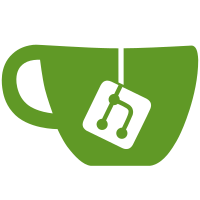
12 changed files with 312 additions and 59 deletions
@ -0,0 +1,29 @@ |
|||
package com.yxt.yythmall.api.lpkcustomerbank; |
|||
|
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
import java.util.UUID; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2024/1/28 |
|||
**/ |
|||
@Data |
|||
public class LpkCustomerBank { |
|||
private String id; |
|||
private String sid = UUID.randomUUID().toString(); |
|||
private String createTime; |
|||
private String remarks; |
|||
private String isEnable; |
|||
private String name; |
|||
private String shortName; |
|||
private String linker; |
|||
private String linkPhone; |
|||
private String address; |
|||
@ApiModelProperty("经度") |
|||
private String lon; |
|||
@ApiModelProperty("纬度") |
|||
private String lat; |
|||
} |
@ -0,0 +1,19 @@ |
|||
package com.yxt.yythmall.api.lpkcustomerbank; |
|||
|
|||
import com.yxt.common.core.query.Query; |
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2024/1/28 |
|||
**/ |
|||
@Data |
|||
public class LpkCustomerBankQuery implements Query { |
|||
|
|||
@ApiModelProperty("名称") |
|||
private String name; |
|||
|
|||
|
|||
} |
@ -0,0 +1,17 @@ |
|||
package com.yxt.yythmall.api.lpkcustomerbank; |
|||
|
|||
import io.swagger.annotations.ApiModelProperty; |
|||
import lombok.Data; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2024/1/28 |
|||
**/ |
|||
@Data |
|||
public class LpkCustomerBankVo { |
|||
@ApiModelProperty("支行sid") |
|||
private String bankSid; |
|||
@ApiModelProperty("支行名称") |
|||
private String bankName; |
|||
} |
@ -0,0 +1,24 @@ |
|||
package com.yxt.yythmall.biz.lpkcustomerbank; |
|||
|
|||
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import com.baomidou.mybatisplus.core.toolkit.Constants; |
|||
import com.yxt.yythmall.api.lpkcustomerbank.LpkCustomerBank; |
|||
import com.yxt.yythmall.api.lpkcustomerbank.LpkCustomerBankVo; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
import org.apache.ibatis.annotations.Param; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2024/1/28 |
|||
**/ |
|||
@Mapper |
|||
public interface LpkCustomerBankMapper extends BaseMapper<LpkCustomerBank> { |
|||
IPage<LpkCustomerBankVo> selectPageVo(IPage<LpkCustomerBank> page, @Param(Constants.WRAPPER)QueryWrapper<LpkCustomerBank> qw); |
|||
|
|||
List<LpkCustomerBankVo> getBankList(@Param(Constants.WRAPPER)QueryWrapper<LpkCustomerBank> qw); |
|||
} |
@ -0,0 +1,21 @@ |
|||
<?xml version="1.0" encoding="UTF-8" ?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.biz.lpkcustomerbank.LpkCustomerBankMapper"> |
|||
<select id="selectPageVo" resultType="com.yxt.yythmall.api.lpkcustomerbank.LpkCustomerBankVo"> |
|||
select lb.sid bankSid, |
|||
lb.name bankName |
|||
from lpk_customer_bank lb |
|||
<where> |
|||
${ew.sqlSegment} |
|||
</where> |
|||
</select> |
|||
|
|||
<select id="getBankList" resultType="com.yxt.yythmall.api.lpkcustomerbank.LpkCustomerBankVo"> |
|||
select lb.sid bankSid, |
|||
lb.name bankName |
|||
from lpk_customer_bank lb |
|||
<where> |
|||
${ew.sqlSegment} |
|||
</where> |
|||
</select> |
|||
</mapper> |
@ -0,0 +1,45 @@ |
|||
package com.yxt.yythmall.biz.lpkcustomerbank; |
|||
|
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import com.yxt.yythmall.api.lpkbank.LpkBankQuery; |
|||
import com.yxt.yythmall.api.lpkbank.LpkBankVo; |
|||
import com.yxt.yythmall.api.lpkcustomerbank.LpkCustomerBankQuery; |
|||
import com.yxt.yythmall.api.lpkcustomerbank.LpkCustomerBankVo; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2024/1/28 |
|||
**/ |
|||
@Api(tags = "支行信息(十八家支行)") |
|||
@RestController |
|||
@RequestMapping("LpkCustomerBank") |
|||
public class LpkCustomerBankRest { |
|||
|
|||
@Autowired |
|||
private LpkCustomerBankService lpkCustomerBankService; |
|||
|
|||
@ApiOperation("分页列表") |
|||
@PostMapping("/listPage") |
|||
public ResultBean<PagerVo<LpkCustomerBankVo>> ListPageVo(@RequestBody PagerQuery<LpkCustomerBankQuery> pq) { |
|||
ResultBean rb = ResultBean.fireFail(); |
|||
PagerVo<LpkCustomerBankVo> pv = lpkCustomerBankService.listPageVo(pq); |
|||
return rb.success().setData(pv); |
|||
} |
|||
|
|||
@ApiOperation("支行信息列表") |
|||
@GetMapping("/getBankList") |
|||
ResultBean<List<LpkCustomerBankVo>> getBankList(@RequestParam(value = "name", required = false) String name) { |
|||
return lpkCustomerBankService.getBankList(name); |
|||
} |
|||
|
|||
|
|||
} |
@ -0,0 +1,54 @@ |
|||
package com.yxt.yythmall.biz.lpkcustomerbank; |
|||
|
|||
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
|||
import com.baomidou.mybatisplus.core.metadata.IPage; |
|||
import com.yxt.common.base.service.MybatisBaseService; |
|||
import com.yxt.common.base.utils.PagerUtil; |
|||
import com.yxt.common.core.query.PagerQuery; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.common.core.vo.PagerVo; |
|||
import com.yxt.yythmall.api.lpkbank.LpkBankQuery; |
|||
import com.yxt.yythmall.api.lpkbank.LpkBankVo; |
|||
import com.yxt.yythmall.api.lpkcustomerbank.LpkCustomerBank; |
|||
import com.yxt.yythmall.api.lpkcustomerbank.LpkCustomerBankQuery; |
|||
import com.yxt.yythmall.api.lpkcustomerbank.LpkCustomerBankVo; |
|||
import org.apache.commons.lang3.StringUtils; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
import java.util.Collections; |
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @description: |
|||
* @author: dimengzhe |
|||
* @date: 2024/1/28 |
|||
**/ |
|||
@Service |
|||
public class LpkCustomerBankService extends MybatisBaseService<LpkCustomerBankMapper, LpkCustomerBank> { |
|||
|
|||
|
|||
public PagerVo<LpkCustomerBankVo> listPageVo(PagerQuery<LpkCustomerBankQuery> pq) { |
|||
LpkCustomerBankQuery query = pq.getParams(); |
|||
QueryWrapper<LpkCustomerBank> qw = new QueryWrapper<>(); |
|||
if (query != null) { |
|||
if (StringUtils.isNotBlank(query.getName())) { |
|||
qw.like("lb.name", query.getName()); |
|||
} |
|||
} |
|||
IPage<LpkCustomerBank> page = PagerUtil.queryToPage(pq); |
|||
IPage<LpkCustomerBankVo> pagging = baseMapper.selectPageVo(page, qw); |
|||
PagerVo<LpkCustomerBankVo> p = PagerUtil.pageToVo(pagging, null); |
|||
return p; |
|||
} |
|||
|
|||
public ResultBean<List<LpkCustomerBankVo>> getBankList(String name) { |
|||
ResultBean<List<LpkCustomerBankVo>> rb = ResultBean.fireFail(); |
|||
QueryWrapper<LpkCustomerBank> qw = new QueryWrapper<>(); |
|||
if (StringUtils.isNotBlank(name)) { |
|||
qw.like("lb.name", name); |
|||
} |
|||
List<LpkCustomerBankVo> list = baseMapper.getBankList(qw); |
|||
list.removeAll(Collections.singleton(null)); |
|||
return rb.success().setData(list); |
|||
} |
|||
} |
Loading…
Reference in new issue