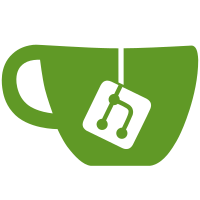
8 changed files with 477 additions and 171 deletions
@ -0,0 +1,24 @@ |
|||
package com.yxt.yythmall.adminapi; |
|||
|
|||
|
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.yythmall.adminapi.ordertools.OrdertoolsService; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.web.bind.annotation.*; |
|||
import org.springframework.web.multipart.MultipartFile; |
|||
|
|||
@RestController("com.yxt.yythmall.adminapi.OrdertoolsRest") |
|||
@RequestMapping("/adminapi/ordertools") |
|||
public class OrdertoolsRest { |
|||
@Autowired |
|||
private OrdertoolsService ordertoolsService; |
|||
|
|||
@ApiOperation("上传和导出") |
|||
@PostMapping("/uploadExcelFile") |
|||
public ResultBean uploadExcelFile(@RequestParam("file") MultipartFile file) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
String fileUrl = ordertoolsService.uploadAndResetData(file); |
|||
return rb.success().setData(fileUrl); |
|||
} |
|||
} |
@ -0,0 +1,71 @@ |
|||
package com.yxt.yythmall.adminapi.ordertools; |
|||
|
|||
import cn.hutool.core.bean.BeanUtil; |
|||
import cn.hutool.core.util.StrUtil; |
|||
import com.alibaba.excel.EasyExcel; |
|||
import com.alibaba.excel.context.AnalysisContext; |
|||
import com.alibaba.excel.read.builder.ExcelReaderBuilder; |
|||
import com.alibaba.excel.read.listener.ReadListener; |
|||
import com.yxt.common.base.config.component.FileUploadComponent; |
|||
import com.yxt.common.core.result.FileUploadResult; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.yythmall.biz.ordorder.OrdOrderService; |
|||
import org.apache.commons.lang3.StringUtils; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.stereotype.Service; |
|||
import org.springframework.web.multipart.MultipartFile; |
|||
|
|||
import java.util.ArrayList; |
|||
import java.util.List; |
|||
|
|||
@Service |
|||
public class OrdertoolsService { |
|||
|
|||
@Autowired |
|||
private FileUploadComponent fileUploadComponent; |
|||
|
|||
@Autowired |
|||
private OrdOrderService ordOrderService; |
|||
|
|||
public String uploadAndResetData(MultipartFile file) { |
|||
ResultBean<FileUploadResult> fub = fileUploadComponent.uploadFile(file, "zfdd"); |
|||
String filePath = fub.getData().getFilePath(); |
|||
String fp = fileUploadComponent.getUploadPath() + filePath; |
|||
String outFilePath = "/zfdd/fhd" + System.currentTimeMillis() + ".xlsx"; |
|||
String outFileName = fileUploadComponent.getUploadPath() + outFilePath; |
|||
String outFileUrl = fileUploadComponent.getUrlPrefix() + outFilePath; |
|||
|
|||
List<WxOrderIn> inList = new ArrayList<>(); |
|||
ExcelReaderBuilder read = EasyExcel.read(fp, WxOrderIn.class, createReadListener(inList)); |
|||
|
|||
List<ToImportOrderExcel> toList = new ArrayList<>(); |
|||
inList.forEach(inObj -> { |
|||
ToImportOrderExcel toObj = new ToImportOrderExcel(); |
|||
toObj.setTranOrderNo(inObj.getTranOrderNo()); |
|||
toObj.setMerchantOrderNo(inObj.getMerchantOrderNo()); |
|||
toObj.setMerchantNo(inObj.getMerchantNo()); |
|||
toObj.setGoodsNames(ordOrderService.orderGoodsNames(inObj.getMerchantOrderNo())); |
|||
toList.add(toObj); |
|||
}); |
|||
|
|||
// ArrayList EasyExcel.write(response.getOutputStream(), ReserveCustomerExcel.class).sheet("预约单明细").doWrite(list);
|
|||
|
|||
|
|||
read.sheet().doRead(); |
|||
return ""; |
|||
} |
|||
|
|||
private ReadListener<WxOrderIn> createReadListener(List<WxOrderIn> inList) { |
|||
|
|||
return new ReadListener<WxOrderIn>() { |
|||
@Override |
|||
public void invoke(WxOrderIn obj, AnalysisContext analysisContext) { |
|||
inList.add(obj); |
|||
} |
|||
|
|||
@Override |
|||
public void doAfterAllAnalysed(AnalysisContext analysisContext) { |
|||
} |
|||
}; |
|||
} |
|||
} |
@ -0,0 +1,44 @@ |
|||
package com.yxt.yythmall.adminapi.ordertools; |
|||
|
|||
import com.alibaba.excel.annotation.ExcelProperty; |
|||
import com.alibaba.excel.annotation.write.style.ColumnWidth; |
|||
import lombok.EqualsAndHashCode; |
|||
import lombok.Getter; |
|||
import lombok.Setter; |
|||
|
|||
@Getter |
|||
@Setter |
|||
@EqualsAndHashCode |
|||
public class ToImportOrderExcel { |
|||
@ColumnWidth(20) |
|||
@ExcelProperty(value = "交易单号",index = 0) |
|||
private String tranOrderNo; // 交易单号
|
|||
@ColumnWidth(20) |
|||
@ExcelProperty(value = "商户单号",index = 1) |
|||
private String merchantOrderNo; // 商户单号
|
|||
@ColumnWidth(20) |
|||
@ExcelProperty(value = "商户号",index = 2) |
|||
private String merchantNo;// 商户号
|
|||
@ColumnWidth(20) |
|||
@ExcelProperty(value = "发货方式",index = 3) |
|||
private String modeOfDespatch = "用户自提";// 发货方式
|
|||
@ColumnWidth(20) |
|||
@ExcelProperty(value = "发货模式",index = 4) |
|||
private String deliveryMode = "统一发货";// 发货模式
|
|||
@ColumnWidth(20) |
|||
@ExcelProperty(value = "快递公司",index = 5) |
|||
private String kdgs = ""; // 快递公司
|
|||
@ColumnWidth(20) |
|||
@ExcelProperty(value = "快递单号(多个快递单使用;分隔)",index = 6) |
|||
private String kddh = ""; // 快递单号(多个快递单使用;分隔)
|
|||
@ColumnWidth(20) |
|||
@ExcelProperty(value = "是否完成发货",index = 7) |
|||
private String sfwcfh = ""; // 是否完成发货
|
|||
@ColumnWidth(20) |
|||
@ExcelProperty(value = "是否重新发货",index = 8) |
|||
private String sfcxfh = ""; // 是否重新发货
|
|||
@ColumnWidth(20) |
|||
@ExcelProperty(value = "商品信息",index = 9) |
|||
private String goodsNames; // 商品信息
|
|||
|
|||
} |
@ -0,0 +1,12 @@ |
|||
package com.yxt.yythmall.adminapi.ordertools; |
|||
|
|||
import lombok.Data; |
|||
|
|||
@Data |
|||
public class WxOrderIn { |
|||
private String tranTime; // 支付时间
|
|||
private String tranOrderNo; // 交易单号
|
|||
private String merchantOrderNo; // 商户单号
|
|||
private String merchantNo;// 商户号
|
|||
private String status; // 状态
|
|||
} |
@ -0,0 +1,9 @@ |
|||
package com.yxt.yythmall.biz.ordorder; |
|||
|
|||
import lombok.Data; |
|||
|
|||
@Data |
|||
public class OrdOrderGoodsItem { |
|||
private String goodsName; |
|||
private int goodsNumber; |
|||
} |
Loading…
Reference in new issue