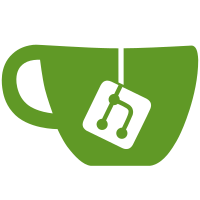
27 changed files with 4193 additions and 86 deletions
@ -0,0 +1,37 @@ |
|||
package com.yxt.anrui.buscenter.api.bussalesorderloancontract; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author Administrator |
|||
* @description |
|||
* @date 2023/9/1 11:29 |
|||
*/ |
|||
@Data |
|||
public class BusLoancontractForRepayQuery implements Query { |
|||
|
|||
@ApiModelProperty("销售部门") |
|||
private String orgName; |
|||
@ApiModelProperty("销售专员") |
|||
private String staffName; |
|||
@ApiModelProperty("分公司名称") |
|||
private String useOrgName; |
|||
@ApiModelProperty("消贷合同编号") |
|||
private String loanContractNo; // 消贷合同编号
|
|||
@ApiModelProperty("借款人名称") |
|||
private String borrowerName; // 借款人名称
|
|||
@ApiModelProperty("资方名称") |
|||
private String bankName; |
|||
@ApiModelProperty("金融产品名称") |
|||
private String policyName; // 金融产品名称
|
|||
@ApiModelProperty("组织全路径") |
|||
private String orgPath; |
|||
@ApiModelProperty("菜单sid") |
|||
private String menuSid; |
|||
@ApiModelProperty("菜单url") |
|||
private String menuUrl; |
|||
@ApiModelProperty("用户sid") |
|||
private String userSid; |
|||
} |
@ -0,0 +1,36 @@ |
|||
package com.yxt.anrui.buscenter.api.bussalesorderloancontract; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author Administrator |
|||
* @description |
|||
* @date 2023/9/1 11:21 |
|||
*/ |
|||
@Data |
|||
public class BusLoancontractForRepayVo implements Vo { |
|||
|
|||
@ApiModelProperty("销售订单sid") |
|||
private String salesOrderSid; // 销售订单sid
|
|||
@ApiModelProperty("销售部门") |
|||
private String orgName; |
|||
@ApiModelProperty("销售专员") |
|||
private String staffName; |
|||
@ApiModelProperty("分公司名称") |
|||
private String useOrgName; |
|||
@ApiModelProperty("消贷合同编号") |
|||
private String loanContractNo; // 消贷合同编号
|
|||
@ApiModelProperty("借款人名称") |
|||
private String borrowerName; // 借款人名称
|
|||
@ApiModelProperty("车架号") |
|||
private String vinNo; // 台数
|
|||
@ApiModelProperty("资方名称") |
|||
private String bankName; |
|||
@ApiModelProperty("资方合同号") |
|||
private String bankContractNo; // 资方合同号
|
|||
@ApiModelProperty("金融产品名称") |
|||
private String policyName; // 金融产品名称
|
|||
|
|||
} |
@ -1,13 +1,34 @@ |
|||
<?xml version="1.0" encoding="UTF-8" ?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.anrui.buscenter.biz.bussalesorderloancontract.BusSalesOrderLoancontractMapper"> |
|||
<!-- <where> ${ew.sqlSegment} </where>--> |
|||
<!-- ${ew.customSqlSegment} --> |
|||
<select id="selectPageVo" resultType="com.yxt.anrui.buscenter.api.bussalesorderloancontract.BusSalesOrderLoancontractVo"> |
|||
SELECT * FROM bus_sales_order_loancontract <where> ${ew.sqlSegment} </where> |
|||
<!-- <where> ${ew.sqlSegment} </where>--> |
|||
<!-- ${ew.customSqlSegment} --> |
|||
<select id="selectPageVo" |
|||
resultType="com.yxt.anrui.buscenter.api.bussalesorderloancontract.BusSalesOrderLoancontractVo"> |
|||
SELECT * FROM bus_sales_order_loancontract |
|||
<where>${ew.sqlSegment}</where> |
|||
</select> |
|||
|
|||
<select id="selectListAllVo" resultType="com.yxt.anrui.buscenter.api.bussalesorderloancontract.BusSalesOrderLoancontractVo"> |
|||
SELECT * FROM bus_sales_order_loancontract <where> ${ew.sqlSegment} </where> |
|||
<select id="selectListAllVo" |
|||
resultType="com.yxt.anrui.buscenter.api.bussalesorderloancontract.BusSalesOrderLoancontractVo"> |
|||
SELECT * FROM bus_sales_order_loancontract |
|||
<where>${ew.sqlSegment}</where> |
|||
</select> |
|||
<select id="listPageForRepay" |
|||
resultType="com.yxt.anrui.buscenter.api.bussalesorderloancontract.BusLoancontractForRepayVo"> |
|||
SELECT |
|||
bs.sid as salesOrderSid, |
|||
bs.orgName as orgName, |
|||
bs.staffName as staffName, |
|||
bs.useOrgName as useOrgName, |
|||
co.loanCotractNo as loanContractNo, |
|||
co.borrowerName as borrowerName, |
|||
co.bankName as bankName, |
|||
co.bankContractNo as bankContractNo, |
|||
co.policyName as policyName |
|||
FROM |
|||
bus_sales_order_loancontract AS co |
|||
LEFT JOIN bus_sales_order AS bs ON co.salesOrderSid = bs.sid |
|||
<where>${ew.sqlSegment}</where> |
|||
</select> |
|||
</mapper> |
@ -0,0 +1,54 @@ |
|||
package com.yxt.anrui.riskcenter.api.loanrepaymentschedule; |
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author Administrator |
|||
* @description |
|||
* @date 2023/9/4 14:04 |
|||
*/ |
|||
@Data |
|||
public class LoanCreateSchedulePdfDto implements Dto { |
|||
@ApiModelProperty("销售订单sid") |
|||
private String salesOrderSid; |
|||
@ApiModelProperty("金融方案sid") |
|||
private String solutionsSid; |
|||
@ApiModelProperty("消贷合同编号") |
|||
private String loanContractNo; |
|||
@ApiModelProperty("借款人名称") |
|||
private String borrowerName; |
|||
@ApiModelProperty("车辆台数") |
|||
private String vehCount; |
|||
@ApiModelProperty("资方名称") |
|||
private String policyName; |
|||
@ApiModelProperty("主产品贷款金额") |
|||
private String loanAmount; |
|||
@ApiModelProperty("主产品期数") |
|||
private String mainPeriod; |
|||
@ApiModelProperty("主产品首期还款日") |
|||
private String mainRepayDate; |
|||
@ApiModelProperty("主产品首期月还") |
|||
private String mainFirstRepay; |
|||
@ApiModelProperty("主产品期间月还") |
|||
private String mainMidRepay; |
|||
@ApiModelProperty("主产品期末月还") |
|||
private String mainLastRepay; |
|||
@ApiModelProperty("其他融资方") |
|||
private String otherPolicyName; |
|||
@ApiModelProperty("其他融贷款金额") |
|||
private String otherAmount; |
|||
@ApiModelProperty("其他融期数") |
|||
private String otherPeriod; |
|||
@ApiModelProperty("其他融首期还款日") |
|||
private String otherRepayDate; |
|||
@ApiModelProperty("其他融首期月还") |
|||
private String otherFirstRepay; |
|||
@ApiModelProperty("其他融期间月还") |
|||
private String otherMidRepay; |
|||
@ApiModelProperty("其他融期末月还") |
|||
private String otherLastRepay; |
|||
@ApiModelProperty("是否有其他融 0没有1有") |
|||
private String isOtherPolicy; |
|||
} |
@ -0,0 +1,59 @@ |
|||
package com.yxt.anrui.riskcenter.api.loanrepaymentschedule; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.math.BigDecimal; |
|||
import java.util.Date; |
|||
|
|||
/** |
|||
* @author Administrator |
|||
* @description |
|||
* @date 2023/9/1 15:01 |
|||
*/ |
|||
@Data |
|||
public class LoanCreateSchedulePdfVo implements Vo { |
|||
|
|||
@ApiModelProperty("销售订单sid") |
|||
private String salesOrderSid; |
|||
@ApiModelProperty("金融方案sid") |
|||
private String solutionsSid; |
|||
@ApiModelProperty("消贷合同编号") |
|||
private String loanContractNo; |
|||
@ApiModelProperty("借款人名称") |
|||
private String borrowerName; |
|||
@ApiModelProperty("车辆台数") |
|||
private String vehCount; |
|||
@ApiModelProperty("资方名称") |
|||
private String policyName; |
|||
@ApiModelProperty("主产品贷款金额") |
|||
private String loanAmount; |
|||
@ApiModelProperty("主产品期数") |
|||
private String mainPeriod; |
|||
@ApiModelProperty("主产品首期还款日") |
|||
private String mainRepayDate; |
|||
@ApiModelProperty("主产品首期月还") |
|||
private String mainFirstRepay; |
|||
@ApiModelProperty("主产品期间月还") |
|||
private String mainMidRepay; |
|||
@ApiModelProperty("主产品期末月还") |
|||
private String mainLastRepay; |
|||
@ApiModelProperty("其他融资方") |
|||
private String otherPolicyName; |
|||
@ApiModelProperty("其他融贷款金额") |
|||
private String otherAmount; |
|||
@ApiModelProperty("其他融期数") |
|||
private String otherPeriod; |
|||
@ApiModelProperty("其他融首期还款日") |
|||
private String otherRepayDate; |
|||
@ApiModelProperty("其他融首期月还") |
|||
private String otherFirstRepay; |
|||
@ApiModelProperty("其他融期间月还") |
|||
private String otherMidRepay; |
|||
@ApiModelProperty("其他融期末月还") |
|||
private String otherLastRepay; |
|||
@ApiModelProperty("是否有其他融 0没有1有") |
|||
private String isOtherPolicy; |
|||
|
|||
} |
@ -0,0 +1,28 @@ |
|||
package com.yxt.anrui.riskcenter.api.loanrepaymentschedule; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.ArrayList; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author Administrator |
|||
* @description |
|||
* @date 2023/9/5 14:34 |
|||
*/ |
|||
@Data |
|||
public class LoanCreateScheduleVinOneVo implements Vo { |
|||
@ApiModelProperty("借款人名称") |
|||
private String borrowerName; |
|||
@ApiModelProperty("车架号") |
|||
private String vinNo; |
|||
@ApiModelProperty("是否有其他融 0没有1有") |
|||
private String isOtherPolicy; |
|||
@ApiModelProperty("主产品首期还款日") |
|||
private String mainRepayDate; |
|||
@ApiModelProperty("其他融首期还款日") |
|||
private String otherRepayDate; |
|||
private List<LoanCreateScheduleVinsListVo> scheduleVins = new ArrayList<>(); |
|||
} |
@ -0,0 +1,25 @@ |
|||
package com.yxt.anrui.riskcenter.api.loanrepaymentschedule; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @author Administrator |
|||
* @description |
|||
* @date 2023/9/4 14:15 |
|||
*/ |
|||
@Data |
|||
public class LoanCreateScheduleVinsListVo implements Vo { |
|||
|
|||
@ApiModelProperty("期数") |
|||
private String period; |
|||
@ApiModelProperty("还款月份") |
|||
private String repayMonth; |
|||
@ApiModelProperty("主产品月还") |
|||
private String mainRepay; |
|||
@ApiModelProperty("其他融期间月还") |
|||
private String otherRepay; |
|||
@ApiModelProperty("合计") |
|||
private String amount; |
|||
} |
@ -0,0 +1,37 @@ |
|||
package com.yxt.anrui.riskcenter.api.loanrepaymentschedule; |
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.ArrayList; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author Administrator |
|||
* @description |
|||
* @date 2023/9/4 14:11 |
|||
*/ |
|||
@Data |
|||
public class LoanCreateScheduleVinsVo implements Vo { |
|||
@ApiModelProperty("销售订单sid") |
|||
private String salesOrderSid; |
|||
@ApiModelProperty("消贷合同编号") |
|||
private String loanContractNo; |
|||
@ApiModelProperty("借款人名称") |
|||
private String borrowerName; |
|||
@ApiModelProperty("资方") |
|||
private String bankName; |
|||
@ApiModelProperty("车辆台数") |
|||
private String vehCount; |
|||
@ApiModelProperty("车架号") |
|||
private String vinNo; |
|||
@ApiModelProperty("是否有其他融 0没有1有") |
|||
private String isOtherPolicy; |
|||
@ApiModelProperty("主产品首期还款日") |
|||
private String mainRepayDate; |
|||
@ApiModelProperty("其他融首期还款日") |
|||
private String otherRepayDate; |
|||
private List<LoanCreateScheduleVinsListVo> scheduleVins = new ArrayList<>(); |
|||
|
|||
} |
@ -0,0 +1,60 @@ |
|||
package com.yxt.anrui.riskcenter.api.loanrepaymentschedule; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.math.BigDecimal; |
|||
import java.util.Date; |
|||
|
|||
/** |
|||
* @author Administrator |
|||
* @description |
|||
* @date 2023/8/16 14:34 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "还款计划表", description = "还款计划表") |
|||
@TableName("loan_repayment_schedule") |
|||
public class LoanRepaymentSchedule extends BaseEntity { |
|||
@ApiModelProperty("销售订单sid") |
|||
private String salesOrderSid; |
|||
@ApiModelProperty("借款人sid") |
|||
private String borrowerSid; |
|||
@ApiModelProperty("借款人名称") |
|||
private String borrowerName; |
|||
@ApiModelProperty("车架号") |
|||
private String vinNo; |
|||
@ApiModelProperty("车辆台数") |
|||
private String vehCount; |
|||
@ApiModelProperty("消贷合同编号") |
|||
private String loanContractNo; |
|||
@ApiModelProperty("资方名称") |
|||
private String bankName; |
|||
@ApiModelProperty("主产品期数") |
|||
private String mainPeriod; |
|||
@ApiModelProperty("主产品首期还款日") |
|||
private Date mainRepayDate; |
|||
@ApiModelProperty("主产品首期月还") |
|||
private BigDecimal mainFirstRepay; |
|||
@ApiModelProperty("主产品期间月还") |
|||
private BigDecimal mainMidRepay; |
|||
@ApiModelProperty("主产品期末月还") |
|||
private BigDecimal mainLastRepay; |
|||
@ApiModelProperty("其他融期数") |
|||
private String otherPeriod; |
|||
@ApiModelProperty("其他融首期还款日") |
|||
private Date otherRepayDate; |
|||
@ApiModelProperty("其他融首期月还") |
|||
private BigDecimal otherFirstRepay; |
|||
@ApiModelProperty("其他融期间月还") |
|||
private BigDecimal otherMidRepay; |
|||
@ApiModelProperty("其他融期末月还") |
|||
private BigDecimal otherLastRepay; |
|||
@ApiModelProperty("是否有其他融 0没有1有") |
|||
private String isOtherPolicy; |
|||
@ApiModelProperty("还款计划单下载地址") |
|||
private String schedulePath; |
|||
|
|||
} |
@ -0,0 +1,47 @@ |
|||
package com.yxt.anrui.riskcenter.api.loanrepaymentschedule; |
|||
|
|||
import com.yxt.common.core.result.ResultBean; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import java.util.List; |
|||
|
|||
|
|||
/** |
|||
* @description: 还款计划表 |
|||
* @author: fan |
|||
* @date: 2023/7/6 |
|||
**/ |
|||
@Api(tags = "还款计划表") |
|||
@FeignClient( |
|||
contextId = "anrui-riskcenter-LoanRepaymentSchedule", |
|||
name = "anrui-riskcenter", |
|||
path = "v1/loanrepaymentschedule", |
|||
fallback = LoanRepaymentScheduleFeignFallback.class) |
|||
public interface LoanRepaymentScheduleFeign { |
|||
|
|||
|
|||
@ApiOperation("根据销售订单SID生成还款计划表回显") |
|||
@GetMapping("/loanCreateSchedule") |
|||
@ResponseBody |
|||
public ResultBean<LoanCreateSchedulePdfVo> loanCreateSchedule(@RequestParam("salesOrderSid") String salesOrderSid); |
|||
|
|||
@ApiOperation("生成还款计划表") |
|||
@PostMapping("/loanCreateSchedulePdf") |
|||
@ResponseBody |
|||
public ResultBean<String> loanCreateSchedulePdf(@RequestBody LoanCreateSchedulePdfVo dto); |
|||
|
|||
@ApiOperation("查看多台还款计划表") |
|||
@GetMapping("/viewVinsSchedule") |
|||
@ResponseBody |
|||
public ResultBean<LoanCreateScheduleVinsVo> viewVinsSchedule(@RequestParam("salesOrderSid") String salesOrderSid); |
|||
|
|||
@ApiOperation("查看单台还款计划表") |
|||
@GetMapping("/viewVinOneSchedule") |
|||
@ResponseBody |
|||
public ResultBean<List<LoanCreateScheduleVinOneVo>> viewVinOneSchedule(@RequestParam("salesOrderSid") String salesOrderSid); |
|||
|
|||
|
|||
} |
@ -0,0 +1,35 @@ |
|||
package com.yxt.anrui.riskcenter.api.loanrepaymentschedule; |
|||
|
|||
import com.yxt.common.core.result.ResultBean; |
|||
import org.springframework.stereotype.Component; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author Administrator |
|||
* @description |
|||
* @date 2023/8/16 14:36 |
|||
*/ |
|||
@Component |
|||
public class LoanRepaymentScheduleFeignFallback implements LoanRepaymentScheduleFeign { |
|||
|
|||
@Override |
|||
public ResultBean<LoanCreateSchedulePdfVo> loanCreateSchedule(String salesOrderSid) { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<String> loanCreateSchedulePdf(LoanCreateSchedulePdfVo dto) { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<LoanCreateScheduleVinsVo> viewVinsSchedule(String salesOrderSid) { |
|||
return null; |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<LoanCreateScheduleVinOneVo>> viewVinOneSchedule(String salesOrderSid) { |
|||
return null; |
|||
} |
|||
} |
@ -0,0 +1,24 @@ |
|||
package com.yxt.anrui.riskcenter.biz.loanrepaymentschedule; |
|||
|
|||
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import com.baomidou.mybatisplus.core.toolkit.Constants; |
|||
import com.yxt.anrui.riskcenter.api.loanparameter.LoanParameter; |
|||
import com.yxt.anrui.riskcenter.api.loanparameter.LoanParameterVo; |
|||
import com.yxt.anrui.riskcenter.api.loanrepaymentschedule.LoanRepaymentSchedule; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
import org.apache.ibatis.annotations.Param; |
|||
|
|||
|
|||
/** |
|||
* @description: |
|||
* @author: fan |
|||
* @date: 2023/8/21 |
|||
**/ |
|||
@Mapper |
|||
public interface LoanRepaymentScheduleMapper extends BaseMapper<LoanRepaymentSchedule> { |
|||
|
|||
|
|||
LoanRepaymentSchedule selectByOrderSid(String salesOrderSid); |
|||
} |
@ -0,0 +1,11 @@ |
|||
<?xml version="1.0" encoding="UTF-8" ?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.anrui.riskcenter.biz.loanrepaymentschedule.LoanRepaymentScheduleMapper"> |
|||
|
|||
<select id="selectByOrderSid" |
|||
resultType="com.yxt.anrui.riskcenter.api.loanrepaymentschedule.LoanRepaymentSchedule"> |
|||
select * |
|||
from loan_repayment_schedule |
|||
where salesOrderSid = #{salesOrderSid} |
|||
</select> |
|||
</mapper> |
@ -0,0 +1,45 @@ |
|||
package com.yxt.anrui.riskcenter.biz.loanrepaymentschedule; |
|||
|
|||
|
|||
import com.yxt.anrui.riskcenter.api.loanrepaymentschedule.*; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import io.swagger.annotations.Api; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.web.bind.annotation.RequestMapping; |
|||
import org.springframework.web.bind.annotation.RestController; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: fan |
|||
* @date: 2023/8/21 |
|||
**/ |
|||
@Api(tags = "还款计划表") |
|||
@RestController |
|||
@RequestMapping("v1/loanrepaymentschedule") |
|||
public class LoanRepaymentScheduleRest implements LoanRepaymentScheduleFeign { |
|||
|
|||
@Autowired |
|||
private LoanRepaymentScheduleService loanRepaymentScheduleService; |
|||
|
|||
@Override |
|||
public ResultBean<LoanCreateSchedulePdfVo> loanCreateSchedule(String salesOrderSid) { |
|||
return loanRepaymentScheduleService.loanCreateSchedule(salesOrderSid); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<String> loanCreateSchedulePdf(LoanCreateSchedulePdfVo dto) { |
|||
return loanRepaymentScheduleService.loanCreateSchedulePdf(dto); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<LoanCreateScheduleVinsVo> viewVinsSchedule(String salesOrderSid) { |
|||
return loanRepaymentScheduleService.viewVinsSchedule(salesOrderSid); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<LoanCreateScheduleVinOneVo>> viewVinOneSchedule(String salesOrderSid) { |
|||
return loanRepaymentScheduleService.viewVinOneSchedule(salesOrderSid); |
|||
} |
|||
} |
@ -0,0 +1,510 @@ |
|||
package com.yxt.anrui.riskcenter.biz.loanrepaymentschedule; |
|||
|
|||
import cn.hutool.core.bean.BeanUtil; |
|||
import cn.hutool.core.date.DateUtil; |
|||
import com.yxt.anrui.buscenter.api.bussalesorderloancontract.BusSalesOrderLoancontract; |
|||
import com.yxt.anrui.buscenter.api.bussalesorderloancontract.BusSalesOrderLoancontractFeign; |
|||
import com.yxt.anrui.buscenter.api.bussalesordervehicle.BusSalesOrderVehicle; |
|||
import com.yxt.anrui.buscenter.api.bussalesordervehicle.BusSalesOrderVehicleFeign; |
|||
import com.yxt.anrui.buscenter.api.busvehicledatahandover.BusDataListPdfVo; |
|||
import com.yxt.anrui.buscenter.api.busvehicledatahandover.BusVehicleDataHandoverPdfVo; |
|||
import com.yxt.anrui.portal.api.sysorganization.SysOrganizationFeign; |
|||
import com.yxt.anrui.portal.api.sysstafforg.SysStaffOrgFeign; |
|||
import com.yxt.anrui.portal.api.sysuser.SysUserFeign; |
|||
import com.yxt.anrui.riskcenter.api.loanrepaymentschedule.*; |
|||
import com.yxt.anrui.riskcenter.api.loansolutions.LoanSolutions; |
|||
import com.yxt.anrui.riskcenter.api.loansolutionsotherpolicy.LoanSolutionsOtherpolicy; |
|||
import com.yxt.anrui.riskcenter.biz.loansolutions.LoanSolutionsService; |
|||
import com.yxt.anrui.riskcenter.biz.loansolutionsotherpolicy.LoanSolutionsOtherpolicyService; |
|||
import com.yxt.common.base.config.component.DocPdfComponent; |
|||
import com.yxt.common.base.service.MybatisBaseService; |
|||
import com.yxt.common.base.utils.StringUtils; |
|||
import com.yxt.common.base.utils.WordConvertUtils; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.stereotype.Service; |
|||
import org.springframework.transaction.annotation.Transactional; |
|||
|
|||
import java.io.File; |
|||
import java.io.InputStream; |
|||
import java.math.BigDecimal; |
|||
import java.text.SimpleDateFormat; |
|||
import java.util.*; |
|||
import java.util.stream.Collectors; |
|||
|
|||
|
|||
/** |
|||
* @description: |
|||
* @author: fan |
|||
* @date: 2023/8/21 |
|||
**/ |
|||
@Service |
|||
public class LoanRepaymentScheduleService extends MybatisBaseService<LoanRepaymentScheduleMapper, LoanRepaymentSchedule> { |
|||
|
|||
@Autowired |
|||
private SysUserFeign sysUserFeign; |
|||
@Autowired |
|||
private SysStaffOrgFeign sysStaffOrgFeign; |
|||
@Autowired |
|||
private SysOrganizationFeign sysOrganizationFeign; |
|||
@Autowired |
|||
private BusSalesOrderLoancontractFeign busSalesOrderLoancontractFeign; |
|||
@Autowired |
|||
private LoanSolutionsService loanSolutionsService; |
|||
@Autowired |
|||
private LoanSolutionsOtherpolicyService loanSolutionsOtherpolicyService; |
|||
@Autowired |
|||
private BusSalesOrderVehicleFeign busSalesOrderVehicleFeign; |
|||
@Autowired |
|||
private DocPdfComponent docPdfComponent; |
|||
|
|||
/** |
|||
* 根据销售订单SID生成还款计划表回显 |
|||
* |
|||
* @param salesOrderSid |
|||
* @return |
|||
*/ |
|||
public ResultBean<LoanCreateSchedulePdfVo> loanCreateSchedule(String salesOrderSid) { |
|||
ResultBean<LoanCreateSchedulePdfVo> rb = ResultBean.fireFail(); |
|||
LoanCreateSchedulePdfVo vo = new LoanCreateSchedulePdfVo(); |
|||
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); |
|||
vo.setSalesOrderSid(salesOrderSid); |
|||
BusSalesOrderLoancontract loancontract = busSalesOrderLoancontractFeign.fetchDetailsByOrderSid(salesOrderSid).getData(); |
|||
if (null != loancontract) { |
|||
// 消贷合同编号
|
|||
if (StringUtils.isNotBlank(loancontract.getLoanCotractNo())) { |
|||
vo.setLoanContractNo(loancontract.getLoanCotractNo()); |
|||
} |
|||
// 贷款人车辆台数
|
|||
if (null != (loancontract.getVehCount())) { |
|||
vo.setVehCount(loancontract.getVehCount().toString()); |
|||
} |
|||
// 贷款人
|
|||
if (StringUtils.isNotBlank(loancontract.getBorrowerName())) { |
|||
vo.setBorrowerName(loancontract.getBorrowerName()); |
|||
} |
|||
} |
|||
LoanSolutions loanSolutions = loanSolutionsService.selectBySaleOrderSid(salesOrderSid); |
|||
if (null != loanSolutions) { |
|||
// 产品方案sid
|
|||
vo.setSolutionsSid(loanSolutions.getSid()); |
|||
// 主金融产品
|
|||
if (StringUtils.isNotBlank(loanSolutions.getPolicyName())) { |
|||
vo.setPolicyName(loanSolutions.getPolicyName()); |
|||
} |
|||
// 主产品贷款金额
|
|||
if (null != loanSolutions.getLoanAmount()) { |
|||
vo.setLoanAmount(loanSolutions.getLoanAmount().toString()); |
|||
} |
|||
// 主产品期数
|
|||
if (StringUtils.isNotBlank(loanSolutions.getLoanPeriod())) { |
|||
vo.setMainPeriod(loanSolutions.getLoanPeriod()); |
|||
} |
|||
LoanRepaymentSchedule schedule = baseMapper.selectByOrderSid(salesOrderSid); |
|||
if (null != schedule) { |
|||
// 主产品首期还款日
|
|||
if (null != schedule.getMainRepayDate()) { |
|||
vo.setMainRepayDate(sdf.format(schedule.getMainRepayDate())); |
|||
} |
|||
// 主产品首期月还
|
|||
if (null != schedule.getMainFirstRepay()) { |
|||
vo.setMainFirstRepay(schedule.getMainFirstRepay().toString()); |
|||
} |
|||
// 主产品期间月还
|
|||
if (null != schedule.getMainMidRepay()) { |
|||
vo.setMainMidRepay(schedule.getMainMidRepay().toString()); |
|||
} |
|||
// 主产品期末月还
|
|||
if (null != schedule.getMainLastRepay()) { |
|||
vo.setMainLastRepay(schedule.getMainLastRepay().toString()); |
|||
} |
|||
} |
|||
//查看是否有其他融
|
|||
LoanSolutionsOtherpolicy otherpolicy = loanSolutionsOtherpolicyService.selectByLoanSid(loanSolutions.getSid()); |
|||
if (null != otherpolicy) { |
|||
if (StringUtils.isNotBlank(otherpolicy.getOtherPolicyPeriod())) { |
|||
vo.setIsOtherPolicy("1"); |
|||
// 其他融产品
|
|||
if (StringUtils.isNotBlank(otherpolicy.getOtherPolicyName())) { |
|||
vo.setOtherPolicyName(otherpolicy.getOtherPolicyName()); |
|||
} |
|||
// 其他融贷款金额
|
|||
if (null != otherpolicy.getOtherPolicyAmount()) { |
|||
vo.setOtherAmount(otherpolicy.getOtherPolicyAmount().toString()); |
|||
} |
|||
// 其他融期数
|
|||
if (StringUtils.isNotBlank(otherpolicy.getOtherPolicyPeriod())) { |
|||
vo.setOtherPeriod(otherpolicy.getOtherPolicyPeriod()); |
|||
} |
|||
if (null != schedule) { |
|||
// 其他融首期还款日
|
|||
if (null != schedule.getOtherRepayDate()) { |
|||
vo.setOtherRepayDate(sdf.format(schedule.getOtherRepayDate())); |
|||
} |
|||
// 其他融首期月还
|
|||
if (null != schedule.getOtherFirstRepay()) { |
|||
vo.setOtherFirstRepay(schedule.getOtherFirstRepay().toString()); |
|||
} |
|||
// 其他融期间月还
|
|||
if (null != schedule.getOtherMidRepay()) { |
|||
vo.setOtherMidRepay(schedule.getOtherMidRepay().toString()); |
|||
} |
|||
// 其他融期末月还
|
|||
if (null != schedule.getOtherLastRepay()) { |
|||
vo.setOtherLastRepay(schedule.getOtherLastRepay().toString()); |
|||
} |
|||
} |
|||
} else { |
|||
vo.setIsOtherPolicy("0"); |
|||
} |
|||
} |
|||
} |
|||
return rb.success().setData(vo); |
|||
} |
|||
|
|||
/** |
|||
* 生成还款计划表pdf |
|||
* |
|||
* @param dto |
|||
* @return |
|||
*/ |
|||
@Transactional(rollbackFor = Exception.class) |
|||
public ResultBean<String> loanCreateSchedulePdf(LoanCreateSchedulePdfVo dto) { |
|||
ResultBean<String> rb = ResultBean.fireFail(); |
|||
LoanRepaymentSchedule loanRepaymentSchedule = baseMapper.selectByOrderSid(dto.getSalesOrderSid()); |
|||
if (StringUtils.isBlank(dto.getMainRepayDate())) { |
|||
return rb.setMsg("请选择主金融产品首期还款日!"); |
|||
} |
|||
if (StringUtils.isBlank(dto.getMainFirstRepay())) { |
|||
return rb.setMsg("请填写主金融产品首期月还!"); |
|||
} |
|||
if (StringUtils.isBlank(dto.getMainMidRepay())) { |
|||
return rb.setMsg("请填写主金融产品期间月还!"); |
|||
} |
|||
if (StringUtils.isBlank(dto.getMainLastRepay())) { |
|||
return rb.setMsg("请填写主金融产品末期月还!"); |
|||
} |
|||
if (dto.getIsOtherPolicy().equals("1")) { |
|||
if (StringUtils.isBlank(dto.getOtherRepayDate())) { |
|||
return rb.setMsg("请选择其他融首期还款日!"); |
|||
} |
|||
if (StringUtils.isBlank(dto.getOtherFirstRepay())) { |
|||
return rb.setMsg("请填写其他融首期月还!"); |
|||
} |
|||
if (StringUtils.isBlank(dto.getOtherMidRepay())) { |
|||
return rb.setMsg("请填写其他融期间月还!"); |
|||
} |
|||
if (StringUtils.isBlank(dto.getOtherLastRepay())) { |
|||
return rb.setMsg("请填写其他融末期月还!"); |
|||
} |
|||
} |
|||
if (null != loanRepaymentSchedule) { |
|||
BeanUtil.copyProperties(dto, loanRepaymentSchedule, "id", "sid"); |
|||
baseMapper.updateById(loanRepaymentSchedule); |
|||
} else { |
|||
LoanRepaymentSchedule entity = new LoanRepaymentSchedule(); |
|||
BeanUtil.copyProperties(dto, entity, "id", "sid"); |
|||
List<BusSalesOrderVehicle> busSalesOrderVehicles = busSalesOrderVehicleFeign.selectListByOrderSid(dto.getSalesOrderSid()).getData(); |
|||
busSalesOrderVehicles.removeAll(Collections.singleton(null)); |
|||
if (!busSalesOrderVehicles.isEmpty()) { |
|||
List<String> stringVinNos = busSalesOrderVehicles.stream().map(c -> c.getLinkNo()).collect(Collectors.toList()); |
|||
if (!stringVinNos.isEmpty()) { |
|||
entity.setVinNo(String.join(",", stringVinNos)); |
|||
} |
|||
} |
|||
BusSalesOrderLoancontract loancontract = busSalesOrderLoancontractFeign.fetchDetailsByOrderSid(dto.getSalesOrderSid()).getData(); |
|||
if (null != loancontract) { |
|||
if (StringUtils.isNotBlank(loancontract.getBorrowerSid())) { |
|||
entity.setBorrowerSid(loancontract.getBorrowerSid()); |
|||
} |
|||
if (StringUtils.isNotBlank(loancontract.getBankName())) { |
|||
entity.setBankName(loancontract.getBankName()); |
|||
} |
|||
} |
|||
baseMapper.insert(entity); |
|||
} |
|||
// 生成还款计划表pdf
|
|||
String template = "/template/"; |
|||
String returnPath = ""; |
|||
LoanCreateScheduleVinsVo createScheduleVinsVo = viewVinsSchedule(dto.getSalesOrderSid()).getData(); |
|||
if (null != createScheduleVinsVo) { |
|||
String pdfPath = commonCreatePdf(createScheduleVinsVo); |
|||
String filePath = pdfPath.substring(docPdfComponent.getUploadTemplateUrl().length()); |
|||
returnPath = template + filePath; |
|||
LoanRepaymentSchedule entity = baseMapper.selectByOrderSid(dto.getSalesOrderSid()); |
|||
if (null != entity) { |
|||
entity.setSchedulePath(filePath); |
|||
baseMapper.updateById(entity); |
|||
} |
|||
} |
|||
return rb.success().setData(returnPath); |
|||
} |
|||
|
|||
/** |
|||
* 还款计划表多台回显 |
|||
* |
|||
* @param salesOrderSid |
|||
* @return |
|||
*/ |
|||
public ResultBean<LoanCreateScheduleVinsVo> viewVinsSchedule(String salesOrderSid) { |
|||
ResultBean<LoanCreateScheduleVinsVo> rb = ResultBean.fireFail(); |
|||
LoanCreateScheduleVinsVo vo = new LoanCreateScheduleVinsVo(); |
|||
BusSalesOrderLoancontract loancontract = busSalesOrderLoancontractFeign.fetchDetailsByOrderSid(salesOrderSid).getData(); |
|||
if (null != loancontract) { |
|||
if (StringUtils.isNotBlank(loancontract.getBankName())) { |
|||
vo.setBankName(loancontract.getBankName()); |
|||
} |
|||
} |
|||
LoanRepaymentSchedule schedule = baseMapper.selectByOrderSid(salesOrderSid); |
|||
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); |
|||
String mainDate = ""; |
|||
String otherDate = ""; |
|||
String isOther = ""; |
|||
BigDecimal mainF = new BigDecimal(0); |
|||
BigDecimal otherF = new BigDecimal(0); |
|||
BigDecimal mainM = new BigDecimal(0); |
|||
BigDecimal otherM = new BigDecimal(0); |
|||
BigDecimal mainL = new BigDecimal(0); |
|||
BigDecimal otherL = new BigDecimal(0); |
|||
if (null != schedule) { |
|||
BeanUtil.copyProperties(schedule, vo); |
|||
if (null != schedule.getMainRepayDate()) { |
|||
mainDate = sdf.format(schedule.getMainRepayDate()); |
|||
} |
|||
if (null != schedule.getOtherRepayDate()) { |
|||
otherDate = sdf.format(schedule.getOtherRepayDate()); |
|||
} |
|||
//主产品月还日
|
|||
if (StringUtils.isNotBlank(mainDate)) { |
|||
String[] splitMainDate = mainDate.split("-"); |
|||
String day = splitMainDate[2]; |
|||
if (day.length() == 2 && Integer.parseInt(day) >= 10) { |
|||
vo.setMainRepayDate(day); |
|||
} else { |
|||
vo.setMainRepayDate(day.replace("0", "")); |
|||
} |
|||
} |
|||
//其他融月还日
|
|||
if (StringUtils.isNotBlank(otherDate)) { |
|||
String[] splitOtherDate = otherDate.split("-"); |
|||
String day = splitOtherDate[2]; |
|||
if (day.length() == 2 && Integer.parseInt(day) >= 10) { |
|||
vo.setOtherRepayDate(day); |
|||
} else { |
|||
vo.setOtherRepayDate(day.replace("0", "")); |
|||
} |
|||
} |
|||
//月还金额
|
|||
if (null != schedule.getMainFirstRepay()) { |
|||
mainF = schedule.getMainFirstRepay(); |
|||
} |
|||
if (null != schedule.getMainMidRepay()) { |
|||
mainM = schedule.getMainMidRepay(); |
|||
} |
|||
if (null != schedule.getMainLastRepay()) { |
|||
mainL = schedule.getMainLastRepay(); |
|||
} |
|||
if (null != schedule.getOtherFirstRepay()) { |
|||
otherF = schedule.getOtherFirstRepay(); |
|||
} |
|||
if (null != schedule.getOtherMidRepay()) { |
|||
otherM = schedule.getOtherMidRepay(); |
|||
} |
|||
if (null != schedule.getOtherLastRepay()) { |
|||
otherL = schedule.getOtherLastRepay(); |
|||
} |
|||
//-----还款计划表列表信息-------
|
|||
List<LoanCreateScheduleVinsListVo> scheduleVins = new ArrayList<>(); |
|||
if (StringUtils.isNotBlank(schedule.getMainPeriod())) { |
|||
int mainPeriod = Integer.parseInt(schedule.getMainPeriod()); |
|||
if (StringUtils.isNotBlank(schedule.getIsOtherPolicy())) { |
|||
isOther = schedule.getIsOtherPolicy(); |
|||
} |
|||
String otherPer = ""; |
|||
if (StringUtils.isNotBlank(schedule.getOtherPeriod())) { |
|||
otherPer = schedule.getOtherPeriod(); |
|||
} |
|||
int otherMidPer = 0; |
|||
int otherLastPer = 0; |
|||
String month = ""; |
|||
String year = ""; |
|||
String moth = ""; |
|||
String otherMoth = ""; |
|||
String otherFDate = ""; |
|||
String otherMonth = ""; |
|||
if (StringUtils.isNotBlank(mainDate)) { |
|||
String[] splitMain = mainDate.split("-"); |
|||
year = splitMain[0]; |
|||
moth = splitMain[1]; |
|||
if (moth.length() == 2 && Integer.parseInt(moth) >= 10) { |
|||
month = moth; |
|||
} else { |
|||
month = moth.replace("0", ""); |
|||
} |
|||
} |
|||
if (StringUtils.isNotBlank(otherDate)) { |
|||
String[] splitOther = otherDate.split("-"); |
|||
|
|||
otherMoth = splitOther[1]; |
|||
if (otherMoth.length() == 2 && Integer.parseInt(otherMoth) >= 10) { |
|||
otherMonth = otherMoth; |
|||
} else { |
|||
otherMonth = otherMoth.replace("0", ""); |
|||
} |
|||
otherFDate = splitOther[0] + otherMonth; |
|||
} |
|||
for (int i = 1; i <= mainPeriod; i++) { |
|||
String yearMonth = year + month; |
|||
LoanCreateScheduleVinsListVo scheduleVo = new LoanCreateScheduleVinsListVo(); |
|||
scheduleVo.setRepayMonth(year + "年" + month + "月"); |
|||
scheduleVo.setPeriod(String.valueOf(i)); |
|||
int m = Integer.parseInt(month); |
|||
month = String.valueOf(++m); |
|||
if (Integer.parseInt(month) > 12) { |
|||
int y = Integer.parseInt(year); |
|||
year = String.valueOf(++y); |
|||
month = "1"; |
|||
} |
|||
if (isOther.equals("1")) { |
|||
//首期
|
|||
if (i == 1) { |
|||
scheduleVo.setMainRepay(mainF.toString()); |
|||
} else if (i == mainPeriod) { |
|||
scheduleVo.setMainRepay(mainL.toString()); |
|||
} else { |
|||
scheduleVo.setMainRepay(mainM.toString()); |
|||
} |
|||
if ((yearMonth.equals(otherFDate))) { |
|||
scheduleVo.setOtherRepay(otherF.toString()); |
|||
scheduleVo.setAmount((new BigDecimal(scheduleVo.getMainRepay()).add(otherF)).toString()); |
|||
otherMidPer = (i + Integer.parseInt(otherPer)) - 2; |
|||
otherLastPer = (i + Integer.parseInt(otherPer)) - 1; |
|||
} else if (i <= otherMidPer && otherMidPer != 0) { |
|||
scheduleVo.setOtherRepay(otherM.toString()); |
|||
scheduleVo.setAmount((new BigDecimal(scheduleVo.getMainRepay()).add(otherM)).toString()); |
|||
} else if (i == otherLastPer && otherLastPer != 0) { |
|||
scheduleVo.setOtherRepay(otherL.toString()); |
|||
scheduleVo.setAmount((new BigDecimal(scheduleVo.getMainRepay()).add(otherL)).toString()); |
|||
} else if (scheduleVo.getOtherRepay() == null || "".equals(scheduleVo.getOtherRepay())) { |
|||
scheduleVo.setAmount(scheduleVo.getMainRepay()); |
|||
scheduleVo.setOtherRepay(""); |
|||
} |
|||
} else if (isOther.equals("0")) { |
|||
//首期
|
|||
if (i == 1) { |
|||
scheduleVo.setMainRepay(mainF.toString()); |
|||
} else if (i == mainPeriod) { |
|||
scheduleVo.setMainRepay(mainL.toString()); |
|||
} else { |
|||
scheduleVo.setMainRepay(mainM.toString()); |
|||
} |
|||
if (scheduleVo.getOtherRepay() == null || "".equals(scheduleVo.getOtherRepay())) { |
|||
scheduleVo.setAmount(scheduleVo.getMainRepay()); |
|||
scheduleVo.setOtherRepay(""); |
|||
} |
|||
} |
|||
scheduleVins.add(scheduleVo); |
|||
} |
|||
if (!scheduleVins.isEmpty()) { |
|||
vo.setScheduleVins(scheduleVins); |
|||
} |
|||
} |
|||
} |
|||
return rb.success().setData(vo); |
|||
} |
|||
|
|||
/** |
|||
* 生成pdf |
|||
* |
|||
* @param pdfVo |
|||
* @return |
|||
*/ |
|||
public String commonCreatePdf(LoanCreateScheduleVinsVo pdfVo) { |
|||
Map<String, Object> dataMap = new HashMap<String, Object>(); |
|||
List<LoanCreateScheduleVinsListVo> pdfVos = pdfVo.getScheduleVins(); |
|||
List<Map<String, Object>> newList = new ArrayList<>(); |
|||
dataMap.put("loanContractNo", pdfVo.getLoanContractNo()); |
|||
dataMap.put("borrowerName", pdfVo.getBorrowerName()); |
|||
dataMap.put("vehCount", pdfVo.getVehCount()); |
|||
dataMap.put("bankName", pdfVo.getBankName()); |
|||
dataMap.put("vinNo", pdfVo.getVinNo()); |
|||
dataMap.put("mainRepayDate", pdfVo.getMainRepayDate()); |
|||
if (StringUtils.isNotBlank(pdfVo.getOtherRepayDate())) { |
|||
dataMap.put("otherRepayDate", pdfVo.getOtherRepayDate()); |
|||
} |
|||
if (!pdfVos.isEmpty()) { |
|||
for (LoanCreateScheduleVinsListVo detailsVo : pdfVos) { |
|||
Map<String, Object> map = new HashMap<>(); |
|||
map.put("period", detailsVo.getPeriod()); |
|||
map.put("repayMonth", detailsVo.getRepayMonth()); |
|||
map.put("mainRepay", detailsVo.getMainRepay()); |
|||
if (StringUtils.isNotBlank(detailsVo.getOtherRepay())) { |
|||
map.put("otherRepay", detailsVo.getOtherRepay()); |
|||
} |
|||
map.put("amount", detailsVo.getAmount()); |
|||
newList.add(map); |
|||
} |
|||
} |
|||
dataMap.put("newList", newList); |
|||
String path = ""; |
|||
String targetPath = docPdfComponent.getUploadTemplateUrl(); |
|||
try { |
|||
String ftl = ""; |
|||
if (pdfVo.getIsOtherPolicy().equals("0")) { |
|||
ftl = "ftl/mainSchedule.ftl"; |
|||
} else if (pdfVo.getIsOtherPolicy().equals("1")) { |
|||
ftl = "ftl/otherSchedule.ftl"; |
|||
} |
|||
//获取模板
|
|||
InputStream inputStream = this.getClass().getClassLoader().getResourceAsStream(ftl); |
|||
//生成word文件名
|
|||
String dateStr = DateUtil.format(new Date(), "yyyyMMdd"); |
|||
long seconds = System.currentTimeMillis(); |
|||
String typeName = dateStr + seconds + ".doc"; |
|||
File file = new File(targetPath + "repaymentSchedule" + seconds + ".ftl"); |
|||
File dir = new File(targetPath); |
|||
WordConvertUtils.inputStreamToFile(inputStream, file); |
|||
WordConvertUtils.creatWord1(dataMap, file, targetPath, typeName, dir); |
|||
//新生成的word路径
|
|||
String wordPath = targetPath + typeName; |
|||
//生成出门证文件名
|
|||
String pdfName = "还款计划表" + dateStr + seconds + ".pdf"; |
|||
WordConvertUtils.doc2pdf(wordPath, targetPath, pdfName); |
|||
path = targetPath + pdfName; |
|||
} catch (NoClassDefFoundError e) { |
|||
e.printStackTrace(); |
|||
path = targetPath + "temp"; |
|||
} |
|||
return path; |
|||
} |
|||
|
|||
public ResultBean<List<LoanCreateScheduleVinOneVo>> viewVinOneSchedule(String salesOrderSid) { |
|||
ResultBean<List<LoanCreateScheduleVinOneVo>> rb = ResultBean.fireFail(); |
|||
List<LoanCreateScheduleVinOneVo> list = new ArrayList<>(); |
|||
LoanRepaymentSchedule schedule = baseMapper.selectByOrderSid(salesOrderSid); |
|||
LoanCreateScheduleVinsVo scheduleVinsVo = viewVinsSchedule(salesOrderSid).getData(); |
|||
if (null != schedule) { |
|||
if (StringUtils.isNotBlank(schedule.getVinNo())) { |
|||
if (!schedule.getVinNo().contains(",")) { |
|||
LoanCreateScheduleVinOneVo vinOneVo = new LoanCreateScheduleVinOneVo(); |
|||
if (null != scheduleVinsVo) { |
|||
BeanUtil.copyProperties(scheduleVinsVo, vinOneVo); |
|||
list.add(vinOneVo); |
|||
} |
|||
} else { |
|||
String vinNos = schedule.getVinNo(); |
|||
String[] split = vinNos.split(","); |
|||
for (int i = 0; i < split.length; i++) { |
|||
LoanCreateScheduleVinOneVo vinOneVo = new LoanCreateScheduleVinOneVo(); |
|||
if (null != scheduleVinsVo) { |
|||
BeanUtil.copyProperties(scheduleVinsVo, vinOneVo); |
|||
vinOneVo.setVinNo(split[i]); |
|||
list.add(vinOneVo); |
|||
} |
|||
} |
|||
} |
|||
} |
|||
} |
|||
return rb.success().setData(list); |
|||
} |
|||
} |
File diff suppressed because it is too large
File diff suppressed because it is too large
Loading…
Reference in new issue