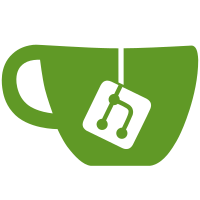
22 changed files with 709 additions and 325 deletions
@ -0,0 +1,31 @@ |
|||
package com.yxt.yythmall.api.vegereplenish; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
import java.util.UUID; |
|||
|
|||
/** |
|||
* @author Fan |
|||
* @description |
|||
* @date 2024/1/21 10:49 |
|||
*/ |
|||
|
|||
@ApiModel(value = "绑卡记录", description = "绑卡记录") |
|||
@TableName("vege_replenish") |
|||
@Data |
|||
public class VegeReplenish { |
|||
|
|||
private String id; |
|||
private String sid= UUID.randomUUID().toString(); |
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone="GMT+8") |
|||
private Date createTime; |
|||
private String remarks; |
|||
private String customerSid;//客户sid
|
|||
private String cardCode;//卡编码,个人卡、企业卡是卡编码,转赠记录的是转赠编码
|
|||
private String cardtype;//0 个人卡 1 企业卡 2 转赠的
|
|||
|
|||
} |
@ -0,0 +1,32 @@ |
|||
package com.yxt.yythmall.api.vegereplenishdetail; |
|||
|
|||
import com.baomidou.mybatisplus.annotation.TableName; |
|||
import com.fasterxml.jackson.annotation.JsonFormat; |
|||
import io.swagger.annotations.ApiModel; |
|||
import lombok.Data; |
|||
|
|||
import java.util.Date; |
|||
import java.util.UUID; |
|||
|
|||
/** |
|||
* @author Fan |
|||
* @description |
|||
* @date 2024/1/21 10:49 |
|||
*/ |
|||
|
|||
@ApiModel(value = "绑卡记录商品信息", description = "绑卡记录商品信息") |
|||
@TableName("vege_replenish_detail") |
|||
@Data |
|||
public class VegeReplenishDetail { |
|||
|
|||
private String id; |
|||
private String sid = UUID.randomUUID().toString(); |
|||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss", timezone = "GMT+8") |
|||
private Date createTime; |
|||
private String remarks; |
|||
private String orderSid;//订单SID
|
|||
private String goodsSid;//商品Sid
|
|||
private String goodsName;//商品名称
|
|||
private Integer goodsNumber;//商品数量
|
|||
|
|||
} |
@ -0,0 +1,19 @@ |
|||
package com.yxt.yythmall.biz.vegereplenish; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.yxt.yythmall.api.vegecallerreservedetails.VegeCellarReserveDetails; |
|||
import com.yxt.yythmall.api.vegereplenish.VegeReplenish; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
import org.apache.ibatis.annotations.Param; |
|||
import org.apache.ibatis.annotations.Select; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/23 10:36 |
|||
*/ |
|||
@Mapper |
|||
public interface VegeReplenishMapper extends BaseMapper<VegeReplenish> { |
|||
|
|||
} |
@ -0,0 +1,8 @@ |
|||
<?xml version="1.0" encoding="UTF-8" ?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.biz.vegereplenish.VegeReplenishMapper"> |
|||
<!-- <where> ${ew.sqlSegment} </where>--> |
|||
<!-- ${ew.customSqlSegment} --> |
|||
|
|||
|
|||
</mapper> |
@ -0,0 +1,27 @@ |
|||
package com.yxt.yythmall.biz.vegereplenish; |
|||
|
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.yythmall.api.lpkgiftcard.BindCardDto; |
|||
import io.swagger.annotations.Api; |
|||
import io.swagger.annotations.ApiOperation; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.web.bind.annotation.*; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/23 10:35 |
|||
*/ |
|||
@Api(tags = "绑卡记录") |
|||
@RestController |
|||
@RequestMapping("vegereplenish") |
|||
public class VegeReplenishRest { |
|||
@Autowired |
|||
VegeReplenishService vegeReplenishService; |
|||
|
|||
@ApiOperation("保存") |
|||
@PostMapping("/saveBindRecord") |
|||
public ResultBean<String> saveBindRecord(@RequestBody BindCardDto dto, @RequestParam("type") String type) { |
|||
return vegeReplenishService.saveBindRecord(dto,type); |
|||
} |
|||
|
|||
} |
@ -0,0 +1,129 @@ |
|||
package com.yxt.yythmall.biz.vegereplenish; |
|||
|
|||
import cn.hutool.core.bean.BeanUtil; |
|||
import cn.hutool.core.date.DateTime; |
|||
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
|||
import com.yxt.common.base.service.MybatisBaseService; |
|||
import com.yxt.common.core.result.ResultBean; |
|||
import com.yxt.yythmall.api.customerstore.CustomerStoreDto; |
|||
import com.yxt.yythmall.api.empcard.EmpCard; |
|||
import com.yxt.yythmall.api.empcardgift.EmpCardGift; |
|||
import com.yxt.yythmall.api.empcardgiftgoods.EmpCardGiftGoodsVo; |
|||
import com.yxt.yythmall.api.lpkgiftbag.GiftBagGoods; |
|||
import com.yxt.yythmall.api.lpkgiftbag.LpkGiftBagInitVo; |
|||
import com.yxt.yythmall.api.lpkgiftcard.BindCardDto; |
|||
import com.yxt.yythmall.api.lpkgiftcard.GoodsVo; |
|||
import com.yxt.yythmall.api.lpkgiftcard.LpkGiftCard; |
|||
import com.yxt.yythmall.api.lpkgoods.LpkGoods; |
|||
import com.yxt.yythmall.api.vegecallerreservedetails.VegeCellarReserveDetails; |
|||
import com.yxt.yythmall.api.vegecallerreserveorder.VegeCellarReserveOrderDto; |
|||
import com.yxt.yythmall.api.vegereplenish.VegeReplenish; |
|||
import com.yxt.yythmall.api.vegereplenishdetail.VegeReplenishDetail; |
|||
import com.yxt.yythmall.api.vegetablecellar.VegetableCellar; |
|||
import com.yxt.yythmall.biz.customerstore.CustomerStoreService; |
|||
import com.yxt.yythmall.biz.empcard.EmpCardService; |
|||
import com.yxt.yythmall.biz.empcardgift.EmpCardGiftService; |
|||
import com.yxt.yythmall.biz.empcardgiftgoods.EmpCardGiftGoodsService; |
|||
import com.yxt.yythmall.biz.lpkgiftbag.LpkGiftBagService; |
|||
import com.yxt.yythmall.biz.lpkgiftcard.LpkGiftCardService; |
|||
import com.yxt.yythmall.biz.lpkgoods.LpkGoodsService; |
|||
import com.yxt.yythmall.biz.vegereplenishdetail.VegeReplenishDetailService; |
|||
import com.yxt.yythmall.biz.vegetablecellar.VegetableCellarService; |
|||
import com.yxt.yythmall.mallplus.biz.util.StringUtils; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.stereotype.Service; |
|||
import org.springframework.transaction.annotation.Transactional; |
|||
|
|||
import java.util.List; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/23 10:36 |
|||
*/ |
|||
@Service |
|||
public class VegeReplenishService extends MybatisBaseService<VegeReplenishMapper, VegeReplenish> { |
|||
|
|||
@Autowired |
|||
private VegeReplenishDetailService vegeReplenishDetailService; |
|||
@Autowired |
|||
private LpkGiftCardService lpkGiftCardService; |
|||
@Autowired |
|||
private LpkGiftBagService lpkGiftBagService; |
|||
@Autowired |
|||
private EmpCardService empCardService; |
|||
@Autowired |
|||
private EmpCardGiftService empCardGiftService; |
|||
@Autowired |
|||
private EmpCardGiftGoodsService empCardGiftGoodsService; |
|||
|
|||
@Transactional(rollbackFor = Exception.class) |
|||
public ResultBean<String> saveBindRecord(BindCardDto dto, String type) { |
|||
ResultBean rb = new ResultBean().fail(); |
|||
VegeReplenish entity = new VegeReplenish(); |
|||
if (StringUtils.isNotBlank(dto.getCustomerSid())) { |
|||
entity.setCustomerSid(dto.getCustomerSid()); |
|||
} |
|||
if (StringUtils.isNotBlank(dto.getCode())) { |
|||
entity.setCardCode(dto.getCode()); |
|||
} |
|||
entity.setCreateTime(new DateTime()); |
|||
entity.setCardtype(type); |
|||
baseMapper.insert(entity); |
|||
if (type.equals("0")) { |
|||
//个人卡
|
|||
LpkGiftCard card = lpkGiftCardService.selectOneByCodeAndCodeKey(dto.getCode(), dto.getCodeKey()); |
|||
if (null != card) { |
|||
LpkGiftBagInitVo giftBagInitVo = lpkGiftBagService.giftBagInit(card.getGiftbagSid()).getData(); |
|||
if (null != giftBagInitVo) { |
|||
List<GiftBagGoods> goods = giftBagInitVo.getGoods(); |
|||
if (!goods.isEmpty()) { |
|||
goods.stream().forEach(g -> { |
|||
VegeReplenishDetail detail = new VegeReplenishDetail(); |
|||
detail.setGoodsSid(g.getGoodsSid()); |
|||
detail.setGoodsNumber(Integer.parseInt(g.getGoodsNumber())); |
|||
detail.setOrderSid(entity.getSid()); |
|||
detail.setCreateTime(new DateTime()); |
|||
vegeReplenishDetailService.insert(detail); |
|||
}); |
|||
} |
|||
} |
|||
} |
|||
} else if (type.equals("1")) { |
|||
//企业卡
|
|||
EmpCard card = empCardService.selectOneByCodeAndCodeKey(dto.getCode(), dto.getCodeKey()); |
|||
if (null != card) { |
|||
LpkGiftBagInitVo giftBagInitVo = lpkGiftBagService.giftBagInit(card.getGiftbagSid()).getData(); |
|||
if (null != giftBagInitVo) { |
|||
List<GiftBagGoods> goods = giftBagInitVo.getGoods(); |
|||
if (!goods.isEmpty()) { |
|||
goods.stream().forEach(g -> { |
|||
VegeReplenishDetail detail = new VegeReplenishDetail(); |
|||
detail.setGoodsSid(g.getGoodsSid()); |
|||
detail.setGoodsNumber(Integer.parseInt(g.getGoodsNumber())); |
|||
detail.setOrderSid(entity.getSid()); |
|||
detail.setCreateTime(new DateTime()); |
|||
vegeReplenishDetailService.insert(detail); |
|||
}); |
|||
} |
|||
} |
|||
} |
|||
} else if (type.equals("2")) { |
|||
//转赠卡
|
|||
EmpCardGift card = empCardGiftService.selectOneByCodeAndCodeKey(dto.getCode(), dto.getCodeKey()); |
|||
if (null != card) { |
|||
List<EmpCardGiftGoodsVo> goods = empCardGiftGoodsService.getGoodsDetailsByEmpCardGiftSid(card.getSid()).getData(); |
|||
if (!goods.isEmpty()) { |
|||
goods.stream().forEach(g -> { |
|||
VegeReplenishDetail detail = new VegeReplenishDetail(); |
|||
detail.setGoodsSid(g.getGoodsSid()); |
|||
detail.setGoodsNumber(g.getGoodsNumber()); |
|||
detail.setOrderSid(entity.getSid()); |
|||
detail.setCreateTime(new DateTime()); |
|||
vegeReplenishDetailService.insert(detail); |
|||
}); |
|||
} |
|||
} |
|||
} |
|||
return rb.success(); |
|||
} |
|||
} |
@ -0,0 +1,15 @@ |
|||
package com.yxt.yythmall.biz.vegereplenishdetail; |
|||
|
|||
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
|||
import com.yxt.yythmall.api.vegereplenish.VegeReplenish; |
|||
import com.yxt.yythmall.api.vegereplenishdetail.VegeReplenishDetail; |
|||
import org.apache.ibatis.annotations.Mapper; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/23 10:36 |
|||
*/ |
|||
@Mapper |
|||
public interface VegeReplenishDetailMapper extends BaseMapper<VegeReplenishDetail> { |
|||
|
|||
} |
@ -0,0 +1,8 @@ |
|||
<?xml version="1.0" encoding="UTF-8" ?> |
|||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|||
<mapper namespace="com.yxt.yythmall.biz.vegereplenishdetail.VegeReplenishDetailMapper"> |
|||
<!-- <where> ${ew.sqlSegment} </where>--> |
|||
<!-- ${ew.customSqlSegment} --> |
|||
|
|||
|
|||
</mapper> |
@ -0,0 +1,19 @@ |
|||
package com.yxt.yythmall.biz.vegereplenishdetail; |
|||
|
|||
import io.swagger.annotations.Api; |
|||
import org.springframework.beans.factory.annotation.Autowired; |
|||
import org.springframework.web.bind.annotation.RequestMapping; |
|||
import org.springframework.web.bind.annotation.RestController; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/23 10:35 |
|||
*/ |
|||
@Api(tags = "绑卡记录详情") |
|||
@RestController |
|||
@RequestMapping("vegereplenishdetail") |
|||
public class VegeReplenishDetailRest { |
|||
@Autowired |
|||
VegeReplenishDetailService vegeReplenishDetailService; |
|||
|
|||
} |
@ -0,0 +1,15 @@ |
|||
package com.yxt.yythmall.biz.vegereplenishdetail; |
|||
|
|||
import com.yxt.common.base.service.MybatisBaseService; |
|||
import com.yxt.yythmall.api.vegereplenish.VegeReplenish; |
|||
import com.yxt.yythmall.api.vegereplenishdetail.VegeReplenishDetail; |
|||
import org.springframework.stereotype.Service; |
|||
|
|||
/** |
|||
* @author wangpengfei |
|||
* @date 2023/11/23 10:36 |
|||
*/ |
|||
@Service |
|||
public class VegeReplenishDetailService extends MybatisBaseService<VegeReplenishDetailMapper, VegeReplenishDetail> { |
|||
|
|||
} |
Loading…
Reference in new issue