/ |
|||
@Slf4j |
|||
@RestController |
|||
@Api(tags = "SmsCouponProductRelationController", description = "优惠券和产品的关系表管理") |
|||
@RequestMapping("/sms/SmsCouponProductRelation") |
|||
public class SmsCouponProductRelationController { |
|||
@Resource |
|||
private com.yxt.yythmall.biz.smscoupon.ISmsCouponProductRelationService ISmsCouponProductRelationService; |
|||
|
|||
// @SysLog(MODULE = "sms", REMARK = "根据条件查询所有优惠券和产品的关系表列表")
|
|||
@ApiOperation("根据条件查询所有优惠券和产品的关系表列表") |
|||
@GetMapping(value = "/list") |
|||
// @PreAuthorize("hasAuthority('sms:SmsCouponProductRelation:read')")
|
|||
public Object getSmsCouponProductRelationByPage(SmsCouponProductRelation entity, |
|||
@RequestParam(value = "pageNum", defaultValue = "1") Integer pageNum, |
|||
@RequestParam(value = "pageSize", defaultValue = "10") Integer pageSize |
|||
) { |
|||
try { |
|||
return new CommonResult().success(ISmsCouponProductRelationService.page(new Page<SmsCouponProductRelation>(pageNum, pageSize), new QueryWrapper<>(entity))); |
|||
} catch (Exception e) { |
|||
log.error("根据条件查询所有优惠券和产品的关系表列表:%s", e.getMessage(), e); |
|||
} |
|||
return new CommonResult().failed(); |
|||
} |
|||
|
|||
// @SysLog(MODULE = "sms", REMARK = "保存优惠券和产品的关系表")
|
|||
@ApiOperation("保存优惠券和产品的关系表") |
|||
@PostMapping(value = "/create") |
|||
// @PreAuthorize("hasAuthority('sms:SmsCouponProductRelation:create')")
|
|||
public Object saveSmsCouponProductRelation(@RequestBody SmsCouponProductRelation entity) { |
|||
try { |
|||
if (ISmsCouponProductRelationService.save(entity)) { |
|||
return new CommonResult().success(); |
|||
} |
|||
} catch (Exception e) { |
|||
log.error("保存优惠券和产品的关系表:%s", e.getMessage(), e); |
|||
return new CommonResult().failed(); |
|||
} |
|||
return new CommonResult().failed(); |
|||
} |
|||
|
|||
// @SysLog(MODULE = "sms", REMARK = "更新优惠券和产品的关系表")
|
|||
@ApiOperation("更新优惠券和产品的关系表") |
|||
@PostMapping(value = "/update/{id}") |
|||
// @PreAuthorize("hasAuthority('sms:SmsCouponProductRelation:update')")
|
|||
public Object updateSmsCouponProductRelation(@RequestBody SmsCouponProductRelation entity) { |
|||
try { |
|||
if (ISmsCouponProductRelationService.updateById(entity)) { |
|||
return new CommonResult().success(); |
|||
} |
|||
} catch (Exception e) { |
|||
log.error("更新优惠券和产品的关系表:%s", e.getMessage(), e); |
|||
return new CommonResult().failed(); |
|||
} |
|||
return new CommonResult().failed(); |
|||
} |
|||
|
|||
// @SysLog(MODULE = "sms", REMARK = "删除优惠券和产品的关系表")
|
|||
@ApiOperation("删除优惠券和产品的关系表") |
|||
@GetMapping(value = "/delete/{id}") |
|||
// @PreAuthorize("hasAuthority('sms:SmsCouponProductRelation:delete')")
|
|||
public Object deleteSmsCouponProductRelation(@ApiParam("优惠券和产品的关系表id") @PathVariable Long id) { |
|||
try { |
|||
if (ValidatorUtils.empty(id)) { |
|||
return new CommonResult().paramFailed("优惠券和产品的关系表id"); |
|||
} |
|||
if (ISmsCouponProductRelationService.removeById(id)) { |
|||
return new CommonResult().success(); |
|||
} |
|||
} catch (Exception e) { |
|||
log.error("删除优惠券和产品的关系表:%s", e.getMessage(), e); |
|||
return new CommonResult().failed(); |
|||
} |
|||
return new CommonResult().failed(); |
|||
} |
|||
|
|||
// @SysLog(MODULE = "sms", REMARK = "给优惠券和产品的关系表分配优惠券和产品的关系表")
|
|||
@ApiOperation("查询优惠券和产品的关系表明细") |
|||
@GetMapping(value = "/{id}") |
|||
// @PreAuthorize("hasAuthority('sms:SmsCouponProductRelation:read')")
|
|||
public Object getSmsCouponProductRelationById(@ApiParam("优惠券和产品的关系表id") @PathVariable Long id) { |
|||
try { |
|||
if (ValidatorUtils.empty(id)) { |
|||
return new CommonResult().paramFailed("优惠券和产品的关系表id"); |
|||
} |
|||
SmsCouponProductRelation coupon = ISmsCouponProductRelationService.getById(id); |
|||
return new CommonResult().success(coupon); |
|||
} catch (Exception e) { |
|||
log.error("查询优惠券和产品的关系表明细:%s", e.getMessage(), e); |
|||
return new CommonResult().failed(); |
|||
} |
|||
|
|||
} |
|||
|
|||
@ApiOperation(value = "批量删除优惠券和产品的关系表") |
|||
@RequestMapping(value = "/delete/batch", method = RequestMethod.GET) |
|||
@ResponseBody |
|||
// @SysLog(MODULE = "pms", REMARK = "批量删除优惠券和产品的关系表")
|
|||
// @PreAuthorize("hasAuthority('sms:SmsCouponProductRelation:delete')")
|
|||
public Object deleteBatch(@RequestParam("ids") List<Long> ids) { |
|||
boolean count = ISmsCouponProductRelationService.removeByIds(ids); |
|||
if (count) { |
|||
return new CommonResult().success(count); |
|||
} else { |
|||
return new CommonResult().failed(); |
|||
} |
|||
} |
|||
|
|||
} |
@ -0,0 +1,17 @@ |
|||
package com.yxt.yythmall.biz.smscoupon; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCouponProductRelation; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
|
|||
/** |
|||
* <p> |
|||
* 优惠券和产品的关系表 Mapper 接口 |
|||
* </p> |
|||
* |
|||
* @author zscat |
|||
* @since 2019-04-19 |
|||
*/@Mapper |
|||
public interface SmsCouponProductRelationMapper extends BaseMapper<SmsCouponProductRelation> { |
|||
|
|||
} |
@ -0,0 +1,19 @@ |
|||
<?xml version="1.0" encoding="UTF-8"?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.biz.smscoupon.SmsCouponProductRelationMapper"> |
|||
|
|||
<!-- 通用查询映射结果 --> |
|||
<resultMap id="BaseResultMap" type="com.yxt.yythmall.api.sms.entity.SmsCouponProductRelation"> |
|||
<id column="id" property="id"/> |
|||
<result column="coupon_id" property="couponId"/> |
|||
<result column="product_id" property="productId"/> |
|||
<result column="product_name" property="productName"/> |
|||
<result column="product_sn" property="productSn"/> |
|||
</resultMap> |
|||
|
|||
<!-- 通用查询结果列 --> |
|||
<sql id="Base_Column_List"> |
|||
id, coupon_id, product_id, product_name, product_sn |
|||
</sql> |
|||
|
|||
</mapper> |
@ -0,0 +1,18 @@ |
|||
package com.yxt.yythmall.biz.smscoupon; |
|||
|
|||
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCouponProductRelation; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
/** |
|||
* <p> |
|||
* 优惠券和产品的关系表 服务实现类 |
|||
* </p> |
|||
* |
|||
* @author zscat |
|||
* @since 2019-04-19 |
|||
*/ |
|||
@Service |
|||
public class SmsCouponProductRelationServiceImpl extends ServiceImpl<SmsCouponProductRelationMapper, SmsCouponProductRelation> implements ISmsCouponProductRelationService { |
|||
|
|||
} |
@ -0,0 +1,105 @@ |
|||
package com.yxt.yythmall.biz.smscoupon; |
|||
|
|||
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
|||
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCoupon; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCouponProductCategoryRelation; |
|||
import com.yxt.yythmall.api.sms.entity.SmsCouponProductRelation; |
|||
import com.yxt.yythmall.api.sms.vo.SmsCouponParam; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
import javax.annotation.Resource; |
|||
|
|||
/** |
|||
* <p> |
|||
* 优惠卷表 服务实现类 |
|||
* </p> |
|||
* |
|||
* @author zscat |
|||
* @since 2019-04-19 |
|||
*/ |
|||
@Service |
|||
public class SmsCouponServiceImpl extends ServiceImpl<SmsCouponMapper, SmsCoupon> implements ISmsCouponService { |
|||
|
|||
@Resource |
|||
private SmsCouponMapper couponMapper; |
|||
@Resource |
|||
private SmsCouponProductRelationMapper productRelationMapper; |
|||
@Resource |
|||
private SmsCouponProductCategoryRelationMapper productCategoryRelationMapper; |
|||
@Resource |
|||
private ISmsCouponProductRelationService productRelationDao; |
|||
@Resource |
|||
private ISmsCouponProductCategoryRelationService productCategoryRelationDao; |
|||
|
|||
@Override |
|||
public boolean saves(SmsCouponParam couponParam) { |
|||
couponParam.setCount(couponParam.getPublishCount()); |
|||
couponParam.setUseCount(0); |
|||
couponParam.setReceiveCount(0); |
|||
//插入优惠券表
|
|||
int count = couponMapper.insert(couponParam); |
|||
//插入优惠券和商品关系表
|
|||
if (couponParam.getUseType().equals(2)) { |
|||
for (SmsCouponProductRelation productRelation : couponParam.getProductRelationList()) { |
|||
productRelation.setCouponId(couponParam.getId()); |
|||
} |
|||
productRelationDao.saveBatch(couponParam.getProductRelationList()); |
|||
} |
|||
//插入优惠券和商品分类关系表
|
|||
if (couponParam.getUseType().equals(1)) { |
|||
for (SmsCouponProductCategoryRelation couponProductCategoryRelation : couponParam.getProductCategoryRelationList()) { |
|||
couponProductCategoryRelation.setCouponId(couponParam.getId()); |
|||
} |
|||
productCategoryRelationDao.saveBatch(couponParam.getProductCategoryRelationList()); |
|||
} |
|||
return true; |
|||
} |
|||
|
|||
@Override |
|||
public boolean updateByIds(SmsCouponParam couponParam) { |
|||
couponParam.setId(couponParam.getId()); |
|||
int count = couponMapper.updateById(couponParam); |
|||
//删除后插入优惠券和商品关系表
|
|||
if (couponParam.getUseType().equals(2)) { |
|||
for (SmsCouponProductRelation productRelation : couponParam.getProductRelationList()) { |
|||
productRelation.setCouponId(couponParam.getId()); |
|||
} |
|||
deleteProductRelation(couponParam.getId()); |
|||
productRelationDao.saveBatch(couponParam.getProductRelationList()); |
|||
} |
|||
//删除后插入优惠券和商品分类关系表
|
|||
if (couponParam.getUseType().equals(1)) { |
|||
for (SmsCouponProductCategoryRelation couponProductCategoryRelation : couponParam.getProductCategoryRelationList()) { |
|||
couponProductCategoryRelation.setCouponId(couponParam.getId()); |
|||
} |
|||
deleteProductCategoryRelation(couponParam.getId()); |
|||
productCategoryRelationDao.saveBatch(couponParam.getProductCategoryRelationList()); |
|||
} |
|||
return true; |
|||
} |
|||
|
|||
private void deleteProductCategoryRelation(Long id) { |
|||
productCategoryRelationMapper.delete(new QueryWrapper<>(new SmsCouponProductCategoryRelation()).eq("coupon_id", id)); |
|||
} |
|||
|
|||
private void deleteProductRelation(Long id) { |
|||
productRelationMapper.delete(new QueryWrapper<>(new SmsCouponProductRelation()).eq("coupon_id", id)); |
|||
} |
|||
|
|||
@Override |
|||
public int delete(Long id) { |
|||
//删除优惠券
|
|||
int count = couponMapper.deleteById(id); |
|||
//删除商品关联
|
|||
deleteProductRelation(id); |
|||
//删除商品分类关联
|
|||
deleteProductCategoryRelation(id); |
|||
return count; |
|||
} |
|||
|
|||
@Override |
|||
public SmsCouponParam getItem(Long id) { |
|||
return couponMapper.getItem(id); |
|||
} |
|||
} |
Loading…
Reference in new issue