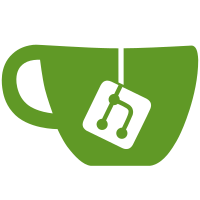
51 changed files with 3303 additions and 186 deletions
@ -0,0 +1,76 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.crm.api.crmcustomerorg; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.math.BigDecimal; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户单位) <br/> |
|||
* File: CrmCustomerOrg.java <br/> |
|||
* Class: com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrg <br/> |
|||
* Description: 客户单位. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 09:41:42 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "客户单位", description = "客户单位") |
|||
@TableName("crm_customer_org") |
|||
public class CrmCustomerOrg extends BaseEntity { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty("单位名称") |
|||
private String orgName; // 单位名称
|
|||
@ApiModelProperty("单位编码(拼音缩写)") |
|||
private String orgCode; // 单位编码(拼音缩写)
|
|||
@ApiModelProperty("联系人") |
|||
private String contacts; // 联系人
|
|||
@ApiModelProperty("联系电话") |
|||
private String mobile; // 联系电话
|
|||
@ApiModelProperty("地址") |
|||
private String address; // 地址
|
|||
@ApiModelProperty("信用额度") |
|||
private Integer creditLimit; // 信用额度
|
|||
@ApiModelProperty("期初欠款") |
|||
private BigDecimal initialDebt; // 期初欠款
|
|||
@ApiModelProperty("排序号") |
|||
private Integer sortNo; // 排序号
|
|||
@ApiModelProperty("使用组织sid") |
|||
private String useOrgSid; // 使用组织sid
|
|||
@ApiModelProperty("创建组织sid") |
|||
private String createOrgSid; // 创建组织sid
|
|||
|
|||
} |
@ -0,0 +1,78 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.crm.api.crmcustomerorg; |
|||
|
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
|
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户单位) <br/> |
|||
* File: CrmCustomerOrgVo.java <br/> |
|||
* Class: com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrgVo <br/> |
|||
* Description: 客户单位 视图数据对象. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 09:41:42 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "客户单位 视图数据详情", description = "客户单位 视图数据详情") |
|||
public class CrmCustomerOrgDetailsVo implements Vo { |
|||
|
|||
private String sid; // sid
|
|||
@ApiModelProperty("创建人sid") |
|||
private String createBySid; // 创建人sid
|
|||
@ApiModelProperty("备注") |
|||
private String remarks; // 备注
|
|||
@ApiModelProperty("单位名称") |
|||
private String orgName; // 单位名称
|
|||
@ApiModelProperty("单位编码(拼音缩写)") |
|||
private String orgCode; // 单位编码(拼音缩写)
|
|||
@ApiModelProperty("联系人") |
|||
private String contacts; // 联系人
|
|||
@ApiModelProperty("联系电话") |
|||
private String mobile; // 联系电话
|
|||
@ApiModelProperty("地址") |
|||
private String address; // 地址
|
|||
@ApiModelProperty("信用额度") |
|||
private String creditLimit; // 信用额度
|
|||
@ApiModelProperty("排序号") |
|||
private String sortNo; // 排序号
|
|||
@ApiModelProperty("期初欠款") |
|||
private String initialDebt; // 排序号
|
|||
@ApiModelProperty("使用组织sid") |
|||
private String useOrgSid; // 使用组织sid
|
|||
@ApiModelProperty("创建组织sid") |
|||
private String createOrgSid; // 创建组织sid
|
|||
|
|||
} |
@ -0,0 +1,78 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.crm.api.crmcustomerorg; |
|||
|
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
|
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户单位) <br/> |
|||
* File: CrmCustomerOrgDto.java <br/> |
|||
* Class: com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrgDto <br/> |
|||
* Description: 客户单位 数据传输对象. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 09:41:42 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "客户单位 数据传输对象", description = "客户单位 数据传输对象") |
|||
public class CrmCustomerOrgDto implements Dto { |
|||
|
|||
private String sid; // sid
|
|||
@ApiModelProperty("创建人sid") |
|||
private String createBySid; // 创建人sid
|
|||
@ApiModelProperty("备注") |
|||
private String remarks; // 备注
|
|||
@ApiModelProperty("单位名称") |
|||
private String orgName; // 单位名称
|
|||
@ApiModelProperty("单位编码(拼音缩写)") |
|||
private String orgCode; // 单位编码(拼音缩写)
|
|||
@ApiModelProperty("联系人") |
|||
private String contacts; // 联系人
|
|||
@ApiModelProperty("联系电话") |
|||
private String mobile; // 联系电话
|
|||
@ApiModelProperty("地址") |
|||
private String address; // 地址
|
|||
@ApiModelProperty("信用额度") |
|||
private String creditLimit; // 信用额度
|
|||
@ApiModelProperty("排序号") |
|||
private String sortNo; // 排序号
|
|||
@ApiModelProperty("期初欠款") |
|||
private String initialDebt; // 排序号
|
|||
@ApiModelProperty("使用组织sid") |
|||
private String useOrgSid; // 使用组织sid
|
|||
@ApiModelProperty("创建组织sid") |
|||
private String createOrgSid; // 创建组织sid
|
|||
|
|||
} |
@ -0,0 +1,84 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.crm.api.crmcustomerorg; |
|||
|
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户单位) <br/> |
|||
* File: CrmCustomerOrgFeign.java <br/> |
|||
* Class: com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrgFeign <br/> |
|||
* Description: 客户单位. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 09:41:42 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Api(tags = "客户单位") |
|||
@FeignClient( |
|||
contextId = "anrui-crm-CrmCustomerOrg", |
|||
name = "anrui-crm", |
|||
path = "v1/crmcustomerorg", |
|||
fallback = CrmCustomerOrgFeignFallback.class) |
|||
public interface CrmCustomerOrgFeign { |
|||
|
|||
@ApiOperation("根据条件分页查询数据的列表") |
|||
@PostMapping("/listPage") |
|||
@ResponseBody |
|||
public ResultBean<PagerVo<CrmCustomerOrgVo>> listPage(@RequestBody PagerQuery<CrmCustomerOrgQuery> pq); |
|||
|
|||
@ApiOperation("新增或修改") |
|||
@PostMapping("/save") |
|||
@ResponseBody |
|||
public ResultBean save(@RequestBody CrmCustomerOrgDto dto); |
|||
|
|||
@ApiOperation("根据sid删除记录") |
|||
@DeleteMapping("/delBySids") |
|||
@ResponseBody |
|||
public ResultBean delBySids(@RequestBody String[] sids); |
|||
|
|||
@ApiOperation("根据SID获取一条记录") |
|||
@GetMapping("/fetchDetailsBySid/{sid}") |
|||
@ResponseBody |
|||
public ResultBean<CrmCustomerOrgDetailsVo> fetchDetailsBySid(@PathVariable("sid") String sid); |
|||
|
|||
@ApiOperation("根据部门sid查询客户单位") |
|||
@GetMapping("/selCustomerOrgList") |
|||
@ResponseBody |
|||
public ResultBean<List<CrmCustomerOrgVo>> selCustomerOrgList(@RequestParam("useOrgSid") String useOrgSid); |
|||
|
|||
} |
@ -0,0 +1,77 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.crm.api.crmcustomerorg; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import org.springframework.stereotype.Component; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户单位) <br/> |
|||
* File: CrmCustomerOrgFeignFallback.java <br/> |
|||
* Class: com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrgFeignFallback <br/> |
|||
* Description: 客户单位. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 09:41:42 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Component |
|||
public class CrmCustomerOrgFeignFallback implements CrmCustomerOrgFeign { |
|||
|
|||
@Override |
|||
public ResultBean<PagerVo<CrmCustomerOrgVo>> listPage(PagerQuery<CrmCustomerOrgQuery> pq){ |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
return rb.setMsg("接口anrui-crm/crmcustomerorg/listPage无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean save(CrmCustomerOrgDto dto){ |
|||
return ResultBean.fireFail().setMsg("接口anrui-crm/crmcustomerorg/save无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean delBySids( String[] sids){ |
|||
return ResultBean.fireFail().setMsg("接口anrui-crm/crmcustomerorg/delBySids无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<CrmCustomerOrgDetailsVo> fetchDetailsBySid(String sid){ |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
return rb.setMsg("接口anrui-crm/crmcustomerorg/fetchDetailsBySid无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<CrmCustomerOrgVo>> selCustomerOrgList(String useOrgSid) { |
|||
return null; |
|||
} |
|||
} |
@ -0,0 +1,67 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.crm.api.crmcustomerorg; |
|||
|
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
|
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户单位) <br/> |
|||
* File: CrmCustomerOrgQuery.java <br/> |
|||
* Class: com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrgQuery <br/> |
|||
* Description: 客户单位 查询条件. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 09:41:42 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "客户单位 查询条件", description = "客户单位 查询条件") |
|||
public class CrmCustomerOrgQuery implements Query { |
|||
|
|||
@ApiModelProperty("单位名称") |
|||
private String orgName; // 单位名称
|
|||
@ApiModelProperty("单位编码(拼音缩写)") |
|||
private String orgCode; // 单位编码(拼音缩写)
|
|||
@ApiModelProperty("联系人") |
|||
private String contacts; // 联系人
|
|||
@ApiModelProperty("联系电话") |
|||
private String mobile; // 联系电话
|
|||
|
|||
@ApiModelProperty("全路径") |
|||
private String orgPath; |
|||
private String userSid; |
|||
@ApiModelProperty("菜单url") |
|||
private String menuUrl; |
|||
|
|||
} |
@ -0,0 +1,76 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.crm.api.crmcustomerorg; |
|||
|
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
|
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.math.BigDecimal; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户单位) <br/> |
|||
* File: CrmCustomerOrgVo.java <br/> |
|||
* Class: com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrgVo <br/> |
|||
* Description: 客户单位 视图数据对象. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 09:41:42 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "客户单位 视图数据对象", description = "客户单位 视图数据对象") |
|||
public class CrmCustomerOrgVo implements Vo { |
|||
|
|||
private String sid; // sid
|
|||
|
|||
@ApiModelProperty("单位名称") |
|||
private String orgName; // 单位名称
|
|||
@ApiModelProperty("单位编码(拼音缩写)") |
|||
private String orgCode; // 单位编码(拼音缩写)
|
|||
@ApiModelProperty("联系人") |
|||
private String contacts; // 联系人
|
|||
@ApiModelProperty("联系电话") |
|||
private String mobile; // 联系电话
|
|||
@ApiModelProperty("地址") |
|||
private String address; // 地址
|
|||
@ApiModelProperty("信用额度") |
|||
private String creditLimit; // 信用额度
|
|||
@ApiModelProperty("排序号") |
|||
private String sortNo; // 排序号
|
|||
@ApiModelProperty("期初欠款") |
|||
private String initialDebt; // 期初欠款
|
|||
@ApiModelProperty("备注") |
|||
private String remarks; // 备注
|
|||
|
|||
|
|||
} |
@ -0,0 +1,58 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.crm.api.crmcustomerorglink; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户单位) <br/> |
|||
* File: CrmCustomerOrgLink.java <br/> |
|||
* Class: com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLink <br/> |
|||
* Description: 客户、单位关联表. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 09:41:42 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "客户、单位关联表", description = "客户、单位关联表") |
|||
@TableName("crm_customer_org_link") |
|||
public class CrmCustomerOrgLink extends BaseEntity { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty("customerSid") |
|||
private String customerSid; //
|
|||
@ApiModelProperty("orgSid") |
|||
private String orgSid; //
|
|||
|
|||
} |
@ -0,0 +1,59 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.crm.api.crmcustomerorglink; |
|||
|
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
|
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户单位) <br/> |
|||
* File: CrmCustomerOrgLinkVo.java <br/> |
|||
* Class: com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLinkVo <br/> |
|||
* Description: 客户、单位关联表 视图数据对象. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 09:41:42 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "客户、单位关联表 视图数据详情", description = "客户、单位关联表 视图数据详情") |
|||
public class CrmCustomerOrgLinkDetailsVo implements Vo { |
|||
|
|||
private String sid; // sid
|
|||
|
|||
@ApiModelProperty("customerSid") |
|||
private String customerSid; //
|
|||
@ApiModelProperty("orgSid") |
|||
private String orgSid; //
|
|||
|
|||
} |
@ -0,0 +1,59 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.crm.api.crmcustomerorglink; |
|||
|
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
|
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户单位) <br/> |
|||
* File: CrmCustomerOrgLinkDto.java <br/> |
|||
* Class: com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLinkDto <br/> |
|||
* Description: 客户、单位关联表 数据传输对象. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 09:41:42 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "客户、单位关联表 数据传输对象", description = "客户、单位关联表 数据传输对象") |
|||
public class CrmCustomerOrgLinkDto implements Dto { |
|||
|
|||
private String sid; // sid
|
|||
|
|||
@ApiModelProperty("customerSid") |
|||
private String customerSid; //
|
|||
@ApiModelProperty("orgSid") |
|||
private String orgSid; //
|
|||
|
|||
} |
@ -0,0 +1,78 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.crm.api.crmcustomerorglink; |
|||
|
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import org.springframework.cloud.openfeign.FeignClient; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户单位) <br/> |
|||
* File: CrmCustomerOrgLinkFeign.java <br/> |
|||
* Class: com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLinkFeign <br/> |
|||
* Description: 客户、单位关联表. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 09:41:42 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Api(tags = "客户、单位关联表") |
|||
@FeignClient( |
|||
contextId = "anrui-crm-CrmCustomerOrgLink", |
|||
name = "anrui-crm", |
|||
path = "v1/crmcustomerorglink", |
|||
fallback = CrmCustomerOrgLinkFeignFallback.class) |
|||
public interface CrmCustomerOrgLinkFeign { |
|||
|
|||
@ApiOperation("根据条件分页查询数据的列表") |
|||
@PostMapping("/listPage") |
|||
@ResponseBody |
|||
public ResultBean<PagerVo<CrmCustomerOrgLinkVo>> listPage(@RequestBody PagerQuery<CrmCustomerOrgLinkQuery> pq); |
|||
|
|||
@ApiOperation("新增或修改") |
|||
@PostMapping("/save") |
|||
@ResponseBody |
|||
public ResultBean save(@RequestBody CrmCustomerOrgLinkDto dto); |
|||
|
|||
@ApiOperation("根据sid删除记录") |
|||
@DeleteMapping("/delBySids") |
|||
@ResponseBody |
|||
public ResultBean delBySids(@RequestBody String[] sids); |
|||
|
|||
@ApiOperation("根据SID获取一条记录") |
|||
@GetMapping("/fetchDetailsBySid/{sid}") |
|||
@ResponseBody |
|||
public ResultBean<CrmCustomerOrgLinkDetailsVo> fetchDetailsBySid(@PathVariable("sid") String sid); |
|||
} |
@ -0,0 +1,72 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.crm.api.crmcustomerorglink; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import org.springframework.stereotype.Component; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户单位) <br/> |
|||
* File: CrmCustomerOrgLinkFeignFallback.java <br/> |
|||
* Class: com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLinkFeignFallback <br/> |
|||
* Description: 客户、单位关联表. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 09:41:42 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Component |
|||
public class CrmCustomerOrgLinkFeignFallback implements CrmCustomerOrgLinkFeign { |
|||
|
|||
@Override |
|||
public ResultBean<PagerVo<CrmCustomerOrgLinkVo>> listPage(PagerQuery<CrmCustomerOrgLinkQuery> pq){ |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
return rb.setMsg("接口anrui-crm/crmcustomerorglink/listPage无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean save(CrmCustomerOrgLinkDto dto){ |
|||
return ResultBean.fireFail().setMsg("接口anrui-crm/crmcustomerorglink/save无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean delBySids( String[] sids){ |
|||
return ResultBean.fireFail().setMsg("接口anrui-crm/crmcustomerorglink/delBySids无法访问"); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<CrmCustomerOrgLinkDetailsVo> fetchDetailsBySid(String sid){ |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
return rb.setMsg("接口anrui-crm/crmcustomerorglink/fetchDetailsBySid无法访问"); |
|||
} |
|||
} |
@ -0,0 +1,57 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.crm.api.crmcustomerorglink; |
|||
|
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
|
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户单位) <br/> |
|||
* File: CrmCustomerOrgLinkQuery.java <br/> |
|||
* Class: com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLinkQuery <br/> |
|||
* Description: 客户、单位关联表 查询条件. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 09:41:42 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "客户、单位关联表 查询条件", description = "客户、单位关联表 查询条件") |
|||
public class CrmCustomerOrgLinkQuery implements Query { |
|||
|
|||
@ApiModelProperty("customerSid") |
|||
private String customerSid; //
|
|||
@ApiModelProperty("orgSid") |
|||
private String orgSid; //
|
|||
|
|||
} |
@ -0,0 +1,59 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.crm.api.crmcustomerorglink; |
|||
|
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
|
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户单位) <br/> |
|||
* File: CrmCustomerOrgLinkVo.java <br/> |
|||
* Class: com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLinkVo <br/> |
|||
* Description: 客户、单位关联表 视图数据对象. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 09:41:42 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "客户、单位关联表 视图数据对象", description = "客户、单位关联表 视图数据对象") |
|||
public class CrmCustomerOrgLinkVo implements Vo { |
|||
|
|||
private String sid; // sid
|
|||
|
|||
@ApiModelProperty("customerSid") |
|||
private String customerSid; //
|
|||
@ApiModelProperty("orgSid") |
|||
private String orgSid; //
|
|||
|
|||
} |
@ -0,0 +1,36 @@ |
|||
package com.yxt.anrui.crm.api.crmcustomertemp; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author Fan |
|||
* @description |
|||
* @date 2024/6/3 13:42 |
|||
*/ |
|||
@Data |
|||
public class AsCustomerListQuery implements Query { |
|||
@ApiModelProperty("客户名称") |
|||
private String name; |
|||
@ApiModelProperty("客户类型key(自然人/法人)") |
|||
private String customerTypeKey; |
|||
@ApiModelProperty("联系电话") |
|||
private String mobile; |
|||
@ApiModelProperty("客户级别(意向客户/准客户/成交客户/集团内销/黑名单客户)") |
|||
private String levelKey; |
|||
@ApiModelProperty("提醒开始日期") |
|||
private String remindStartDay; |
|||
@ApiModelProperty("提醒结束日期") |
|||
private String remindEndDay; |
|||
|
|||
@ApiModelProperty("全路径") |
|||
private String orgPath; |
|||
private String userSid; |
|||
@ApiModelProperty("菜单url") |
|||
private String menuUrl; |
|||
|
|||
|
|||
} |
@ -0,0 +1,39 @@ |
|||
package com.yxt.anrui.crm.api.crmcustomertemp; |
|||
|
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import com.yxt.anrui.crm.api.crmbusiness.CrmBusinessVo; |
|||
import com.yxt.common.core.vo.Vo; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
|
|||
/** |
|||
* @author Fan |
|||
* @description |
|||
* @date 2024/6/3 13:42 |
|||
*/ |
|||
@Data |
|||
public class AsCustomerListVo implements Vo { |
|||
|
|||
@ApiModelProperty("潜在客户sid") |
|||
private String sid; |
|||
@ApiModelProperty("客户编号(部门编码+客户类型(1位,0个人,1企业)+部门内部流水号(6位))") |
|||
private String customerNo; |
|||
@ApiModelProperty("客户名称") |
|||
private String name; |
|||
@ApiModelProperty("客户类型(自然人/法人)") |
|||
private String customerType; |
|||
@ApiModelProperty("联系电话") |
|||
private String mobile; |
|||
@ApiModelProperty("微信号码") |
|||
private String weixin; |
|||
@ApiModelProperty("客户级别(意向客户/准客户/成交客户/集团内销/黑名单客户)") |
|||
private String level; |
|||
@ApiModelProperty("提醒日期") |
|||
private String remind_day; |
|||
private String vehCount; //车辆数量
|
|||
|
|||
|
|||
|
|||
} |
@ -0,0 +1,73 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.crm.biz.crmcustomerorg; |
|||
|
|||
import com.baomidou.mybatisplus.core.conditions.Wrapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.baomidou.mybatisplus.core.toolkit.Constants; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
import org.apache.ibatis.annotations.Param; |
|||
import org.apache.ibatis.annotations.Select; |
|||
import com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrg; |
|||
import com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrgVo; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户单位) <br/> |
|||
* File: CrmCustomerOrgMapper.java <br/> |
|||
* Class: com.yxt.anrui.crm.biz.crmcustomerorg.CrmCustomerOrgMapper <br/> |
|||
* Description: 客户单位. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 09:41:42 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Mapper |
|||
public interface CrmCustomerOrgMapper extends BaseMapper<CrmCustomerOrg> { |
|||
|
|||
//@Update("update crm_customer_org set name=#{msg} where id=#{id}")
|
|||
//IPage<CrmCustomerOrgVo> voPage(IPage<CrmCustomerOrg> page, @Param(Constants.WRAPPER) QueryWrapper<CrmCustomerOrg> qw);
|
|||
|
|||
IPage<CrmCustomerOrgVo> selectPageVo(IPage<CrmCustomerOrg> page, @Param(Constants.WRAPPER) Wrapper<CrmCustomerOrg> qw); |
|||
|
|||
List<CrmCustomerOrgVo> selectListAllVo(@Param(Constants.WRAPPER) Wrapper<CrmCustomerOrg> qw); |
|||
|
|||
@Select("select * from crm_customer_org") |
|||
List<CrmCustomerOrgVo> selectListVo(); |
|||
|
|||
CrmCustomerOrg checkForInsert(@Param("orgName") String orgName,@Param("useOrgSid") String useOrgSid); |
|||
|
|||
CrmCustomerOrg checkForUpdate(@Param("orgName")String orgName, @Param("useOrgSid")String useOrgSid, @Param("sid")String sid); |
|||
|
|||
int updateBySidIsDelete(List<String> list); |
|||
|
|||
List<CrmCustomerOrgVo> selCustomerOrgList(@Param("useOrgSid") String useOrgSid); |
|||
} |
@ -0,0 +1,43 @@ |
|||
<?xml version="1.0" encoding="UTF-8" ?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.anrui.crm.biz.crmcustomerorg.CrmCustomerOrgMapper"> |
|||
<!-- <where> ${ew.sqlSegment} </where>--> |
|||
<!-- ${ew.customSqlSegment} --> |
|||
<select id="selectPageVo" resultType="com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrgVo"> |
|||
SELECT * FROM crm_customer_org as a LEFT JOIN anrui_portal.sys_organization as s ON a.useOrgSid = s.sid |
|||
<where>${ew.sqlSegment}</where> |
|||
</select> |
|||
|
|||
<select id="selectListAllVo" resultType="com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrgVo"> |
|||
SELECT * FROM crm_customer_org |
|||
<where>${ew.sqlSegment}</where> |
|||
</select> |
|||
<select id="checkForInsert" resultType="com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrg"> |
|||
select * |
|||
from crm_customer_org |
|||
where orgName = #{orgName} |
|||
and useOrgSid = #{useOrgSid} |
|||
and isDelete = 0 |
|||
</select> |
|||
<select id="checkForUpdate" resultType="com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrg"> |
|||
select * |
|||
from crm_customer_org |
|||
where orgName = #{orgName} |
|||
and useOrgSid = #{useOrgSid} |
|||
and isDelete = 0 |
|||
and sid !=#{sid} |
|||
</select> |
|||
<select id="selCustomerOrgList" resultType="com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrgVo"> |
|||
select * |
|||
from crm_customer_org |
|||
where useOrgSid = #{useOrgSid} |
|||
</select> |
|||
<update id="updateBySidIsDelete"> |
|||
UPDATE crm_customer_org |
|||
SET isDelete=1 |
|||
where sid in |
|||
<foreach collection="list" item="item" index="index" open="(" separator="," close=")"> |
|||
#{item} |
|||
</foreach> |
|||
</update> |
|||
</mapper> |
@ -0,0 +1,104 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.crm.biz.crmcustomerorg; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import java.util.List; |
|||
import com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrg; |
|||
import com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrgQuery; |
|||
import com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrgVo; |
|||
import com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrgDetailsVo; |
|||
import com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrgDto; |
|||
import com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrgFeign; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户单位) <br/> |
|||
* File: CrmCustomerOrgFeignFallback.java <br/> |
|||
* Class: com.yxt.anrui.crm.biz.crmcustomerorg.CrmCustomerOrgRest <br/> |
|||
* Description: 客户单位. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 09:41:42 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Api(tags = "客户单位") |
|||
@RestController("com.yxt.anrui.crm.biz.crmcustomerorg.CrmCustomerOrgRest") |
|||
@RequestMapping("v1/crmcustomerorg") |
|||
public class CrmCustomerOrgRest implements CrmCustomerOrgFeign { |
|||
|
|||
@Autowired |
|||
private CrmCustomerOrgService crmCustomerOrgService; |
|||
|
|||
@Override |
|||
@ApiOperation("根据条件分页查询数据的列表") |
|||
@PostMapping("/listPage") |
|||
public ResultBean<PagerVo<CrmCustomerOrgVo>> listPage(@RequestBody PagerQuery<CrmCustomerOrgQuery> pq){ |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
PagerVo<CrmCustomerOrgVo> pv = crmCustomerOrgService.listPageVo(pq); |
|||
return rb.success().setData(pv); |
|||
} |
|||
|
|||
@Override |
|||
@ApiOperation("新增或修改") |
|||
@PostMapping("/save") |
|||
public ResultBean save(@RequestBody CrmCustomerOrgDto dto){ |
|||
return crmCustomerOrgService.saveOrUpdateDto(dto); |
|||
} |
|||
|
|||
@ApiOperation("根据sid批量删除") |
|||
@DeleteMapping("/delBySids") |
|||
public ResultBean delBySids(@RequestBody String[] sids){ |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
crmCustomerOrgService.delAll(sids); |
|||
return rb.success(); |
|||
} |
|||
|
|||
@Override |
|||
@ApiOperation("根据SID获取一条记录") |
|||
@GetMapping("/fetchDetailsBySid/{sid}") |
|||
public ResultBean<CrmCustomerOrgDetailsVo> fetchDetailsBySid(@PathVariable("sid") String sid){ |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
CrmCustomerOrgDetailsVo vo = crmCustomerOrgService.fetchDetailsVoBySid(sid); |
|||
return rb.success().setData(vo); |
|||
} |
|||
|
|||
@Override |
|||
public ResultBean<List<CrmCustomerOrgVo>> selCustomerOrgList(String useOrgSid) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
List<CrmCustomerOrgVo> list = crmCustomerOrgService.selCustomerOrgList(useOrgSid); |
|||
return rb.success().setData(list); |
|||
} |
|||
} |
@ -0,0 +1,201 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.crm.biz.crmcustomerorg; |
|||
|
|||
import cn.hutool.core.bean.BeanUtil; |
|||
import cn.hutool.core.date.DateTime; |
|||
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import com.yxt.anrui.crm.utils.PinYinUtils; |
|||
import com.yxt.anrui.portal.api.sysorganization.SysOrganizationVo; |
|||
import com.yxt.anrui.portal.api.sysuser.PrivilegeQuery; |
|||
import com.yxt.anrui.portal.api.sysuser.SysUserFeign; |
|||
import org.apache.commons.lang3.StringUtils; |
|||
import com.yxt.common.base.service.MybatisBaseService; |
|||
import com.yxt.common.base.utils.PagerUtil; |
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrg; |
|||
import com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrgQuery; |
|||
import com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrgVo; |
|||
import com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrgDetailsVo; |
|||
import com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrgDto; |
|||
import com.yxt.anrui.crm.api.crmcustomerorg.CrmCustomerOrgFeign; |
|||
|
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
import java.util.Arrays; |
|||
import java.util.Date; |
|||
import java.util.List; |
|||
import java.util.stream.Collectors; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户单位) <br/> |
|||
* File: CrmCustomerOrgService.java <br/> |
|||
* Class: com.yxt.anrui.crm.biz.crmcustomerorg.CrmCustomerOrgService <br/> |
|||
* Description: 客户单位 业务逻辑. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 09:41:42 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Service |
|||
public class CrmCustomerOrgService extends MybatisBaseService<CrmCustomerOrgMapper, CrmCustomerOrg> { |
|||
|
|||
@Autowired |
|||
private SysUserFeign sysUserFeign; |
|||
|
|||
private QueryWrapper<CrmCustomerOrg> createQueryWrapper(CrmCustomerOrgQuery query) { |
|||
// todo: 这里根据具体业务调整查询条件
|
|||
// 多字段Like示例:qw.and(wrapper -> wrapper.like("name", query.getName()).or().like("remark", query.getName()));
|
|||
QueryWrapper<CrmCustomerOrg> qw = new QueryWrapper<>(); |
|||
return qw; |
|||
} |
|||
|
|||
public PagerVo<CrmCustomerOrgVo> listPageVo(PagerQuery<CrmCustomerOrgQuery> pq) { |
|||
CrmCustomerOrgQuery query = pq.getParams(); |
|||
QueryWrapper<CrmCustomerOrg> qw = new QueryWrapper<>(); |
|||
PrivilegeQuery privilegeQuery = new PrivilegeQuery(); |
|||
privilegeQuery.setOrgPath(query.getOrgPath()); |
|||
privilegeQuery.setMenuUrl(query.getMenuUrl()); |
|||
privilegeQuery.setUserSid(query.getUserSid()); |
|||
ResultBean<String> defaultIdReltBean = sysUserFeign.selectPrivilegeLevel(privilegeQuery); |
|||
if (StringUtils.isNotBlank(query.getMenuUrl())) { |
|||
if (StringUtils.isNotBlank(defaultIdReltBean.getData())) { |
|||
//数据权限ID(1集团、2事业部、3分公司、4部门、5个人)
|
|||
String orgSidPath = query.getOrgPath(); |
|||
orgSidPath = orgSidPath + "/"; |
|||
int i1 = orgSidPath.indexOf("/"); |
|||
int i2 = orgSidPath.indexOf("/", i1 + 1); |
|||
int i3 = orgSidPath.indexOf("/", i2 + 1); |
|||
int i4 = orgSidPath.indexOf("/", i3 + 1); |
|||
String orgLevelKey = defaultIdReltBean.getData(); |
|||
if ("1".equals(orgLevelKey)) { |
|||
orgSidPath = orgSidPath.substring(0, i1); |
|||
qw.like("s.orgSidPath", orgSidPath); |
|||
} else if ("2".equals(orgLevelKey)) { |
|||
orgSidPath = orgSidPath.substring(0, i2); |
|||
qw.like("s.orgSidPath", orgSidPath); |
|||
} else if ("3".equals(orgLevelKey)) { |
|||
orgSidPath = orgSidPath.substring(0, i3); |
|||
qw.like("s.orgSidPath", orgSidPath); |
|||
} else if ("4".equals(orgLevelKey)) { |
|||
orgSidPath = orgSidPath.substring(0, i4); |
|||
qw.like("s.orgSidPath", orgSidPath); |
|||
} else if ("5".equals(orgLevelKey)) { |
|||
qw.eq("a.createBySid", query.getUserSid()); |
|||
} else { |
|||
PagerVo<CrmCustomerOrgVo> p = new PagerVo<>(); |
|||
return p; |
|||
} |
|||
} else { |
|||
PagerVo<CrmCustomerOrgVo> p = new PagerVo<>(); |
|||
return p; |
|||
} |
|||
} |
|||
if (StringUtils.isNotBlank(query.getOrgName())) { |
|||
qw.like("a.orgName", query.getOrgName()); |
|||
} |
|||
if (StringUtils.isNotBlank(query.getOrgCode())) { |
|||
qw.like("a.orgCode", query.getOrgCode()); |
|||
} |
|||
if (StringUtils.isNotBlank(query.getContacts())) { |
|||
qw.like("a.contacts", query.getContacts()); |
|||
} |
|||
if (StringUtils.isNotBlank(query.getMobile())) { |
|||
qw.like("a.mobile", query.getMobile()); |
|||
} |
|||
qw.eq("a.isDelete", 0); |
|||
qw.orderByAsc("a.sortNo"); |
|||
IPage<CrmCustomerOrg> page = PagerUtil.queryToPage(pq); |
|||
IPage<CrmCustomerOrgVo> pagging = baseMapper.selectPageVo(page, qw); |
|||
PagerVo<CrmCustomerOrgVo> p = PagerUtil.pageToVo(pagging, null); |
|||
return p; |
|||
} |
|||
|
|||
public ResultBean saveOrUpdateDto(CrmCustomerOrgDto dto) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
String sid = ""; |
|||
if (StringUtils.isNotBlank(dto.getSid())) { |
|||
sid = dto.getSid(); |
|||
CrmCustomerOrg crmCustomerOrg = fetchBySid(dto.getSid()); |
|||
BeanUtil.copyProperties(dto, crmCustomerOrg, "id", "sid"); |
|||
crmCustomerOrg.setModifyTime(new Date()); |
|||
crmCustomerOrg.setOrgCode(PinYinUtils.sx(dto.getOrgName())); |
|||
CrmCustomerOrg check = baseMapper.checkForUpdate(dto.getOrgName(), crmCustomerOrg.getUseOrgSid(), sid); |
|||
if (null != check) { |
|||
return rb.setMsg("单位名称不能重复"); |
|||
} |
|||
baseMapper.updateById(crmCustomerOrg); |
|||
} else { |
|||
CrmCustomerOrg crmCustomerOrg = new CrmCustomerOrg(); |
|||
BeanUtil.copyProperties(dto, crmCustomerOrg, "id", "sid"); |
|||
crmCustomerOrg.setOrgCode(PinYinUtils.sx(dto.getOrgName())); |
|||
CrmCustomerOrg check = baseMapper.checkForInsert(dto.getOrgName(), dto.getUseOrgSid()); |
|||
if (null != check) { |
|||
return rb.setMsg("单位名称不能重复"); |
|||
} |
|||
baseMapper.insert(crmCustomerOrg); |
|||
} |
|||
return rb.success(); |
|||
} |
|||
|
|||
public void insertByDto(CrmCustomerOrgDto dto) { |
|||
CrmCustomerOrg entity = new CrmCustomerOrg(); |
|||
BeanUtil.copyProperties(dto, entity, "id", "sid"); |
|||
baseMapper.insert(entity); |
|||
} |
|||
|
|||
public void updateByDto(CrmCustomerOrgDto dto) { |
|||
String dtoSid = dto.getSid(); |
|||
if (StringUtils.isBlank(dtoSid)) { |
|||
return; |
|||
} |
|||
CrmCustomerOrg entity = fetchBySid(dtoSid); |
|||
BeanUtil.copyProperties(dto, entity, "id", "sid"); |
|||
baseMapper.updateById(entity); |
|||
} |
|||
|
|||
public CrmCustomerOrgDetailsVo fetchDetailsVoBySid(String sid) { |
|||
CrmCustomerOrg entity = fetchBySid(sid); |
|||
CrmCustomerOrgDetailsVo vo = new CrmCustomerOrgDetailsVo(); |
|||
BeanUtil.copyProperties(entity, vo); |
|||
return vo; |
|||
} |
|||
|
|||
public void delAll(String[] sids) { |
|||
int count = baseMapper.updateBySidIsDelete(Arrays.stream(sids).collect(Collectors.toList())); |
|||
} |
|||
|
|||
public List<CrmCustomerOrgVo> selCustomerOrgList(String useOrgSid) { |
|||
return baseMapper.selCustomerOrgList(useOrgSid); |
|||
} |
|||
} |
@ -0,0 +1,67 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.crm.biz.crmcustomerorglink; |
|||
|
|||
import com.baomidou.mybatisplus.core.conditions.Wrapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.baomidou.mybatisplus.core.toolkit.Constants; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
import org.apache.ibatis.annotations.Param; |
|||
import org.apache.ibatis.annotations.Select; |
|||
import com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLink; |
|||
import com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLinkVo; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户单位) <br/> |
|||
* File: CrmCustomerOrgLinkMapper.java <br/> |
|||
* Class: com.yxt.anrui.crm.biz.crmcustomerorglink.CrmCustomerOrgLinkMapper <br/> |
|||
* Description: 客户、单位关联表. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 09:41:42 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Mapper |
|||
public interface CrmCustomerOrgLinkMapper extends BaseMapper<CrmCustomerOrgLink> { |
|||
|
|||
//@Update("update crm_customer_org_link set name=#{msg} where id=#{id}")
|
|||
//IPage<CrmCustomerOrgLinkVo> voPage(IPage<CrmCustomerOrgLink> page, @Param(Constants.WRAPPER) QueryWrapper<CrmCustomerOrgLink> qw);
|
|||
|
|||
IPage<CrmCustomerOrgLinkVo> selectPageVo(IPage<CrmCustomerOrgLink> page, @Param(Constants.WRAPPER) Wrapper<CrmCustomerOrgLink> qw); |
|||
|
|||
List<CrmCustomerOrgLinkVo> selectListAllVo(@Param(Constants.WRAPPER) Wrapper<CrmCustomerOrgLink> qw); |
|||
|
|||
@Select("select * from crm_customer_org_link") |
|||
List<CrmCustomerOrgLinkVo> selectListVo(); |
|||
|
|||
CrmCustomerOrgLink fetchByCustomerSid(@Param("customerSid") String customerSid); |
|||
} |
@ -0,0 +1,16 @@ |
|||
<?xml version="1.0" encoding="UTF-8" ?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.anrui.crm.biz.crmcustomerorglink.CrmCustomerOrgLinkMapper"> |
|||
<!-- <where> ${ew.sqlSegment} </where>--> |
|||
<!-- ${ew.customSqlSegment} --> |
|||
<select id="selectPageVo" resultType="com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLinkVo"> |
|||
SELECT * FROM crm_customer_org_link <where> ${ew.sqlSegment} </where> |
|||
</select> |
|||
|
|||
<select id="selectListAllVo" resultType="com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLinkVo"> |
|||
SELECT * FROM crm_customer_org_link <where> ${ew.sqlSegment} </where> |
|||
</select> |
|||
<select id="fetchByCustomerSid" resultType="com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLink"> |
|||
SELECT * FROM crm_customer_org_link where customerSid =#{customerSid} |
|||
</select> |
|||
</mapper> |
@ -0,0 +1,100 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.crm.biz.crmcustomerorglink; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import java.util.List; |
|||
import com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLink; |
|||
import com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLinkQuery; |
|||
import com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLinkVo; |
|||
import com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLinkDetailsVo; |
|||
import com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLinkDto; |
|||
import com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLinkFeign; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户单位) <br/> |
|||
* File: CrmCustomerOrgLinkFeignFallback.java <br/> |
|||
* Class: com.yxt.anrui.crm.biz.crmcustomerorglink.CrmCustomerOrgLinkRest <br/> |
|||
* Description: 客户、单位关联表. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 09:41:42 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Api(tags = "客户、单位关联表") |
|||
@RestController("com.yxt.anrui.crm.biz.crmcustomerorglink.CrmCustomerOrgLinkRest") |
|||
@RequestMapping("v1/crmcustomerorglink") |
|||
public class CrmCustomerOrgLinkRest implements CrmCustomerOrgLinkFeign { |
|||
|
|||
@Autowired |
|||
private CrmCustomerOrgLinkService crmCustomerOrgLinkService; |
|||
|
|||
@Override |
|||
@ApiOperation("根据条件分页查询数据的列表") |
|||
@PostMapping("/listPage") |
|||
public ResultBean<PagerVo<CrmCustomerOrgLinkVo>> listPage(@RequestBody PagerQuery<CrmCustomerOrgLinkQuery> pq){ |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
PagerVo<CrmCustomerOrgLinkVo> pv = crmCustomerOrgLinkService.listPageVo(pq); |
|||
return rb.success().setData(pv); |
|||
} |
|||
|
|||
@Override |
|||
@ApiOperation("新增或修改") |
|||
@PostMapping("/save") |
|||
public ResultBean save(@RequestBody CrmCustomerOrgLinkDto dto){ |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
crmCustomerOrgLinkService.saveOrUpdateDto(dto); |
|||
return rb.success(); |
|||
} |
|||
|
|||
@Override |
|||
@ApiOperation("根据sid批量删除") |
|||
@PostMapping("/delBySids") |
|||
public ResultBean delBySids(@RequestBody String[] sids){ |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
crmCustomerOrgLinkService.delBySids(sids); |
|||
return rb.success(); |
|||
} |
|||
|
|||
@Override |
|||
@ApiOperation("根据SID获取一条记录") |
|||
@GetMapping("/fetchDetailsBySid/{sid}") |
|||
public ResultBean<CrmCustomerOrgLinkDetailsVo> fetchDetailsBySid(@PathVariable("sid") String sid){ |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
CrmCustomerOrgLinkDetailsVo vo = crmCustomerOrgLinkService.fetchDetailsVoBySid(sid); |
|||
return rb.success().setData(vo); |
|||
} |
|||
} |
@ -0,0 +1,128 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.crm.biz.crmcustomerorglink; |
|||
|
|||
import cn.hutool.core.bean.BeanUtil; |
|||
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import org.apache.commons.lang3.StringUtils; |
|||
import com.yxt.common.base.service.MybatisBaseService; |
|||
import com.yxt.common.base.utils.PagerUtil; |
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLink; |
|||
import com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLinkQuery; |
|||
import com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLinkVo; |
|||
import com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLinkDetailsVo; |
|||
import com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLinkDto; |
|||
import com.yxt.anrui.crm.api.crmcustomerorglink.CrmCustomerOrgLinkFeign; |
|||
|
|||
import org.springframework.stereotype.Service; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* Project: anrui-crm(客户单位) <br/> |
|||
* File: CrmCustomerOrgLinkService.java <br/> |
|||
* Class: com.yxt.anrui.crm.biz.crmcustomerorglink.CrmCustomerOrgLinkService <br/> |
|||
* Description: 客户、单位关联表 业务逻辑. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 09:41:42 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Service |
|||
public class CrmCustomerOrgLinkService extends MybatisBaseService<CrmCustomerOrgLinkMapper, CrmCustomerOrgLink> { |
|||
private QueryWrapper<CrmCustomerOrgLink> createQueryWrapper(CrmCustomerOrgLinkQuery query) { |
|||
// todo: 这里根据具体业务调整查询条件
|
|||
// 多字段Like示例:qw.and(wrapper -> wrapper.like("name", query.getName()).or().like("remark", query.getName()));
|
|||
QueryWrapper<CrmCustomerOrgLink> qw = new QueryWrapper<>(); |
|||
return qw; |
|||
} |
|||
|
|||
public PagerVo<CrmCustomerOrgLinkVo> listPageVo(PagerQuery<CrmCustomerOrgLinkQuery> pq) { |
|||
CrmCustomerOrgLinkQuery query = pq.getParams(); |
|||
QueryWrapper<CrmCustomerOrgLink> qw = createQueryWrapper(query); |
|||
IPage<CrmCustomerOrgLink> page = PagerUtil.queryToPage(pq); |
|||
IPage<CrmCustomerOrgLinkVo> pagging = baseMapper.selectPageVo(page, qw); |
|||
PagerVo<CrmCustomerOrgLinkVo> p = PagerUtil.pageToVo(pagging, null); |
|||
return p; |
|||
} |
|||
|
|||
public void saveOrUpdateDto(CrmCustomerOrgLinkDto dto) { |
|||
String dtoSid = dto.getSid(); |
|||
if (StringUtils.isBlank(dtoSid)) { |
|||
this.insertByDto(dto); |
|||
return; |
|||
} |
|||
this.updateByDto(dto); |
|||
} |
|||
|
|||
public void insertByDto(CrmCustomerOrgLinkDto dto) { |
|||
CrmCustomerOrgLink entity = new CrmCustomerOrgLink(); |
|||
BeanUtil.copyProperties(dto, entity, "id", "sid"); |
|||
baseMapper.insert(entity); |
|||
} |
|||
|
|||
public void updateByDto(CrmCustomerOrgLinkDto dto) { |
|||
String dtoSid = dto.getSid(); |
|||
if (StringUtils.isBlank(dtoSid)) { |
|||
return; |
|||
} |
|||
CrmCustomerOrgLink entity = fetchBySid(dtoSid); |
|||
BeanUtil.copyProperties(dto, entity, "id", "sid"); |
|||
baseMapper.updateById(entity); |
|||
} |
|||
|
|||
public CrmCustomerOrgLinkDetailsVo fetchDetailsVoBySid(String sid) { |
|||
CrmCustomerOrgLink entity = fetchBySid(sid); |
|||
CrmCustomerOrgLinkDetailsVo vo = new CrmCustomerOrgLinkDetailsVo(); |
|||
BeanUtil.copyProperties(entity, vo); |
|||
return vo; |
|||
} |
|||
|
|||
public CrmCustomerOrgLink fetchByCustomerSid(String customerSid) { |
|||
return baseMapper.fetchByCustomerSid(customerSid); |
|||
} |
|||
|
|||
public void saveOrgLink(String customerSid, String orgSid) { |
|||
CrmCustomerOrgLink crmCustomerOrgLink = baseMapper.fetchByCustomerSid(customerSid); |
|||
if (null != crmCustomerOrgLink) { |
|||
crmCustomerOrgLink.setOrgSid(orgSid); |
|||
baseMapper.updateById(crmCustomerOrgLink); |
|||
} else { |
|||
CrmCustomerOrgLink entity = new CrmCustomerOrgLink(); |
|||
entity.setCustomerSid(customerSid); |
|||
entity.setOrgSid(orgSid); |
|||
baseMapper.insert(entity); |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,292 @@ |
|||
package com.yxt.anrui.crm.utils; |
|||
|
|||
import net.sourceforge.pinyin4j.PinyinHelper; |
|||
import net.sourceforge.pinyin4j.format.HanyuPinyinCaseType; |
|||
import net.sourceforge.pinyin4j.format.HanyuPinyinOutputFormat; |
|||
import net.sourceforge.pinyin4j.format.HanyuPinyinToneType; |
|||
import net.sourceforge.pinyin4j.format.HanyuPinyinVCharType; |
|||
import org.apache.commons.lang3.StringUtils; |
|||
|
|||
import java.util.*; |
|||
import java.util.stream.Collectors; |
|||
import java.util.stream.Stream; |
|||
|
|||
/** |
|||
* @Description |
|||
* @Author liuguohui |
|||
* @Date 2021/9/16 |
|||
*/ |
|||
public class PinYinUtils { |
|||
|
|||
private static HanyuPinyinOutputFormat format = null; |
|||
|
|||
static { |
|||
format = new HanyuPinyinOutputFormat(); |
|||
//拼音小写
|
|||
format.setCaseType(HanyuPinyinCaseType.LOWERCASE); |
|||
//无音标方式;WITH_TONE_NUMBER:1-4数字表示英标;WITH_TONE_MARK:直接用音标符(必须WITH_U_UNICODE否则异常
|
|||
format.setToneType(HanyuPinyinToneType.WITHOUT_TONE); |
|||
//用v表示ü
|
|||
format.setVCharType(HanyuPinyinVCharType.WITH_V); |
|||
} |
|||
|
|||
// 多音字
|
|||
private static final Map<String, Object> DYZMAP = setDYZMap(); |
|||
|
|||
private static Map<String, Object> setDYZMap(){ |
|||
Map<String, Object> map = new HashMap<>(); |
|||
map.put("仇","QIU"); |
|||
map.put("柏", "BO"); |
|||
map.put("牟", "MU"); |
|||
map.put("颉", "XIE"); |
|||
map.put("解", "XIE"); |
|||
map.put("尉", "YU"); |
|||
map.put("奇", "JI"); |
|||
map.put("单", "SHAN"); |
|||
map.put("谌", "SHEN"); |
|||
map.put("乐", "YUE"); |
|||
map.put("召", "SHAO"); |
|||
map.put("朴", "PIAO"); |
|||
map.put("区", "OU"); |
|||
map.put("查", "ZHA"); |
|||
map.put("曾", "ZENG"); |
|||
map.put("缪", "MIAO"); |
|||
map.put("晟", "CHENG"); |
|||
map.put("员", "YUN"); |
|||
map.put("贠", "YUN"); |
|||
map.put("黑", "HE"); |
|||
map.put("重", "CHONG"); |
|||
map.put("秘", "BI"); |
|||
map.put("冼", "XIAN"); |
|||
map.put("折", "SHE"); |
|||
map.put("翟", "ZHAI"); |
|||
map.put("盖", "GE"); |
|||
map.put("万俟", "MOQI"); |
|||
map.put("尉迟", "YUCHI"); |
|||
return map; |
|||
} |
|||
|
|||
|
|||
|
|||
/** |
|||
* 返回字符串的拼音 |
|||
* @param str |
|||
* @return |
|||
*/ |
|||
public static String[] getCharPinYinString(String str) { |
|||
if (str == null || str.length() < 1) { |
|||
return null; |
|||
} |
|||
List<String> result = new ArrayList<String>(); |
|||
//对字符串中的记录逐个分析
|
|||
for (int i = 0; i < str.length(); i++) { |
|||
result = getCharPinYinString(str.charAt(i), result); |
|||
} |
|||
return result.toArray(new String[result.size()]); |
|||
} |
|||
|
|||
/** |
|||
* 将字符c的拼音拼接到list中的记录中 |
|||
* @param c |
|||
* @param list |
|||
* @return |
|||
*/ |
|||
private static List<String> getCharPinYinString(char c, List<String> list) { |
|||
String[] strs = getCharPinYinString(c); |
|||
List<String> result = new ArrayList<String>(); |
|||
// strs去重(多音字)
|
|||
strs = disdinctStr(strs); |
|||
|
|||
//如果解析出的拼音为空,判断字符C是否为英文字母,如果是英文字母则添加值拼音结果中
|
|||
if (strs == null) { |
|||
if ((c >= 'a' && c <= 'z') || (c >= 'A' && c <= 'Z')) { |
|||
c = c <= 91 ? (char)(c + 32) : c; |
|||
if (list == null || list.size() == 0) { |
|||
result.add(c + ""); |
|||
} else { |
|||
for (String s : list) { |
|||
result.add(s + c); |
|||
} |
|||
} |
|||
return result; |
|||
} |
|||
return list; |
|||
} |
|||
//将字符C的拼音首和已存在的拼音首组合成新的记录
|
|||
for (String str : strs) { |
|||
if (list == null || list.size() == 0) { |
|||
result.add(str); |
|||
} else { |
|||
for (String s : list) { |
|||
result.add(s + str); |
|||
} |
|||
} |
|||
} |
|||
return result; |
|||
} |
|||
|
|||
/** |
|||
* 返回汉字的拼音 |
|||
* @param c |
|||
* @return |
|||
*/ |
|||
public static String[] getCharPinYinString(char c) { |
|||
try { |
|||
//返回字符C的拼音
|
|||
return PinyinHelper.toHanyuPinyinStringArray(c, format); |
|||
} catch (Exception e) { |
|||
e.printStackTrace(); |
|||
} |
|||
return null; |
|||
} |
|||
/** |
|||
* 缩写 |
|||
* @param args |
|||
*/ |
|||
public static String sx(String hz) { |
|||
char [] nameArray = hz.toCharArray(); |
|||
String b=""; |
|||
for (int i = 0; i < nameArray.length; i++) { |
|||
if (Character.toString(nameArray[i]).matches("^[\u4e00-\u9fa5]+$")) { |
|||
b=b+PinYinUtils.getCharDuoPinYinChar(String.valueOf(nameArray[i])); |
|||
}else{ |
|||
b=b+String.valueOf(nameArray[i]); |
|||
} |
|||
} |
|||
return b; |
|||
} |
|||
/** |
|||
* 返回字符串的拼音的首字母 |
|||
* @param str |
|||
* @return |
|||
*/ |
|||
public static String[] getCharPinYinChar(String str) { |
|||
if (str == null || str.length() < 1) { |
|||
return null; |
|||
} |
|||
List<String> result = new ArrayList<String>(); |
|||
//对字符串中的记录逐个分析
|
|||
for (int i = 0; i < str.length(); i++) { |
|||
result = getCharPinYinChar(str.charAt(i), result); |
|||
} |
|||
return result.toArray(new String[result.size()]); |
|||
} |
|||
|
|||
/** |
|||
* 将字符c的拼音首字母拼接到list中的记录中 |
|||
* @param c |
|||
* @param list |
|||
* @return |
|||
*/ |
|||
private static List<String> getCharPinYinChar(char c, List<String> list) { |
|||
char[] chars = getCharPinYinChar(c); |
|||
List<String> result = new ArrayList<String>(); |
|||
//如果解析出的拼音为空,判断字符C是否为英文字母,如果是英文字母则添加值拼音结果中
|
|||
if (chars == null) { |
|||
if ((c >= 'a' && c <= 'z') || (c >= 'A' && c <= 'Z')) { |
|||
c = c < 91 ? (char)(c + 32) : c; |
|||
if (list == null || list.size() == 0) { |
|||
result.add(c + ""); |
|||
} else { |
|||
for (String s : list) { |
|||
result.add(s + c); |
|||
} |
|||
} |
|||
return result; |
|||
} |
|||
return list; |
|||
} |
|||
//将字符C的拼音首字母和已存在的拼音首字母组合成新的记录
|
|||
for (char ch : chars) { |
|||
if (list == null || list.size() == 0) { |
|||
result.add(ch + ""); |
|||
} else { |
|||
for (String s : list) { |
|||
result.add(s + ch); |
|||
} |
|||
} |
|||
} |
|||
return result; |
|||
} |
|||
|
|||
/** |
|||
* 返回汉字拼音首字母 |
|||
* @param c |
|||
* @return |
|||
*/ |
|||
public static char[] getCharPinYinChar(char c) { |
|||
//字符C的拼音
|
|||
String[] strs = getCharPinYinString(c); |
|||
if (strs != null) { |
|||
//截取拼音的首字母
|
|||
char[] chars = new char[strs.length]; |
|||
for(int i = 0; i <chars.length; i++) { |
|||
chars[i] = strs[i].charAt(0); |
|||
} |
|||
return chars; |
|||
} |
|||
return null; |
|||
} |
|||
|
|||
/** |
|||
* 去重 |
|||
* @param str |
|||
* @return |
|||
*/ |
|||
private static String [] disdinctStr(String str[]){ |
|||
Stream<String> arrStream = Arrays.stream(str); |
|||
List<String> arrList = arrStream.distinct().collect(Collectors.toList()); |
|||
str = new String[arrList.size()]; |
|||
arrList.toArray(str); |
|||
return str; |
|||
} |
|||
|
|||
/** |
|||
* 判断传入的字符串是否为中文,将中文的转换为拼音 |
|||
* @param name |
|||
* @return |
|||
*/ |
|||
public static String getPinYinName(String name){ |
|||
char[] nameArray = name.toCharArray(); |
|||
String newName = ""; |
|||
for (int i = 0; i < nameArray.length; i++) { |
|||
if (Character.toString(nameArray[i]).matches("^[\u4e00-\u9fa5]+$")) { // 如果字符是中文,则将中文转为汉语拼音
|
|||
String[] pinYinString = PinYinUtils.getCharPinYinString(nameArray[i]); |
|||
// strs去重(多音字)
|
|||
pinYinString = disdinctStr(pinYinString); |
|||
String pinYinStr = StringUtils.join(pinYinString); |
|||
newName = newName + pinYinStr; |
|||
}else { |
|||
newName = newName + Character.toString(nameArray[i]); |
|||
} |
|||
} |
|||
return newName; |
|||
} |
|||
|
|||
/** |
|||
* 返回字符串的拼音的首字母(包括多音字) |
|||
* @param str |
|||
* @return |
|||
*/ |
|||
public static String getCharDuoPinYinChar(String str) { |
|||
if (str == null || str.length() < 1) { |
|||
return null; |
|||
} |
|||
char firstChar = str.toCharArray()[0]; |
|||
if(Character.toString(firstChar).matches("^[\u4e00-\u9fa5]+$")){ // 为中文
|
|||
char[] charPinYinChar = getCharPinYinChar(firstChar); |
|||
String result = ""; |
|||
if (DYZMAP.containsKey(Character.toString(firstChar))) { |
|||
result = DYZMAP.get(Character.toString(firstChar)).toString().substring(0, 1); |
|||
}else { |
|||
result = StringUtils.join(charPinYinChar[0]); |
|||
} |
|||
return result.toUpperCase(); |
|||
}else if(Character.toString(firstChar).matches("^[a-zA-Z]")){ // 为英文字母
|
|||
return Character.toString(firstChar).toUpperCase(); |
|||
}else { // 特殊符号
|
|||
return "#"; |
|||
} |
|||
} |
|||
|
|||
} |
@ -0,0 +1,106 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.as.api.ascustomervehmaintenance; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableField; |
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.fasterxml.jackson.annotation.JsonProperty; |
|||
import com.yxt.common.core.domain.BaseEntity; |
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import java.util.Date; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: as(车辆保养明细) <br/> |
|||
* File: AsCustomervehMaintenance.java <br/> |
|||
* Class: com.yxt.anrui.as.api.ascustomervehmaintenance.AsCustomervehMaintenance <br/> |
|||
* Description: 客户保养记录(到期明细). <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 10:04:53 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "客户保养记录(到期明细)", description = "客户保养记录(到期明细)") |
|||
@TableName("as_customerveh_maintenance") |
|||
public class AsCustomervehMaintenance extends BaseEntity { |
|||
private static final long serialVersionUID = 1L; |
|||
|
|||
@ApiModelProperty("车辆sid") |
|||
private String vehSid; // 车辆sid
|
|||
@ApiModelProperty("行驶里程") |
|||
private Integer currentMileage; // 行驶里程
|
|||
@ApiModelProperty("每月公里") |
|||
private Integer MonthKm; // 每月公里
|
|||
|
|||
@ApiModelProperty("滤芯到期公里") |
|||
private Integer filter_km; // 滤芯到期公里
|
|||
|
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone = "GMT+8") |
|||
@ApiModelProperty("滤芯到期日期") |
|||
private Date filter_date; // 滤芯到期日期
|
|||
|
|||
@ApiModelProperty("机油到期公里") |
|||
private Integer engineoil_km; // 机油到期公里
|
|||
|
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone = "GMT+8") |
|||
@ApiModelProperty("机油到期日期") |
|||
private Date engineoil_date; // 机油到期日期
|
|||
|
|||
@ApiModelProperty("齿轮油到期公里") |
|||
private Integer gearoil_km; // 齿轮油到期公里
|
|||
|
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone = "GMT+8") |
|||
@ApiModelProperty("齿轮油到期日期") |
|||
private Date gearoil_date; // 齿轮油到期日期
|
|||
|
|||
@ApiModelProperty("宝轮到期公里") |
|||
private Integer treasurewheel_km; // 宝轮到期公里
|
|||
|
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone = "GMT+8") |
|||
@ApiModelProperty("宝轮到期日期") |
|||
private Date treasurewheel_date; // 宝轮到期日期
|
|||
|
|||
@ApiModelProperty("风扇皮带到期公里") |
|||
private Integer fanbelt_km; // 风扇皮带到期公里
|
|||
|
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone = "GMT+8") |
|||
@ApiModelProperty("风扇皮带到期日期") |
|||
private Date fanbelt_date; // 风扇皮带到期日期
|
|||
|
|||
@ApiModelProperty("刹车片到期公里") |
|||
private Integer brakepads_km; // 刹车片到期公里
|
|||
|
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone = "GMT+8") |
|||
@ApiModelProperty("刹车片到期日期") |
|||
private Date brakepads_date; // 刹车片到期日期
|
|||
|
|||
} |
@ -0,0 +1,99 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.as.api.ascustomervehmaintenance; |
|||
|
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableField; |
|||
import com.yxt.common.core.vo.Vo; |
|||
|
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import java.util.Date; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: as(车辆保养明细) <br/> |
|||
* File: AsCustomervehMaintenanceVo.java <br/> |
|||
* Class: com.yxt.anrui.as.api.ascustomervehmaintenance.AsCustomervehMaintenanceVo <br/> |
|||
* Description: 客户保养记录(到期明细) 视图数据对象. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 10:04:53 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "客户保养记录(到期明细) 视图数据详情", description = "客户保养记录(到期明细) 视图数据详情") |
|||
public class AsCustomervehMaintenanceDetailsVo implements Vo { |
|||
|
|||
private String sid; // sid
|
|||
|
|||
@ApiModelProperty("车辆sid") |
|||
private String vehSid; // 车辆sid
|
|||
@ApiModelProperty("行驶里程") |
|||
private String currentMileage; // 行驶里程
|
|||
@ApiModelProperty("每月公里") |
|||
private String MonthKm; // 每月公里
|
|||
@ApiModelProperty("滤芯到期公里") |
|||
private String filterKm; // 滤芯到期公里
|
|||
|
|||
@ApiModelProperty("滤芯到期日期") |
|||
private String filterDate; // 滤芯到期日期
|
|||
|
|||
@ApiModelProperty("机油到期公里") |
|||
private String engineoilKm; // 机油到期公里
|
|||
|
|||
@ApiModelProperty("机油到期日期") |
|||
private String engineoilDate; // 机油到期日期
|
|||
|
|||
@ApiModelProperty("齿轮油到期公里") |
|||
private String gearoilKm; // 齿轮油到期公里
|
|||
|
|||
@ApiModelProperty("齿轮油到期日期") |
|||
private String gearoilDate; // 齿轮油到期日期
|
|||
|
|||
@ApiModelProperty("宝轮到期公里") |
|||
private String treasurewheelKm; // 宝轮到期公里
|
|||
|
|||
@ApiModelProperty("宝轮到期日期") |
|||
private String treasurewheelDate; // 宝轮到期日期
|
|||
|
|||
@ApiModelProperty("风扇皮带到期公里") |
|||
private String fanbeltKm; // 风扇皮带到期公里
|
|||
|
|||
@ApiModelProperty("风扇皮带到期日期") |
|||
private String fanbeltDate; // 风扇皮带到期日期
|
|||
|
|||
@ApiModelProperty("刹车片到期公里") |
|||
private String brakepadsKm; // 刹车片到期公里
|
|||
|
|||
@ApiModelProperty("刹车片到期日期") |
|||
private String brakepadsDate; // 刹车片到期日期
|
|||
|
|||
} |
@ -0,0 +1,98 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.as.api.ascustomervehmaintenance; |
|||
|
|||
|
|||
import com.yxt.common.core.dto.Dto; |
|||
|
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import java.util.Date; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: as(车辆保养明细) <br/> |
|||
* File: AsCustomervehMaintenanceDto.java <br/> |
|||
* Class: com.yxt.anrui.as.api.ascustomervehmaintenance.AsCustomervehMaintenanceDto <br/> |
|||
* Description: 客户保养记录(到期明细) 数据传输对象. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 10:04:53 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "客户保养记录(到期明细) 数据传输对象", description = "客户保养记录(到期明细) 数据传输对象") |
|||
public class AsCustomervehMaintenanceDto implements Dto { |
|||
|
|||
private String sid; // sid
|
|||
|
|||
@ApiModelProperty("车辆sid") |
|||
private String vehSid; // 车辆sid
|
|||
@ApiModelProperty("行驶里程") |
|||
private String currentMileage; // 行驶里程
|
|||
@ApiModelProperty("每月公里") |
|||
private String MonthKm; // 每月公里
|
|||
@ApiModelProperty("滤芯到期公里") |
|||
private String filterKm; // 滤芯到期公里
|
|||
|
|||
@ApiModelProperty("滤芯到期日期") |
|||
private String filterDate; // 滤芯到期日期
|
|||
|
|||
@ApiModelProperty("机油到期公里") |
|||
private String engineoilKm; // 机油到期公里
|
|||
|
|||
@ApiModelProperty("机油到期日期") |
|||
private String engineoilDate; // 机油到期日期
|
|||
|
|||
@ApiModelProperty("齿轮油到期公里") |
|||
private String gearoilKm; // 齿轮油到期公里
|
|||
|
|||
@ApiModelProperty("齿轮油到期日期") |
|||
private String gearoilDate; // 齿轮油到期日期
|
|||
|
|||
@ApiModelProperty("宝轮到期公里") |
|||
private String treasurewheelKm; // 宝轮到期公里
|
|||
|
|||
@ApiModelProperty("宝轮到期日期") |
|||
private String treasurewheelDate; // 宝轮到期日期
|
|||
|
|||
@ApiModelProperty("风扇皮带到期公里") |
|||
private String fanbeltKm; // 风扇皮带到期公里
|
|||
|
|||
@ApiModelProperty("风扇皮带到期日期") |
|||
private String fanbeltDate; // 风扇皮带到期日期
|
|||
|
|||
@ApiModelProperty("刹车片到期公里") |
|||
private String brakepadsKm; // 刹车片到期公里
|
|||
|
|||
@ApiModelProperty("刹车片到期日期") |
|||
private String brakepadsDate; // 刹车片到期日期
|
|||
|
|||
} |
@ -0,0 +1,97 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.as.api.ascustomervehmaintenance; |
|||
|
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
|
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import java.util.Date; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: as(车辆保养明细) <br/> |
|||
* File: AsCustomervehMaintenanceQuery.java <br/> |
|||
* Class: com.yxt.anrui.as.api.ascustomervehmaintenance.AsCustomervehMaintenanceQuery <br/> |
|||
* Description: 客户保养记录(到期明细) 查询条件. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 10:04:53 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "客户保养记录(到期明细) 查询条件", description = "客户保养记录(到期明细) 查询条件") |
|||
public class AsCustomervehMaintenanceQuery implements Query { |
|||
|
|||
@ApiModelProperty("车辆sid") |
|||
private String vehSid; // 车辆sid
|
|||
@ApiModelProperty("行驶里程") |
|||
private Integer currentMileage; // 行驶里程
|
|||
@ApiModelProperty("每月公里") |
|||
private Integer MonthKm; // 每月公里
|
|||
@ApiModelProperty("滤芯到期公里") |
|||
private Integer filter_km; // 滤芯到期公里
|
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone = "GMT+8") |
|||
@ApiModelProperty("滤芯到期日期") |
|||
private Date filter_dateStart; // 滤芯到期日期
|
|||
private Date filter_dateEnd; // 滤芯到期日期
|
|||
@ApiModelProperty("机油到期公里") |
|||
private Integer engineoil_km; // 机油到期公里
|
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone = "GMT+8") |
|||
@ApiModelProperty("机油到期日期") |
|||
private Date engineoil_dateStart; // 机油到期日期
|
|||
private Date engineoil_dateEnd; // 机油到期日期
|
|||
@ApiModelProperty("齿轮油到期公里") |
|||
private Integer gearoil_km; // 齿轮油到期公里
|
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone = "GMT+8") |
|||
@ApiModelProperty("齿轮油到期日期") |
|||
private Date gearoil_dateStart; // 齿轮油到期日期
|
|||
private Date gearoil_dateEnd; // 齿轮油到期日期
|
|||
@ApiModelProperty("宝轮到期公里") |
|||
private Integer treasurewheel_km; // 宝轮到期公里
|
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone = "GMT+8") |
|||
@ApiModelProperty("宝轮到期日期") |
|||
private Date treasurewheel_dateStart; // 宝轮到期日期
|
|||
private Date treasurewheel_dateEnd; // 宝轮到期日期
|
|||
@ApiModelProperty("风扇皮带到期公里") |
|||
private Integer fanbelt_km; // 风扇皮带到期公里
|
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone = "GMT+8") |
|||
@ApiModelProperty("风扇皮带到期日期") |
|||
private Date fanbelt_dateStart; // 风扇皮带到期日期
|
|||
private Date fanbelt_dateEnd; // 风扇皮带到期日期
|
|||
@ApiModelProperty("刹车片到期公里") |
|||
private Integer brakepads_km; // 刹车片到期公里
|
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone = "GMT+8") |
|||
@ApiModelProperty("刹车片到期日期") |
|||
private Date brakepads_dateStart; // 刹车片到期日期
|
|||
private Date brakepads_dateEnd; // 刹车片到期日期
|
|||
|
|||
} |
@ -0,0 +1,99 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.as.api.ascustomervehmaintenance; |
|||
|
|||
|
|||
import com.yxt.common.core.vo.Vo; |
|||
|
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import java.util.Date; |
|||
import io.swagger.annotations.ApiModel; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* Project: as(车辆保养明细) <br/> |
|||
* File: AsCustomervehMaintenanceVo.java <br/> |
|||
* Class: com.yxt.anrui.as.api.ascustomervehmaintenance.AsCustomervehMaintenanceVo <br/> |
|||
* Description: 客户保养记录(到期明细) 视图数据对象. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 10:04:53 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Data |
|||
@ApiModel(value = "客户保养记录(到期明细) 视图数据对象", description = "客户保养记录(到期明细) 视图数据对象") |
|||
public class AsCustomervehMaintenanceVo implements Vo { |
|||
|
|||
private String sid; // sid
|
|||
|
|||
@ApiModelProperty("车辆sid") |
|||
private String vehSid; // 车辆sid
|
|||
@ApiModelProperty("行驶里程") |
|||
private Integer currentMileage; // 行驶里程
|
|||
@ApiModelProperty("每月公里") |
|||
private Integer MonthKm; // 每月公里
|
|||
@ApiModelProperty("滤芯到期公里") |
|||
private Integer filter_km; // 滤芯到期公里
|
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone = "GMT+8") |
|||
@ApiModelProperty("滤芯到期日期") |
|||
private Date filter_dateStart; // 滤芯到期日期
|
|||
private Date filter_dateEnd; // 滤芯到期日期
|
|||
@ApiModelProperty("机油到期公里") |
|||
private Integer engineoil_km; // 机油到期公里
|
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone = "GMT+8") |
|||
@ApiModelProperty("机油到期日期") |
|||
private Date engineoil_dateStart; // 机油到期日期
|
|||
private Date engineoil_dateEnd; // 机油到期日期
|
|||
@ApiModelProperty("齿轮油到期公里") |
|||
private Integer gearoil_km; // 齿轮油到期公里
|
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone = "GMT+8") |
|||
@ApiModelProperty("齿轮油到期日期") |
|||
private Date gearoil_dateStart; // 齿轮油到期日期
|
|||
private Date gearoil_dateEnd; // 齿轮油到期日期
|
|||
@ApiModelProperty("宝轮到期公里") |
|||
private Integer treasurewheel_km; // 宝轮到期公里
|
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone = "GMT+8") |
|||
@ApiModelProperty("宝轮到期日期") |
|||
private Date treasurewheel_dateStart; // 宝轮到期日期
|
|||
private Date treasurewheel_dateEnd; // 宝轮到期日期
|
|||
@ApiModelProperty("风扇皮带到期公里") |
|||
private Integer fanbelt_km; // 风扇皮带到期公里
|
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone = "GMT+8") |
|||
@ApiModelProperty("风扇皮带到期日期") |
|||
private Date fanbelt_dateStart; // 风扇皮带到期日期
|
|||
private Date fanbelt_dateEnd; // 风扇皮带到期日期
|
|||
@ApiModelProperty("刹车片到期公里") |
|||
private Integer brakepads_km; // 刹车片到期公里
|
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone = "GMT+8") |
|||
@ApiModelProperty("刹车片到期日期") |
|||
private Date brakepads_dateStart; // 刹车片到期日期
|
|||
private Date brakepads_dateEnd; // 刹车片到期日期
|
|||
|
|||
} |
@ -0,0 +1,72 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.as.biz.ascustomervehmaintenance; |
|||
|
|||
import com.baomidou.mybatisplus.core.conditions.Wrapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.baomidou.mybatisplus.core.toolkit.Constants; |
|||
import org.apache.ibatis.annotations.Delete; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
import org.apache.ibatis.annotations.Param; |
|||
import org.apache.ibatis.annotations.Select; |
|||
import com.yxt.anrui.as.api.ascustomervehmaintenance.AsCustomervehMaintenance; |
|||
import com.yxt.anrui.as.api.ascustomervehmaintenance.AsCustomervehMaintenanceVo; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* Project: as(车辆保养明细) <br/> |
|||
* File: AsCustomervehMaintenanceMapper.java <br/> |
|||
* Class: com.yxt.anrui.as.biz.ascustomervehmaintenance.AsCustomervehMaintenanceMapper <br/> |
|||
* Description: 客户保养记录(到期明细). <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 10:04:53 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Mapper |
|||
public interface AsCustomervehMaintenanceMapper extends BaseMapper<AsCustomervehMaintenance> { |
|||
|
|||
//@Update("update as_customerveh_maintenance set name=#{msg} where id=#{id}")
|
|||
//IPage<AsCustomervehMaintenanceVo> voPage(IPage<AsCustomervehMaintenance> page, @Param(Constants.WRAPPER) QueryWrapper<AsCustomervehMaintenance> qw);
|
|||
|
|||
IPage<AsCustomervehMaintenanceVo> selectPageVo(IPage<AsCustomervehMaintenance> page, @Param(Constants.WRAPPER) Wrapper<AsCustomervehMaintenance> qw); |
|||
|
|||
List<AsCustomervehMaintenanceVo> selectListAllVo(@Param(Constants.WRAPPER) Wrapper<AsCustomervehMaintenance> qw); |
|||
|
|||
@Select("select * from as_customerveh_maintenance") |
|||
List<AsCustomervehMaintenanceVo> selectListVo(); |
|||
|
|||
@Select("select * from as_customerveh_maintenance where vehSid =#{vehSid}") |
|||
AsCustomervehMaintenance fetchByVehSid(@Param("vehSid") String vehSid); |
|||
|
|||
@Delete("delete from as_customerveh_maintenance where vehSid =#{vehSid}") |
|||
void delByVehSid(@Param("vehSid") String vehSid); |
|||
} |
@ -0,0 +1,13 @@ |
|||
<?xml version="1.0" encoding="UTF-8" ?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.anrui.as.biz.ascustomervehmaintenance.AsCustomervehMaintenanceMapper"> |
|||
<!-- <where> ${ew.sqlSegment} </where>--> |
|||
<!-- ${ew.customSqlSegment} --> |
|||
<select id="selectPageVo" resultType="com.yxt.anrui.as.api.ascustomervehmaintenance.AsCustomervehMaintenanceVo"> |
|||
SELECT * FROM as_customerveh_maintenance <where> ${ew.sqlSegment} </where> |
|||
</select> |
|||
|
|||
<select id="selectListAllVo" resultType="com.yxt.anrui.as.api.ascustomervehmaintenance.AsCustomervehMaintenanceVo"> |
|||
SELECT * FROM as_customerveh_maintenance <where> ${ew.sqlSegment} </where> |
|||
</select> |
|||
</mapper> |
@ -0,0 +1,97 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.as.biz.ascustomervehmaintenance; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import com.yxt.anrui.as.api.ascustomervehmaintenance.AsCustomervehMaintenanceQuery; |
|||
import com.yxt.anrui.as.api.ascustomervehmaintenance.AsCustomervehMaintenanceVo; |
|||
import com.yxt.anrui.as.api.ascustomervehmaintenance.AsCustomervehMaintenanceDetailsVo; |
|||
import com.yxt.anrui.as.api.ascustomervehmaintenance.AsCustomervehMaintenanceDto; |
|||
|
|||
/** |
|||
* Project: as(车辆保养明细) <br/> |
|||
* File: AsCustomervehMaintenanceFeignFallback.java <br/> |
|||
* Class: com.yxt.anrui.as.biz.ascustomervehmaintenance.AsCustomervehMaintenanceRest <br/> |
|||
* Description: 客户保养记录(到期明细). <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 10:04:53 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Api(tags = "客户保养记录(到期明细)") |
|||
@RestController("com.yxt.anrui.as.biz.ascustomervehmaintenance.AsCustomervehMaintenanceRest") |
|||
@RequestMapping("v1/ascustomervehmaintenance") |
|||
public class AsCustomervehMaintenanceRest{ |
|||
|
|||
@Autowired |
|||
private AsCustomervehMaintenanceService asCustomervehMaintenanceService; |
|||
|
|||
|
|||
@ApiOperation("根据条件分页查询数据的列表") |
|||
@PostMapping("/listPage") |
|||
public ResultBean<PagerVo<AsCustomervehMaintenanceVo>> listPage(@RequestBody PagerQuery<AsCustomervehMaintenanceQuery> pq){ |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
PagerVo<AsCustomervehMaintenanceVo> pv = asCustomervehMaintenanceService.listPageVo(pq); |
|||
return rb.success().setData(pv); |
|||
} |
|||
|
|||
|
|||
@ApiOperation("新增或修改") |
|||
@PostMapping("/save") |
|||
public ResultBean save(@RequestBody AsCustomervehMaintenanceDto dto){ |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
asCustomervehMaintenanceService.saveOrUpdateDto(dto); |
|||
return rb.success(); |
|||
} |
|||
|
|||
|
|||
@ApiOperation("根据sid批量删除") |
|||
@PostMapping("/delBySids") |
|||
public ResultBean delBySids(@RequestBody String[] sids){ |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
asCustomervehMaintenanceService.delBySids(sids); |
|||
return rb.success(); |
|||
} |
|||
|
|||
|
|||
@ApiOperation("根据SID获取一条记录") |
|||
@GetMapping("/fetchDetailsBySid/{sid}") |
|||
public ResultBean<AsCustomervehMaintenanceDetailsVo> fetchDetailsBySid(@PathVariable("sid") String sid){ |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
AsCustomervehMaintenanceDetailsVo vo = asCustomervehMaintenanceService.fetchDetailsVoBySid(sid); |
|||
return rb.success().setData(vo); |
|||
} |
|||
} |
@ -0,0 +1,114 @@ |
|||
/********************************************************* |
|||
********************************************************* |
|||
******************** ******************* |
|||
************* ************ |
|||
******* _oo0oo_ ******* |
|||
*** o8888888o *** |
|||
* 88" . "88 * |
|||
* (| -_- |) * |
|||
* 0\ = /0 * |
|||
* ___/`---'\___ * |
|||
* .' \\| |// '. *
|
|||
* / \\||| : |||// \ *
|
|||
* / _||||| -:- |||||- \ * |
|||
* | | \\\ - /// | | *
|
|||
* | \_| ''\---/'' |_/ | * |
|||
* \ .-\__ '-' ___/-. / * |
|||
* ___'. .' /--.--\ `. .'___ * |
|||
* ."" '< `.___\_<|>_/___.' >' "". * |
|||
* | | : `- \`.;`\ _ /`;.`/ - ` : | | * |
|||
* \ \ `_. \_ __\ /__ _/ .-` / / * |
|||
* =====`-.____`.___ \_____/___.-`___.-'===== * |
|||
* `=---=' * |
|||
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ * |
|||
*********__佛祖保佑__永无BUG__验收通过__钞票多多__********* |
|||
*********************************************************/ |
|||
package com.yxt.anrui.as.biz.ascustomervehmaintenance; |
|||
|
|||
import cn.hutool.core.bean.BeanUtil; |
|||
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import org.apache.commons.lang3.StringUtils; |
|||
import com.yxt.common.base.service.MybatisBaseService; |
|||
import com.yxt.common.base.utils.PagerUtil; |
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import com.yxt.anrui.as.api.ascustomervehmaintenance.AsCustomervehMaintenance; |
|||
import com.yxt.anrui.as.api.ascustomervehmaintenance.AsCustomervehMaintenanceQuery; |
|||
import com.yxt.anrui.as.api.ascustomervehmaintenance.AsCustomervehMaintenanceVo; |
|||
import com.yxt.anrui.as.api.ascustomervehmaintenance.AsCustomervehMaintenanceDetailsVo; |
|||
import com.yxt.anrui.as.api.ascustomervehmaintenance.AsCustomervehMaintenanceDto; |
|||
|
|||
import org.springframework.stereotype.Service; |
|||
|
|||
/** |
|||
* Project: as(车辆保养明细) <br/> |
|||
* File: AsCustomervehMaintenanceService.java <br/> |
|||
* Class: com.yxt.anrui.as.biz.ascustomervehmaintenance.AsCustomervehMaintenanceService <br/> |
|||
* Description: 客户保养记录(到期明细) 业务逻辑. <br/> |
|||
* Copyright: Copyright (c) 2011 <br/> |
|||
* Company: https://gitee.com/liuzp315 <br/>
|
|||
* Makedate: 2024-06-03 10:04:53 <br/> |
|||
* |
|||
* @author liupopo |
|||
* @version 1.0 |
|||
* @since 1.0 |
|||
*/ |
|||
@Service |
|||
public class AsCustomervehMaintenanceService extends MybatisBaseService<AsCustomervehMaintenanceMapper, AsCustomervehMaintenance> { |
|||
private QueryWrapper<AsCustomervehMaintenance> createQueryWrapper(AsCustomervehMaintenanceQuery query) { |
|||
// todo: 这里根据具体业务调整查询条件
|
|||
// 多字段Like示例:qw.and(wrapper -> wrapper.like("name", query.getName()).or().like("remark", query.getName()));
|
|||
QueryWrapper<AsCustomervehMaintenance> qw = new QueryWrapper<>(); |
|||
return qw; |
|||
} |
|||
|
|||
public AsCustomervehMaintenance fetchByVehSid(String vehSid) { |
|||
return baseMapper.fetchByVehSid(vehSid); |
|||
} |
|||
|
|||
public PagerVo<AsCustomervehMaintenanceVo> listPageVo(PagerQuery<AsCustomervehMaintenanceQuery> pq) { |
|||
AsCustomervehMaintenanceQuery query = pq.getParams(); |
|||
QueryWrapper<AsCustomervehMaintenance> qw = createQueryWrapper(query); |
|||
IPage<AsCustomervehMaintenance> page = PagerUtil.queryToPage(pq); |
|||
IPage<AsCustomervehMaintenanceVo> pagging = baseMapper.selectPageVo(page, qw); |
|||
PagerVo<AsCustomervehMaintenanceVo> p = PagerUtil.pageToVo(pagging, null); |
|||
return p; |
|||
} |
|||
|
|||
public void saveOrUpdateDto(AsCustomervehMaintenanceDto dto){ |
|||
String dtoSid = dto.getSid(); |
|||
if (StringUtils.isBlank(dtoSid)) { |
|||
this.insertByDto(dto); |
|||
return; |
|||
} |
|||
this.updateByDto(dto); |
|||
} |
|||
|
|||
public void insertByDto(AsCustomervehMaintenanceDto dto){ |
|||
AsCustomervehMaintenance entity = new AsCustomervehMaintenance(); |
|||
BeanUtil.copyProperties(dto, entity, "id", "sid"); |
|||
baseMapper.insert(entity); |
|||
} |
|||
|
|||
public void updateByDto(AsCustomervehMaintenanceDto dto){ |
|||
String dtoSid = dto.getSid(); |
|||
if (StringUtils.isBlank(dtoSid)) { |
|||
return; |
|||
} |
|||
AsCustomervehMaintenance entity = fetchBySid(dtoSid); |
|||
BeanUtil.copyProperties(dto, entity, "id", "sid"); |
|||
baseMapper.updateById(entity); |
|||
} |
|||
|
|||
public AsCustomervehMaintenanceDetailsVo fetchDetailsVoBySid(String sid){ |
|||
AsCustomervehMaintenance entity = fetchBySid(sid); |
|||
AsCustomervehMaintenanceDetailsVo vo = new AsCustomervehMaintenanceDetailsVo(); |
|||
BeanUtil.copyProperties(entity, vo); |
|||
return vo; |
|||
} |
|||
|
|||
public void delByVehSid(String sid) { |
|||
baseMapper.delByVehSid(sid); |
|||
} |
|||
} |
Loading…
Reference in new issue